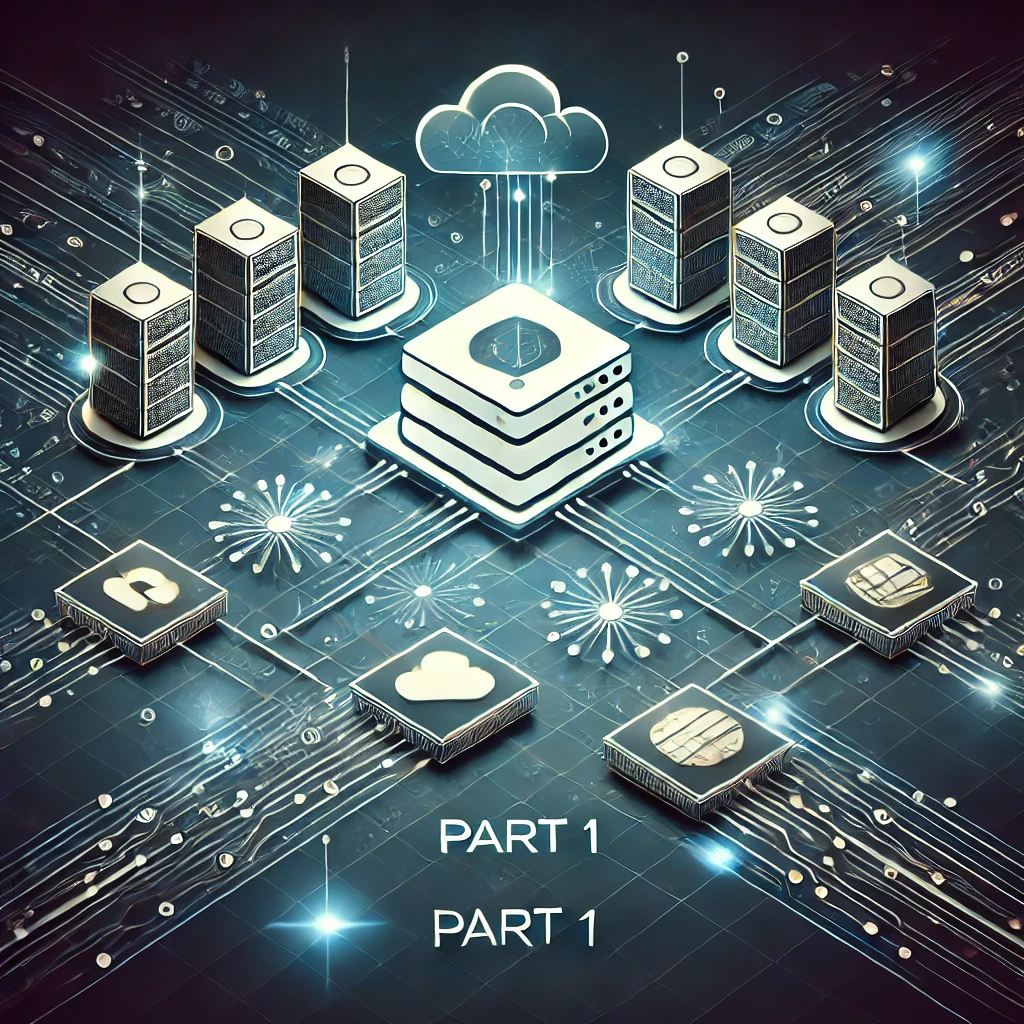
Load balancing is a fundamental component of system design, crucial for distributing network traffic across multiple servers to ensure optimal resource utilization, reduce latency, and prevent any single server from becoming a point of failure. By providing redundancy and scaling capacity, load balancers enhance both the reliability and performance of applications, making them resilient to high traffic and unexpected spikes in demand.
In This Session, We Will:
- Create an initial API
- Clone the first API for a second instance
- Set up an Nginx server
- Run docker compose up
The APIs
For this demonstration, we’ll use FastAPI due to its simplicity and Python’s robust package ecosystem, which makes it easy to demonstrate these concepts. Start by creating a file named api1.py
:
from fastapi import FastAPI
import uvicorn
app = FastAPI()
@app.get("/hc")
def healthcheck():
return 'API-1 Health - OK'
if __name__ == "__main__":
uvicorn.run(app, host="0.0.0.0", port=8001)
Here, FastAPI
is our web framework, and we’ll use uvicorn
as the http server to run the API. Both are listed in the requirements.txt
file. This example features a simple health check endpoint. In real-world applications, the implementation could be some CRUD method that is far more complex.
To avoid configuration issues and permissions on your machine, we’ll use Docker for a clean setup. Here’s the Dockerfile
for api1.py
:
FROM python:3.11
COPY ./requirements.txt /requirements.txt
WORKDIR /
RUN pip install -r requirements.txt
COPY . /
ENTRYPOINT ["python"]
CMD ["api1.py"]
EXPOSE 8001
Now, let’s create a second API by duplicating everything except the port. This second API will be named api2.py
and will run on port 8002:
from fastapi import FastAPI
import uvicorn
app = FastAPI()
@app.get("/hc")
def healthcheck():
return 'API-2 Health - OK'
if __name__ == "__main__":
uvicorn.run(app, host="0.0.0.0", port=8002)
The Dockerfile
for api2.py
is identical except for the port number is 8002
FROM python:3.11
COPY ./requirements.txt /requirements.txt
WORKDIR /
RUN pip install -r requirements.txt
COPY . /
ENTRYPOINT ["python"]
CMD ["api2.py"]
EXPOSE 8002
Setting Up the Load Balancer
For this demonstration, we’ll use Nginx, a powerful open-source web server that can also handle load balancing. Although there are other options, including AWS’s Application Load Balancer, Nginx is sufficient for illustrating the basic concepts.
The goal is to have two identical APIs taking requests in a round-robin fashion. While this may seem trivial at a small scale, it becomes crucial as the number of users increases. Technologies like AWS Fargate allow you to scale dynamically based on load, starting with two services and automatically spinning up more as needed.
Here’s the Dockerfile
for Nginx:
FROM nginx
RUN rm /etc/nginx/conf.d/default.conf
COPY nginx.conf /etc/nginx/conf.d/default.conf
EXPOSE 80
EXPOSE 8080
Next, we need to configure the Nginx load balancer by specifying the API IP addresses and ports. This is all specified in the docker_compose.yml which we will get to in a minute. Create a file called nginx.conf and add the code below.
upstream loadbalancer {
server 172.20.0.4:8001;
server 172.20.0.5:8002;
}
server {
listen 80;
location / {
proxy_pass http://loadbalancer;
}
}
The ip addresses above are from the docker containers.
Final Setup with Docker Compose
Now, let’s set up a small, multi-service application using a docker-compose.yml
file. This file will configure the infrastructure, specifying IPs, ports, and references to the APIs and load balancer:
version: '3'
networks:
frontend:
ipam:
config:
- subnet: 172.20.0.0/24
gateway: 172.20.0.1
services:
api1:
build: ./api1
networks:
frontend:
ipv4_address: 172.20.0.4
ports:
- "8001:8001"
api2:
build: ./api2
networks:
frontend:
ipv4_address: 172.20.0.5
ports:
- "8002:8002"
nginx:
build: ./nginx
networks:
frontend:
ipv4_address: 172.20.0.2
ports:
- "80:80"
depends_on:
- api1
- api2
Breakdown:
- Networks Section: Defines custom networks for our services, with specific IP address management (IPAM) configuration.
- Subnet: Specifies a range of 256 IP addresses (from 172.20.0.0 to 172.20.0.255).
- Gateway: Defines the default gateway at 172.20.0.1.
- Services Section: Specifies the containers that will make up this application.
- API1 and API2: Each has a fixed IP address and port mapping.
- Nginx: Connects to the frontend network, maps port 80, and depends on the two API services.
Running the Setup
Finally, run docker-compose
up on the docker-compose.yml
file to start all Docker instances.
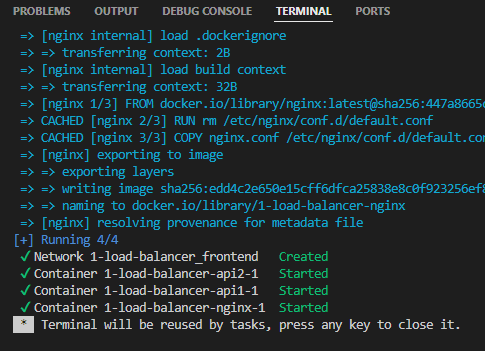
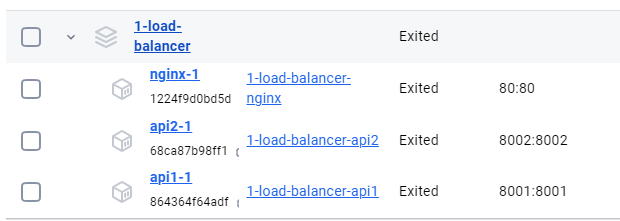
Once everything is up and running, you can test the load balancer by accessing http://localhost/hc
. You’ll notice that requests are distributed between the two servers in a round-robin manner, effectively demonstrating the basics of load balancing.
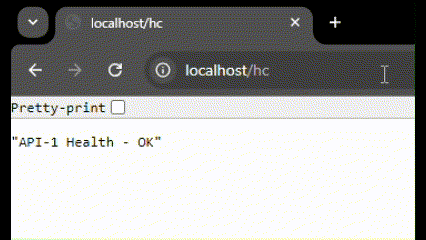
As you scale, more advanced solutions can provide geographic load balancing, dynamic scaling, and more.
Thank you for following my blog
Please find the code here
Pretty section of content. I just stumbled upon your blog and in accession capital to assert that I get in fact enjoyed account your blog posts. Any way I will be subscribing to your feeds and even I achievement you access consistently rapidly.
нажмите, чтобы подробнее
[url=https://euroshop18.ru/katalog/xolodilniki/vstraivaemye.html]встраиваемый холодильник цена[/url]
[url=https://quarklab.ru/]coin drain[/url] – crypto drainer, crypto drainer download
Экономия времени и финансов при использовании современного оборудования.
Барабанный просеиватель песка [url=http://barabaniy-grohot.moscow/]http://barabaniy-grohot.moscow/[/url] .
why not try here https://toruswallet.org/
опубликовано здесь
[url=https://stmb-trucks.ru/]китайский экскаватор погрузчик[/url]
Источник
[url=https://dbshop.ru/services/ustanovka-avtozvuka/ustanovka-magnitoly/]установка магнитолы[/url]
click this https://toruswallet.org
continue reading this https://web-freewallet.com
have a peek at this web-site https://jaxx-wallet.net
CapCut считается мощным приложением для редактирования видео, который открыл новые возможности в области видеомонтажа. Доступный как в онлайн-версии через capcut.com, так и в виде софта для PC и телефонов, он обеспечивает мощные функции редактирования для авторов любого уровня. Детальное описание функций представлено на сайте [url=https://aggam.xyz/]https://aggam.xyz/[/url] и в официальных соцсетях.
Уникальным преимуществом CapCut является богатая коллекция встроенных темплейтов, которые позволяют даже начинающим пользователям создавать привлекательные видео в быстром темпе.
Приложение постоянно развивается – от базовой версии до расширенной CapCut Pro, предлагая пользователям дополнительные инструменты и креативные решения.
Я готов помочь по вопросам как делать эдиты в capcut бесплатно – стучите в Телеграм srp60
directory https://jaxx-wallet.net/
Continued https://web-freewallet.com
One thing I have actually noticed is the fact that there are plenty of common myths regarding the financial institutions intentions any time talking about home foreclosure. One fantasy in particular is always that the bank would like your house. Your banker wants your hard earned dollars, not the home. They want the bucks they lent you having interest. Avoiding the bank will draw some sort of foreclosed final result. Thanks for your post.
Американские власти могут прекратить распространение известные сетевые устройства TP-Link из-за проблем с защищенностью. Как сообщает [url=https://netgate-kiev.blogspot.com/2024/12/TP-Link-pod-pritselom-spetssluzhb-SSHA.html ]Источник[/url], регуляторы США изучают возможность блокировки распространения сетевого оборудования TP-Link на территории США. Главным основанием называются угрозы безопасности страны и причастность оборудования компании с частыми цифровыми угрозами.
По данным издания, продукция китайского производителя часто продаются с брешами безопасности, а компания не проявляет должного усердия в их ликвидации. Особую тревогу вызывает тот факт, что маршрутизаторы TP-Link задействованы не только в частных сетях, но и в стратегической инфраструктуре, включая Пентагон США.
TP-Link удерживает около 65% рынка США маршрутизаторов для домашнего использования и малого бизнеса. В компании сообщили, что готовы к сотрудничеству с властями США и намерены продемонстрировать соответствие своих способов обеспечения безопасности принятым нормам.
Окончательное решение о запрете может быть вынесено в 2025 году.
Источник: [url=https://netgate-kiev.blogspot.com/2024/12/TP-Link-pod-pritselom-spetssluzhb-SSHA.html ]https://telegra.ph/Riski-kiberbezopasnosti-analiz-situacii-s-zapretom-TP-Link-v-SSHA-12-18 [/url]
Готов выручить в трудную минуту по вопросам 2025: США без TP-Link – стучите в Телеграм tds56
Recommended Site [url=https://sites.google.com/mycryptowalletus.com/metamaskwalletapp-extension/]Metamask Extension[/url]
her comment is here [url=https://sites.google.com/mycryptowalletus.com/metamask-walletapp-extension/]Metamask Extension[/url]
Get More Info [url=https://sites.google.com/mycryptowalletus.com/phantomwalletapp-extension/]phantom wallet[/url]
useful source [url=https://sites.google.com/mycryptowalletus.com/phantom-walletapp-extension/]phantom wallet[/url]
hop over to here [url=https://sites.google.com/mycryptowalletus.com/metamaskwalletapp-extension/]Metamask Extension[/url]
look at this website [url=https://sites.google.com/mycryptowalletus.com/phantom-walletapp-extension/]phantom Download[/url]
useful reference [url=https://sites.google.com/mycryptowalletus.com/metamask-walletapp-extension/]MetaMask Download[/url]
visit homepage [url=https://sites.google.com/mycryptowalletus.com/phantomwalletapp-extension/]phantom Extension[/url]
I like the valuable information you supply on your articles. I will bookmark your blog and take a look at once more here regularly. I am somewhat sure I抣l learn a lot of new stuff proper here! Best of luck for the next!
нажмите здесь [url=https://lk.mangoo-office.com]Mango-Office отзывы клиентов[/url]
пояснения [url=https://lk.mangoo-office.com]Манго Офис личный кабинет[/url]
перенаправляется сюда [url=https://mango-offlce.com/]Манго Офис облачные решения[/url]
I’m not sure exactly why but this blog is loading very slow for me. Is anyone else having this problem or is it a problem on my end? I’ll check back later and see if the problem still exists.
You helped me a lot with this post. I love the subject and I hope you continue to write excellent articles like this.
Discover the secrets of effective marketing: precise contact information! I can help you gather it all. https://telegra.ph/Personalized-Contact-Data-Extraction-from-Google-Maps-10-03 (or telegram: @chamerion)
веб-сайте [url=https://lk.mangoo-office.com]Манго Офис телефония[/url]
узнать больше https://forum.hpc.name/thread/19290/nujen-chistyy-djimbot.html
[url=https://quarklab.ru]EVM drainer[/url] – crypto drainer download, Crypto scam
Источник https://plaan.ai/category/cases/
click here to read [url=https://the-immediateaffinity.com]Immediate Affinity[/url]
Thanks for making me to acquire new suggestions about computers. I also hold the belief that certain of the best ways to keep your laptop in perfect condition is with a hard plastic case, and also shell, which fits over the top of the computer. A majority of these protective gear usually are model specific since they are manufactured to fit perfectly within the natural covering. You can buy all of them directly from the owner, or via third party places if they are available for your laptop, however not every laptop can have a cover on the market. Once more, thanks for your points.
dig this [url=https://trusteewallet.org]trustee plus[/url]
Click Here
[url=https://my-sollet.com/]sollet wallet extension[/url]
I must voice my love for your kindness supporting those individuals that should have help on this important topic. Your real commitment to getting the message all over turned out to be rather helpful and has all the time allowed employees just like me to get to their desired goals. The useful useful information entails a whole lot to me and especially to my colleagues. Thanks a lot; from everyone of us.
Homepage [url=https://brd-wallet.io]bread-wallet[/url]
You made certain good points there. I did a search on the matter and found a good number of folks will consent with your blog.
web [url=https://web-counterparty.io]counterparty bitcoin[/url]
В современную цифровую эпоху все больше пользователей встречаются с сложным выбором: что делать со устаревшим домашним кинотеатром при покупке нового телевизора? Многие заблуждаются, что придется покупать новую акустическую систему. Однако есть несколько работающих способов объединения прежней, но качественной техники с новыми устройствами. Такое решение не только сбережет значительную сумму, но и позволит продолжить использование проверенным временем оборудованием. Для тех, кому любопытно, то по url можно прочитать о том [url=https://1wyws.top/ ]как включить без пульта домашний кинотеатр bbk [/url] и много других познавательных публикаций в блоге 1wyws.top.
В материале подробно описываются различные варианты соединения старых домашних кинотеатров к современным телевизорам – от использования HDMI-интерфейса с поддержкой технологии ARC до подключения через оптический выход или традиционные аналоговые разъемы. Каждый метод сопровождается детальными руководствами и практическими рекомендациями. Особое внимание уделяется совместимости различных типов соединений и возможным техническим ограничениям. Читатели найдут полезную таблицу совместимости разъемов, которая поможет быстро выбрать оптимальный способ подключения.
Материал также включает подробное описание типичных сложностей, возникающих при подключении устройств разных поколений, и действенные способы их решения. Авторы делятся практическими советами по настройке звука и оптимизации работы системы. Пошаговые инструкции помогут даже неопытным пользователям успешно выполнить подключение самостоятельно. Особенно важным является раздел с описанием возможных сложностей и методов их устранения, что поможет избежать многих распространенных ошибок при настройке оборудования.
Источник: [url=https://1wyws.top/tehnologii/330-kak-podklyuchit-staryj-domashnij-kinoteatr-k-novomu-televizoru/ ]https://1wyws.top/tehnologii/330-kak-podklyuchit-staryj-domashnij-kinoteatr-k-novomu-televizoru/ [/url]
Не буду равнодушен к вашей просьбе о помощи по вопросам как подключить телевизор к домашний кинотеатр sony – пишите в Телеграм uku02
[url=https://kgmstrategy.com/]cross-border sourcing strategies[/url] was the missing piece in our approach—it’s worth exploring.
When I initially commented I clicked the “Notify me when new comments are added” checkbox and now each time a comment is added I get three emails with the same comment. Is there any way you can remove people from that service? Cheers!
[url=https://arroyostrategy.com]procurement software[/url] is an innovative option we’ve recently explored, and it’s proven highly effective.
Related Site
[url=https://toruswallet.org/]evm wallet[/url]
I really like your writing style, fantastic info, thanks for putting up :D. “Silence is more musical than any song.” by Christina G. Rossetti.
Let’s make tonight unforgettable… your place or mine? – https://rb.gy/es66fc?braima
Преди време пътувах с туристическа агенция Мистрал Травел и останах изключително доволна! Организацията беше безупречна – от записването до самото пътуване. Избрах туристическа програма до чешката столица Прага и всичко беше изпълнено с професионализъм – мястото за настаняване беше удобен, гидът с висока квалификация, а програмата интересна и добре балансирана. Благодарение на тях имах възможност да се насладя на културата и забележителностите на града без никакви грижи. С радост бих се използвала услугите на Мистрал отново и препоръчвам на всички да изберат техните услуги!
hi!,I like your writing so much! share we communicate more about your post on AOL? I require an expert on this area to solve my problem. May be that’s you! Looking forward to see you.
Ahoj! I’m curious about what’s in store for us.
Visiting your site was like soaking in the golden sun on a Moroccan beach, with beautiful patterns and warm designs wrapping around me like a cozy blanket. You’ve clearly got the eye of a seasoned travel designer, ensuring every detail enhances the overall vibe.
I would love to hear your insights on my site buy institute diploma online
See you soon, and may peace be your guiding star
Окраска бампера машины требует тщательной подготовительной работы и правильного подбора составов. Сначала необходимо определить, сколько краски нужно на бампер, учитывая его размеры и состояние поверхности. [url=https://emmanuelbibletraining.info/ ]Слезает краска на бампере на emmanuelbibletraining.info [/url] считается подходящим выбором для достижения качественного результата. Важно помнить, что перед нанесением нового покрытия следует тщательно подготовить поверхность, очистив старую краску и обезжирив пластик. Текстурная краска гарантирует не только эстетичный внешний вид, но и дополнительную защиту от механических повреждений.
Для тех, кто столкнулся с необходимостью удаления следов краски после ДТП, существуют различные методы решения проблемы. Чтобы оттереть краску с бампера от другой машины, специалисты рекомендуют использовать специальные смывки для краски с пластика бампера, которые эффективно справляются с задачей без повреждения основного покрытия. При работе с черной структурной краской важно учитывать время высыхания каждого слоя и следовать технологию нанесения, что позволит добиться однородного покрытия и долговечного результата.
Источник: [url=https://emmanuelbibletraining.info/ ]https://emmanuelbibletraining.info/ [/url]
по вопросам Краска для бампера в баллончиках купить – пишите в Telegram ehl14
Sup, bro? It’s awesome running into you, my dude.
I felt as if I were lounging on a private beach in the Maldives while browsing your site! The vibrant tropical color scheme and engaging content made me want to sip a coconut drink under the sun. Well done, master of tropical relaxation!
Your code is smooth—let’s see if we can make magic on my site too buy certified diploma
Goodbye for now, and may your spirit soar like an eagle
check this [url=https://cryptomatrix.money/]copy trading[/url]
Как безопасно купить диплом колледжа или ПТУ в России, что важно знать
I want to show you one exclusive program called (BTC PROFIT SEARCH AND MINING PHRASES), which can make you a rich man!
This program searches for Bitcoin wallets with a balance, and tries to find a secret phrase for them to get full access to the lost wallet!
Run the program and wait, and in order to increase your chances, install the program on all computers available to you, at work, with your friends, with your relatives, you can also ask your classmates to use the program, so your chances will increase tenfold!
Remember the more computers you use, the higher your chances of getting the treasure!
DOWNLOAD FOR FREE
Telegram:
https://t.me/btc_profit_search
Nice i really enjoyed reading your blogs. Keep on posting. Thanks
Nice i really enjoyed reading your blogs. Keep on posting. Thanks
в этом разделе [url=https://vk.com/brows_makeup_kotlas]Брови Вычегодский[/url]
Thank you for sharing indeed great looking !
нажмите http://lapplebi.com/
why not try this out https://my-sollet.com/
go now https://web-lumiwallet.com/
go now https://myjaxxwallet.us
visit the site https://abacusmarket.me
[url=https://kra020.shop]kra8.at[/url] – kraken актуальные ссылки, kraken маркетплейс официальный сайт
you could check here https://web-sollet.com/
[url=http://bs2siite2.at/]blacksprut com ссылка[/url] – bs2web nl, как открыть ссылку bs2web at
[url=https://bbqate.com]bbgate.com[/url] – breaking bad forum, Speciality Chemicals
[url=https://alt-coins.cc/]Альткоин обменник[/url] – Alt coin bestchange, Alt coin bestchange
[url=https://fermacc.org/]обменять доге[/url] – обменять dogecoin, ферма обменник
Empowering Investors with EtherBank
Investing in cryptocurrency doesn’t have to be complicated. EtherBank crypto investment simplifies the process, offering a secure and efficient way to grow your assets.
Key Features of EtherBank
Transparency: Real-time updates and blockchain integration.
Support: Expert guidance through the EtherTalk investment platform.
Flexibility: Customizable investment plans to suit your needs.
Why Choose EtherTalk Investment?
EtherTalk investment connects you with valuable insights and analytics. Whether you’re tracking market trends or exploring new opportunities, EtherTalk empowers you to make smarter choices.
Take control of your financial future with EtherBank crypto investment. Join us today and experience the difference.
На нашей платформе вы получите доступ к всё, что нужно для удобного использования к популярным букмекерским конторам. Мы гарантируем актуальный [url=https://trinixy.ru/249332-sloty-leonbets-igrovye-avtomaty-bk-leon-v-onlayn-kazino-voyti-na-sayt-leonbets.html]слоты леон[/url] где представлены такие популярные бренды, как Марафонбет. Наш сайт включает дополнительные ресурсы и решения, предоставляющие доступ легко избегать ограничений и с комфортом играть без ограничений.
Помимо этого, вы имеете возможность воспользоваться бонусами, разобраться в процессе регистрации и скачать удобные приложения. Для конкретных брендов, как 1хБет, мы обеспечиваем проверенные решения для [url=https://trinixy.ru/239145-legalnye-bukmekerskie-kontory-reyting-bk-rossii.html]лучшие букмекерские конторы в россии[/url] чтобы вы всегда были на связи. Всё это предлагается для того, чтобы ваш доступ к беттингу было гарантированно защищённым и простым.
Новейшие технологии GPON открывают новые возможности для высокоскоростного интернет-соединения, и многие клиенты интересуются о правильном подключении роутера к такой сети. На сайте [url=https://netgate-kiev.blogspot.com/ ]Как подключиться к gpon роутеру мгтс на netgate-kiev.blogspot.com [/url] опубликована подробная информация о разных способах настройки оборудования. Вне зависимости от того, эксплуатируете ли вы оборудование от провайдеров МГТС или Ростелеком, следует понимать основные принципы подключения вашего роутера к GPON-терминалу для обеспечения стабильного и быстрого интернет-соединения.
Процесс подключения роутера к GPON может отличаться в зависимости от конкретного оператора и установленного оборудования. При подключении своего роутера к GPON-терминалу необходимо учитывать особенности настройки VLAN, MAC-адресов и других конфигураций сети. Пристальное внимание следует уделить настройке Wi-Fi параметров и настройке безопасности сети, чтобы обеспечить надежную защиту домашней сети. Многие абоненты успешно настраивают как стандартные роутеры провайдера, так и свои собственные устройства, важно – следовать инструкциям и правильно настраивать все параметры подключения.
Источник: [url=https://netgate-kiev.blogspot.com/ ]https://netgate-kiev.blogspot.com/ [/url]
по вопросам Как подключить роутер к gpon мгтс – пишите в Telegram koq80
Unlock Your Potential with EtherBank Crypto Investment
As the digital economy evolves, EtherBank offers unparalleled opportunities for individuals and businesses. EtherBank crypto investment is designed to provide secure and lucrative options for anyone looking to thrive in the blockchain era.
Why EtherBank Is the Right Choice
EtherBank simplifies the complexities of cryptocurrency. With an intuitive platform and transparent policies, investors can enjoy peace of mind while accessing cutting-edge tools.
The Power of EtherTalk Investment
For those seeking guidance, EtherTalk investment offers a wealth of resources. From beginner-friendly guides to advanced analytics, EtherTalk ensures you’re equipped with the knowledge to succeed in the crypto world.
Making Crypto Accessible to All
EtherBank’s mission is to democratize crypto investments. With low entry barriers and customizable plans, EtherBank crypto investment caters to all levels of expertise. Whether you’re starting small or managing a portfolio, EtherBank has you covered.
Start your journey with EtherBank today. Discover why thousands trust us as their gateway to financial growth.
Your blog is a testament to your dedication to your craft. Your commitment to excellence is evident in every aspect of your writing. Thank you for being such a positive influence in the online community. SLOT DANA GOPAY
проверить сайт https://zelenka.guru/forums/587/
нажмите, чтобы подробнее https://lzt.market/
веб-сайте https://zelenka.guru/articles/
узнать https://zelenka.guru/forums/767/
Присоединяйтесь к [url=https://www.kv.by/information/2023/08/05/1067839-mostbet-zerkalo-vhod-na-sayt-mostbet-obzor-i-registraciya]Mostbet[/url], чтобы открыть двери в мир спорта и выигрышей! Надежная платформа, быстрый доступ через зеркало и бонусы для новых игроков ждут вас уже сегодня.
Success starts with accurate information! Order data extraction and enhance your chances of growth. https://telegra.ph/Personalized-Contact-Data-Extraction-from-Google-Maps-10-03 (or telegram: @chamerion)
узнать https://lzt.market
In Jodo Do Tigrinho online slots, every spin is a step towards victory! Incredible graphics, exciting themes and many bonuses await you. Fortune favors the brave – try your hand and discover the world of winnings with Tigrinho!
onlinepharmacy
Hi there! Do you know if they make any plugins to protect against hackers? I’m kinda paranoid about losing everything I’ve worked hard on. Any suggestions?
I know that there are countless plug-ins designed to make the comments do-follow, but I’m looking for something that will make the links in the blog-posts themselves do-follow. Please include a link or detailed instructions on how to do this. Thanks!. Do you have any examples of themes that you know for a fact have do-follow links in the posts? I’m having a hard time finding good information about this by searching..
Стоимость металлокерамического имплантанта.
Металлокерамическая коронка на имплантате цена [url=http://www.belfamilydent.ru/services/metallokeramicheskie-koronki/]http://www.belfamilydent.ru/services/metallokeramicheskie-koronki/[/url] .
If you’re looking for a secure and reliable wallet, MetaMask Wallet is the perfect choice. Easy to set up and supports Chrome, Firefox, and Opera!
I really like your blog.. very nice colors & theme. Did you make this website yourself or did you hire someone to do it for you? Plz respond as I’m looking to construct my own blog and would like to find out where u got this from. kudos
Новая эра покера начинается здесь! [url=https://ru.nztpoker.com/]AI покерная помощь[/url] поможет вам принимать лучшие решения и добиваться высоких результатов. Попробуйте и ощутите разницу!
Подробнее здесь [url=https://xn--90aipggcf.org]obmenko[/url] – Обменко отзывы, Обменко
Перейти на сайт [url=https://vodkabetslot.ru]vodka casino[/url] – водка бет, водкабет
взломанный первобытный парк [url=https://apk-smart.com/igry/arkady/1487-vzlomannyj-pervobytnyj-park-mod-mnogo-deneg.html]https://apk-smart.com/igry/arkady/1487-vzlomannyj-pervobytnyj-park-mod-mnogo-deneg.html[/url] взломанный первобытный парк
P.S Live ID: K89Io9blWX1UfZWv3ajv
P.S.S [url=https://tehnopage.ru/jsfiddle-error]Программы и игры для Андроид телефона[/url] [url=https://wowjp.net/forum/41-207582-84#4299082]Программы и игры для Андроид телефона[/url] [url=http://gpnmall.gp114.net/bbs/board.php?bo_table=qa&wr_id=421665]Программы и игры дл[/url] 6378a5d
WONDERFUL Post.thanks for share..extra wait .. ?
Using Metamask on Chrome has been a great experience! If you’re looking for step-by-step instructions, https://metamenu.org/ has you covered.
https://virtual-local-numbers.com/virtualnumber/virtual-sms-number.html
Индивидуальный подход к каждому пациенту при базальной имплантации зубов в Минске.
Базальная имплантация Минск цены [url=https://belfamilydent.ru/services/basal-implantation/]Базальная имплантация Минск цены[/url] .
Уникальные свойства циркония для установки коронок, будут служить вам долгие годы.
Поставить цирконий коронку [url=http://www.belfamilydent.ru/services/koronki-iz-cirkoniya]http://www.belfamilydent.ru/services/koronki-iz-cirkoniya[/url] .
Иногда так хочется отвлечься от повседневных забот и окунуться в мир захватывающих историй. Восточные сериалы — это настоящий источник эмоций, романтики и неожиданных сюжетных поворотов. Если тебе близка культура Азии и ты не можешь устоять перед драматическими сюжетами, то стоит заглянуть на этот сайт. Здесь собраны лучшие [url=https://doramahit.tv/]дорамы[/url], которые можно смотреть в любое время без ограничений.
My brother suggested I might like this blog He was totally right This post actually made my day You can not imagine simply how much time I had spent for this info Thanks.
find out this here https://playslotrealmoney.com/hu/arrival-real-money/
Эта информационная публикация освещает широкий спектр тем из мира медицины. Мы предлагаем читателям ясные и понятные объяснения современных заболеваний, методов профилактики и лечения. Информация будет полезна как пациентам, так и медицинским работникам, желающим поддержать уровень своих знаний.
Выяснить больше – https://nakroklinikatest.ru/
Читать далее [url=https://casino-o.pro]обзор казино с бесплатными фриспинами[/url]
https://athens-rentalcars.com/ru/
Уникальный способ изменить свой гардероб – печать на ткани, добавьте индивидуальность и креативность.
Инновационные технологии печати на ткани, для придания уникальности вашей одежде.
Трендовая технология для ценителей моды, которые не боятся выделяться.
Сделайте свою одежду уникальной с помощью печати на ткани, которые вы сами выберете.
Удивительные дизайны для нанесения на одежду, которые вдохновят вас на новые образы.
Печать на ткани: какие методы выбрать, для идеального результата.
печать рисунка на ткани на заказ [url=http://www.pechat-nadpisi-na-tkani.ru]http://www.pechat-nadpisi-na-tkani.ru[/url] .
navigate to this web-site https://demofreeslot.com/tr/
веб-сайт [url=https://casino-o.pro]1WIN[/url]
этот контент
[url=https://xn--90aipggcf.org/]Обменко официальный сайт[/url] – obmenko bestchange, obmenko bestchange
view https://playslotrealmoney.com/ko/hot-safari-real-money/
[url=https://meqa.fo/]мега купить[/url] – megaweb14.at, mega onion как зайти
узнать больше Здесь [url=https://vodkabetslot.ru]vodka официальный сайт[/url] – водка бет, vodka bet
Алкогольный запой – это серьёзное состояние, при котором человек теряет контроль над употреблением алкоголя, что приводит к тяжёлой интоксикации организма. Во время запоя нарушаются функции внутренних органов, страдает нервная система, и повышается риск развития опасных осложнений, таких как алкогольный делирий. Это состояние требует незамедлительного медицинского вмешательства.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]вывод из запоя вызов на дом екатеринбург[/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Выяснить больше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-v-stacionare-v-chelyabinske/]быстрый вывод из запоя в стационаре [/url]
Запой — это состояние, при котором человек продолжает употреблять алкоголь на протяжении нескольких дней или даже недель, не контролируя объемы потребляемого напитка. Этот процесс сопровождается тяжелыми физическими и психическими последствиями, такими как обезвоживание организма, отравление продуктами распада алкоголя, нервные расстройства и потеря контроля над ситуацией. Вывод из запоя — это медицинская процедура, направленная на прекращение употребления алкоголя и восстановление организма пациента.
Детальнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-stacionare-v-krasnodare/]vyvod iz zapoya v stacionare anonimno v-krasnodare[/url]
Проблема зависимостей остаётся актуальной в современном обществе. С каждым годом увеличивается число людей, страдающих от алкоголизма, наркомании и других форм зависимостей, что негативно отражается на их жизни и благополучии близких. Зависимость — это не просто физическое заболевание, но и глубокая психологическая проблема. Для эффективного лечения требуется помощь профессионалов, способных обеспечить комплексный подход.
Подробнее тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-stacionare-v-voronezhe/]вывод из запоя в стационаре [/url]
Создайте уникальные и запоминающиеся 3D-модели для своих товаров с помощью [url=https://arigami.io/]сделать 3D товара[/url]. Команда Arigami поможет вам воплотить любые идеи в жизнь, создавая высококачественные и точные модели, которые соответствуют всем вашим требованиям. Это отличный способ улучшить восприятие вашего бренда и привлечь больше клиентов.
Алкогольный запой — это тяжелое состояние, при котором человек теряет контроль над количеством потребляемого алкоголя. Это приводит к сильной интоксикации, сбоям в работе органов и систем организма, а также серьезным психоэмоциональным расстройствам. Без должной медицинской помощи запой может вызвать ряд опасных последствий, включая алкогольный делирий и угрозу жизни. Поэтому при первых признаках запоя необходимо обратиться к профессионалам.
Подробнее тут – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]vyvod iz zapoya na domu [/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Получить больше информации – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]срочный вывод из запоя на дому в челябинске[/url]
В этой статье мы расскажем о возможностях клиники для лечения алкогольной интоксикации круглосуточно, включая вывод из запоя в стационаре и на дому, а также о роли профессионалов, таких как нарколог, в процессе лечения.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]vyvod iz zapoya na domu nedorogo krasnodar[/url]
Алкогольная зависимость представляет собой серьёзную медицинскую проблему, требующую специализированного подхода. Запойное состояние характеризуется длительным и бесконтрольным потреблением спиртных напитков, что приводит к возникновению как физической, так и психической зависимости. Данное явление может вызвать нарушения в работе внутренних органов, значительно увеличивая вероятность развития алкогольного делирия, который представляет собой острое состояние, требующее неотложной медицинской помощи. Вывод из запоя это ключевая часть лечения зависимости, которая может осуществляться как в стационаре, так и в амбулаторных условиях, в зависимости от конкретной ситуации и состояния пациента.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-12.ru/]наркологическая клиника[/url]
Алкогольная зависимость — это серьезная проблема, требующая немедленного вмешательства. Если вы или ваши близкие столкнулись с запоем, важно получить профессиональную помощь как можно скорее, чтобы избежать ухудшения здоровья. Наркологическая клиника «Шаг к Трезвости» в Краснодаре предлагает услугу вывода из запоя на дому, что является удобным и эффективным решением для тех, кто не может или не хочет посещать стационар.
Детальнее – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]анонимный вывод из запоя на дому краснодар[/url]
Наркологическая клиника «Союз Здоровья» в Воронеже специализируется на лечении алкогольной зависимости и предлагает профессиональные услуги по выводу из запоя. Мы стремимся создать атмосферу доверия, где каждый пациент сможет получить необходимую поддержку.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-stacionare-v-voronezhe/]вывод из запоя в стационаре анонимно воронеж[/url]
Алкогольная зависимость представляет собой серьёзную медицинскую проблему, требующую специализированного подхода. Запойное состояние характеризуется длительным и бесконтрольным потреблением спиртных напитков, что приводит к возникновению как физической, так и психической зависимости. Данное явление может вызвать нарушения в работе внутренних органов, значительно увеличивая вероятность развития алкогольного делирия, который представляет собой острое состояние, требующее неотложной медицинской помощи. Вывод из запоя это ключевая часть лечения зависимости, которая может осуществляться как в стационаре, так и в амбулаторных условиях, в зависимости от конкретной ситуации и состояния пациента.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-12.ru/]narkologicheskij centr v-krasnodare[/url]
Запой — это тяжелое состояние, возникающее вследствие длительного и непрерывного употребления алкоголя, которое сопровождается физической и психической зависимостью. Это опасное явление требует незамедлительного вмешательства специалистов. Вывод из запоя — это комплексный процесс медицинской детоксикации, который включает в себя очищение организма от токсинов, нормализацию функций внутренних органов и поддержку психоэмоционального состояния пациента. В случае тяжелого запоя стационарное лечение становится оптимальным решением для безопасного и эффективного вывода из этого состояния. В этой статье мы рассмотрим, как проходит вывод из запоя в стационаре клиники «Перекресток Надежды» в Челябинске, а также особенности анонимности, скорости лечения и участия нарколога.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-v-kruglosutochno-v-chelyabinske/]vyvod iz zapoya kruglosutochno [/url]
В современном мире проблема зависимостей остаётся одной из наиболее острых. Всё больше людей сталкиваются с трудностями, связанными с алкоголизмом, наркоманией и другими формами зависимости, что разрушительно сказывается как на их жизни, так и на взаимоотношениях с окружающими. Это сложное явление, затрагивающее не только физическое здоровье, но и психоэмоциональное состояние, требует профессионального подхода для эффективного решения.
Выяснить больше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-anonimno-v-voronezhe/]наркология вывод из запоя анонимно в воронеже[/url]
В клинике «Излечение» понимают важность анонимности и индивидуального подхода при лечении алкогольной зависимости. Полная конфиденциальность гарантируется как в стационаре, так и при выезде на дом. Это позволяет пациентам чувствовать себя защищенными и уверенными на пути к выздоровлению. Процесс вывода из запоя включает в себя устранение токсического воздействия алкоголя, восстановление нормального функционирования организма и психологическую поддержку.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя с выездом на дом краснодар[/url]
Запой — это состояние, при котором человек не может прекратить употребление алкоголя на протяжении длительного времени, что вызывает физическую и психологическую зависимость. Это крайне опасное состояние, требующее немедленного вмешательства специалистов. Наркологическая клиника «Преображение» в Екатеринбурге предоставляет профессиональную помощь в лечении запоя, используя самые эффективные и безопасные методы.
Подробнее – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-cena-v-ekaterinburge/]narkolog vyvod iz zapoya cena ekaterinburg[/url]
Алкогольная зависимость становится всё более распространенной проблемой, требующей внимания как со стороны медицинского сообщества, так и общества в целом. Вывод из запоя — это сложный и ответственный процесс, необходимый для восстановления здоровья пациента и его интеграции в нормальную жизнь. Профессиональное вмешательство позволяет минимизировать последствия длительного употребления алкоголя и нормализовать состояние организма.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-anonimno-v-ekaterinburge/]narkologiya vyvod iz zapoya anonimno ekaterinburg[/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-17.ru/]vyvod iz zapoya s vyezdom v-chelyabinske[/url]
Алкогольные запои — это одно из самых сложных проявлений зависимости, требующее профессионального подхода и незамедлительных действий. В такие периоды близкие люди часто не знают, как помочь, но своевременное обращение за медицинской помощью может стать ключом к восстановлению. В Челябинске клиника «Перекресток Надежды» предоставляет квалифицированные услуги по выводу из запоя, сочетая современные методики лечения с поддержкой на психологическом уровне.
Выяснить больше – [url=https://vyvod-iz-zapoya-17.ru/]narkologicheskaya klinika chelyabinsk[/url]
Гарантированная анонимность при выводе из запоя в Челябинске. В клинике «Перекресток Надежды» мы строго соблюдаем политику конфиденциальности. Информация о пациенте и его состоянии здоровья остаётся закрытой. Это особенно важно для тех, кто ценит свою репутацию и не хочет разглашения. Мы обеспечиваем анонимность как в стационаре, так и при оказании услуг на дому, сохраняя высокий уровень медицинской помощи.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-cena-v-chelyabinske/]вывод из запоя цена в челябинске[/url]
Запойное пьянство характеризуется продолжительным и интенсивным потреблением алкоголя, что приводит к серьезным физическим и психологическим последствиям. Часто пациенты сталкиваются с различными заболеваниями, такими как цирроз печени, панкреатит, алкогольный делирий и другие. Алкогольное опьянение не только ухудшает качество жизни, но также может угрожать жизни пациента. В условиях стационара лечение может быть более эффективным, однако многие люди предпочитают проходить данную процедуру на дому, чтобы избежать стигматизации и сохранить чувство приватности.
Разобраться лучше – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]vyvod iz zapoya na domu kruglosutochno ekaterinburg[/url]
Алкогольная зависимость представляет собой серьезную медицинскую проблему, которая влечет за собой не только физические, но и психологические проблемы. Периоды запоя, характеризующиеся бесконтрольным употреблением алкоголя, могут привести к тяжелым последствиям. Симптомы абстиненции требуют незамедлительного вмешательства. Анонимность в процессе реабилитации становится важным аспектом, помогающим пациентам избежать стыда и социального осуждения.
Получить больше информации – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-cena-v-krasnodare/]нарколог вывод из запоя цена [/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Выяснить больше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya na domu nedorogo chelyabinsk[/url]
Для достижения эффективных результатов в лечении запоя необходима квалифицированная помощь специалистов. Наркологическая клиника «Излечение» в Краснодаре предоставляет профессиональные услуги по выводу из запоя, гарантируя безопасность, комфорт и индивидуальный подход к каждому пациенту.
Получить больше информации – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]vyvod iz zapoya vyzov na dom v-krasnodare[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя с выездом на дом [/url]
В таких ситуациях вывод из запоя на дому становится оптимальным решением, особенно если пациент не может или не хочет посещать стационар. Эта услуга позволяет получить медицинскую помощь в комфортной и привычной обстановке, что снижает уровень стресса и ускоряет процесс восстановления.
Разобраться лучше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-anonimno-v-voronezhe/]анонимный вывод из запоя на дому в воронеже[/url]
Алкогольная зависимость — это серьезная проблема, требующая немедленного вмешательства. Если вы или ваши близкие столкнулись с запоем, важно получить профессиональную помощь как можно скорее, чтобы избежать ухудшения здоровья. Наркологическая клиника «Шаг к Трезвости» в Краснодаре предлагает услугу вывода из запоя на дому, что является удобным и эффективным решением для тех, кто не может или не хочет посещать стационар.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]vyvod iz zapoya kruglosutochno [/url]
В Воронеже специалисты клиники «Союз Здоровья» оказывают профессиональную помощь пациентам, страдающим от алкогольной зависимости, включая выведение из запоя. Особое внимание уделяется созданию атмосферы доверия и комфорта, что помогает пациентам быстрее адаптироваться к процессу лечения.
Детальнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-kruglosutochno-v-voronezhe/]vyvod iz zapoya kruglosutochno [/url]
Алкогольная зависимость представляет собой актуальную медицинскую проблему, требующую тщательного подхода. Запой характеризуется длительным, непрерывным употреблением спиртных напитков, что ведёт к физической и психической зависимости. На фоне запойного состояния могут возникнуть тяжёлые нарушения функций органов, увеличивается риск развития таких состояний, как алкогольный делирий. Вывод из запоя становится ключевым этапом в лечении зависимостей, который может осуществляться как в стационарных условиях, так и на дому, в зависимости от состояния пациента. В клинике «Перекресток Надежды» в Челябинске мы предлагаем круглосуточную помощь, чтобы обеспечить максимально эффективное и безопасное лечение при любом варианте вывода из запоя.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя с выездом на дом [/url]
Запой — это тяжелое состояние, при котором человек теряет контроль над количеством употребляемого алкоголя, что приводит к серьезным нарушениям здоровья. Процесс вывода из запоя требует профессионального подхода, медицинского контроля и тщательного восстановления организма. Наркологическая клиника «Шаг к Трезвости» в Краснодаре предлагает квалифицированную помощь для тех, кто нуждается в срочном и безопасном выходе из запоя. Мы предоставляем комплексное лечение, ориентированное на каждый этап восстановления, с максимальной заботой о здоровье пациента.
Подробнее тут – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]vyvod iz zapoya srochno kruglosutochno krasnodar[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьёзное воздействие этанола. Это приводит к нарушениям нормальной физиологической деятельности и ухудшению состояния здоровья. Анонимный вывод из запоя — это медицинская помощь, направленная на устранение последствий чрезмерного употребления алкоголя с соблюдением строгой конфиденциальности.
Детальнее – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-v-stacionare-v-ekaterinburge/]быстрый вывод из запоя в стационаре [/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Детальнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya vrach na dom chelyabinsk[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Узнать больше – [url=https://vyvod-iz-zapoya-19.ru/]наркологический центр в санкт-петербурге[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьёзное воздействие этанола. Это приводит к нарушениям нормальной физиологической деятельности и ухудшению состояния здоровья. Анонимный вывод из запоя — это медицинская помощь, направленная на устранение последствий чрезмерного употребления алкоголя с соблюдением строгой конфиденциальности.
Подробнее – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]srochny vyvod iz zapoya na domu [/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Узнать больше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя с выездом на дом [/url]
В современном мире зависимость стала серьёзным вызовом, с которым сталкиваются многие. Жизненные трудности, постоянный стресс, финансовые проблемы — всё это способствует развитию таких заболеваний, как алкоголизм, наркомания и игромания. Клиника “Второй Шанс” специализируется на оказании медицинской помощи людям, страдающим от этих проблем, и помогает восстановить здоровье, адаптироваться к нормальной жизни и предотвратить рецидивы.
Подробнее тут – [url=https://vyvod-iz-zapoya-14.ru/]вывод из запоя с выездом[/url]
В современном мире зависимость стала серьёзным вызовом, с которым сталкиваются многие. Жизненные трудности, постоянный стресс, финансовые проблемы — всё это способствует развитию таких заболеваний, как алкоголизм, наркомания и игромания. Клиника “Второй Шанс” специализируется на оказании медицинской помощи людям, страдающим от этих проблем, и помогает восстановить здоровье, адаптироваться к нормальной жизни и предотвратить рецидивы.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya vrach na dom v-sankt-peterburge[/url]
Выход из запоя — это важнейший этап на пути к избавлению от алкогольной зависимости. Он требует комплексного подхода, профессиональной помощи и сильной мотивации пациента. В условиях, когда анонимность и комфорт становятся ключевыми факторами для многих людей, вывод из запоя на дому приобретает все большее значение. Это решение позволяет человеку получить необходимую помощь, не покидая дома, и избежать стресса, связанного с госпитализацией.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя на дому круглосуточно краснодар[/url]
Стоимость вывода из запоя зависит от ряда факторов, таких как состояние пациента, выбранная программа лечения и место проведения процедуры. Мы предлагаем прозрачное ценообразование, чтобы вы могли заранее ознакомиться с возможными затратами на лечение.
Подробнее – [url=https://vyvod-iz-zapoya-17.ru/]наркологический центр[/url]
Запой — это тяжелое состояние, возникающее вследствие длительного и непрерывного употребления алкоголя, которое сопровождается физической и психической зависимостью. Это опасное явление требует незамедлительного вмешательства специалистов. Вывод из запоя — это комплексный процесс медицинской детоксикации, который включает в себя очищение организма от токсинов, нормализацию функций внутренних органов и поддержку психоэмоционального состояния пациента. В случае тяжелого запоя стационарное лечение становится оптимальным решением для безопасного и эффективного вывода из этого состояния. В этой статье мы рассмотрим, как проходит вывод из запоя в стационаре клиники «Перекресток Надежды» в Челябинске, а также особенности анонимности, скорости лечения и участия нарколога.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-17.ru/]narkologicheskij centr chelyabinsk[/url]
Клиника «Шаг к Трезвости» в Краснодаре предлагает анонимный вывод из запоя, обеспечивая полную конфиденциальность и безопасность на каждом этапе лечения. Мы понимаем, что для многих людей важно сохранить свою личную информацию в тайне, и гарантируем, что все данные о пациенте и его состоянии здоровья останутся закрытыми. Независимо от того, выбрал ли пациент стационарное лечение или вывод из запоя на дому, мы обеспечиваем высококачественную медицинскую помощь с полным соблюдением принципов конфиденциальности.
Узнать больше – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]anonimny vyvod iz zapoya na domu krasnodar[/url]
Запойное состояние сопряжено с множеством рисков, включая вероятность возникновения алкогольного делирия и других опасных осложнений. Поэтому своевременное обращение к наркологу помогает минимизировать эти угрозы. Круглосуточная доступность специалистов клиники позволяет оперативно реагировать на любые ситуации, обеспечивая пациентам безопасность и комфорт на всех этапах лечения.
Детальнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya na domu nedorogo kapelnica v-chelyabinske[/url]
Запой представляет собой состояние, характеризующееся длительным непрерывным употреблением алкоголя, что приводит к физической и психической зависимости. Этот процесс может длиться от нескольких дней до недель. Алкогольные напитки негативно влияют на функционирование внутренних органов, вызывая серьёзные нарушения, включая алкоголизм. При необходимости вывода из запоя важно обратиться за медицинской помощью, что можно осуществить на дому. В данной статье рассмотрим ключевые аспекты данной процедуры.
Выяснить больше – [url=https://vyvod-iz-zapoya-17.ru/]наркологическая клиника в челябинске[/url]
Алкогольная зависимость — одна из самых разрушительных форм аддикции, способная нанести непоправимый вред здоровью. Запой, как крайнее проявление этой зависимости, требует немедленного вмешательства, чтобы избежать серьёзных последствий, включая интоксикацию организма и психические расстройства. Обращение за профессиональной помощью становится ключевым шагом на пути к выздоровлению.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя врач на дом санкт-петербург[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя вызов на дом санкт-петербург[/url]
Запой — это тяжелое состояние, возникающее вследствие длительного и непрерывного употребления алкоголя, которое сопровождается физической и психической зависимостью. Это опасное явление требует незамедлительного вмешательства специалистов. Вывод из запоя — это комплексный процесс медицинской детоксикации, который включает в себя очищение организма от токсинов, нормализацию функций внутренних органов и поддержку психоэмоционального состояния пациента. В случае тяжелого запоя стационарное лечение становится оптимальным решением для безопасного и эффективного вывода из этого состояния. В этой статье мы рассмотрим, как проходит вывод из запоя в стационаре клиники «Перекресток Надежды» в Челябинске, а также особенности анонимности, скорости лечения и участия нарколога.
Детальнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]narkologiya vyvod iz zapoya na domu chelyabinsk[/url]
Алкогольная зависимость — это серьезная проблема, требующая незамедлительного вмешательства, чтобы избежать тяжелых последствий для здоровья. Запойное пьянство может привести к различным заболеваниям, таким как цирроз печени, панкреатит и другие опасные состояния. К счастью, современная медицина предлагает эффективные методы решения этой проблемы. Для тех, кто не хочет или не может посетить стационар, есть возможность пройти процедуру капельницы от запоя на дому в Твери.
Разобраться лучше – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-cena-v-tveri/]vyvod iz zapoya cena v-tveri[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Разобраться лучше – [url=https://vyvod-iz-zapoya-11.ru/]vyvod iz zapoya kapelnica voronezh[/url]
В Воронеже специалисты клиники «Союз Здоровья» оказывают профессиональную помощь пациентам, страдающим от алкогольной зависимости, включая выведение из запоя. Особое внимание уделяется созданию атмосферы доверия и комфорта, что помогает пациентам быстрее адаптироваться к процессу лечения.
Детальнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-stacionare-v-voronezhe/]vyvod iz zapoya v stacionare anonimno v-voronezhe[/url]
Алкогольная зависимость — это длительное и прогрессирующее заболевание, при котором развивается как физическая, так и психическая зависимость от алкоголя. Одним из самых тяжелых проявлений болезни является запой — длительное употребление алкоголя, которое может сопровождаться нарушениями работы организма и абстинентным синдромом, представляющим серьезную угрозу для здоровья. Эффективное лечение запоя требует комплексного подхода, включающего медикаментозную терапию, психотерапию и реабилитационные мероприятия.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]narkolog na dom vyvod iz zapoya tver[/url]
Алкогольная интоксикация, или запой, представляет собой крайне опасное состояние, при котором организм страдает от токсического воздействия алкоголя. Это ведет к нарушению нормальных физиологических процессов и ухудшению общего состояния здоровья. Капельница от запоя — это медицинская процедура, направленная на выведение токсинов из организма и восстановление нормальной функции организма при соблюдении полной конфиденциальности.
Детальнее – [url=https://vyvod-iz-zapoya-21.ru/]вывод из запоя[/url]
Алкогольная зависимость представляет собой актуальную медицинскую проблему, требующую тщательного подхода. Запой характеризуется длительным, непрерывным употреблением спиртных напитков, что ведёт к физической и психической зависимости. На фоне запойного состояния могут возникнуть тяжёлые нарушения функций органов, увеличивается риск развития таких состояний, как алкогольный делирий. Вывод из запоя становится ключевым этапом в лечении зависимостей, который может осуществляться как в стационарных условиях, так и на дому, в зависимости от состояния пациента. В клинике «Перекресток Надежды» в Челябинске мы предлагаем круглосуточную помощь, чтобы обеспечить максимально эффективное и безопасное лечение при любом варианте вывода из запоя.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]срочный вывод из запоя на дому [/url]
Запой — это состояние, при котором человек не может прекратить употребление алкоголя на протяжении длительного времени, что вызывает физическую и психологическую зависимость. Это крайне опасное состояние, требующее немедленного вмешательства специалистов. Наркологическая клиника «Преображение» в Екатеринбурге предоставляет профессиональную помощь в лечении запоя, используя самые эффективные и безопасные методы.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-20.ru/]наркологический центр в екатеринбурге[/url]
Круглосуточная медицинская помощь при алкогольном запое играет важнейшую роль в спасении жизни пациентов и их возвращении к нормальному состоянию. В данной статье рассмотрим ключевые аспекты вывода из запоя в домашних условиях, срочной помощи и работы нарколога.
Узнать больше – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-anonimno-v-ekaterinburge/]анонимный вывод из запоя на дому в екатеринбурге[/url]
Алкогольный запой — одно из тяжелейших состояний, связанное с длительным и неконтролируемым употреблением спиртного. Это не только разрушает физическое и психическое здоровье, но и может стать причиной серьёзных осложнений, таких как поражение печени, проблемы с нервной системой и другие опасные заболевания. Вывод из запоя требует квалифицированной помощи и медицинского вмешательства, чтобы минимизировать риски для здоровья.
Подробнее – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]srochny vyvod iz zapoya na domu [/url]
Алкогольная зависимость — коварное заболевание, которое захватывает не только физическое здоровье, но и разрушает психику, лишает человека воли и заставляет его жить в плену у собственной зависимости. Запой — один из наиболее тяжелых периодов в жизни человека, столкнувшегося с алкоголизмом. Он характеризуется длительным и интенсивным употреблением спиртных напитков, приводящим к глубокой интоксикации организма, нарушению физиологических функций, психическим расстройствам, а также обострению сопутствующих заболеваний.
Углубиться в тему – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]вывод из запоя анонимно недорого краснодар[/url]
Алкогольная зависимость представляет собой серьёзную медицинскую проблему, требующую специализированного подхода. Запойное состояние характеризуется длительным и бесконтрольным потреблением спиртных напитков, что приводит к возникновению как физической, так и психической зависимости. Данное явление может вызвать нарушения в работе внутренних органов, значительно увеличивая вероятность развития алкогольного делирия, который представляет собой острое состояние, требующее неотложной медицинской помощи. Вывод из запоя это ключевая часть лечения зависимости, которая может осуществляться как в стационаре, так и в амбулаторных условиях, в зависимости от конкретной ситуации и состояния пациента.
Узнать больше – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-cena-v-krasnodare/]вывод из запоя цена наркология в краснодаре[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Углубиться в тему – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-anonimno-v-chelyabinske/]vyvod iz zapoya anonimno nedorogo v-chelyabinske[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьёзное воздействие этанола. Это приводит к нарушениям нормальной физиологической деятельности и ухудшению состояния здоровья. Анонимный вывод из запоя — это медицинская помощь, направленная на устранение последствий чрезмерного употребления алкоголя с соблюдением строгой конфиденциальности.
Выяснить больше – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-cena-v-ekaterinburge/]вывод из запоя цена в екатеринбурге[/url]
Клиника «Шаг к Трезвости» в Краснодаре предлагает анонимный вывод из запоя, обеспечивая полную конфиденциальность и безопасность на каждом этапе лечения. Мы понимаем, что для многих людей важно сохранить свою личную информацию в тайне, и гарантируем, что все данные о пациенте и его состоянии здоровья останутся закрытыми. Независимо от того, выбрал ли пациент стационарное лечение или вывод из запоя на дому, мы обеспечиваем высококачественную медицинскую помощь с полным соблюдением принципов конфиденциальности.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-12.ru/]vyvod iz zapoya kapelnica krasnodar[/url]
Клиника «Преображение» предлагает услуги анонимного вывода из запоя в Екатеринбурге, обеспечивая высокое качество медицинской помощи и соблюдение всех этических норм.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]srochny vyvod iz zapoya na domu ekaterinburg[/url]
Алкогольная зависимость представляет собой актуальную медицинскую проблему, требующую тщательного подхода. Запой характеризуется длительным, непрерывным употреблением спиртных напитков, что ведёт к физической и психической зависимости. На фоне запойного состояния могут возникнуть тяжёлые нарушения функций органов, увеличивается риск развития таких состояний, как алкогольный делирий. Вывод из запоя становится ключевым этапом в лечении зависимостей, который может осуществляться как в стационарных условиях, так и на дому, в зависимости от состояния пациента. В клинике «Перекресток Надежды» в Челябинске мы предлагаем круглосуточную помощь, чтобы обеспечить максимально эффективное и безопасное лечение при любом варианте вывода из запоя.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-anonimno-v-chelyabinske/]вывод из запоя анонимно [/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Детальнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]нарколог вывод из запоя цена [/url]
Запой — это тяжелое состояние, возникающее вследствие длительного и непрерывного употребления алкоголя, которое сопровождается физической и психической зависимостью. Это опасное явление требует незамедлительного вмешательства специалистов. Вывод из запоя — это комплексный процесс медицинской детоксикации, который включает в себя очищение организма от токсинов, нормализацию функций внутренних органов и поддержку психоэмоционального состояния пациента. В случае тяжелого запоя стационарное лечение становится оптимальным решением для безопасного и эффективного вывода из этого состояния. В этой статье мы рассмотрим, как проходит вывод из запоя в стационаре клиники «Перекресток Надежды» в Челябинске, а также особенности анонимности, скорости лечения и участия нарколога.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя на дому челябинск[/url]
Гарантированная анонимность при выводе из запоя в Челябинске. В клинике «Перекресток Надежды» мы строго соблюдаем политику конфиденциальности. Информация о пациенте и его состоянии здоровья остаётся закрытой. Это особенно важно для тех, кто ценит свою репутацию и не хочет разглашения. Мы обеспечиваем анонимность как в стационаре, так и при оказании услуг на дому, сохраняя высокий уровень медицинской помощи.
Детальнее – [url=https://vyvod-iz-zapoya-17.ru/]наркологический центр в челябинске[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya na domu nedorogo kapelnica chelyabinsk[/url]
Процедура вывода из запоя направлена на очищение организма от токсинов, нормализацию работы внутренних органов и стабилизацию психоэмоционального состояния. Она может быть проведена как в условиях стационара, так и на дому, в зависимости от степени тяжести состояния. При этом анонимность лечения играет важную роль. Клиника «Перекресток Надежды» гарантирует полную конфиденциальность, что особенно важно для тех, кто ценит приватность. Независимо от выбранного варианта, каждый пациент получает высококачественную помощь, соответствующую современным стандартам медицины.
Выяснить больше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-cena-v-chelyabinske/]нарколог вывод из запоя цена в челябинске[/url]
Вывод из запоя – это важнейший шаг на пути к избавлению от алкогольной зависимости, требующий комплексного подхода и поддержки специалистов. В современных условиях все больше людей предпочитают услуги вывода из запоя на дому, что позволяет избежать стресса от госпитализации и сохранить привычный ритм жизни. Такой подход делает процесс более комфортным и анонимным, что особенно важно для тех, кто стремится сохранить конфиденциальность.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]срочный вывод из запоя на дому в краснодаре[/url]
Мы предлагаем варианты лечения, которые учитывают индивидуальное состояние пациента: от комфортного домашнего выезда до комплексной помощи в стационаре. Стоимость процедур определяется исходя из необходимого объёма вмешательства и всегда остаётся прозрачной, чтобы пациенты могли сосредоточиться на главном — возвращении к полноценной жизни.
Узнать больше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]vyvod iz zapoya kruglosutochno sankt-peterburg[/url]
Выведение из запоя — это важный шаг на пути к восстановлению. Состояние, сопровождаемое как физическими, так и эмоциональными нарушениями, требует внимательного профессионального подхода. Квалифицированная медицинская помощь помогает эффективно устранить симптомы абстиненции и ускорить процесс реабилитации, давая пациентам возможность вернуться к полноценной жизни.
Узнать больше – [url=https://vyvod-iz-zapoya-11.ru/]vyvod iz zapoya s vyezdom[/url]
Проблема зависимостей остаётся актуальной в современном обществе. С каждым годом увеличивается число людей, страдающих от алкоголизма, наркомании и других форм зависимостей, что негативно отражается на их жизни и благополучии близких. Зависимость — это не просто физическое заболевание, но и глубокая психологическая проблема. Для эффективного лечения требуется помощь профессионалов, способных обеспечить комплексный подход.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]narkologiya vyvod iz zapoya na domu [/url]
Алкогольный запой оказывает разрушительное воздействие на физическое и психическое здоровье человека, формируя зависимость и усугубляя общее состояние. Профессиональная помощь при выводе из запоя становится необходимой мерой для восстановления организма. Наркологи выполняют не только медицинские процедуры, но и оказывают психологическую поддержку, помогая пациенту справляться с абстинентным синдромом и другими последствиями длительного употребления алкоголя.
Узнать больше – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]vyvod iz zapoya anonimno krasnodar[/url]
Алкогольный запой — одно из тяжелейших состояний, связанное с длительным и неконтролируемым употреблением спиртного. Это не только разрушает физическое и психическое здоровье, но и может стать причиной серьёзных осложнений, таких как поражение печени, проблемы с нервной системой и другие опасные заболевания. Вывод из запоя требует квалифицированной помощи и медицинского вмешательства, чтобы минимизировать риски для здоровья.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]вывод из запоя цена [/url]
В клинике «Излечение» понимают важность анонимности и индивидуального подхода при лечении алкогольной зависимости. Полная конфиденциальность гарантируется как в стационаре, так и при выезде на дом. Это позволяет пациентам чувствовать себя защищенными и уверенными на пути к выздоровлению. Процесс вывода из запоя включает в себя устранение токсического воздействия алкоголя, восстановление нормального функционирования организма и психологическую поддержку.
Углубиться в тему – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя на дому [/url]
Запой — это состояние, характеризующееся утратой контроля над употреблением алкоголя, что приводит к тяжелой интоксикации организма. Вывод из запоя — это комплексный процесс, направленный на устранение последствий интоксикации и восстановление нормального функционирования организма. Цена данной процедуры может значительно различаться в зависимости от нескольких факторов, и в этой статье мы подробно рассмотрим, что влияет на стоимость вывода из запоя в Краснодаре, в том числе в клинике «Шаг к Трезвости».
Получить больше информации – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-v-stacionare-v-krasnodare/]bystry vyvod iz zapoya v stacionare v-krasnodare[/url]
В клинике «Излечение» мы предлагаем широкий спектр услуг по выводу из запоя, включая как стационарное лечение, так и помощь на дому. В этой статье мы подробно рассмотрим, от чего зависит цена вывода из запоя и какие услуги входят в стоимость.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-16.ru/]вывод из запоя краснодар[/url]
В клинике «Заря Будущего» мы понимаем, насколько важен своевременный и профессиональный подход к лечению зависимости. Наши специалисты готовы оказать квалифицированную помощь прямо у вас дома, обеспечивая полную безопасность и конфиденциальность на каждом этапе лечения.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя с выездом на дом [/url]
Алкогольная зависимость — это серьезная проблема, требующая незамедлительного вмешательства, чтобы избежать тяжелых последствий для здоровья. Запойное пьянство может привести к различным заболеваниям, таким как цирроз печени, панкреатит и другие опасные состояния. К счастью, современная медицина предлагает эффективные методы решения этой проблемы. Для тех, кто не хочет или не может посетить стационар, есть возможность пройти процедуру капельницы от запоя на дому в Твери.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]vyvod iz zapoya s vyezdom na dom tver[/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-17.ru/]vyvod iz zapoya[/url]
В клинике «Рассвет» в Твери мы предлагаем круглосуточную помощь при алкогольных запоях, обеспечивая быстрый вывод токсинов из организма с помощью капельниц и других эффективных методик.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-v-kruglosutochno-v-tveri/]vyvod iz zapoya srochno kruglosutochno v-tveri[/url]
Алкогольная зависимость представляет собой актуальную медицинскую проблему, требующую тщательного подхода. Запой характеризуется длительным, непрерывным употреблением спиртных напитков, что ведёт к физической и психической зависимости. На фоне запойного состояния могут возникнуть тяжёлые нарушения функций органов, увеличивается риск развития таких состояний, как алкогольный делирий. Вывод из запоя становится ключевым этапом в лечении зависимостей, который может осуществляться как в стационарных условиях, так и на дому, в зависимости от состояния пациента. В клинике «Перекресток Надежды» в Челябинске мы предлагаем круглосуточную помощь, чтобы обеспечить максимально эффективное и безопасное лечение при любом варианте вывода из запоя.
Разобраться лучше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя врач на дом челябинск[/url]
Запой — это опасное состояние, которое развивается у людей с алкогольной зависимостью и характеризуется длительным непрерывным потреблением алкоголя. Это состояние приводит к серьезным нарушениям в организме, как физическим, так и психоэмоциональным. Длительный запой может вызвать множество осложнений, таких как поражение внутренних органов, нервной системы, а также проблемы в социальной и семейной жизни. Однако современная медицина предоставляет эффективные методы вывода из запоя, и одним из наиболее удобных и востребованных является вывод из запоя на дому.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-19.ru/]наркологическая клиника в санкт-петербурге[/url]
Вывод из запоя — сложный процесс, включающий несколько этапов. Синдром абстиненции, сопровождающий это состояние, может проявляться в виде как лёгкой нервозности, так и тяжёлых психических расстройств. Только профессиональный подход и современные медицинские методики способны обеспечить успешное восстановление и предотвратить осложнения.
Углубиться в тему – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]вывод из запоя круглосуточно наркология [/url]
Клиника «Преображение» предлагает услуги анонимного вывода из запоя в Екатеринбурге, обеспечивая высокое качество медицинской помощи и соблюдение всех этических норм.
Подробнее тут – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-cena-v-ekaterinburge/]вывод из запоя цена [/url]
Запойное состояние сопряжено с множеством рисков, включая вероятность возникновения алкогольного делирия и других опасных осложнений. Поэтому своевременное обращение к наркологу помогает минимизировать эти угрозы. Круглосуточная доступность специалистов клиники позволяет оперативно реагировать на любые ситуации, обеспечивая пациентам безопасность и комфорт на всех этапах лечения.
Разобраться лучше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя на дому недорого челябинск[/url]
Выведение из запоя — это важный шаг на пути к восстановлению. Состояние, сопровождаемое как физическими, так и эмоциональными нарушениями, требует внимательного профессионального подхода. Квалифицированная медицинская помощь помогает эффективно устранить симптомы абстиненции и ускорить процесс реабилитации, давая пациентам возможность вернуться к полноценной жизни.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-anonimno-v-voronezhe/]anonimny vyvod iz zapoya na domu voronezh[/url]
Запой — это тяжелое состояние, возникающее вследствие длительного и непрерывного употребления алкоголя, которое сопровождается физической и психической зависимостью. Это опасное явление требует незамедлительного вмешательства специалистов. Вывод из запоя — это комплексный процесс медицинской детоксикации, который включает в себя очищение организма от токсинов, нормализацию функций внутренних органов и поддержку психоэмоционального состояния пациента. В случае тяжелого запоя стационарное лечение становится оптимальным решением для безопасного и эффективного вывода из этого состояния. В этой статье мы рассмотрим, как проходит вывод из запоя в стационаре клиники «Перекресток Надежды» в Челябинске, а также особенности анонимности, скорости лечения и участия нарколога.
Детальнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-v-stacionare-v-chelyabinske/]быстрый вывод из запоя в стационаре [/url]
Гарантированная анонимность при выводе из запоя в Челябинске. В клинике «Перекресток Надежды» мы строго соблюдаем политику конфиденциальности. Информация о пациенте и его состоянии здоровья остаётся закрытой. Это особенно важно для тех, кто ценит свою репутацию и не хочет разглашения. Мы обеспечиваем анонимность как в стационаре, так и при оказании услуг на дому, сохраняя высокий уровень медицинской помощи.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-17.ru/]narkologicheski centr[/url]
Запой — это тяжелое состояние, возникающее вследствие длительного и непрерывного употребления алкоголя, которое сопровождается физической и психической зависимостью. Это опасное явление требует незамедлительного вмешательства специалистов. Вывод из запоя — это комплексный процесс медицинской детоксикации, который включает в себя очищение организма от токсинов, нормализацию функций внутренних органов и поддержку психоэмоционального состояния пациента. В случае тяжелого запоя стационарное лечение становится оптимальным решением для безопасного и эффективного вывода из этого состояния. В этой статье мы рассмотрим, как проходит вывод из запоя в стационаре клиники «Перекресток Надежды» в Челябинске, а также особенности анонимности, скорости лечения и участия нарколога.
Узнать больше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-v-kruglosutochno-v-chelyabinske/]вывод из запоя срочно круглосуточно в челябинске[/url]
Наркологическая клиника «Союз Здоровья» в Воронеже специализируется на лечении алкогольной зависимости и предлагает профессиональные услуги по выводу из запоя. Мы стремимся создать атмосферу доверия, где каждый пациент сможет получить необходимую поддержку.
Выяснить больше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]vyvod iz zapoya na domu cena voronezh[/url]
Алкогольный запой оказывает разрушительное воздействие на физическое и психическое здоровье человека, формируя зависимость и усугубляя общее состояние. Профессиональная помощь при выводе из запоя становится необходимой мерой для восстановления организма. Наркологи выполняют не только медицинские процедуры, но и оказывают психологическую поддержку, помогая пациенту справляться с абстинентным синдромом и другими последствиями длительного употребления алкоголя.
Разобраться лучше – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]anonimny vyvod iz zapoya na domu v-krasnodare[/url]
Выход из запоя — это важнейший этап на пути к избавлению от алкогольной зависимости. Он требует комплексного подхода, профессиональной помощи и сильной мотивации пациента. В условиях, когда анонимность и комфорт становятся ключевыми факторами для многих людей, вывод из запоя на дому приобретает все большее значение. Это решение позволяет человеку получить необходимую помощь, не покидая дома, и избежать стресса, связанного с госпитализацией.
Разобраться лучше – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя на дому недорого в краснодаре[/url]
Для эффективного лечения важно обратиться к профессионалам. Наркологическая клиника «Излечение» в Краснодаре предлагает помощь в безопасном и комфортном выведении из запоя, применяя индивидуальный подход к каждому случаю. Алкогольная зависимость разрушает не только физическое здоровье, но и психику, заставляя человека терять волю и становиться заложником собственной зависимости. Запой является одним из самых тяжелых этапов этой болезни: он сопровождается глубокой интоксикацией, нарушением жизненно важных функций организма, психическими расстройствами и обострением хронических заболеваний.
Выяснить больше – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]narkologiya vyvod iz zapoya anonimno [/url]
Алкогольная зависимость представляет собой актуальную медицинскую проблему, требующую тщательного подхода. Запой характеризуется длительным, непрерывным употреблением спиртных напитков, что ведёт к физической и психической зависимости. На фоне запойного состояния могут возникнуть тяжёлые нарушения функций органов, увеличивается риск развития таких состояний, как алкогольный делирий. Вывод из запоя становится ключевым этапом в лечении зависимостей, который может осуществляться как в стационарных условиях, так и на дому, в зависимости от состояния пациента. В клинике «Перекресток Надежды» в Челябинске мы предлагаем круглосуточную помощь, чтобы обеспечить максимально эффективное и безопасное лечение при любом варианте вывода из запоя.
Детальнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя на дому недорого капельница челябинск[/url]
Стоимость вывода из запоя зависит от ряда факторов, таких как состояние пациента, выбранная программа лечения и место проведения процедуры. Мы предлагаем прозрачное ценообразование, чтобы вы могли заранее ознакомиться с возможными затратами на лечение.
Детальнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya s vyezdom na dom v-chelyabinske[/url]
Гарантированная анонимность при выводе из запоя в Челябинске. В клинике «Перекресток Надежды» мы строго соблюдаем политику конфиденциальности. Информация о пациенте и его состоянии здоровья остаётся закрытой. Это особенно важно для тех, кто ценит свою репутацию и не хочет разглашения. Мы обеспечиваем анонимность как в стационаре, так и при оказании услуг на дому, сохраняя высокий уровень медицинской помощи.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya kapelnica na domu v-chelyabinske[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Подробнее – [url=https://vyvod-iz-zapoya-19.ru/]вывод из запоя капельница в санкт-петербурге[/url]
Алкогольный запой — это тяжелое состояние, при котором человек теряет контроль над количеством потребляемого алкоголя. Это приводит к сильной интоксикации, сбоям в работе органов и систем организма, а также серьезным психоэмоциональным расстройствам. Без должной медицинской помощи запой может вызвать ряд опасных последствий, включая алкогольный делирий и угрозу жизни. Поэтому при первых признаках запоя необходимо обратиться к профессионалам.
Подробнее – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-v-stacionare-v-tveri/]вывод из запоя в стационаре тверь[/url]
Алкогольная интоксикация, или запой, представляет собой крайне опасное состояние, при котором организм страдает от токсического воздействия алкоголя. Это ведет к нарушению нормальных физиологических процессов и ухудшению общего состояния здоровья. Капельница от запоя — это медицинская процедура, направленная на выведение токсинов из организма и восстановление нормальной функции организма при соблюдении полной конфиденциальности.
Подробнее тут – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-anonimno-v-tveri/]наркология вывод из запоя анонимно тверь[/url]
Алкогольная зависимость — одна из самых разрушительных форм аддикции, способная нанести непоправимый вред здоровью. Запой, как крайнее проявление этой зависимости, требует немедленного вмешательства, чтобы избежать серьёзных последствий, включая интоксикацию организма и психические расстройства. Обращение за профессиональной помощью становится ключевым шагом на пути к выздоровлению.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]вывод из запоя круглосуточно наркология в санкт-петербурге[/url]
Клиника «Преображение» предлагает услуги анонимного вывода из запоя в Екатеринбурге, обеспечивая высокое качество медицинской помощи и соблюдение всех этических норм.
Выяснить больше – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-anonimno-v-ekaterinburge/]анонимный вывод из запоя на дому в екатеринбурге[/url]
Запой представляет собой состояние, характеризующееся длительным непрерывным употреблением алкоголя, что приводит к физической и психической зависимости. Этот процесс может длиться от нескольких дней до недель. Алкогольные напитки негативно влияют на функционирование внутренних органов, вызывая серьёзные нарушения, включая алкоголизм. При необходимости вывода из запоя важно обратиться за медицинской помощью, что можно осуществить на дому. В данной статье рассмотрим ключевые аспекты данной процедуры.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-cena-v-chelyabinske/]вывод из запоя цена наркология [/url]
Мы предлагаем варианты лечения, которые учитывают индивидуальное состояние пациента: от комфортного домашнего выезда до комплексной помощи в стационаре. Стоимость процедур определяется исходя из необходимого объёма вмешательства и всегда остаётся прозрачной, чтобы пациенты могли сосредоточиться на главном — возвращении к полноценной жизни.
Выяснить больше – [url=https://vyvod-iz-zapoya-14.ru/]наркологический центр в санкт-петербурге[/url]
В Воронеже специалисты клиники «Союз Здоровья» оказывают профессиональную помощь пациентам, страдающим от алкогольной зависимости, включая выведение из запоя. Особое внимание уделяется созданию атмосферы доверия и комфорта, что помогает пациентам быстрее адаптироваться к процессу лечения.
Подробнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]narkologiya vyvod iz zapoya na domu v-voronezhe[/url]
Стоимость вывода из запоя зависит от ряда факторов, таких как состояние пациента, выбранная программа лечения и место проведения процедуры. Мы предлагаем прозрачное ценообразование, чтобы вы могли заранее ознакомиться с возможными затратами на лечение.
Получить больше информации – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-cena-v-chelyabinske/]narkolog vyvod iz zapoya cena chelyabinsk[/url]
Гарантированная анонимность при выводе из запоя в Челябинске. В клинике «Перекресток Надежды» мы строго соблюдаем политику конфиденциальности. Информация о пациенте и его состоянии здоровья остаётся закрытой. Это особенно важно для тех, кто ценит свою репутацию и не хочет разглашения. Мы обеспечиваем анонимность как в стационаре, так и при оказании услуг на дому, сохраняя высокий уровень медицинской помощи.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-v-stacionare-v-chelyabinske/]вывод из запоя в стационаре [/url]
Запой — это состояние, характеризующееся утратой контроля над употреблением алкоголя, что приводит к тяжелой интоксикации организма. Вывод из запоя — это комплексный процесс, направленный на устранение последствий интоксикации и восстановление нормального функционирования организма. Цена данной процедуры может значительно различаться в зависимости от нескольких факторов, и в этой статье мы подробно рассмотрим, что влияет на стоимость вывода из запоя в Краснодаре, в том числе в клинике «Шаг к Трезвости».
Разобраться лучше – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-cena-v-krasnodare/]vyvod iz zapoya s vyezdom cena [/url]
Алкогольные запои — это одно из самых сложных проявлений зависимости, требующее профессионального подхода и незамедлительных действий. В такие периоды близкие люди часто не знают, как помочь, но своевременное обращение за медицинской помощью может стать ключом к восстановлению. В Челябинске клиника «Перекресток Надежды» предоставляет квалифицированные услуги по выводу из запоя, сочетая современные методики лечения с поддержкой на психологическом уровне.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya na domu kruglosutochno chelyabinsk[/url]
Запой — это серьезное состояние, при котором человек не может прекратить употребление алкоголя на протяжении длительного времени, что вызывает физическую и психологическую зависимость. Это крайне опасное состояние, требующее немедленного вмешательства специалистов. Для безопасного и эффективного вывода из запоя необходима квалифицированная медицинская помощь, которую предоставляет наркологическая клиника «Рассвет» в Твери. Наши опытные специалисты используют современные методы детоксикации и восстановления организма, обеспечивая полный медицинский контроль и поддержку на всех этапах лечения.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-v-stacionare-v-tveri/]bystry vyvod iz zapoya v stacionare tver[/url]
В клинике «Рассвет» в Твери мы предлагаем круглосуточную помощь при алкогольных запоях, обеспечивая быстрый вывод токсинов из организма с помощью капельниц и других эффективных методик.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-v-kruglosutochno-v-tveri/]вывод из запоя круглосуточно тверь[/url]
Запойное состояние представляет собой серьёзную угрозу для здоровья, поскольку сопровождается тяжёлой интоксикацией организма и нарушением психоэмоционального состояния. Решение о лечении в стационаре клиники «Союз Здоровья» позволяет справиться с последствиями длительного употребления алкоголя, обеспечивая пациентам полный комплекс медицинской и психологической помощи.
Разобраться лучше – [url=https://vyvod-iz-zapoya-11.ru/]https://vyvod-iz-zapoya-11.ru[/url]
В клинике работает команда квалифицированных специалистов, которые осуществляют вывод из запоя, обеспечивая полный контроль над состоянием пациента и устраняя все последствия длительного употребления алкоголя.
Подробнее тут – [url=https://vyvod-iz-zapoya-20.ru/]vyvod iz zapoya gorod ekaterinburg[/url]
Алкогольный запой — это серьезное заболевание, которое требует немедленного и профессионального вмешательства. Запой сопровождается не только физическими страданиями, но и эмоциональными трудностями. Процесс выведения из запоя требует срочного внимания, так как состояние пациента может ухудшиться, если не получить квалифицированную помощь вовремя. Наркологическая клиника «Излечение» в Краснодаре предоставляет круглосуточную помощь при алкогольном запое, чтобы восстановить здоровье пациента в самых сложных ситуациях.
Узнать больше – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]vyvod iz zapoya srochno kruglosutochno krasnodar[/url]
Алкогольная зависимость представляет собой серьёзную медицинскую проблему, требующую специализированного подхода. Запойное состояние характеризуется длительным и бесконтрольным потреблением спиртных напитков, что приводит к возникновению как физической, так и психической зависимости. Данное явление может вызвать нарушения в работе внутренних органов, значительно увеличивая вероятность развития алкогольного делирия, который представляет собой острое состояние, требующее неотложной медицинской помощи. Вывод из запоя это ключевая часть лечения зависимости, которая может осуществляться как в стационаре, так и в амбулаторных условиях, в зависимости от конкретной ситуации и состояния пациента.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]srochny vyvod iz zapoya na domu v-krasnodare[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя вызов на дом [/url]
Запой представляет собой сложное состояние, связанное с длительным употреблением алкоголя, что приводит к формированию зависимости. Это явление не только нарушает нормальную жизнедеятельность, но также вызывает серьезные физические и психологические расстройства. Стационарное лечение становится необходимым для восстановления здоровья и профилактики осложнений. В стационаре клиники «Шаг к Трезвости» в Краснодаре мы предлагаем комплексный подход, включающий медикаментозное лечение, психотерапию и постоянный контроль со стороны квалифицированных специалистов.
Узнать больше – [url=https://vyvod-iz-zapoya-12.ru/]vyvod iz zapoya v-krasnodare[/url]
Мы предлагаем варианты лечения, которые учитывают индивидуальное состояние пациента: от комфортного домашнего выезда до комплексной помощи в стационаре. Стоимость процедур определяется исходя из необходимого объёма вмешательства и всегда остаётся прозрачной, чтобы пациенты могли сосредоточиться на главном — возвращении к полноценной жизни.
Разобраться лучше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя на дому недорого [/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Выяснить больше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-v-stacionare-v-chelyabinske/]vyvod iz zapoya v stacionare anonimno v-chelyabinske[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьезное воздействие этанола, что приводит к нарушению нормальной физиологической деятельности. Это состояние сопровождается сильными физическими и психологическими расстройствами, которые требуют профессионального вмешательства. Однако многие люди, страдающие алкогольной зависимостью, сталкиваются с трудностью признания своей проблемы, опасаясь утраты репутации и конфиденциальности. В клинике «Заря Будущего» мы предлагаем анонимный вывод из запоя в Санкт-Петербурге, предоставляя высококачественное лечение с гарантией сохранения личной информации.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]vyvod iz zapoya kruglosutochno narkologiya sankt-peterburg[/url]
Вывод из запоя – это важнейший шаг на пути к избавлению от алкогольной зависимости, требующий комплексного подхода и поддержки специалистов. В современных условиях все больше людей предпочитают услуги вывода из запоя на дому, что позволяет избежать стресса от госпитализации и сохранить привычный ритм жизни. Такой подход делает процесс более комфортным и анонимным, что особенно важно для тех, кто стремится сохранить конфиденциальность.
Узнать больше – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]narkologiya vyvod iz zapoya na domu krasnodar[/url]
Алкогольный запой оказывает разрушительное воздействие на физическое и психическое здоровье человека, формируя зависимость и усугубляя общее состояние. Профессиональная помощь при выводе из запоя становится необходимой мерой для восстановления организма. Наркологи выполняют не только медицинские процедуры, но и оказывают психологическую поддержку, помогая пациенту справляться с абстинентным синдромом и другими последствиями длительного употребления алкоголя.
Разобраться лучше – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя на дому недорого краснодар[/url]
Выведение из запоя — это важный шаг на пути к восстановлению. Состояние, сопровождаемое как физическими, так и эмоциональными нарушениями, требует внимательного профессионального подхода. Квалифицированная медицинская помощь помогает эффективно устранить симптомы абстиненции и ускорить процесс реабилитации, давая пациентам возможность вернуться к полноценной жизни.
Углубиться в тему – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]vyvod iz zapoya cena narkologiya v-voronezhe[/url]
Алкоголизм — это хроническое прогрессирующее заболевание, связанное с физической и психической зависимостью от этанола. Запой, как его крайнее проявление, представляет собой длительное употребление алкоголя, сопровождающееся тяжелыми нарушениями работы организма, включая абстинентный синдром и потенциальные угрозы для жизни. Лечение запоя требует комплексного подхода, включающего медицинские процедуры, психотерапию и реабилитационные меры.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-v-kruglosutochno-v-ekaterinburge/]vyvod iz zapoya srochno kruglosutochno [/url]
Клиника «Шаг к Трезвости» в Краснодаре предлагает анонимный вывод из запоя, обеспечивая полную конфиденциальность и безопасность на каждом этапе лечения. Мы понимаем, что для многих людей важно сохранить свою личную информацию в тайне, и гарантируем, что все данные о пациенте и его состоянии здоровья останутся закрытыми. Независимо от того, выбрал ли пациент стационарное лечение или вывод из запоя на дому, мы обеспечиваем высококачественную медицинскую помощь с полным соблюдением принципов конфиденциальности.
Получить больше информации – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]vyvod iz zapoya s vyezdom na dom krasnodar[/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Подробнее тут – [url=https://vyvod-iz-zapoya-19.ru/]vyvod iz zapoya[/url]
Алкогольная зависимость представляет собой серьезную медицинскую проблему, которая влечет за собой не только физические, но и психологические проблемы. Периоды запоя, характеризующиеся бесконтрольным употреблением алкоголя, могут привести к тяжелым последствиям. Симптомы абстиненции требуют незамедлительного вмешательства. Анонимность в процессе реабилитации становится важным аспектом, помогающим пациентам избежать стыда и социального осуждения.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]нарколог на дом вывод из запоя [/url]
Запойное состояние сопряжено с множеством рисков, включая вероятность возникновения алкогольного делирия и других опасных осложнений. Поэтому своевременное обращение к наркологу помогает минимизировать эти угрозы. Круглосуточная доступность специалистов клиники позволяет оперативно реагировать на любые ситуации, обеспечивая пациентам безопасность и комфорт на всех этапах лечения.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]narkolog na dom vyvod iz zapoya [/url]
В клинике «Излечение» мы предлагаем широкий спектр услуг по выводу из запоя, включая как стационарное лечение, так и помощь на дому. В этой статье мы подробно рассмотрим, от чего зависит цена вывода из запоя и какие услуги входят в стоимость.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]вывод из запоя анонимно краснодар[/url]
Запой — это тяжелое состояние, возникающее вследствие длительного и непрерывного употребления алкоголя, которое сопровождается физической и психической зависимостью. Это опасное явление требует незамедлительного вмешательства специалистов. Вывод из запоя — это комплексный процесс медицинской детоксикации, который включает в себя очищение организма от токсинов, нормализацию функций внутренних органов и поддержку психоэмоционального состояния пациента. В случае тяжелого запоя стационарное лечение становится оптимальным решением для безопасного и эффективного вывода из этого состояния. В этой статье мы рассмотрим, как проходит вывод из запоя в стационаре клиники «Перекресток Надежды» в Челябинске, а также особенности анонимности, скорости лечения и участия нарколога.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя на дому круглосуточно челябинск[/url]
Willingly I accept. In my opinion, it is an interesting question, I will take part in discussion. Together we can come to a right answer. I am assured.
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Подробнее тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-anonimno-v-voronezhe/]vyvod iz zapoya anonimno nedorogo [/url]
Гарантированная анонимность при выводе из запоя в Челябинске. В клинике «Перекресток Надежды» мы строго соблюдаем политику конфиденциальности. Информация о пациенте и его состоянии здоровья остаётся закрытой. Это особенно важно для тех, кто ценит свою репутацию и не хочет разглашения. Мы обеспечиваем анонимность как в стационаре, так и при оказании услуг на дому, сохраняя высокий уровень медицинской помощи.
Узнать больше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya na domu nedorogo kapelnica v-chelyabinske[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьёзное воздействие этанола. Это приводит к нарушениям нормальной физиологической деятельности и ухудшению состояния здоровья. Анонимный вывод из запоя — это медицинская помощь, направленная на устранение последствий чрезмерного употребления алкоголя с соблюдением строгой конфиденциальности.
Выяснить больше – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]вывод из запоя врач на дом [/url]
Вывод из запоя – это важнейший шаг на пути к избавлению от алкогольной зависимости, требующий комплексного подхода и поддержки специалистов. В современных условиях все больше людей предпочитают услуги вывода из запоя на дому, что позволяет избежать стресса от госпитализации и сохранить привычный ритм жизни. Такой подход делает процесс более комфортным и анонимным, что особенно важно для тех, кто стремится сохранить конфиденциальность.
Углубиться в тему – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]срочный вывод из запоя на дому краснодар[/url]
Запой — это состояние, характеризующееся утратой контроля над употреблением алкоголя, что приводит к тяжелой интоксикации организма. Вывод из запоя — это комплексный процесс, направленный на устранение последствий интоксикации и восстановление нормального функционирования организма. Цена данной процедуры может значительно различаться в зависимости от нескольких факторов, и в этой статье мы подробно рассмотрим, что влияет на стоимость вывода из запоя в Краснодаре, в том числе в клинике «Шаг к Трезвости».
Получить больше информации – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]анонимный вывод из запоя на дому краснодар[/url]
Вывод из запоя – это важнейший шаг на пути к избавлению от алкогольной зависимости, требующий комплексного подхода и поддержки специалистов. В современных условиях все больше людей предпочитают услуги вывода из запоя на дому, что позволяет избежать стресса от госпитализации и сохранить привычный ритм жизни. Такой подход делает процесс более комфортным и анонимным, что особенно важно для тех, кто стремится сохранить конфиденциальность.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-stacionare-v-krasnodare/]http://www.vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-stacionare-v-krasnodare/[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Получить больше информации – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya vyzov na dom [/url]
Алкогольная зависимость становится всё более распространенной проблемой, требующей внимания как со стороны медицинского сообщества, так и общества в целом. Вывод из запоя — это сложный и ответственный процесс, необходимый для восстановления здоровья пациента и его интеграции в нормальную жизнь. Профессиональное вмешательство позволяет минимизировать последствия длительного употребления алкоголя и нормализовать состояние организма.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-v-stacionare-v-ekaterinburge/]bystry vyvod iz zapoya v stacionare [/url]
Запойное пьянство характеризуется продолжительным и интенсивным потреблением алкоголя, что приводит к серьезным физическим и психологическим последствиям. Часто пациенты сталкиваются с различными заболеваниями, такими как цирроз печени, панкреатит, алкогольный делирий и другие. Алкогольное опьянение не только ухудшает качество жизни, но также может угрожать жизни пациента. В условиях стационара лечение может быть более эффективным, однако многие люди предпочитают проходить данную процедуру на дому, чтобы избежать стигматизации и сохранить чувство приватности.
Подробнее тут – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]vyvod iz zapoya vrach na dom ekaterinburg[/url]
Запой — это тяжелое состояние, при котором человек теряет контроль над количеством употребляемого алкоголя, что приводит к серьезным нарушениям здоровья. Процесс вывода из запоя требует профессионального подхода, медицинского контроля и тщательного восстановления организма. Наркологическая клиника «Шаг к Трезвости» в Краснодаре предлагает квалифицированную помощь для тех, кто нуждается в срочном и безопасном выходе из запоя. Мы предоставляем комплексное лечение, ориентированное на каждый этап восстановления, с максимальной заботой о здоровье пациента.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя капельница на дому [/url]
Запой — это тяжелое состояние, возникающее вследствие длительного и непрерывного употребления алкоголя, которое сопровождается физической и психической зависимостью. Это опасное явление требует незамедлительного вмешательства специалистов. Вывод из запоя — это комплексный процесс медицинской детоксикации, который включает в себя очищение организма от токсинов, нормализацию функций внутренних органов и поддержку психоэмоционального состояния пациента. В случае тяжелого запоя стационарное лечение становится оптимальным решением для безопасного и эффективного вывода из этого состояния. В этой статье мы рассмотрим, как проходит вывод из запоя в стационаре клиники «Перекресток Надежды» в Челябинске, а также особенности анонимности, скорости лечения и участия нарколога.
Выяснить больше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-v-kruglosutochno-v-chelyabinske/]вывод из запоя срочно круглосуточно [/url]
Алкогольная интоксикация, или запой, представляет собой крайне опасное состояние, при котором организм страдает от токсического воздействия алкоголя. Это ведет к нарушению нормальных физиологических процессов и ухудшению общего состояния здоровья. Капельница от запоя — это медицинская процедура, направленная на выведение токсинов из организма и восстановление нормальной функции организма при соблюдении полной конфиденциальности.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-anonimno-v-tveri/]narkologiya vyvod iz zapoya anonimno tver[/url]
Клиника «Преображение» предлагает услуги анонимного вывода из запоя в Екатеринбурге, обеспечивая высокое качество медицинской помощи и соблюдение всех этических норм.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-anonimno-v-ekaterinburge/]anonimny vyvod iz zapoya na domu [/url]
Выведение из запоя — это важный шаг на пути к восстановлению. Состояние, сопровождаемое как физическими, так и эмоциональными нарушениями, требует внимательного профессионального подхода. Квалифицированная медицинская помощь помогает эффективно устранить симптомы абстиненции и ускорить процесс реабилитации, давая пациентам возможность вернуться к полноценной жизни.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-kruglosutochno-v-voronezhe/]vyvod iz zapoya kruglosutochno narkologiya [/url]
Алкогольная зависимость представляет собой серьёзную медицинскую проблему, требующую специализированного подхода. Запойное состояние характеризуется длительным и бесконтрольным потреблением спиртных напитков, что приводит к возникновению как физической, так и психической зависимости. Данное явление может вызвать нарушения в работе внутренних органов, значительно увеличивая вероятность развития алкогольного делирия, который представляет собой острое состояние, требующее неотложной медицинской помощи. Вывод из запоя это ключевая часть лечения зависимости, которая может осуществляться как в стационаре, так и в амбулаторных условиях, в зависимости от конкретной ситуации и состояния пациента.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]vyvod iz zapoya vrach na dom [/url]
[url=https://meqa.fo]mega sb вход[/url] – megaweb12.at, как зайти на мегу
Стоимость вывода из запоя зависит от ряда факторов, таких как состояние пациента, выбранная программа лечения и место проведения процедуры. Мы предлагаем прозрачное ценообразование, чтобы вы могли заранее ознакомиться с возможными затратами на лечение.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-v-stacionare-v-chelyabinske/]bystry vyvod iz zapoya v stacionare v-chelyabinske[/url]
Запой представляет собой состояние, связанное с длительным употреблением алкогольных напитков, что приводит к значительным физическим и психическим расстройствам. Это явление формирует зависимость, оказывая негативное воздействие на здоровье. Вывод из запоя в стационаре становится необходимостью для восстановительных мероприятий, направленных на реабилитацию пациента. В этой статье рассматриваются аспекты анонимного вывода, скорость процедур, а также роль нарколога в процессе лечения.
Детальнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]вывод из запоя круглосуточно краснодар[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-19.ru/]вывод из запоя капельница[/url]
Клиника «Преображение» предлагает услуги анонимного вывода из запоя в Екатеринбурге, обеспечивая высокое качество медицинской помощи и соблюдение всех этических норм.
Углубиться в тему – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-anonimno-v-ekaterinburge/]наркология вывод из запоя анонимно екатеринбург[/url]
Стоимость процедур зависит от состояния пациента, программы терапии и выбранных условий — стационар или вызов врача на дом. Клиника предоставляет прозрачную информацию о ценах, чтобы вы могли заранее оценить предстоящие затраты. Профессиональный подход и индивидуальные решения для каждого пациента помогают не только выйти из запойного состояния, но и сделать шаг к полноценной жизни.
Углубиться в тему – [url=https://vyvod-iz-zapoya-17.ru/]vyvod iz zapoya s vyezdom chelyabinsk[/url]
Запойное состояние представляет собой серьёзную угрозу для здоровья, поскольку сопровождается тяжёлой интоксикацией организма и нарушением психоэмоционального состояния. Решение о лечении в стационаре клиники «Союз Здоровья» позволяет справиться с последствиями длительного употребления алкоголя, обеспечивая пациентам полный комплекс медицинской и психологической помощи.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-stacionare-v-voronezhe/]vyvod iz zapoya v stacionare v-voronezhe[/url]
read review [url=https://sites.google.com/mycryptowalletus.com/metamask-walletlogin/]Metamask Extension[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-v-stacionare-v-chelyabinske/]вывод из запоя в стационаре анонимно челябинск[/url]
resource [url=https://sites.google.com/mycryptowalletus.com/phantomwalletlogin/]phantom Extension[/url]
Многие люди избегают обращения за помощью из-за страха утраты конфиденциальности. В клинике строго соблюдается анонимность, что позволяет пациентам чувствовать себя защищёнными и уверенными. Высокий уровень профессионализма и индивидуальный подход создают комфортные условия для выздоровления.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]vyvod iz zapoya na domu nedorogo [/url]
Для тех, кто не готов к госпитализации, клиника “Второй Шанс” предлагает услугу вывода из запоя на дому. Высококвалифицированные специалисты обеспечивают комфортное и безопасное лечение в привычных для пациента условиях, применяя современные методы, которые гарантируют эффективность терапии. Однако в ряде случаев, особенно при наличии осложнений, может потребоваться круглосуточный стационарный контроль и специализированная поддержка.
Выяснить больше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя капельница на дому санкт-петербург[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Узнать больше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]narkologiya vyvod iz zapoya na domu chelyabinsk[/url]
В этой статье мы расскажем о возможностях клиники для лечения алкогольной интоксикации круглосуточно, включая вывод из запоя в стационаре и на дому, а также о роли профессионалов, таких как нарколог, в процессе лечения.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]http://www.vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/[/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]вывод из запоя на дому круглосуточно в воронеже[/url]
imp source [url=https://sites.google.com/mycryptowalletus.com/metamaskwalletlogin/]MetaMask Download[/url]
В клинике «Излечение» понимают важность анонимности и индивидуального подхода при лечении алкогольной зависимости. Полная конфиденциальность гарантируется как в стационаре, так и при выезде на дом. Это позволяет пациентам чувствовать себя защищенными и уверенными на пути к выздоровлению. Процесс вывода из запоя включает в себя устранение токсического воздействия алкоголя, восстановление нормального функционирования организма и психологическую поддержку.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]narkologiya vyvod iz zapoya na domu krasnodar[/url]
Мы предлагаем широкий спектр услуг: от амбулаторной помощи до стационарного лечения. Анонимность, оперативность и индивидуальный подход — главные принципы нашей работы. Независимо от сложности ситуации, мы стремимся оказать помощь каждому, кто обратился за поддержкой, и обеспечить восстановление физического и психологического состояния.
Получить больше информации – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]анонимный вывод из запоя на дому в санкт-петербурге[/url]
Запойное состояние сопряжено с множеством рисков, включая вероятность возникновения алкогольного делирия и других опасных осложнений. Поэтому своевременное обращение к наркологу помогает минимизировать эти угрозы. Круглосуточная доступность специалистов клиники позволяет оперативно реагировать на любые ситуации, обеспечивая пациентам безопасность и комфорт на всех этапах лечения.
Детальнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya na domu kruglosutochno [/url]
blog link [url=https://phantom-wallet.at/]phantom Extension[/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-anonimno-v-chelyabinske/]вывод из запоя анонимно недорого челябинск[/url]
why not check here [url=https://sites.google.com/mycryptowalletus.com/metamask-wallet-login/]MetaMask Download[/url]
check this link right here now [url=https://keplr-extension.com]keplr wallet[/url]
my website [url=https://sites.google.com/mycryptowalletus.com/phantom-walletlogin/]phantom Download[/url]
more tips here [url=https://sites.google.com/mycryptowalletus.com/phantom-wallet-login/]phantom wallet[/url]
Source [url=https://Keplr.at]keplr Extension[/url]
Discover More Here [url=https://rabby-wallet.net/]rabby wallet[/url]
hop over to here [url=https://phantom-wallet.net]phantom Extension[/url]
Клиника «Рассвет» в Твери предоставляет услугу анонимного вывода из запоя, обеспечивая высокое качество медицинского обслуживания и строгое соблюдение всех этических норм.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-21.ru/]вывод из запоя тверь[/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Детальнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu nedorogo kapelnica [/url]
Алкогольная зависимость — это одна из самых распространённых форм аддиктивного поведения, которая оказывает разрушительное воздействие на физическое и психическое здоровье человека. Запой, как тяжелое состояние, характеризуется длительным и бесконтрольным употреблением спиртных напитков, что приводит к серьёзным последствиям, таким как разрушение органов, психические расстройства и высокий риск летального исхода. В таких случаях необходимо срочное и квалифицированное вмешательство специалистов, чтобы предотвратить осложнения и восстановить здоровье пациента.
Подробнее – [url=https://vyvod-iz-zapoya-14.ru/]http://www.vyvod-iz-zapoya-14.ru[/url]
Лучшие стоматологические клиники в Минске, где вы можете получить качественное лечение, и улучшить состояние вашей полости рта.
Услуги стоматолога цены [url=https://belamed.ru]https://belamed.ru[/url] .
Для достижения эффективных результатов в лечении запоя необходима квалифицированная помощь специалистов. Наркологическая клиника «Излечение» в Краснодаре предоставляет профессиональные услуги по выводу из запоя, гарантируя безопасность, комфорт и индивидуальный подход к каждому пациенту.
Подробнее тут – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-cena-v-krasnodare/]вывод из запоя с выездом цена краснодар[/url]
Актуальные цены на стоматологию в Минске, ознакомьтесь на нашем ресурсе.
Стоматология недорого цены на услуги [url=https://www.dentistblog.ru/]https://www.dentistblog.ru/[/url] .
Запой представляет собой сложное состояние, связанное с длительным употреблением алкоголя, что приводит к формированию зависимости. Это явление не только нарушает нормальную жизнедеятельность, но также вызывает серьезные физические и психологические расстройства. Стационарное лечение становится необходимым для восстановления здоровья и профилактики осложнений. В стационаре клиники «Шаг к Трезвости» в Краснодаре мы предлагаем комплексный подход, включающий медикаментозное лечение, психотерапию и постоянный контроль со стороны квалифицированных специалистов.
Получить больше информации – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]вывод из запоя срочно круглосуточно [/url]
Алкогольный запой — это серьезное заболевание, которое требует немедленного и профессионального вмешательства. Запой сопровождается не только физическими страданиями, но и эмоциональными трудностями. Процесс выведения из запоя требует срочного внимания, так как состояние пациента может ухудшиться, если не получить квалифицированную помощь вовремя. Наркологическая клиника «Излечение» в Краснодаре предоставляет круглосуточную помощь при алкогольном запое, чтобы восстановить здоровье пациента в самых сложных ситуациях.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/[/url]
В центре доступны различные программы лечения, от амбулаторного наблюдения до интенсивного стационарного курса. Основные принципы работы – анонимность, оперативность и персонализированный подход. Независимо от сложности ситуации, каждый пациент получает квалифицированную помощь, направленную на восстановление физического и психического здоровья.
Разобраться лучше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]srochny vyvod iz zapoya na domu v-sankt-peterburge[/url]
Медицинская помощь при запоях направлена не только на устранение симптомов интоксикации, но и на предотвращение рецидивов. Современные методики лечения включают медикаментозную терапию, психологическую поддержку и индивидуальный подход к каждому пациенту. Одним из ключевых аспектов эффективного лечения является анонимность, позволяющая избежать социального осуждения и дискомфорта, связанного с обращением к наркологам.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-v-stacionare-v-krasnodare/]bystry vyvod iz zapoya v stacionare v-krasnodare[/url]
Стоимость процедур формируется в зависимости от состояния пациента, выбранного способа лечения и дополнительных медицинских услуг. Прозрачность ценообразования позволяет заранее оценить предстоящие расходы и выбрать оптимальный вариант. Комплексный подход, профессионализм врачей и индивидуальные схемы терапии помогают не только выйти из запойного состояния, но и сделать уверенный шаг к здоровой и полноценной жизни.
Углубиться в тему – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-cena-v-chelyabinske/]нарколог вывод из запоя цена в челябинске[/url]
Запой представляет собой сложное состояние, связанное с длительным употреблением алкоголя, что приводит к формированию зависимости. Это явление не только нарушает нормальную жизнедеятельность, но также вызывает серьезные физические и психологические расстройства. Стационарное лечение становится необходимым для восстановления здоровья и профилактики осложнений. В стационаре клиники «Шаг к Трезвости» в Краснодаре мы предлагаем комплексный подход, включающий медикаментозное лечение, психотерапию и постоянный контроль со стороны квалифицированных специалистов.
Выяснить больше – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]наркология вывод из запоя анонимно в краснодаре[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Подробнее тут – [url=https://vyvod-iz-zapoya-11.ru/]https://vyvod-iz-zapoya-11.ru[/url]
Алкогольный запой представляет собой критическое состояние, требующее немедленного вмешательства квалифицированных специалистов. Клиника «Союз Здоровья» в Воронеже предлагает круглосуточную помощь, чтобы обеспечить своевременное и эффективное лечение. Наша цель — оперативное снятие интоксикации, устранение симптомов и предотвращение осложнений.
Подробнее тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-kruglosutochno-v-voronezhe/]вывод из запоя круглосуточно воронеж[/url]
Алкогольное злоупотребление, перетекающее в длительные запои, представляет серьезную угрозу как физическому, так и психическому здоровью. Потеря контроля над количеством выпитого приводит к глубокой интоксикации организма, ухудшению самочувствия и развитию зависимости. В таких случаях необходима срочная медицинская помощь, чтобы избежать тяжелых последствий и стабилизировать состояние пациента.
Узнать больше – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя на дому краснодар[/url]
Наркологическая клиника «Освобождение» в Рязани предлагает профессиональные услуги по выводу из запоя и комплексной реабилитации. Мы понимаем, что зависимость — это болезнь, требующая индивидуального подхода и поддержки не только для пациента, но и для его близких.
Подробнее тут – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-cena-v-ryazani/]vyvod iz zapoya cena [/url]
Алкоголизм — это хроническое заболевание, которое характеризуется патологической зависимостью от этанола. Периоды запоя, когда человек неконтролируемо потребляет алкоголь, могут привести к разнообразным медицинским осложнениям, как физическим, так и психологическим. Запой становится не только причиной ухудшения здоровья, но и причиной множества социальных и личных проблем. На этом фоне возникает необходимость вывода из запоя, процесс, который должен учитывать индивидуальные особенности пациента. Важно, что анонимность в реабилитации играет ключевую роль, помогая избежать стигматизации и увеличивая шансы на успешное восстановление.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-anonimno-v-ryazani/]vyvod iz zapoya anonimno [/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-stacionare-v-voronezhe/]быстрый вывод из запоя в стационаре [/url]
Стоимость процедур формируется в зависимости от состояния пациента, выбранного способа лечения и дополнительных медицинских услуг. Прозрачность ценообразования позволяет заранее оценить предстоящие расходы и выбрать оптимальный вариант. Комплексный подход, профессионализм врачей и индивидуальные схемы терапии помогают не только выйти из запойного состояния, но и сделать уверенный шаг к здоровой и полноценной жизни.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя на дому челябинск[/url]
Особую опасность представляет алкогольный запой – состояние, сопровождающееся многодневным бесконтрольным употреблением спиртного. Оно разрушает здоровье, негативно влияет на нервную систему, печень и другие органы, а также может привести к тяжёлым осложнениям. Чтобы минимизировать риски, необходимо медицинское вмешательство. Вывести человека из запоя самостоятельно крайне сложно и небезопасно, поэтому важно вовремя обратиться за квалифицированной помощью.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]вывод из запоя круглосуточно [/url]
Алкогольная зависимость — это хроническое заболевание, которое сопровождается неконтролируемым стремлением к употреблению спиртного. Регулярные запои приводят не только к разрушению организма, но и к ухудшению психоэмоционального состояния, социальным проблемам и конфликтам. Чтобы избавиться от этой зависимости, необходимо начать с детоксикации и постепенной реабилитации, адаптированной под конкретного пациента. Важным аспектом в этом процессе является сохранение анонимности, позволяющее человеку избежать осуждения и сосредоточиться на выздоровлении.
Детальнее – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-v-kruglosutochno-v-ryazani/]вывод из запоя круглосуточно наркология рязань[/url]
Зависимость – серьёзная проблема, с которой сталкиваются люди по всему миру. Повседневные трудности, постоянный стресс и финансовые неурядицы нередко становятся катализаторами таких расстройств, как алкоголизм, наркомания и игромания. Медицинский центр «Второй Шанс» специализируется на помощи людям, стремящимся избавиться от этих заболеваний, восстановить здоровье, адаптироваться к жизни без вредных привычек и избежать рецидивов.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]vyvod iz zapoya kruglosutochno sankt-peterburg[/url]
Процедура вывода из запоя — это не просто очищение организма от токсинов, а комплекс мер, направленных на восстановление физического состояния и психического равновесия. Специалисты клиники помогают минимизировать последствия длительного употребления алкоголя, снижая риск осложнений и помогая пациенту вернуться к нормальной жизни. Чем быстрее начато лечение, тем выше шансы на успешное восстановление и отказ от пагубной привычки.
Детальнее – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]срочный вывод из запоя на дому [/url]
Вывод из запоя представляет собой важный этап в процессе лечения алкогольной зависимости. Это медицинская процедура, необходимая для устранения токсических веществ, накопленных в организме в результате длительного употребления алкоголя. Алкогольная зависимость может вызывать множество проблем, включая физические и психоэмоциональные расстройства. Своевременное вмешательство специалистов помогает минимизировать риски и способствует восстановлению здоровья пациента.
Выяснить больше – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]vyvod iz zapoya vyzov na dom ryazan[/url]
В медицинском центре «Второй Шанс» помощь оказывается круглосуточно. Применяются эффективные и проверенные методы лечения, направленные на безопасность пациента и достижение устойчивого результата. Полная конфиденциальность позволяет людям, обратившимся за поддержкой, чувствовать себя комфортно и сосредоточиться на своём выздоровлении.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]наркология вывод из запоя на дому [/url]
Клиника «Шаг к Трезвости» в Краснодаре предлагает анонимный вывод из запоя, обеспечивая полную конфиденциальность и безопасность на каждом этапе лечения. Мы понимаем, что для многих людей важно сохранить свою личную информацию в тайне, и гарантируем, что все данные о пациенте и его состоянии здоровья останутся закрытыми. Независимо от того, выбрал ли пациент стационарное лечение или вывод из запоя на дому, мы обеспечиваем высококачественную медицинскую помощь с полным соблюдением принципов конфиденциальности.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]anonimny vyvod iz zapoya na domu [/url]
Запой представляет серьёзную угрозу, поэтому откладывать обращение за медицинской помощью нельзя. Чем раньше будет проведена детоксикация и поддерживающая терапия, тем ниже риск осложнений. В клинике «Освобождение» работают круглосуточно, оперативно реагируя на экстренные случаи и предлагая как стационарное лечение, так и выездные услуги — в зависимости от состояния пациента и его пожеланий.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]наркология вывод из запоя на дому [/url]
Стоимость процедуры зависит от множества факторов, включая тяжесть состояния пациента, необходимость дополнительных медицинских манипуляций и выбранный формат лечения. Однако главное – не откладывать обращение за помощью, поскольку запойное состояние несет серьезные риски для жизни и здоровья.
Подробнее – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]vyvod iz zapoya na domu [/url]
Зависимость – серьёзная проблема, с которой сталкиваются люди по всему миру. Повседневные трудности, постоянный стресс и финансовые неурядицы нередко становятся катализаторами таких расстройств, как алкоголизм, наркомания и игромания. Медицинский центр «Второй Шанс» специализируется на помощи людям, стремящимся избавиться от этих заболеваний, восстановить здоровье, адаптироваться к жизни без вредных привычек и избежать рецидивов.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]vyvod iz zapoya v stacionare [/url]
Запойное состояние представляет собой серьёзную угрозу для здоровья, поскольку сопровождается тяжёлой интоксикацией организма и нарушением психоэмоционального состояния. Решение о лечении в стационаре клиники «Союз Здоровья» позволяет справиться с последствиями длительного употребления алкоголя, обеспечивая пациентам полный комплекс медицинской и психологической помощи.
Подробнее тут – [url=https://vyvod-iz-zapoya-11.ru/]vyvod iz zapoya s vyezdom v-voronezhe[/url]
Комплексный подход к лечению включает в себя не только медицинское вмешательство, но и психологическую поддержку. Зависимость – это хроническое состояние, требующее долгосрочной терапии и контроля. В работе используются современные методики, основанные на научных исследованиях, что позволяет оказывать квалифицированную помощь на всех этапах выздоровления. Пациентам предлагаются индивидуальные решения, учитывающие особенности их состояния и обстоятельств.
Разобраться лучше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya vyzov na dom v-sankt-peterburge[/url]
Процедура вывода из запоя включает очищение организма от токсинов, восстановление работы жизненно важных органов и стабилизацию нервной системы. В зависимости от тяжести состояния пациента лечение может проводиться как в клинике, так и на дому. Для многих людей крайне важно сохранить конфиденциальность, поэтому анонимность таких услуг играет ключевую роль. Современные медицинские центры, предоставляющие помощь при запоях, обеспечивают высокий уровень приватности и профессионального подхода.
Подробнее тут – [url=https://vyvod-iz-zapoya-17.ru/]вывод из запоя капельница челябинск[/url]
Вывод из запоя представляет собой важный этап в процессе лечения алкогольной зависимости. Это медицинская процедура, необходимая для устранения токсических веществ, накопленных в организме в результате длительного употребления алкоголя. Алкогольная зависимость может вызывать множество проблем, включая физические и психоэмоциональные расстройства. Своевременное вмешательство специалистов помогает минимизировать риски и способствует восстановлению здоровья пациента.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-13.ru/]vyvod iz zapoya s vyezdom ryazan[/url]
Современный мир сталкивается с множеством сложных проблем, среди которых зависимость от алкоголя занимает особое место. Это разрушительное состояние негативно сказывается на здоровье, разрушает семьи и затрудняет адаптацию в обществе, требуя грамотного и своевременного вмешательства специалистов.
Детальнее – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]нарколог на дом вывод из запоя рязань[/url]
Запой представляет собой сложное состояние, связанное с длительным употреблением алкоголя, что приводит к формированию зависимости. Это явление не только нарушает нормальную жизнедеятельность, но также вызывает серьезные физические и психологические расстройства. Стационарное лечение становится необходимым для восстановления здоровья и профилактики осложнений. В стационаре клиники «Шаг к Трезвости» в Краснодаре мы предлагаем комплексный подход, включающий медикаментозное лечение, психотерапию и постоянный контроль со стороны квалифицированных специалистов.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]vyvod iz zapoya kruglosutochno narkologiya [/url]
Запой – это состояние, которое возникает вследствие длительного употребления алкоголя, приводящее к потере контроля над количеством выпиваемого и формированию как физической, так и психологической зависимости. Оно сопровождается серьёзными последствиями для здоровья и требует немедленного вмешательства.
Выяснить больше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]наркология вывод из запоя на дому [/url]
Комплексный подход к лечению включает в себя не только медицинское вмешательство, но и психологическую поддержку. Зависимость – это хроническое состояние, требующее долгосрочной терапии и контроля. В работе используются современные методики, основанные на научных исследованиях, что позволяет оказывать квалифицированную помощь на всех этапах выздоровления. Пациентам предлагаются индивидуальные решения, учитывающие особенности их состояния и обстоятельств.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]vyvod iz zapoya cena narkologiya v-sankt-peterburge[/url]
Комплексный подход к лечению включает в себя не только медицинское вмешательство, но и психологическую поддержку. Зависимость – это хроническое состояние, требующее долгосрочной терапии и контроля. В работе используются современные методики, основанные на научных исследованиях, что позволяет оказывать квалифицированную помощь на всех этапах выздоровления. Пациентам предлагаются индивидуальные решения, учитывающие особенности их состояния и обстоятельств.
Разобраться лучше – [url=https://vyvod-iz-zapoya-14.ru/]вывод из запоя в санкт-петербурге[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Углубиться в тему – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]вывод из запоя на дому цена воронеж[/url]
Для эффективного выхода из запоя требуется профессиональный подход, включающий детоксикацию, поддержку внутренних органов и восстановление психоэмоционального состояния. Самостоятельные попытки прекратить употребление алкоголя могут привести к осложнениям, таким как сильный абстинентный синдром или алкогольный делирий. Поэтому важно обратиться за квалифицированной помощью, которая может быть оказана как в стационарных условиях, так и на дому.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]vyvod iz zapoya na domu nedorogo [/url]
В Рязани помощь людям, страдающим от алкогольной зависимости, оказывает наркологическая клиника «Освобождение». Здесь понимают, что борьба с этим заболеванием требует не только медицинского вмешательства, но и комплексной поддержки, учитывающей как состояние самого пациента, так и влияние зависимости на его окружение.
Разобраться лучше – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-anonimno-v-ryazani/]anonimny vyvod iz zapoya na domu [/url]
Процесс вывода из запоя требует быстрого реагирования. Запой, особенно в его тяжелых формах, представляет угрозу не только для психического состояния пациента, но и для его физического здоровья. Чтобы минимизировать риски и предотвратить осложнения, важно начинать лечение как можно скорее. Клинике «Освобождение» в Рязани доступна круглосуточная помощь — мы работаем 24/7, чтобы оперативно вмешаться в любой момент, когда это необходимо. Врачебная помощь может быть предоставлена как в стационаре клиники, так и на дому, в зависимости от состояния пациента и его предпочтений.
Получить больше информации – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]narkolog na dom vyvod iz zapoya v-ryazani[/url]
Алкогольная зависимость – одно из самых разрушительных заболеваний, наносящее серьёзный ущерб организму. Запойные состояния представляют особую опасность, так как ведут к сильной интоксикации, нарушениям психики и необратимым последствиям. Чем раньше будет оказана медицинская помощь, тем выше шанс избежать тяжёлых осложнений и быстрее вернуться к нормальной жизни.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-14.ru/]narkologicheskij centr v-sankt-peterburge[/url]
Для тех, кто по разным причинам не может поехать в стационар, предлагается услуга вывода из запоя на дому. Опытные специалисты проводят детоксикацию в комфортных условиях, применяя современные препараты, снижающие нагрузку на организм и уменьшающие неприятные симптомы. Однако при наличии осложнений или тяжёлого состояния может потребоваться круглосуточный медицинский контроль в стационаре, где пациенту будет обеспечена всесторонняя поддержка.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя на дому недорого капельница санкт-петербург[/url]
Алкогольная зависимость — это хроническое заболевание, которое сопровождается неконтролируемым стремлением к употреблению спиртного. Регулярные запои приводят не только к разрушению организма, но и к ухудшению психоэмоционального состояния, социальным проблемам и конфликтам. Чтобы избавиться от этой зависимости, необходимо начать с детоксикации и постепенной реабилитации, адаптированной под конкретного пациента. Важным аспектом в этом процессе является сохранение анонимности, позволяющее человеку избежать осуждения и сосредоточиться на выздоровлении.
Детальнее – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-cena-v-ryazani/]narkolog vyvod iz zapoya cena v-ryazani[/url]
Процедура вывода из запоя включает очищение организма от токсинов, восстановление работы жизненно важных органов и стабилизацию нервной системы. В зависимости от тяжести состояния пациента лечение может проводиться как в клинике, так и на дому. Для многих людей крайне важно сохранить конфиденциальность, поэтому анонимность таких услуг играет ключевую роль. Современные медицинские центры, предоставляющие помощь при запоях, обеспечивают высокий уровень приватности и профессионального подхода.
Подробнее тут – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-cena-v-chelyabinske/]vyvod iz zapoya s vyezdom cena [/url]
Вывод из запоя представляет собой важный этап в процессе лечения алкогольной зависимости. Это медицинская процедура, необходимая для устранения токсических веществ, накопленных в организме в результате длительного употребления алкоголя. Алкогольная зависимость может вызывать множество проблем, включая физические и психоэмоциональные расстройства. Своевременное вмешательство специалистов помогает минимизировать риски и способствует восстановлению здоровья пациента.
Подробнее – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-v-kruglosutochno-v-ryazani/]вывод из запоя срочно круглосуточно в рязани[/url]
Клиника «Шаг к Трезвости» в Краснодаре предлагает анонимный вывод из запоя, обеспечивая полную конфиденциальность и безопасность на каждом этапе лечения. Мы понимаем, что для многих людей важно сохранить свою личную информацию в тайне, и гарантируем, что все данные о пациенте и его состоянии здоровья останутся закрытыми. Независимо от того, выбрал ли пациент стационарное лечение или вывод из запоя на дому, мы обеспечиваем высококачественную медицинскую помощь с полным соблюдением принципов конфиденциальности.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]vyvod iz zapoya vrach na dom v-krasnodare[/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Углубиться в тему – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-anonimno-v-voronezhe/]narkologiya vyvod iz zapoya anonimno v-voronezhe[/url]
Для тех, кто не может или не хочет посещать клинику, доступна услуга вывода из запоя на дому. Этот вариант удобен и позволяет пациенту получить необходимую помощь в привычной обстановке. Однако в сложных случаях, особенно при наличии осложнений, рекомендуется стационарное лечение, обеспечивающее круглосуточный контроль специалистов и комплексный подход к восстановлению здоровья.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя на дому круглосуточно [/url]
Алкогольная зависимость — это хроническое заболевание, которое сопровождается неконтролируемым стремлением к употреблению спиртного. Регулярные запои приводят не только к разрушению организма, но и к ухудшению психоэмоционального состояния, социальным проблемам и конфликтам. Чтобы избавиться от этой зависимости, необходимо начать с детоксикации и постепенной реабилитации, адаптированной под конкретного пациента. Важным аспектом в этом процессе является сохранение анонимности, позволяющее человеку избежать осуждения и сосредоточиться на выздоровлении.
Подробнее тут – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]срочный вывод из запоя на дому [/url]
Алкогольные запои представляют собой сложное состояние, при котором человек на протяжении нескольких дней или даже недель бесконтрольно употребляет спиртное. Это не просто вредная привычка, а серьезная зависимость, ведущая к разрушению организма и психики. Родные и близкие зачастую оказываются в растерянности, не зная, как помочь. В такие моменты важно как можно скорее обратиться за квалифицированной медицинской помощью, так как своевременное вмешательство может предотвратить тяжелые последствия.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-cena-v-chelyabinske/]vyvod iz zapoya na domu cena [/url]
Зависимость – серьёзная проблема, с которой сталкиваются люди по всему миру. Повседневные трудности, постоянный стресс и финансовые неурядицы нередко становятся катализаторами таких расстройств, как алкоголизм, наркомания и игромания. Медицинский центр «Второй Шанс» специализируется на помощи людям, стремящимся избавиться от этих заболеваний, восстановить здоровье, адаптироваться к жизни без вредных привычек и избежать рецидивов.
Углубиться в тему – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu kruglosutochno v-sankt-peterburge[/url]
Клиника «Освобождение» в Рязани предоставляет услугу круглосуточного вывода из запоя на дому, что позволяет пациенту получить медицинскую помощь в привычной обстановке, избегая лишнего стресса, связанного с госпитализацией.
Углубиться в тему – [url=https://vyvod-iz-zapoya-13.ru/]вывод из запоя в рязани[/url]
Алкогольный запой представляет собой критическое состояние, требующее немедленного вмешательства квалифицированных специалистов. Клиника «Союз Здоровья» в Воронеже предлагает круглосуточную помощь, чтобы обеспечить своевременное и эффективное лечение. Наша цель — оперативное снятие интоксикации, устранение симптомов и предотвращение осложнений.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]вывод из запоя вызов на дом в воронеже[/url]
Комплексный подход к лечению включает в себя не только медицинское вмешательство, но и психологическую поддержку. Зависимость – это хроническое состояние, требующее долгосрочной терапии и контроля. В работе используются современные методики, основанные на научных исследованиях, что позволяет оказывать квалифицированную помощь на всех этапах выздоровления. Пациентам предлагаются индивидуальные решения, учитывающие особенности их состояния и обстоятельств.
Детальнее – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]вывод из запоя срочно круглосуточно санкт-петербург[/url]
Для эффективного выхода из запоя требуется профессиональный подход, включающий детоксикацию, поддержку внутренних органов и восстановление психоэмоционального состояния. Самостоятельные попытки прекратить употребление алкоголя могут привести к осложнениям, таким как сильный абстинентный синдром или алкогольный делирий. Поэтому важно обратиться за квалифицированной помощью, которая может быть оказана как в стационарных условиях, так и на дому.
Углубиться в тему – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя вызов на дом в краснодаре[/url]
Запой представляет серьёзную угрозу, поэтому откладывать обращение за медицинской помощью нельзя. Чем раньше будет проведена детоксикация и поддерживающая терапия, тем ниже риск осложнений. В клинике «Освобождение» работают круглосуточно, оперативно реагируя на экстренные случаи и предлагая как стационарное лечение, так и выездные услуги — в зависимости от состояния пациента и его пожеланий.
Подробнее тут – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-cena-v-ryazani/]vyvod iz zapoya cena narkologiya ryazan[/url]
Стоимость процедур формируется в зависимости от состояния пациента, выбранного способа лечения и дополнительных медицинских услуг. Прозрачность ценообразования позволяет заранее оценить предстоящие расходы и выбрать оптимальный вариант. Комплексный подход, профессионализм врачей и индивидуальные схемы терапии помогают не только выйти из запойного состояния, но и сделать уверенный шаг к здоровой и полноценной жизни.
Выяснить больше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-cena-v-chelyabinske/]вывод из запоя с выездом цена [/url]
Запой представляет серьёзную угрозу, поэтому откладывать обращение за медицинской помощью нельзя. Чем раньше будет проведена детоксикация и поддерживающая терапия, тем ниже риск осложнений. В клинике «Освобождение» работают круглосуточно, оперативно реагируя на экстренные случаи и предлагая как стационарное лечение, так и выездные услуги — в зависимости от состояния пациента и его пожеланий.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-13.ru/]vyvod-iz-zapoya-13.ru[/url]
I recommend to you to come for a site on which there is a lot of information on this question.
Комплексный подход к лечению включает в себя не только медицинское вмешательство, но и психологическую поддержку. Зависимость – это хроническое состояние, требующее долгосрочной терапии и контроля. В работе используются современные методики, основанные на научных исследованиях, что позволяет оказывать квалифицированную помощь на всех этапах выздоровления. Пациентам предлагаются индивидуальные решения, учитывающие особенности их состояния и обстоятельств.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]быстрый вывод из запоя в стационаре в санкт-петербурге[/url]
Алкогольный запой представляет собой серьезное состояние, которое требует немедленного медицинского вмешательства. Это состояние характеризуется длительным и бесконтрольным потреблением алкоголя, что приводит к разрушению как физического, так и психоэмоционального здоровья человека. Для эффективного и безопасного вывода из запоя стационарное лечение является оптимальным решением, особенно при тяжелых случаях интоксикации. В стационаре клиники «Заря Будущего» мы предлагаем комплексную программу вывода из запоя, которая включает детоксикацию, восстановление организма и психотерапевтическую помощь.
Получить больше информации – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]вывод из запоя в стационаре [/url]
Запой представляет собой состояние, связанное с длительным употреблением алкогольных напитков, что приводит к значительным физическим и психическим расстройствам. Это явление формирует зависимость, оказывая негативное воздействие на здоровье. Вывод из запоя в стационаре становится необходимостью для восстановительных мероприятий, направленных на реабилитацию пациента. В этой статье рассматриваются аспекты анонимного вывода, скорость процедур, а также роль нарколога в процессе лечения.
Получить больше информации – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя на дому в краснодаре[/url]
Сочные, первоклассные и качественные суши теперь ещё ближе! Наша сеть доставки в Иркутске и Красноярске предлагает широкий выбор суши-роллов, суши и запечённых сетов. Попробуйте популярные “Филадельфию”, “Калифорнию”, “Дракона” и известные вкусы. Мы используем только натуральные ингредиенты от проверенных поставщиков. Постоянные выгодные скидки, приятные сюрпризы делают заказ ещё интереснее. А моментальная [url=https://sushi-holl.ru/catalog/rolly]суши роллы доставка[/url] всегда бесплатная в удобное для вас время от “Sushi-Holl”!
Если хотите порадовать себя или устроить вкусный ужин, у нас можно [url=https://sushi-holl.ru/]роллы в иркутске[/url] с доставкой на дом. Кроме традиционных суши и роллов, в меню есть разнообразные виды WOK, оригинальные салаты, тёплые блюда и даже раздел для детей. Оформляйте заказ на сайте – мы привезём всё свежеприготовленным, вкусным и в удобное место!
Запой представляет собой состояние, характеризующееся длительным непрерывным употреблением алкоголя, что приводит к физической и психической зависимости. Этот процесс может длиться от нескольких дней до недель. Алкогольные напитки негативно влияют на функционирование внутренних органов, вызывая серьёзные нарушения, включая алкоголизм. При необходимости вывода из запоя важно обратиться за медицинской помощью, что можно осуществить на дому. В данной статье рассмотрим ключевые аспекты данной процедуры.
Выяснить больше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]наркология вывод из запоя на дому челябинск[/url]
Клиника “Второй Шанс” в Санкт-Петербурге предлагает услугу вывода из запоя, как в стационаре, так и на дому. Для пациентов, не желающих посещать клинику, квалифицированные врачи готовы предоставить помощь в комфортных домашних условиях. Используя современные методы, специалисты обеспечивают безопасность, анонимность и высокий уровень медицинской поддержки.
Получить больше информации – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya s vyezdom na dom sankt-peterburg[/url]
Наркологическая клиника «Союз Здоровья» в Воронеже специализируется на лечении алкогольной зависимости и предлагает профессиональные услуги по выводу из запоя. Мы стремимся создать атмосферу доверия, где каждый пациент сможет получить необходимую поддержку.
Получить больше информации – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-stacionare-v-voronezhe/]вывод из запоя в стационаре в воронеже[/url]
Алкогольная интоксикация, или запой, представляет собой крайне опасное состояние, при котором организм страдает от токсического воздействия алкоголя. Это ведет к нарушению нормальных физиологических процессов и ухудшению общего состояния здоровья. Капельница от запоя — это медицинская процедура, направленная на выведение токсинов из организма и восстановление нормальной функции организма при соблюдении полной конфиденциальности.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-cena-v-tveri/]нарколог вывод из запоя цена тверь[/url]
Запой — это опасное состояние, которое развивается у людей с алкогольной зависимостью и характеризуется длительным непрерывным потреблением алкоголя. Это состояние приводит к серьезным нарушениям в организме, как физическим, так и психоэмоциональным. Длительный запой может вызвать множество осложнений, таких как поражение внутренних органов, нервной системы, а также проблемы в социальной и семейной жизни. Однако современная медицина предоставляет эффективные методы вывода из запоя, и одним из наиболее удобных и востребованных является вывод из запоя на дому.
Разобраться лучше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]vyvod iz zapoya anonimno nedorogo v-sankt-peterburge[/url]
Запой – это тяжелое состояние, возникающее из-за длительного и неконтролируемого употребления алкоголя. Оно характеризуется физической и психологической зависимостью, что приводит к серьезным последствиям для организма и психики. Для восстановления нормального самочувствия и здоровья необходимо профессиональное лечение. Одним из наиболее удобных и эффективных методов является вывод из запоя на дому.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-13.ru/]вывод из запоя[/url]
Вывод из запоя представляет собой важный этап в процессе лечения алкогольной зависимости. Это медицинская процедура, необходимая для устранения токсических веществ, накопленных в организме в результате длительного употребления алкоголя. Алкогольная зависимость может вызывать множество проблем, включая физические и психоэмоциональные расстройства. Своевременное вмешательство специалистов помогает минимизировать риски и способствует восстановлению здоровья пациента.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-v-kruglosutochno-v-ryazani/]вывод из запоя срочно круглосуточно [/url]
Современное общество сталкивается с серьёзными вызовами, связанными с зависимостями. Алкоголизм, как одна из самых распространённых проблем, оказывает разрушительное воздействие на здоровье человека, разрушая семейные отношения и затрудняя социальную адаптацию. В такие моменты крайне важно обратиться за квалифицированной помощью.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]вывод из запоя на дому недорого [/url]
Одной из распространенных проблем является запой, состояние, при котором человек длительное время бесконтрольно употребляет алкоголь, что приводит к тяжелой физической и психологической зависимости. Такое поведение чревато серьезными последствиями для здоровья, включая поражение внутренних органов, нервные расстройства и другие заболевания. Важно своевременно обратиться за профессиональной помощью, чтобы минимизировать риски и предотвратить осложнения.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu kruglosutochno sankt-peterburg[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]вывод из запоя с выездом цена [/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Подробнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя с выездом на дом санкт-петербург[/url]
Зависимость — это серьёзная проблема, которая затрагивает не только человека, но и его семью и окружение. Алкоголизм, наркомания и другие виды зависимостей могут разрушить жизнь, но с профессиональной помощью можно вернуть её в русло нормального функционирования. Наркологическая клиника «Заря Будущего» в Санкт-Петербурге предлагает уникальные программы лечения зависимостей, которые сочетает медико-психологический подход, индивидуальные методики и квалифицированную поддержку.
Получить больше информации – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu nedorogo kapelnica v-sankt-peterburge[/url]
Запой представляет собой сложное состояние, связанное с длительным употреблением алкоголя, что приводит к формированию зависимости. Это явление не только нарушает нормальную жизнедеятельность, но также вызывает серьезные физические и психологические расстройства. Стационарное лечение становится необходимым для восстановления здоровья и профилактики осложнений. В стационаре клиники «Шаг к Трезвости» в Краснодаре мы предлагаем комплексный подход, включающий медикаментозное лечение, психотерапию и постоянный контроль со стороны квалифицированных специалистов.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-12.ru/]vyvod iz zapoya v-krasnodare[/url]
Алкогольная зависимость представляет собой серьёзную медицинскую проблему, требующую специализированного подхода. Запойное состояние характеризуется длительным и бесконтрольным потреблением спиртных напитков, что приводит к возникновению как физической, так и психической зависимости. Данное явление может вызвать нарушения в работе внутренних органов, значительно увеличивая вероятность развития алкогольного делирия, который представляет собой острое состояние, требующее неотложной медицинской помощи. Вывод из запоя это ключевая часть лечения зависимости, которая может осуществляться как в стационаре, так и в амбулаторных условиях, в зависимости от конкретной ситуации и состояния пациента.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-v-stacionare-v-krasnodare/]vyvod iz zapoya v stacionare anonimno [/url]
Вывод из запоя представляет собой важный этап в процессе лечения алкогольной зависимости. Это медицинская процедура, необходимая для устранения токсических веществ, накопленных в организме в результате длительного употребления алкоголя. Алкогольная зависимость может вызывать множество проблем, включая физические и психоэмоциональные расстройства. Своевременное вмешательство специалистов помогает минимизировать риски и способствует восстановлению здоровья пациента.
Детальнее – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-v-kruglosutochno-v-ryazani/]вывод из запоя круглосуточно рязань[/url]
Запой — это тяжелое состояние, при котором человек теряет контроль над количеством употребляемого алкоголя, что приводит к серьезным нарушениям здоровья. Процесс вывода из запоя требует профессионального подхода, медицинского контроля и тщательного восстановления организма. Наркологическая клиника «Шаг к Трезвости» в Краснодаре предлагает квалифицированную помощь для тех, кто нуждается в срочном и безопасном выходе из запоя. Мы предоставляем комплексное лечение, ориентированное на каждый этап восстановления, с максимальной заботой о здоровье пациента.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]срочный вывод из запоя на дому в краснодаре[/url]
Выход из запоя — это важнейший этап на пути к избавлению от алкогольной зависимости. Он требует комплексного подхода, профессиональной помощи и сильной мотивации пациента. В условиях, когда анонимность и комфорт становятся ключевыми факторами для многих людей, вывод из запоя на дому приобретает все большее значение. Это решение позволяет человеку получить необходимую помощь, не покидая дома, и избежать стресса, связанного с госпитализацией.
Получить больше информации – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя на дому недорого [/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьезное воздействие этанола, что приводит к нарушению нормальной физиологической деятельности. Это состояние сопровождается сильными физическими и психологическими расстройствами, которые требуют профессионального вмешательства. Однако многие люди, страдающие алкогольной зависимостью, сталкиваются с трудностью признания своей проблемы, опасаясь утраты репутации и конфиденциальности. В клинике «Заря Будущего» мы предлагаем анонимный вывод из запоя в Санкт-Петербурге, предоставляя высококачественное лечение с гарантией сохранения личной информации.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-19.ru/]narkologicheskaya klinika[/url]
Запой нарушает привычный ритм жизни, вызывая серьезные физические и эмоциональные проблемы. Для его лечения требуется не только медикаментозное вмешательство, но и постоянное наблюдение специалистов. Стационарное лечение в клинике позволяет обеспечить полный контроль над состоянием пациента, что минимизирует риски и способствует более эффективной реабилитации.
Подробнее – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu nedorogo kapelnica [/url]
Запой сопровождается сильной интоксикацией организма, что негативно сказывается на внутренних органах и нервной системе, повышая вероятность осложнений, таких как алкогольный делирий. Это требует немедленного медицинского вмешательства, так как своевременная помощь способна спасти жизнь пациента.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-20.ru/]narkologicheskaya klinika[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-cena-v-chelyabinske/]вывод из запоя на дому цена в челябинске[/url]
Для достижения эффективных результатов в лечении запоя необходима квалифицированная помощь специалистов. Наркологическая клиника «Излечение» в Краснодаре предоставляет профессиональные услуги по выводу из запоя, гарантируя безопасность, комфорт и индивидуальный подход к каждому пациенту.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-16.ru/]вывод из запоя[/url]
Для достижения эффективных результатов в лечении запоя необходима квалифицированная помощь специалистов. Наркологическая клиника «Излечение» в Краснодаре предоставляет профессиональные услуги по выводу из запоя, гарантируя безопасность, комфорт и индивидуальный подход к каждому пациенту.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя на дому недорого капельница [/url]
Современное общество сталкивается с серьёзными вызовами, связанными с зависимостями. Алкоголизм, как одна из самых распространённых проблем, оказывает разрушительное воздействие на здоровье человека, разрушая семейные отношения и затрудняя социальную адаптацию. В такие моменты крайне важно обратиться за квалифицированной помощью.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-13.ru/]вывод из запоя в рязани[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]вывод из запоя с выездом на дом воронеж[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Узнать больше – [url=https://vyvod-iz-zapoya-17.ru/]наркологический центр в челябинске[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Разобраться лучше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya na domu kruglosutochno v-chelyabinske[/url]
Процесс вывода из запоя включает несколько этапов, направленных на детоксикацию и восстановление нормальной работы организма. Для этого необходимы высококвалифицированные специалисты, которые помогают избавиться от последствий токсического воздействия алкоголя и восстанавливают здоровье пациента. Стоимость лечения зависит от необходимого объема услуг и условий их предоставления. В клинике «Излечение» предлагаются доступные цены, с учетом индивидуальных потребностей каждого пациента.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя на дому круглосуточно [/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьёзное воздействие этанола. Это приводит к нарушениям нормальной физиологической деятельности и ухудшению состояния здоровья. Анонимный вывод из запоя — это медицинская помощь, направленная на устранение последствий чрезмерного употребления алкоголя с соблюдением строгой конфиденциальности.
Получить больше информации – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-anonimno-v-ekaterinburge/]narkologiya vyvod iz zapoya anonimno [/url]
Запойное пьянство характеризуется продолжительным и интенсивным потреблением алкоголя, что приводит к серьезным физическим и психологическим последствиям. Часто пациенты сталкиваются с различными заболеваниями, такими как цирроз печени, панкреатит, алкогольный делирий и другие. Алкогольное опьянение не только ухудшает качество жизни, но также может угрожать жизни пациента. В условиях стационара лечение может быть более эффективным, однако многие люди предпочитают проходить данную процедуру на дому, чтобы избежать стигматизации и сохранить чувство приватности.
Углубиться в тему – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-v-stacionare-v-ekaterinburge/]vyvod iz zapoya v stacionare ekaterinburg[/url]
Алкоголизм — это хроническое заболевание, которое характеризуется патологической зависимостью от этанола. Периоды запоя, когда человек неконтролируемо потребляет алкоголь, могут привести к разнообразным медицинским осложнениям, как физическим, так и психологическим. Запой становится не только причиной ухудшения здоровья, но и причиной множества социальных и личных проблем. На этом фоне возникает необходимость вывода из запоя, процесс, который должен учитывать индивидуальные особенности пациента. Важно, что анонимность в реабилитации играет ключевую роль, помогая избежать стигматизации и увеличивая шансы на успешное восстановление.
Получить больше информации – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]vyvod iz zapoya na domu ryazan[/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Выяснить больше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu sankt-peterburg[/url]
Алкогольная зависимость становится всё более распространенной проблемой, требующей внимания как со стороны медицинского сообщества, так и общества в целом. Вывод из запоя — это сложный и ответственный процесс, необходимый для восстановления здоровья пациента и его интеграции в нормальную жизнь. Профессиональное вмешательство позволяет минимизировать последствия длительного употребления алкоголя и нормализовать состояние организма.
Детальнее – [url=https://vyvod-iz-zapoya-20.ru/]vyvod iz zapoya kapelnica[/url]
Специалисты клиники «Рассвет» в Твери оказывают комплексную поддержку людям, страдающим от запоев. Пациенты получают круглосуточное медицинское наблюдение, эффективную детоксикацию и индивидуально подобранные программы восстановления. Гарантируется высокий уровень обслуживания, анонимность и полное сопровождение на пути к выздоровлению.
Углубиться в тему – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-v-kruglosutochno-v-tveri/]vyvod iz zapoya kruglosutochno narkologiya v-tveri[/url]
Алкогольная зависимость представляет собой серьезную медицинскую проблему, которая влечет за собой не только физические, но и психологические проблемы. Периоды запоя, характеризующиеся бесконтрольным употреблением алкоголя, могут привести к тяжелым последствиям. Симптомы абстиненции требуют незамедлительного вмешательства. Анонимность в процессе реабилитации становится важным аспектом, помогающим пациентам избежать стыда и социального осуждения.
Получить больше информации – [url=https://vyvod-iz-zapoya-12.ru/]вывод из запоя капельница[/url]
Алкогольная зависимость представляет собой сложное и хроническое заболевание, которое влияет не только на физическое, но и на психологическое состояние пациента. Периоды запоя, влекущие за собой неконтролируемое употребление алкоголя, могут привести к опасным последствиям для здоровья и жизни. Абстинентные симптомы, такие как тремор, тошнота, головная боль и другие проявления, требуют немедленного медицинского вмешательства. В таких ситуациях важно не только быстро устранить физическое воздействие алкоголя, но и предоставить пациенту психологическую поддержку.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]narkolog na dom vyvod iz zapoya [/url]
Запой – это состояние, возникающее из-за длительного употребления алкоголя и характеризующееся физической и психологической зависимостью. Оно требует комплексного медицинского вмешательства для устранения последствий и предотвращения дальнейшего ухудшения здоровья. Стационарное лечение в клинике «Освобождение» в Рязани – это эффективный способ вернуть пациента к нормальной жизни, предоставляя ему комфортные условия и круглосуточную помощь специалистов.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]srochny vyvod iz zapoya na domu ryazan[/url]
Одной из распространенных проблем является запой, состояние, при котором человек длительное время бесконтрольно употребляет алкоголь, что приводит к тяжелой физической и психологической зависимости. Такое поведение чревато серьезными последствиями для здоровья, включая поражение внутренних органов, нервные расстройства и другие заболевания. Важно своевременно обратиться за профессиональной помощью, чтобы минимизировать риски и предотвратить осложнения.
Подробнее – [url=https://vyvod-iz-zapoya-14.ru/]narkologicheskaya klinika sankt-peterburg[/url]
Алкогольная зависимость представляет собой сложное и хроническое заболевание, которое влияет не только на физическое, но и на психологическое состояние пациента. Периоды запоя, влекущие за собой неконтролируемое употребление алкоголя, могут привести к опасным последствиям для здоровья и жизни. Абстинентные симптомы, такие как тремор, тошнота, головная боль и другие проявления, требуют немедленного медицинского вмешательства. В таких ситуациях важно не только быстро устранить физическое воздействие алкоголя, но и предоставить пациенту психологическую поддержку.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]vyvod iz zapoya s vyezdom na dom v-krasnodare[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьезное воздействие этанола, что приводит к нарушению нормальной физиологической деятельности. Это состояние сопровождается сильными физическими и психологическими расстройствами, которые требуют профессионального вмешательства. Однако многие люди, страдающие алкогольной зависимостью, сталкиваются с трудностью признания своей проблемы, опасаясь утраты репутации и конфиденциальности. В клинике «Заря Будущего» мы предлагаем анонимный вывод из запоя в Санкт-Петербурге, предоставляя высококачественное лечение с гарантией сохранения личной информации.
Узнать больше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]srochny vyvod iz zapoya na domu v-sankt-peterburge[/url]
Гарантированная анонимность при выводе из запоя в Челябинске. В клинике «Перекресток Надежды» мы строго соблюдаем политику конфиденциальности. Информация о пациенте и его состоянии здоровья остаётся закрытой. Это особенно важно для тех, кто ценит свою репутацию и не хочет разглашения. Мы обеспечиваем анонимность как в стационаре, так и при оказании услуг на дому, сохраняя высокий уровень медицинской помощи.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя на дому недорого капельница челябинск[/url]
Запойное состояние представляет собой серьёзную угрозу для здоровья, поскольку сопровождается тяжёлой интоксикацией организма и нарушением психоэмоционального состояния. Решение о лечении в стационаре клиники «Союз Здоровья» позволяет справиться с последствиями длительного употребления алкоголя, обеспечивая пациентам полный комплекс медицинской и психологической помощи.
Получить больше информации – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]vyvod iz zapoya cena narkologiya [/url]
Запой — это серьезное состояние, при котором человек не может прекратить употребление алкоголя на протяжении длительного времени, что вызывает физическую и психологическую зависимость. Это крайне опасное состояние, требующее немедленного вмешательства специалистов. Для безопасного и эффективного вывода из запоя необходима квалифицированная медицинская помощь, которую предоставляет наркологическая клиника «Рассвет» в Твери. Наши опытные специалисты используют современные методы детоксикации и восстановления организма, обеспечивая полный медицинский контроль и поддержку на всех этапах лечения.
Выяснить больше – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]vyvod iz zapoya s vyezdom na dom v-tveri[/url]
Для лечения запоев требуется помощь квалифицированных специалистов. В наркологической клинике «Излечение» в Краснодаре обеспечивают безопасный и комфортный выход из запоя, учитывая особенности состояния каждого пациента. Запой — одно из самых тяжелых проявлений алкогольной зависимости, сопровождающееся сильной интоксикацией, нарушением работы внутренних органов и психическими расстройствами. В этот период могут обостряться хронические заболевания, что усиливает необходимость срочной медицинской помощи.
Детальнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-cena-v-krasnodare/]вывод из запоя цена наркология [/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Разобраться лучше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-anonimno-v-chelyabinske/]narkologiya vyvod iz zapoya anonimno chelyabinsk[/url]
Запой — это состояние, характеризующееся утратой контроля над употреблением алкоголя, что приводит к тяжелой интоксикации организма. Вывод из запоя — это комплексный процесс, направленный на устранение последствий интоксикации и восстановление нормального функционирования организма. Цена данной процедуры может значительно различаться в зависимости от нескольких факторов, и в этой статье мы подробно рассмотрим, что влияет на стоимость вывода из запоя в Краснодаре, в том числе в клинике «Шаг к Трезвости».
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-v-stacionare-v-krasnodare/]vyvod iz zapoya v stacionare anonimno [/url]
В клинике «Рассвет» в Твери мы предлагаем круглосуточную помощь при алкогольных запоях, обеспечивая быстрый вывод токсинов из организма с помощью капельниц и других эффективных методик.
Разобраться лучше – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-cena-v-tveri/]vyvod iz zapoya cena tver[/url]
Клиника «Рассвет» в Твери предоставляет услугу анонимного вывода из запоя, обеспечивая высокое качество медицинского обслуживания и строгое соблюдение всех этических норм.
Подробнее – [url=https://vyvod-iz-zapoya-21.ru/]vyvod iz zapoya s vyezdom[/url]
Алкогольная зависимость представляет собой серьёзную медицинскую проблему, требующую специализированного подхода. Запойное состояние характеризуется длительным и бесконтрольным потреблением спиртных напитков, что приводит к возникновению как физической, так и психической зависимости. Данное явление может вызвать нарушения в работе внутренних органов, значительно увеличивая вероятность развития алкогольного делирия, который представляет собой острое состояние, требующее неотложной медицинской помощи. Вывод из запоя это ключевая часть лечения зависимости, которая может осуществляться как в стационаре, так и в амбулаторных условиях, в зависимости от конкретной ситуации и состояния пациента.
Подробнее тут – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]вывод из запоя круглосуточно наркология краснодар[/url]
В клинике работает команда квалифицированных специалистов, которые осуществляют вывод из запоя, обеспечивая полный контроль над состоянием пациента и устраняя все последствия длительного употребления алкоголя.
Получить больше информации – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]вывод из запоя на дому [/url]
В клинике «Заря Будущего» мы понимаем, насколько важен своевременный и профессиональный подход к лечению зависимости. Наши специалисты готовы оказать квалифицированную помощь прямо у вас дома, обеспечивая полную безопасность и конфиденциальность на каждом этапе лечения.
Подробнее тут – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]vyvod iz zapoya v stacionare anonimno v-sankt-peterburge[/url]
Современное общество сталкивается с серьёзными вызовами, связанными с зависимостями. Алкоголизм, как одна из самых распространённых проблем, оказывает разрушительное воздействие на здоровье человека, разрушая семейные отношения и затрудняя социальную адаптацию. В такие моменты крайне важно обратиться за квалифицированной помощью.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-13.ru/]наркологическая клиника[/url]
Клиника «Освобождение» в Рязани предоставляет услугу круглосуточного вывода из запоя на дому, что позволяет пациенту получить медицинскую помощь в привычной обстановке, избегая лишнего стресса, связанного с госпитализацией.
Выяснить больше – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]vyvod iz zapoya vyzov na dom [/url]
Процесс выхода из запоя имеет большое значение в борьбе с алкоголизмом. Он требует не только профессионального медицинского вмешательства, но и внутренней мотивации пациента. Важным моментом является создание комфортных и анонимных условий лечения, особенно для тех, кто опасается осуждения со стороны окружающих. Одним из удобных решений является выведение из запоя на дому, что позволяет пациенту находиться в привычной обстановке и избегать стресса от госпитализации.
Получить больше информации – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]срочный вывод из запоя на дому в челябинске[/url]
В клинике также уделяется внимание конфиденциальности лечения. Понимая деликатность проблемы, врачи гарантируют полную анонимность на всех этапах терапии. Благодаря профессиональному подходу и использованию проверенных методик пациенты могут восстановить здоровье и вернуться к полноценной жизни.
Детальнее – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]vyvod iz zapoya cena [/url]
Процесс выхода из запоя имеет большое значение в борьбе с алкоголизмом. Он требует не только профессионального медицинского вмешательства, но и внутренней мотивации пациента. Важным моментом является создание комфортных и анонимных условий лечения, особенно для тех, кто опасается осуждения со стороны окружающих. Одним из удобных решений является выведение из запоя на дому, что позволяет пациенту находиться в привычной обстановке и избегать стресса от госпитализации.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-anonimno-v-chelyabinske/]vyvod iz zapoya anonimno nedorogo chelyabinsk[/url]
Длительное запойное состояние крайне опасно, поскольку провоцирует серьезные заболевания, включая цирроз печени, воспаление поджелудочной железы и нарушения работы нервной системы. В тяжелых случаях возможно развитие алкогольного делирия – состояния, угрожающего жизни. Именно поэтому важно своевременно обратиться за медицинской помощью при первых признаках ухудшения самочувствия.
Разобраться лучше – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-v-stacionare-v-tveri/]bystry vyvod iz zapoya v stacionare tver[/url]
Алкогольный запой — это серьезное заболевание, которое требует немедленного и профессионального вмешательства. Запой сопровождается не только физическими страданиями, но и эмоциональными трудностями. Процесс выведения из запоя требует срочного внимания, так как состояние пациента может ухудшиться, если не получить квалифицированную помощь вовремя. Наркологическая клиника «Излечение» в Краснодаре предоставляет круглосуточную помощь при алкогольном запое, чтобы восстановить здоровье пациента в самых сложных ситуациях.
Получить больше информации – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя врач на дом в краснодаре[/url]
Для достижения эффективных результатов в лечении запоя необходима квалифицированная помощь специалистов. Наркологическая клиника «Излечение» в Краснодаре предоставляет профессиональные услуги по выводу из запоя, гарантируя безопасность, комфорт и индивидуальный подход к каждому пациенту.
Получить больше информации – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя на дому недорого капельница в краснодаре[/url]
В клинике «Заря Будущего» мы понимаем, насколько важен своевременный и профессиональный подход к лечению зависимости. Наши специалисты готовы оказать квалифицированную помощь прямо у вас дома, обеспечивая полную безопасность и конфиденциальность на каждом этапе лечения.
Подробнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]вывод из запоя анонимно санкт-петербург[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Детальнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]narkolog na dom vyvod iz zapoya voronezh[/url]
Для лечения запоев требуется помощь квалифицированных специалистов. В наркологической клинике «Излечение» в Краснодаре обеспечивают безопасный и комфортный выход из запоя, учитывая особенности состояния каждого пациента. Запой — одно из самых тяжелых проявлений алкогольной зависимости, сопровождающееся сильной интоксикацией, нарушением работы внутренних органов и психическими расстройствами. В этот период могут обостряться хронические заболевания, что усиливает необходимость срочной медицинской помощи.
Разобраться лучше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя с выездом на дом [/url]
Алкогольный запой – это серьёзное состояние, при котором человек теряет контроль над употреблением алкоголя, что приводит к тяжёлой интоксикации организма. Во время запоя нарушаются функции внутренних органов, страдает нервная система, и повышается риск развития опасных осложнений, таких как алкогольный делирий. Это состояние требует незамедлительного медицинского вмешательства.
Подробнее тут – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-anonimno-v-ekaterinburge/]наркология вывод из запоя анонимно екатеринбург[/url]
Процесс вывода из запоя включает несколько этапов, направленных на детоксикацию и восстановление нормальной работы организма. Для этого необходимы высококвалифицированные специалисты, которые помогают избавиться от последствий токсического воздействия алкоголя и восстанавливают здоровье пациента. Стоимость лечения зависит от необходимого объема услуг и условий их предоставления. В клинике «Излечение» предлагаются доступные цены, с учетом индивидуальных потребностей каждого пациента.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-cena-v-krasnodare/]вывод из запоя цена наркология в краснодаре[/url]
Продолжительное употребление алкоголя приводит к состоянию, при котором человек теряет способность самостоятельно остановиться, оказываясь в плену физической и психологической зависимости. Такое положение дел представляет серьёзную угрозу для здоровья и требует незамедлительного вмешательства профессионалов. В Екатеринбурге наркологическая клиника «Преображение» предлагает специализированную помощь, используя проверенные и безопасные методы лечения.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]срочный вывод из запоя на дому [/url]
Выход из запоя — это важнейший этап на пути к избавлению от алкогольной зависимости. Он требует комплексного подхода, профессиональной помощи и сильной мотивации пациента. В условиях, когда анонимность и комфорт становятся ключевыми факторами для многих людей, вывод из запоя на дому приобретает все большее значение. Это решение позволяет человеку получить необходимую помощь, не покидая дома, и избежать стресса, связанного с госпитализацией.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-stacionare-v-krasnodare/]vyvod iz zapoya v stacionare krasnodar[/url]
Алкогольная зависимость представляет собой сложное и хроническое заболевание, которое влияет не только на физическое, но и на психологическое состояние пациента. Периоды запоя, влекущие за собой неконтролируемое употребление алкоголя, могут привести к опасным последствиям для здоровья и жизни. Абстинентные симптомы, такие как тремор, тошнота, головная боль и другие проявления, требуют немедленного медицинского вмешательства. В таких ситуациях важно не только быстро устранить физическое воздействие алкоголя, но и предоставить пациенту психологическую поддержку.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]срочный вывод из запоя на дому [/url]
Алкогольная зависимость — это серьезная проблема, требующая немедленного вмешательства. Если вы или ваши близкие столкнулись с запоем, важно получить профессиональную помощь как можно скорее, чтобы избежать ухудшения здоровья. Наркологическая клиника «Шаг к Трезвости» в Краснодаре предлагает услугу вывода из запоя на дому, что является удобным и эффективным решением для тех, кто не может или не хочет посещать стационар.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-12.ru/]вывод из запоя[/url]
Клиника «Преображение» в Екатеринбурге предлагает анонимные услуги по выведению из запоя, придерживаясь высоких стандартов медицинской этики. Алкогольная зависимость — это серьёзная проблема, требующая комплексного подхода и внимания со стороны общества. Профессиональное вмешательство позволяет значительно снизить вредное воздействие алкоголя и способствует возвращению человека к нормальной жизни.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-cena-v-ekaterinburge/]vyvod iz zapoya cena narkologiya ekaterinburg[/url]
Чего нельзя делать при синдроме беспокойных ног, типичные обманы.
Неизвестные способы лечения синдрома беспокойных ног, какие из них работают?
синдром беспокойных [url=https://sindrom-vitmaka.ru/]синдром беспокойных[/url] .
Алкогольная зависимость становится всё более распространенной проблемой, требующей внимания как со стороны медицинского сообщества, так и общества в целом. Вывод из запоя — это сложный и ответственный процесс, необходимый для восстановления здоровья пациента и его интеграции в нормальную жизнь. Профессиональное вмешательство позволяет минимизировать последствия длительного употребления алкоголя и нормализовать состояние организма.
Разобраться лучше – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-v-kruglosutochno-v-ekaterinburge/]vyvod iz zapoya srochno kruglosutochno [/url]
Запой — это серьезное состояние, при котором человек не может прекратить употребление алкоголя на протяжении длительного времени, что вызывает физическую и психологическую зависимость. Это крайне опасное состояние, требующее немедленного вмешательства специалистов. Для безопасного и эффективного вывода из запоя необходима квалифицированная медицинская помощь, которую предоставляет наркологическая клиника «Рассвет» в Твери. Наши опытные специалисты используют современные методы детоксикации и восстановления организма, обеспечивая полный медицинский контроль и поддержку на всех этапах лечения.
Детальнее – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]вывод из запоя на дому круглосуточно [/url]
Алкоголизм — это хроническое заболевание, которое характеризуется патологической зависимостью от этанола. Периоды запоя, когда человек неконтролируемо потребляет алкоголь, могут привести к разнообразным медицинским осложнениям, как физическим, так и психологическим. Запой становится не только причиной ухудшения здоровья, но и причиной множества социальных и личных проблем. На этом фоне возникает необходимость вывода из запоя, процесс, который должен учитывать индивидуальные особенности пациента. Важно, что анонимность в реабилитации играет ключевую роль, помогая избежать стигматизации и увеличивая шансы на успешное восстановление.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-cena-v-ryazani/]вывод из запоя на дому цена рязань[/url]
Алкогольная зависимость представляет собой актуальную медицинскую проблему, требующую тщательного подхода. Запой характеризуется длительным, непрерывным употреблением спиртных напитков, что ведёт к физической и психической зависимости. На фоне запойного состояния могут возникнуть тяжёлые нарушения функций органов, увеличивается риск развития таких состояний, как алкогольный делирий. Вывод из запоя становится ключевым этапом в лечении зависимостей, который может осуществляться как в стационарных условиях, так и на дому, в зависимости от состояния пациента. В клинике «Перекресток Надежды» в Челябинске мы предлагаем круглосуточную помощь, чтобы обеспечить максимально эффективное и безопасное лечение при любом варианте вывода из запоя.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-v-kruglosutochno-v-chelyabinske/]вывод из запоя срочно круглосуточно [/url]
Стоимость вывода из запоя зависит от ряда факторов, таких как состояние пациента, выбранная программа лечения и место проведения процедуры. Мы предлагаем прозрачное ценообразование, чтобы вы могли заранее ознакомиться с возможными затратами на лечение.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya na domu nedorogo kapelnica [/url]
Запой — это опасное состояние, которое развивается у людей с алкогольной зависимостью и характеризуется длительным непрерывным потреблением алкоголя. Это состояние приводит к серьезным нарушениям в организме, как физическим, так и психоэмоциональным. Длительный запой может вызвать множество осложнений, таких как поражение внутренних органов, нервной системы, а также проблемы в социальной и семейной жизни. Однако современная медицина предоставляет эффективные методы вывода из запоя, и одним из наиболее удобных и востребованных является вывод из запоя на дому.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя капельница на дому санкт-петербург[/url]
Алкогольная зависимость остаётся серьёзной проблемой, требующей квалифицированного медицинского вмешательства. Капельница от запоя — это ключевой этап в процессе лечения, направленный на стабилизацию состояния пациента и его возвращение к полноценной жизни. Профессиональная помощь позволяет эффективно справиться с последствиями длительного употребления алкоголя и восстановить физическое и психологическое здоровье.
Углубиться в тему – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]срочный вывод из запоя на дому [/url]
I wanted to download Metamask safely, and https://sites.google.com/view/metamask-extension-dfkasdkfdnt/download helped me do just that. Their guide is detailed and straightforward.
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Узнать больше – [url=https://vyvod-iz-zapoya-17.ru/]наркологическая клиника челябинск[/url]
В клинике также уделяется внимание конфиденциальности лечения. Понимая деликатность проблемы, врачи гарантируют полную анонимность на всех этапах терапии. Благодаря профессиональному подходу и использованию проверенных методик пациенты могут восстановить здоровье и вернуться к полноценной жизни.
Детальнее – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]быстрый вывод из запоя в стационаре в санкт-петербурге[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Выяснить больше – [url=https://vyvod-iz-zapoya-17.ru/]vyvod iz zapoya[/url]
Алкогольная зависимость — это хроническое заболевание, которое требует комплексного подхода и квалифицированного лечения. В клинике «Излечение» гарантируют полную анонимность на всех этапах лечения, что особенно важно для тех, кто хочет избежать общественного осуждения. Пациенты могут получить помощь как в стационаре, так и на дому, при этом обеспечивается максимальный комфорт и конфиденциальность.
Разобраться лучше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-anonimno-v-chelyabinske/]наркология вывод из запоя анонимно [/url]
В таких ситуациях вывод из запоя на дому становится оптимальным решением, особенно если пациент не может или не хочет посещать стационар. Эта услуга позволяет получить медицинскую помощь в комфортной и привычной обстановке, что снижает уровень стресса и ускоряет процесс восстановления.
Подробнее тут – [url=https://vyvod-iz-zapoya-11.ru/]vyvod iz zapoya s vyezdom[/url]
В клинике «Заря Будущего» мы понимаем, насколько важен своевременный и профессиональный подход к лечению зависимости. Наши специалисты готовы оказать квалифицированную помощь прямо у вас дома, обеспечивая полную безопасность и конфиденциальность на каждом этапе лечения.
Узнать больше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu v-sankt-peterburge[/url]
Клиника «Преображение» в Екатеринбурге предлагает анонимные услуги по выведению из запоя, придерживаясь высоких стандартов медицинской этики. Алкогольная зависимость — это серьёзная проблема, требующая комплексного подхода и внимания со стороны общества. Профессиональное вмешательство позволяет значительно снизить вредное воздействие алкоголя и способствует возвращению человека к нормальной жизни.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-v-stacionare-v-ekaterinburge/]vyvod iz zapoya v stacionare anonimno [/url]
Процесс вывода из запоя включает несколько этапов, направленных на детоксикацию и восстановление нормальной работы организма. Для этого необходимы высококвалифицированные специалисты, которые помогают избавиться от последствий токсического воздействия алкоголя и восстанавливают здоровье пациента. Стоимость лечения зависит от необходимого объема услуг и условий их предоставления. В клинике «Излечение» предлагаются доступные цены, с учетом индивидуальных потребностей каждого пациента.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]вывод из запоя круглосуточно краснодар[/url]
Алкогольная зависимость – это состояние, при котором человек теряет способность контролировать употребление спиртных напитков, что со временем приводит к глубокой физической и психической привязанности. Одна из наиболее опасных стадий – запой, когда алкоголь потребляется бесконтрольно, вызывая сильную интоксикацию и дестабилизацию работы организма. Выйти из такого состояния самостоятельно не только трудно, но и небезопасно, поэтому необходима профессиональная помощь.
Детальнее – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]vyvod iz zapoya na domu nedorogo kapelnica [/url]
Круглосуточная поддержка при алкогольной зависимости помогает восстановить нормальное состояние здоровья и вернуться к полноценной жизни. Анонимное выведение из запоя позволяет получить необходимую медицинскую помощь с соблюдением конфиденциальности, минимизируя последствия алкоголизма и нормализуя общее состояние пациента.
Узнать больше – [url=https://vyvod-iz-zapoya-20.ru/]vyvod-iz-zapoya-20.ru[/url]
Запой представляет собой сложное состояние, связанное с длительным употреблением алкоголя, что приводит к формированию зависимости. Это явление не только нарушает нормальную жизнедеятельность, но также вызывает серьезные физические и психологические расстройства. Стационарное лечение становится необходимым для восстановления здоровья и профилактики осложнений. В стационаре клиники «Шаг к Трезвости» в Краснодаре мы предлагаем комплексный подход, включающий медикаментозное лечение, психотерапию и постоянный контроль со стороны квалифицированных специалистов.
Детальнее – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя на дому краснодар[/url]
Алкоголизм — это хроническое прогрессирующее заболевание, связанное с физической и психической зависимостью от этанола. Запой, как его крайнее проявление, представляет собой длительное употребление алкоголя, сопровождающееся тяжелыми нарушениями работы организма, включая абстинентный синдром и потенциальные угрозы для жизни. Лечение запоя требует комплексного подхода, включающего медицинские процедуры, психотерапию и реабилитационные меры.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]narkologiya vyvod iz zapoya na domu ekaterinburg[/url]
Запой представляет собой сложное состояние, связанное с длительным употреблением алкоголя, что приводит к формированию зависимости. Это явление не только нарушает нормальную жизнедеятельность, но также вызывает серьезные физические и психологические расстройства. Стационарное лечение становится необходимым для восстановления здоровья и профилактики осложнений. В стационаре клиники «Шаг к Трезвости» в Краснодаре мы предлагаем комплексный подход, включающий медикаментозное лечение, психотерапию и постоянный контроль со стороны квалифицированных специалистов.
Детальнее – [url=https://vyvod-iz-zapoya-12.ru/]наркологическая клиника краснодар[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Детальнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/[/url]
Для лечения запоев требуется помощь квалифицированных специалистов. В наркологической клинике «Излечение» в Краснодаре обеспечивают безопасный и комфортный выход из запоя, учитывая особенности состояния каждого пациента. Запой — одно из самых тяжелых проявлений алкогольной зависимости, сопровождающееся сильной интоксикацией, нарушением работы внутренних органов и психическими расстройствами. В этот период могут обостряться хронические заболевания, что усиливает необходимость срочной медицинской помощи.
Детальнее – [url=https://vyvod-iz-zapoya-17.ru/]наркологический центр в челябинске[/url]
В клинике также уделяется внимание конфиденциальности лечения. Понимая деликатность проблемы, врачи гарантируют полную анонимность на всех этапах терапии. Благодаря профессиональному подходу и использованию проверенных методик пациенты могут восстановить здоровье и вернуться к полноценной жизни.
Узнать больше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]narkolog vyvod iz zapoya cena v-sankt-peterburge[/url]
Для достижения эффективных результатов в лечении запоя необходима квалифицированная помощь специалистов. Наркологическая клиника «Излечение» в Краснодаре предоставляет профессиональные услуги по выводу из запоя, гарантируя безопасность, комфорт и индивидуальный подход к каждому пациенту.
Разобраться лучше – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-cena-v-krasnodare/]http://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-cena-v-krasnodare/[/url]
Запой — это продолжительное, неконтролируемое потребление алкоголя, которое часто приводит к серьезным физическим и психологическим проблемам. Во время запоя организм подвергается интоксикации, обезвоживанию, нарушениям работы нервной системы, а также утрате способности к самоконтролю. Для успешного преодоления этого состояния необходима профессиональная медицинская помощь, направленная на прекращение употребления алкоголя и восстановление нормального функционирования организма.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-17.ru/]vyvod iz zapoya s vyezdom v-chelyabinske[/url]
Наркологическая клиника «Союз Здоровья» в Воронеже специализируется на лечении алкогольной зависимости и предлагает профессиональные услуги по выводу из запоя. Мы стремимся создать атмосферу доверия, где каждый пациент сможет получить необходимую поддержку.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]вывод из запоя с выездом на дом в воронеже[/url]
Клиника «Рассвет» в Твери предоставляет услугу анонимного вывода из запоя, обеспечивая высокое качество медицинского обслуживания и строгое соблюдение всех этических норм.
Разобраться лучше – [url=https://vyvod-iz-zapoya-21.ru/]наркологическая клиника в твери[/url]
Алкогольная зависимость — это серьезная проблема, требующая незамедлительного вмешательства, чтобы избежать тяжелых последствий для здоровья. Запойное пьянство может привести к различным заболеваниям, таким как цирроз печени, панкреатит и другие опасные состояния. К счастью, современная медицина предлагает эффективные методы решения этой проблемы. Для тех, кто не хочет или не может посетить стационар, есть возможность пройти процедуру капельницы от запоя на дому в Твери.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]vyvod iz zapoya vrach na dom [/url]
Процесс выхода из запоя имеет большое значение в борьбе с алкоголизмом. Он требует не только профессионального медицинского вмешательства, но и внутренней мотивации пациента. Важным моментом является создание комфортных и анонимных условий лечения, особенно для тех, кто опасается осуждения со стороны окружающих. Одним из удобных решений является выведение из запоя на дому, что позволяет пациенту находиться в привычной обстановке и избегать стресса от госпитализации.
Разобраться лучше – [url=https://vyvod-iz-zapoya-16.ru/]наркологическая клиника в краснодаре[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Детальнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]срочный вывод из запоя на дому [/url]
Наркологическая клиника «Освобождение» в Рязани предлагает профессиональные услуги по выводу из запоя и комплексной реабилитации. Мы понимаем, что зависимость — это болезнь, требующая индивидуального подхода и поддержки не только для пациента, но и для его близких.
Выяснить больше – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-v-stacionare-v-ryazani/]вывод из запоя в стационаре анонимно в рязани[/url]
Круглосуточная поддержка при алкогольной зависимости помогает восстановить нормальное состояние здоровья и вернуться к полноценной жизни. Анонимное выведение из запоя позволяет получить необходимую медицинскую помощь с соблюдением конфиденциальности, минимизируя последствия алкоголизма и нормализуя общее состояние пациента.
Узнать больше – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-cena-v-ekaterinburge/]вывод из запоя с выездом цена екатеринбург[/url]
Клиника «Преображение» в Екатеринбурге предлагает анонимные услуги по выведению из запоя, придерживаясь высоких стандартов медицинской этики. Алкогольная зависимость — это серьёзная проблема, требующая комплексного подхода и внимания со стороны общества. Профессиональное вмешательство позволяет значительно снизить вредное воздействие алкоголя и способствует возвращению человека к нормальной жизни.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-20.ru/]vyvod iz zapoya s vyezdom[/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Детальнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-v-stacionare-v-chelyabinske/]вывод из запоя в стационаре челябинск[/url]
Запой — это тяжелое состояние, при котором человек теряет контроль над количеством употребляемого алкоголя, что приводит к серьезным нарушениям здоровья. Процесс вывода из запоя требует профессионального подхода, медицинского контроля и тщательного восстановления организма. Наркологическая клиника «Шаг к Трезвости» в Краснодаре предлагает квалифицированную помощь для тех, кто нуждается в срочном и безопасном выходе из запоя. Мы предоставляем комплексное лечение, ориентированное на каждый этап восстановления, с максимальной заботой о здоровье пациента.
Углубиться в тему – [url=https://vyvod-iz-zapoya-12.ru/]http://vyvod-iz-zapoya-12.ru[/url]
Клиника «Рассвет» в Твери предоставляет услугу анонимного вывода из запоя, обеспечивая высокое качество медицинского обслуживания и строгое соблюдение всех этических норм.
Подробнее – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]нарколог на дом вывод из запоя [/url]
Алкогольная зависимость остаётся серьёзной проблемой, требующей квалифицированного медицинского вмешательства. Капельница от запоя — это ключевой этап в процессе лечения, направленный на стабилизацию состояния пациента и его возвращение к полноценной жизни. Профессиональная помощь позволяет эффективно справиться с последствиями длительного употребления алкоголя и восстановить физическое и психологическое здоровье.
Подробнее тут – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]narkologiya vyvod iz zapoya na domu v-tveri[/url]
Наркологическая клиника «Освобождение» в Рязани предлагает профессиональные услуги по выводу из запоя и комплексной реабилитации. Мы понимаем, что зависимость — это болезнь, требующая индивидуального подхода и поддержки не только для пациента, но и для его близких.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-13.ru/]vyvod iz zapoya s vyezdom ryazan[/url]
Алкогольный запой представляет собой серьезное состояние, которое требует немедленного медицинского вмешательства. Это состояние характеризуется длительным и бесконтрольным потреблением алкоголя, что приводит к разрушению как физического, так и психоэмоционального здоровья человека. Для эффективного и безопасного вывода из запоя стационарное лечение является оптимальным решением, особенно при тяжелых случаях интоксикации. В стационаре клиники «Заря Будущего» мы предлагаем комплексную программу вывода из запоя, которая включает детоксикацию, восстановление организма и психотерапевтическую помощь.
Углубиться в тему – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя на дому санкт-петербург[/url]
Хроническая алкогольная зависимость проявляется как длительное употребление спиртных напитков, которое сопровождается серьёзными нарушениями в организме, включая абстинентный синдром и риск для жизни. Эффективная терапия требует комплексного подхода, включающего медицинское вмешательство, психологическую поддержку и реабилитацию.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-20.ru/]http://www.vyvod-iz-zapoya-20.ru[/url]
Продолжительное употребление алкоголя приводит к состоянию, при котором человек теряет способность самостоятельно остановиться, оказываясь в плену физической и психологической зависимости. Такое положение дел представляет серьёзную угрозу для здоровья и требует незамедлительного вмешательства профессионалов. В Екатеринбурге наркологическая клиника «Преображение» предлагает специализированную помощь, используя проверенные и безопасные методы лечения.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]вывод из запоя капельница на дому екатеринбург[/url]
Алкогольная зависимость – это состояние, при котором человек теряет способность контролировать употребление спиртных напитков, что со временем приводит к глубокой физической и психической привязанности. Одна из наиболее опасных стадий – запой, когда алкоголь потребляется бесконтрольно, вызывая сильную интоксикацию и дестабилизацию работы организма. Выйти из такого состояния самостоятельно не только трудно, но и небезопасно, поэтому необходима профессиональная помощь.
Узнать больше – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]vyvod iz zapoya na domu nedorogo kapelnica [/url]
Алкогольная зависимость — это хроническое заболевание, которое требует комплексного подхода и квалифицированного лечения. В клинике «Излечение» гарантируют полную анонимность на всех этапах лечения, что особенно важно для тех, кто хочет избежать общественного осуждения. Пациенты могут получить помощь как в стационаре, так и на дому, при этом обеспечивается максимальный комфорт и конфиденциальность.
Получить больше информации – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]срочный вывод из запоя на дому [/url]
Процедура вывода из запоя направлена на устранение последствий длительного употребления алкоголя и восстановления работы организма. Этот процесс включает не только медикаментозное лечение, но и психологическую поддержку, что позволяет минимизировать симптомы абстиненции и предотвратить возможные осложнения.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]narkolog na dom vyvod iz zapoya sankt-peterburg[/url]
Алкогольная зависимость становится всё более распространенной проблемой, требующей внимания как со стороны медицинского сообщества, так и общества в целом. Вывод из запоя — это сложный и ответственный процесс, необходимый для восстановления здоровья пациента и его интеграции в нормальную жизнь. Профессиональное вмешательство позволяет минимизировать последствия длительного употребления алкоголя и нормализовать состояние организма.
Выяснить больше – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]наркология вывод из запоя на дому [/url]
В клинике работает команда квалифицированных специалистов, которые осуществляют вывод из запоя, обеспечивая полный контроль над состоянием пациента и устраняя все последствия длительного употребления алкоголя.
Получить больше информации – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-cena-v-ekaterinburge/]вывод из запоя с выездом цена в екатеринбурге[/url]
В таких ситуациях вывод из запоя на дому становится оптимальным решением, особенно если пациент не может или не хочет посещать стационар. Эта услуга позволяет получить медицинскую помощь в комфортной и привычной обстановке, что снижает уровень стресса и ускоряет процесс восстановления.
Узнать больше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]вывод из запоя с выездом на дом [/url]
Запой – это тяжелое состояние, возникающее из-за длительного и неконтролируемого употребления алкоголя. Оно характеризуется физической и психологической зависимостью, что приводит к серьезным последствиям для организма и психики. Для восстановления нормального самочувствия и здоровья необходимо профессиональное лечение. Одним из наиболее удобных и эффективных методов является вывод из запоя на дому.
Подробнее – [url=https://vyvod-iz-zapoya-13.ru/]vyvod iz zapoya[/url]
Запой — это продолжительное, неконтролируемое потребление алкоголя, которое часто приводит к серьезным физическим и психологическим проблемам. Во время запоя организм подвергается интоксикации, обезвоживанию, нарушениям работы нервной системы, а также утрате способности к самоконтролю. Для успешного преодоления этого состояния необходима профессиональная медицинская помощь, направленная на прекращение употребления алкоголя и восстановление нормального функционирования организма.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]наркология вывод из запоя анонимно краснодар[/url]
Запой — это состояние, при котором человек продолжает употреблять алкоголь на протяжении нескольких дней или даже недель, не контролируя объемы потребляемого напитка. Этот процесс сопровождается тяжелыми физическими и психическими последствиями, такими как обезвоживание организма, отравление продуктами распада алкоголя, нервные расстройства и потеря контроля над ситуацией. Вывод из запоя — это медицинская процедура, направленная на прекращение употребления алкоголя и восстановление организма пациента.
Подробнее тут – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]наркология вывод из запоя на дому [/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]срочный вывод из запоя на дому санкт-петербург[/url]
Алкоголизм — это хроническое заболевание, которое характеризуется патологической зависимостью от этанола. Периоды запоя, когда человек неконтролируемо потребляет алкоголь, могут привести к разнообразным медицинским осложнениям, как физическим, так и психологическим. Запой становится не только причиной ухудшения здоровья, но и причиной множества социальных и личных проблем. На этом фоне возникает необходимость вывода из запоя, процесс, который должен учитывать индивидуальные особенности пациента. Важно, что анонимность в реабилитации играет ключевую роль, помогая избежать стигматизации и увеличивая шансы на успешное восстановление.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]vyvod iz zapoya vyzov na dom ryazan[/url]
Современное общество сталкивается с серьёзными вызовами, связанными с зависимостями. Алкоголизм, как одна из самых распространённых проблем, оказывает разрушительное воздействие на здоровье человека, разрушая семейные отношения и затрудняя социальную адаптацию. В такие моменты крайне важно обратиться за квалифицированной помощью.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-v-stacionare-v-ryazani/]вывод из запоя в стационаре рязань[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Получить больше информации – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-v-kruglosutochno-v-chelyabinske/]vyvod iz zapoya srochno kruglosutochno [/url]
Вывод из запоя представляет собой важный этап в процессе лечения алкогольной зависимости. Это медицинская процедура, необходимая для устранения токсических веществ, накопленных в организме в результате длительного употребления алкоголя. Алкогольная зависимость может вызывать множество проблем, включая физические и психоэмоциональные расстройства. Своевременное вмешательство специалистов помогает минимизировать риски и способствует восстановлению здоровья пациента.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-13.ru/]vyvod iz zapoya kapelnica ryazan[/url]
Клиника “Второй Шанс” в Санкт-Петербурге предлагает услугу вывода из запоя, как в стационаре, так и на дому. Для пациентов, не желающих посещать клинику, квалифицированные врачи готовы предоставить помощь в комфортных домашних условиях. Используя современные методы, специалисты обеспечивают безопасность, анонимность и высокий уровень медицинской поддержки.
Углубиться в тему – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]vyvod iz zapoya kruglosutochno sankt-peterburg[/url]
Процесс выхода из запоя имеет большое значение в борьбе с алкоголизмом. Он требует не только профессионального медицинского вмешательства, но и внутренней мотивации пациента. Важным моментом является создание комфортных и анонимных условий лечения, особенно для тех, кто опасается осуждения со стороны окружающих. Одним из удобных решений является выведение из запоя на дому, что позволяет пациенту находиться в привычной обстановке и избегать стресса от госпитализации.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-17.ru/]вывод из запоя капельница[/url]
Выход из запоя — это важнейший этап на пути к избавлению от алкогольной зависимости. Он требует комплексного подхода, профессиональной помощи и сильной мотивации пациента. В условиях, когда анонимность и комфорт становятся ключевыми факторами для многих людей, вывод из запоя на дому приобретает все большее значение. Это решение позволяет человеку получить необходимую помощь, не покидая дома, и избежать стресса, связанного с госпитализацией.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-stacionare-v-krasnodare/]вывод из запоя в стационаре анонимно [/url]
Затянувшийся запой может вызвать серьезные проблемы со здоровьем, включая абстинентный синдром, который проявляется такими симптомами, как тошнота, тремор и головная боль. Эти состояния требуют немедленного вмешательства медицинских специалистов. Эффективное лечение запоя предполагает не только снятие физической зависимости, но и оказание психологической поддержки. В сложных случаях, когда состояние пациента требует постоянного наблюдения, может быть рекомендовано стационарное лечение с интенсивной терапией.
Подробнее тут – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya na domu nedorogo kapelnica chelyabinsk[/url]
Процесс вывода из запоя требует быстрого реагирования. Запой, особенно в его тяжелых формах, представляет угрозу не только для психического состояния пациента, но и для его физического здоровья. Чтобы минимизировать риски и предотвратить осложнения, важно начинать лечение как можно скорее. Клинике «Освобождение» в Рязани доступна круглосуточная помощь — мы работаем 24/7, чтобы оперативно вмешаться в любой момент, когда это необходимо. Врачебная помощь может быть предоставлена как в стационаре клиники, так и на дому, в зависимости от состояния пациента и его предпочтений.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-v-stacionare-v-ryazani/]вывод из запоя в стационаре анонимно рязань[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Детальнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]narkolog na dom vyvod iz zapoya sankt-peterburg[/url]
Запойное состояние представляет собой серьёзную угрозу для здоровья, поскольку сопровождается тяжёлой интоксикацией организма и нарушением психоэмоционального состояния. Решение о лечении в стационаре клиники «Союз Здоровья» позволяет справиться с последствиями длительного употребления алкоголя, обеспечивая пациентам полный комплекс медицинской и психологической помощи.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-kruglosutochno-v-voronezhe/]вывод из запоя круглосуточно наркология [/url]
Процесс вывода из запоя включает несколько этапов, направленных на детоксикацию и восстановление нормальной работы организма. Для этого необходимы высококвалифицированные специалисты, которые помогают избавиться от последствий токсического воздействия алкоголя и восстанавливают здоровье пациента. Стоимость лечения зависит от необходимого объема услуг и условий их предоставления. В клинике «Излечение» предлагаются доступные цены, с учетом индивидуальных потребностей каждого пациента.
Подробнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-cena-v-krasnodare/]вывод из запоя на дому цена [/url]
В некоторых ситуациях, когда запой длится несколько дней или приводит к серьезным последствиям для здоровья, необходим срочный вызов нарколога. Среди признаков, при которых требуется неотложная помощь:
Выяснить больше – [url=https://kapelnica-ot-zapoya-moskva2.ru/]капельница от запоя с выездом цена в москве[/url]
Процесс вывода из запоя требует быстрого реагирования. Запой, особенно в его тяжелых формах, представляет угрозу не только для психического состояния пациента, но и для его физического здоровья. Чтобы минимизировать риски и предотвратить осложнения, важно начинать лечение как можно скорее. Клинике «Освобождение» в Рязани доступна круглосуточная помощь — мы работаем 24/7, чтобы оперативно вмешаться в любой момент, когда это необходимо. Врачебная помощь может быть предоставлена как в стационаре клиники, так и на дому, в зависимости от состояния пациента и его предпочтений.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-v-kruglosutochno-v-ryazani/]вывод из запоя срочно круглосуточно [/url]
Если затянувшееся употребление алкоголя не прерывается, организм начинает испытывать тяжелые последствия. Возникает абстинентный синдром, проявляющийся в виде тошноты, сильной головной боли, дрожи в теле и других неприятных симптомов. Без своевременной помощи состояние может ухудшиться, поэтому важна быстрая медицинская реакция. Комплексное лечение направлено не только на избавление от физической зависимости, но и на поддержку человека в эмоциональном плане. В случаях, когда требуется постоянное наблюдение, рекомендуется стационарная терапия.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]vyvod iz zapoya srochno kruglosutochno v-krasnodare[/url]
Запой — это опасное состояние, которое развивается у людей с алкогольной зависимостью и характеризуется длительным непрерывным потреблением алкоголя. Это состояние приводит к серьезным нарушениям в организме, как физическим, так и психоэмоциональным. Длительный запой может вызвать множество осложнений, таких как поражение внутренних органов, нервной системы, а также проблемы в социальной и семейной жизни. Однако современная медицина предоставляет эффективные методы вывода из запоя, и одним из наиболее удобных и востребованных является вывод из запоя на дому.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]vyvod iz zapoya v stacionare anonimno [/url]
Алкогольная зависимость — это серьезное хроническое заболевание, которое характеризуется непреодолимым стремлением к употреблению спиртных напитков, потерей контроля над их потреблением и развитием толерантности. Запойное состояние, при котором человек продолжает пить без перерыва в течение нескольких дней, приводит к серьезной интоксикации организма и может вызвать опасные осложнения. Капельница от запоя — один из наиболее эффективных методов лечения, направленный на быструю детоксикацию и стабилизацию состояния пациента. В наркологической клинике “Восстановление души” в Иркутске мы предлагаем профессиональную медицинскую помощь с учетом индивидуальных потребностей каждого пациента.
Углубиться в тему – [url=https://kapelnica-ot-zapoya-irkutsk3.ru/kapelnica-ot-zapoya-na-domu-v-irkutske/]kapelnica ot zapoya na domu kruglosutochno[/url]
Клиника “Восстановление души” предлагает услугу капельницы от запоя на дому в Иркутске и Иркутской области, что является удобным решением для тех, кто не может или не хочет лечиться в стационаре. Мы понимаем, как важно поддерживать пациента в момент, когда его организм нуждается в быстрой и квалифицированной помощи.
Выяснить больше – [url=https://kapelnica-ot-zapoya-moskva2.ru/]kapelnica ot zapoya na domu cena[/url]
В этой статье мы расскажем о возможностях клиники для лечения алкогольной интоксикации круглосуточно, включая вывод из запоя в стационаре и на дому, а также о роли профессионалов, таких как нарколог, в процессе лечения.
Детальнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-cena-v-krasnodare/]нарколог вывод из запоя цена краснодар[/url]
Мы понимаем, что каждая зависимость уникальна, поэтому основой нашего подхода является индивидуализация лечения. После тщательной диагностики, включающей анализ медицинской истории, психологического состояния и социальных факторов, наши специалисты разрабатывают персонализированный план терапии. Этот план может включать медикаментозную детоксикацию, психотерапевтические методы и социальные программы, направленные на изменение поведения и мышления пациента.
В клинике “Восстановление души” работает команда высококвалифицированных специалистов, готовых предложить современное и эффективное лечение зависимостей. Наши врачи-наркологи обладают обширным опытом работы и постоянно совершенствуют свои навыки, чтобы использовать самые передовые методы терапии.
Зависимость — это серьёзная проблема, которая затрагивает не только человека, но и его семью и окружение. Алкоголизм, наркомания и другие виды зависимостей могут разрушить жизнь, но с профессиональной помощью можно вернуть её в русло нормального функционирования. Наркологическая клиника «Заря Будущего» в Санкт-Петербурге предлагает уникальные программы лечения зависимостей, которые сочетает медико-психологический подход, индивидуальные методики и квалифицированную поддержку.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya kapelnica na domu sankt-peterburg[/url]
Одной из распространенных проблем является запой, состояние, при котором человек длительное время бесконтрольно употребляет алкоголь, что приводит к тяжелой физической и психологической зависимости. Такое поведение чревато серьезными последствиями для здоровья, включая поражение внутренних органов, нервные расстройства и другие заболевания. Важно своевременно обратиться за профессиональной помощью, чтобы минимизировать риски и предотвратить осложнения.
Выяснить больше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]vyvod iz zapoya na domu cena [/url]
Клиника “Второй Шанс” в Санкт-Петербурге предлагает услугу вывода из запоя, как в стационаре, так и на дому. Для пациентов, не желающих посещать клинику, квалифицированные врачи готовы предоставить помощь в комфортных домашних условиях. Используя современные методы, специалисты обеспечивают безопасность, анонимность и высокий уровень медицинской поддержки.
Выяснить больше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]vyvod iz zapoya kruglosutochno narkologiya v-sankt-peterburge[/url]
В клинике “Восстановление души” работает команда высококвалифицированных специалистов, готовых предложить современное и эффективное лечение зависимостей. Наши врачи-наркологи обладают обширным опытом работы и постоянно совершенствуют свои навыки, чтобы использовать самые передовые методы терапии.
Детальнее – [url=https://kapelnica-ot-zapoya-irkutsk.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-irkutske/]kapelnica ot zapoya anonimno irkutsk[/url]
В таких ситуациях вывод из запоя на дому становится оптимальным решением, особенно если пациент не может или не хочет посещать стационар. Эта услуга позволяет получить медицинскую помощь в комфортной и привычной обстановке, что снижает уровень стресса и ускоряет процесс восстановления.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]вывод из запоя с выездом на дом в воронеже[/url]
Запой — это опасное состояние, которое развивается у людей с алкогольной зависимостью и характеризуется длительным непрерывным потреблением алкоголя. Это состояние приводит к серьезным нарушениям в организме, как физическим, так и психоэмоциональным. Длительный запой может вызвать множество осложнений, таких как поражение внутренних органов, нервной системы, а также проблемы в социальной и семейной жизни. Однако современная медицина предоставляет эффективные методы вывода из запоя, и одним из наиболее удобных и востребованных является вывод из запоя на дому.
Подробнее тут – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu nedorogo kapelnica sankt-peterburg[/url]
В современном мире зависимость становится все более распространенной проблемой. Стресс, жизненные трудности и финансовые проблемы часто провоцируют развитие таких серьезных состояний, как алкоголизм, наркомания и игромания. Наркологическая клиника “Второй Шанс” предоставляет помощь людям, столкнувшимся с этими заболеваниями. Основное направление работы клиники — восстановление здоровья пациентов, их социальная адаптация и предотвращение повторных рецидивов.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]анонимный вывод из запоя на дому в санкт-петербурге[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Детальнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]vyvod iz zapoya s vyezdom cena v-sankt-peterburge[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]vyvod iz zapoya kruglosutochno sankt-peterburg[/url]
Современное общество сталкивается с серьёзными вызовами, связанными с зависимостями. Алкоголизм, как одна из самых распространённых проблем, оказывает разрушительное воздействие на здоровье человека, разрушая семейные отношения и затрудняя социальную адаптацию. В такие моменты крайне важно обратиться за квалифицированной помощью.
Получить больше информации – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-cena-v-ryazani/]narkolog vyvod iz zapoya cena ryazan[/url]
Поддержка — ключевой элемент на пути к выздоровлению. Мы предлагаем программы, которые продолжаются даже после завершения основного курса лечения. Пациенты имеют возможность участвовать в регулярных встречах с психологами и наркологами, где они могут делиться своими успехами и получать необходимую помощь.
Ознакомиться с деталями – [url=https://kapelnica-ot-zapoya-voronezh2.ru/kapelnica-ot-zapoya-anonimno-v-voronezhe/]kapelnica ot zapoya kruglosutochno[/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Разобраться лучше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]вывод из запоя анонимно недорого в санкт-петербурге[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Выяснить больше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]narkolog vyvod iz zapoya cena [/url]
Запой – это состояние, возникающее из-за длительного употребления алкоголя и характеризующееся физической и психологической зависимостью. Оно требует комплексного медицинского вмешательства для устранения последствий и предотвращения дальнейшего ухудшения здоровья. Стационарное лечение в клинике «Освобождение» в Рязани – это эффективный способ вернуть пациента к нормальной жизни, предоставляя ему комфортные условия и круглосуточную помощь специалистов.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-v-kruglosutochno-v-ryazani/]vyvod iz zapoya kruglosutochno ryazan[/url]
Вывод из запойного состояния – сложный процесс, включающий несколько последовательных этапов. Основная цель – детоксикация организма и восстановление его нормального функционирования. Важно, чтобы процессом руководили опытные специалисты, способные минимизировать последствия интоксикации и помочь человеку вернуться к здоровой жизни. Стоимость лечения зависит от выбранных методов и уровня медицинского обслуживания, а в клинике «Излечение» предлагаются доступные варианты с учетом индивидуальных потребностей пациентов.
Детальнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]vyvod iz zapoya na domu nedorogo kapelnica krasnodar[/url]
Одним из главных преимуществ стационарного лечения в нашей клинике является полная анонимность. Мы понимаем, что многие пациенты боятся раскрытия своей зависимости и осуждения со стороны окружающих. В “Восстановление души” мы гарантируем конфиденциальность всех процедур и защиту личных данных пациента. Это создает безопасную и доверительную атмосферу, необходимую для успешного лечения и психологического восстановления.
Исследовать вопрос подробнее – [url=https://kapelnica-ot-zapoya-moskva2.ru/kapelnica-ot-zapoya-anonimno-v-moskve/]быстрая капельница от запоя в стационаре в москве[/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]vyvod iz zapoya anonimno nedorogo v-sankt-peterburge[/url]
Клиника “Восстановление души” предлагает услугу капельницы от запоя на дому в Иркутске и Иркутской области, что является удобным решением для тех, кто не может или не хочет лечиться в стационаре. Мы понимаем, как важно поддерживать пациента в момент, когда его организм нуждается в быстрой и квалифицированной помощи.
Подробнее – [url=https://kapelnica-ot-zapoya-voronezh2.ru/]anonimnaya kapelnica ot zapoya na domu v voronezhe[/url]
В современном мире зависимость становится все более распространенной проблемой. Стресс, жизненные трудности и финансовые проблемы часто провоцируют развитие таких серьезных состояний, как алкоголизм, наркомания и игромания. Наркологическая клиника “Второй Шанс” предоставляет помощь людям, столкнувшимся с этими заболеваниями. Основное направление работы клиники — восстановление здоровья пациентов, их социальная адаптация и предотвращение повторных рецидивов.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu nedorogo kapelnica v-sankt-peterburge[/url]
Современное общество сталкивается с серьёзными вызовами, связанными с зависимостями. Алкоголизм, как одна из самых распространённых проблем, оказывает разрушительное воздействие на здоровье человека, разрушая семейные отношения и затрудняя социальную адаптацию. В такие моменты крайне важно обратиться за квалифицированной помощью.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-cena-v-ryazani/]vyvod iz zapoya s vyezdom cena [/url]
Если затянувшееся употребление алкоголя не прерывается, организм начинает испытывать тяжелые последствия. Возникает абстинентный синдром, проявляющийся в виде тошноты, сильной головной боли, дрожи в теле и других неприятных симптомов. Без своевременной помощи состояние может ухудшиться, поэтому важна быстрая медицинская реакция. Комплексное лечение направлено не только на избавление от физической зависимости, но и на поддержку человека в эмоциональном плане. В случаях, когда требуется постоянное наблюдение, рекомендуется стационарная терапия.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]vyvod iz zapoya kruglosutochno narkologiya krasnodar[/url]
Запой – это тяжелое состояние, возникающее из-за длительного и неконтролируемого употребления алкоголя. Оно характеризуется физической и психологической зависимостью, что приводит к серьезным последствиям для организма и психики. Для восстановления нормального самочувствия и здоровья необходимо профессиональное лечение. Одним из наиболее удобных и эффективных методов является вывод из запоя на дому.
Получить больше информации – [url=https://vyvod-iz-zapoya-13.ru/]вывод из запоя с выездом в рязани[/url]
Алкогольная зависимость представляет собой актуальную медицинскую проблему, требующую тщательного подхода. Запой характеризуется длительным, непрерывным употреблением спиртных напитков, что ведёт к физической и психической зависимости. На фоне запойного состояния могут возникнуть тяжёлые нарушения функций органов, увеличивается риск развития таких состояний, как алкогольный делирий. Вывод из запоя становится ключевым этапом в лечении зависимостей, который может осуществляться как в стационарных условиях, так и на дому, в зависимости от состояния пациента. В клинике «Перекресток Надежды» в Челябинске мы предлагаем круглосуточную помощь, чтобы обеспечить максимально эффективное и безопасное лечение при любом варианте вывода из запоя.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-17.ru/]vyvod iz zapoya kapelnica[/url]
Запой — это опасное состояние, которое развивается у людей с алкогольной зависимостью и характеризуется длительным непрерывным потреблением алкоголя. Это состояние приводит к серьезным нарушениям в организме, как физическим, так и психоэмоциональным. Длительный запой может вызвать множество осложнений, таких как поражение внутренних органов, нервной системы, а также проблемы в социальной и семейной жизни. Однако современная медицина предоставляет эффективные методы вывода из запоя, и одним из наиболее удобных и востребованных является вывод из запоя на дому.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя вызов на дом в санкт-петербурге[/url]
В клинике «Заря Будущего» мы понимаем, насколько важен своевременный и профессиональный подход к лечению зависимости. Наши специалисты готовы оказать квалифицированную помощь прямо у вас дома, обеспечивая полную безопасность и конфиденциальность на каждом этапе лечения.
Узнать больше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]bystry vyvod iz zapoya v stacionare v-sankt-peterburge[/url]
Зависимость от алкоголя – серьезная проблема, требующая системного подхода и профессионального лечения. В клинике «Излечение» гарантируется полная конфиденциальность на всех этапах процесса, что позволяет пациентам чувствовать себя в безопасности. Лечение может проходить как в стационаре, так и на дому, при этом соблюдаются все условия для максимального комфорта.
Детальнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя капельница на дому в краснодаре[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Выяснить больше – [url=https://vyvod-iz-zapoya-19.ru/]narkologicheskij centr v-sankt-peterburge[/url]
Запой — это серьезное состояние, при котором человек не может прекратить употребление алкоголя на протяжении длительного времени, что вызывает физическую и психологическую зависимость. Это крайне опасное состояние, требующее немедленного вмешательства специалистов. Для безопасного и эффективного вывода из запоя необходима квалифицированная медицинская помощь, которую предоставляет наркологическая клиника «Рассвет» в Твери. Наши опытные специалисты используют современные методы детоксикации и восстановления организма, обеспечивая полный медицинский контроль и поддержку на всех этапах лечения.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-v-kruglosutochno-v-tveri/]vyvod iz zapoya srochno kruglosutochno tver[/url]
Процесс возвращения к нормальной жизни после длительного употребления алкоголя играет ключевую роль в избавлении от зависимости. Медицинское сопровождение должно сочетаться с осознанным желанием человека изменить свою жизнь. Важно, чтобы лечение проходило в условиях, исключающих стресс и дискомфорт. Для этого предусмотрены как стационарные программы, так и возможность получить медицинскую помощь на дому. Такой вариант подходит тем, кто предпочитает находиться в привычной обстановке и избегать огласки.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]vyvod iz zapoya kapelnica na domu [/url]
Мы понимаем, что каждая зависимость уникальна, поэтому основой нашего подхода является индивидуализация лечения. После тщательной диагностики, включающей анализ медицинской истории, психологического состояния и социальных факторов, наши специалисты разрабатывают персонализированный план терапии. Этот план может включать медикаментозную детоксикацию, психотерапевтические методы и социальные программы, направленные на изменение поведения и мышления пациента.
Разобраться лучше – [url=https://kapelnica-ot-zapoya-voronezh2.ru/kapelnica-ot-zapoya-anonimno-v-voronezhe/]kapelnica ot zapoya kruglosutochno narkologiya v voronezhe[/url]
This theme is simply matchless :), it is interesting to me)))
Клиника предлагает гибкие варианты лечения, включая стационарное и амбулаторное. Стоимость услуг зависит от индивидуальных потребностей пациента, степени тяжести состояния и объемов необходимых процедур. Специалисты стремятся создать максимально комфортные условия для каждого пациента, обеспечивая прозрачное ценообразование и высокое качество обслуживания.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя на дому санкт-петербург[/url]
Клиника «Преображение» предлагает услуги анонимного вывода из запоя в Екатеринбурге, обеспечивая высокое качество медицинской помощи и соблюдение всех этических норм.
Детальнее – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]вывод из запоя вызов на дом екатеринбург[/url]
После успешной детоксикации начинается этап реабилитации, который включает восстановление социального статуса пациента и формирование новых здоровых привычек. Мы предлагаем как групповые, так и индивидуальные занятия, направленные на изменение поведения и мышления, что способствует долгосрочному удержанию пациента от возвращения к зависимостям.
Алкогольная зависимость — это серьезное заболевание, при котором человек теряет контроль над потреблением алкоголя, что приводит к его физической и психологической зависимости. Одним из наиболее выраженных проявлений алкоголизма является состояние запоя — длительное и неконтролируемое употребление спиртных напитков. В таких случаях капельница от запоя становится не только быстрым, но и безопасным методом для избавления от последствий интоксикации.
Запой нарушает привычный ритм жизни, вызывая серьезные физические и эмоциональные проблемы. Для его лечения требуется не только медикаментозное вмешательство, но и постоянное наблюдение специалистов. Стационарное лечение в клинике позволяет обеспечить полный контроль над состоянием пациента, что минимизирует риски и способствует более эффективной реабилитации.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-14.ru/]наркологический центр[/url]
Алкогольный запой представляет собой серьезное состояние, которое требует немедленного медицинского вмешательства. Это состояние характеризуется длительным и бесконтрольным потреблением алкоголя, что приводит к разрушению как физического, так и психоэмоционального здоровья человека. Для эффективного и безопасного вывода из запоя стационарное лечение является оптимальным решением, особенно при тяжелых случаях интоксикации. В стационаре клиники «Заря Будущего» мы предлагаем комплексную программу вывода из запоя, которая включает детоксикацию, восстановление организма и психотерапевтическую помощь.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu kruglosutochno [/url]
Процесс вывода из запоя требует быстрого реагирования. Запой, особенно в его тяжелых формах, представляет угрозу не только для психического состояния пациента, но и для его физического здоровья. Чтобы минимизировать риски и предотвратить осложнения, важно начинать лечение как можно скорее. Клинике «Освобождение» в Рязани доступна круглосуточная помощь — мы работаем 24/7, чтобы оперативно вмешаться в любой момент, когда это необходимо. Врачебная помощь может быть предоставлена как в стационаре клиники, так и на дому, в зависимости от состояния пациента и его предпочтений.
Подробнее – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-anonimno-v-ryazani/]vyvod iz zapoya anonimno v-ryazani[/url]
Мы понимаем, что каждая зависимость уникальна, поэтому основой нашего подхода является индивидуализация лечения. После тщательной диагностики, включающей анализ медицинской истории, психологического состояния и социальных факторов, наши специалисты разрабатывают персонализированный план терапии. Этот план может включать медикаментозную детоксикацию, психотерапевтические методы и социальные программы, направленные на изменение поведения и мышления пациента.
Разобраться лучше – [url=https://kapelnica-ot-zapoya-moskva3.ru/kapelnica-ot-zapoya-na-domu-v-moskve/]https://kapelnica-ot-zapoya-moskva3.ru/kapelnica-ot-zapoya-na-domu-v-moskve[/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]вывод из запоя круглосуточно санкт-петербург[/url]
Запой – это состояние, возникающее из-за длительного употребления алкоголя и характеризующееся физической и психологической зависимостью. Оно требует комплексного медицинского вмешательства для устранения последствий и предотвращения дальнейшего ухудшения здоровья. Стационарное лечение в клинике «Освобождение» в Рязани – это эффективный способ вернуть пациента к нормальной жизни, предоставляя ему комфортные условия и круглосуточную помощь специалистов.
Узнать больше – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-anonimno-v-ryazani/]anonimny vyvod iz zapoya na domu v-ryazani[/url]
[url=https://noves-shop.biz/]купить аккаунт инстаграм[/url] – аккаунт фейсбук купить фанпей, фейковый аккаунт инстаграм купить
Злоупотребление алкоголем в течение продолжительного времени приводит к состоянию, при котором человек теряет контроль над собой, а его организм подвергается серьезным нагрузкам. Последствия такого состояния проявляются на физическом и психическом уровнях: организм страдает от отравления, обезвоживания и сбоев в работе нервной системы, а человек теряет способность адекватно оценивать ситуацию. Выйти из этого состояния без медицинской помощи крайне сложно, так как требуется не только прекратить употребление алкоголя, но и восстановить нормальные функции организма.
Получить больше информации – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-cena-v-krasnodare/]vyvod iz zapoya cena narkologiya [/url]
Запой – это тяжелое состояние, возникающее из-за длительного и неконтролируемого употребления алкоголя. Оно характеризуется физической и психологической зависимостью, что приводит к серьезным последствиям для организма и психики. Для восстановления нормального самочувствия и здоровья необходимо профессиональное лечение. Одним из наиболее удобных и эффективных методов является вывод из запоя на дому.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-v-stacionare-v-ryazani/]vyvod iz zapoya v stacionare [/url]
В современном мире зависимость становится все более распространенной проблемой. Стресс, жизненные трудности и финансовые проблемы часто провоцируют развитие таких серьезных состояний, как алкоголизм, наркомания и игромания. Наркологическая клиника “Второй Шанс” предоставляет помощь людям, столкнувшимся с этими заболеваниями. Основное направление работы клиники — восстановление здоровья пациентов, их социальная адаптация и предотвращение повторных рецидивов.
Получить больше информации – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu kruglosutochno [/url]
Запой – это состояние, которое возникает вследствие длительного употребления алкоголя, приводящее к потере контроля над количеством выпиваемого и формированию как физической, так и психологической зависимости. Оно сопровождается серьёзными последствиями для здоровья и требует немедленного вмешательства.
Получить больше информации – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-stacionare-v-voronezhe/]vyvod iz zapoya v stacionare voronezh[/url]
Алкогольная зависимость — это серьезное заболевание, при котором человек теряет контроль над потреблением алкоголя, что приводит к его физической и психологической зависимости. Одним из наиболее выраженных проявлений алкоголизма является состояние запоя — длительное и неконтролируемое употребление спиртных напитков. В таких случаях капельница от запоя становится не только быстрым, но и безопасным методом для избавления от последствий интоксикации.
Выяснить больше – [url=https://kapelnica-ot-zapoya-voronezh2.ru/kapelnica-ot-zapoya-anonimno-v-voronezhe/]kapelnica ot zapoya kruglosutochno v voronezhe[/url]
[url=http://lordfilm-w.com/multfilmy/]онлайн мультфильмы в хорошем качестве[/url] – смотреть фильмы бесплатно, онлайн мультфильмы в хорошем качестве
Зависимость от алкоголя – серьезная проблема, требующая системного подхода и профессионального лечения. В клинике «Излечение» гарантируется полная конфиденциальность на всех этапах процесса, что позволяет пациентам чувствовать себя в безопасности. Лечение может проходить как в стационаре, так и на дому, при этом соблюдаются все условия для максимального комфорта.
Узнать больше – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-cena-v-krasnodare/]vyvod iz zapoya na domu cena [/url]
Запой — это опасное состояние, которое развивается у людей с алкогольной зависимостью и характеризуется длительным непрерывным потреблением алкоголя. Это состояние приводит к серьезным нарушениям в организме, как физическим, так и психоэмоциональным. Длительный запой может вызвать множество осложнений, таких как поражение внутренних органов, нервной системы, а также проблемы в социальной и семейной жизни. Однако современная медицина предоставляет эффективные методы вывода из запоя, и одним из наиболее удобных и востребованных является вывод из запоя на дому.
Выяснить больше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya kapelnica na domu [/url]
Вывод из запойного состояния – сложный процесс, включающий несколько последовательных этапов. Основная цель – детоксикация организма и восстановление его нормального функционирования. Важно, чтобы процессом руководили опытные специалисты, способные минимизировать последствия интоксикации и помочь человеку вернуться к здоровой жизни. Стоимость лечения зависит от выбранных методов и уровня медицинского обслуживания, а в клинике «Излечение» предлагаются доступные варианты с учетом индивидуальных потребностей пациентов.
Узнать больше – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]вывод из запоя круглосуточно в краснодаре[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-19.ru/]наркологический центр[/url]
Запой — это тяжелое состояние, возникающее вследствие длительного и непрерывного употребления алкоголя, которое сопровождается физической и психической зависимостью. Это опасное явление требует незамедлительного вмешательства специалистов. Вывод из запоя — это комплексный процесс медицинской детоксикации, который включает в себя очищение организма от токсинов, нормализацию функций внутренних органов и поддержку психоэмоционального состояния пациента. В случае тяжелого запоя стационарное лечение становится оптимальным решением для безопасного и эффективного вывода из этого состояния. В этой статье мы рассмотрим, как проходит вывод из запоя в стационаре клиники «Перекресток Надежды» в Челябинске, а также особенности анонимности, скорости лечения и участия нарколога.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-cena-v-chelyabinske/]вывод из запоя цена в челябинске[/url]
Проблема зависимости от алкоголя, наркотиков и азартных игр остается одной из наиболее острых в современном обществе. Эти состояния оказывают значительное воздействие не только на здоровье самого человека, но и на его семью, друзей и общественные связи. Наркологическая клиника “Восстановление души” предлагает широкий спектр услуг для тех, кто борется с различными формами зависимости, такими как алкоголизм, наркомания и игромания. Наша цель — предоставить комплексный подход к лечению, что обеспечивает высокие показатели успешности среди наших пациентов.
Исследовать вопрос подробнее – [url=https://kapelnica-ot-zapoya-irkutsk.ru/]срочная капельница от запоя на дому[/url]
Зависимость — это серьёзная проблема, которая затрагивает не только человека, но и его семью и окружение. Алкоголизм, наркомания и другие виды зависимостей могут разрушить жизнь, но с профессиональной помощью можно вернуть её в русло нормального функционирования. Наркологическая клиника «Заря Будущего» в Санкт-Петербурге предлагает уникальные программы лечения зависимостей, которые сочетает медико-психологический подход, индивидуальные методики и квалифицированную поддержку.
Выяснить больше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]вывод из запоя в стационаре анонимно в санкт-петербурге[/url]
Запой нарушает привычный ритм жизни, вызывая серьезные физические и эмоциональные проблемы. Для его лечения требуется не только медикаментозное вмешательство, но и постоянное наблюдение специалистов. Стационарное лечение в клинике позволяет обеспечить полный контроль над состоянием пациента, что минимизирует риски и способствует более эффективной реабилитации.
Выяснить больше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]vyvod iz zapoya na domu cena sankt-peterburg[/url]
В современном мире зависимость становится все более распространенной проблемой. Стресс, жизненные трудности и финансовые проблемы часто провоцируют развитие таких серьезных состояний, как алкоголизм, наркомания и игромания. Наркологическая клиника “Второй Шанс” предоставляет помощь людям, столкнувшимся с этими заболеваниями. Основное направление работы клиники — восстановление здоровья пациентов, их социальная адаптация и предотвращение повторных рецидивов.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]наркология вывод из запоя на дому [/url]
Запой – это тяжелое состояние, возникающее из-за длительного и неконтролируемого употребления алкоголя. Оно характеризуется физической и психологической зависимостью, что приводит к серьезным последствиям для организма и психики. Для восстановления нормального самочувствия и здоровья необходимо профессиональное лечение. Одним из наиболее удобных и эффективных методов является вывод из запоя на дому.
Подробнее – [url=https://vyvod-iz-zapoya-13.ru/]вывод из запоя с выездом[/url]
Процесс вывода из запоя требует быстрого реагирования. Запой, особенно в его тяжелых формах, представляет угрозу не только для психического состояния пациента, но и для его физического здоровья. Чтобы минимизировать риски и предотвратить осложнения, важно начинать лечение как можно скорее. Клинике «Освобождение» в Рязани доступна круглосуточная помощь — мы работаем 24/7, чтобы оперативно вмешаться в любой момент, когда это необходимо. Врачебная помощь может быть предоставлена как в стационаре клиники, так и на дому, в зависимости от состояния пациента и его предпочтений.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-v-stacionare-v-ryazani/]вывод из запоя в стационаре анонимно [/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]вывод из запоя на дому недорого воронеж[/url]
В клинике «Заря Будущего» мы понимаем, насколько важен своевременный и профессиональный подход к лечению зависимости. Наши специалисты готовы оказать квалифицированную помощь прямо у вас дома, обеспечивая полную безопасность и конфиденциальность на каждом этапе лечения.
Углубиться в тему – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]вывод из запоя в стационаре в санкт-петербурге[/url]
Наркологическая клиника «Освобождение» в Рязани предлагает профессиональные услуги по выводу из запоя и комплексной реабилитации. Мы понимаем, что зависимость — это болезнь, требующая индивидуального подхода и поддержки не только для пациента, но и для его близких.
Выяснить больше – [url=https://vyvod-iz-zapoya-13.ru/]наркологическая клиника рязань[/url]
Злоупотребление алкоголем в течение продолжительного времени приводит к состоянию, при котором человек теряет контроль над собой, а его организм подвергается серьезным нагрузкам. Последствия такого состояния проявляются на физическом и психическом уровнях: организм страдает от отравления, обезвоживания и сбоев в работе нервной системы, а человек теряет способность адекватно оценивать ситуацию. Выйти из этого состояния без медицинской помощи крайне сложно, так как требуется не только прекратить употребление алкоголя, но и восстановить нормальные функции организма.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-cena-v-krasnodare/]narkolog vyvod iz zapoya cena v-krasnodare[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьёзное воздействие этанола. Это приводит к нарушениям нормальной физиологической деятельности и ухудшению состояния здоровья. Анонимный вывод из запоя — это медицинская помощь, направленная на устранение последствий чрезмерного употребления алкоголя с соблюдением строгой конфиденциальности.
Углубиться в тему – [url=https://vyvod-iz-zapoya-20.ru/]наркологическая клиника в екатеринбурге[/url]
Запой представляет собой состояние, характеризующееся длительным непрерывным употреблением алкоголя, что приводит к физической и психической зависимости. Этот процесс может длиться от нескольких дней до недель. Алкогольные напитки негативно влияют на функционирование внутренних органов, вызывая серьёзные нарушения, включая алкоголизм. При необходимости вывода из запоя важно обратиться за медицинской помощью, что можно осуществить на дому. В данной статье рассмотрим ключевые аспекты данной процедуры.
Углубиться в тему – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]наркология вывод из запоя на дому [/url]
Одной из распространенных проблем является запой, состояние, при котором человек длительное время бесконтрольно употребляет алкоголь, что приводит к тяжелой физической и психологической зависимости. Такое поведение чревато серьезными последствиями для здоровья, включая поражение внутренних органов, нервные расстройства и другие заболевания. Важно своевременно обратиться за профессиональной помощью, чтобы минимизировать риски и предотвратить осложнения.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-14.ru/]vyvod iz zapoya s vyezdom v-sankt-peterburge[/url]
Вывод из запойного состояния – сложный процесс, включающий несколько последовательных этапов. Основная цель – детоксикация организма и восстановление его нормального функционирования. Важно, чтобы процессом руководили опытные специалисты, способные минимизировать последствия интоксикации и помочь человеку вернуться к здоровой жизни. Стоимость лечения зависит от выбранных методов и уровня медицинского обслуживания, а в клинике «Излечение» предлагаются доступные варианты с учетом индивидуальных потребностей пациентов.
Подробнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя вызов на дом [/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Подробнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]быстрый вывод из запоя в стационаре [/url]
Одним из главных преимуществ стационарного лечения в нашей клинике является полная анонимность. Мы понимаем, что многие пациенты боятся раскрытия своей зависимости и осуждения со стороны окружающих. В “Восстановление души” мы гарантируем конфиденциальность всех процедур и защиту личных данных пациента. Это создает безопасную и доверительную атмосферу, необходимую для успешного лечения и психологического восстановления.
Выяснить больше – [url=https://kapelnica-ot-zapoya-irkutsk3.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-irkutske/]https://www.kapelnica-ot-zapoya-irkutsk3.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-irkutske[/url]
Вывод из запойного состояния – сложный процесс, включающий несколько последовательных этапов. Основная цель – детоксикация организма и восстановление его нормального функционирования. Важно, чтобы процессом руководили опытные специалисты, способные минимизировать последствия интоксикации и помочь человеку вернуться к здоровой жизни. Стоимость лечения зависит от выбранных методов и уровня медицинского обслуживания, а в клинике «Излечение» предлагаются доступные варианты с учетом индивидуальных потребностей пациентов.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-16.ru/]narkologicheskaya klinika[/url]
После успешной детоксикации начинается этап реабилитации, который включает восстановление социального статуса пациента и формирование новых здоровых привычек. Мы предлагаем как групповые, так и индивидуальные занятия, направленные на изменение поведения и мышления, что способствует долгосрочному удержанию пациента от возвращения к зависимостям.
Углубиться в тему – [url=https://kapelnica-ot-zapoya-moskva3.ru/kapelnica-ot-zapoya-na-domu-v-moskve/]anonimnaya kapelnica ot zapoya na domu moskva[/url]
Запой представляет собой состояние, связанное с длительным употреблением алкогольных напитков, что приводит к значительным физическим и психическим расстройствам. Это явление формирует зависимость, оказывая негативное воздействие на здоровье. Вывод из запоя в стационаре становится необходимостью для восстановительных мероприятий, направленных на реабилитацию пациента. В этой статье рассматриваются аспекты анонимного вывода, скорость процедур, а также роль нарколога в процессе лечения.
Узнать больше – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]срочный вывод из запоя на дому краснодар[/url]
Купить Хавейл – только у нас вы найдете цены ниже рынка. Быстрей всего сделать заказ на хавал джулиан купить в уфе можно только у нас!
[url=https://jolion-ufa1.ru]хавал джолион купить в уфе[/url]
новый хавал джолион цена – [url=http://www.jolion-ufa1.ru/]http://www.jolion-ufa1.ru/[/url]
Алкоголизм — это хроническое заболевание, которое характеризуется патологической зависимостью от этанола. Периоды запоя, когда человек неконтролируемо потребляет алкоголь, могут привести к разнообразным медицинским осложнениям, как физическим, так и психологическим. Запой становится не только причиной ухудшения здоровья, но и причиной множества социальных и личных проблем. На этом фоне возникает необходимость вывода из запоя, процесс, который должен учитывать индивидуальные особенности пациента. Важно, что анонимность в реабилитации играет ключевую роль, помогая избежать стигматизации и увеличивая шансы на успешное восстановление.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-v-kruglosutochno-v-ryazani/]вывод из запоя круглосуточно рязань[/url]
Запой – это состояние, которое возникает вследствие длительного употребления алкоголя, приводящее к потере контроля над количеством выпиваемого и формированию как физической, так и психологической зависимости. Оно сопровождается серьёзными последствиями для здоровья и требует немедленного вмешательства.
Углубиться в тему – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]srochny vyvod iz zapoya na domu v-voronezhe[/url]
Зависимость — это серьёзная проблема, которая затрагивает не только человека, но и его семью и окружение. Алкоголизм, наркомания и другие виды зависимостей могут разрушить жизнь, но с профессиональной помощью можно вернуть её в русло нормального функционирования. Наркологическая клиника «Заря Будущего» в Санкт-Петербурге предлагает уникальные программы лечения зависимостей, которые сочетает медико-психологический подход, индивидуальные методики и квалифицированную поддержку.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя на дому недорого [/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя с выездом на дом челябинск[/url]
[url=https://rustud.com/catalog/recenziya]Купить написание рецензии недорого[/url] – заказать доклад с презентацией, написать отчет по практике заказать
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Подробнее тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-anonimno-v-voronezhe/]vyvod iz zapoya anonimno voronezh[/url]
Запой представляет собой состояние, характеризующееся длительным непрерывным употреблением алкоголя, что приводит к физической и психической зависимости. Этот процесс может длиться от нескольких дней до недель. Алкогольные напитки негативно влияют на функционирование внутренних органов, вызывая серьёзные нарушения, включая алкоголизм. При необходимости вывода из запоя важно обратиться за медицинской помощью, что можно осуществить на дому. В данной статье рассмотрим ключевые аспекты данной процедуры.
Подробнее тут – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]narkolog na dom vyvod iz zapoya chelyabinsk[/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Разобраться лучше – [url=https://vyvod-iz-zapoya-19.ru/]vyvod iz zapoya s vyezdom[/url]
Проблема зависимости от алкоголя, наркотиков и азартных игр остается одной из наиболее острых в современном обществе. Эти состояния оказывают значительное воздействие не только на здоровье самого человека, но и на его семью, друзей и общественные связи. Наркологическая клиника “Восстановление души” предлагает широкий спектр услуг для тех, кто борется с различными формами зависимости, такими как алкоголизм, наркомания и игромания. Наша цель — предоставить комплексный подход к лечению, что обеспечивает высокие показатели успешности среди наших пациентов.
Подробнее – [url=https://kapelnica-ot-zapoya-voronezh2.ru/kapelnica-ot-zapoya-v-stacionare-v-voronezhe/]капельница от запоя на дому цена воронеж[/url]
Вывод из запойного состояния – сложный процесс, включающий несколько последовательных этапов. Основная цель – детоксикация организма и восстановление его нормального функционирования. Важно, чтобы процессом руководили опытные специалисты, способные минимизировать последствия интоксикации и помочь человеку вернуться к здоровой жизни. Стоимость лечения зависит от выбранных методов и уровня медицинского обслуживания, а в клинике «Излечение» предлагаются доступные варианты с учетом индивидуальных потребностей пациентов.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-16.ru/]vyvod iz zapoya s vyezdom v-krasnodare[/url]
Круглосуточная медицинская помощь при алкогольном запое играет важнейшую роль в спасении жизни пациентов и их возвращении к нормальному состоянию. В данной статье рассмотрим ключевые аспекты вывода из запоя в домашних условиях, срочной помощи и работы нарколога.
Получить больше информации – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]вывод из запоя на дому недорого капельница [/url]
Запой – это состояние, возникающее из-за длительного употребления алкоголя и характеризующееся физической и психологической зависимостью. Оно требует комплексного медицинского вмешательства для устранения последствий и предотвращения дальнейшего ухудшения здоровья. Стационарное лечение в клинике «Освобождение» в Рязани – это эффективный способ вернуть пациента к нормальной жизни, предоставляя ему комфортные условия и круглосуточную помощь специалистов.
Узнать больше – [url=https://vyvod-iz-zapoya-13.ru/]наркологический центр рязань[/url]
Зависимость — это серьёзная проблема, которая затрагивает не только человека, но и его семью и окружение. Алкоголизм, наркомания и другие виды зависимостей могут разрушить жизнь, но с профессиональной помощью можно вернуть её в русло нормального функционирования. Наркологическая клиника «Заря Будущего» в Санкт-Петербурге предлагает уникальные программы лечения зависимостей, которые сочетает медико-психологический подход, индивидуальные методики и квалифицированную поддержку.
Углубиться в тему – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]быстрый вывод из запоя в стационаре [/url]
Запой нарушает привычный ритм жизни, вызывая серьезные физические и эмоциональные проблемы. Для его лечения требуется не только медикаментозное вмешательство, но и постоянное наблюдение специалистов. Стационарное лечение в клинике позволяет обеспечить полный контроль над состоянием пациента, что минимизирует риски и способствует более эффективной реабилитации.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]vyvod iz zapoya srochno kruglosutochno sankt-peterburg[/url]
Алкогольный запой — это серьезное заболевание, которое требует немедленного и профессионального вмешательства. Запой сопровождается не только физическими страданиями, но и эмоциональными трудностями. Процесс выведения из запоя требует срочного внимания, так как состояние пациента может ухудшиться, если не получить квалифицированную помощь вовремя. Наркологическая клиника «Излечение» в Краснодаре предоставляет круглосуточную помощь при алкогольном запое, чтобы восстановить здоровье пациента в самых сложных ситуациях.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-cena-v-krasnodare/]вывод из запоя на дому цена в краснодаре[/url]
Вывод из запойного состояния – сложный процесс, включающий несколько последовательных этапов. Основная цель – детоксикация организма и восстановление его нормального функционирования. Важно, чтобы процессом руководили опытные специалисты, способные минимизировать последствия интоксикации и помочь человеку вернуться к здоровой жизни. Стоимость лечения зависит от выбранных методов и уровня медицинского обслуживания, а в клинике «Излечение» предлагаются доступные варианты с учетом индивидуальных потребностей пациентов.
Узнать больше – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]vyvod iz zapoya na domu nedorogo kapelnica krasnodar[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]вывод из запоя цена наркология санкт-петербург[/url]
В клинике «Излечение» мы предлагаем широкий спектр услуг по выводу из запоя, включая как стационарное лечение, так и помощь на дому. В этой статье мы подробно рассмотрим, от чего зависит цена вывода из запоя и какие услуги входят в стоимость.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя на дому круглосуточно [/url]
Наши наркологи приезжают к пациенту в удобное для него время и проводят необходимую терапию. Капельница от запоя помогает:
Получить дополнительные сведения – [url=https://kapelnica-ot-zapoya-moskva1.ru/kapelnica-ot-zapoya-cena-v-moskve/]капельница от запоя круглосуточно в москве[/url]
Запой нарушает привычный ритм жизни, вызывая серьезные физические и эмоциональные проблемы. Для его лечения требуется не только медикаментозное вмешательство, но и постоянное наблюдение специалистов. Стационарное лечение в клинике позволяет обеспечить полный контроль над состоянием пациента, что минимизирует риски и способствует более эффективной реабилитации.
Разобраться лучше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya kapelnica na domu sankt-peterburg[/url]
Алкогольный запой представляет собой серьезное состояние, которое требует немедленного медицинского вмешательства. Это состояние характеризуется длительным и бесконтрольным потреблением алкоголя, что приводит к разрушению как физического, так и психоэмоционального здоровья человека. Для эффективного и безопасного вывода из запоя стационарное лечение является оптимальным решением, особенно при тяжелых случаях интоксикации. В стационаре клиники «Заря Будущего» мы предлагаем комплексную программу вывода из запоя, которая включает детоксикацию, восстановление организма и психотерапевтическую помощь.
Узнать больше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu v-sankt-peterburge[/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-cena-v-chelyabinske/]вывод из запоя цена в челябинске[/url]
В таких ситуациях вывод из запоя на дому становится оптимальным решением, особенно если пациент не может или не хочет посещать стационар. Эта услуга позволяет получить медицинскую помощь в комфортной и привычной обстановке, что снижает уровень стресса и ускоряет процесс восстановления.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]vyvod iz zapoya na domu nedorogo kapelnica [/url]
Алкогольный запой представляет собой серьезное состояние, которое требует немедленного медицинского вмешательства. Это состояние характеризуется длительным и бесконтрольным потреблением алкоголя, что приводит к разрушению как физического, так и психоэмоционального здоровья человека. Для эффективного и безопасного вывода из запоя стационарное лечение является оптимальным решением, особенно при тяжелых случаях интоксикации. В стационаре клиники «Заря Будущего» мы предлагаем комплексную программу вывода из запоя, которая включает детоксикацию, восстановление организма и психотерапевтическую помощь.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]vyvod iz zapoya cena sankt-peterburg[/url]
Алкоголизм — это хроническое прогрессирующее заболевание, связанное с физической и психической зависимостью от этанола. Запой, как его крайнее проявление, представляет собой длительное употребление алкоголя, сопровождающееся тяжелыми нарушениями работы организма, включая абстинентный синдром и потенциальные угрозы для жизни. Лечение запоя требует комплексного подхода, включающего медицинские процедуры, психотерапию и реабилитационные меры.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-cena-v-ekaterinburge/]нарколог вывод из запоя цена в екатеринбурге[/url]
Процедура вывода из запоя направлена на устранение последствий длительного употребления алкоголя и восстановления работы организма. Этот процесс включает не только медикаментозное лечение, но и психологическую поддержку, что позволяет минимизировать симптомы абстиненции и предотвратить возможные осложнения.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]vyvod iz zapoya kruglosutochno sankt-peterburg[/url]
I had no idea how to set up Metamask on Chrome, but https://sites.google.com/view/metamask-extension-download-oa/chrome explained everything in detail. The instructions are easy to understand, making it perfect for beginners like me!
Запой представляет собой состояние, характеризующееся длительным непрерывным употреблением алкоголя, что приводит к физической и психической зависимости. Этот процесс может длиться от нескольких дней до недель. Алкогольные напитки негативно влияют на функционирование внутренних органов, вызывая серьёзные нарушения, включая алкоголизм. При необходимости вывода из запоя важно обратиться за медицинской помощью, что можно осуществить на дому. В данной статье рассмотрим ключевые аспекты данной процедуры.
Детальнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя на дому круглосуточно [/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьезное воздействие этанола, что приводит к нарушению нормальной физиологической деятельности. Это состояние сопровождается сильными физическими и психологическими расстройствами, которые требуют профессионального вмешательства. Однако многие люди, страдающие алкогольной зависимостью, сталкиваются с трудностью признания своей проблемы, опасаясь утраты репутации и конфиденциальности. В клинике «Заря Будущего» мы предлагаем анонимный вывод из запоя в Санкт-Петербурге, предоставляя высококачественное лечение с гарантией сохранения личной информации.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]быстрый вывод из запоя в стационаре санкт-петербург[/url]
Запой нарушает привычный ритм жизни, вызывая серьезные физические и эмоциональные проблемы. Для его лечения требуется не только медикаментозное вмешательство, но и постоянное наблюдение специалистов. Стационарное лечение в клинике позволяет обеспечить полный контроль над состоянием пациента, что минимизирует риски и способствует более эффективной реабилитации.
Углубиться в тему – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя врач на дом [/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьёзное воздействие этанола. Это приводит к нарушениям нормальной физиологической деятельности и ухудшению состояния здоровья. Анонимный вывод из запоя — это медицинская помощь, направленная на устранение последствий чрезмерного употребления алкоголя с соблюдением строгой конфиденциальности.
Выяснить больше – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]вывод из запоя на дому недорого [/url]
Клиника “Второй Шанс” в Санкт-Петербурге предлагает услугу вывода из запоя, как в стационаре, так и на дому. Для пациентов, не желающих посещать клинику, квалифицированные врачи готовы предоставить помощь в комфортных домашних условиях. Используя современные методы, специалисты обеспечивают безопасность, анонимность и высокий уровень медицинской поддержки.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]анонимный вывод из запоя на дому в санкт-петербурге[/url]
Процесс вывода из запоя требует быстрого реагирования. Запой, особенно в его тяжелых формах, представляет угрозу не только для психического состояния пациента, но и для его физического здоровья. Чтобы минимизировать риски и предотвратить осложнения, важно начинать лечение как можно скорее. Клинике «Освобождение» в Рязани доступна круглосуточная помощь — мы работаем 24/7, чтобы оперативно вмешаться в любой момент, когда это необходимо. Врачебная помощь может быть предоставлена как в стационаре клиники, так и на дому, в зависимости от состояния пациента и его предпочтений.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]vyvod iz zapoya s vyezdom na dom ryazan[/url]
В этой статье мы расскажем о возможностях клиники для лечения алкогольной интоксикации круглосуточно, включая вывод из запоя в стационаре и на дому, а также о роли профессионалов, таких как нарколог, в процессе лечения.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]vyvod iz zapoya vrach na dom [/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Выяснить больше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]vyvod iz zapoya cena [/url]
Мы понимаем, что каждая зависимость уникальна, поэтому основой нашего подхода является индивидуализация лечения. После тщательной диагностики, включающей анализ медицинской истории, психологического состояния и социальных факторов, наши специалисты разрабатывают персонализированный план терапии. Этот план может включать медикаментозную детоксикацию, психотерапевтические методы и социальные программы, направленные на изменение поведения и мышления пациента.
Углубиться в тему – [url=https://kapelnica-ot-zapoya-moskva3.ru/kapelnica-ot-zapoya-v-stacionare-v-moskve/]kapelnica ot zapoya na domu kruglosutochno v moskve[/url]
Алкогольная зависимость — это серьезная проблема, требующая незамедлительного вмешательства, чтобы избежать тяжелых последствий для здоровья. Запойное пьянство может привести к различным заболеваниям, таким как цирроз печени, панкреатит и другие опасные состояния. К счастью, современная медицина предлагает эффективные методы решения этой проблемы. Для тех, кто не хочет или не может посетить стационар, есть возможность пройти процедуру капельницы от запоя на дому в Твери.
Выяснить больше – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]vyvod iz zapoya na domu nedorogo [/url]
Одной из распространенных проблем является запой, состояние, при котором человек длительное время бесконтрольно употребляет алкоголь, что приводит к тяжелой физической и психологической зависимости. Такое поведение чревато серьезными последствиями для здоровья, включая поражение внутренних органов, нервные расстройства и другие заболевания. Важно своевременно обратиться за профессиональной помощью, чтобы минимизировать риски и предотвратить осложнения.
Подробнее тут – [url=https://vyvod-iz-zapoya-14.ru/]http://www.vyvod-iz-zapoya-14.ru[/url]
В клинике «Заря Будущего» мы понимаем, насколько важен своевременный и профессиональный подход к лечению зависимости. Наши специалисты готовы оказать квалифицированную помощь прямо у вас дома, обеспечивая полную безопасность и конфиденциальность на каждом этапе лечения.
Детальнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]вывод из запоя с выездом цена в санкт-петербурге[/url]
Запой – это тяжелое состояние, возникающее из-за длительного и неконтролируемого употребления алкоголя. Оно характеризуется физической и психологической зависимостью, что приводит к серьезным последствиям для организма и психики. Для восстановления нормального самочувствия и здоровья необходимо профессиональное лечение. Одним из наиболее удобных и эффективных методов является вывод из запоя на дому.
Разобраться лучше – [url=https://vyvod-iz-zapoya-13.ru/]http://www.vyvod-iz-zapoya-13.ru[/url]
Выход из запоя — это важнейший этап на пути к избавлению от алкогольной зависимости. Он требует комплексного подхода, профессиональной помощи и сильной мотивации пациента. В условиях, когда анонимность и комфорт становятся ключевыми факторами для многих людей, вывод из запоя на дому приобретает все большее значение. Это решение позволяет человеку получить необходимую помощь, не покидая дома, и избежать стресса, связанного с госпитализацией.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-16.ru/]вывод из запоя капельница[/url]
Запой — это опасное состояние, которое развивается у людей с алкогольной зависимостью и характеризуется длительным непрерывным потреблением алкоголя. Это состояние приводит к серьезным нарушениям в организме, как физическим, так и психоэмоциональным. Длительный запой может вызвать множество осложнений, таких как поражение внутренних органов, нервной системы, а также проблемы в социальной и семейной жизни. Однако современная медицина предоставляет эффективные методы вывода из запоя, и одним из наиболее удобных и востребованных является вывод из запоя на дому.
Подробнее тут – [url=https://vyvod-iz-zapoya-19.ru/]narkologicheskaya klinika v-sankt-peterburge[/url]
Процедура вывода из запоя направлена на устранение последствий длительного употребления алкоголя и восстановления работы организма. Этот процесс включает не только медикаментозное лечение, но и психологическую поддержку, что позволяет минимизировать симптомы абстиненции и предотвратить возможные осложнения.
Получить больше информации – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]вывод из запоя круглосуточно наркология [/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает сильное воздействие этанола, что приводит к нарушению нормального функционирования физиологических систем. Это состояние может быть опасным и требует квалифицированной медицинской помощи. Вывод из запоя — важная медицинская процедура, направленная на устранение последствий чрезмерного употребления алкоголя и восстановление здоровья пациента.
Подробнее тут – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]наркология вывод из запоя на дому [/url]
Процесс возвращения к нормальной жизни после длительного употребления алкоголя играет ключевую роль в избавлении от зависимости. Медицинское сопровождение должно сочетаться с осознанным желанием человека изменить свою жизнь. Важно, чтобы лечение проходило в условиях, исключающих стресс и дискомфорт. Для этого предусмотрены как стационарные программы, так и возможность получить медицинскую помощь на дому. Такой вариант подходит тем, кто предпочитает находиться в привычной обстановке и избегать огласки.
Углубиться в тему – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]vyvod iz zapoya vyzov na dom [/url]
В некоторых ситуациях, когда запой длится несколько дней или приводит к серьезным последствиям для здоровья, необходим срочный вызов нарколога. Среди признаков, при которых требуется неотложная помощь:
Подробнее – [url=https://kapelnica-ot-zapoya-moskva1.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-moskve/]narkolog kapelnica ot zapoya cena[/url]
[url=https://avtoshkola-car.ru]Онлайн изучение теории на права[/url] – Категория А мотоцикл Красноярск, Записать в автошколу красноярска онлайн
Алкогольная зависимость представляет собой актуальную медицинскую проблему, требующую тщательного подхода. Запой характеризуется длительным, непрерывным употреблением спиртных напитков, что ведёт к физической и психической зависимости. На фоне запойного состояния могут возникнуть тяжёлые нарушения функций органов, увеличивается риск развития таких состояний, как алкогольный делирий. Вывод из запоя становится ключевым этапом в лечении зависимостей, который может осуществляться как в стационарных условиях, так и на дому, в зависимости от состояния пациента. В клинике «Перекресток Надежды» в Челябинске мы предлагаем круглосуточную помощь, чтобы обеспечить максимально эффективное и безопасное лечение при любом варианте вывода из запоя.
Узнать больше – [url=https://vyvod-iz-zapoya-17.ru/]вывод из запоя в челябинске[/url]
В клинике также уделяется внимание конфиденциальности лечения. Понимая деликатность проблемы, врачи гарантируют полную анонимность на всех этапах терапии. Благодаря профессиональному подходу и использованию проверенных методик пациенты могут восстановить здоровье и вернуться к полноценной жизни.
Выяснить больше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]vyvod iz zapoya kruglosutochno sankt-peterburg[/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Получить больше информации – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-kruglosutochno-v-voronezhe/]вывод из запоя срочно круглосуточно воронеж[/url]
В клинике «Излечение» мы предлагаем широкий спектр услуг по выводу из запоя, включая как стационарное лечение, так и помощь на дому. В этой статье мы подробно рассмотрим, от чего зависит цена вывода из запоя и какие услуги входят в стоимость.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-cena-v-krasnodare/]vyvod iz zapoya cena narkologiya [/url]
Зависимость от алкоголя – серьезная проблема, требующая системного подхода и профессионального лечения. В клинике «Излечение» гарантируется полная конфиденциальность на всех этапах процесса, что позволяет пациентам чувствовать себя в безопасности. Лечение может проходить как в стационаре, так и на дому, при этом соблюдаются все условия для максимального комфорта.
Детальнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]vyvod iz zapoya anonimno [/url]
Запой – это состояние, возникающее из-за длительного употребления алкоголя и характеризующееся физической и психологической зависимостью. Оно требует комплексного медицинского вмешательства для устранения последствий и предотвращения дальнейшего ухудшения здоровья. Стационарное лечение в клинике «Освобождение» в Рязани – это эффективный способ вернуть пациента к нормальной жизни, предоставляя ему комфортные условия и круглосуточную помощь специалистов.
Подробнее тут – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]narkologiya vyvod iz zapoya na domu ryazan[/url]
Поддержка — ключевой элемент на пути к выздоровлению. Мы предлагаем программы, которые продолжаются даже после завершения основного курса лечения. Пациенты имеют возможность участвовать в регулярных встречах с психологами и наркологами, где они могут делиться своими успехами и получать необходимую помощь.
Получить больше информации – [url=https://kapelnica-ot-zapoya-moskva2.ru/kapelnica-ot-zapoya-na-domu-v-moskve/]kapelnica ot zapoya v moskve[/url]
Запойное состояние представляет собой серьёзную угрозу для здоровья, поскольку сопровождается тяжёлой интоксикацией организма и нарушением психоэмоционального состояния. Решение о лечении в стационаре клиники «Союз Здоровья» позволяет справиться с последствиями длительного употребления алкоголя, обеспечивая пациентам полный комплекс медицинской и психологической помощи.
Разобраться лучше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]vyvod iz zapoya cena narkologiya voronezh[/url]
Запой — это состояние, при котором человек не может прекратить употребление алкоголя на протяжении длительного времени, что вызывает физическую и психологическую зависимость. Это крайне опасное состояние, требующее немедленного вмешательства специалистов. Наркологическая клиника «Преображение» в Екатеринбурге предоставляет профессиональную помощь в лечении запоя, используя самые эффективные и безопасные методы.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]vyvod iz zapoya na domu nedorogo kapelnica ekaterinburg[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]срочный вывод из запоя на дому [/url]
Алкогольная интоксикация, или запой, представляет собой крайне опасное состояние, при котором организм страдает от токсического воздействия алкоголя. Это ведет к нарушению нормальных физиологических процессов и ухудшению общего состояния здоровья. Капельница от запоя — это медицинская процедура, направленная на выведение токсинов из организма и восстановление нормальной функции организма при соблюдении полной конфиденциальности.
Узнать больше – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-v-kruglosutochno-v-tveri/]вывод из запоя круглосуточно тверь[/url]
Алкогольный запой — это тяжелое состояние, при котором человек теряет контроль над количеством потребляемого алкоголя. Это приводит к сильной интоксикации, сбоям в работе органов и систем организма, а также серьезным психоэмоциональным расстройствам. Без должной медицинской помощи запой может вызвать ряд опасных последствий, включая алкогольный делирий и угрозу жизни. Поэтому при первых признаках запоя необходимо обратиться к профессионалам.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-cena-v-tveri/]vyvod iz zapoya s vyezdom cena v-tveri[/url]
Алкогольный запой — это серьезное заболевание, которое требует немедленного и профессионального вмешательства. Запой сопровождается не только физическими страданиями, но и эмоциональными трудностями. Процесс выведения из запоя требует срочного внимания, так как состояние пациента может ухудшиться, если не получить квалифицированную помощь вовремя. Наркологическая клиника «Излечение» в Краснодаре предоставляет круглосуточную помощь при алкогольном запое, чтобы восстановить здоровье пациента в самых сложных ситуациях.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-stacionare-v-krasnodare/]вывод из запоя в стационаре [/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает сильное воздействие этанола, что приводит к нарушению нормального функционирования физиологических систем. Это состояние может быть опасным и требует квалифицированной медицинской помощи. Вывод из запоя — важная медицинская процедура, направленная на устранение последствий чрезмерного употребления алкоголя и восстановление здоровья пациента.
Разобраться лучше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-v-kruglosutochno-v-chelyabinske/]vyvod iz zapoya kruglosutochno chelyabinsk[/url]
Долговечность и надежность дизайнерской мебели премиум-класса.
Дизайнерская мебель премиум-класса [url=http://www.byfurniture.by/]http://www.byfurniture.by/[/url] .
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Детальнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu kruglosutochno sankt-peterburg[/url]
Алкоголизм — это хроническое прогрессирующее заболевание, связанное с физической и психической зависимостью от этанола. Запой, как его крайнее проявление, представляет собой длительное употребление алкоголя, сопровождающееся тяжелыми нарушениями работы организма, включая абстинентный синдром и потенциальные угрозы для жизни. Лечение запоя требует комплексного подхода, включающего медицинские процедуры, психотерапию и реабилитационные меры.
Детальнее – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]vyvod iz zapoya na domu kruglosutochno v-ekaterinburge[/url]
Одним из главных преимуществ стационарного лечения в нашей клинике является полная анонимность. Мы понимаем, что многие пациенты боятся раскрытия своей зависимости и осуждения со стороны окружающих. В “Восстановление души” мы гарантируем конфиденциальность всех процедур и защиту личных данных пациента. Это создает безопасную и доверительную атмосферу, необходимую для успешного лечения и психологического восстановления.
Разобраться лучше – [url=https://kapelnica-ot-zapoya-voronezh2.ru/kapelnica-ot-zapoya-cena-v-voronezhe/]kapelnica ot zapoya na domu kruglosutochno[/url]
Если затянувшееся употребление алкоголя не прерывается, организм начинает испытывать тяжелые последствия. Возникает абстинентный синдром, проявляющийся в виде тошноты, сильной головной боли, дрожи в теле и других неприятных симптомов. Без своевременной помощи состояние может ухудшиться, поэтому важна быстрая медицинская реакция. Комплексное лечение направлено не только на избавление от физической зависимости, но и на поддержку человека в эмоциональном плане. В случаях, когда требуется постоянное наблюдение, рекомендуется стационарная терапия.
Выяснить больше – [url=https://vyvod-iz-zapoya-16.ru/]наркологическая клиника[/url]
Проблема зависимостей остаётся актуальной в современном обществе. С каждым годом увеличивается число людей, страдающих от алкоголизма, наркомании и других форм зависимостей, что негативно отражается на их жизни и благополучии близких. Зависимость — это не просто физическое заболевание, но и глубокая психологическая проблема. Для эффективного лечения требуется помощь профессионалов, способных обеспечить комплексный подход.
Детальнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]narkologiya vyvod iz zapoya na domu [/url]
Одним из важнейших аспектов, который помогает пациентам справиться с алкогольной зависимостью, является анонимность в процессе лечения. В клинике «Излечение» мы понимаем, как важно сохранить конфиденциальность и гарантировать защиту личной информации, особенно для тех, кто опасается общественного осуждения. Мы обеспечиваем полную анонимность при выводе из запоя, как в стационарных условиях, так и на дому.
Детальнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-stacionare-v-krasnodare/]vyvod iz zapoya v stacionare anonimno v-krasnodare[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьезное воздействие этанола, что приводит к нарушению нормальной физиологической деятельности. Это состояние сопровождается сильными физическими и психологическими расстройствами, которые требуют профессионального вмешательства. Однако многие люди, страдающие алкогольной зависимостью, сталкиваются с трудностью признания своей проблемы, опасаясь утраты репутации и конфиденциальности. В клинике «Заря Будущего» мы предлагаем анонимный вывод из запоя в Санкт-Петербурге, предоставляя высококачественное лечение с гарантией сохранения личной информации.
Подробнее тут – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu nedorogo [/url]
Вывод из запоя представляет собой важный этап в процессе лечения алкогольной зависимости. Это медицинская процедура, необходимая для устранения токсических веществ, накопленных в организме в результате длительного употребления алкоголя. Алкогольная зависимость может вызывать множество проблем, включая физические и психоэмоциональные расстройства. Своевременное вмешательство специалистов помогает минимизировать риски и способствует восстановлению здоровья пациента.
Углубиться в тему – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]vyvod iz zapoya na domu nedorogo kapelnica [/url]
Выход из запоя — это важнейший этап на пути к избавлению от алкогольной зависимости. Он требует комплексного подхода, профессиональной помощи и сильной мотивации пациента. В условиях, когда анонимность и комфорт становятся ключевыми факторами для многих людей, вывод из запоя на дому приобретает все большее значение. Это решение позволяет человеку получить необходимую помощь, не покидая дома, и избежать стресса, связанного с госпитализацией.
Детальнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]вывод из запоя анонимно краснодар[/url]
Алкогольный запой представляет собой критическое состояние, требующее немедленного вмешательства квалифицированных специалистов. Клиника «Союз Здоровья» в Воронеже предлагает круглосуточную помощь, чтобы обеспечить своевременное и эффективное лечение. Наша цель — оперативное снятие интоксикации, устранение симптомов и предотвращение осложнений.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]vyvod iz zapoya s vyezdom na dom voronezh[/url]
[url=https://kra-32-at.ru/]kra30.at[/url] – кракен купить, Kra30.cc
В некоторых ситуациях, когда запой длится несколько дней или приводит к серьезным последствиям для здоровья, необходим срочный вызов нарколога. Среди признаков, при которых требуется неотложная помощь:
Подробнее можно узнать тут – [url=https://kapelnica-ot-zapoya-irkutsk3.ru/kapelnica-ot-zapoya-v-stacionare-v-irkutske/]http://kapelnica-ot-zapoya-irkutsk3.ru/kapelnica-ot-zapoya-v-stacionare-v-irkutske[/url]
Алкогольная зависимость остаётся серьёзной проблемой, требующей квалифицированного медицинского вмешательства. Капельница от запоя — это ключевой этап в процессе лечения, направленный на стабилизацию состояния пациента и его возвращение к полноценной жизни. Профессиональная помощь позволяет эффективно справиться с последствиями длительного употребления алкоголя и восстановить физическое и психологическое здоровье.
Детальнее – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]вывод из запоя на дому тверь[/url]
Запой представляет собой состояние, характеризующееся длительным непрерывным употреблением алкоголя, что приводит к физической и психической зависимости. Этот процесс может длиться от нескольких дней до недель. Алкогольные напитки негативно влияют на функционирование внутренних органов, вызывая серьёзные нарушения, включая алкоголизм. При необходимости вывода из запоя важно обратиться за медицинской помощью, что можно осуществить на дому. В данной статье рассмотрим ключевые аспекты данной процедуры.
Узнать больше – [url=https://vyvod-iz-zapoya-17.ru/]vyvod iz zapoya kapelnica v-chelyabinske[/url]
Запой представляет собой состояние, характеризующееся длительным непрерывным употреблением алкоголя, что приводит к физической и психической зависимости. Этот процесс может длиться от нескольких дней до недель. Алкогольные напитки негативно влияют на функционирование внутренних органов, вызывая серьёзные нарушения, включая алкоголизм. При необходимости вывода из запоя важно обратиться за медицинской помощью, что можно осуществить на дому. В данной статье рассмотрим ключевые аспекты данной процедуры.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-17.ru/]vyvod iz zapoya v-chelyabinske[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает сильное воздействие этанола, что приводит к нарушению нормального функционирования физиологических систем. Это состояние может быть опасным и требует квалифицированной медицинской помощи. Вывод из запоя — важная медицинская процедура, направленная на устранение последствий чрезмерного употребления алкоголя и восстановление здоровья пациента.
Детальнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя на дому круглосуточно [/url]
Запой — это опасное состояние, которое развивается у людей с алкогольной зависимостью и характеризуется длительным непрерывным потреблением алкоголя. Это состояние приводит к серьезным нарушениям в организме, как физическим, так и психоэмоциональным. Длительный запой может вызвать множество осложнений, таких как поражение внутренних органов, нервной системы, а также проблемы в социальной и семейной жизни. Однако современная медицина предоставляет эффективные методы вывода из запоя, и одним из наиболее удобных и востребованных является вывод из запоя на дому.
Подробнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]vyvod iz zapoya kruglosutochno narkologiya sankt-peterburg[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-19.ru/]наркологический центр в санкт-петербурге[/url]
Алкогольный запой — это серьезное заболевание, которое требует немедленного и профессионального вмешательства. Запой сопровождается не только физическими страданиями, но и эмоциональными трудностями. Процесс выведения из запоя требует срочного внимания, так как состояние пациента может ухудшиться, если не получить квалифицированную помощь вовремя. Наркологическая клиника «Излечение» в Краснодаре предоставляет круглосуточную помощь при алкогольном запое, чтобы восстановить здоровье пациента в самых сложных ситуациях.
Разобраться лучше – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя вызов на дом в краснодаре[/url]
Алкогольная зависимость становится всё более распространенной проблемой, требующей внимания как со стороны медицинского сообщества, так и общества в целом. Вывод из запоя — это сложный и ответственный процесс, необходимый для восстановления здоровья пациента и его интеграции в нормальную жизнь. Профессиональное вмешательство позволяет минимизировать последствия длительного употребления алкоголя и нормализовать состояние организма.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]вывод из запоя капельница на дому [/url]
Клиника «Рассвет» в Твери предоставляет услугу анонимного вывода из запоя, обеспечивая высокое качество медицинского обслуживания и строгое соблюдение всех этических норм.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-21.ru/]вывод из запоя[/url]
Поддержка — ключевой элемент на пути к выздоровлению. Мы предлагаем программы, которые продолжаются даже после завершения основного курса лечения. Пациенты имеют возможность участвовать в регулярных встречах с психологами и наркологами, где они могут делиться своими успехами и получать необходимую помощь.
Получить дополнительные сведения – [url=https://kapelnica-ot-zapoya-irkutsk.ru/kapelnica-ot-zapoya-na-domu-v-irkutske/]капельница от запоя на дому цена в иркутске[/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-cena-v-chelyabinske/]вывод из запоя цена [/url]
Проблема зависимостей остаётся актуальной в современном обществе. С каждым годом увеличивается число людей, страдающих от алкоголизма, наркомании и других форм зависимостей, что негативно отражается на их жизни и благополучии близких. Зависимость — это не просто физическое заболевание, но и глубокая психологическая проблема. Для эффективного лечения требуется помощь профессионалов, способных обеспечить комплексный подход.
Получить больше информации – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]вывод из запоя врач на дом воронеж[/url]
После успешной детоксикации начинается этап реабилитации, который включает восстановление социального статуса пациента и формирование новых здоровых привычек. Мы предлагаем как групповые, так и индивидуальные занятия, направленные на изменение поведения и мышления, что способствует долгосрочному удержанию пациента от возвращения к зависимостям.
Детальнее – [url=https://kapelnica-ot-zapoya-moskva2.ru/kapelnica-ot-zapoya-cena-v-moskve/]https://www.kapelnica-ot-zapoya-moskva2.ru/kapelnica-ot-zapoya-cena-v-moskve[/url]
В таких ситуациях вывод из запоя на дому становится оптимальным решением, особенно если пациент не может или не хочет посещать стационар. Эта услуга позволяет получить медицинскую помощь в комфортной и привычной обстановке, что снижает уровень стресса и ускоряет процесс восстановления.
Углубиться в тему – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-anonimno-v-voronezhe/]anonimny vyvod iz zapoya na domu voronezh[/url]
Запой — это опасное состояние, которое развивается у людей с алкогольной зависимостью и характеризуется длительным непрерывным потреблением алкоголя. Это состояние приводит к серьезным нарушениям в организме, как физическим, так и психоэмоциональным. Длительный запой может вызвать множество осложнений, таких как поражение внутренних органов, нервной системы, а также проблемы в социальной и семейной жизни. Однако современная медицина предоставляет эффективные методы вывода из запоя, и одним из наиболее удобных и востребованных является вывод из запоя на дому.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]нарколог вывод из запоя цена санкт-петербург[/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Получить больше информации – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-anonimno-v-voronezhe/]вывод из запоя анонимно [/url]
Алкогольная зависимость становится всё более распространенной проблемой, требующей внимания как со стороны медицинского сообщества, так и общества в целом. Вывод из запоя — это сложный и ответственный процесс, необходимый для восстановления здоровья пациента и его интеграции в нормальную жизнь. Профессиональное вмешательство позволяет минимизировать последствия длительного употребления алкоголя и нормализовать состояние организма.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-v-stacionare-v-ekaterinburge/]vyvod iz zapoya v stacionare [/url]
Если затянувшееся употребление алкоголя не прерывается, организм начинает испытывать тяжелые последствия. Возникает абстинентный синдром, проявляющийся в виде тошноты, сильной головной боли, дрожи в теле и других неприятных симптомов. Без своевременной помощи состояние может ухудшиться, поэтому важна быстрая медицинская реакция. Комплексное лечение направлено не только на избавление от физической зависимости, но и на поддержку человека в эмоциональном плане. В случаях, когда требуется постоянное наблюдение, рекомендуется стационарная терапия.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]vyvod iz zapoya s vyezdom na dom v-krasnodare[/url]
Наркологическая клиника «Освобождение» в Рязани предлагает профессиональные услуги по выводу из запоя и комплексной реабилитации. Мы понимаем, что зависимость — это болезнь, требующая индивидуального подхода и поддержки не только для пациента, но и для его близких.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-v-kruglosutochno-v-ryazani/]вывод из запоя круглосуточно рязань[/url]
Процедура вывода из запоя направлена на устранение последствий длительного употребления алкоголя и восстановления работы организма. Этот процесс включает не только медикаментозное лечение, но и психологическую поддержку, что позволяет минимизировать симптомы абстиненции и предотвратить возможные осложнения.
Углубиться в тему – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]bystry vyvod iz zapoya v stacionare [/url]
Алкогольная зависимость представляет собой сложное и хроническое заболевание, которое влияет не только на физическое, но и на психологическое состояние пациента. Периоды запоя, влекущие за собой неконтролируемое употребление алкоголя, могут привести к опасным последствиям для здоровья и жизни. Абстинентные симптомы, такие как тремор, тошнота, головная боль и другие проявления, требуют немедленного медицинского вмешательства. В таких ситуациях важно не только быстро устранить физическое воздействие алкоголя, но и предоставить пациенту психологическую поддержку.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]вывод из запоя круглосуточно в краснодаре[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя на дому круглосуточно в санкт-петербурге[/url]
Запой представляет собой состояние, характеризующееся длительным непрерывным употреблением алкоголя, что приводит к физической и психической зависимости. Этот процесс может длиться от нескольких дней до недель. Алкогольные напитки негативно влияют на функционирование внутренних органов, вызывая серьёзные нарушения, включая алкоголизм. При необходимости вывода из запоя важно обратиться за медицинской помощью, что можно осуществить на дому. В данной статье рассмотрим ключевые аспекты данной процедуры.
Узнать больше – [url=https://vyvod-iz-zapoya-17.ru/]вывод из запоя капельница челябинск[/url]
Алкогольная зависимость остаётся серьёзной проблемой, требующей квалифицированного медицинского вмешательства. Капельница от запоя — это ключевой этап в процессе лечения, направленный на стабилизацию состояния пациента и его возвращение к полноценной жизни. Профессиональная помощь позволяет эффективно справиться с последствиями длительного употребления алкоголя и восстановить физическое и психологическое здоровье.
Углубиться в тему – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-cena-v-tveri/]вывод из запоя с выездом цена [/url]
В клинике “Восстановление души” работает команда высококвалифицированных специалистов, готовых предложить современное и эффективное лечение зависимостей. Наши врачи-наркологи обладают обширным опытом работы и постоянно совершенствуют свои навыки, чтобы использовать самые передовые методы терапии.
Мы понимаем, что каждая зависимость уникальна, поэтому основой нашего подхода является индивидуализация лечения. После тщательной диагностики, включающей анализ медицинской истории, психологического состояния и социальных факторов, наши специалисты разрабатывают персонализированный план терапии. Этот план может включать медикаментозную детоксикацию, психотерапевтические методы и социальные программы, направленные на изменение поведения и мышления пациента.
Проблема зависимостей остаётся актуальной в современном обществе. С каждым годом увеличивается число людей, страдающих от алкоголизма, наркомании и других форм зависимостей, что негативно отражается на их жизни и благополучии близких. Зависимость — это не просто физическое заболевание, но и глубокая психологическая проблема. Для эффективного лечения требуется помощь профессионалов, способных обеспечить комплексный подход.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-11.ru/]vyvod iz zapoya gorod voronezh[/url]
Зависимость — это хроническое заболевание, требующее долгосрочного лечения и регулярной поддержки. В клинике используют современные научные методы в наркологии и психотерапии, предлагая помощь на всех этапах — от диагностики до реабилитации. Команда профессионалов работает с каждым пациентом индивидуально, обеспечивая комплексный подход к терапии.
Подробнее – [url=https://vyvod-iz-zapoya-14.ru/]вывод из запоя капельница санкт-петербург[/url]
После успешной детоксикации начинается этап реабилитации, который включает восстановление социального статуса пациента и формирование новых здоровых привычек. Мы предлагаем как групповые, так и индивидуальные занятия, направленные на изменение поведения и мышления, что способствует долгосрочному удержанию пациента от возвращения к зависимостям.
Подробнее – [url=https://kapelnica-ot-zapoya-voronezh2.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-voronezhe/]narkologicheskaya klinika voronezh[/url]
Клиника «Преображение» предлагает услуги анонимного вывода из запоя в Екатеринбурге, обеспечивая высокое качество медицинской помощи и соблюдение всех этических норм.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-20.ru/]наркологическая клиника екатеринбург[/url]
Запойное состояние представляет собой серьёзную угрозу для здоровья, поскольку сопровождается тяжёлой интоксикацией организма и нарушением психоэмоционального состояния. Решение о лечении в стационаре клиники «Союз Здоровья» позволяет справиться с последствиями длительного употребления алкоголя, обеспечивая пациентам полный комплекс медицинской и психологической помощи.
Подробнее – [url=https://vyvod-iz-zapoya-11.ru/]http://vyvod-iz-zapoya-11.ru[/url]
Алкогольная зависимость — это серьезное заболевание, при котором человек теряет контроль над потреблением алкоголя, что приводит к его физической и психологической зависимости. Одним из наиболее выраженных проявлений алкоголизма является состояние запоя — длительное и неконтролируемое употребление спиртных напитков. В таких случаях капельница от запоя становится не только быстрым, но и безопасным методом для избавления от последствий интоксикации.
Изучить вопрос глубже – [url=https://kapelnica-ot-zapoya-irkutsk3.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-irkutske/]kapelnica ot zapoya anonimno irkutsk[/url]
Одной из распространенных проблем является запой, состояние, при котором человек длительное время бесконтрольно употребляет алкоголь, что приводит к тяжелой физической и психологической зависимости. Такое поведение чревато серьезными последствиями для здоровья, включая поражение внутренних органов, нервные расстройства и другие заболевания. Важно своевременно обратиться за профессиональной помощью, чтобы минимизировать риски и предотвратить осложнения.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]вывод из запоя анонимно в санкт-петербурге[/url]
Когда человек сталкивается с затяжными алкогольными эпизодами, ему необходима квалифицированная помощь. Специалисты наркологической клиники «Излечение» в Краснодаре разрабатывают индивидуальные методы вывода из этого состояния, создавая комфортные и безопасные условия для пациентов. Длительное употребление спиртного оказывает разрушительное воздействие на внутренние органы, приводит к психическим нарушениям и обостряет хронические болезни. В таких случаях медицинское вмешательство становится неотложной необходимостью.
Получить больше информации – [url=https://vyvod-iz-zapoya-16.ru/]vyvod iz zapoya s vyezdom krasnodar[/url]
Клиника «Рассвет» в Твери предоставляет услугу анонимного вывода из запоя, обеспечивая высокое качество медицинского обслуживания и строгое соблюдение всех этических норм.
Подробнее тут – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-anonimno-v-tveri/]vyvod iz zapoya anonimno v-tveri[/url]
Современное общество сталкивается с серьёзными вызовами, связанными с зависимостями. Алкоголизм, как одна из самых распространённых проблем, оказывает разрушительное воздействие на здоровье человека, разрушая семейные отношения и затрудняя социальную адаптацию. В такие моменты крайне важно обратиться за квалифицированной помощью.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]срочный вывод из запоя на дому рязань[/url]
Запой нарушает привычный ритм жизни, вызывая серьезные физические и эмоциональные проблемы. Для его лечения требуется не только медикаментозное вмешательство, но и постоянное наблюдение специалистов. Стационарное лечение в клинике позволяет обеспечить полный контроль над состоянием пациента, что минимизирует риски и способствует более эффективной реабилитации.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-14.ru/]вывод из запоя капельница санкт-петербург[/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Подробнее – [url=https://vyvod-iz-zapoya-17.ru/]наркологическая клиника в челябинске[/url]
Запой представляет собой состояние, связанное с длительным употреблением алкогольных напитков, что приводит к значительным физическим и психическим расстройствам. Это явление формирует зависимость, оказывая негативное воздействие на здоровье. Вывод из запоя в стационаре становится необходимостью для восстановительных мероприятий, направленных на реабилитацию пациента. В этой статье рассматриваются аспекты анонимного вывода, скорость процедур, а также роль нарколога в процессе лечения.
Подробнее тут – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]vyvod iz zapoya vyzov na dom [/url]
В клинике работает команда квалифицированных специалистов, которые осуществляют вывод из запоя, обеспечивая полный контроль над состоянием пациента и устраняя все последствия длительного употребления алкоголя.
Выяснить больше – [url=https://vyvod-iz-zapoya-20.ru/]вывод из запоя капельница в екатеринбурге[/url]
Клиника «Рассвет» в Твери предоставляет услугу анонимного вывода из запоя, обеспечивая высокое качество медицинского обслуживания и строгое соблюдение всех этических норм.
Разобраться лучше – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]vyvod iz zapoya na domu nedorogo [/url]
[url=https://kra-32-at.ru/]kra32.cc[/url] – kra32, kra28.at
Наркологическая клиника «Освобождение» в Рязани предлагает профессиональные услуги по выводу из запоя и комплексной реабилитации. Мы понимаем, что зависимость — это болезнь, требующая индивидуального подхода и поддержки не только для пациента, но и для его близких.
Подробнее тут – [url=https://vyvod-iz-zapoya-13.ru/]http://www.vyvod-iz-zapoya-13.ru[/url]
Алкогольная зависимость остаётся серьёзной проблемой, требующей квалифицированного медицинского вмешательства. Капельница от запоя — это ключевой этап в процессе лечения, направленный на стабилизацию состояния пациента и его возвращение к полноценной жизни. Профессиональная помощь позволяет эффективно справиться с последствиями длительного употребления алкоголя и восстановить физическое и психологическое здоровье.
Подробнее тут – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]вывод из запоя врач на дом тверь[/url]
Проблема зависимостей остаётся актуальной в современном обществе. С каждым годом увеличивается число людей, страдающих от алкоголизма, наркомании и других форм зависимостей, что негативно отражается на их жизни и благополучии близких. Зависимость — это не просто физическое заболевание, но и глубокая психологическая проблема. Для эффективного лечения требуется помощь профессионалов, способных обеспечить комплексный подход.
Узнать больше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]вывод из запоя на дому недорого в воронеже[/url]
Процедура вывода из запоя направлена на устранение последствий длительного употребления алкоголя и восстановления работы организма. Этот процесс включает не только медикаментозное лечение, но и психологическую поддержку, что позволяет минимизировать симптомы абстиненции и предотвратить возможные осложнения.
Детальнее – [url=https://vyvod-iz-zapoya-14.ru/]https://www.vyvod-iz-zapoya-14.ru[/url]
Запой представляет собой состояние, характеризующееся длительным непрерывным употреблением алкоголя, что приводит к физической и психической зависимости. Этот процесс может длиться от нескольких дней до недель. Алкогольные напитки негативно влияют на функционирование внутренних органов, вызывая серьёзные нарушения, включая алкоголизм. При необходимости вывода из запоя важно обратиться за медицинской помощью, что можно осуществить на дому. В данной статье рассмотрим ключевые аспекты данной процедуры.
Выяснить больше – [url=https://vyvod-iz-zapoya-17.ru/]vyvod iz zapoya kapelnica chelyabinsk[/url]
Клиника “Второй Шанс” в Санкт-Петербурге предлагает услугу вывода из запоя, как в стационаре, так и на дому. Для пациентов, не желающих посещать клинику, квалифицированные врачи готовы предоставить помощь в комфортных домашних условиях. Используя современные методы, специалисты обеспечивают безопасность, анонимность и высокий уровень медицинской поддержки.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu nedorogo kapelnica [/url]
Алкогольная зависимость — это длительное и прогрессирующее заболевание, при котором развивается как физическая, так и психическая зависимость от алкоголя. Одним из самых тяжелых проявлений болезни является запой — длительное употребление алкоголя, которое может сопровождаться нарушениями работы организма и абстинентным синдромом, представляющим серьезную угрозу для здоровья. Эффективное лечение запоя требует комплексного подхода, включающего медикаментозную терапию, психотерапию и реабилитационные мероприятия.
Подробнее – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]vyvod iz zapoya na domu nedorogo kapelnica [/url]
В современном мире зависимость становится все более распространенной проблемой. Стресс, жизненные трудности и финансовые проблемы часто провоцируют развитие таких серьезных состояний, как алкоголизм, наркомания и игромания. Наркологическая клиника “Второй Шанс” предоставляет помощь людям, столкнувшимся с этими заболеваниями. Основное направление работы клиники — восстановление здоровья пациентов, их социальная адаптация и предотвращение повторных рецидивов.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]наркология вывод из запоя анонимно в санкт-петербурге[/url]
Зависимость от алкоголя – серьезная проблема, требующая системного подхода и профессионального лечения. В клинике «Излечение» гарантируется полная конфиденциальность на всех этапах процесса, что позволяет пациентам чувствовать себя в безопасности. Лечение может проходить как в стационаре, так и на дому, при этом соблюдаются все условия для максимального комфорта.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]вывод из запоя круглосуточно наркология [/url]
Алкоголизм — это хроническое прогрессирующее заболевание, связанное с физической и психической зависимостью от этанола. Запой, как его крайнее проявление, представляет собой длительное употребление алкоголя, сопровождающееся тяжелыми нарушениями работы организма, включая абстинентный синдром и потенциальные угрозы для жизни. Лечение запоя требует комплексного подхода, включающего медицинские процедуры, психотерапию и реабилитационные меры.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-v-stacionare-v-ekaterinburge/]vyvod iz zapoya v stacionare anonimno [/url]
Запойное пьянство характеризуется продолжительным и интенсивным потреблением алкоголя, что приводит к серьезным физическим и психологическим последствиям. Часто пациенты сталкиваются с различными заболеваниями, такими как цирроз печени, панкреатит, алкогольный делирий и другие. Алкогольное опьянение не только ухудшает качество жизни, но также может угрожать жизни пациента. В условиях стационара лечение может быть более эффективным, однако многие люди предпочитают проходить данную процедуру на дому, чтобы избежать стигматизации и сохранить чувство приватности.
Получить больше информации – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-cena-v-ekaterinburge/]vyvod iz zapoya s vyezdom cena ekaterinburg[/url]
Запой — это состояние, при котором человек не может прекратить употребление алкоголя на протяжении длительного времени, что вызывает физическую и психологическую зависимость. Это крайне опасное состояние, требующее немедленного вмешательства специалистов. Наркологическая клиника «Преображение» в Екатеринбурге предоставляет профессиональную помощь в лечении запоя, используя самые эффективные и безопасные методы.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]наркология вывод из запоя на дому [/url]
Мы понимаем, что каждая зависимость уникальна, поэтому основой нашего подхода является индивидуализация лечения. После тщательной диагностики, включающей анализ медицинской истории, психологического состояния и социальных факторов, наши специалисты разрабатывают персонализированный план терапии. Этот план может включать медикаментозную детоксикацию, психотерапевтические методы и социальные программы, направленные на изменение поведения и мышления пациента.
Подробнее – [url=https://kapelnica-ot-zapoya-irkutsk3.ru/kapelnica-ot-zapoya-na-domu-v-irkutske/]срочная капельница от запоя на дому в иркутске[/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Детальнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]вывод из запоя капельница на дому воронеж[/url]
Алкоголизм — это хроническое заболевание, которое характеризуется патологической зависимостью от этанола. Периоды запоя, когда человек неконтролируемо потребляет алкоголь, могут привести к разнообразным медицинским осложнениям, как физическим, так и психологическим. Запой становится не только причиной ухудшения здоровья, но и причиной множества социальных и личных проблем. На этом фоне возникает необходимость вывода из запоя, процесс, который должен учитывать индивидуальные особенности пациента. Важно, что анонимность в реабилитации играет ключевую роль, помогая избежать стигматизации и увеличивая шансы на успешное восстановление.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-anonimno-v-ryazani/]vyvod iz zapoya anonimno nedorogo v-ryazani[/url]
В клинике «Излечение» мы предлагаем широкий спектр услуг по выводу из запоя, включая как стационарное лечение, так и помощь на дому. В этой статье мы подробно рассмотрим, от чего зависит цена вывода из запоя и какие услуги входят в стоимость.
Углубиться в тему – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]anonimny vyvod iz zapoya na domu krasnodar[/url]
В клинике «Заря Будущего» мы понимаем, насколько важен своевременный и профессиональный подход к лечению зависимости. Наши специалисты готовы оказать квалифицированную помощь прямо у вас дома, обеспечивая полную безопасность и конфиденциальность на каждом этапе лечения.
Получить больше информации – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu sankt-peterburg[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Подробнее тут – [url=https://vyvod-iz-zapoya-19.ru/]vyvod iz zapoya s vyezdom sankt-peterburg[/url]
Запой — это состояние, при котором человек не может прекратить употребление алкоголя на протяжении длительного времени, что вызывает физическую и психологическую зависимость. Это крайне опасное состояние, требующее немедленного вмешательства специалистов. Наркологическая клиника «Преображение» в Екатеринбурге предоставляет профессиональную помощь в лечении запоя, используя самые эффективные и безопасные методы.
Узнать больше – [url=https://vyvod-iz-zapoya-20.ru/]narkologicheskij centr v-ekaterinburge[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает сильное воздействие этанола, что приводит к нарушению нормального функционирования физиологических систем. Это состояние может быть опасным и требует квалифицированной медицинской помощи. Вывод из запоя — важная медицинская процедура, направленная на устранение последствий чрезмерного употребления алкоголя и восстановление здоровья пациента.
Детальнее – [url=https://vyvod-iz-zapoya-17.ru/]http://www.vyvod-iz-zapoya-17.ru[/url]
Запой — это серьезное состояние, при котором человек не может прекратить употребление алкоголя на протяжении длительного времени, что вызывает физическую и психологическую зависимость. Это крайне опасное состояние, требующее немедленного вмешательства специалистов. Для безопасного и эффективного вывода из запоя необходима квалифицированная медицинская помощь, которую предоставляет наркологическая клиника «Рассвет» в Твери. Наши опытные специалисты используют современные методы детоксикации и восстановления организма, обеспечивая полный медицинский контроль и поддержку на всех этапах лечения.
Выяснить больше – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-v-stacionare-v-tveri/]вывод из запоя в стационаре в твери[/url]
Злоупотребление алкоголем в течение продолжительного времени приводит к состоянию, при котором человек теряет контроль над собой, а его организм подвергается серьезным нагрузкам. Последствия такого состояния проявляются на физическом и психическом уровнях: организм страдает от отравления, обезвоживания и сбоев в работе нервной системы, а человек теряет способность адекватно оценивать ситуацию. Выйти из этого состояния без медицинской помощи крайне сложно, так как требуется не только прекратить употребление алкоголя, но и восстановить нормальные функции организма.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]вывод из запоя круглосуточно в краснодаре[/url]
Нарколог, приехавший на дом, первым делом проводит осмотр пациента, оценивает его состояние и выбирает подходящие препараты для детоксикации организма. Это может включать капельницы с солевыми растворами, витаминами и глюкозой, которые способствуют очищению организма.
Изучить вопрос глубже – [url=https://kapelnica-ot-zapoya-irkutsk.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-irkutske/]анонимная капельница от запоя на дому в иркутске[/url]
Алкогольный запой сопровождается опасной интоксикацией, которая без своевременной помощи может привести к необратимым последствиям, включая поражение печени, сердца, почек и психические расстройства. В критических случаях промедление может стоить жизни. Именно поэтому специалисты клиники готовы оказывать поддержку круглосуточно.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-14.ru/]vyvod iz zapoya kapelnica sankt-peterburg[/url]
Запой – это тяжелое состояние, возникающее из-за длительного и неконтролируемого употребления алкоголя. Оно характеризуется физической и психологической зависимостью, что приводит к серьезным последствиям для организма и психики. Для восстановления нормального самочувствия и здоровья необходимо профессиональное лечение. Одним из наиболее удобных и эффективных методов является вывод из запоя на дому.
Подробнее тут – [url=https://vyvod-iz-zapoya-13.ru/]vyvod iz zapoya kapelnica v-ryazani[/url]
Наркологическая клиника «Союз Здоровья» в Воронеже специализируется на лечении алкогольной зависимости и предлагает профессиональные услуги по выводу из запоя. Мы стремимся создать атмосферу доверия, где каждый пациент сможет получить необходимую поддержку.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]vyvod iz zapoya s vyezdom cena [/url]
В клинике работает команда квалифицированных специалистов, которые осуществляют вывод из запоя, обеспечивая полный контроль над состоянием пациента и устраняя все последствия длительного употребления алкоголя.
Получить больше информации – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-cena-v-ekaterinburge/]vyvod iz zapoya s vyezdom cena [/url]
Нарколог, приехавший на дом, первым делом проводит осмотр пациента, оценивает его состояние и выбирает подходящие препараты для детоксикации организма. Это может включать капельницы с солевыми растворами, витаминами и глюкозой, которые способствуют очищению организма.
Подробнее – [url=https://kapelnica-ot-zapoya-moskva2.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-moskve/]капельница от запоя круглосуточно москва[/url]
Одним из главных преимуществ стационарного лечения в нашей клинике является полная анонимность. Мы понимаем, что многие пациенты боятся раскрытия своей зависимости и осуждения со стороны окружающих. В “Восстановление души” мы гарантируем конфиденциальность всех процедур и защиту личных данных пациента. Это создает безопасную и доверительную атмосферу, необходимую для успешного лечения и психологического восстановления.
Выяснить больше – [url=https://kapelnica-ot-zapoya-moskva1.ru/kapelnica-ot-zapoya-na-domu-v-moskve/]наркология капельница от запоя на дому[/url]
Процесс возвращения к нормальной жизни после длительного употребления алкоголя играет ключевую роль в избавлении от зависимости. Медицинское сопровождение должно сочетаться с осознанным желанием человека изменить свою жизнь. Важно, чтобы лечение проходило в условиях, исключающих стресс и дискомфорт. Для этого предусмотрены как стационарные программы, так и возможность получить медицинскую помощь на дому. Такой вариант подходит тем, кто предпочитает находиться в привычной обстановке и избегать огласки.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]вывод из запоя анонимно краснодар[/url]
Мы понимаем, что каждая зависимость уникальна, поэтому основой нашего подхода является индивидуализация лечения. После тщательной диагностики, включающей анализ медицинской истории, психологического состояния и социальных факторов, наши специалисты разрабатывают персонализированный план терапии. Этот план может включать медикаментозную детоксикацию, психотерапевтические методы и социальные программы, направленные на изменение поведения и мышления пациента.
Углубиться в тему – [url=https://kapelnica-ot-zapoya-irkutsk.ru/kapelnica-ot-zapoya-anonimno-v-irkutske/]bystry kapelnica ot zapoya v stacionare irkutsk[/url]
Вывод из запоя представляет собой важный этап в процессе лечения алкогольной зависимости. Это медицинская процедура, необходимая для устранения токсических веществ, накопленных в организме в результате длительного употребления алкоголя. Алкогольная зависимость может вызывать множество проблем, включая физические и психоэмоциональные расстройства. Своевременное вмешательство специалистов помогает минимизировать риски и способствует восстановлению здоровья пациента.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-anonimno-v-ryazani/]narkologiya vyvod iz zapoya anonimno ryazan[/url]
Клиника предлагает гибкие варианты лечения, включая стационарное и амбулаторное. Стоимость услуг зависит от индивидуальных потребностей пациента, степени тяжести состояния и объемов необходимых процедур. Специалисты стремятся создать максимально комфортные условия для каждого пациента, обеспечивая прозрачное ценообразование и высокое качество обслуживания.
Подробнее – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]вывод из запоя цена наркология санкт-петербург[/url]
Алкогольная зависимость — это серьезное хроническое заболевание, которое характеризуется непреодолимым стремлением к употреблению спиртных напитков, потерей контроля над их потреблением и развитием толерантности. Запойное состояние, при котором человек продолжает пить без перерыва в течение нескольких дней, приводит к серьезной интоксикации организма и может вызвать опасные осложнения. Капельница от запоя — один из наиболее эффективных методов лечения, направленный на быструю детоксикацию и стабилизацию состояния пациента. В наркологической клинике “Восстановление души” в Иркутске мы предлагаем профессиональную медицинскую помощь с учетом индивидуальных потребностей каждого пациента.
Углубиться в тему – [url=https://kapelnica-ot-zapoya-irkutsk3.ru/kapelnica-ot-zapoya-v-stacionare-v-irkutske/]kapelnica ot zapoya v stacionare v irkutske[/url]
Выход из запоя — это важнейший этап на пути к избавлению от алкогольной зависимости. Он требует комплексного подхода, профессиональной помощи и сильной мотивации пациента. В условиях, когда анонимность и комфорт становятся ключевыми факторами для многих людей, вывод из запоя на дому приобретает все большее значение. Это решение позволяет человеку получить необходимую помощь, не покидая дома, и избежать стресса, связанного с госпитализацией.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя на дому [/url]
Наркологическая клиника «Освобождение» в Рязани предлагает профессиональные услуги по выводу из запоя и комплексной реабилитации. Мы понимаем, что зависимость — это болезнь, требующая индивидуального подхода и поддержки не только для пациента, но и для его близких.
Детальнее – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-anonimno-v-ryazani/]vyvod iz zapoya anonimno nedorogo [/url]
Алкогольная зависимость остаётся серьёзной проблемой, требующей квалифицированного медицинского вмешательства. Капельница от запоя — это ключевой этап в процессе лечения, направленный на стабилизацию состояния пациента и его возвращение к полноценной жизни. Профессиональная помощь позволяет эффективно справиться с последствиями длительного употребления алкоголя и восстановить физическое и психологическое здоровье.
Подробнее – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-anonimno-v-tveri/]anonimny vyvod iz zapoya na domu tver[/url]
Запой — это опасное состояние, которое развивается у людей с алкогольной зависимостью и характеризуется длительным непрерывным потреблением алкоголя. Это состояние приводит к серьезным нарушениям в организме, как физическим, так и психоэмоциональным. Длительный запой может вызвать множество осложнений, таких как поражение внутренних органов, нервной системы, а также проблемы в социальной и семейной жизни. Однако современная медицина предоставляет эффективные методы вывода из запоя, и одним из наиболее удобных и востребованных является вывод из запоя на дому.
Углубиться в тему – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]narkologiya vyvod iz zapoya na domu v-sankt-peterburge[/url]
Процесс возвращения к нормальной жизни после длительного употребления алкоголя играет ключевую роль в избавлении от зависимости. Медицинское сопровождение должно сочетаться с осознанным желанием человека изменить свою жизнь. Важно, чтобы лечение проходило в условиях, исключающих стресс и дискомфорт. Для этого предусмотрены как стационарные программы, так и возможность получить медицинскую помощь на дому. Такой вариант подходит тем, кто предпочитает находиться в привычной обстановке и избегать огласки.
Подробнее тут – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-cena-v-krasnodare/]vyvod iz zapoya cena narkologiya krasnodar[/url]
Запойное пьянство характеризуется продолжительным и интенсивным потреблением алкоголя, что приводит к серьезным физическим и психологическим последствиям. Часто пациенты сталкиваются с различными заболеваниями, такими как цирроз печени, панкреатит, алкогольный делирий и другие. Алкогольное опьянение не только ухудшает качество жизни, но также может угрожать жизни пациента. В условиях стационара лечение может быть более эффективным, однако многие люди предпочитают проходить данную процедуру на дому, чтобы избежать стигматизации и сохранить чувство приватности.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]нарколог на дом вывод из запоя в екатеринбурге[/url]
Одной из распространенных проблем является запой, состояние, при котором человек длительное время бесконтрольно употребляет алкоголь, что приводит к тяжелой физической и психологической зависимости. Такое поведение чревато серьезными последствиями для здоровья, включая поражение внутренних органов, нервные расстройства и другие заболевания. Важно своевременно обратиться за профессиональной помощью, чтобы минимизировать риски и предотвратить осложнения.
Получить больше информации – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu nedorogo sankt-peterburg[/url]
Запой – это состояние, возникающее из-за длительного употребления алкоголя и характеризующееся физической и психологической зависимостью. Оно требует комплексного медицинского вмешательства для устранения последствий и предотвращения дальнейшего ухудшения здоровья. Стационарное лечение в клинике «Освобождение» в Рязани – это эффективный способ вернуть пациента к нормальной жизни, предоставляя ему комфортные условия и круглосуточную помощь специалистов.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-13.ru/]vyvod iz zapoya s vyezdom[/url]
В клинике «Излечение» мы предлагаем широкий спектр услуг по выводу из запоя, включая как стационарное лечение, так и помощь на дому. В этой статье мы подробно рассмотрим, от чего зависит цена вывода из запоя и какие услуги входят в стоимость.
Детальнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-stacionare-v-krasnodare/]быстрый вывод из запоя в стационаре [/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает сильное воздействие этанола, что приводит к нарушению нормального функционирования физиологических систем. Это состояние может быть опасным и требует квалифицированной медицинской помощи. Вывод из запоя — важная медицинская процедура, направленная на устранение последствий чрезмерного употребления алкоголя и восстановление здоровья пациента.
Разобраться лучше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-anonimno-v-chelyabinske/]narkologiya vyvod iz zapoya anonimno chelyabinsk[/url]
Если затянувшееся употребление алкоголя не прерывается, организм начинает испытывать тяжелые последствия. Возникает абстинентный синдром, проявляющийся в виде тошноты, сильной головной боли, дрожи в теле и других неприятных симптомов. Без своевременной помощи состояние может ухудшиться, поэтому важна быстрая медицинская реакция. Комплексное лечение направлено не только на избавление от физической зависимости, но и на поддержку человека в эмоциональном плане. В случаях, когда требуется постоянное наблюдение, рекомендуется стационарная терапия.
Выяснить больше – [url=https://vyvod-iz-zapoya-16.ru/]вывод из запоя с выездом краснодар[/url]
В современном мире зависимость становится все более распространенной проблемой. Стресс, жизненные трудности и финансовые проблемы часто провоцируют развитие таких серьезных состояний, как алкоголизм, наркомания и игромания. Наркологическая клиника “Второй Шанс” предоставляет помощь людям, столкнувшимся с этими заболеваниями. Основное направление работы клиники — восстановление здоровья пациентов, их социальная адаптация и предотвращение повторных рецидивов.
Углубиться в тему – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]анонимный вывод из запоя на дому санкт-петербург[/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya kapelnica na domu chelyabinsk[/url]
В клинике «Излечение» мы предлагаем широкий спектр услуг по выводу из запоя, включая как стационарное лечение, так и помощь на дому. В этой статье мы подробно рассмотрим, от чего зависит цена вывода из запоя и какие услуги входят в стоимость.
Детальнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]vyvod iz zapoya kruglosutochno narkologiya [/url]
Запой представляет собой состояние, связанное с длительным употреблением алкогольных напитков, что приводит к значительным физическим и психическим расстройствам. Это явление формирует зависимость, оказывая негативное воздействие на здоровье. Вывод из запоя в стационаре становится необходимостью для восстановительных мероприятий, направленных на реабилитацию пациента. В этой статье рассматриваются аспекты анонимного вывода, скорость процедур, а также роль нарколога в процессе лечения.
Подробнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-stacionare-v-krasnodare/]вывод из запоя в стационаре [/url]
Клиника “Второй Шанс” в Санкт-Петербурге предлагает услугу вывода из запоя, как в стационаре, так и на дому. Для пациентов, не желающих посещать клинику, квалифицированные врачи готовы предоставить помощь в комфортных домашних условиях. Используя современные методы, специалисты обеспечивают безопасность, анонимность и высокий уровень медицинской поддержки.
Выяснить больше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]вывод из запоя в стационаре анонимно в санкт-петербурге[/url]
Зависимость от алкоголя – серьезная проблема, требующая системного подхода и профессионального лечения. В клинике «Излечение» гарантируется полная конфиденциальность на всех этапах процесса, что позволяет пациентам чувствовать себя в безопасности. Лечение может проходить как в стационаре, так и на дому, при этом соблюдаются все условия для максимального комфорта.
Получить больше информации – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-stacionare-v-krasnodare/]вывод из запоя в стационаре анонимно [/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Разобраться лучше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya kapelnica na domu [/url]
Запой – это тяжелое состояние, возникающее из-за длительного и неконтролируемого употребления алкоголя. Оно характеризуется физической и психологической зависимостью, что приводит к серьезным последствиям для организма и психики. Для восстановления нормального самочувствия и здоровья необходимо профессиональное лечение. Одним из наиболее удобных и эффективных методов является вывод из запоя на дому.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-cena-v-ryazani/]вывод из запоя с выездом цена в рязани[/url]
Алкогольная зависимость становится всё более распространенной проблемой, требующей внимания как со стороны медицинского сообщества, так и общества в целом. Вывод из запоя — это сложный и ответственный процесс, необходимый для восстановления здоровья пациента и его интеграции в нормальную жизнь. Профессиональное вмешательство позволяет минимизировать последствия длительного употребления алкоголя и нормализовать состояние организма.
Узнать больше – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-cena-v-ekaterinburge/]нарколог вывод из запоя цена [/url]
Наркологическая клиника «Союз Здоровья» в Воронеже специализируется на лечении алкогольной зависимости и предлагает профессиональные услуги по выводу из запоя. Мы стремимся создать атмосферу доверия, где каждый пациент сможет получить необходимую поддержку.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]нарколог вывод из запоя цена воронеж[/url]
Запой – это тяжелое состояние, возникающее из-за длительного и неконтролируемого употребления алкоголя. Оно характеризуется физической и психологической зависимостью, что приводит к серьезным последствиям для организма и психики. Для восстановления нормального самочувствия и здоровья необходимо профессиональное лечение. Одним из наиболее удобных и эффективных методов является вывод из запоя на дому.
Выяснить больше – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]вывод из запоя на дому круглосуточно [/url]
Алкогольный запой сопровождается опасной интоксикацией, которая без своевременной помощи может привести к необратимым последствиям, включая поражение печени, сердца, почек и психические расстройства. В критических случаях промедление может стоить жизни. Именно поэтому специалисты клиники готовы оказывать поддержку круглосуточно.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]вывод из запоя в стационаре анонимно в санкт-петербурге[/url]
В клинике «Заря Будущего» мы понимаем, насколько важен своевременный и профессиональный подход к лечению зависимости. Наши специалисты готовы оказать квалифицированную помощь прямо у вас дома, обеспечивая полную безопасность и конфиденциальность на каждом этапе лечения.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]вывод из запоя анонимно [/url]
Алкогольная зависимость — это длительное и прогрессирующее заболевание, при котором развивается как физическая, так и психическая зависимость от алкоголя. Одним из самых тяжелых проявлений болезни является запой — длительное употребление алкоголя, которое может сопровождаться нарушениями работы организма и абстинентным синдромом, представляющим серьезную угрозу для здоровья. Эффективное лечение запоя требует комплексного подхода, включающего медикаментозную терапию, психотерапию и реабилитационные мероприятия.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-cena-v-tveri/]vyvod iz zapoya cena narkologiya [/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Углубиться в тему – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-anonimno-v-chelyabinske/]вывод из запоя анонимно [/url]
Awesome site you have here but I was curious if you knew of any user discussion forums that cover the same topics discussed in this article? I’d really love to be a part of group where I can get responses from other experienced people that share the same interest. If you have any recommendations, please let me know. Appreciate it!
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Подробнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]вывод из запоя на дому круглосуточно в воронеже[/url]
Зависимость от алкоголя – серьезная проблема, требующая системного подхода и профессионального лечения. В клинике «Излечение» гарантируется полная конфиденциальность на всех этапах процесса, что позволяет пациентам чувствовать себя в безопасности. Лечение может проходить как в стационаре, так и на дому, при этом соблюдаются все условия для максимального комфорта.
Подробнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]vyvod iz zapoya kruglosutochno narkologiya krasnodar[/url]
Алкогольная зависимость — это серьезное хроническое заболевание, которое характеризуется непреодолимым стремлением к употреблению спиртных напитков, потерей контроля над их потреблением и развитием толерантности. Запойное состояние, при котором человек продолжает пить без перерыва в течение нескольких дней, приводит к серьезной интоксикации организма и может вызвать опасные осложнения. Капельница от запоя — один из наиболее эффективных методов лечения, направленный на быструю детоксикацию и стабилизацию состояния пациента. В наркологической клинике “Восстановление души” в Иркутске мы предлагаем профессиональную медицинскую помощь с учетом индивидуальных потребностей каждого пациента.
Получить больше информации – [url=https://kapelnica-ot-zapoya-moskva1.ru/kapelnica-ot-zapoya-cena-v-moskve/]kapelnica ot zapoya srochno kruglosutochno moskva[/url]
Мы понимаем, что каждая зависимость уникальна, поэтому основой нашего подхода является индивидуализация лечения. После тщательной диагностики, включающей анализ медицинской истории, психологического состояния и социальных факторов, наши специалисты разрабатывают персонализированный план терапии. Этот план может включать медикаментозную детоксикацию, психотерапевтические методы и социальные программы, направленные на изменение поведения и мышления пациента.
Выяснить больше – [url=https://kapelnica-ot-zapoya-voronezh2.ru/]http://www.kapelnica-ot-zapoya-voronezh2.ru[/url]
[url=http://lordfilm-w.com/]фильмы в хорошем качестве [/url] – смотреть фильмы бесплатно, онлайн сериалы бесплатно
Алкогольный запой представляет собой критическое состояние, требующее немедленного вмешательства квалифицированных специалистов. Клиника «Союз Здоровья» в Воронеже предлагает круглосуточную помощь, чтобы обеспечить своевременное и эффективное лечение. Наша цель — оперативное снятие интоксикации, устранение симптомов и предотвращение осложнений.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]vyvod iz zapoya cena [/url]
В клинике «Излечение» мы предлагаем широкий спектр услуг по выводу из запоя, включая как стационарное лечение, так и помощь на дому. В этой статье мы подробно рассмотрим, от чего зависит цена вывода из запоя и какие услуги входят в стоимость.
Разобраться лучше – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]anonimny vyvod iz zapoya na domu [/url]
Для достижения эффективных результатов в лечении запоя необходима квалифицированная помощь специалистов. Наркологическая клиника «Излечение» в Краснодаре предоставляет профессиональные услуги по выводу из запоя, гарантируя безопасность, комфорт и индивидуальный подход к каждому пациенту.
Получить больше информации – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]srochny vyvod iz zapoya na domu v-krasnodare[/url]
Зависимость — это серьёзная проблема, которая затрагивает не только человека, но и его семью и окружение. Алкоголизм, наркомания и другие виды зависимостей могут разрушить жизнь, но с профессиональной помощью можно вернуть её в русло нормального функционирования. Наркологическая клиника «Заря Будущего» в Санкт-Петербурге предлагает уникальные программы лечения зависимостей, которые сочетает медико-психологический подход, индивидуальные методики и квалифицированную поддержку.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя врач на дом [/url]
Алкогольная зависимость становится всё более распространенной проблемой, требующей внимания как со стороны медицинского сообщества, так и общества в целом. Вывод из запоя — это сложный и ответственный процесс, необходимый для восстановления здоровья пациента и его интеграции в нормальную жизнь. Профессиональное вмешательство позволяет минимизировать последствия длительного употребления алкоголя и нормализовать состояние организма.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]срочный вывод из запоя на дому [/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Подробнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]narkologiya vyvod iz zapoya na domu voronezh[/url]
Процесс возвращения к нормальной жизни после длительного употребления алкоголя играет ключевую роль в избавлении от зависимости. Медицинское сопровождение должно сочетаться с осознанным желанием человека изменить свою жизнь. Важно, чтобы лечение проходило в условиях, исключающих стресс и дискомфорт. Для этого предусмотрены как стационарные программы, так и возможность получить медицинскую помощь на дому. Такой вариант подходит тем, кто предпочитает находиться в привычной обстановке и избегать огласки.
Разобраться лучше – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-stacionare-v-krasnodare/]быстрый вывод из запоя в стационаре краснодар[/url]
Клиника предлагает гибкие варианты лечения, включая стационарное и амбулаторное. Стоимость услуг зависит от индивидуальных потребностей пациента, степени тяжести состояния и объемов необходимых процедур. Специалисты стремятся создать максимально комфортные условия для каждого пациента, обеспечивая прозрачное ценообразование и высокое качество обслуживания.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]vyvod iz zapoya cena sankt-peterburg[/url]
Зависимость — это хроническое заболевание, требующее долгосрочного лечения и регулярной поддержки. В клинике используют современные научные методы в наркологии и психотерапии, предлагая помощь на всех этапах — от диагностики до реабилитации. Команда профессионалов работает с каждым пациентом индивидуально, обеспечивая комплексный подход к терапии.
Узнать больше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]vyvod iz zapoya v stacionare sankt-peterburg[/url]
Вывод из запойного состояния – сложный процесс, включающий несколько последовательных этапов. Основная цель – детоксикация организма и восстановление его нормального функционирования. Важно, чтобы процессом руководили опытные специалисты, способные минимизировать последствия интоксикации и помочь человеку вернуться к здоровой жизни. Стоимость лечения зависит от выбранных методов и уровня медицинского обслуживания, а в клинике «Излечение» предлагаются доступные варианты с учетом индивидуальных потребностей пациентов.
Узнать больше – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]вывод из запоя круглосуточно краснодар[/url]
В клинике «Заря Будущего» мы понимаем, насколько важен своевременный и профессиональный подход к лечению зависимости. Наши специалисты готовы оказать квалифицированную помощь прямо у вас дома, обеспечивая полную безопасность и конфиденциальность на каждом этапе лечения.
Узнать больше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]анонимный вывод из запоя на дому санкт-петербург[/url]
Запой нарушает привычный ритм жизни, вызывая серьезные физические и эмоциональные проблемы. Для его лечения требуется не только медикаментозное вмешательство, но и постоянное наблюдение специалистов. Стационарное лечение в клинике позволяет обеспечить полный контроль над состоянием пациента, что минимизирует риски и способствует более эффективной реабилитации.
Выяснить больше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]vyvod iz zapoya srochno kruglosutochno v-sankt-peterburge[/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Разобраться лучше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-cena-v-chelyabinske/]vyvod iz zapoya na domu cena chelyabinsk[/url]
В этой статье мы расскажем о возможностях клиники для лечения алкогольной интоксикации круглосуточно, включая вывод из запоя в стационаре и на дому, а также о роли профессионалов, таких как нарколог, в процессе лечения.
Разобраться лучше – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]вывод из запоя круглосуточно краснодар[/url]
Алкогольный запой представляет собой серьезное состояние, которое требует немедленного медицинского вмешательства. Это состояние характеризуется длительным и бесконтрольным потреблением алкоголя, что приводит к разрушению как физического, так и психоэмоционального здоровья человека. Для эффективного и безопасного вывода из запоя стационарное лечение является оптимальным решением, особенно при тяжелых случаях интоксикации. В стационаре клиники «Заря Будущего» мы предлагаем комплексную программу вывода из запоя, которая включает детоксикацию, восстановление организма и психотерапевтическую помощь.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]вывод из запоя анонимно санкт-петербург[/url]
Проблема зависимости от алкоголя, наркотиков и азартных игр остается одной из наиболее острых в современном обществе. Эти состояния оказывают значительное воздействие не только на здоровье самого человека, но и на его семью, друзей и общественные связи. Наркологическая клиника “Восстановление души” предлагает широкий спектр услуг для тех, кто борется с различными формами зависимости, такими как алкоголизм, наркомания и игромания. Наша цель — предоставить комплексный подход к лечению, что обеспечивает высокие показатели успешности среди наших пациентов.
Получить дополнительную информацию – [url=https://kapelnica-ot-zapoya-voronezh2.ru/kapelnica-ot-zapoya-cena-v-voronezhe/]kapelnica ot zapoya s vyezdom na dom v voronezhe[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]вывод из запоя цена наркология воронеж[/url]
Запой – это состояние, возникающее из-за длительного употребления алкоголя и характеризующееся физической и психологической зависимостью. Оно требует комплексного медицинского вмешательства для устранения последствий и предотвращения дальнейшего ухудшения здоровья. Стационарное лечение в клинике «Освобождение» в Рязани – это эффективный способ вернуть пациента к нормальной жизни, предоставляя ему комфортные условия и круглосуточную помощь специалистов.
Подробнее – [url=https://vyvod-iz-zapoya-13.ru/]вывод из запоя в рязани[/url]
Алкогольный запой — это тяжелое состояние, при котором человек теряет контроль над количеством потребляемого алкоголя. Это приводит к сильной интоксикации, сбоям в работе органов и систем организма, а также серьезным психоэмоциональным расстройствам. Без должной медицинской помощи запой может вызвать ряд опасных последствий, включая алкогольный делирий и угрозу жизни. Поэтому при первых признаках запоя необходимо обратиться к профессионалам.
Углубиться в тему – [url=https://vyvod-iz-zapoya-21.ru/]https://vyvod-iz-zapoya-21.ru[/url]
Одной из распространенных проблем является запой, состояние, при котором человек длительное время бесконтрольно употребляет алкоголь, что приводит к тяжелой физической и психологической зависимости. Такое поведение чревато серьезными последствиями для здоровья, включая поражение внутренних органов, нервные расстройства и другие заболевания. Важно своевременно обратиться за профессиональной помощью, чтобы минимизировать риски и предотвратить осложнения.
Получить больше информации – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]нарколог на дом вывод из запоя [/url]
Клиника “Второй Шанс” в Санкт-Петербурге предлагает услугу вывода из запоя, как в стационаре, так и на дому. Для пациентов, не желающих посещать клинику, квалифицированные врачи готовы предоставить помощь в комфортных домашних условиях. Используя современные методы, специалисты обеспечивают безопасность, анонимность и высокий уровень медицинской поддержки.
Выяснить больше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]вывод из запоя в стационаре санкт-петербург[/url]
Одним из важнейших аспектов, который помогает пациентам справиться с алкогольной зависимостью, является анонимность в процессе лечения. В клинике «Излечение» мы понимаем, как важно сохранить конфиденциальность и гарантировать защиту личной информации, особенно для тех, кто опасается общественного осуждения. Мы обеспечиваем полную анонимность при выводе из запоя, как в стационарных условиях, так и на дому.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]vyvod iz zapoya kruglosutochno narkologiya [/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Узнать больше – [url=https://vyvod-iz-zapoya-17.ru/]вывод из запоя с выездом челябинск[/url]
После успешной детоксикации начинается этап реабилитации, который включает восстановление социального статуса пациента и формирование новых здоровых привычек. Мы предлагаем как групповые, так и индивидуальные занятия, направленные на изменение поведения и мышления, что способствует долгосрочному удержанию пациента от возвращения к зависимостям.
Исследовать вопрос подробнее – [url=https://kapelnica-ot-zapoya-moskva3.ru/]http://www.kapelnica-ot-zapoya-moskva3.ru[/url]
Клиника «Освобождение» в Рязани предоставляет услугу круглосуточного вывода из запоя на дому, что позволяет пациенту получить медицинскую помощь в привычной обстановке, избегая лишнего стресса, связанного с госпитализацией.
Подробнее тут – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-v-kruglosutochno-v-ryazani/]vyvod iz zapoya kruglosutochno narkologiya [/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьезное воздействие этанола, что приводит к нарушению нормальной физиологической деятельности. Это состояние сопровождается сильными физическими и психологическими расстройствами, которые требуют профессионального вмешательства. Однако многие люди, страдающие алкогольной зависимостью, сталкиваются с трудностью признания своей проблемы, опасаясь утраты репутации и конфиденциальности. В клинике «Заря Будущего» мы предлагаем анонимный вывод из запоя в Санкт-Петербурге, предоставляя высококачественное лечение с гарантией сохранения личной информации.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-19.ru/]narkologicheskaya klinika sankt-peterburg[/url]
В клинике «Излечение» мы предлагаем широкий спектр услуг по выводу из запоя, включая как стационарное лечение, так и помощь на дому. В этой статье мы подробно рассмотрим, от чего зависит цена вывода из запоя и какие услуги входят в стоимость.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-cena-v-krasnodare/]vyvod iz zapoya cena [/url]
В клинике «Излечение» мы предлагаем широкий спектр услуг по выводу из запоя, включая как стационарное лечение, так и помощь на дому. В этой статье мы подробно рассмотрим, от чего зависит цена вывода из запоя и какие услуги входят в стоимость.
Подробнее тут – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]narkologiya vyvod iz zapoya anonimno v-krasnodare[/url]
Алкогольная зависимость — это серьезная проблема, требующая незамедлительного вмешательства, чтобы избежать тяжелых последствий для здоровья. Запойное пьянство может привести к различным заболеваниям, таким как цирроз печени, панкреатит и другие опасные состояния. К счастью, современная медицина предлагает эффективные методы решения этой проблемы. Для тех, кто не хочет или не может посетить стационар, есть возможность пройти процедуру капельницы от запоя на дому в Твери.
Подробнее – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-v-stacionare-v-tveri/]vyvod iz zapoya v stacionare anonimno tver[/url]
Одной из распространенных проблем является запой, состояние, при котором человек длительное время бесконтрольно употребляет алкоголь, что приводит к тяжелой физической и психологической зависимости. Такое поведение чревато серьезными последствиями для здоровья, включая поражение внутренних органов, нервные расстройства и другие заболевания. Важно своевременно обратиться за профессиональной помощью, чтобы минимизировать риски и предотвратить осложнения.
Выяснить больше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu [/url]
Алкогольный запой – это серьёзное состояние, при котором человек теряет контроль над употреблением алкоголя, что приводит к тяжёлой интоксикации организма. Во время запоя нарушаются функции внутренних органов, страдает нервная система, и повышается риск развития опасных осложнений, таких как алкогольный делирий. Это состояние требует незамедлительного медицинского вмешательства.
Подробнее – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-v-stacionare-v-ekaterinburge/]bystry vyvod iz zapoya v stacionare v-ekaterinburge[/url]
В некоторых ситуациях, когда запой длится несколько дней или приводит к серьезным последствиям для здоровья, необходим срочный вызов нарколога. Среди признаков, при которых требуется неотложная помощь:
Подробнее можно узнать тут – [url=https://kapelnica-ot-zapoya-voronezh2.ru/kapelnica-ot-zapoya-anonimno-v-voronezhe/]kapelnica ot zapoya kruglosutochno narkologiya v voronezhe[/url]
Алкогольный запой представляет собой критическое состояние, требующее немедленного вмешательства квалифицированных специалистов. Клиника «Союз Здоровья» в Воронеже предлагает круглосуточную помощь, чтобы обеспечить своевременное и эффективное лечение. Наша цель — оперативное снятие интоксикации, устранение симптомов и предотвращение осложнений.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-11.ru/]вывод из запоя с выездом воронеж[/url]
Вывод из запоя представляет собой важный этап в процессе лечения алкогольной зависимости. Это медицинская процедура, необходимая для устранения токсических веществ, накопленных в организме в результате длительного употребления алкоголя. Алкогольная зависимость может вызывать множество проблем, включая физические и психоэмоциональные расстройства. Своевременное вмешательство специалистов помогает минимизировать риски и способствует восстановлению здоровья пациента.
Выяснить больше – [url=https://vyvod-iz-zapoya-13.ru/]vyvod iz zapoya kapelnica[/url]
Наркологическая клиника «Освобождение» в Рязани предлагает профессиональные услуги по выводу из запоя и комплексной реабилитации. Мы понимаем, что зависимость — это болезнь, требующая индивидуального подхода и поддержки не только для пациента, но и для его близких.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-cena-v-ryazani/]vyvod iz zapoya s vyezdom cena [/url]
take a look at the site here https://abacusmarket.me/
Алкогольная зависимость — это серьезное заболевание, при котором человек теряет контроль над потреблением алкоголя, что приводит к его физической и психологической зависимости. Одним из наиболее выраженных проявлений алкоголизма является состояние запоя — длительное и неконтролируемое употребление спиртных напитков. В таких случаях капельница от запоя становится не только быстрым, но и безопасным методом для избавления от последствий интоксикации.
Подробнее можно узнать тут – [url=https://kapelnica-ot-zapoya-voronezh2.ru/kapelnica-ot-zapoya-v-stacionare-v-voronezhe/]капельница от запоя с выездом цена[/url]
Вывод из запойного состояния – сложный процесс, включающий несколько последовательных этапов. Основная цель – детоксикация организма и восстановление его нормального функционирования. Важно, чтобы процессом руководили опытные специалисты, способные минимизировать последствия интоксикации и помочь человеку вернуться к здоровой жизни. Стоимость лечения зависит от выбранных методов и уровня медицинского обслуживания, а в клинике «Излечение» предлагаются доступные варианты с учетом индивидуальных потребностей пациентов.
Выяснить больше – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]narkologiya vyvod iz zapoya anonimno v-krasnodare[/url]
Запой — это состояние, при котором человек не может прекратить употребление алкоголя на протяжении длительного времени, что вызывает физическую и психологическую зависимость. Это крайне опасное состояние, требующее немедленного вмешательства специалистов. Наркологическая клиника «Преображение» в Екатеринбурге предоставляет профессиональную помощь в лечении запоя, используя самые эффективные и безопасные методы.
Получить больше информации – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-cena-v-ekaterinburge/]vyvod iz zapoya cena narkologiya v-ekaterinburge[/url]
В этой статье мы расскажем о возможностях клиники для лечения алкогольной интоксикации круглосуточно, включая вывод из запоя в стационаре и на дому, а также о роли профессионалов, таких как нарколог, в процессе лечения.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/[/url]
Алкогольная интоксикация, или запой, представляет собой крайне опасное состояние, при котором организм страдает от токсического воздействия алкоголя. Это ведет к нарушению нормальных физиологических процессов и ухудшению общего состояния здоровья. Капельница от запоя — это медицинская процедура, направленная на выведение токсинов из организма и восстановление нормальной функции организма при соблюдении полной конфиденциальности.
Подробнее тут – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-cena-v-tveri/]вывод из запоя цена в твери[/url]
Алкогольная зависимость остаётся серьёзной проблемой, требующей квалифицированного медицинского вмешательства. Капельница от запоя — это ключевой этап в процессе лечения, направленный на стабилизацию состояния пациента и его возвращение к полноценной жизни. Профессиональная помощь позволяет эффективно справиться с последствиями длительного употребления алкоголя и восстановить физическое и психологическое здоровье.
Выяснить больше – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]vyvod iz zapoya na domu nedorogo tver[/url]
Алкогольный запой – это серьёзное состояние, при котором человек теряет контроль над употреблением алкоголя, что приводит к тяжёлой интоксикации организма. Во время запоя нарушаются функции внутренних органов, страдает нервная система, и повышается риск развития опасных осложнений, таких как алкогольный делирий. Это состояние требует незамедлительного медицинского вмешательства.
Разобраться лучше – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]вывод из запоя на дому екатеринбург[/url]
Эти меры позволяют пациенту быстро и безопасно восстановиться, минимизируя негативные последствия запойного состояния.
Получить дополнительные сведения – [url=https://kapelnica-ot-zapoya-moskva1.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-moskve/]нарколог капельница от запоя цена в москве[/url]
Процесс вывода из запоя требует быстрого реагирования. Запой, особенно в его тяжелых формах, представляет угрозу не только для психического состояния пациента, но и для его физического здоровья. Чтобы минимизировать риски и предотвратить осложнения, важно начинать лечение как можно скорее. Клинике «Освобождение» в Рязани доступна круглосуточная помощь — мы работаем 24/7, чтобы оперативно вмешаться в любой момент, когда это необходимо. Врачебная помощь может быть предоставлена как в стационаре клиники, так и на дому, в зависимости от состояния пациента и его предпочтений.
Детальнее – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-v-stacionare-v-ryazani/]вывод из запоя в стационаре анонимно рязань[/url]
Зависимость — это хроническое заболевание, требующее долгосрочного лечения и регулярной поддержки. В клинике используют современные научные методы в наркологии и психотерапии, предлагая помощь на всех этапах — от диагностики до реабилитации. Команда профессионалов работает с каждым пациентом индивидуально, обеспечивая комплексный подход к терапии.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]наркология вывод из запоя анонимно [/url]
Запой — это опасное состояние, которое развивается у людей с алкогольной зависимостью и характеризуется длительным непрерывным потреблением алкоголя. Это состояние приводит к серьезным нарушениям в организме, как физическим, так и психоэмоциональным. Длительный запой может вызвать множество осложнений, таких как поражение внутренних органов, нервной системы, а также проблемы в социальной и семейной жизни. Однако современная медицина предоставляет эффективные методы вывода из запоя, и одним из наиболее удобных и востребованных является вывод из запоя на дому.
Узнать больше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]анонимный вывод из запоя на дому санкт-петербург[/url]
Запой нарушает привычный ритм жизни, вызывая серьезные физические и эмоциональные проблемы. Для его лечения требуется не только медикаментозное вмешательство, но и постоянное наблюдение специалистов. Стационарное лечение в клинике позволяет обеспечить полный контроль над состоянием пациента, что минимизирует риски и способствует более эффективной реабилитации.
Разобраться лучше – [url=https://vyvod-iz-zapoya-14.ru/]вывод из запоя[/url]
Запойное пьянство характеризуется продолжительным и интенсивным потреблением алкоголя, что приводит к серьезным физическим и психологическим последствиям. Часто пациенты сталкиваются с различными заболеваниями, такими как цирроз печени, панкреатит, алкогольный делирий и другие. Алкогольное опьянение не только ухудшает качество жизни, но также может угрожать жизни пациента. В условиях стационара лечение может быть более эффективным, однако многие люди предпочитают проходить данную процедуру на дому, чтобы избежать стигматизации и сохранить чувство приватности.
Подробнее тут – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-v-kruglosutochno-v-ekaterinburge/]vyvod iz zapoya kruglosutochno [/url]
Алкоголизм — это хроническое заболевание, которое характеризуется патологической зависимостью от этанола. Периоды запоя, когда человек неконтролируемо потребляет алкоголь, могут привести к разнообразным медицинским осложнениям, как физическим, так и психологическим. Запой становится не только причиной ухудшения здоровья, но и причиной множества социальных и личных проблем. На этом фоне возникает необходимость вывода из запоя, процесс, который должен учитывать индивидуальные особенности пациента. Важно, что анонимность в реабилитации играет ключевую роль, помогая избежать стигматизации и увеличивая шансы на успешное восстановление.
Получить больше информации – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]наркология вывод из запоя на дому [/url]
Алкогольная зависимость представляет собой актуальную медицинскую проблему, требующую тщательного подхода. Запой характеризуется длительным, непрерывным употреблением спиртных напитков, что ведёт к физической и психической зависимости. На фоне запойного состояния могут возникнуть тяжёлые нарушения функций органов, увеличивается риск развития таких состояний, как алкогольный делирий. Вывод из запоя становится ключевым этапом в лечении зависимостей, который может осуществляться как в стационарных условиях, так и на дому, в зависимости от состояния пациента. В клинике «Перекресток Надежды» в Челябинске мы предлагаем круглосуточную помощь, чтобы обеспечить максимально эффективное и безопасное лечение при любом варианте вывода из запоя.
Выяснить больше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]наркология вывод из запоя на дому челябинск[/url]
Если затянувшееся употребление алкоголя не прерывается, организм начинает испытывать тяжелые последствия. Возникает абстинентный синдром, проявляющийся в виде тошноты, сильной головной боли, дрожи в теле и других неприятных симптомов. Без своевременной помощи состояние может ухудшиться, поэтому важна быстрая медицинская реакция. Комплексное лечение направлено не только на избавление от физической зависимости, но и на поддержку человека в эмоциональном плане. В случаях, когда требуется постоянное наблюдение, рекомендуется стационарная терапия.
Разобраться лучше – [url=https://vyvod-iz-zapoya-16.ru/]наркологический центр краснодар[/url]
Процедура вывода из запоя направлена на устранение последствий длительного употребления алкоголя и восстановления работы организма. Этот процесс включает не только медикаментозное лечение, но и психологическую поддержку, что позволяет минимизировать симптомы абстиненции и предотвратить возможные осложнения.
Углубиться в тему – [url=https://vyvod-iz-zapoya-14.ru/]наркологическая клиника в санкт-петербурге[/url]
После успешной детоксикации начинается этап реабилитации, который включает восстановление социального статуса пациента и формирование новых здоровых привычек. Мы предлагаем как групповые, так и индивидуальные занятия, направленные на изменение поведения и мышления, что способствует долгосрочному удержанию пациента от возвращения к зависимостям.
Получить дополнительную информацию – [url=https://kapelnica-ot-zapoya-irkutsk3.ru/kapelnica-ot-zapoya-cena-v-irkutske/]капельница от запоя круглосуточно наркология в иркутске[/url]
Неожиданные расходы могут застать врасплох, и тогда выручат [url=https://expl0it.ru/]микрозаймы[/url] . Многие сервисы предлагают первые займы без процентов, что особенно выгодно. Главное – внимательно изучить условия и не затягивать с возвратом.
В этой статье мы расскажем о возможностях клиники для лечения алкогольной интоксикации круглосуточно, включая вывод из запоя в стационаре и на дому, а также о роли профессионалов, таких как нарколог, в процессе лечения.
Выяснить больше – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]анонимный вывод из запоя на дому [/url]
Запой — это состояние, при котором человек продолжает употреблять алкоголь на протяжении нескольких дней или даже недель, не контролируя объемы потребляемого напитка. Этот процесс сопровождается тяжелыми физическими и психическими последствиями, такими как обезвоживание организма, отравление продуктами распада алкоголя, нервные расстройства и потеря контроля над ситуацией. Вывод из запоя — это медицинская процедура, направленная на прекращение употребления алкоголя и восстановление организма пациента.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-16.ru/]вывод из запоя с выездом[/url]
Алкогольная интоксикация, или запой, представляет собой крайне опасное состояние, при котором организм страдает от токсического воздействия алкоголя. Это ведет к нарушению нормальных физиологических процессов и ухудшению общего состояния здоровья. Капельница от запоя — это медицинская процедура, направленная на выведение токсинов из организма и восстановление нормальной функции организма при соблюдении полной конфиденциальности.
Получить больше информации – [url=https://vyvod-iz-zapoya-21.ru/]vyvod iz zapoya[/url]
Запой — это серьезное состояние, при котором человек не может прекратить употребление алкоголя на протяжении длительного времени, что вызывает физическую и психологическую зависимость. Это крайне опасное состояние, требующее немедленного вмешательства специалистов. Для безопасного и эффективного вывода из запоя необходима квалифицированная медицинская помощь, которую предоставляет наркологическая клиника «Рассвет» в Твери. Наши опытные специалисты используют современные методы детоксикации и восстановления организма, обеспечивая полный медицинский контроль и поддержку на всех этапах лечения.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-anonimno-v-tveri/]вывод из запоя анонимно в твери[/url]
Алкогольная зависимость представляет собой актуальную медицинскую проблему, требующую тщательного подхода. Запой характеризуется длительным, непрерывным употреблением спиртных напитков, что ведёт к физической и психической зависимости. На фоне запойного состояния могут возникнуть тяжёлые нарушения функций органов, увеличивается риск развития таких состояний, как алкогольный делирий. Вывод из запоя становится ключевым этапом в лечении зависимостей, который может осуществляться как в стационарных условиях, так и на дому, в зависимости от состояния пациента. В клинике «Перекресток Надежды» в Челябинске мы предлагаем круглосуточную помощь, чтобы обеспечить максимально эффективное и безопасное лечение при любом варианте вывода из запоя.
Получить больше информации – [url=https://vyvod-iz-zapoya-17.ru/]vyvod iz zapoya s vyezdom[/url]
Алкогольный запой представляет собой критическое состояние, требующее немедленного вмешательства квалифицированных специалистов. Клиника «Союз Здоровья» в Воронеже предлагает круглосуточную помощь, чтобы обеспечить своевременное и эффективное лечение. Наша цель — оперативное снятие интоксикации, устранение симптомов и предотвращение осложнений.
Разобраться лучше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-anonimno-v-voronezhe/]вывод из запоя анонимно воронеж[/url]
После успешной детоксикации начинается этап реабилитации, который включает восстановление социального статуса пациента и формирование новых здоровых привычек. Мы предлагаем как групповые, так и индивидуальные занятия, направленные на изменение поведения и мышления, что способствует долгосрочному удержанию пациента от возвращения к зависимостям.
Узнать больше – [url=https://kapelnica-ot-zapoya-voronezh2.ru/kapelnica-ot-zapoya-na-domu-v-voronezhe/]капельница от запоя в стационаре[/url]
Вывод из запойного состояния – сложный процесс, включающий несколько последовательных этапов. Основная цель – детоксикация организма и восстановление его нормального функционирования. Важно, чтобы процессом руководили опытные специалисты, способные минимизировать последствия интоксикации и помочь человеку вернуться к здоровой жизни. Стоимость лечения зависит от выбранных методов и уровня медицинского обслуживания, а в клинике «Излечение» предлагаются доступные варианты с учетом индивидуальных потребностей пациентов.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]srochny vyvod iz zapoya na domu v-krasnodare[/url]
Когда человек сталкивается с затяжными алкогольными эпизодами, ему необходима квалифицированная помощь. Специалисты наркологической клиники «Излечение» в Краснодаре разрабатывают индивидуальные методы вывода из этого состояния, создавая комфортные и безопасные условия для пациентов. Длительное употребление спиртного оказывает разрушительное воздействие на внутренние органы, приводит к психическим нарушениям и обостряет хронические болезни. В таких случаях медицинское вмешательство становится неотложной необходимостью.
Выяснить больше – [url=https://vyvod-iz-zapoya-16.ru/]наркологическая клиника[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-cena-v-chelyabinske/]vyvod iz zapoya na domu cena v-chelyabinske[/url]
В клинике работает команда квалифицированных специалистов, которые осуществляют вывод из запоя, обеспечивая полный контроль над состоянием пациента и устраняя все последствия длительного употребления алкоголя.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]vyvod iz zapoya na domu ekaterinburg[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-11.ru/]vyvod iz zapoya kapelnica[/url]
Алкогольная зависимость — коварное заболевание, которое захватывает не только физическое здоровье, но и разрушает психику, лишает человека воли и заставляет его жить в плену у собственной зависимости. Запой — один из наиболее тяжелых периодов в жизни человека, столкнувшегося с алкоголизмом. Он характеризуется длительным и интенсивным употреблением спиртных напитков, приводящим к глубокой интоксикации организма, нарушению физиологических функций, психическим расстройствам, а также обострению сопутствующих заболеваний.
Углубиться в тему – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя на дому недорого краснодар[/url]
В этой статье мы расскажем о возможностях клиники для лечения алкогольной интоксикации круглосуточно, включая вывод из запоя в стационаре и на дому, а также о роли профессионалов, таких как нарколог, в процессе лечения.
Подробнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-stacionare-v-krasnodare/]вывод из запоя в стационаре в краснодаре[/url]
Злоупотребление алкоголем в течение продолжительного времени приводит к состоянию, при котором человек теряет контроль над собой, а его организм подвергается серьезным нагрузкам. Последствия такого состояния проявляются на физическом и психическом уровнях: организм страдает от отравления, обезвоживания и сбоев в работе нервной системы, а человек теряет способность адекватно оценивать ситуацию. Выйти из этого состояния без медицинской помощи крайне сложно, так как требуется не только прекратить употребление алкоголя, но и восстановить нормальные функции организма.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]вывод из запоя круглосуточно наркология краснодар[/url]
Запой — это опасное состояние, которое развивается у людей с алкогольной зависимостью и характеризуется длительным непрерывным потреблением алкоголя. Это состояние приводит к серьезным нарушениям в организме, как физическим, так и психоэмоциональным. Длительный запой может вызвать множество осложнений, таких как поражение внутренних органов, нервной системы, а также проблемы в социальной и семейной жизни. Однако современная медицина предоставляет эффективные методы вывода из запоя, и одним из наиболее удобных и востребованных является вывод из запоя на дому.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]vyvod iz zapoya anonimno nedorogo v-sankt-peterburge[/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]vyvod iz zapoya cena [/url]
В некоторых ситуациях, когда запой длится несколько дней или приводит к серьезным последствиям для здоровья, необходим срочный вызов нарколога. Среди признаков, при которых требуется неотложная помощь:
Мы понимаем, что каждая зависимость уникальна, поэтому основой нашего подхода является индивидуализация лечения. После тщательной диагностики, включающей анализ медицинской истории, психологического состояния и социальных факторов, наши специалисты разрабатывают персонализированный план терапии. Этот план может включать медикаментозную детоксикацию, психотерапевтические методы и социальные программы, направленные на изменение поведения и мышления пациента.
Клиника «Рассвет» в Твери предоставляет услугу анонимного вывода из запоя, обеспечивая высокое качество медицинского обслуживания и строгое соблюдение всех этических норм.
Углубиться в тему – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-v-kruglosutochno-v-tveri/]вывод из запоя круглосуточно наркология [/url]
Вывод из запойного состояния – сложный процесс, включающий несколько последовательных этапов. Основная цель – детоксикация организма и восстановление его нормального функционирования. Важно, чтобы процессом руководили опытные специалисты, способные минимизировать последствия интоксикации и помочь человеку вернуться к здоровой жизни. Стоимость лечения зависит от выбранных методов и уровня медицинского обслуживания, а в клинике «Излечение» предлагаются доступные варианты с учетом индивидуальных потребностей пациентов.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-16.ru/]вывод из запоя с выездом краснодар[/url]
pop over to this website https://jaxx-liberty.com/
Алкоголизм — это хроническое прогрессирующее заболевание, связанное с физической и психической зависимостью от этанола. Запой, как его крайнее проявление, представляет собой длительное употребление алкоголя, сопровождающееся тяжелыми нарушениями работы организма, включая абстинентный синдром и потенциальные угрозы для жизни. Лечение запоя требует комплексного подхода, включающего медицинские процедуры, психотерапию и реабилитационные меры.
Детальнее – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-anonimno-v-ekaterinburge/]narkologiya vyvod iz zapoya anonimno [/url]
Запой – это состояние, возникающее из-за длительного употребления алкоголя и характеризующееся физической и психологической зависимостью. Оно требует комплексного медицинского вмешательства для устранения последствий и предотвращения дальнейшего ухудшения здоровья. Стационарное лечение в клинике «Освобождение» в Рязани – это эффективный способ вернуть пациента к нормальной жизни, предоставляя ему комфортные условия и круглосуточную помощь специалистов.
Углубиться в тему – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]vyvod iz zapoya na domu kruglosutochno [/url]
Вывод из запоя представляет собой важный этап в процессе лечения алкогольной зависимости. Это медицинская процедура, необходимая для устранения токсических веществ, накопленных в организме в результате длительного употребления алкоголя. Алкогольная зависимость может вызывать множество проблем, включая физические и психоэмоциональные расстройства. Своевременное вмешательство специалистов помогает минимизировать риски и способствует восстановлению здоровья пациента.
Получить больше информации – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]narkolog na dom vyvod iz zapoya v-ryazani[/url]
Алкогольная зависимость представляет собой актуальную медицинскую проблему, требующую тщательного подхода. Запой характеризуется длительным, непрерывным употреблением спиртных напитков, что ведёт к физической и психической зависимости. На фоне запойного состояния могут возникнуть тяжёлые нарушения функций органов, увеличивается риск развития таких состояний, как алкогольный делирий. Вывод из запоя становится ключевым этапом в лечении зависимостей, который может осуществляться как в стационарных условиях, так и на дому, в зависимости от состояния пациента. В клинике «Перекресток Надежды» в Челябинске мы предлагаем круглосуточную помощь, чтобы обеспечить максимально эффективное и безопасное лечение при любом варианте вывода из запоя.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya na domu nedorogo chelyabinsk[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьезное воздействие этанола, что приводит к нарушению нормальной физиологической деятельности. Это состояние сопровождается сильными физическими и психологическими расстройствами, которые требуют профессионального вмешательства. Однако многие люди, страдающие алкогольной зависимостью, сталкиваются с трудностью признания своей проблемы, опасаясь утраты репутации и конфиденциальности. В клинике «Заря Будущего» мы предлагаем анонимный вывод из запоя в Санкт-Петербурге, предоставляя высококачественное лечение с гарантией сохранения личной информации.
Подробнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]vyvod iz zapoya s vyezdom cena [/url]
Алкогольная зависимость — это длительное и прогрессирующее заболевание, при котором развивается как физическая, так и психическая зависимость от алкоголя. Одним из самых тяжелых проявлений болезни является запой — длительное употребление алкоголя, которое может сопровождаться нарушениями работы организма и абстинентным синдромом, представляющим серьезную угрозу для здоровья. Эффективное лечение запоя требует комплексного подхода, включающего медикаментозную терапию, психотерапию и реабилитационные мероприятия.
Выяснить больше – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]vyvod iz zapoya na domu kruglosutochno [/url]
Выход из запоя — это важнейший этап на пути к избавлению от алкогольной зависимости. Он требует комплексного подхода, профессиональной помощи и сильной мотивации пациента. В условиях, когда анонимность и комфорт становятся ключевыми факторами для многих людей, вывод из запоя на дому приобретает все большее значение. Это решение позволяет человеку получить необходимую помощь, не покидая дома, и избежать стресса, связанного с госпитализацией.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]vyvod iz zapoya kapelnica na domu v-krasnodare[/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-cena-v-chelyabinske/]вывод из запоя цена [/url]
Наркологическая клиника «Союз Здоровья» в Воронеже специализируется на лечении алкогольной зависимости и предлагает профессиональные услуги по выводу из запоя. Мы стремимся создать атмосферу доверия, где каждый пациент сможет получить необходимую поддержку.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-kruglosutochno-v-voronezhe/]vyvod iz zapoya srochno kruglosutochno v-voronezhe[/url]
Запой представляет собой состояние, связанное с длительным употреблением алкогольных напитков, что приводит к значительным физическим и психическим расстройствам. Это явление формирует зависимость, оказывая негативное воздействие на здоровье. Вывод из запоя в стационаре становится необходимостью для восстановительных мероприятий, направленных на реабилитацию пациента. В этой статье рассматриваются аспекты анонимного вывода, скорость процедур, а также роль нарколога в процессе лечения.
Подробнее тут – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]anonimny vyvod iz zapoya na domu krasnodar[/url]
Алкогольная зависимость — это серьезная проблема, требующая незамедлительного вмешательства, чтобы избежать тяжелых последствий для здоровья. Запойное пьянство может привести к различным заболеваниям, таким как цирроз печени, панкреатит и другие опасные состояния. К счастью, современная медицина предлагает эффективные методы решения этой проблемы. Для тех, кто не хочет или не может посетить стационар, есть возможность пройти процедуру капельницы от запоя на дому в Твери.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]srochny vyvod iz zapoya na domu v-tveri[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Выяснить больше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-cena-v-chelyabinske/]vyvod iz zapoya cena narkologiya chelyabinsk[/url]
Запой — это серьезное состояние, при котором человек не может прекратить употребление алкоголя на протяжении длительного времени, что вызывает физическую и психологическую зависимость. Это крайне опасное состояние, требующее немедленного вмешательства специалистов. Для безопасного и эффективного вывода из запоя необходима квалифицированная медицинская помощь, которую предоставляет наркологическая клиника «Рассвет» в Твери. Наши опытные специалисты используют современные методы детоксикации и восстановления организма, обеспечивая полный медицинский контроль и поддержку на всех этапах лечения.
Разобраться лучше – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]срочный вывод из запоя на дому [/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]нарколог на дом вывод из запоя в челябинске[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-v-stacionare-v-chelyabinske/]вывод из запоя в стационаре анонимно в челябинске[/url]
Запой — это тяжелое состояние, возникающее вследствие длительного и непрерывного употребления алкоголя, которое сопровождается физической и психической зависимостью. Это опасное явление требует незамедлительного вмешательства специалистов. Вывод из запоя — это комплексный процесс медицинской детоксикации, который включает в себя очищение организма от токсинов, нормализацию функций внутренних органов и поддержку психоэмоционального состояния пациента. В случае тяжелого запоя стационарное лечение становится оптимальным решением для безопасного и эффективного вывода из этого состояния. В этой статье мы рассмотрим, как проходит вывод из запоя в стационаре клиники «Перекресток Надежды» в Челябинске, а также особенности анонимности, скорости лечения и участия нарколога.
Подробнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-v-kruglosutochno-v-chelyabinske/]вывод из запоя круглосуточно наркология челябинск[/url]
В таких ситуациях вывод из запоя на дому становится оптимальным решением, особенно если пациент не может или не хочет посещать стационар. Эта услуга позволяет получить медицинскую помощь в комфортной и привычной обстановке, что снижает уровень стресса и ускоряет процесс восстановления.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-stacionare-v-voronezhe/]вывод из запоя в стационаре анонимно в воронеже[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]narkolog na dom vyvod iz zapoya [/url]
В таких ситуациях вывод из запоя на дому становится оптимальным решением, особенно если пациент не может или не хочет посещать стационар. Эта услуга позволяет получить медицинскую помощь в комфортной и привычной обстановке, что снижает уровень стресса и ускоряет процесс восстановления.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-kruglosutochno-v-voronezhe/]vyvod iz zapoya kruglosutochno v-voronezhe[/url]
Запой представляет собой состояние, связанное с длительным употреблением алкогольных напитков, что приводит к значительным физическим и психическим расстройствам. Это явление формирует зависимость, оказывая негативное воздействие на здоровье. Вывод из запоя в стационаре становится необходимостью для восстановительных мероприятий, направленных на реабилитацию пациента. В этой статье рассматриваются аспекты анонимного вывода, скорость процедур, а также роль нарколога в процессе лечения.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-16.ru/]вывод из запоя с выездом в краснодаре[/url]
В таких ситуациях вывод из запоя на дому становится оптимальным решением, особенно если пациент не может или не хочет посещать стационар. Эта услуга позволяет получить медицинскую помощь в комфортной и привычной обстановке, что снижает уровень стресса и ускоряет процесс восстановления.
Подробнее тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]вывод из запоя вызов на дом воронеж[/url]
Наркологическая клиника «Союз Здоровья» в Воронеже специализируется на лечении алкогольной зависимости и предлагает профессиональные услуги по выводу из запоя. Мы стремимся создать атмосферу доверия, где каждый пациент сможет получить необходимую поддержку.
Подробнее тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]вывод из запоя цена в воронеже[/url]
Наркологическая клиника «Союз Здоровья» в Воронеже специализируется на лечении алкогольной зависимости и предлагает профессиональные услуги по выводу из запоя. Мы стремимся создать атмосферу доверия, где каждый пациент сможет получить необходимую поддержку.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]vyvod iz zapoya s vyezdom cena [/url]
Запой — это тяжелое состояние, возникающее вследствие длительного и непрерывного употребления алкоголя, которое сопровождается физической и психической зависимостью. Это опасное явление требует незамедлительного вмешательства специалистов. Вывод из запоя — это комплексный процесс медицинской детоксикации, который включает в себя очищение организма от токсинов, нормализацию функций внутренних органов и поддержку психоэмоционального состояния пациента. В случае тяжелого запоя стационарное лечение становится оптимальным решением для безопасного и эффективного вывода из этого состояния. В этой статье мы рассмотрим, как проходит вывод из запоя в стационаре клиники «Перекресток Надежды» в Челябинске, а также особенности анонимности, скорости лечения и участия нарколога.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya kapelnica na domu v-chelyabinske[/url]
Алкогольный запой — это тяжелое состояние, при котором человек теряет контроль над количеством потребляемого алкоголя. Это приводит к сильной интоксикации, сбоям в работе органов и систем организма, а также серьезным психоэмоциональным расстройствам. Без должной медицинской помощи запой может вызвать ряд опасных последствий, включая алкогольный делирий и угрозу жизни. Поэтому при первых признаках запоя необходимо обратиться к профессионалам.
Выяснить больше – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-v-kruglosutochno-v-tveri/]vyvod iz zapoya kruglosutochno v-tveri[/url]
find https://jaxx-liberty.com/
Алкогольная зависимость становится всё более распространенной проблемой, требующей внимания как со стороны медицинского сообщества, так и общества в целом. Вывод из запоя — это сложный и ответственный процесс, необходимый для восстановления здоровья пациента и его интеграции в нормальную жизнь. Профессиональное вмешательство позволяет минимизировать последствия длительного употребления алкоголя и нормализовать состояние организма.
Получить больше информации – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-v-kruglosutochno-v-ekaterinburge/]vyvod iz zapoya srochno kruglosutochno ekaterinburg[/url]
see here https://web-lumiwallet.com
В этой статье мы расскажем о возможностях клиники для лечения алкогольной интоксикации круглосуточно, включая вывод из запоя в стационаре и на дому, а также о роли профессионалов, таких как нарколог, в процессе лечения.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/[/url]
Алкогольная зависимость представляет собой серьезную медицинскую проблему, которая влечет за собой не только физические, но и психологические проблемы. Периоды запоя, характеризующиеся бесконтрольным употреблением алкоголя, могут привести к тяжелым последствиям. Симптомы абстиненции требуют незамедлительного вмешательства. Анонимность в процессе реабилитации становится важным аспектом, помогающим пациентам избежать стыда и социального осуждения.
Подробнее тут – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]vyvod iz zapoya kruglosutochno [/url]
Проблема зависимостей остаётся актуальной в современном обществе. С каждым годом увеличивается число людей, страдающих от алкоголизма, наркомании и других форм зависимостей, что негативно отражается на их жизни и благополучии близких. Зависимость — это не просто физическое заболевание, но и глубокая психологическая проблема. Для эффективного лечения требуется помощь профессионалов, способных обеспечить комплексный подход.
Подробнее – [url=https://vyvod-iz-zapoya-11.ru/]vyvod iz zapoya kapelnica v-voronezhe[/url]
Запой – это тяжелое состояние, возникающее из-за длительного и неконтролируемого употребления алкоголя. Оно характеризуется физической и психологической зависимостью, что приводит к серьезным последствиям для организма и психики. Для восстановления нормального самочувствия и здоровья необходимо профессиональное лечение. Одним из наиболее удобных и эффективных методов является вывод из запоя на дому.
Выяснить больше – [url=https://vyvod-iz-zapoya-13.ru/]http://www.vyvod-iz-zapoya-13.ru[/url]
Проблема зависимостей остаётся актуальной в современном обществе. С каждым годом увеличивается число людей, страдающих от алкоголизма, наркомании и других форм зависимостей, что негативно отражается на их жизни и благополучии близких. Зависимость — это не просто физическое заболевание, но и глубокая психологическая проблема. Для эффективного лечения требуется помощь профессионалов, способных обеспечить комплексный подход.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]srochny vyvod iz zapoya na domu [/url]
Выход из запоя — это важнейший этап на пути к избавлению от алкогольной зависимости. Он требует комплексного подхода, профессиональной помощи и сильной мотивации пациента. В условиях, когда анонимность и комфорт становятся ключевыми факторами для многих людей, вывод из запоя на дому приобретает все большее значение. Это решение позволяет человеку получить необходимую помощь, не покидая дома, и избежать стресса, связанного с госпитализацией.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]vyvod iz zapoya vyzov na dom [/url]
Запой – это состояние, которое возникает вследствие длительного употребления алкоголя, приводящее к потере контроля над количеством выпиваемого и формированию как физической, так и психологической зависимости. Оно сопровождается серьёзными последствиями для здоровья и требует немедленного вмешательства.
Выяснить больше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-kruglosutochno-v-voronezhe/]вывод из запоя круглосуточно в воронеже[/url]
Мы понимаем, что успешное лечение невозможно без индивидуального подхода. Каждый случай уникален, поэтому мы разрабатываем персонализированные программы, учитывающие особенности каждого человека. Наши специалисты проводят детальное обследование и подбирают оптимальные методы лечения, обеспечивая комплексное внимание к состоянию пациента.
Изучить вопрос глубже – [url=https://kapelnica-ot-zapoya-voronezh3.ru/kapelnica-ot-zapoya-v-stacionare-v-voronezhe/]нарколог на дом капельница от запоя в воронеже[/url]
Наркологическая клиника «Союз Здоровья» в Воронеже специализируется на лечении алкогольной зависимости и предлагает профессиональные услуги по выводу из запоя. Мы стремимся создать атмосферу доверия, где каждый пациент сможет получить необходимую поддержку.
Получить больше информации – [url=https://vyvod-iz-zapoya-11.ru/]vyvod iz zapoya s vyezdom[/url]
Запой – это состояние, которое возникает вследствие длительного употребления алкоголя, приводящее к потере контроля над количеством выпиваемого и формированию как физической, так и психологической зависимости. Оно сопровождается серьёзными последствиями для здоровья и требует немедленного вмешательства.
Углубиться в тему – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]vyvod iz zapoya kapelnica na domu [/url]
Запой — это состояние, характеризующееся утратой контроля над употреблением алкоголя, что приводит к тяжелой интоксикации организма. Вывод из запоя — это комплексный процесс, направленный на устранение последствий интоксикации и восстановление нормального функционирования организма. Цена данной процедуры может значительно различаться в зависимости от нескольких факторов, и в этой статье мы подробно рассмотрим, что влияет на стоимость вывода из запоя в Краснодаре, в том числе в клинике «Шаг к Трезвости».
Разобраться лучше – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-cena-v-krasnodare/]narkolog vyvod iz zapoya cena [/url]
Запой представляет собой состояние, связанное с длительным употреблением алкогольных напитков, что приводит к значительным физическим и психическим расстройствам. Это явление формирует зависимость, оказывая негативное воздействие на здоровье. Вывод из запоя в стационаре становится необходимостью для восстановительных мероприятий, направленных на реабилитацию пациента. В этой статье рассматриваются аспекты анонимного вывода, скорость процедур, а также роль нарколога в процессе лечения.
Детальнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]нарколог на дом вывод из запоя в краснодаре[/url]
Запойное состояние представляет собой серьёзную угрозу для здоровья, поскольку сопровождается тяжёлой интоксикацией организма и нарушением психоэмоционального состояния. Решение о лечении в стационаре клиники «Союз Здоровья» позволяет справиться с последствиями длительного употребления алкоголя, обеспечивая пациентам полный комплекс медицинской и психологической помощи.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]срочный вывод из запоя на дому воронеж[/url]
Наркологическая клиника «Союз Здоровья» в Воронеже специализируется на лечении алкогольной зависимости и предлагает профессиональные услуги по выводу из запоя. Мы стремимся создать атмосферу доверия, где каждый пациент сможет получить необходимую поддержку.
Детальнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-anonimno-v-voronezhe/]anonimny vyvod iz zapoya na domu [/url]
Алкогольная зависимость — коварное заболевание, которое захватывает не только физическое здоровье, но и разрушает психику, лишает человека воли и заставляет его жить в плену у собственной зависимости. Запой — один из наиболее тяжелых периодов в жизни человека, столкнувшегося с алкоголизмом. Он характеризуется длительным и интенсивным употреблением спиртных напитков, приводящим к глубокой интоксикации организма, нарушению физиологических функций, психическим расстройствам, а также обострению сопутствующих заболеваний.
Выяснить больше – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-cena-v-krasnodare/]нарколог вывод из запоя цена [/url]
Запой – это состояние, которое возникает вследствие длительного употребления алкоголя, приводящее к потере контроля над количеством выпиваемого и формированию как физической, так и психологической зависимости. Оно сопровождается серьёзными последствиями для здоровья и требует немедленного вмешательства.
Разобраться лучше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]vyvod iz zapoya cena narkologiya v-voronezhe[/url]
Алкогольная зависимость — коварное заболевание, которое захватывает не только физическое здоровье, но и разрушает психику, лишает человека воли и заставляет его жить в плену у собственной зависимости. Запой — один из наиболее тяжелых периодов в жизни человека, столкнувшегося с алкоголизмом. Он характеризуется длительным и интенсивным употреблением спиртных напитков, приводящим к глубокой интоксикации организма, нарушению физиологических функций, психическим расстройствам, а также обострению сопутствующих заболеваний.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя капельница на дому в краснодаре[/url]
Алкогольная зависимость — это серьезная проблема, требующая немедленного вмешательства. Если вы или ваши близкие столкнулись с запоем, важно получить профессиональную помощь как можно скорее, чтобы избежать ухудшения здоровья. Наркологическая клиника «Шаг к Трезвости» в Краснодаре предлагает услугу вывода из запоя на дому, что является удобным и эффективным решением для тех, кто не может или не хочет посещать стационар.
Разобраться лучше – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-cena-v-krasnodare/]вывод из запоя с выездом цена краснодар[/url]
Алкоголизм — это хроническое заболевание, которое характеризуется патологической зависимостью от этанола. Периоды запоя, когда человек неконтролируемо потребляет алкоголь, могут привести к разнообразным медицинским осложнениям, как физическим, так и психологическим. Запой становится не только причиной ухудшения здоровья, но и причиной множества социальных и личных проблем. На этом фоне возникает необходимость вывода из запоя, процесс, который должен учитывать индивидуальные особенности пациента. Важно, что анонимность в реабилитации играет ключевую роль, помогая избежать стигматизации и увеличивая шансы на успешное восстановление.
Детальнее – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-anonimno-v-ryazani/]вывод из запоя анонимно рязань[/url]
Клиника «Шаг к Трезвости» в Краснодаре предлагает анонимный вывод из запоя, обеспечивая полную конфиденциальность и безопасность на каждом этапе лечения. Мы понимаем, что для многих людей важно сохранить свою личную информацию в тайне, и гарантируем, что все данные о пациенте и его состоянии здоровья останутся закрытыми. Независимо от того, выбрал ли пациент стационарное лечение или вывод из запоя на дому, мы обеспечиваем высококачественную медицинскую помощь с полным соблюдением принципов конфиденциальности.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя на дому [/url]
Запойное состояние представляет собой серьёзную угрозу для здоровья, поскольку сопровождается тяжёлой интоксикацией организма и нарушением психоэмоционального состояния. Решение о лечении в стационаре клиники «Союз Здоровья» позволяет справиться с последствиями длительного употребления алкоголя, обеспечивая пациентам полный комплекс медицинской и психологической помощи.
Получить больше информации – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]вывод из запоя вызов на дом воронеж[/url]
Клиника «Шаг к Трезвости» в Краснодаре предлагает анонимный вывод из запоя, обеспечивая полную конфиденциальность и безопасность на каждом этапе лечения. Мы понимаем, что для многих людей важно сохранить свою личную информацию в тайне, и гарантируем, что все данные о пациенте и его состоянии здоровья останутся закрытыми. Независимо от того, выбрал ли пациент стационарное лечение или вывод из запоя на дому, мы обеспечиваем высококачественную медицинскую помощь с полным соблюдением принципов конфиденциальности.
Углубиться в тему – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]srochny vyvod iz zapoya na domu v-krasnodare[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]вывод из запоя на дому круглосуточно воронеж[/url]
Клиника «Шаг к Трезвости» в Краснодаре предлагает анонимный вывод из запоя, обеспечивая полную конфиденциальность и безопасность на каждом этапе лечения. Мы понимаем, что для многих людей важно сохранить свою личную информацию в тайне, и гарантируем, что все данные о пациенте и его состоянии здоровья останутся закрытыми. Независимо от того, выбрал ли пациент стационарное лечение или вывод из запоя на дому, мы обеспечиваем высококачественную медицинскую помощь с полным соблюдением принципов конфиденциальности.
Углубиться в тему – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-cena-v-krasnodare/]vyvod iz zapoya na domu cena krasnodar[/url]
Запой представляет собой состояние, связанное с длительным употреблением алкогольных напитков, что приводит к значительным физическим и психическим расстройствам. Это явление формирует зависимость, оказывая негативное воздействие на здоровье. Вывод из запоя в стационаре становится необходимостью для восстановительных мероприятий, направленных на реабилитацию пациента. В этой статье рассматриваются аспекты анонимного вывода, скорость процедур, а также роль нарколога в процессе лечения.
Узнать больше – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя на дому недорого [/url]
Проблема зависимостей остаётся актуальной в современном обществе. С каждым годом увеличивается число людей, страдающих от алкоголизма, наркомании и других форм зависимостей, что негативно отражается на их жизни и благополучии близких. Зависимость — это не просто физическое заболевание, но и глубокая психологическая проблема. Для эффективного лечения требуется помощь профессионалов, способных обеспечить комплексный подход.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]vyvod iz zapoya na domu nedorogo kapelnica v-voronezhe[/url]
В клинике «Излечение» мы предлагаем широкий спектр услуг по выводу из запоя, включая как стационарное лечение, так и помощь на дому. В этой статье мы подробно рассмотрим, от чего зависит цена вывода из запоя и какие услуги входят в стоимость.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]vyvod iz zapoya anonimno [/url]
Зависимость – это сложное испытание, с которым сталкиваются люди во всём мире. Ежедневные стрессы, проблемы в семье и на работе, финансовые трудности часто становятся почвой для развития пагубных привычек, таких как алкоголизм, наркомания и игромания. Медицинский центр «Второй Шанс» предоставляет помощь тем, кто хочет избавиться от этих состояний, вернуть здоровье и научиться жить без разрушительных зависимостей.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]srochny vyvod iz zapoya na domu sankt-peterburg[/url]
Алкогольная зависимость представляет собой серьезную медицинскую проблему, которая влечет за собой не только физические, но и психологические проблемы. Периоды запоя, характеризующиеся бесконтрольным употреблением алкоголя, могут привести к тяжелым последствиям. Симптомы абстиненции требуют незамедлительного вмешательства. Анонимность в процессе реабилитации становится важным аспектом, помогающим пациентам избежать стыда и социального осуждения.
Детальнее – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-cena-v-krasnodare/]вывод из запоя с выездом цена краснодар[/url]
Алкогольный запой представляет собой критическое состояние, требующее немедленного вмешательства квалифицированных специалистов. Клиника «Союз Здоровья» в Воронеже предлагает круглосуточную помощь, чтобы обеспечить своевременное и эффективное лечение. Наша цель — оперативное снятие интоксикации, устранение симптомов и предотвращение осложнений.
Разобраться лучше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]vyvod iz zapoya vyzov na dom [/url]
В этой статье мы расскажем о возможностях клиники для лечения алкогольной интоксикации круглосуточно, включая вывод из запоя в стационаре и на дому, а также о роли профессионалов, таких как нарколог, в процессе лечения.
Углубиться в тему – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]наркология вывод из запоя анонимно краснодар[/url]
Запой — это состояние, характеризующееся утратой контроля над употреблением алкоголя, что приводит к тяжелой интоксикации организма. Вывод из запоя — это комплексный процесс, направленный на устранение последствий интоксикации и восстановление нормального функционирования организма. Цена данной процедуры может значительно различаться в зависимости от нескольких факторов, и в этой статье мы подробно рассмотрим, что влияет на стоимость вывода из запоя в Краснодаре, в том числе в клинике «Шаг к Трезвости».
Узнать больше – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя на дому в краснодаре[/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Подробнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]narkologiya vyvod iz zapoya na domu voronezh[/url]
Алкогольная зависимость — это серьезная проблема, требующая немедленного вмешательства. Если вы или ваши близкие столкнулись с запоем, важно получить профессиональную помощь как можно скорее, чтобы избежать ухудшения здоровья. Наркологическая клиника «Шаг к Трезвости» в Краснодаре предлагает услугу вывода из запоя на дому, что является удобным и эффективным решением для тех, кто не может или не хочет посещать стационар.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]нарколог на дом вывод из запоя в краснодаре[/url]
Запой — это длительное и неконтролируемое употребление алкоголя, которое приводит к серьёзному отравлению организма. Этанол и его метаболиты оказывают негативное воздействие на различные внутренние органы, ухудшая не только физическое состояние, но и психическое здоровье. При этом запой является не только медицинской, но и социальной проблемой. Он может сказаться на взаимоотношениях с близкими, привести к потере работы и другим серьёзным последствиям. Поэтому так важно вовремя распознать симптомы и обратиться за помощью.
Подробнее – [url=https://kapelnica-ot-zapoya-voronezh3.ru/kapelnica-ot-zapoya-cena-v-voronezhe/]капельница от запоя круглосуточно в воронеже[/url]
Лечение зависимости требует комплексного подхода, сочетающего медицинские методы и психологическую поддержку. Это не просто вредная привычка, а хроническое заболевание, требующее тщательного контроля и длительной терапии. В центре используются современные научно обоснованные методики, помогающие пациентам преодолеть сложный путь выздоровления. Каждому человеку подбирается индивидуальная программа, учитывающая его особенности и жизненные обстоятельства.
Разобраться лучше – [url=https://vyvod-iz-zapoya-14.ru/]вывод из запоя в санкт-петербурге[/url]
Для тех, кто не может приехать в клинику, специалисты проводят детоксикацию на дому. С помощью современных препаратов организм мягко очищается от токсинов, уменьшается нагрузка на внутренние органы, снимаются неприятные симптомы абстиненции. Однако при тяжелом состоянии или наличии осложнений может потребоваться круглосуточное наблюдение в стационаре, где пациенту обеспечивается полная медицинская поддержка.
Углубиться в тему – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]вывод из запоя анонимно [/url]
В клинике «Излечение» мы предлагаем широкий спектр услуг по выводу из запоя, включая как стационарное лечение, так и помощь на дому. В этой статье мы подробно рассмотрим, от чего зависит цена вывода из запоя и какие услуги входят в стоимость.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]narkologiya vyvod iz zapoya anonimno [/url]
В медицинском центре «Второй Шанс» помощь оказывается круглосуточно. Применяются проверенные и эффективные методы, обеспечивающие безопасность пациента и устойчивый результат. Конфиденциальность гарантирует комфорт и спокойствие тем, кто обратился за поддержкой, позволяя сосредоточиться на выздоровлении.
Разобраться лучше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]вывод из запоя анонимно в санкт-петербурге[/url]
В медицинском центре «Второй Шанс» помощь оказывается круглосуточно. Применяются проверенные и эффективные методы, обеспечивающие безопасность пациента и устойчивый результат. Конфиденциальность гарантирует комфорт и спокойствие тем, кто обратился за поддержкой, позволяя сосредоточиться на выздоровлении.
Разобраться лучше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu nedorogo kapelnica v-sankt-peterburge[/url]
В этой статье мы расскажем о возможностях клиники для лечения алкогольной интоксикации круглосуточно, включая вывод из запоя в стационаре и на дому, а также о роли профессионалов, таких как нарколог, в процессе лечения.
Углубиться в тему – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-cena-v-krasnodare/]narkolog vyvod iz zapoya cena [/url]
Запой – это состояние, которое возникает вследствие длительного употребления алкоголя, приводящее к потере контроля над количеством выпиваемого и формированию как физической, так и психологической зависимости. Оно сопровождается серьёзными последствиями для здоровья и требует немедленного вмешательства.
Подробнее тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]narkolog na dom vyvod iz zapoya [/url]
Выведение из запоя – это процесс, включающий несколько этапов. Симптомы могут варьироваться от тревожности и бессонницы до серьезных психических расстройств. Только профессиональный подход и современные медицинские технологии помогают минимизировать риски, стабилизировать состояние и избежать осложнений.
Углубиться в тему – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]vyvod iz zapoya v stacionare [/url]
Капельница от запоя – это метод внутривенного введения специальных растворов, направленных на восстановление водно-электролитного баланса организма, выведение токсинов и снижение симптомов абстинентного синдрома. Эта процедура позволяет быстро стабилизировать состояние пациента, ускорить процессы детоксикации и предотвратить возможные осложнения, связанные с длительным употреблением алкоголя.
Ознакомиться с деталями – [url=https://kapelnica-ot-zapoya-voronezh3.ru/kapelnica-ot-zapoya-v-stacionare-v-voronezhe/]kapelnica ot zapoya s vyezdom cena[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]вывод из запоя цена наркология в воронеже[/url]
Одним из важнейших аспектов, который помогает пациентам справиться с алкогольной зависимостью, является анонимность в процессе лечения. В клинике «Излечение» мы понимаем, как важно сохранить конфиденциальность и гарантировать защиту личной информации, особенно для тех, кто опасается общественного осуждения. Мы обеспечиваем полную анонимность при выводе из запоя, как в стационарных условиях, так и на дому.
Подробнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя на дому недорого капельница краснодар[/url]
Алкогольная зависимость — это серьезная проблема, требующая немедленного вмешательства. Если вы или ваши близкие столкнулись с запоем, важно получить профессиональную помощь как можно скорее, чтобы избежать ухудшения здоровья. Наркологическая клиника «Шаг к Трезвости» в Краснодаре предлагает услугу вывода из запоя на дому, что является удобным и эффективным решением для тех, кто не может или не хочет посещать стационар.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-cena-v-krasnodare/]vyvod iz zapoya na domu cena krasnodar[/url]
Запой — это длительное и неконтролируемое употребление алкоголя, которое приводит к серьёзному отравлению организма. Этанол и его метаболиты оказывают негативное воздействие на различные внутренние органы, ухудшая не только физическое состояние, но и психическое здоровье. При этом запой является не только медицинской, но и социальной проблемой. Он может сказаться на взаимоотношениях с близкими, привести к потере работы и другим серьёзным последствиям. Поэтому так важно вовремя распознать симптомы и обратиться за помощью.
Разобраться лучше – [url=https://kapelnica-ot-zapoya-voronezh3.ru/kapelnica-ot-zapoya-cena-v-voronezhe/]kapelnica ot zapoya srochno kruglosutochno v voronezhe[/url]
В медицинском центре «Второй Шанс» помощь оказывается круглосуточно. Применяются проверенные и эффективные методы, обеспечивающие безопасность пациента и устойчивый результат. Конфиденциальность гарантирует комфорт и спокойствие тем, кто обратился за поддержкой, позволяя сосредоточиться на выздоровлении.
Углубиться в тему – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]вывод из запоя анонимно [/url]
Алкоголизм – одно из наиболее разрушительных заболеваний, наносящих серьезный ущерб организму. Запойные состояния особенно опасны, так как приводят к глубокой интоксикации, психическим нарушениям и необратимым последствиям. Чем раньше начато лечение, тем выше вероятность избежать осложнений и быстрее восстановиться.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]наркология вывод из запоя на дому санкт-петербург[/url]
Алкогольная зависимость представляет собой серьёзную медицинскую проблему, требующую специализированного подхода. Запойное состояние характеризуется длительным и бесконтрольным потреблением спиртных напитков, что приводит к возникновению как физической, так и психической зависимости. Данное явление может вызвать нарушения в работе внутренних органов, значительно увеличивая вероятность развития алкогольного делирия, который представляет собой острое состояние, требующее неотложной медицинской помощи. Вывод из запоя это ключевая часть лечения зависимости, которая может осуществляться как в стационаре, так и в амбулаторных условиях, в зависимости от конкретной ситуации и состояния пациента.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]вывод из запоя круглосуточно наркология [/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Узнать больше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]вывод из запоя вызов на дом в воронеже[/url]
Запой — это состояние, характеризующееся утратой контроля над употреблением алкоголя, что приводит к тяжелой интоксикации организма. Вывод из запоя — это комплексный процесс, направленный на устранение последствий интоксикации и восстановление нормального функционирования организма. Цена данной процедуры может значительно различаться в зависимости от нескольких факторов, и в этой статье мы подробно рассмотрим, что влияет на стоимость вывода из запоя в Краснодаре, в том числе в клинике «Шаг к Трезвости».
Подробнее тут – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-v-stacionare-v-krasnodare/]bystry vyvod iz zapoya v stacionare v-krasnodare[/url]
Алкогольная зависимость представляет собой серьезную медицинскую проблему, которая влечет за собой не только физические, но и психологические проблемы. Периоды запоя, характеризующиеся бесконтрольным употреблением алкоголя, могут привести к тяжелым последствиям. Симптомы абстиненции требуют незамедлительного вмешательства. Анонимность в процессе реабилитации становится важным аспектом, помогающим пациентам избежать стыда и социального осуждения.
Подробнее тут – [url=https://vyvod-iz-zapoya-12.ru/]vyvod iz zapoya s vyezdom v-krasnodare[/url]
Выход из запоя — это важнейший этап на пути к избавлению от алкогольной зависимости. Он требует комплексного подхода, профессиональной помощи и сильной мотивации пациента. В условиях, когда анонимность и комфорт становятся ключевыми факторами для многих людей, вывод из запоя на дому приобретает все большее значение. Это решение позволяет человеку получить необходимую помощь, не покидая дома, и избежать стресса, связанного с госпитализацией.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-16.ru/]vyvod iz zapoya gorod krasnodar[/url]
Для тех, кто не может приехать в клинику, специалисты проводят детоксикацию на дому. С помощью современных препаратов организм мягко очищается от токсинов, уменьшается нагрузка на внутренние органы, снимаются неприятные симптомы абстиненции. Однако при тяжелом состоянии или наличии осложнений может потребоваться круглосуточное наблюдение в стационаре, где пациенту обеспечивается полная медицинская поддержка.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-14.ru/]narkologicheskaya klinika v-sankt-peterburge[/url]
Запой – это тяжелое состояние, возникающее из-за длительного и неконтролируемого употребления алкоголя. Оно характеризуется физической и психологической зависимостью, что приводит к серьезным последствиям для организма и психики. Для восстановления нормального самочувствия и здоровья необходимо профессиональное лечение. Одним из наиболее удобных и эффективных методов является вывод из запоя на дому.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-cena-v-ryazani/]vyvod iz zapoya cena narkologiya ryazan[/url]
Алкоголизм – одно из наиболее разрушительных заболеваний, наносящих серьезный ущерб организму. Запойные состояния особенно опасны, так как приводят к глубокой интоксикации, психическим нарушениям и необратимым последствиям. Чем раньше начато лечение, тем выше вероятность избежать осложнений и быстрее восстановиться.
Детальнее – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya s vyezdom na dom sankt-peterburg[/url]
В современном обществе проблема алкоголизма остаётся одной из самых острых, требуя профессионального и комплексного подхода к лечению. Наркологическая клиника “Трезвый Мир” в Воронеже и Воронежской области предлагает эффективное решение для тех, кто столкнулся с запоем и стремится вернуть себе ясность ума и здоровье. Одной из ключевых методик, используемых в нашей клинике, является капельница от запоя – безопасный и быстрый способ восстановить организм и начать путь к трезвости.
Получить дополнительные сведения – [url=https://kapelnica-ot-zapoya-voronezh3.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-voronezhe/]http://kapelnica-ot-zapoya-voronezh3.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-voronezhe[/url]
Выведение из запоя – это процесс, включающий несколько этапов. Симптомы могут варьироваться от тревожности и бессонницы до серьезных психических расстройств. Только профессиональный подход и современные медицинские технологии помогают минимизировать риски, стабилизировать состояние и избежать осложнений.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]вывод из запоя анонимно недорого санкт-петербург[/url]
Алкогольный запой представляет собой критическое состояние, требующее немедленного вмешательства квалифицированных специалистов. Клиника «Союз Здоровья» в Воронеже предлагает круглосуточную помощь, чтобы обеспечить своевременное и эффективное лечение. Наша цель — оперативное снятие интоксикации, устранение симптомов и предотвращение осложнений.
Выяснить больше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]narkolog vyvod iz zapoya cena [/url]
Наша миссия заключается в предоставлении качественной медицинской помощи тем, кто страдает от зависимостей. Мы стремимся создать безопасную и поддерживающую атмосферу, где каждый человек сможет получить необходимую помощь. Наша основная цель — это восстановление здоровья, психоэмоционального состояния и социальной адаптации.
Получить дополнительную информацию – [url=https://kapelnica-ot-zapoya-voronezh3.ru/kapelnica-ot-zapoya-anonimno-v-voronezhe/]kapelnica ot zapoya anonimno [/url]
Алкогольная зависимость представляет собой сложное и хроническое заболевание, которое влияет не только на физическое, но и на психологическое состояние пациента. Периоды запоя, влекущие за собой неконтролируемое употребление алкоголя, могут привести к опасным последствиям для здоровья и жизни. Абстинентные симптомы, такие как тремор, тошнота, головная боль и другие проявления, требуют немедленного медицинского вмешательства. В таких ситуациях важно не только быстро устранить физическое воздействие алкоголя, но и предоставить пациенту психологическую поддержку.
Получить больше информации – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-stacionare-v-krasnodare/]вывод из запоя в стационаре в краснодаре[/url]
Зависимость – это сложное испытание, с которым сталкиваются люди во всём мире. Ежедневные стрессы, проблемы в семье и на работе, финансовые трудности часто становятся почвой для развития пагубных привычек, таких как алкоголизм, наркомания и игромания. Медицинский центр «Второй Шанс» предоставляет помощь тем, кто хочет избавиться от этих состояний, вернуть здоровье и научиться жить без разрушительных зависимостей.
Подробнее тут – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]наркология вывод из запоя анонимно в санкт-петербурге[/url]
Запой — это тяжелое состояние, при котором человек теряет контроль над количеством употребляемого алкоголя, что приводит к серьезным нарушениям здоровья. Процесс вывода из запоя требует профессионального подхода, медицинского контроля и тщательного восстановления организма. Наркологическая клиника «Шаг к Трезвости» в Краснодаре предлагает квалифицированную помощь для тех, кто нуждается в срочном и безопасном выходе из запоя. Мы предоставляем комплексное лечение, ориентированное на каждый этап восстановления, с максимальной заботой о здоровье пациента.
Детальнее – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]vyvod iz zapoya kapelnica na domu v-krasnodare[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Узнать больше – [url=https://vyvod-iz-zapoya-11.ru/]вывод из запоя[/url]
Для тех, кто не может приехать в клинику, специалисты проводят детоксикацию на дому. С помощью современных препаратов организм мягко очищается от токсинов, уменьшается нагрузка на внутренние органы, снимаются неприятные симптомы абстиненции. Однако при тяжелом состоянии или наличии осложнений может потребоваться круглосуточное наблюдение в стационаре, где пациенту обеспечивается полная медицинская поддержка.
Разобраться лучше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]srochny vyvod iz zapoya na domu v-sankt-peterburge[/url]
Наркологическая клиника «Освобождение» в Рязани предлагает профессиональные услуги по выводу из запоя и комплексной реабилитации. Мы понимаем, что зависимость — это болезнь, требующая индивидуального подхода и поддержки не только для пациента, но и для его близких.
Детальнее – [url=https://vyvod-iz-zapoya-13.ru/]http://vyvod-iz-zapoya-13.ru[/url]
Запой – это тяжелое состояние, возникающее из-за длительного и неконтролируемого употребления алкоголя. Оно характеризуется физической и психологической зависимостью, что приводит к серьезным последствиям для организма и психики. Для восстановления нормального самочувствия и здоровья необходимо профессиональное лечение. Одним из наиболее удобных и эффективных методов является вывод из запоя на дому.
Детальнее – [url=https://vyvod-iz-zapoya-13.ru/]наркологическая клиника рязань[/url]
Запой — это состояние, характеризующееся утратой контроля над употреблением алкоголя, что приводит к тяжелой интоксикации организма. Вывод из запоя — это комплексный процесс, направленный на устранение последствий интоксикации и восстановление нормального функционирования организма. Цена данной процедуры может значительно различаться в зависимости от нескольких факторов, и в этой статье мы подробно рассмотрим, что влияет на стоимость вывода из запоя в Краснодаре, в том числе в клинике «Шаг к Трезвости».
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-12.ru/]vyvod iz zapoya s vyezdom v-krasnodare[/url]
Клиника «Освобождение» в Рязани предоставляет услугу круглосуточного вывода из запоя на дому, что позволяет пациенту получить медицинскую помощь в привычной обстановке, избегая лишнего стресса, связанного с госпитализацией.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]срочный вывод из запоя на дому в рязани[/url]
Проблема зависимостей остаётся актуальной в современном обществе. С каждым годом увеличивается число людей, страдающих от алкоголизма, наркомании и других форм зависимостей, что негативно отражается на их жизни и благополучии близких. Зависимость — это не просто физическое заболевание, но и глубокая психологическая проблема. Для эффективного лечения требуется помощь профессионалов, способных обеспечить комплексный подход.
Узнать больше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]вывод из запоя с выездом на дом в воронеже[/url]
Наша миссия заключается в предоставлении качественной медицинской помощи тем, кто страдает от зависимостей. Мы стремимся создать безопасную и поддерживающую атмосферу, где каждый человек сможет получить необходимую помощь. Наша основная цель — это восстановление здоровья, психоэмоционального состояния и социальной адаптации.
Ознакомиться с деталями – [url=https://kapelnica-ot-zapoya-voronezh3.ru/kapelnica-ot-zapoya-na-domu-v-voronezhe/]наркологический центр воронеж[/url]
Проблема зависимостей остаётся актуальной в современном обществе. С каждым годом увеличивается число людей, страдающих от алкоголизма, наркомании и других форм зависимостей, что негативно отражается на их жизни и благополучии близких. Зависимость — это не просто физическое заболевание, но и глубокая психологическая проблема. Для эффективного лечения требуется помощь профессионалов, способных обеспечить комплексный подход.
Выяснить больше – [url=https://vyvod-iz-zapoya-11.ru/]наркологический центр[/url]
В центре доступны различные варианты лечения – от амбулаторного наблюдения до интенсивных стационарных программ. Ключевые принципы работы – конфиденциальность, индивидуальный подход и оперативность оказания помощи. Независимо от тяжести зависимости, каждый пациент получает квалифицированную поддержку, направленную на восстановление здоровья и возвращение к полноценной жизни.
Детальнее – [url=https://vyvod-iz-zapoya-14.ru/]vyvod iz zapoya kapelnica[/url]
Алкогольный запой — это серьезное заболевание, которое требует немедленного и профессионального вмешательства. Запой сопровождается не только физическими страданиями, но и эмоциональными трудностями. Процесс выведения из запоя требует срочного внимания, так как состояние пациента может ухудшиться, если не получить квалифицированную помощь вовремя. Наркологическая клиника «Излечение» в Краснодаре предоставляет круглосуточную помощь при алкогольном запое, чтобы восстановить здоровье пациента в самых сложных ситуациях.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-cena-v-krasnodare/]вывод из запоя на дому цена в краснодаре[/url]
Для тех, кто не может приехать в клинику, специалисты проводят детоксикацию на дому. С помощью современных препаратов организм мягко очищается от токсинов, уменьшается нагрузка на внутренние органы, снимаются неприятные симптомы абстиненции. Однако при тяжелом состоянии или наличии осложнений может потребоваться круглосуточное наблюдение в стационаре, где пациенту обеспечивается полная медицинская поддержка.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-14.ru/]вывод из запоя санкт-петербург[/url]
В клинике “Трезвый Мир” доступны следующие виды помощи:
Получить дополнительную информацию – [url=https://kapelnica-ot-zapoya-voronezh3.ru/kapelnica-ot-zapoya-na-domu-v-voronezhe/]narkologicheski centr v voronezhe[/url]
Алкогольный запой — это серьезное заболевание, которое требует немедленного и профессионального вмешательства. Запой сопровождается не только физическими страданиями, но и эмоциональными трудностями. Процесс выведения из запоя требует срочного внимания, так как состояние пациента может ухудшиться, если не получить квалифицированную помощь вовремя. Наркологическая клиника «Излечение» в Краснодаре предоставляет круглосуточную помощь при алкогольном запое, чтобы восстановить здоровье пациента в самых сложных ситуациях.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]нарколог на дом вывод из запоя в краснодаре[/url]
Вывод из запоя представляет собой важный этап в процессе лечения алкогольной зависимости. Это медицинская процедура, необходимая для устранения токсических веществ, накопленных в организме в результате длительного употребления алкоголя. Алкогольная зависимость может вызывать множество проблем, включая физические и психоэмоциональные расстройства. Своевременное вмешательство специалистов помогает минимизировать риски и способствует восстановлению здоровья пациента.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-v-kruglosutochno-v-ryazani/]вывод из запоя круглосуточно наркология в рязани[/url]
Запойное состояние представляет собой серьёзную угрозу для здоровья, поскольку сопровождается тяжёлой интоксикацией организма и нарушением психоэмоционального состояния. Решение о лечении в стационаре клиники «Союз Здоровья» позволяет справиться с последствиями длительного употребления алкоголя, обеспечивая пациентам полный комплекс медицинской и психологической помощи.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-kruglosutochno-v-voronezhe/]вывод из запоя круглосуточно наркология [/url]
Лечение зависимости требует комплексного подхода, сочетающего медицинские методы и психологическую поддержку. Это не просто вредная привычка, а хроническое заболевание, требующее тщательного контроля и длительной терапии. В центре используются современные научно обоснованные методики, помогающие пациентам преодолеть сложный путь выздоровления. Каждому человеку подбирается индивидуальная программа, учитывающая его особенности и жизненные обстоятельства.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]анонимный вывод из запоя на дому [/url]
Современное общество сталкивается с серьёзными вызовами, связанными с зависимостями. Алкоголизм, как одна из самых распространённых проблем, оказывает разрушительное воздействие на здоровье человека, разрушая семейные отношения и затрудняя социальную адаптацию. В такие моменты крайне важно обратиться за квалифицированной помощью.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]вывод из запоя на дому недорого рязань[/url]
Алкогольный запой — это серьезное заболевание, которое требует немедленного и профессионального вмешательства. Запой сопровождается не только физическими страданиями, но и эмоциональными трудностями. Процесс выведения из запоя требует срочного внимания, так как состояние пациента может ухудшиться, если не получить квалифицированную помощь вовремя. Наркологическая клиника «Излечение» в Краснодаре предоставляет круглосуточную помощь при алкогольном запое, чтобы восстановить здоровье пациента в самых сложных ситуациях.
Выяснить больше – [url=https://vyvod-iz-zapoya-16.ru/]vyvod iz zapoya gorod krasnodar[/url]
Запой – это тяжелое состояние, возникающее из-за длительного и неконтролируемого употребления алкоголя. Оно характеризуется физической и психологической зависимостью, что приводит к серьезным последствиям для организма и психики. Для восстановления нормального самочувствия и здоровья необходимо профессиональное лечение. Одним из наиболее удобных и эффективных методов является вывод из запоя на дому.
Углубиться в тему – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]vyvod iz zapoya vrach na dom ryazan[/url]
Алкоголизм — это хроническое заболевание, которое характеризуется патологической зависимостью от этанола. Периоды запоя, когда человек неконтролируемо потребляет алкоголь, могут привести к разнообразным медицинским осложнениям, как физическим, так и психологическим. Запой становится не только причиной ухудшения здоровья, но и причиной множества социальных и личных проблем. На этом фоне возникает необходимость вывода из запоя, процесс, который должен учитывать индивидуальные особенности пациента. Важно, что анонимность в реабилитации играет ключевую роль, помогая избежать стигматизации и увеличивая шансы на успешное восстановление.
Детальнее – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-cena-v-ryazani/]vyvod iz zapoya s vyezdom cena [/url]
Алкогольный запой представляет собой критическое состояние, требующее немедленного вмешательства квалифицированных специалистов. Клиника «Союз Здоровья» в Воронеже предлагает круглосуточную помощь, чтобы обеспечить своевременное и эффективное лечение. Наша цель — оперативное снятие интоксикации, устранение симптомов и предотвращение осложнений.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-kruglosutochno-v-voronezhe/]вывод из запоя срочно круглосуточно в воронеже[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-kruglosutochno-v-voronezhe/]vyvod iz zapoya kruglosutochno voronezh[/url]
Запойное состояние представляет собой серьёзную угрозу для здоровья, поскольку сопровождается тяжёлой интоксикацией организма и нарушением психоэмоционального состояния. Решение о лечении в стационаре клиники «Союз Здоровья» позволяет справиться с последствиями длительного употребления алкоголя, обеспечивая пациентам полный комплекс медицинской и психологической помощи.
Детальнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]vyvod iz zapoya vrach na dom [/url]
В таких ситуациях вывод из запоя на дому становится оптимальным решением, особенно если пациент не может или не хочет посещать стационар. Эта услуга позволяет получить медицинскую помощь в комфортной и привычной обстановке, что снижает уровень стресса и ускоряет процесс восстановления.
Разобраться лучше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-kruglosutochno-v-voronezhe/]вывод из запоя круглосуточно [/url]
Only free access 18+ – https://t.me/+ijV3M3OHHIowNzcx
Bypairl
Алкогольный запой представляет собой критическое состояние, требующее немедленного вмешательства квалифицированных специалистов. Клиника «Союз Здоровья» в Воронеже предлагает круглосуточную помощь, чтобы обеспечить своевременное и эффективное лечение. Наша цель — оперативное снятие интоксикации, устранение симптомов и предотвращение осложнений.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]vyvod iz zapoya na domu nedorogo voronezh[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]вывод из запоя на дому [/url]
Проведение лечения в домашней обстановке значительно снижает уровень стресса и тревожности. Пациенту не нужно тратить силы на поездки в медицинские учреждения, что особенно важно при ограниченной мобильности. Домашняя атмосфера создает идеальные условия для открытого диалога с врачом, что существенно повышает эффективность терапии и шансы на успешное выздоровление.
Узнать больше – [url=https://narcolog-na-dom-msk55.ru/]https://narcolog-na-dom-msk55.ru/narkolog-na-dom-kruglosutochno-moskva[/url]
Ситуации, требующие вмешательства нарколога, могут быть различными. Наиболее распространенным поводом является состояние тяжелого алкогольного или наркотического опьянения, при котором пациент уже не может самостоятельно справляться с последствиями интоксикации. Это может быть вызвано передозировкой, длительным употреблением веществ или острой реакцией организма на токсичные соединения.
Выяснить больше – [url=https://narcolog-na-dom-v-moskve55.ru/]http://narcolog-na-dom-v-moskve55.ru/narkolog-na-dom-kruglosutochno-moskva/[/url]
Основой лечения становится индивидуальный подход. Врач-нарколог проводит диагностику, разрабатывает план терапии, подбирает необходимые препараты и внимательно следит за состоянием пациента на всех этапах. Учитывается не только физическое, но и психоэмоциональное состояние человека. Психологическая поддержка и мотивация на отказ от алкоголя играют важную роль, помогая закрепить результат и избежать рецидивов.
Узнать больше – [url=https://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-cena-v-voronezhe/]kapelnica ot zapoya anonimno nedorogo v voronezhe[/url]
Процедура необходима в случае появления симптомов алкогольной интоксикации, таких как тошнота, головная боль, слабость, тревожность или нарушения сна. Особенно важно провести детоксикацию, если у человека наблюдаются хронические заболевания, которые могут обостриться под воздействием токсинов.Процесс детоксикации с помощью капельницы существенно облегчает симптомы похмелья: головную боль, тошноту, слабость, беспокойство и другие неприятные проявления. В результате пациент начинает чувствовать себя значительно лучше в короткие сроки, и процесс восстановления проходит гораздо быстрее.
Ознакомиться с деталями – [url=https://kapelnica-ot-zapoya-krasnoyarsk55.ru/]после капельницы от запоя на дому красноярск[/url]
Когда речь идет о лечении зависимостей, многие пациенты испытывают стресс от визита в медицинские учреждения, особенно если это касается такой деликатной темы, как алкоголизм. Лечение на дому помогает избежать всех этих трудностей и обеспечивает комфорт для пациента. Привычная домашняя обстановка снижает тревожность, что позитивно влияет на процесс выздоровления.
Получить больше информации – [url=https://narcolog-na-dom-ektb55.ru/]нарколог на дом цены в екатеринбурге[/url]
Проблемы с алкоголем или наркотиками могут появиться в самый неподходящий момент, и зачастую требуется срочная помощь специалиста. Нередко необходим срочный вызов врача в следующих ситуациях:
Получить дополнительные сведения – [url=https://narcolog-na-dom-msk55.ru/]нарколог на дом москва[/url]
Нарколог на дом в Москве предоставляет комплексную помощь, адаптированную под каждого пациента:
Получить дополнительные сведения – [url=https://narcolog-na-dom-msk55.ru/]вызов нарколога на дом цена в москве[/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Детальнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-v-stacionare-v-chelyabinske/]быстрый вывод из запоя в стационаре челябинск[/url]
Для тех, кто по разным причинам не может посетить медицинский центр, существует возможность вызова нарколога на дом. Такая услуга позволяет получить необходимую помощь в комфортных условиях, сохраняя при этом полную анонимность. Опытные врачи проведут детоксикацию, назначат поддерживающую терапию и дадут рекомендации по дальнейшему восстановлению.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-v-stacionare-v-tveri/]вывод из запоя в стационаре тверь[/url]
Запойное состояние является крайней стадией зависимости, требующей немедленного медицинского вмешательства. В «Союзе Здоровья» организована круглосуточная помощь, что позволяет оперативно стабилизировать состояние пациента, минимизировать риск осложнений и облегчить процесс восстановления.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]вывод из запоя вызов на дом [/url]
Ритм жизни в мегаполисе и постоянный стресс часто становятся причиной развития зависимостей у жителей города. В такой ситуации необходима профессиональная консультация врача. Оптимальное решение – вызов нарколога на дом в Москве. Это обеспечивает не только получение медицинской помощи в привычной обстановке, но и полную конфиденциальность.
Выяснить больше – [url=https://narcolog-na-dom-msk55.ru/]вызов нарколога на дом цена москва[/url]
“Наркозависимость разрушила мою жизнь. Я потратила все силы, и не знала, что делать дальше. В клинике “Пульс жизни” я получила необходимую помощь. Программа лечения действительно работает, и я снова чувствую себя свободной.”— Мария, 29 лет
Исследовать вопрос подробнее – [url=https://kapelnica-ot-zapoya-voronezh2.ru/kapelnica-ot-zapoya-anonimno-v-voronezhe/]капельница от запоя срочно круглосуточно[/url]
Проведение лечения в домашней обстановке значительно снижает уровень стресса и тревожности. Пациенту не нужно тратить силы на поездки в медицинские учреждения, что особенно важно при ограниченной мобильности. Домашняя атмосфера создает идеальные условия для открытого диалога с врачом, что существенно повышает эффективность терапии и шансы на успешное выздоровление.
Разобраться лучше – [url=https://narcolog-na-dom-msk55.ru/]вызов нарколога на дом москва[/url]
В первую очередь это вывод из запоя, который проводится с применением современных инфузионных растворов. Процедура включает внутривенное введение препаратов, устраняющих абстиненцию, восстанавливающих водно-солевой баланс и нормализующих работу внутренних органов. Это помогает снять тревожность, нормализовать сон и восстановить общее состояние.
Подробнее можно узнать тут – [url=https://narcolog-na-dom-v-moskve55.ru/]вызов нарколога на дом москва[/url]
Для многих пациентов важным моментом является сохранение конфиденциальности. Врач, приехавший на дом, работает с полной защитой данных. Пациент может быть уверен, что его проблема останется личной и не станет достоянием общественности. Врачи приезжают на неприметных автомобилях и избегают всяческих ситуаций, которые могут привлечь лишнее внимание.
Подробнее – [url=https://narcolog-na-dom-moskva55.ru/]вызов нарколога ценамосква[/url]
Лечение может проходить как дома, так и в стационаре. Амбулаторный вариант удобен для тех, кто предпочитает находиться в привычной обстановке, тогда как стационарное лечение подходит для более сложных случаев, требующих постоянного медицинского наблюдения. В стационаре проводятся все необходимые исследования, включая анализы, ЭКГ и УЗИ, что позволяет минимизировать риски и ускорить восстановление.
Углубиться в тему – [url=https://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-voronezhe/]http://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-voronezhe[/url]
Проведение лечения в домашней обстановке значительно снижает уровень стресса и тревожности. Пациенту не нужно тратить силы на поездки в медицинские учреждения, что особенно важно при ограниченной мобильности. Домашняя атмосфера создает идеальные условия для открытого диалога с врачом, что существенно повышает эффективность терапии и шансы на успешное выздоровление.
Подробнее – [url=https://narcolog-na-dom-msk55.ru/]http://narcolog-na-dom-msk55.ru[/url]
Процесс детоксикации включает снятие абстиненции, очищение организма и стабилизацию его работы. На всех этапах лечения врач тщательно контролирует состояние пациента, подбирая эффективные препараты для восстановления. Психологическая помощь также важна для снижения уровня тревожности, преодоления депрессии и формирования устойчивой мотивации к трезвости.
Ознакомиться с деталями – [url=https://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-na-domu-v-voronezhe/]наркология капельница от запоя на дому[/url]
3.Уточните наличие акций и скидок. Многие клиники предлагают специальные условия для новых клиентов или на определенные виды услуг.
Разобраться лучше – [url=https://narcolog-na-dom-msk55.ru/]http://narcolog-na-dom-msk55.ru/narkolog-na-dom-kruglosutochno-moskva[/url]
Обращение к специалисту требуется в ситуациях, когда самостоятельное справление с проблемой становится невозможным или небезопасным. Одной из наиболее распространенных причин вызова нарколога является алкогольная интоксикация, связанная с запоем. В таких случаях состояние пациента может быть опасным, а попытки справиться с ситуацией без профессионального вмешательства чреваты осложнениями.
Изучить вопрос глубже – [url=https://narcolog-na-dom-v-moskve55.ru/]https://narcolog-na-dom-v-moskve55.ru/narkolog-na-dom-kruglosutochno-moskva/[/url]
Лечение может проходить как дома, так и в стационаре. Амбулаторный вариант удобен для тех, кто предпочитает находиться в привычной обстановке, тогда как стационарное лечение подходит для более сложных случаев, требующих постоянного медицинского наблюдения. В стационаре проводятся все необходимые исследования, включая анализы, ЭКГ и УЗИ, что позволяет минимизировать риски и ускорить восстановление.
Выяснить больше – [url=https://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-voronezhe/]http://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-voronezhe[/url]
Когда речь идет о лечении зависимостей, многие пациенты испытывают стресс от визита в медицинские учреждения, особенно если это касается такой деликатной темы, как алкоголизм. Лечение на дому помогает избежать всех этих трудностей и обеспечивает комфорт для пациента. Привычная домашняя обстановка снижает тревожность, что позитивно влияет на процесс выздоровления.
Подробнее – [url=https://narcolog-na-dom-ektb55.ru/]частный нарколог на дом екатеринбург[/url]
Процесс вызова нарколога достаточно прост и удобен для пациента и его близких.
Получить дополнительные сведения – [url=https://narcolog-na-dom-msk55.ru/]платный нарколог на дом москва[/url]
Лечение может проходить как дома, так и в стационаре. Амбулаторный вариант удобен для тех, кто предпочитает находиться в привычной обстановке, тогда как стационарное лечение подходит для более сложных случаев, требующих постоянного медицинского наблюдения. В стационаре проводятся все необходимые исследования, включая анализы, ЭКГ и УЗИ, что позволяет минимизировать риски и ускорить восстановление.
Получить дополнительную информацию – [url=https://kapelnica-ot-zapoya-voronezh.ru/]narkolog kapelnica ot zapoya cena v voronezhe[/url]
Запойное состояние представляет собой серьёзную угрозу для здоровья, поскольку сопровождается тяжёлой интоксикацией организма и нарушением психоэмоционального состояния. Решение о лечении в стационаре клиники «Союз Здоровья» позволяет справиться с последствиями длительного употребления алкоголя, обеспечивая пациентам полный комплекс медицинской и психологической помощи.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]narkolog na dom vyvod iz zapoya [/url]
Клиника “Трезвость и Надежда” оказывает профессиональную помощь в избавлении от запоев, как на дому, так и в стационарных условиях. Опытные специалисты используют современные методики, чтобы быстро очистить организм от токсинов и стабилизировать состояние пациента. В ходе процедуры капельного введения препаратов восстанавливается баланс жидкости и электролитов, нормализуется работа внутренних органов, а также устраняются основные симптомы похмельного синдрома: головная боль, тошнота, раздражительность.
Изучить вопрос глубже – [url=https://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-na-domu-v-voronezhe/]kapelnica ot zapoya na domu nedorogo[/url]
Команда “Трезвости и Надежды” предлагает комплексный подход, направленный на полноценное восстановление. Процедура капельницы становится первым шагом к избавлению от зависимости, помогая очистить организм, вернуть силы и начать жизнь с чистого листа.
Узнать больше – [url=https://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-anonimno-v-voronezhe/]kapelnica ot zapoya kruglosutochno narkologiya[/url]
В первую очередь это вывод из запоя, который проводится с применением современных инфузионных растворов. Процедура включает внутривенное введение препаратов, устраняющих абстиненцию, восстанавливающих водно-солевой баланс и нормализующих работу внутренних органов. Это помогает снять тревожность, нормализовать сон и восстановить общее состояние.
Ознакомиться с деталями – [url=https://narcolog-na-dom-v-moskve55.ru/]narkolog na dom [/url]
Клиника работает круглосуточно, предоставляя помощь в любое время. Стоимость услуг зависит от времени вызова и степени срочности, а ночные или праздничные выезды требуют дополнительных затрат. В особо сложных случаях капельница помогает не только снять интоксикацию, но и предотвратить возможные осложнения.
Детальнее – [url=https://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-voronezhe/]bystry kapelnica ot zapoya v stacionare voronezh[/url]
Зависимости продолжают оставаться одной из самых серьёзных проблем современности, затрагивая не только здоровье человека, но и его социальные связи. Алкоголизм и наркомания способны разрушить жизнь, приводя к тяжёлым последствиям, требующим профессионального вмешательства. Комплексный подход к лечению подобных состояний позволяет не только справиться с физическими проявлениями зависимости, но и восстановить психоэмоциональное равновесие.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]vyvod iz zapoya kapelnica na domu v-voronezhe[/url]
Еще одной причиной вызова нарколога может быть абстинентный синдром, возникающий после прекращения употребления алкоголя или наркотиков. Это состояние сопровождается физическими и психологическими проявлениями, такими как дрожь, тревога, бессонница, боли в мышцах.
Разобраться лучше – [url=https://narcolog-na-dom-v-moskve55.ru/]частный нарколог на дом в москве[/url]
Нарколог на дом в Москве предоставляет комплексную помощь, адаптированную под каждого пациента:
Узнать больше – [url=https://narcolog-na-dom-msk55.ru/]http://narcolog-na-dom-msk55.ru[/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-11.ru/]https://www.vyvod-iz-zapoya-11.ru[/url]
Алкогольная зависимость представляет собой актуальную медицинскую проблему, требующую тщательного подхода. Запой характеризуется длительным, непрерывным употреблением спиртных напитков, что ведёт к физической и психической зависимости. На фоне запойного состояния могут возникнуть тяжёлые нарушения функций органов, увеличивается риск развития таких состояний, как алкогольный делирий. Вывод из запоя становится ключевым этапом в лечении зависимостей, который может осуществляться как в стационарных условиях, так и на дому, в зависимости от состояния пациента. В клинике «Перекресток Надежды» в Челябинске мы предлагаем круглосуточную помощь, чтобы обеспечить максимально эффективное и безопасное лечение при любом варианте вывода из запоя.
Узнать больше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-cena-v-chelyabinske/]narkolog vyvod iz zapoya cena chelyabinsk[/url]
С увеличением темпа жизни и нарастающим стрессом, многие люди в Екатеринбурге сталкиваются с зависимостями, особенно с алкогольной. Алкогольная зависимость является одной из наиболее распространённых проблем, требующих немедленного вмешательства специалистов. Важно помнить, что в сложных ситуациях, связанных с зависимостью, помощь не должна откладываться. Вызов нарколога на дом в Екатеринбурге становится идеальным вариантом для тех, кто не может или не хочет покидать свой дом, но нуждается в медицинской помощи.
Подробнее можно узнать тут – [url=https://narcolog-na-dom-ektb55.ru/]нарколог на дом недорого екатеринбург[/url]
Для начала необходимо связаться с выбранной клиникой. Вызов нарколога на дом по телефону в Москве доступен любое время, так как большинство учреждений предлагают услуги круглосуточно. При звонке важно иметь под рукой информацию о состоянии пациента, чтобы оператор мог быстрее и точнее сориентироваться в ситуации. Зачастую клиники предлагают несколько номеров телефонов, чтобы минимизировать время ожидания на линии. Также некоторые учреждения предоставляют возможность заказа обратного звонка через официальный сайт, что особенно удобно, если линия перегружена.
Углубиться в тему – [url=https://narcolog-na-dom-msk55.ru/]выезд нарколога на дом цена москва[/url]
Команда “Трезвости и Надежды” предлагает комплексный подход, направленный на полноценное восстановление. Процедура капельницы становится первым шагом к избавлению от зависимости, помогая очистить организм, вернуть силы и начать жизнь с чистого листа.
Подробнее можно узнать тут – [url=https://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-na-domu-v-voronezhe/]kapelnica ot zapoya kapelnica na domu[/url]
Миссия клиники «Пульс жизни» заключается в предоставлении комплексной помощи пациентам с зависимостями. Мы уверены, что зависимость — это не приговор, а болезнь, которую можно и нужно лечить. «Пульс жизни» создаёт атмосферу, способствующую психологическому комфорту и эмоциональной поддержке. Каждый пациент получает индивидуальный подход, что является важным аспектом успешного лечения.
Получить больше информации – [url=https://kapelnica-ot-zapoya-voronezh2.ru/kapelnica-ot-zapoya-na-domu-v-voronezhe/]kapelnica ot zapoya v stacionare v voronezhe[/url]
Процесс вызова нарколога достаточно прост и удобен для пациента и его близких.
Ознакомиться с деталями – [url=https://narcolog-na-dom-msk55.ru/]выезд нарколога на дом цена в москве[/url]
Лечение может проходить как дома, так и в стационаре. Амбулаторный вариант удобен для тех, кто предпочитает находиться в привычной обстановке, тогда как стационарное лечение подходит для более сложных случаев, требующих постоянного медицинского наблюдения. В стационаре проводятся все необходимые исследования, включая анализы, ЭКГ и УЗИ, что позволяет минимизировать риски и ускорить восстановление.
Узнать больше – [url=https://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-na-domu-v-voronezhe/]срочная капельница от запоя на дому воронеж[/url]
Запой – это состояние, которое возникает вследствие длительного употребления алкоголя, приводящее к потере контроля над количеством выпиваемого и формированию как физической, так и психологической зависимости. Оно сопровождается серьёзными последствиями для здоровья и требует немедленного вмешательства.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]вывод из запоя цена наркология воронеж[/url]
Проблема зависимостей остаётся актуальной в современном обществе. С каждым годом увеличивается число людей, страдающих от алкоголизма, наркомании и других форм зависимостей, что негативно отражается на их жизни и благополучии близких. Зависимость — это не просто физическое заболевание, но и глубокая психологическая проблема. Для эффективного лечения требуется помощь профессионалов, способных обеспечить комплексный подход.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-kruglosutochno-v-voronezhe/]вывод из запоя срочно круглосуточно воронеж[/url]
Основой лечения становится индивидуальный подход. Врач-нарколог проводит диагностику, разрабатывает план терапии, подбирает необходимые препараты и внимательно следит за состоянием пациента на всех этапах. Учитывается не только физическое, но и психоэмоциональное состояние человека. Психологическая поддержка и мотивация на отказ от алкоголя играют важную роль, помогая закрепить результат и избежать рецидивов.
Изучить вопрос глубже – [url=https://kapelnica-ot-zapoya-voronezh.ru/]капельница от запоя на дому цена воронеж[/url]
Когда речь идет о лечении зависимостей, многие пациенты испытывают стресс от визита в медицинские учреждения, особенно если это касается такой деликатной темы, как алкоголизм. Лечение на дому помогает избежать всех этих трудностей и обеспечивает комфорт для пациента. Привычная домашняя обстановка снижает тревожность, что позитивно влияет на процесс выздоровления.
Ознакомиться с деталями – [url=https://narcolog-na-dom-ektb55.ru/]нарколог в екатеринбурге[/url]
Многие люди боятся обращаться за помощью, опасаясь нарушения конфиденциальности. В клинике строго соблюдаются правила анонимности, что создаёт атмосферу безопасности и доверия. Высокий уровень профессионализма специалистов и индивидуальный подход к каждому пациенту способствуют комфортному и эффективному лечению.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]нарколог на дом вывод из запоя воронеж[/url]
Алкогольная зависимость остается одной из самых серьезных проблем современного общества. Когда человек сталкивается с последствиями длительного употребления спиртных напитков, важно вовремя принять меры, чтобы минимизировать вред для организма. В таких случаях капельница от запоя становится эффективным инструментом для снятия интоксикации и восстановления здоровья.Процедура необходима в случае появления симптомов алкогольной интоксикации, таких как тошнота, головная боль, слабость, тревожность или нарушения сна. Особенно важно провести детоксикацию, если у человека наблюдаются хронические заболевания, которые могут обостриться под воздействием токсинов.
Подробнее – [url=https://kapelnica-ot-zapoya-krasnoyarsk55.ru/]капельница от запоя на дому в красноярске[/url]
Ритм жизни в мегаполисе и постоянный стресс часто становятся причиной развития зависимостей у жителей города. В такой ситуации необходима профессиональная консультация врача. Оптимальное решение – вызов нарколога на дом в Москве. Это обеспечивает не только получение медицинской помощи в привычной обстановке, но и полную конфиденциальность.
Получить больше информации – [url=https://narcolog-na-dom-msk55.ru/]вызов нарколога на дом цена москва[/url]
Когда речь идет о лечении зависимостей, многие пациенты испытывают стресс от визита в медицинские учреждения, особенно если это касается такой деликатной темы, как алкоголизм. Лечение на дому помогает избежать всех этих трудностей и обеспечивает комфорт для пациента. Привычная домашняя обстановка снижает тревожность, что позитивно влияет на процесс выздоровления.
Подробнее – [url=https://narcolog-na-dom-ektb55.ru/]нарколог екатеринбург[/url]
Лечение может проходить как дома, так и в стационаре. Амбулаторный вариант удобен для тех, кто предпочитает находиться в привычной обстановке, тогда как стационарное лечение подходит для более сложных случаев, требующих постоянного медицинского наблюдения. В стационаре проводятся все необходимые исследования, включая анализы, ЭКГ и УЗИ, что позволяет минимизировать риски и ускорить восстановление.
Подробнее можно узнать тут – [url=https://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-voronezhe/]bystry kapelnica ot zapoya v stacionare voronezh[/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya na domu nedorogo kapelnica v-chelyabinske[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-11.ru/]https://www.vyvod-iz-zapoya-11.ru[/url]
Запойное состояние представляет собой серьёзную угрозу для здоровья, поскольку сопровождается тяжёлой интоксикацией организма и нарушением психоэмоционального состояния. Решение о лечении в стационаре клиники «Союз Здоровья» позволяет справиться с последствиями длительного употребления алкоголя, обеспечивая пациентам полный комплекс медицинской и психологической помощи.
Узнать больше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-stacionare-v-voronezhe/]bystry vyvod iz zapoya v stacionare [/url]
Наркологическая клиника “Пульс жизни” в Воронеже предлагает услугу капельницы от запоя на дому, которая представляет собой эффективный и безопасный способ вывода пациента из состояния алкогольного отравления. Процедура не только помогает облегчить физическое состояние, но и способствует восстановлению психоэмоционального фона, что крайне важно для успешной реабилитации.
Разобраться лучше – [url=https://kapelnica-ot-zapoya-voronezh2.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-voronezhe/]http://www.kapelnica-ot-zapoya-voronezh2.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-voronezhe[/url]
Алкогольная зависимость – это серьезное заболевание, которое затрагивает как тело, так и психику. Особую опасность представляет запой, при котором длительное употребление алкоголя приводит к сильнейшей интоксикации организма. В таких ситуациях капельное введение препаратов становится жизненно необходимым.
Разобраться лучше – [url=https://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-na-domu-v-voronezhe/]kapelnica ot zapoya vyzov na dom[/url]
Вывод из запоя – это не просто разовое медицинское вмешательство, а важный шаг на пути к полному избавлению от алкогольной зависимости. Комплексный подход, включающий не только устранение симптомов интоксикации, но и последующую психологическую реабилитацию, позволяет добиться устойчивого результата и вернуть человека к полноценной жизни.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-v-stacionare-v-tveri/]вывод из запоя в стационаре анонимно в твери[/url]
Жизнь в мегаполисе, таком как Москва, предъявляет к человеку множество требований и вызовов. В условиях постоянного стресса и давления вероятность столкнуться с проблемами, связанными с алкоголизмом или наркоманией, возрастает. В таких ситуациях вызов нарколога на дом в Москве становится не роскошью, а необходимостью. В этой статье рассмотрим, когда и как можно воспользоваться этой услугой, а также разберем стоимость и основные преимущества.
Ознакомиться с деталями – [url=https://narcolog-na-dom-msk55.ru/]нарколог на дом в москве[/url]
Запой — это серьёзное состояние, вызванное длительным употреблением алкоголя и сопровождающееся утратой контроля над своим поведением. Оно несёт угрозу как для организма, так и для психики, а потому требует своевременного медицинского вмешательства. Для удобства пациентов предусмотрена возможность оказания помощи на дому, что снижает уровень стресса и позволяет провести процедуру в привычной обстановке.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-kruglosutochno-v-voronezhe/]vyvod iz zapoya srochno kruglosutochno voronezh[/url]
Злоупотребление алкоголем опасно не только физическими последствиями, но и разрушительным воздействием на психику. Лечение в стационаре даёт возможность не только справиться с интоксикацией, но и получить полноценную поддержку специалистов. Благодаря комплексному подходу пациент получает не только медицинскую помощь, но и психологическую поддержку, что повышает эффективность реабилитации.
Углубиться в тему – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]нарколог вывод из запоя цена в воронеже[/url]
Наркологическая помощь на дому необходима в ряде случаев, связанных с острыми состояниями пациента или невозможностью посетить клинику. Часто это ситуации, когда человек испытывает тяжелые последствия запоя, сильную интоксикацию или симптомы, угрожающие здоровью.
Детальнее – [url=https://narcolog-na-dom-moskva55.ru/]платный нарколог на дом в москве[/url]
Процедура необходима в случае появления симптомов алкогольной интоксикации, таких как тошнота, головная боль, слабость, тревожность или нарушения сна. Особенно важно провести детоксикацию, если у человека наблюдаются хронические заболевания, которые могут обостриться под воздействием токсинов.Эта процедура доступна как в условиях клиники, так и на дому, что делает ее универсальным решением для различных жизненных ситуаций. При этом важно доверять свое здоровье только профессиональным врачам, которые могут обеспечить безопасное и качественное лечение.
Углубиться в тему – [url=https://kapelnica-ot-zapoya-krasnoyarsk55.ru/]после капельницы от запоя красноярск[/url]
Алкогольная зависимость представляет собой актуальную медицинскую проблему, требующую тщательного подхода. Запой характеризуется длительным, непрерывным употреблением спиртных напитков, что ведёт к физической и психической зависимости. На фоне запойного состояния могут возникнуть тяжёлые нарушения функций органов, увеличивается риск развития таких состояний, как алкогольный делирий. Вывод из запоя становится ключевым этапом в лечении зависимостей, который может осуществляться как в стационарных условиях, так и на дому, в зависимости от состояния пациента. В клинике «Перекресток Надежды» в Челябинске мы предлагаем круглосуточную помощь, чтобы обеспечить максимально эффективное и безопасное лечение при любом варианте вывода из запоя.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-17.ru/]vyvod iz zapoya kapelnica chelyabinsk[/url]
Процедура необходима в случае появления симптомов алкогольной интоксикации, таких как тошнота, головная боль, слабость, тревожность или нарушения сна. Особенно важно провести детоксикацию, если у человека наблюдаются хронические заболевания, которые могут обостриться под воздействием токсинов.Одним из наиболее удобных вариантов лечения является проведение процедуры в домашних условиях. Это особенно актуально для пациентов, которые чувствуют себя слишком плохо, чтобы отправиться в клинику, или желают сохранить анонимность.
Детальнее – [url=https://kapelnica-ot-zapoya-krasnoyarsk55.ru/]капельница от запоя стоимость в красноярске[/url]
Стоимость услуги формируется на основе различных факторов, включая сложность состояния пациента, срочность вызова, квалификацию врача и расстояние до места вызова. Чтобы сделать правильный выбор, важно учитывать все аспекты, влияющие на цену, и понимать, как получить качественную помощь по справедливой стоимости.
Узнать больше – [url=https://narcolog-na-dom-ektb55.ru/]врач нарколог на дом платный екатеринбург[/url]
Запой — это тяжелое состояние, возникающее вследствие длительного и непрерывного употребления алкоголя, которое сопровождается физической и психической зависимостью. Это опасное явление требует незамедлительного вмешательства специалистов. Вывод из запоя — это комплексный процесс медицинской детоксикации, который включает в себя очищение организма от токсинов, нормализацию функций внутренних органов и поддержку психоэмоционального состояния пациента. В случае тяжелого запоя стационарное лечение становится оптимальным решением для безопасного и эффективного вывода из этого состояния. В этой статье мы рассмотрим, как проходит вывод из запоя в стационаре клиники «Перекресток Надежды» в Челябинске, а также особенности анонимности, скорости лечения и участия нарколога.
Разобраться лучше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя на дому круглосуточно [/url]
Website [url=https://tlo-lookup.com]ATM[/url]
Капельница от запоя – это метод внутривенного введения специальных растворов, направленных на восстановление водно-электролитного баланса организма, выведение токсинов и снижение симптомов абстинентного синдрома. Эта процедура позволяет быстро стабилизировать состояние пациента, ускорить процессы детоксикации и предотвратить возможные осложнения, связанные с длительным употреблением алкоголя.
Детальнее – [url=https://kapelnica-ot-zapoya-voronezh2.ru/]kapelnica ot zapoya anonimno v voronezhe[/url]
Детоксикация организма представляет собой комплексное очищение от токсинов. Для этого используются инфузионные растворы, содержащие витамины, антиоксиданты и препараты для поддержки печени. Процедура помогает снизить нагрузку на органы и улучшить общее самочувствие.
Подробнее тут – [url=https://narcolog-na-dom-ektb55.ru/]narcolog-na-dom-ektb55.ru/[/url]
Запой – это не просто временное состояние, а серьёзная медицинская проблема, требующая своевременного вмешательства. Капельница от запоя в клинике «Пульс жизни» – один из самых эффективных методов восстановления, который помогает решить сразу несколько проблем:
Углубиться в тему – [url=https://kapelnica-ot-zapoya-voronezh2.ru/kapelnica-ot-zapoya-cena-v-voronezhe/]нарколог на дом капельница от запоя[/url]
Для эффективного и безопасного выхода из запоя необходимо квалифицированное лечение, включающее детоксикацию организма, восстановление работы внутренних органов и поддержку психоэмоционального состояния. В этом случае важно обратиться к специалистам, которые смогут подобрать оптимальные методы терапии, исходя из состояния пациента. Один из наиболее эффективных способов снятия алкогольной интоксикации – это внутривенная инфузионная терапия (капельницы), позволяющая быстро очистить организм от токсинов, восстановить водно-солевой баланс и улучшить общее самочувствие.
Углубиться в тему – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-anonimno-v-tveri/]vyvod iz zapoya anonimno nedorogo v-tveri[/url]
Процесс детоксикации с помощью капельницы существенно облегчает симптомы похмелья: головную боль, тошноту, слабость, беспокойство и другие неприятные проявления. В результате пациент начинает чувствовать себя значительно лучше в короткие сроки, и процесс восстановления проходит гораздо быстрее.Алкогольная зависимость остается одной из самых серьезных проблем современного общества. Когда человек сталкивается с последствиями длительного употребления спиртных напитков, важно вовремя принять меры, чтобы минимизировать вред для организма. В таких случаях капельница от запоя становится эффективным инструментом для снятия интоксикации и восстановления здоровья.
Исследовать вопрос подробнее – [url=https://kapelnica-ot-zapoya-krasnoyarsk55.ru/]после капельницы от запоя на дому в красноярске[/url]
Проведение лечения в домашней обстановке значительно снижает уровень стресса и тревожности. Пациенту не нужно тратить силы на поездки в медицинские учреждения, что особенно важно при ограниченной мобильности. Домашняя атмосфера создает идеальные условия для открытого диалога с врачом, что существенно повышает эффективность терапии и шансы на успешное выздоровление.
Получить больше информации – [url=https://narcolog-na-dom-msk55.ru/]https://narcolog-na-dom-msk55.ru/[/url]
[url=https://midnight.im/store/chity-cs-1-6/]скачать приватные читы для кс 1.6[/url] – чит на кс го скачать, купить приватный чит для кс го
Запой – это состояние, которое возникает вследствие длительного употребления алкоголя, приводящее к потере контроля над количеством выпиваемого и формированию как физической, так и психологической зависимости. Оно сопровождается серьёзными последствиями для здоровья и требует немедленного вмешательства.
Углубиться в тему – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-anonimno-v-voronezhe/]вывод из запоя анонимно [/url]
Запойное состояние представляет собой серьёзную угрозу для здоровья, поскольку сопровождается тяжёлой интоксикацией организма и нарушением психоэмоционального состояния. Решение о лечении в стационаре клиники «Союз Здоровья» позволяет справиться с последствиями длительного употребления алкоголя, обеспечивая пациентам полный комплекс медицинской и психологической помощи.
Получить больше информации – [url=https://vyvod-iz-zapoya-11.ru/]vyvod iz zapoya gorod voronezh[/url]
More Help
[url=https://galaxy-swapper.org/]galaxy swapper[/url]
[url=https://betslive.ru/twin-casino-promokod/]Приветственные бонусы по промокоду казино Twin[/url] – Хани мани казино промокод, Покерок бонусный код при регистрации
Read More Here [url=https://tlo-ssndob.com]TLO lookup[/url]
Смотреть здесь https://nn.benevobis.su/price/
Алкогольная зависимость — это тяжелое состояние, требующее срочного медицинского вмешательства. В столице растет число пациентов, предпочитающих получать медицинскую помощь в домашних условиях, и капельница от запоя на дому в Москве становится востребованным и результативным методом лечения. Данный подход позволяет эффективно восстановить организм пациента в привычной обстановке без необходимости пребывания в больнице.
Выяснить больше – [url=https://kapelnica-ot-zapoya-msk55.ru/]капельница от запоя стоимость москва[/url]
Алкогольная зависимость — комплексная проблема, которая нуждается в квалифицированном лечении. В Красноярске функционирует широкая сеть медицинских учреждений, готовых оказать поддержку тем, кто столкнулся с этой проблемой. Вывод из запоя в Красноярске осуществляется как в клинических условиях, так и при выезде специалистов на дом, что дает возможность подобрать оптимальный вариант лечения для каждого пациента.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]http://vyvod-iz-zapoya-krasnoyarsk55.ru/vyvod-iz-zapoya-na-domu-krasnoyarsk/[/url]
читать https://nsk.benevobis.su/services/bronkhialnaya-astma/
Медикаментозное лечение — это еще один важный этап. Оно включает использование препаратов, которые помогают пациенту восстановиться как физически, так и психологически. Нарколог контролирует процесс лечения, чтобы исключить побочные эффекты и скорректировать терапию при необходимости.
Детальнее – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]narcolog-na-dom-v-krasnoyarske55.ru/[/url]
Зависимости становятся всё более актуальной проблемой нашего времени, влияя не только на физическое, но и на психическое здоровье. Количество людей, страдающих от алкоголизма, наркозависимости и других форм зависимости, растёт с каждым годом, разрушая их жизнь и причиняя вред близким. Для эффективного решения этой проблемы необходим профессиональный подход, охватывающий все аспекты восстановления.
Выяснить больше – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]вывод из запоя срочно круглосуточно [/url]
Услуга вызова нарколога на дом в Красноярске — это удобный и современный способ получения медицинской помощи. Она идеально подходит для тех, кто нуждается в профессиональной поддержке, но по каким-либо причинам не может или не хочет посещать клинику.
Детальнее – [url=https://narcolog-na-dom-krasnoyarsk55.ru/]narkolog na dom krasnoyarsk[/url]
Стационарное лечение обеспечивает постоянный контроль медицинского персонала, что гарантирует максимальную безопасность и мониторинг состояния пациента. Это критически важно для пациентов с сопутствующими заболеваниями или осложнениями алкоголизма. Клиники также предоставляют дополнительные услуги: консультации психолога, групповые и индивидуальные терапевтические занятия, способствующие скорейшему выздоровлению и профилактике рецидивов.
Разобраться лучше – [url=https://kapelnica-ot-zapoya-msk55.ru/]стоимость капельницы от запоя в москве[/url]
Алкогольная зависимость — это серьезное заболевание, которое затрагивает не только физическое здоровье, но и психику. Особенно опасен запой, при котором длительное употребление алкоголя вызывает сильную интоксикацию организма. В таких случаях капельница становится жизненно необходимой процедурой.
Ознакомиться с деталями – [url=https://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-cena-v-voronezhe/]анонимная капельница от запоя на дому воронеж[/url]
Алкогольный запой представляет собой критическое состояние, требующее немедленного вмешательства квалифицированных специалистов. Клиника «Союз Здоровья» в Воронеже предлагает круглосуточную помощь, чтобы обеспечить своевременное и эффективное лечение. Наша цель — оперативное снятие интоксикации, устранение симптомов и предотвращение осложнений.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]narkologiya vyvod iz zapoya na domu v-voronezhe[/url]
Клиника «Преображение» предлагает услуги анонимного вывода из запоя в Екатеринбурге, обеспечивая высокое качество медицинской помощи и соблюдение всех этических норм.
Детальнее – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-anonimno-v-ekaterinburge/]наркология вывод из запоя анонимно [/url]
Врач-нарколог проводит комплексное обследование пациента, оценивая степень зависимости и общее состояние организма. В ходе первичного осмотра специалист не только определяет тяжесть алкогольной зависимости, но и выявляет сопутствующие патологии, которые могут повлиять на процесс лечения. Обследование включает лабораторные анализы, электрокардиограмму и другие необходимые диагностические процедуры. Врач тщательно изучает анамнез для выявления возможных противопоказаний к определенным методам терапии. Особое внимание уделяется психологическим аспектам, которые могли способствовать формированию зависимости, что помогает разработать эффективную программу лечения.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]вывод из запоя цена красноярск[/url]
Выведение из запоя возможно как в условиях стационара, так и при выезде специалиста на дом. Стоимость этих вариантов лечения может существенно отличаться.
Детальнее – [url=https://kapelnica-ot-zapoya-msk55.ru/]после капельницы от запоя на дому в москве[/url]
Отсутствует потребность в длительных поездках в медицинские учреждения или ожидании приема в специализированных центрах. Более того, стоимость домашнего лечения часто оказывается значительно ниже по сравнению с пребыванием в стационаре. Лечение на дому позволяет избежать дополнительных затрат на транспортировку и проживание в медицинских учреждениях. Это приобретает особую значимость для жителей удаленных районов, где доступ к стационарному лечению может быть существенно ограничен. Кроме того, получение медицинской помощи в домашних условиях дает возможность пациенту сохранить привычный рабочий график, что существенно снижает финансовые потери. Выезд специалиста предполагает удобные схемы оплаты и гибкие финансовые условия, делая услугу доступной для различных категорий населения.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]вывод из запоя в красноярске[/url]
Такая терапия помогает подготовить организм к дальнейшему лечению от зависимости, улучшить физическое состояние и предотвратить развитие осложнений, таких как обезвоживание или нарушение работы внутренних органов.
Подробнее тут – [url=https://kapelnica-ot-zapoya-voronezh2.ru/]anonimnaya kapelnica ot zapoya na domu [/url]
Оптимальный вариант получить помощь круглосуточно анонимно в привычной обстановке. Цены капельницы от запоя на дому в Москве составляют от 7 000 до 20 000 руб., учитывая расстояние и время вызова.
Получить дополнительные сведения – [url=https://kapelnica-ot-zapoya-msk55.ru/]капельницы от запоя в москве[/url]
Запой — это тяжелое состояние, требующее комплексного медицинского вмешательства. Он может приводить к различным нарушениям здоровья, как физическим, так и психоэмоциональным. Стационарное лечение в нашей клинике является эффективным способом помочь пациенту вернуться к нормальной жизни, обеспечивая комфортные условия и круглосуточную поддержку специалистов.
Получить больше информации – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]вывод из запоя на дому [/url]
Алкогольная зависимость — это тяжелое состояние, требующее срочного медицинского вмешательства. В столице растет число пациентов, предпочитающих получать медицинскую помощь в домашних условиях, и капельница от запоя на дому в Москве становится востребованным и результативным методом лечения. Данный подход позволяет эффективно восстановить организм пациента в привычной обстановке без необходимости пребывания в больнице.
Получить дополнительную информацию – [url=https://kapelnica-ot-zapoya-msk55.ru/]после капельницы от запоя москва[/url]
Многие пациенты интересуются возможностью быстрого вывода из запоя в стационаре Красноярска. Современные медицинские учреждения используют инновационные методики и препараты, существенно ускоряющие процесс выздоровления. Ключевые достоинства ускоренного лечения:
Подробнее тут – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]http://vyvod-iz-zapoya-krasnoyarsk55.ru/vyvod-iz-zapoya-krasnoyarsk-kruglosutochno[/url]
Алкогольная зависимость — комплексная проблема, которая нуждается в квалифицированном лечении. В Красноярске функционирует широкая сеть медицинских учреждений, готовых оказать поддержку тем, кто столкнулся с этой проблемой. Вывод из запоя в Красноярске осуществляется как в клинических условиях, так и при выезде специалистов на дом, что дает возможность подобрать оптимальный вариант лечения для каждого пациента.
Детальнее – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]http://vyvod-iz-zapoya-krasnoyarsk55.ru/vyvod-iz-zapoya-krasnoyarsk-kruglosutochno[/url]
Запой — это опасное состояние, возникающее вследствие длительного алкоголизма, когда человек теряет контроль над собой, а зависимость становится всё сильнее. Это сопровождается серьёзной интоксикацией и нарушениями психоэмоционального состояния. Быстрое вмешательство профессионалов позволяет минимизировать вред для организма и вернуть пациента к нормальной жизни.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]нарколог на дом вывод из запоя в краснодаре[/url]
Для тех, кто не готов или не может пройти стационарное лечение, есть возможность получения медицинской помощи на дому. Это помогает уменьшить стресс, так как пациент остаётся в привычной обстановке. Тем не менее, в более тяжёлых случаях лечение в клинике является наилучшим выбором, так как предоставляет доступ ко всем необходимым медицинским и психологическим ресурсам.
Узнать больше – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-cena-v-krasnodare/]narkolog vyvod iz zapoya cena [/url]
Капельница от запоя — это терапевтическая процедура, обеспечивающая очищение организма, элиминацию токсических веществ и возвращение пациента к нормальному состоянию после алкогольной интоксикации. В состав капельницы входят следующие компоненты:
Детальнее – [url=https://kapelnica-ot-zapoya-msk55.ru/]стоимость капельницы от запоя москва[/url]
Процесс лечения алкогольной зависимости состоит из последовательных этапов, каждый из которых критически важен для успешного выздоровления.
Подробнее тут – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]вывод из запоя стационар красноярск[/url]
Запой — это опасное состояние, возникающее вследствие длительного алкоголизма, когда человек теряет контроль над собой, а зависимость становится всё сильнее. Это сопровождается серьёзной интоксикацией и нарушениями психоэмоционального состояния. Быстрое вмешательство профессионалов позволяет минимизировать вред для организма и вернуть пациента к нормальной жизни.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-cena-v-krasnodare/]вывод из запоя цена наркология в краснодаре[/url]
Для тех, кто не готов или не может пройти стационарное лечение, есть возможность получения медицинской помощи на дому. Это помогает уменьшить стресс, так как пациент остаётся в привычной обстановке. Тем не менее, в более тяжёлых случаях лечение в клинике является наилучшим выбором, так как предоставляет доступ ко всем необходимым медицинским и психологическим ресурсам.
Получить больше информации – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]вывод из запоя срочно круглосуточно краснодар[/url]
[url=https://midnight.im/store/chity-cs-go/]cs go приватный чит[/url] – cs go приватные читы, купить приват читы для кс го
Процесс лечения алкогольной зависимости состоит из последовательных этапов, каждый из которых критически важен для успешного выздоровления.
Узнать больше – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]http://vyvod-iz-zapoya-krasnoyarsk55.ru/vyvod-iz-zapoya-na-domu-krasnoyarsk[/url]
Специалист проводит тщательное обследование, включающее оценку общего состояния и сбор анамнеза для определения степени интоксикации. Измеряются основные показатели: артериальное давление, частота пульса, уровень насыщения крови кислородом и другие жизненно важные параметры. Врач собирает подробную информацию о длительности употребления алкоголя, его количестве, наличии хронических заболеваний и возможных аллергических реакциях на лекарства. Эти данные позволяют оценить тяжесть интоксикации и предвидеть потенциальные осложнения. У пациентов могут наблюдаться признаки абстинентного синдрома: дрожь, повышенное потоотделение, беспокойство и даже галлюцинаторные явления, требующие особого медицинского контроля.
Подробнее можно узнать тут – [url=https://kapelnica-ot-zapoya-msk55.ru/]капельница от запоя в москве[/url]
Одной из самых востребованных процедур является снятие абстинентного синдрома. Это состояние сопровождается сильными физическими и психологическими симптомами, такими как тревожность, тремор, головная боль и бессонница. Врач, прибывший на вызов, проводит диагностику состояния пациента и подбирает необходимые препараты. Вводятся инфузионные растворы с витаминами, седативными и противорвотными средствами.
Изучить вопрос глубже – [url=https://narcolog-na-dom-krasnoyarsk55.ru/]нарколог красноярск[/url]
Алкогольная зависимость — комплексная проблема, которая нуждается в квалифицированном лечении. В Красноярске функционирует широкая сеть медицинских учреждений, готовых оказать поддержку тем, кто столкнулся с этой проблемой. Вывод из запоя в Красноярске осуществляется как в клинических условиях, так и при выезде специалистов на дом, что дает возможность подобрать оптимальный вариант лечения для каждого пациента.
Детальнее – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]вывод из запоя на дому в красноярске[/url]
Капельница от запоя — это терапевтическая процедура, обеспечивающая очищение организма, элиминацию токсических веществ и возвращение пациента к нормальному состоянию после алкогольной интоксикации. В состав капельницы входят следующие компоненты:
Получить дополнительные сведения – [url=https://kapelnica-ot-zapoya-msk55.ru/]капельница от запоя москва[/url]
Преодоление запоя — это длительный и сложный процесс, который требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными, от нервозности до тяжёлых нарушений работы органов. Чем раньше пациент получит помощь, тем быстрее он вернётся к нормальной жизни и минимизирует возможные риски для здоровья.
Детальнее – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]vyvod iz zapoya vrach na dom [/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Получить больше информации – [url=https://vyvod-iz-zapoya-11.ru/]наркологический центр[/url]
Зависимости становятся всё более актуальной проблемой нашего времени, влияя не только на физическое, но и на психическое здоровье. Количество людей, страдающих от алкоголизма, наркозависимости и других форм зависимости, растёт с каждым годом, разрушая их жизнь и причиняя вред близким. Для эффективного решения этой проблемы необходим профессиональный подход, охватывающий все аспекты восстановления.
Получить больше информации – [url=https://vyvod-iz-zapoya-12.ru/]narkologicheskaya klinika v-krasnodare[/url]
Преодоление запоя — это длительный и сложный процесс, который требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными, от нервозности до тяжёлых нарушений работы органов. Чем раньше пациент получит помощь, тем быстрее он вернётся к нормальной жизни и минимизирует возможные риски для здоровья.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-12.ru/]наркологический центр краснодар[/url]
Клиника работает круглосуточно, обеспечивая помощь в любое время. Стоимость услуг зависит от времени вызова и срочности. Ночные или праздничные выезды могут потребовать дополнительных затрат. В сложных случаях капельница не только помогает избавиться от интоксикации, но и предотвращает возможные осложнения.
Углубиться в тему – [url=https://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-v-stacionare-v-voronezhe/]narkologicheskaya klinika voronezh[/url]
Для купирования симптомов и профилактики рецидивов врач подбирает индивидуальные препараты, которые могут включать седативные средства, витамины и противосудорожные препараты. Лечение проводится под строгим контролем специалиста, который следит за реакцией пациента и при необходимости корректирует терапию.
Получить дополнительную информацию – [url=https://narcolog-na-dom-krasnoyarsk55.ru/]https://narcolog-na-dom-krasnoyarsk55.ru/[/url]
Специалист проводит тщательное обследование, включающее оценку общего состояния и сбор анамнеза для определения степени интоксикации. Измеряются основные показатели: артериальное давление, частота пульса, уровень насыщения крови кислородом и другие жизненно важные параметры. Врач собирает подробную информацию о длительности употребления алкоголя, его количестве, наличии хронических заболеваний и возможных аллергических реакциях на лекарства. Эти данные позволяют оценить тяжесть интоксикации и предвидеть потенциальные осложнения. У пациентов могут наблюдаться признаки абстинентного синдрома: дрожь, повышенное потоотделение, беспокойство и даже галлюцинаторные явления, требующие особого медицинского контроля.
Получить дополнительные сведения – [url=https://kapelnica-ot-zapoya-msk55.ru/]http://kapelnica-ot-zapoya-msk55.ru/kapelnicza-ot-zapoya-tsena-moskva[/url]
Многие медцентры используют современные методики детоксикации с применением эффективных препаратов для облегчения абстинентного синдрома. Пациентам доступно полное медицинское обследование для выявления сопутствующих патологий и разработки оптимальной схемы лечения.
Выяснить больше – [url=https://kapelnica-ot-zapoya-msk55.ru/]http://kapelnica-ot-zapoya-msk55.ru/kapelnicza-ot-zapoya-na-domu-moskva[/url]
Этот формат позволяет пациентам получить профессиональную помощь в комфортной домашней обстановке. Такой подход не только обеспечивает удобство, но и гарантирует конфиденциальность, что особенно важно для многих людей.
Получить дополнительную информацию – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]narcolog-na-dom-v-krasnoyarske55.ru/[/url]
Капельница от запоя – это метод внутривенного введения специальных растворов, направленных на восстановление водно-электролитного баланса организма, выведение токсинов и снижение симптомов абстинентного синдрома. Эта процедура позволяет быстро стабилизировать состояние пациента, ускорить процессы детоксикации и предотвратить возможные осложнения, связанные с длительным употреблением алкоголя.
Подробнее можно узнать тут – [url=https://kapelnica-ot-zapoya-voronezh2.ru/kapelnica-ot-zapoya-anonimno-v-voronezhe/]капельница от запоя круглосуточно наркология воронеж[/url]
Алкогольный запой представляет собой критическое состояние, требующее немедленного вмешательства квалифицированных специалистов. Клиника «Союз Здоровья» в Воронеже предлагает круглосуточную помощь, чтобы обеспечить своевременное и эффективное лечение. Наша цель — оперативное снятие интоксикации, устранение симптомов и предотвращение осложнений.
Подробнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]vyvod iz zapoya na domu kruglosutochno voronezh[/url]
Проблема алкогольной зависимости затрагивает значительное количество людей. Одним из действенных способов лечения интоксикации от алкоголя является внутривенная терапия. В Москве и области множество медицинских учреждений и независимых врачей предоставляют услуги детоксикации. Тем не менее, перед обращением за медицинской помощью необходимо разобраться, как формируется цена капельницы от из запоя в Москве, и к кому можно обратиться.
Углубиться в тему – [url=https://kapelnica-ot-zapoya-msk55.ru/]http://kapelnica-ot-zapoya-msk55.ru[/url]
Лечение возможно как на дому, так и в стационаре. Амбулаторное лечение идеально подходит для тех, кто предпочитает оставаться в привычной обстановке, в то время как стационар подходит для более сложных случаев, требующих постоянного медицинского контроля. В стационаре проводятся все необходимые исследования, включая анализы, ЭКГ и УЗИ, что помогает минимизировать риски и ускорить процесс восстановления.
Выяснить больше – [url=https://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-anonimno-v-voronezhe/]kapelnica ot zapoya srochno kruglosutochno voronezh[/url]
Клиника “Трезвость и Надежда” предоставляет профессиональную помощь в избавлении от запоев как в домашних условиях, так и в стационаре. Наши специалисты используют передовые методы для быстрого очищения организма от токсинов и стабилизации состояния пациента. В процессе капельного введения препаратов восстанавливаются водно-электролитный баланс и нормализуется работа органов, также устраняются основные симптомы похмелья — головная боль, тошнота, раздражительность.
Ознакомиться с деталями – [url=https://kapelnica-ot-zapoya-voronezh.ru/]нарколог капельница от запоя цена[/url]
Вывод из запоя — одна из самых востребованных процедур. Этот процесс включает в себя не только медикаментозное вмешательство для устранения абстинентного синдрома, но и комплексную диагностику для выявления степени зависимости пациента. Врач может применить бензодиазепины для стабилизации нервной системы, а также витаминные комплексы для восстановления обменных процессов. Важно также обеспечить адекватное увлажнение организма, что поможет избежать обезвоживания и поддержит нормальное функционирование органов.
Подробнее тут – [url=https://narcolog-na-dom-krasnoyarsk55.ru/]выезд нарколога на дом цена в красноярске[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-11.ru/]vyvod iz zapoya[/url]
Одной из самых востребованных процедур является снятие абстинентного синдрома. Это состояние сопровождается сильными физическими и психологическими симптомами, такими как тревожность, тремор, головная боль и бессонница. Врач, прибывший на вызов, проводит диагностику состояния пациента и подбирает необходимые препараты. Вводятся инфузионные растворы с витаминами, седативными и противорвотными средствами.
Детальнее – [url=https://narcolog-na-dom-krasnoyarsk55.ru/]вызов нарколога ценакрасноярск[/url]
Алкогольная зависимость — это серьезное заболевание, которое затрагивает не только физическое здоровье, но и психику. Особенно опасен запой, при котором длительное употребление алкоголя вызывает сильную интоксикацию организма. В таких случаях капельница становится жизненно необходимой процедурой.
Исследовать вопрос подробнее – [url=https://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-anonimno-v-voronezhe/]kapelnica ot zapoya srochno kruglosutochno[/url]
Оптимальный вариант получить помощь круглосуточно анонимно в привычной обстановке. Цены капельницы от запоя на дому в Москве составляют от 7 000 до 20 000 руб., учитывая расстояние и время вызова.
Получить дополнительные сведения – [url=https://kapelnica-ot-zapoya-msk55.ru/]стоимость капельницы от запоя москва[/url]
Детоксикация включает в себя снятие абстиненции, очищение организма и стабилизацию работы внутренних органов. Врач тщательно следит за состоянием пациента на всех этапах лечения, подбирая необходимые препараты для восстановления. Психологическая поддержка помогает снизить тревожность, справиться с депрессией и укрепить мотивацию к трезвости.
Подробнее тут – [url=https://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-cena-v-voronezhe/]наркология капельница от запоя анонимно[/url]
I’ve been looking for a reliable way to install Metamask on Chrome, and https://metanate.org/ provided exactly what I needed. Their guide made the process effortless.
Медикаментозное лечение — это еще один важный этап. Оно включает использование препаратов, которые помогают пациенту восстановиться как физически, так и психологически. Нарколог контролирует процесс лечения, чтобы исключить побочные эффекты и скорректировать терапию при необходимости.
Получить дополнительную информацию – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]выезд нарколога на дом цена красноярск[/url]
Такая терапия помогает подготовить организм к дальнейшему лечению от зависимости, улучшить физическое состояние и предотвратить развитие осложнений, таких как обезвоживание или нарушение работы внутренних органов.
Подробнее можно узнать тут – [url=https://kapelnica-ot-zapoya-voronezh2.ru/kapelnica-ot-zapoya-cena-v-voronezhe/]капельница от запоя на дому недорого[/url]
Медикаментозное лечение — это еще один важный этап. Оно включает использование препаратов, которые помогают пациенту восстановиться как физически, так и психологически. Нарколог контролирует процесс лечения, чтобы исключить побочные эффекты и скорректировать терапию при необходимости.
Подробнее – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]выезд нарколога на дом цена красноярск[/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Углубиться в тему – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]vyvod iz zapoya na domu nedorogo [/url]
Детоксикация включает в себя снятие абстиненции, очищение организма и стабилизацию работы внутренних органов. Врач тщательно следит за состоянием пациента на всех этапах лечения, подбирая необходимые препараты для восстановления. Психологическая поддержка помогает снизить тревожность, справиться с депрессией и укрепить мотивацию к трезвости.
Получить больше информации – [url=https://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-voronezhe/]https://www.kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-voronezhe[/url]
Многие медцентры используют современные методики детоксикации с применением эффективных препаратов для облегчения абстинентного синдрома. Пациентам доступно полное медицинское обследование для выявления сопутствующих патологий и разработки оптимальной схемы лечения.
Получить дополнительную информацию – [url=https://kapelnica-ot-zapoya-msk55.ru/]капельницу от запоя в москве[/url]
Лечение основывается на индивидуальном подходе. Врач-нарколог проводит диагностику, разрабатывает план терапии, подбирает нужные препараты и внимательно следит за состоянием пациента на протяжении всей терапии. При этом учитывается не только физическое, но и психоэмоциональное состояние. Психологическая поддержка и мотивация отказаться от алкоголя играют ключевую роль в достижении устойчивых результатов и предотвращении рецидивов.
Узнать больше – [url=https://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-anonimno-v-voronezhe/]kapelnica ot zapoya srochno kruglosutochno v voronezhe[/url]
Детоксикация организма, проводимая на дому, помогает очистить кровь от токсинов, накопившихся из-за длительного употребления алкоголя или наркотических веществ. Она проводится с использованием специально подобранных медикаментов, которые улучшают работу печени, почек и других органов.
Подробнее – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]нарколог на дом красноярск[/url]
Услуга вызова нарколога на дом в Красноярске — это удобный и современный способ получения медицинской помощи. Она идеально подходит для тех, кто нуждается в профессиональной поддержке, но по каким-либо причинам не может или не хочет посещать клинику.
Получить дополнительную информацию – [url=https://narcolog-na-dom-krasnoyarsk55.ru/]вызов нарколога цена в красноярске[/url]
Стоимость стационарного лечения начинается от 35 000 рублей и варьируется в зависимости от сложности состояния. В клинике пациенты получают комплексную поддержку: от детоксикации до круглосуточного наблюдения и психологической помощи.
Исследовать вопрос подробнее – [url=https://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-na-domu-v-voronezhe/]капельница от запоя вызов на дом в воронеже[/url]
При госпитализации пациента в стационар клиники “Пульс жизни”, первым этапом является введение капельницы для детоксикации организма. Растворы, содержащие электролиты, витамины и другие необходимые компоненты, восстанавливают водно-солевой баланс и поддерживают функции внутренних органов. Также в процессе лечения может быть назначено дополнительное медикаментозное лечение для устранения симптомов абстиненции и нормализации психоэмоционального состояния.
Ознакомиться с деталями – [url=https://kapelnica-ot-zapoya-voronezh2.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-voronezhe/]http://www.kapelnica-ot-zapoya-voronezh2.ru/kapelnica-ot-zapoya-v-kruglosutochno-v-voronezhe[/url]
Нарколог, приезжающий на дом, предоставляет широкий спектр услуг. Основная цель — стабилизировать состояние пациента и начать лечение, направленное на устранение последствий зависимости.
Подробнее – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]вызов нарколога на дом красноярск[/url]
Алкогольная зависимость — это серьезное заболевание, которое затрагивает не только физическое здоровье, но и психику. Особенно опасен запой, при котором длительное употребление алкоголя вызывает сильную интоксикацию организма. В таких случаях капельница становится жизненно необходимой процедурой.
Выяснить больше – [url=https://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-cena-v-voronezhe/]капельница от запоя анонимно[/url]
Запой — это длительное, неконтролируемое употребление алкоголя, часто сопровождающееся тяжелыми физическими и психоэмоциональными симптомами. Капельница от запоя является неотъемлемой частью выведения пациента из состояния алкогольной интоксикации и восстановления его здоровья. Она помогает быстро восстановить водно-солевой баланс, вывести токсины, снизить симптомы абстиненции и улучшить общее самочувствие пациента.
Узнать больше – [url=https://kapelnica-ot-zapoya-voronezh2.ru/kapelnica-ot-zapoya-cena-v-voronezhe/]капельница от запоя врач на дом воронеж[/url]
Алкогольная и наркотическая зависимости — серьезные проблемы, требующие профессионального вмешательства. В Красноярске одной из самых востребованных услуг является вызов нарколога на дом. Этот формат позволяет пациентам получить квалифицированную помощь в комфортной обстановке, избегая стрессов, связанных с посещением клиники.
Получить больше информации – [url=https://narcolog-na-dom-krasnoyarsk55.ru/]вызов нарколога на дом цена в красноярске[/url]
Детоксикация включает в себя снятие абстиненции, очищение организма и стабилизацию работы внутренних органов. Врач тщательно следит за состоянием пациента на всех этапах лечения, подбирая необходимые препараты для восстановления. Психологическая поддержка помогает снизить тревожность, справиться с депрессией и укрепить мотивацию к трезвости.
Получить больше информации – [url=https://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-anonimno-v-voronezhe/]kapelnica ot zapoya srochno kruglosutochno v voronezhe[/url]
Things i have usually told persons is that when you are evaluating a good internet electronics retail store, there are a few variables that you have to take into account. First and foremost, you would like to make sure to find a reputable as well as reliable store that has enjoyed great evaluations and scores from other individuals and industry advisors. This will ensure that you are getting through with a well-known store to provide good program and assistance to the patrons. Many thanks for sharing your thinking on this web site.
Запой – это состояние, которое возникает вследствие длительного употребления алкоголя, приводящее к потере контроля над количеством выпиваемого и формированию как физической, так и психологической зависимости. Оно сопровождается серьёзными последствиями для здоровья и требует немедленного вмешательства.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]vyvod iz zapoya na domu nedorogo kapelnica voronezh[/url]
Алкогольная зависимость — это серьезное заболевание, которое затрагивает не только физическое здоровье, но и психику. Особенно опасен запой, при котором длительное употребление алкоголя вызывает сильную интоксикацию организма. В таких случаях капельница становится жизненно необходимой процедурой.
Подробнее можно узнать тут – [url=https://kapelnica-ot-zapoya-voronezh.ru/kapelnica-ot-zapoya-v-stacionare-v-voronezhe/]kapelnica ot zapoya voronezh[/url]
Наркологическая клиника «Союз Здоровья» в Воронеже специализируется на лечении алкогольной зависимости и предлагает профессиональные услуги по выводу из запоя. Мы стремимся создать атмосферу доверия, где каждый пациент сможет получить необходимую поддержку.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]вывод из запоя на дому круглосуточно в воронеже[/url]
Капельница от запоя представляет собой инъекционную терапию, которая помогает очистить организм от токсинов, вызванных чрезмерным употреблением алкоголя. Состав капельницы включает специальные растворы, которые способствуют восстановлению водно-солевого баланса, а также витамины и препараты для нормализации функций печени и нервной системы. Это помогает пациенту избавиться от головной боли, слабости, тошноты и других симптомов похмелья, ускоряя процесс восстановления.
Подробнее тут – [url=https://kapelnica-ot-zapoya-ektb55.ru/]http://kapelnica-ot-zapoya-ektb55.ru/kapelnicza-na-domu-ekaterinburg-ot-zapoya/[/url]
Стационарное лечение рекомендуется при сильной алкогольной интоксикации, когда пациент испытывает головные боли, тошноту, слабость, повышенное давление и нарушение сна. Если эти симптомы игнорировать, возможны серьезные последствия, включая развитие хронических заболеваний и осложнений.
Детальнее – [url=https://kapelnica-ot-zapoya-ekb55.ru/]капельницу от запоя в екатеринбурге[/url]
Этот вид услуги востребован благодаря своей оперативности, индивидуальному подходу и конфиденциальности. Вызов врача позволяет минимизировать стресс, обеспечить комфортное лечение и своевременно предотвратить возможные осложнения.
Выяснить больше – [url=https://narcolog-na-dom-ekaterinburg55.ru /]нарколог на дом недорого екатеринбург[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьезное воздействие этанола, что приводит к нарушению нормальной физиологической деятельности. Это состояние сопровождается сильными физическими и психологическими расстройствами, которые требуют профессионального вмешательства. Однако многие люди, страдающие алкогольной зависимостью, сталкиваются с трудностью признания своей проблемы, опасаясь утраты репутации и конфиденциальности. В клинике «Заря Будущего» мы предлагаем анонимный вывод из запоя в Санкт-Петербурге, предоставляя высококачественное лечение с гарантией сохранения личной информации.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]vyvod iz zapoya cena narkologiya sankt-peterburg[/url]
Процедура капельницы особенно показана в случаях, когда симптомы похмелья выражены настолько сильно, что пациент не в состоянии справиться с ними самостоятельно. Головная боль, тошнота, рвота, усталость и раздражительность — все это признаки того, что организму необходимо восстановление. Капельница помогает не только ускорить процесс очищения, но и минимизировать риск осложнений, таких как нарушение работы сердца и печени.
Ознакомиться с деталями – [url=https://kapelnica-ot-zapoya-ektb55.ru/]капельницу от запоя в екатеринбурге[/url]
Проблема зависимостей остаётся актуальной в современном обществе. С каждым годом увеличивается число людей, страдающих от алкоголизма, наркомании и других форм зависимостей, что негативно отражается на их жизни и благополучии близких. Зависимость — это не просто физическое заболевание, но и глубокая психологическая проблема. Для эффективного лечения требуется помощь профессионалов, способных обеспечить комплексный подход.
Подробнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]vyvod iz zapoya vrach na dom voronezh[/url]
Алкогольная зависимость – это серьёзная проблема, требующая комплексного медицинского вмешательства. Для эффективного лечения и восстановления здоровья пациенты всё чаще выбирают стационарное лечение в наркологической клинике. Капельница от запоя в стационаре в Екатеринбурге представляет собой одно из наиболее популярных и действенных решений, которое позволяет быстро устранить симптомы интоксикации и нормализовать состояние пациента.
Исследовать вопрос подробнее – [url=https://kapelnica-ot-zapoya-ektb55.ru/]стоимость капельницы от запоя в екатеринбурге[/url]
Алкогольная зависимость требует быстрого и квалифицированного вмешательства, поскольку она может привести к тяжелым последствиям для здоровья. Капельница от запоя — один из наиболее эффективных методов, помогающих вывести организм из состояния алкогольной интоксикации и восстановить нормальное самочувствие. В Екатеринбурге эта процедура доступна как в медицинских учреждениях, так и на дому, что позволяет пациентам выбрать наиболее удобный и комфортный способ лечения.
Детальнее – [url=https://kapelnica-ot-zapoya-ektb55.ru/]после капельницы от запоя в екатеринбурге[/url]
Алкогольная зависимость – сложное и опасное состояние, которое сопровождается как физической, так и психологической привязанностью к спиртному. Одним из самых тяжелых проявлений этой проблемы является запой – длительное бесконтрольное употребление алкоголя, которое приводит к серьезным нарушениям в работе организма. Такое состояние требует срочного медицинского вмешательства, поскольку без профессиональной помощи возможны тяжелые осложнения, вплоть до угрозы жизни.
Разобраться лучше – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-anonimno-v-tveri/]anonimny vyvod iz zapoya na domu [/url]
Алкогольная зависимость требует быстрого и квалифицированного вмешательства, поскольку она может привести к тяжелым последствиям для здоровья. Капельница от запоя — один из наиболее эффективных методов, помогающих вывести организм из состояния алкогольной интоксикации и восстановить нормальное самочувствие. В Екатеринбурге эта процедура доступна как в медицинских учреждениях, так и на дому, что позволяет пациентам выбрать наиболее удобный и комфортный способ лечения.
Узнать больше – [url=https://kapelnica-ot-zapoya-ektb55.ru/]капельницы от запояекатеринбург[/url]
Программы лечения разрабатываются индивидуально и могут включать как визит врача на дом, так и стационарное пребывание. Стоимость услуг формируется в зависимости от необходимых процедур и остается прозрачной, позволяя пациентам сосредоточиться на главном – восстановлении здоровья и возвращении к полноценной жизни.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]вывод из запоя круглосуточно санкт-петербург[/url]
Капельница от запоя представляет собой инъекционную терапию, которая помогает очистить организм от токсинов, вызванных чрезмерным употреблением алкоголя. Состав капельницы включает специальные растворы, которые способствуют восстановлению водно-солевого баланса, а также витамины и препараты для нормализации функций печени и нервной системы. Это помогает пациенту избавиться от головной боли, слабости, тошноты и других симптомов похмелья, ускоряя процесс восстановления.
Подробнее – [url=https://kapelnica-ot-zapoya-ektb55.ru/]kapelnica-ot-zapoya-ektb55.ru/[/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Подробнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя вызов на дом в санкт-петербурге[/url]
Алкогольная зависимость – это серьёзная проблема, требующая комплексного медицинского вмешательства. Для эффективного лечения и восстановления здоровья пациенты всё чаще выбирают стационарное лечение в наркологической клинике. Капельница от запоя в стационаре в Екатеринбурге представляет собой одно из наиболее популярных и действенных решений, которое позволяет быстро устранить симптомы интоксикации и нормализовать состояние пациента.
Исследовать вопрос подробнее – [url=https://kapelnica-ot-zapoya-ektb55.ru/]капельницу от запоя в екатеринбурге[/url]
Алкогольная зависимость — одна из самых распространенных проблем нашего времени, требующая немедленного и грамотного вмешательства. В Екатеринбурге капельница от запоя является одним из самых популярных и действенных методов восстановления после длительного употребления алкоголя. Она помогает организму справиться с интоксикацией, восстанавливает физическое и эмоциональное состояние пациента, улучшает самочувствие в короткие сроки. При этом лечение может быть организовано как в стационаре, так и на дому, в зависимости от удобства пациента.
Получить дополнительную информацию – [url=https://kapelnica-ot-zapoya-ekb55.ru/]капельницу от запоя в екатеринбурге[/url]
Для эффективного и безопасного выхода из запоя необходимо квалифицированное лечение, включающее детоксикацию организма, восстановление работы внутренних органов и поддержку психоэмоционального состояния. В этом случае важно обратиться к специалистам, которые смогут подобрать оптимальные методы терапии, исходя из состояния пациента. Один из наиболее эффективных способов снятия алкогольной интоксикации – это внутривенная инфузионная терапия (капельницы), позволяющая быстро очистить организм от токсинов, восстановить водно-солевой баланс и улучшить общее самочувствие.
Узнать больше – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]вывод из запоя на дому тверь[/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]быстрый вывод из запоя в стационаре [/url]
Алкогольная зависимость требует быстрого и квалифицированного вмешательства, поскольку она может привести к тяжелым последствиям для здоровья. Капельница от запоя — один из наиболее эффективных методов, помогающих вывести организм из состояния алкогольной интоксикации и восстановить нормальное самочувствие. В Екатеринбурге эта процедура доступна как в медицинских учреждениях, так и на дому, что позволяет пациентам выбрать наиболее удобный и комфортный способ лечения.
Разобраться лучше – [url=https://kapelnica-ot-zapoya-ektb55.ru/]капельница от запоя на дому екатеринбург[/url]
Для тех, кто по разным причинам не может посетить медицинский центр, существует возможность вызова нарколога на дом. Такая услуга позволяет получить необходимую помощь в комфортных условиях, сохраняя при этом полную анонимность. Опытные врачи проведут детоксикацию, назначат поддерживающую терапию и дадут рекомендации по дальнейшему восстановлению.
Узнать больше – [url=https://vyvod-iz-zapoya-21.ru/]narkologicheskij centr tver[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьезное воздействие этанола, что приводит к нарушению нормальной физиологической деятельности. Это состояние сопровождается сильными физическими и психологическими расстройствами, которые требуют профессионального вмешательства. Однако многие люди, страдающие алкогольной зависимостью, сталкиваются с трудностью признания своей проблемы, опасаясь утраты репутации и конфиденциальности. В клинике «Заря Будущего» мы предлагаем анонимный вывод из запоя в Санкт-Петербурге, предоставляя высококачественное лечение с гарантией сохранения личной информации.
Подробнее тут – [url=https://vyvod-iz-zapoya-19.ru/]vyvod iz zapoya kapelnica sankt-peterburg[/url]
Для эффективного и безопасного выхода из запоя необходимо квалифицированное лечение, включающее детоксикацию организма, восстановление работы внутренних органов и поддержку психоэмоционального состояния. В этом случае важно обратиться к специалистам, которые смогут подобрать оптимальные методы терапии, исходя из состояния пациента. Один из наиболее эффективных способов снятия алкогольной интоксикации – это внутривенная инфузионная терапия (капельницы), позволяющая быстро очистить организм от токсинов, восстановить водно-солевой баланс и улучшить общее самочувствие.
Разобраться лучше – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-v-stacionare-v-tveri/]vyvod iz zapoya v stacionare anonimno tver[/url]
Запойное пьянство представляет серьезную угрозу для здоровья, провоцируя развитие таких заболеваний, как цирроз печени, панкреатит, сердечно-сосудистые нарушения, а также психические расстройства. В запущенных случаях возможны тяжелые последствия, включая алкогольный делирий (белую горячку), который требует экстренной медицинской помощи. Чтобы избежать подобных рисков, необходимо своевременно обратиться к специалистам, которые помогут стабилизировать состояние и провести грамотное лечение.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-v-stacionare-v-tveri/]vyvod iz zapoya v stacionare anonimno [/url]
В центре доступны различные варианты лечения – от амбулаторного наблюдения до интенсивных стационарных программ. Ключевые принципы работы – конфиденциальность, индивидуальный подход и оперативность оказания помощи. Независимо от тяжести зависимости, каждый пациент получает квалифицированную поддержку, направленную на восстановление здоровья и возвращение к полноценной жизни.
Подробнее – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]вывод из запоя круглосуточно [/url]
Алкогольный запой представляет собой критическое состояние, требующее немедленного вмешательства квалифицированных специалистов. Клиника «Союз Здоровья» в Воронеже предлагает круглосуточную помощь, чтобы обеспечить своевременное и эффективное лечение. Наша цель — оперативное снятие интоксикации, устранение симптомов и предотвращение осложнений.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-anonimno-v-voronezhe/]vyvod iz zapoya anonimno v-voronezhe[/url]
Процедура капельницы помогает не только устранить симптомы похмелья, но и способствует восстановлению нормальной работы организма. Это достигается благодаря введению лекарственных растворов, которые очищают кровь от токсинов, восстанавливают водно-солевой баланс и нормализуют работу жизненно важных органов.
Получить дополнительную информацию – [url=https://kapelnica-ot-zapoya-ekb55.ru/]после капельницы от запоя екатеринбург[/url]
Капельница от запоя представляет собой инъекционную терапию, которая помогает очистить организм от токсинов, вызванных чрезмерным употреблением алкоголя. Состав капельницы включает специальные растворы, которые способствуют восстановлению водно-солевого баланса, а также витамины и препараты для нормализации функций печени и нервной системы. Это помогает пациенту избавиться от головной боли, слабости, тошноты и других симптомов похмелья, ускоряя процесс восстановления.
Узнать больше – [url=https://kapelnica-ot-zapoya-ektb55.ru/]капельница от запоя екатеринбург[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьезное воздействие этанола, что приводит к нарушению нормальной физиологической деятельности. Это состояние сопровождается сильными физическими и психологическими расстройствами, которые требуют профессионального вмешательства. Однако многие люди, страдающие алкогольной зависимостью, сталкиваются с трудностью признания своей проблемы, опасаясь утраты репутации и конфиденциальности. В клинике «Заря Будущего» мы предлагаем анонимный вывод из запоя в Санкт-Петербурге, предоставляя высококачественное лечение с гарантией сохранения личной информации.
Подробнее тут – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya s vyezdom na dom sankt-peterburg[/url]
Вывод из запоя – это не просто разовое медицинское вмешательство, а важный шаг на пути к полному избавлению от алкогольной зависимости. Комплексный подход, включающий не только устранение симптомов интоксикации, но и последующую психологическую реабилитацию, позволяет добиться устойчивого результата и вернуть человека к полноценной жизни.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-cena-v-tveri/]вывод из запоя с выездом цена в твери[/url]
Для тех, кто не может приехать в клинику, специалисты проводят детоксикацию на дому. С помощью современных препаратов организм мягко очищается от токсинов, уменьшается нагрузка на внутренние органы, снимаются неприятные симптомы абстиненции. Однако при тяжелом состоянии или наличии осложнений может потребоваться круглосуточное наблюдение в стационаре, где пациенту обеспечивается полная медицинская поддержка.
Узнать больше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]наркология вывод из запоя на дому [/url]
Процедура капельницы особенно показана в случаях, когда симптомы похмелья выражены настолько сильно, что пациент не в состоянии справиться с ними самостоятельно. Головная боль, тошнота, рвота, усталость и раздражительность — все это признаки того, что организму необходимо восстановление. Капельница помогает не только ускорить процесс очищения, но и минимизировать риск осложнений, таких как нарушение работы сердца и печени.
Углубиться в тему – [url=https://kapelnica-ot-zapoya-ektb55.ru/]капельницу от запоя в екатеринбурге[/url]
Запойное пьянство представляет серьезную угрозу для здоровья, провоцируя развитие таких заболеваний, как цирроз печени, панкреатит, сердечно-сосудистые нарушения, а также психические расстройства. В запущенных случаях возможны тяжелые последствия, включая алкогольный делирий (белую горячку), который требует экстренной медицинской помощи. Чтобы избежать подобных рисков, необходимо своевременно обратиться к специалистам, которые помогут стабилизировать состояние и провести грамотное лечение.
Разобраться лучше – [url=https://vyvod-iz-zapoya-21.ru/]наркологическая клиника[/url]
Алкогольный запой представляет собой серьезное состояние, которое требует немедленного медицинского вмешательства. Это состояние характеризуется длительным и бесконтрольным потреблением алкоголя, что приводит к разрушению как физического, так и психоэмоционального здоровья человека. Для эффективного и безопасного вывода из запоя стационарное лечение является оптимальным решением, особенно при тяжелых случаях интоксикации. В стационаре клиники «Заря Будущего» мы предлагаем комплексную программу вывода из запоя, которая включает детоксикацию, восстановление организма и психотерапевтическую помощь.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-19.ru/]вывод из запоя с выездом санкт-петербург[/url]
Вывод из запоя – это не просто разовое медицинское вмешательство, а важный шаг на пути к полному избавлению от алкогольной зависимости. Комплексный подход, включающий не только устранение симптомов интоксикации, но и последующую психологическую реабилитацию, позволяет добиться устойчивого результата и вернуть человека к полноценной жизни.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-21.ru/]вывод из запоя капельница[/url]
Процедура капельницы особенно показана в случаях, когда симптомы похмелья выражены настолько сильно, что пациент не в состоянии справиться с ними самостоятельно. Головная боль, тошнота, рвота, усталость и раздражительность — все это признаки того, что организму необходимо восстановление. Капельница помогает не только ускорить процесс очищения, но и минимизировать риск осложнений, таких как нарушение работы сердца и печени.
Получить дополнительные сведения – [url=https://kapelnica-ot-zapoya-ektb55.ru/]https://kapelnica-ot-zapoya-ektb55.ru/kapelnicza-ot-zapoya-v-stacionare-ekaterinburg[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]быстрый вывод из запоя в стационаре [/url]
Выведение из запоя – это процесс, включающий несколько этапов. Симптомы могут варьироваться от тревожности и бессонницы до серьезных психических расстройств. Только профессиональный подход и современные медицинские технологии помогают минимизировать риски, стабилизировать состояние и избежать осложнений.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]быстрый вывод из запоя в стационаре санкт-петербург[/url]
Лечение в домашних условиях имеет множество достоинств, среди которых ключевыми являются комфорт, доступность и конфиденциальность. Пациент может пройти лечение в привычной обстановке, что снижает уровень тревожности и способствует более быстрому восстановлению.
Подробнее – [url=https://narcolog-na-dom-ekaterinburg55.ru /]http://narcolog-na-dom-ekaterinburg55.ru [/url]
Программы лечения разрабатываются индивидуально и могут включать как визит врача на дом, так и стационарное пребывание. Стоимость услуг формируется в зависимости от необходимых процедур и остается прозрачной, позволяя пациентам сосредоточиться на главном – восстановлении здоровья и возвращении к полноценной жизни.
Углубиться в тему – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя на дому недорого в санкт-петербурге[/url]
Проблема зависимостей остаётся актуальной в современном обществе. С каждым годом увеличивается число людей, страдающих от алкоголизма, наркомании и других форм зависимостей, что негативно отражается на их жизни и благополучии близких. Зависимость — это не просто физическое заболевание, но и глубокая психологическая проблема. Для эффективного лечения требуется помощь профессионалов, способных обеспечить комплексный подход.
Подробнее тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]narkolog na dom vyvod iz zapoya voronezh[/url]
Процедура капельницы особенно показана в случаях, когда симптомы похмелья выражены настолько сильно, что пациент не в состоянии справиться с ними самостоятельно. Головная боль, тошнота, рвота, усталость и раздражительность — все это признаки того, что организму необходимо восстановление. Капельница помогает не только ускорить процесс очищения, но и минимизировать риск осложнений, таких как нарушение работы сердца и печени.
Получить дополнительную информацию – [url=https://kapelnica-ot-zapoya-ektb55.ru/]капельницы от запояекатеринбург[/url]
Алкогольная зависимость – сложное и опасное состояние, которое сопровождается как физической, так и психологической привязанностью к спиртному. Одним из самых тяжелых проявлений этой проблемы является запой – длительное бесконтрольное употребление алкоголя, которое приводит к серьезным нарушениям в работе организма. Такое состояние требует срочного медицинского вмешательства, поскольку без профессиональной помощи возможны тяжелые осложнения, вплоть до угрозы жизни.
Выяснить больше – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-anonimno-v-tveri/]vyvod iz zapoya anonimno v-tveri[/url]
Для тех, кто по разным причинам не может посетить медицинский центр, существует возможность вызова нарколога на дом. Такая услуга позволяет получить необходимую помощь в комфортных условиях, сохраняя при этом полную анонимность. Опытные врачи проведут детоксикацию, назначат поддерживающую терапию и дадут рекомендации по дальнейшему восстановлению.
Подробнее тут – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]narkolog na dom vyvod iz zapoya [/url]
В клинике «Заря Будущего» мы понимаем, насколько важен своевременный и профессиональный подход к лечению зависимости. Наши специалисты готовы оказать квалифицированную помощь прямо у вас дома, обеспечивая полную безопасность и конфиденциальность на каждом этапе лечения.
Разобраться лучше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]srochny vyvod iz zapoya na domu v-sankt-peterburge[/url]
Алкоголизм – одно из наиболее разрушительных заболеваний, наносящих серьезный ущерб организму. Запойные состояния особенно опасны, так как приводят к глубокой интоксикации, психическим нарушениям и необратимым последствиям. Чем раньше начато лечение, тем выше вероятность избежать осложнений и быстрее восстановиться.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-14.ru/]vyvod iz zapoya[/url]
Алкогольная зависимость – сложное и опасное состояние, которое сопровождается как физической, так и психологической привязанностью к спиртному. Одним из самых тяжелых проявлений этой проблемы является запой – длительное бесконтрольное употребление алкоголя, которое приводит к серьезным нарушениям в работе организма. Такое состояние требует срочного медицинского вмешательства, поскольку без профессиональной помощи возможны тяжелые осложнения, вплоть до угрозы жизни.
Подробнее – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-cena-v-tveri/]нарколог вывод из запоя цена тверь[/url]
Капельница от запоя представляет собой инъекционную терапию, которая помогает очистить организм от токсинов, вызванных чрезмерным употреблением алкоголя. Состав капельницы включает специальные растворы, которые способствуют восстановлению водно-солевого баланса, а также витамины и препараты для нормализации функций печени и нервной системы. Это помогает пациенту избавиться от головной боли, слабости, тошноты и других симптомов похмелья, ускоряя процесс восстановления.
Получить дополнительные сведения – [url=https://kapelnica-ot-zapoya-ektb55.ru/]https://kapelnica-ot-zapoya-ektb55.ru/kapelnicza-na-domu-ekaterinburg-ot-zapoya/[/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Углубиться в тему – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]anonimny vyvod iz zapoya na domu sankt-peterburg[/url]
Зависимость от алкоголя или наркотических веществ — это серьёзная проблема, требующая профессионального вмешательства. В критических ситуациях вызов нарколога на дом становится оптимальным решением. Услуга позволяет получить квалифицированную медицинскую помощь в комфортных условиях, что особенно важно для пациентов, которым трудно или невозможно посетить клинику.
Получить дополнительные сведения – [url=https://narcolog-na-dom-ekaterinburg55.ru /]http://narcolog-na-dom-ekaterinburg55.ru/narkolog-na-dom-kruglosutochno-ekaterinburg/[/url]
Для тех, кто не может приехать в клинику, специалисты проводят детоксикацию на дому. С помощью современных препаратов организм мягко очищается от токсинов, уменьшается нагрузка на внутренние органы, снимаются неприятные симптомы абстиненции. Однако при тяжелом состоянии или наличии осложнений может потребоваться круглосуточное наблюдение в стационаре, где пациенту обеспечивается полная медицинская поддержка.
Подробнее тут – [url=https://vyvod-iz-zapoya-14.ru/]наркологическая клиника[/url]
Наркологическая помощь на дому охватывает широкий спектр мероприятий, направленных на стабилизацию состояния пациента и лечение зависимости. Одним из ключевых направлений является детоксикация. Эта процедура позволяет очистить организм от токсичных веществ, улучшить общее самочувствие и восстановить работу внутренних органов.
Подробнее можно узнать тут – [url=https://narcolog-na-dom-ekaterinburg55.ru /]нарколог на дом цены в екатеринбурге[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Разобраться лучше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]вывод из запоя цена наркология воронеж[/url]
Зависимость — это серьёзная проблема, которая затрагивает не только человека, но и его семью и окружение. Алкоголизм, наркомания и другие виды зависимостей могут разрушить жизнь, но с профессиональной помощью можно вернуть её в русло нормального функционирования. Наркологическая клиника «Заря Будущего» в Санкт-Петербурге предлагает уникальные программы лечения зависимостей, которые сочетает медико-психологический подход, индивидуальные методики и квалифицированную поддержку.
Подробнее – [url=https://vyvod-iz-zapoya-19.ru/]narkologicheskaya klinika sankt-peterburg[/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]нарколог вывод из запоя цена воронеж[/url]
Для эффективного и безопасного выхода из запоя необходимо квалифицированное лечение, включающее детоксикацию организма, восстановление работы внутренних органов и поддержку психоэмоционального состояния. В этом случае важно обратиться к специалистам, которые смогут подобрать оптимальные методы терапии, исходя из состояния пациента. Один из наиболее эффективных способов снятия алкогольной интоксикации – это внутривенная инфузионная терапия (капельницы), позволяющая быстро очистить организм от токсинов, восстановить водно-солевой баланс и улучшить общее самочувствие.
Подробнее – [url=https://vyvod-iz-zapoya-21.ru/]вывод из запоя капельница[/url]
Особенно опасны длительные запои, когда человек в течение нескольких дней бесконтрольно употребляет алкоголь. Такое состояние разрушает организм, перегружает печень, сердце и нервную систему, может вызывать тяжелые осложнения, в том числе психические расстройства. Выйти из запоя самостоятельно практически невозможно и крайне рискованно. Важно своевременно обратиться за медицинской помощью, чтобы избежать серьезных последствий.
Узнать больше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]вывод из запоя в стационаре [/url]
Запойное состояние представляет собой серьёзную угрозу для здоровья, поскольку сопровождается тяжёлой интоксикацией организма и нарушением психоэмоционального состояния. Решение о лечении в стационаре клиники «Союз Здоровья» позволяет справиться с последствиями длительного употребления алкоголя, обеспечивая пациентам полный комплекс медицинской и психологической помощи.
Получить больше информации – [url=https://vyvod-iz-zapoya-11.ru/]наркологическая клиника в воронеже[/url]
Зависимость – это сложное испытание, с которым сталкиваются люди во всём мире. Ежедневные стрессы, проблемы в семье и на работе, финансовые трудности часто становятся почвой для развития пагубных привычек, таких как алкоголизм, наркомания и игромания. Медицинский центр «Второй Шанс» предоставляет помощь тем, кто хочет избавиться от этих состояний, вернуть здоровье и научиться жить без разрушительных зависимостей.
Разобраться лучше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]анонимный вывод из запоя на дому [/url]
Наркологическая помощь на дому охватывает широкий спектр мероприятий, направленных на стабилизацию состояния пациента и лечение зависимости. Одним из ключевых направлений является детоксикация. Эта процедура позволяет очистить организм от токсичных веществ, улучшить общее самочувствие и восстановить работу внутренних органов.
Изучить вопрос глубже – [url=https://narcolog-na-dom-ekaterinburg55.ru /]http://narcolog-na-dom-ekaterinburg55.ru [/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Подробнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]наркология вывод из запоя на дому [/url]
Вывод из запоя – это не просто разовое медицинское вмешательство, а важный шаг на пути к полному избавлению от алкогольной зависимости. Комплексный подход, включающий не только устранение симптомов интоксикации, но и последующую психологическую реабилитацию, позволяет добиться устойчивого результата и вернуть человека к полноценной жизни.
Углубиться в тему – [url=https://vyvod-iz-zapoya-21.ru/]vyvod-iz-zapoya-21.ru[/url]
Лечение в домашних условиях имеет множество достоинств, среди которых ключевыми являются комфорт, доступность и конфиденциальность. Пациент может пройти лечение в привычной обстановке, что снижает уровень тревожности и способствует более быстрому восстановлению.
Разобраться лучше – [url=https://narcolog-na-dom-ekaterinburg55.ru /]нарколог в екатеринбурге[/url]
Особенно опасны длительные запои, когда человек в течение нескольких дней бесконтрольно употребляет алкоголь. Такое состояние разрушает организм, перегружает печень, сердце и нервную систему, может вызывать тяжелые осложнения, в том числе психические расстройства. Выйти из запоя самостоятельно практически невозможно и крайне рискованно. Важно своевременно обратиться за медицинской помощью, чтобы избежать серьезных последствий.
Узнать больше – [url=https://vyvod-iz-zapoya-14.ru/]вывод из запоя с выездом[/url]
I was skeptical about installing Metamask until I found https://metamake.org/. Their guides are thorough and trustworthy, making it easy for me to secure my digital assets with confidence. Definitely recommend!
Наркологическая клиника «Союз Здоровья» в Воронеже специализируется на лечении алкогольной зависимости и предлагает профессиональные услуги по выводу из запоя. Мы стремимся создать атмосферу доверия, где каждый пациент сможет получить необходимую поддержку.
Углубиться в тему – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-kruglosutochno-v-voronezhe/]vyvod iz zapoya kruglosutochno narkologiya voronezh[/url]
Процедура капельницы особенно показана в случаях, когда симптомы похмелья выражены настолько сильно, что пациент не в состоянии справиться с ними самостоятельно. Головная боль, тошнота, рвота, усталость и раздражительность — все это признаки того, что организму необходимо восстановление. Капельница помогает не только ускорить процесс очищения, но и минимизировать риск осложнений, таких как нарушение работы сердца и печени.
Подробнее можно узнать тут – [url=https://kapelnica-ot-zapoya-ektb55.ru/]https://kapelnica-ot-zapoya-ektb55.ru/kapelnicza-na-domu-ekaterinburg-ot-zapoya/[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьезное воздействие этанола, что приводит к нарушению нормальной физиологической деятельности. Это состояние сопровождается сильными физическими и психологическими расстройствами, которые требуют профессионального вмешательства. Однако многие люди, страдающие алкогольной зависимостью, сталкиваются с трудностью признания своей проблемы, опасаясь утраты репутации и конфиденциальности. В клинике «Заря Будущего» мы предлагаем анонимный вывод из запоя в Санкт-Петербурге, предоставляя высококачественное лечение с гарантией сохранения личной информации.
Узнать больше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]нарколог вывод из запоя цена в санкт-петербурге[/url]
Ещё одной важной услугой является детоксикация организма. Она заключается в очищении крови и тканей от токсичных веществ, которые накапливаются в процессе длительного употребления алкоголя. Детоксикация проводится с применением капельниц, содержащих глюкозу, витамины, электролиты и другие необходимые компоненты.
Ознакомиться с деталями – [url=https://narcolog-na-dom-ekaterinburg55.ru /]платный нарколог на дом екатеринбург[/url]
Кроме того, анонимность является важной составляющей этой услуги. Лечение дома исключает возможность столкновения с посторонними людьми и помогает пациентам сохранить свою репутацию. Клиника «Гармония и Свет» обеспечивает полную конфиденциальность, предоставляя пациентам возможность получать помощь без лишних вопросов и обсуждений.
Подробнее можно узнать тут – [url=https://narcolog-na-dom-ektb55.ru/]вызов нарколога на дом цена в екатеринбурге[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]vyvod iz zapoya na domu cena [/url]
В данном тексте вы узнаете, как организовать вызов нарколога в Москве, что влияет на стоимость услуги, а также почему этот формат помощи становится все более популярным.
Узнать больше – [url=https://narcolog-na-dom-moskva55.ru/]нарколог на дом стоимость москва[/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Разобраться лучше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]narkologiya vyvod iz zapoya anonimno v-sankt-peterburge[/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Подробнее тут – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]вывод из запоя на дому цена санкт-петербург[/url]
В клинике «Заря Будущего» мы понимаем, насколько важен своевременный и профессиональный подход к лечению зависимости. Наши специалисты готовы оказать квалифицированную помощь прямо у вас дома, обеспечивая полную безопасность и конфиденциальность на каждом этапе лечения.
Детальнее – [url=https://vyvod-iz-zapoya-19.ru/]наркологический центр[/url]
Алкогольный запой представляет собой серьезное состояние, которое требует немедленного медицинского вмешательства. Это состояние характеризуется длительным и бесконтрольным потреблением алкоголя, что приводит к разрушению как физического, так и психоэмоционального здоровья человека. Для эффективного и безопасного вывода из запоя стационарное лечение является оптимальным решением, особенно при тяжелых случаях интоксикации. В стационаре клиники «Заря Будущего» мы предлагаем комплексную программу вывода из запоя, которая включает детоксикацию, восстановление организма и психотерапевтическую помощь.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]вывод из запоя на дому цена в санкт-петербурге[/url]
Детоксикация организма — еще одна важная процедура, которая позволяет вывести токсины и минимизировать их негативное воздействие на органы. Для этого применяются специальные растворы, содержащие витамины, глюкозу и электролиты. Все компоненты подбираются индивидуально на основе состояния пациента.
Детальнее – [url=https://narcolog-na-dom-v-moskve55.ru/]narkolog na dom [/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьезное воздействие этанола, что приводит к нарушению нормальной физиологической деятельности. Это состояние сопровождается сильными физическими и психологическими расстройствами, которые требуют профессионального вмешательства. Однако многие люди, страдающие алкогольной зависимостью, сталкиваются с трудностью признания своей проблемы, опасаясь утраты репутации и конфиденциальности. В клинике «Заря Будущего» мы предлагаем анонимный вывод из запоя в Санкт-Петербурге, предоставляя высококачественное лечение с гарантией сохранения личной информации.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]bystry vyvod iz zapoya v stacionare [/url]
Детоксикация организма представляет собой комплексное очищение от токсинов. Для этого используются инфузионные растворы, содержащие витамины, антиоксиданты и препараты для поддержки печени. Процедура помогает снизить нагрузку на органы и улучшить общее самочувствие.
Изучить вопрос глубже – [url=https://narcolog-na-dom-ektb55.ru/]https://narcolog-na-dom-ektb55.ru/narkolog-na-dom-ekaterinburg-tseny[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]наркология вывод из запоя анонимно [/url]
Проведение лечения в домашней обстановке значительно снижает уровень стресса и тревожности. Пациенту не нужно тратить силы на поездки в медицинские учреждения, что особенно важно при ограниченной мобильности. Домашняя атмосфера создает идеальные условия для открытого диалога с врачом, что существенно повышает эффективность терапии и шансы на успешное выздоровление.
Подробнее тут – [url=https://narcolog-na-dom-msk55.ru/]платный нарколог на дом москва[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Детальнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]narkologiya vyvod iz zapoya anonimno [/url]
Когда пациент решает обратиться за помощью к наркологу на дом, ему предстоит пройти ряд этапов лечения, каждый из которых будет направлен на устранение последствий алкоголизма. Врач проведет первичный осмотр, выслушает жалобы пациента и на основе собранной информации начнёт индивидуальную программу лечения.
Узнать больше – [url=https://narcolog-na-dom-ektb55.ru/]http://narcolog-na-dom-ektb55.ru/[/url]
Одной из самых частых причин является затяжной запой. Алкогольная интоксикация, сопровождающая это состояние, не только ухудшает общее самочувствие, но и создает угрозу для здоровья. Повышенное давление, нарушения сердечного ритма, головные боли и тошнота — это лишь некоторые симптомы, которые требуют вмешательства врача.
Подробнее тут – [url=https://narcolog-na-dom-moskva55.ru/]http://narcolog-na-dom-moskva55.ru[/url]
Кроме того, анонимность является важной составляющей этой услуги. Лечение дома исключает возможность столкновения с посторонними людьми и помогает пациентам сохранить свою репутацию. Клиника «Гармония и Свет» обеспечивает полную конфиденциальность, предоставляя пациентам возможность получать помощь без лишних вопросов и обсуждений.
Разобраться лучше – [url=https://narcolog-na-dom-ektb55.ru/]нарколог на дом екатеринбург[/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Детальнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]наркология вывод из запоя на дому в санкт-петербурге[/url]
Важно отметить, что некоторые клиники предоставляют скидки для новых клиентов или на отдельные процедуры. Однако стоит избегать слишком низких цен, так как это может свидетельствовать о недостаточном уровне профессионализма или отсутствии лицензии у специалиста.
Изучить вопрос глубже – [url=https://narcolog-na-dom-ektb55.ru/]выезд нарколога на дом цена екатеринбург[/url]
Алкогольный запой представляет собой серьезное состояние, которое требует немедленного медицинского вмешательства. Это состояние характеризуется длительным и бесконтрольным потреблением алкоголя, что приводит к разрушению как физического, так и психоэмоционального здоровья человека. Для эффективного и безопасного вывода из запоя стационарное лечение является оптимальным решением, особенно при тяжелых случаях интоксикации. В стационаре клиники «Заря Будущего» мы предлагаем комплексную программу вывода из запоя, которая включает детоксикацию, восстановление организма и психотерапевтическую помощь.
Углубиться в тему – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/[/url]
Для многих пациентов важным моментом является сохранение конфиденциальности. Врач, приехавший на дом, работает с полной защитой данных. Пациент может быть уверен, что его проблема останется личной и не станет достоянием общественности. Врачи приезжают на неприметных автомобилях и избегают всяческих ситуаций, которые могут привлечь лишнее внимание.
Разобраться лучше – [url=https://narcolog-na-dom-moskva55.ru/]http://narcolog-na-dom-moskva55.ru/narkolog-na-dom-moskva-tseny[/url]
Алкогольный запой – это серьёзное состояние, при котором человек теряет контроль над употреблением алкоголя, что приводит к тяжёлой интоксикации организма. Во время запоя нарушаются функции внутренних органов, страдает нервная система, и повышается риск развития опасных осложнений, таких как алкогольный делирий. Это состояние требует незамедлительного медицинского вмешательства.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-v-stacionare-v-ekaterinburge/]вывод из запоя в стационаре екатеринбург[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьезное воздействие этанола, что приводит к нарушению нормальной физиологической деятельности. Это состояние сопровождается сильными физическими и психологическими расстройствами, которые требуют профессионального вмешательства. Однако многие люди, страдающие алкогольной зависимостью, сталкиваются с трудностью признания своей проблемы, опасаясь утраты репутации и конфиденциальности. В клинике «Заря Будущего» мы предлагаем анонимный вывод из запоя в Санкт-Петербурге, предоставляя высококачественное лечение с гарантией сохранения личной информации.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]vyvod iz zapoya cena narkologiya sankt-peterburg[/url]
Популярные мастерские по изготовлению серебряных колец в Саратове, для тех, кто ценит качество и красоту.
Купить кольцо серебряное женское [url=http://xn--b1acnbnotaei0k.xn--p1ai/]http://xn--b1acnbnotaei0k.xn--p1ai/[/url] .
В некоторых случаях помощь нарколога требуется для выведения пациента из запоя. Это состояние опасно не только ухудшением физического самочувствия, но и риском серьезных осложнений, таких как инсульт или инфаркт.
Углубиться в тему – [url=https://narcolog-na-dom-moskva55.ru/]http://narcolog-na-dom-moskva55.ru/narkolog-na-dom-kruglosutochno-moskva/[/url]
Кроме того, врач, работающий на выезде, может уделить больше времени пациенту, что позволяет индивидуально подойти к решению проблемы.
Исследовать вопрос подробнее – [url=https://narcolog-na-dom-moskva55.ru/]вызов нарколога на дом в москве[/url]
Зависимость — это серьёзная проблема, которая затрагивает не только человека, но и его семью и окружение. Алкоголизм, наркомания и другие виды зависимостей могут разрушить жизнь, но с профессиональной помощью можно вернуть её в русло нормального функционирования. Наркологическая клиника «Заря Будущего» в Санкт-Петербурге предлагает уникальные программы лечения зависимостей, которые сочетает медико-психологический подход, индивидуальные методики и квалифицированную поддержку.
Детальнее – [url=https://vyvod-iz-zapoya-19.ru/]вывод из запоя[/url]
Когда речь идет о лечении зависимостей, многие пациенты испытывают стресс от визита в медицинские учреждения, особенно если это касается такой деликатной темы, как алкоголизм. Лечение на дому помогает избежать всех этих трудностей и обеспечивает комфорт для пациента. Привычная домашняя обстановка снижает тревожность, что позитивно влияет на процесс выздоровления.
Выяснить больше – [url=https://narcolog-na-dom-ektb55.ru/]http://narcolog-na-dom-ektb55.ru/narkolog-na-dom-ekaterinburg-tseny[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Выяснить больше – [url=https://vyvod-iz-zapoya-19.ru/]вывод из запоя санкт-петербург[/url]
Когда речь идет о лечении зависимостей, многие пациенты испытывают стресс от визита в медицинские учреждения, особенно если это касается такой деликатной темы, как алкоголизм. Лечение на дому помогает избежать всех этих трудностей и обеспечивает комфорт для пациента. Привычная домашняя обстановка снижает тревожность, что позитивно влияет на процесс выздоровления.
Получить дополнительные сведения – [url=https://narcolog-na-dom-ektb55.ru/]narkolog na dom [/url]
Стоимость услуг в Екатеринбурге может различаться в зависимости от конкретного случая. Консультация нарколога обычно стоит от 3 000 до 5 000 рублей, тогда как услуга вывода из запоя обойдётся в 5 000–12 000 рублей. Более комплексные программы, такие как детоксикация организма или курсы лечения, могут стоить от 10 000 до 20 000 рублей.
Получить дополнительную информацию – [url=https://narcolog-na-dom-ektb55.ru/]http://narcolog-na-dom-ektb55.ru/narkolog-na-dom-kruglosutochno-ekaterinburg/[/url]
Одной из самых частых причин является затяжной запой. Алкогольная интоксикация, сопровождающая это состояние, не только ухудшает общее самочувствие, но и создает угрозу для здоровья. Повышенное давление, нарушения сердечного ритма, головные боли и тошнота — это лишь некоторые симптомы, которые требуют вмешательства врача.
Получить дополнительную информацию – [url=https://narcolog-na-dom-moskva55.ru/]http://narcolog-na-dom-moskva55.ru/narkolog-na-dom-moskva-tseny[/url]
Цены на услуги зависят от нескольких факторов. Один из ключевых – квалификация специалиста. Опытные врачи, обладающие высоким уровнем профессионализма, оценивают свою работу дороже, но их помощь часто оказывается более эффективной.
Получить дополнительные сведения – [url=https://narcolog-na-dom-moskva55.ru/]нарколог на дом в москве[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьезное воздействие этанола, что приводит к нарушению нормальной физиологической деятельности. Это состояние сопровождается сильными физическими и психологическими расстройствами, которые требуют профессионального вмешательства. Однако многие люди, страдающие алкогольной зависимостью, сталкиваются с трудностью признания своей проблемы, опасаясь утраты репутации и конфиденциальности. В клинике «Заря Будущего» мы предлагаем анонимный вывод из запоя в Санкт-Петербурге, предоставляя высококачественное лечение с гарантией сохранения личной информации.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя капельница на дому санкт-петербург[/url]
Запой — это опасное состояние, которое развивается у людей с алкогольной зависимостью и характеризуется длительным непрерывным потреблением алкоголя. Это состояние приводит к серьезным нарушениям в организме, как физическим, так и психоэмоциональным. Длительный запой может вызвать множество осложнений, таких как поражение внутренних органов, нервной системы, а также проблемы в социальной и семейной жизни. Однако современная медицина предоставляет эффективные методы вывода из запоя, и одним из наиболее удобных и востребованных является вывод из запоя на дому.
Узнать больше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu v-sankt-peterburge[/url]
Одной из самых частых причин является затяжной запой. Алкогольная интоксикация, сопровождающая это состояние, не только ухудшает общее самочувствие, но и создает угрозу для здоровья. Повышенное давление, нарушения сердечного ритма, головные боли и тошнота — это лишь некоторые симптомы, которые требуют вмешательства врача.
Подробнее тут – [url=https://narcolog-na-dom-moskva55.ru/]нарколог на дом стоимость москва[/url]
Уникальные аксессуары для швейных мастерских в Москве по оптовым ценам, завоюйте рынок.
Швейная фурнитура оптом [url=https://sewingsupplies.ru/]https://sewingsupplies.ru/[/url] .
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]vyvod iz zapoya s vyezdom cena [/url]
Как правило, для эффективного устранения токсинов из организма и восстановления нормального функционирования всех органов и систем, назначаются специальные препараты. К таким препаратам относятся растворы для восстановления водно-солевого баланса, витамины и медикаменты для поддержания нормальной работы печени, а также препараты, стабилизирующие психоэмоциональное состояние пациента.
Изучить вопрос глубже – [url=https://narcolog-na-dom-ektb55.ru/]платный нарколог на дом екатеринбург[/url]
Спасибо за информацию.
Предлагаю вам [url=https://rufilmonline.com/kriminal/]русские криминальные фильмы[/url] – это настоящее искусство, которое нравится огромному количество зрителей по всему миру. Русские фильмы и сериалы предлагают уникальный взгляд на русскую культуру, историю и обычаи. В настоящее время смотреть русские фильмы и сериалы онлайн стало очень просто за счет множества онлайн кинотеатров. От ужасов до триллеров, от исторических фильмов до современных детективов – выбор огромен. Погрузитесь в захватывающие сюжеты, профессиональную актерскую работу и красивую работу оператора, наслаждаясь кинематографией из России прямо у себя дома.
Клиника «Гармония и Свет» предоставляет качественные услуги нарколога на дому, обеспечивая пациентам комфортное лечение в привычной обстановке. Это позволяет не только своевременно получить квалифицированную помощь, но и сделать процесс лечения максимально непринужденным и анонимным.
Исследовать вопрос подробнее – [url=https://narcolog-na-dom-ektb55.ru/]narcolog-na-dom-ektb55.ru/[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьёзное воздействие этанола. Это приводит к нарушениям нормальной физиологической деятельности и ухудшению состояния здоровья. Анонимный вывод из запоя — это медицинская помощь, направленная на устранение последствий чрезмерного употребления алкоголя с соблюдением строгой конфиденциальности.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-anonimno-v-ekaterinburge/]vyvod iz zapoya anonimno nedorogo ekaterinburg[/url]
Запой — это опасное состояние, которое развивается у людей с алкогольной зависимостью и характеризуется длительным непрерывным потреблением алкоголя. Это состояние приводит к серьезным нарушениям в организме, как физическим, так и психоэмоциональным. Длительный запой может вызвать множество осложнений, таких как поражение внутренних органов, нервной системы, а также проблемы в социальной и семейной жизни. Однако современная медицина предоставляет эффективные методы вывода из запоя, и одним из наиболее удобных и востребованных является вывод из запоя на дому.
Подробнее тут – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]наркология вывод из запоя анонимно в санкт-петербурге[/url]
Кроме того, анонимность является важной составляющей этой услуги. Лечение дома исключает возможность столкновения с посторонними людьми и помогает пациентам сохранить свою репутацию. Клиника «Гармония и Свет» обеспечивает полную конфиденциальность, предоставляя пациентам возможность получать помощь без лишних вопросов и обсуждений.
Разобраться лучше – [url=https://narcolog-na-dom-ektb55.ru/]вызов нарколога цена в екатеринбурге[/url]
Для многих пациентов важным моментом является сохранение конфиденциальности. Врач, приехавший на дом, работает с полной защитой данных. Пациент может быть уверен, что его проблема останется личной и не станет достоянием общественности. Врачи приезжают на неприметных автомобилях и избегают всяческих ситуаций, которые могут привлечь лишнее внимание.
Подробнее можно узнать тут – [url=https://narcolog-na-dom-moskva55.ru/]платный нарколог на дом в москве[/url]
Цены на услуги зависят от нескольких факторов. Один из ключевых – квалификация специалиста. Опытные врачи, обладающие высоким уровнем профессионализма, оценивают свою работу дороже, но их помощь часто оказывается более эффективной.
Выяснить больше – [url=https://narcolog-na-dom-moskva55.ru/]платный нарколог на дом москва[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]narkolog na dom vyvod iz zapoya sankt-peterburg[/url]
Кроме того, анонимность является важной составляющей этой услуги. Лечение дома исключает возможность столкновения с посторонними людьми и помогает пациентам сохранить свою репутацию. Клиника «Гармония и Свет» обеспечивает полную конфиденциальность, предоставляя пациентам возможность получать помощь без лишних вопросов и обсуждений.
Ознакомиться с деталями – [url=https://narcolog-na-dom-ektb55.ru/]частный нарколог на дом в екатеринбурге[/url]
Цены на услуги зависят от нескольких факторов. Один из ключевых – квалификация специалиста. Опытные врачи, обладающие высоким уровнем профессионализма, оценивают свою работу дороже, но их помощь часто оказывается более эффективной.
Получить больше информации – [url=https://narcolog-na-dom-moskva55.ru/]вызов нарколога на дом москва[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьезное воздействие этанола, что приводит к нарушению нормальной физиологической деятельности. Это состояние сопровождается сильными физическими и психологическими расстройствами, которые требуют профессионального вмешательства. Однако многие люди, страдающие алкогольной зависимостью, сталкиваются с трудностью признания своей проблемы, опасаясь утраты репутации и конфиденциальности. В клинике «Заря Будущего» мы предлагаем анонимный вывод из запоя в Санкт-Петербурге, предоставляя высококачественное лечение с гарантией сохранения личной информации.
Выяснить больше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]vyvod iz zapoya srochno kruglosutochno sankt-peterburg[/url]
Запойное пьянство характеризуется продолжительным и интенсивным потреблением алкоголя, что приводит к серьезным физическим и психологическим последствиям. Часто пациенты сталкиваются с различными заболеваниями, такими как цирроз печени, панкреатит, алкогольный делирий и другие. Алкогольное опьянение не только ухудшает качество жизни, но также может угрожать жизни пациента. В условиях стационара лечение может быть более эффективным, однако многие люди предпочитают проходить данную процедуру на дому, чтобы избежать стигматизации и сохранить чувство приватности.
Подробнее – [url=https://vyvod-iz-zapoya-20.ru/]vyvod iz zapoya s vyezdom[/url]
I was skeptical about installing Metamask until I found https://metaduck.org/. Their guides are thorough and trustworthy, making it easy for me to secure my digital assets with confidence. Definitely recommend!
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьезное воздействие этанола, что приводит к нарушению нормальной физиологической деятельности. Это состояние сопровождается сильными физическими и психологическими расстройствами, которые требуют профессионального вмешательства. Однако многие люди, страдающие алкогольной зависимостью, сталкиваются с трудностью признания своей проблемы, опасаясь утраты репутации и конфиденциальности. В клинике «Заря Будущего» мы предлагаем анонимный вывод из запоя в Санкт-Петербурге, предоставляя высококачественное лечение с гарантией сохранения личной информации.
Подробнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya vyzov na dom [/url]
Как правило, для эффективного устранения токсинов из организма и восстановления нормального функционирования всех органов и систем, назначаются специальные препараты. К таким препаратам относятся растворы для восстановления водно-солевого баланса, витамины и медикаменты для поддержания нормальной работы печени, а также препараты, стабилизирующие психоэмоциональное состояние пациента.
Подробнее тут – [url=https://narcolog-na-dom-ektb55.ru/]https://narcolog-na-dom-ektb55.ru/narkolog-na-dom-ekaterinburg-tseny[/url]
Процесс вызова специалиста на дом продуман до мелочей, чтобы максимально упростить задачу для пациента и его близких.
Подробнее тут – [url=https://narcolog-na-dom-moskva55.ru/]частный нарколог на дом москва[/url]
Алкогольный запой представляет собой серьезное состояние, которое требует немедленного медицинского вмешательства. Это состояние характеризуется длительным и бесконтрольным потреблением алкоголя, что приводит к разрушению как физического, так и психоэмоционального здоровья человека. Для эффективного и безопасного вывода из запоя стационарное лечение является оптимальным решением, особенно при тяжелых случаях интоксикации. В стационаре клиники «Заря Будущего» мы предлагаем комплексную программу вывода из запоя, которая включает детоксикацию, восстановление организма и психотерапевтическую помощь.
Подробнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]вывод из запоя цена наркология санкт-петербург[/url]
При вызове нарколога можно рассчитывать на широкий спектр процедур, направленных на стабилизацию состояния пациента.
Подробнее – [url=https://narcolog-na-dom-v-moskve55.ru/]https://narcolog-na-dom-v-moskve55.ru/vyzov-narkologa-na-dom-moskva[/url]
Зависимость — это серьёзная проблема, которая затрагивает не только человека, но и его семью и окружение. Алкоголизм, наркомания и другие виды зависимостей могут разрушить жизнь, но с профессиональной помощью можно вернуть её в русло нормального функционирования. Наркологическая клиника «Заря Будущего» в Санкт-Петербурге предлагает уникальные программы лечения зависимостей, которые сочетает медико-психологический подход, индивидуальные методики и квалифицированную поддержку.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu sankt-peterburg[/url]
Алкогольная зависимость становится всё более распространенной проблемой, требующей внимания как со стороны медицинского сообщества, так и общества в целом. Вывод из запоя — это сложный и ответственный процесс, необходимый для восстановления здоровья пациента и его интеграции в нормальную жизнь. Профессиональное вмешательство позволяет минимизировать последствия длительного употребления алкоголя и нормализовать состояние организма.
Узнать больше – [url=https://vyvod-iz-zapoya-20.ru/]narkologicheskij centr v-ekaterinburge[/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Получить больше информации – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]вывод из запоя срочно круглосуточно в санкт-петербурге[/url]
Процесс вызова специалиста на дом продуман до мелочей, чтобы максимально упростить задачу для пациента и его близких.
Узнать больше – [url=https://narcolog-na-dom-moskva55.ru/]http://narcolog-na-dom-moskva55.ru/vyzov-narkologa-na-dom-moskva/[/url]
Запой — это состояние, при котором человек не может прекратить употребление алкоголя на протяжении длительного времени, что вызывает физическую и психологическую зависимость. Это крайне опасное состояние, требующее немедленного вмешательства специалистов. Наркологическая клиника «Преображение» в Екатеринбурге предоставляет профессиональную помощь в лечении запоя, используя самые эффективные и безопасные методы.
Углубиться в тему – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]vyvod iz zapoya s vyezdom na dom ekaterinburg[/url]
Алкогольный запой представляет собой серьезное состояние, которое требует немедленного медицинского вмешательства. Это состояние характеризуется длительным и бесконтрольным потреблением алкоголя, что приводит к разрушению как физического, так и психоэмоционального здоровья человека. Для эффективного и безопасного вывода из запоя стационарное лечение является оптимальным решением, особенно при тяжелых случаях интоксикации. В стационаре клиники «Заря Будущего» мы предлагаем комплексную программу вывода из запоя, которая включает детоксикацию, восстановление организма и психотерапевтическую помощь.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-19.ru/]vyvod iz zapoya kapelnica[/url]
If you’re confused about installing the Metamask extension, check out https://metapaws.org/. Their step-by-step guide is super helpful and easy to follow.
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]вывод из запоя на дому цена в санкт-петербурге[/url]
Как правило, для эффективного устранения токсинов из организма и восстановления нормального функционирования всех органов и систем, назначаются специальные препараты. К таким препаратам относятся растворы для восстановления водно-солевого баланса, витамины и медикаменты для поддержания нормальной работы печени, а также препараты, стабилизирующие психоэмоциональное состояние пациента.
Подробнее можно узнать тут – [url=https://narcolog-na-dom-ektb55.ru/]narcolog-na-dom-ektb55.ru/[/url]
Запой — это опасное состояние, которое развивается у людей с алкогольной зависимостью и характеризуется длительным непрерывным потреблением алкоголя. Это состояние приводит к серьезным нарушениям в организме, как физическим, так и психоэмоциональным. Длительный запой может вызвать множество осложнений, таких как поражение внутренних органов, нервной системы, а также проблемы в социальной и семейной жизни. Однако современная медицина предоставляет эффективные методы вывода из запоя, и одним из наиболее удобных и востребованных является вывод из запоя на дому.
Подробнее тут – [url=https://vyvod-iz-zapoya-19.ru/]вывод из запоя капельница санкт-петербург[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Подробнее тут – [url=https://vyvod-iz-zapoya-19.ru/]наркологическая клиника санкт-петербург[/url]
Когда речь идет о лечении зависимостей, многие пациенты испытывают стресс от визита в медицинские учреждения, особенно если это касается такой деликатной темы, как алкоголизм. Лечение на дому помогает избежать всех этих трудностей и обеспечивает комфорт для пациента. Привычная домашняя обстановка снижает тревожность, что позитивно влияет на процесс выздоровления.
Ознакомиться с деталями – [url=https://narcolog-na-dom-ektb55.ru/]https://narcolog-na-dom-ektb55.ru/narkolog-na-dom-ekaterinburg-tseny[/url]
Обращение к специалисту требуется в ситуациях, когда самостоятельное справление с проблемой становится невозможным или небезопасным. Одной из наиболее распространенных причин вызова нарколога является алкогольная интоксикация, связанная с запоем. В таких случаях состояние пациента может быть опасным, а попытки справиться с ситуацией без профессионального вмешательства чреваты осложнениями.
Подробнее – [url=https://narcolog-na-dom-v-moskve55.ru/]нарколог в москве[/url]
В клинике работает команда квалифицированных специалистов, которые осуществляют вывод из запоя, обеспечивая полный контроль над состоянием пациента и устраняя все последствия длительного употребления алкоголя.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]srochny vyvod iz zapoya na domu v-ekaterinburge[/url]
Ситуации, требующие вмешательства нарколога, могут быть различными. Наиболее распространенным поводом является состояние тяжелого алкогольного или наркотического опьянения, при котором пациент уже не может самостоятельно справляться с последствиями интоксикации. Это может быть вызвано передозировкой, длительным употреблением веществ или острой реакцией организма на токсичные соединения.
Разобраться лучше – [url=https://narcolog-na-dom-v-moskve55.ru/]вызов нарколога на дом москва[/url]
С увеличением темпа жизни и нарастающим стрессом, многие люди в Екатеринбурге сталкиваются с зависимостями, особенно с алкогольной. Алкогольная зависимость является одной из наиболее распространённых проблем, требующих немедленного вмешательства специалистов. Важно помнить, что в сложных ситуациях, связанных с зависимостью, помощь не должна откладываться. Вызов нарколога на дом в Екатеринбурге становится идеальным вариантом для тех, кто не может или не хочет покидать свой дом, но нуждается в медицинской помощи.
Узнать больше – [url=https://narcolog-na-dom-ektb55.ru/]http://narcolog-na-dom-ektb55.ru/narkolog-na-dom-ekaterinburg-tseny/[/url]
Основной задачей нарколога, выезжающего на дом, является помощь в любой ситуации, связанной с зависимостью. Все процедуры и методики подбираются индивидуально, в зависимости от состояния пациента.
Получить больше информации – [url=https://narcolog-na-dom-moskva55.ru/]нарколог на дом в москве[/url]
Круглосуточная медицинская помощь при алкогольном запое играет важнейшую роль в спасении жизни пациентов и их возвращении к нормальному состоянию. В данной статье рассмотрим ключевые аспекты вывода из запоя в домашних условиях, срочной помощи и работы нарколога.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-20.ru/]vyvod iz zapoya kapelnica ekaterinburg[/url]
Проведение лечения в домашней обстановке значительно снижает уровень стресса и тревожности. Пациенту не нужно тратить силы на поездки в медицинские учреждения, что особенно важно при ограниченной мобильности. Домашняя атмосфера создает идеальные условия для открытого диалога с врачом, что существенно повышает эффективность терапии и шансы на успешное выздоровление.
Выяснить больше – [url=https://narcolog-na-dom-msk55.ru/]http://narcolog-na-dom-msk55.ru/narkolog-na-dom-moskva-tseny/[/url]
В некоторых случаях помощь нарколога требуется для выведения пациента из запоя. Это состояние опасно не только ухудшением физического самочувствия, но и риском серьезных осложнений, таких как инсульт или инфаркт.
Подробнее – [url=https://narcolog-na-dom-moskva55.ru/]http://narcolog-na-dom-moskva55.ru/narkolog-na-dom-kruglosutochno-moskva/[/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]narkologiya vyvod iz zapoya anonimno [/url]
look here
[url=https://myjaxxwallet.us/]jaxx wallet[/url]
Проведение лечения в домашней обстановке значительно снижает уровень стресса и тревожности. Пациенту не нужно тратить силы на поездки в медицинские учреждения, что особенно важно при ограниченной мобильности. Домашняя атмосфера создает идеальные условия для открытого диалога с врачом, что существенно повышает эффективность терапии и шансы на успешное выздоровление.
Получить больше информации – [url=https://narcolog-na-dom-msk55.ru/]http://narcolog-na-dom-msk55.ru/narkolog-na-dom-kruglosutochno-moskva/[/url]
Алкогольный запой представляет собой серьезное состояние, которое требует немедленного медицинского вмешательства. Это состояние характеризуется длительным и бесконтрольным потреблением алкоголя, что приводит к разрушению как физического, так и психоэмоционального здоровья человека. Для эффективного и безопасного вывода из запоя стационарное лечение является оптимальным решением, особенно при тяжелых случаях интоксикации. В стационаре клиники «Заря Будущего» мы предлагаем комплексную программу вывода из запоя, которая включает детоксикацию, восстановление организма и психотерапевтическую помощь.
Подробнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya kapelnica na domu [/url]
Еще одной причиной вызова нарколога может быть абстинентный синдром, возникающий после прекращения употребления алкоголя или наркотиков. Это состояние сопровождается физическими и психологическими проявлениями, такими как дрожь, тревога, бессонница, боли в мышцах.
Получить дополнительную информацию – [url=https://narcolog-na-dom-v-moskve55.ru/]платный нарколог на дом москва[/url]
С увеличением темпа жизни и нарастающим стрессом, многие люди в Екатеринбурге сталкиваются с зависимостями, особенно с алкогольной. Алкогольная зависимость является одной из наиболее распространённых проблем, требующих немедленного вмешательства специалистов. Важно помнить, что в сложных ситуациях, связанных с зависимостью, помощь не должна откладываться. Вызов нарколога на дом в Екатеринбурге становится идеальным вариантом для тех, кто не может или не хочет покидать свой дом, но нуждается в медицинской помощи.
Ознакомиться с деталями – [url=https://narcolog-na-dom-ektb55.ru/]выезд нарколога на дом цена екатеринбург[/url]
Алкогольный запой – это серьёзное состояние, при котором человек теряет контроль над употреблением алкоголя, что приводит к тяжёлой интоксикации организма. Во время запоя нарушаются функции внутренних органов, страдает нервная система, и повышается риск развития опасных осложнений, таких как алкогольный делирий. Это состояние требует незамедлительного медицинского вмешательства.
Выяснить больше – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-v-stacionare-v-ekaterinburge/]vyvod iz zapoya v stacionare [/url]
В первую очередь это вывод из запоя, который проводится с применением современных инфузионных растворов. Процедура включает внутривенное введение препаратов, устраняющих абстиненцию, восстанавливающих водно-солевой баланс и нормализующих работу внутренних органов. Это помогает снять тревожность, нормализовать сон и восстановить общее состояние.
Получить дополнительную информацию – [url=https://narcolog-na-dom-v-moskve55.ru/]платный нарколог на дом москва[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]вывод из запоя цена [/url]
Процесс вызова специалиста на дом продуман до мелочей, чтобы максимально упростить задачу для пациента и его близких.
Получить дополнительные сведения – [url=https://narcolog-na-dom-moskva55.ru/]https://narcolog-na-dom-moskva55.ru/vyzov-narkologa-na-dom-moskva[/url]
Круглосуточная медицинская помощь при алкогольном запое играет важнейшую роль в спасении жизни пациентов и их возвращении к нормальному состоянию. В данной статье рассмотрим ключевые аспекты вывода из запоя в домашних условиях, срочной помощи и работы нарколога.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-cena-v-ekaterinburge/]vyvod iz zapoya na domu cena ekaterinburg[/url]
read the article [url=https://jaxx-liberty.com]jaxx liberty[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя на дому круглосуточно [/url]
Ситуации, требующие вмешательства нарколога, могут быть различными. Наиболее распространенным поводом является состояние тяжелого алкогольного или наркотического опьянения, при котором пациент уже не может самостоятельно справляться с последствиями интоксикации. Это может быть вызвано передозировкой, длительным употреблением веществ или острой реакцией организма на токсичные соединения.
Разобраться лучше – [url=https://narcolog-na-dom-v-moskve55.ru/]частный нарколог на дом москва[/url]
here are the findings
[url=https://jaxx-wallet.net/]jaxx liberty wallet[/url]
Зависимость — это серьёзная проблема, которая затрагивает не только человека, но и его семью и окружение. Алкоголизм, наркомания и другие виды зависимостей могут разрушить жизнь, но с профессиональной помощью можно вернуть её в русло нормального функционирования. Наркологическая клиника «Заря Будущего» в Санкт-Петербурге предлагает уникальные программы лечения зависимостей, которые сочетает медико-психологический подход, индивидуальные методики и квалифицированную поддержку.
Подробнее тут – [url=https://vyvod-iz-zapoya-19.ru/]наркологический центр санкт-петербург[/url]
С увеличением темпа жизни и нарастающим стрессом, многие люди в Екатеринбурге сталкиваются с зависимостями, особенно с алкогольной. Алкогольная зависимость является одной из наиболее распространённых проблем, требующих немедленного вмешательства специалистов. Важно помнить, что в сложных ситуациях, связанных с зависимостью, помощь не должна откладываться. Вызов нарколога на дом в Екатеринбурге становится идеальным вариантом для тех, кто не может или не хочет покидать свой дом, но нуждается в медицинской помощи.
Подробнее – [url=https://narcolog-na-dom-ektb55.ru/]нарколог на дом екатеринбург[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьёзное воздействие этанола. Это приводит к нарушениям нормальной физиологической деятельности и ухудшению состояния здоровья. Анонимный вывод из запоя — это медицинская помощь, направленная на устранение последствий чрезмерного употребления алкоголя с соблюдением строгой конфиденциальности.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-20.ru/]vyvod iz zapoya gorod ekaterinburg[/url]
Проблемы с алкоголем или наркотиками могут появиться в самый неподходящий момент, и зачастую требуется срочная помощь специалиста. Нередко необходим срочный вызов врача в следующих ситуациях:
Выяснить больше – [url=https://narcolog-na-dom-msk55.ru/]вызов нарколога на дом москва[/url]
Алкоголизм — это хроническое прогрессирующее заболевание, связанное с физической и психической зависимостью от этанола. Запой, как его крайнее проявление, представляет собой длительное употребление алкоголя, сопровождающееся тяжелыми нарушениями работы организма, включая абстинентный синдром и потенциальные угрозы для жизни. Лечение запоя требует комплексного подхода, включающего медицинские процедуры, психотерапию и реабилитационные меры.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]вывод из запоя капельница на дому [/url]
Ситуации, требующие вмешательства нарколога, могут быть различными. Наиболее распространенным поводом является состояние тяжелого алкогольного или наркотического опьянения, при котором пациент уже не может самостоятельно справляться с последствиями интоксикации. Это может быть вызвано передозировкой, длительным употреблением веществ или острой реакцией организма на токсичные соединения.
Подробнее тут – [url=https://narcolog-na-dom-v-moskve55.ru/]https://narcolog-na-dom-v-moskve55.ru/[/url]
Наркологическая помощь на дому необходима в ряде случаев, связанных с острыми состояниями пациента или невозможностью посетить клинику. Часто это ситуации, когда человек испытывает тяжелые последствия запоя, сильную интоксикацию или симптомы, угрожающие здоровью.
Изучить вопрос глубже – [url=https://narcolog-na-dom-moskva55.ru/]платный нарколог на дом в москве[/url]
Алкогольный запой — одно из самых опасных проявлений зависимости, требующее немедленного вмешательства. Длительное употребление спиртного приводит к интоксикации организма, поражает внутренние органы, нарушает работу нервной системы и может привести к необратимым последствиям. Бесконтрольное пьянство сопровождается высокой физической и психологической зависимостью, и выйти из этого состояния самостоятельно практически невозможно.
Подробнее – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]срочный вывод из запоя на дому в санкт-петербурге[/url]
Важно отметить, что некоторые клиники предоставляют скидки для новых клиентов или на отдельные процедуры. Однако стоит избегать слишком низких цен, так как это может свидетельствовать о недостаточном уровне профессионализма или отсутствии лицензии у специалиста.
Выяснить больше – [url=https://narcolog-na-dom-ektb55.ru/]http://narcolog-na-dom-ektb55.ru/[/url]
Ситуации, требующие вмешательства нарколога, могут быть различными. Наиболее распространенным поводом является состояние тяжелого алкогольного или наркотического опьянения, при котором пациент уже не может самостоятельно справляться с последствиями интоксикации. Это может быть вызвано передозировкой, длительным употреблением веществ или острой реакцией организма на токсичные соединения.
Изучить вопрос глубже – [url=https://narcolog-na-dom-v-moskve55.ru/]http://narcolog-na-dom-v-moskve55.ru/narkolog-na-dom-moskva-tseny[/url]
Ситуации, требующие вмешательства нарколога, могут быть различными. Наиболее распространенным поводом является состояние тяжелого алкогольного или наркотического опьянения, при котором пациент уже не может самостоятельно справляться с последствиями интоксикации. Это может быть вызвано передозировкой, длительным употреблением веществ или острой реакцией организма на токсичные соединения.
Изучить вопрос глубже – [url=https://narcolog-na-dom-v-moskve55.ru/]нарколог на дом недорого в москве[/url]
Когда речь идет о лечении зависимостей, многие пациенты испытывают стресс от визита в медицинские учреждения, особенно если это касается такой деликатной темы, как алкоголизм. Лечение на дому помогает избежать всех этих трудностей и обеспечивает комфорт для пациента. Привычная домашняя обстановка снижает тревожность, что позитивно влияет на процесс выздоровления.
Изучить вопрос глубже – [url=https://narcolog-na-dom-ektb55.ru/]частный нарколог на дом екатеринбург[/url]
Алкогольный запой – это серьёзное состояние, при котором человек теряет контроль над употреблением алкоголя, что приводит к тяжёлой интоксикации организма. Во время запоя нарушаются функции внутренних органов, страдает нервная система, и повышается риск развития опасных осложнений, таких как алкогольный делирий. Это состояние требует незамедлительного медицинского вмешательства.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]вывод из запоя врач на дом екатеринбург[/url]
В клинике работает команда квалифицированных специалистов, которые осуществляют вывод из запоя, обеспечивая полный контроль над состоянием пациента и устраняя все последствия длительного употребления алкоголя.
Разобраться лучше – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-cena-v-ekaterinburge/]vyvod iz zapoya na domu cena [/url]
Запой — это состояние, при котором человек не может прекратить употребление алкоголя на протяжении длительного времени, что вызывает физическую и психологическую зависимость. Это крайне опасное состояние, требующее немедленного вмешательства специалистов. Наркологическая клиника «Преображение» в Екатеринбурге предоставляет профессиональную помощь в лечении запоя, используя самые эффективные и безопасные методы.
Подробнее – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]vyvod iz zapoya kapelnica na domu v-ekaterinburge[/url]
Нарколог на дом в Москве предоставляет комплексную помощь, адаптированную под каждого пациента:
Подробнее можно узнать тут – [url=https://narcolog-na-dom-msk55.ru/]вызов нарколога ценамосква[/url]
В первую очередь это вывод из запоя, который проводится с применением современных инфузионных растворов. Процедура включает внутривенное введение препаратов, устраняющих абстиненцию, восстанавливающих водно-солевой баланс и нормализующих работу внутренних органов. Это помогает снять тревожность, нормализовать сон и восстановить общее состояние.
Получить больше информации – [url=https://narcolog-na-dom-v-moskve55.ru/]https://narcolog-na-dom-v-moskve55.ru/narkolog-na-dom-kruglosutochno-moskva/[/url]
Для многих пациентов важным моментом является сохранение конфиденциальности. Врач, приехавший на дом, работает с полной защитой данных. Пациент может быть уверен, что его проблема останется личной и не станет достоянием общественности. Врачи приезжают на неприметных автомобилях и избегают всяческих ситуаций, которые могут привлечь лишнее внимание.
Подробнее – [url=https://narcolog-na-dom-moskva55.ru/]https://narcolog-na-dom-moskva55.ru/[/url]
В клинике «Заря Будущего» мы понимаем, насколько важен своевременный и профессиональный подход к лечению зависимости. Наши специалисты готовы оказать квалифицированную помощь прямо у вас дома, обеспечивая полную безопасность и конфиденциальность на каждом этапе лечения.
Подробнее тут – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]vyvod iz zapoya anonimno nedorogo v-sankt-peterburge[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьезное воздействие этанола, что приводит к нарушению нормальной физиологической деятельности. Это состояние сопровождается сильными физическими и психологическими расстройствами, которые требуют профессионального вмешательства. Однако многие люди, страдающие алкогольной зависимостью, сталкиваются с трудностью признания своей проблемы, опасаясь утраты репутации и конфиденциальности. В клинике «Заря Будущего» мы предлагаем анонимный вывод из запоя в Санкт-Петербурге, предоставляя высококачественное лечение с гарантией сохранения личной информации.
Получить больше информации – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]vyvod iz zapoya kruglosutochno narkologiya [/url]
Запойное пьянство характеризуется продолжительным и интенсивным потреблением алкоголя, что приводит к серьезным физическим и психологическим последствиям. Часто пациенты сталкиваются с различными заболеваниями, такими как цирроз печени, панкреатит, алкогольный делирий и другие. Алкогольное опьянение не только ухудшает качество жизни, но также может угрожать жизни пациента. В условиях стационара лечение может быть более эффективным, однако многие люди предпочитают проходить данную процедуру на дому, чтобы избежать стигматизации и сохранить чувство приватности.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-20.ru/]vyvod iz zapoya s vyezdom v-ekaterinburge[/url]
Детоксикация организма представляет собой комплексное очищение от токсинов. Для этого используются инфузионные растворы, содержащие витамины, антиоксиданты и препараты для поддержки печени. Процедура помогает снизить нагрузку на органы и улучшить общее самочувствие.
Углубиться в тему – [url=https://narcolog-na-dom-ektb55.ru/]нарколог на дом екатеринбург[/url]
find more information
[url=https://jaxx-wallet.net/]jaxx liberty[/url]
В современном мире проблема зависимостей становится всё более острой. Давление повседневных стрессов, сложные жизненные обстоятельства и финансовые трудности нередко приводят людей к алкоголизму, наркомании и игровым расстройствам. Эти состояния не только разрушают здоровье, но и лишают человека социальной стабильности.
Детальнее – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]вывод из запоя анонимно недорого [/url]
В первую очередь это вывод из запоя, который проводится с применением современных инфузионных растворов. Процедура включает внутривенное введение препаратов, устраняющих абстиненцию, восстанавливающих водно-солевой баланс и нормализующих работу внутренних органов. Это помогает снять тревожность, нормализовать сон и восстановить общее состояние.
Детальнее – [url=https://narcolog-na-dom-v-moskve55.ru/]частный нарколог на дом москва[/url]
Запой — это состояние, при котором человек не может прекратить употребление алкоголя на протяжении длительного времени, что вызывает физическую и психологическую зависимость. Это крайне опасное состояние, требующее немедленного вмешательства специалистов. Наркологическая клиника «Преображение» в Екатеринбурге предоставляет профессиональную помощь в лечении запоя, используя самые эффективные и безопасные методы.
Узнать больше – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-v-stacionare-v-ekaterinburge/]bystry vyvod iz zapoya v stacionare ekaterinburg[/url]
Процесс вызова нарколога достаточно прост и удобен для пациента и его близких.
Исследовать вопрос подробнее – [url=https://narcolog-na-dom-msk55.ru/]вызов нарколога на дом цена москва[/url]
В некоторых случаях помощь нарколога требуется для выведения пациента из запоя. Это состояние опасно не только ухудшением физического самочувствия, но и риском серьезных осложнений, таких как инсульт или инфаркт.
Углубиться в тему – [url=https://narcolog-na-dom-moskva55.ru/]вызов нарколога на дом москва[/url]
This Site [url=https://web-freewallet.com]freewallet io[/url]
Важно отметить, что некоторые клиники предоставляют скидки для новых клиентов или на отдельные процедуры. Однако стоит избегать слишком низких цен, так как это может свидетельствовать о недостаточном уровне профессионализма или отсутствии лицензии у специалиста.
Выяснить больше – [url=https://narcolog-na-dom-ektb55.ru/]narkolog na dom [/url]
Клиника «Второй Шанс» создана для комплексной помощи тем, кто столкнулся с зависимостью. Мы не просто снимаем симптомы, а работаем над коренными причинами проблемы, помогая пациентам восстановить физическое и психологическое состояние, адаптироваться к жизни без вредных привычек и избежать рецидивов.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-14.ru/]наркологический центр в санкт-петербурге[/url]
Отсутствие визитов в клинику гарантирует полную конфиденциальность. Это особенно важно для пациентов, беспокоящихся о своей репутации или возможном влиянии на профессиональную деятельность. Вызов врача на дом полностью исключает нежелательные встречи в медицинском центре.
Получить дополнительные сведения – [url=https://narcolog-na-dom-msk55.ru/]нарколог на дом недорого москва[/url]
visite site
[url=https://jaxx-liberty.com/]jaxx io[/url]
Кроме того, врач, работающий на выезде, может уделить больше времени пациенту, что позволяет индивидуально подойти к решению проблемы.
Углубиться в тему – [url=https://narcolog-na-dom-moskva55.ru/]http://narcolog-na-dom-moskva55.ru/narkolog-na-dom-kruglosutochno-moskva/[/url]
С увеличением темпа жизни и нарастающим стрессом, многие люди в Екатеринбурге сталкиваются с зависимостями, особенно с алкогольной. Алкогольная зависимость является одной из наиболее распространённых проблем, требующих немедленного вмешательства специалистов. Важно помнить, что в сложных ситуациях, связанных с зависимостью, помощь не должна откладываться. Вызов нарколога на дом в Екатеринбурге становится идеальным вариантом для тех, кто не может или не хочет покидать свой дом, но нуждается в медицинской помощи.
Разобраться лучше – [url=https://narcolog-na-dom-ektb55.ru/]вызов нарколога цена в екатеринбурге[/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]vyvod iz zapoya cena narkologiya sankt-peterburg[/url]
Важно отметить, что некоторые клиники предоставляют скидки для новых клиентов или на отдельные процедуры. Однако стоит избегать слишком низких цен, так как это может свидетельствовать о недостаточном уровне профессионализма или отсутствии лицензии у специалиста.
Исследовать вопрос подробнее – [url=https://narcolog-na-dom-ektb55.ru/]http://narcolog-na-dom-ektb55.ru/[/url]
При рассмотрении вопроса о наркологической помощи на дому, необходимо четко представлять спектр доступных услуг:
Получить дополнительные сведения – [url=https://narcolog-na-dom-msk55.ru/]нарколог на дом стоимость москва[/url]
Услуги нарколога на дому включают весь спектр мероприятий, направленных на стабилизацию состояния пациента и помощь в борьбе с зависимостью. Среди наиболее востребованных процедур:
Получить дополнительные сведения – [url=https://narcolog-na-dom-v-moskve55.ru/]http://narcolog-na-dom-v-moskve55.ru/narkolog-na-dom-moskva-tseny[/url]
Преимущества домашнего лечения также заключаются в индивидуальном подходе. Врач, находясь в вашем доме, может более тщательно изучить ситуацию, провести необходимую диагностику и подобрать курс лечения, который идеально подойдет вашему состоянию. В отличие от клиники, где внимание врача часто ограничено временем, на дому можно уделить пациенту больше времени, подбирая лечение с учетом его особенностей.
Подробнее – [url=https://narcolog-na-dom-moskva55.ru/]https://narcolog-na-dom-moskva55.ru/narkolog-na-dom-moskva-tseny[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Детальнее – [url=https://vyvod-iz-zapoya-19.ru/]вывод из запоя с выездом в санкт-петербурге[/url]
Когда речь идет о лечении зависимостей, многие пациенты испытывают стресс от визита в медицинские учреждения, особенно если это касается такой деликатной темы, как алкоголизм. Лечение на дому помогает избежать всех этих трудностей и обеспечивает комфорт для пациента. Привычная домашняя обстановка снижает тревожность, что позитивно влияет на процесс выздоровления.
Подробнее тут – [url=https://narcolog-na-dom-ektb55.ru/]выезд нарколога на дом цена екатеринбург[/url]
Алкогольная зависимость становится всё более распространенной проблемой, требующей внимания как со стороны медицинского сообщества, так и общества в целом. Вывод из запоя — это сложный и ответственный процесс, необходимый для восстановления здоровья пациента и его интеграции в нормальную жизнь. Профессиональное вмешательство позволяет минимизировать последствия длительного употребления алкоголя и нормализовать состояние организма.
Получить больше информации – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]вывод из запоя на дому круглосуточно [/url]
Специалисты клиники «Второй Шанс» предлагают профессиональную медицинскую помощь при запоях, обеспечивая безопасный и эффективный вывод из этого состояния. Для удобства пациентов мы оказываем услуги как в условиях стационара, так и на дому. Благодаря современным методам детоксикации и индивидуальному подходу наши врачи помогают минимизировать риски и ускорить процесс восстановления.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]vyvod iz zapoya anonimno [/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Узнать больше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]narkologiya vyvod iz zapoya na domu v-sankt-peterburge[/url]
Стоимость услуги формируется на основе различных факторов, включая сложность состояния пациента, срочность вызова, квалификацию врача и расстояние до места вызова. Чтобы сделать правильный выбор, важно учитывать все аспекты, влияющие на цену, и понимать, как получить качественную помощь по справедливой стоимости.
Выяснить больше – [url=https://narcolog-na-dom-ektb55.ru/]http://narcolog-na-dom-ektb55.ru/narkolog-na-dom-ekaterinburg-tseny[/url]
В современном мире проблема зависимостей становится всё более острой. Давление повседневных стрессов, сложные жизненные обстоятельства и финансовые трудности нередко приводят людей к алкоголизму, наркомании и игровым расстройствам. Эти состояния не только разрушают здоровье, но и лишают человека социальной стабильности.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя на дому круглосуточно в санкт-петербурге[/url]
В клинике «Заря Будущего» мы понимаем, насколько важен своевременный и профессиональный подход к лечению зависимости. Наши специалисты готовы оказать квалифицированную помощь прямо у вас дома, обеспечивая полную безопасность и конфиденциальность на каждом этапе лечения.
Углубиться в тему – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя на дому недорого капельница санкт-петербург[/url]
Стоимость услуг в Екатеринбурге может различаться в зависимости от конкретного случая. Консультация нарколога обычно стоит от 3 000 до 5 000 рублей, тогда как услуга вывода из запоя обойдётся в 5 000–12 000 рублей. Более комплексные программы, такие как детоксикация организма или курсы лечения, могут стоить от 10 000 до 20 000 рублей.
Изучить вопрос глубже – [url=https://narcolog-na-dom-ektb55.ru/]https://narcolog-na-dom-ektb55.ru/narkolog-na-dom-ekaterinburg-tseny/[/url]
Наркологическая помощь на дому необходима в ряде случаев, связанных с острыми состояниями пациента или невозможностью посетить клинику. Часто это ситуации, когда человек испытывает тяжелые последствия запоя, сильную интоксикацию или симптомы, угрожающие здоровью.
Детальнее – [url=https://narcolog-na-dom-moskva55.ru/]https://narcolog-na-dom-moskva55.ru/[/url]
В клинике работает команда квалифицированных специалистов, которые осуществляют вывод из запоя, обеспечивая полный контроль над состоянием пациента и устраняя все последствия длительного употребления алкоголя.
Выяснить больше – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-cena-v-ekaterinburge/]вывод из запоя с выездом цена [/url]
Nice post. I learn something new and challenging on blogs I stumble upon everyday. It will always be useful to read through articles from other writers and practice something from other websites.
온라인바카라
Клиника «Второй Шанс» создана для комплексной помощи тем, кто столкнулся с зависимостью. Мы не просто снимаем симптомы, а работаем над коренными причинами проблемы, помогая пациентам восстановить физическое и психологическое состояние, адаптироваться к жизни без вредных привычек и избежать рецидивов.
Получить больше информации – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]vyvod iz zapoya s vyezdom cena sankt-peterburg[/url]
Преимущества домашнего лечения также заключаются в индивидуальном подходе. Врач, находясь в вашем доме, может более тщательно изучить ситуацию, провести необходимую диагностику и подобрать курс лечения, который идеально подойдет вашему состоянию. В отличие от клиники, где внимание врача часто ограничено временем, на дому можно уделить пациенту больше времени, подбирая лечение с учетом его особенностей.
Исследовать вопрос подробнее – [url=https://narcolog-na-dom-moskva55.ru/]нарколог на дом в москве[/url]
Современный ритм жизни в Москве, с его постоянным стрессом, высокой интенсивностью и зачастую перегрузками, становится одной из причин, провоцирующих развитие зависимостей. Проблемы с алкоголем и наркотиками не всегда решаются быстро, и чаще всего требуют немедленного вмешательства профессионалов. В таких случаях вызов нарколога на дом становится оптимальным решением, которое сочетает комфорт, безопасность и высокий уровень медицинской поддержки.
Выяснить больше – [url=https://narcolog-na-dom-v-moskve55.ru/]нарколог на дом в москве[/url]
Клиника «Второй Шанс» в Санкт-Петербурге работает круглосуточно, предоставляя помощь в любой момент. Мы гарантируем конфиденциальность, внимательное отношение и профессиональный подход на каждом этапе лечения. Наша цель — не просто вывести человека из состояния запоя, а помочь ему вернуться к полноценной жизни без зависимости.
Выяснить больше – [url=https://vyvod-iz-zapoya-14.ru/]http://www.vyvod-iz-zapoya-14.ru[/url]
Кроме того, врач, работающий на выезде, может уделить больше времени пациенту, что позволяет индивидуально подойти к решению проблемы.
Подробнее – [url=https://narcolog-na-dom-moskva55.ru/]вызов нарколога на дом цена москва[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Углубиться в тему – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]вывод из запоя цена санкт-петербург[/url]
Клиника «Второй Шанс» создана для комплексной помощи тем, кто столкнулся с зависимостью. Мы не просто снимаем симптомы, а работаем над коренными причинами проблемы, помогая пациентам восстановить физическое и психологическое состояние, адаптироваться к жизни без вредных привычек и избежать рецидивов.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]narkologiya vyvod iz zapoya anonimno sankt-peterburg[/url]
Для многих пациентов важным моментом является сохранение конфиденциальности. Врач, приехавший на дом, работает с полной защитой данных. Пациент может быть уверен, что его проблема останется личной и не станет достоянием общественности. Врачи приезжают на неприметных автомобилях и избегают всяческих ситуаций, которые могут привлечь лишнее внимание.
Получить дополнительные сведения – [url=https://narcolog-na-dom-moskva55.ru/]https://narcolog-na-dom-moskva55.ru/[/url]
Зависимость — это серьёзная проблема, которая затрагивает не только человека, но и его семью и окружение. Алкоголизм, наркомания и другие виды зависимостей могут разрушить жизнь, но с профессиональной помощью можно вернуть её в русло нормального функционирования. Наркологическая клиника «Заря Будущего» в Санкт-Петербурге предлагает уникальные программы лечения зависимостей, которые сочетает медико-психологический подход, индивидуальные методики и квалифицированную поддержку.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]vyvod iz zapoya anonimno v-sankt-peterburge[/url]
Когда речь идет о лечении зависимостей, многие пациенты испытывают стресс от визита в медицинские учреждения, особенно если это касается такой деликатной темы, как алкоголизм. Лечение на дому помогает избежать всех этих трудностей и обеспечивает комфорт для пациента. Привычная домашняя обстановка снижает тревожность, что позитивно влияет на процесс выздоровления.
Углубиться в тему – [url=https://narcolog-na-dom-ektb55.ru/]https://narcolog-na-dom-ektb55.ru/[/url]
Важно понимать, что алкоголизм — это хроническое заболевание, требующее комплексного лечения. Одной детоксикации недостаточно для полного избавления от зависимости. После выхода из запоя необходимо продолжить терапию, включающую психологическую поддержку, медикаментозное лечение и социальную адаптацию.
Узнать больше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя на дому круглосуточно санкт-петербург[/url]
Клиника «Второй Шанс» создана для комплексной помощи тем, кто столкнулся с зависимостью. Мы не просто снимаем симптомы, а работаем над коренными причинами проблемы, помогая пациентам восстановить физическое и психологическое состояние, адаптироваться к жизни без вредных привычек и избежать рецидивов.
Выяснить больше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя врач на дом в санкт-петербурге[/url]
Одной из самых частых причин является затяжной запой. Алкогольная интоксикация, сопровождающая это состояние, не только ухудшает общее самочувствие, но и создает угрозу для здоровья. Повышенное давление, нарушения сердечного ритма, головные боли и тошнота — это лишь некоторые симптомы, которые требуют вмешательства врача.
Получить больше информации – [url=https://narcolog-na-dom-moskva55.ru/]вызов нарколога на дом цена в москве[/url]
Важно понимать, что алкоголизм — это хроническое заболевание, требующее комплексного лечения. Одной детоксикации недостаточно для полного избавления от зависимости. После выхода из запоя необходимо продолжить терапию, включающую психологическую поддержку, медикаментозное лечение и социальную адаптацию.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]вывод из запоя анонимно в санкт-петербурге[/url]
Для многих пациентов важным моментом является сохранение конфиденциальности. Врач, приехавший на дом, работает с полной защитой данных. Пациент может быть уверен, что его проблема останется личной и не станет достоянием общественности. Врачи приезжают на неприметных автомобилях и избегают всяческих ситуаций, которые могут привлечь лишнее внимание.
Изучить вопрос глубже – [url=https://narcolog-na-dom-moskva55.ru/]платный нарколог на дом москва[/url]
Стоимость услуг в Екатеринбурге может различаться в зависимости от конкретного случая. Консультация нарколога обычно стоит от 3 000 до 5 000 рублей, тогда как услуга вывода из запоя обойдётся в 5 000–12 000 рублей. Более комплексные программы, такие как детоксикация организма или курсы лечения, могут стоить от 10 000 до 20 000 рублей.
Получить дополнительные сведения – [url=https://narcolog-na-dom-ektb55.ru/]вызов нарколога ценаекатеринбург[/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Подробнее тут – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]vyvod iz zapoya anonimno nedorogo [/url]
При вызове нарколога можно рассчитывать на широкий спектр процедур, направленных на стабилизацию состояния пациента.
Узнать больше – [url=https://narcolog-na-dom-v-moskve55.ru/]нарколог москва[/url]
Круглосуточная медицинская помощь при алкогольном запое играет важнейшую роль в спасении жизни пациентов и их возвращении к нормальному состоянию. В данной статье рассмотрим ключевые аспекты вывода из запоя в домашних условиях, срочной помощи и работы нарколога.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-20.ru/]vyvod iz zapoya gorod ekaterinburg[/url]
В современном мире проблема зависимостей становится всё более острой. Давление повседневных стрессов, сложные жизненные обстоятельства и финансовые трудности нередко приводят людей к алкоголизму, наркомании и игровым расстройствам. Эти состояния не только разрушают здоровье, но и лишают человека социальной стабильности.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya kapelnica na domu [/url]
Ритм жизни в мегаполисе и постоянный стресс часто становятся причиной развития зависимостей у жителей города. В такой ситуации необходима профессиональная консультация врача. Оптимальное решение – вызов нарколога на дом в Москве. Это обеспечивает не только получение медицинской помощи в привычной обстановке, но и полную конфиденциальность.
Выяснить больше – [url=https://narcolog-na-dom-msk55.ru/]http://narcolog-na-dom-msk55.ru/vyzov-narkologa-na-dom-moskva[/url]
Важно отметить, что некоторые клиники предоставляют скидки для новых клиентов или на отдельные процедуры. Однако стоит избегать слишком низких цен, так как это может свидетельствовать о недостаточном уровне профессионализма или отсутствии лицензии у специалиста.
Получить больше информации – [url=https://narcolog-na-dom-ektb55.ru/]вызов нарколога на дом цена в екатеринбурге[/url]
Важно отметить, что некоторые клиники предоставляют скидки для новых клиентов или на отдельные процедуры. Однако стоит избегать слишком низких цен, так как это может свидетельствовать о недостаточном уровне профессионализма или отсутствии лицензии у специалиста.
Подробнее – [url=https://narcolog-na-dom-ektb55.ru/]платный нарколог на дом в екатеринбурге[/url]
По завершению напряжённого дня, так хочется расслабиться, зарядиться позитивом и подарить себе полноценное восстановление. В Termburg.ru для этого созданы комфортная атмосфера: огромный водный комплекс с гидромассажем, тёплые бассейны на свежем воздухе и комфортные лаунж-пространства. Для любителей прогреть мышцы и укрепить иммунитет работают [url=https://termburg.ru/timetable/]термбург график работы[/url] включая русскую парную, финскую сауну и турецкую баню. Здесь можно не просто насладиться тишиной, но и получить процедуры с лечебным воздействием, укрепляя иммунитет.
Посетители ценят нас за уютную обстановку и разнообразие спа-процедур – [url=https://termburg.ru/]термбург ул гурьянова 30[/url] предлагает сеансы массажа, контрастные купели, терапию эфирными маслами и уникальные программы восстановления для души и тела. Подарите себе день расслабления в “Термбург” уже сегодня и познайте место, где забота о здоровье сочетается с настоящим отдыхом!
Круглосуточная медицинская помощь при алкогольном запое играет важнейшую роль в спасении жизни пациентов и их возвращении к нормальному состоянию. В данной статье рассмотрим ключевые аспекты вывода из запоя в домашних условиях, срочной помощи и работы нарколога.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-cena-v-ekaterinburge/]вывод из запоя цена в екатеринбурге[/url]
Интоксикация организма, вызванная наркотиками, также является весомой причиной для вызова врача. Передозировка, смешение веществ или использование низкокачественных препаратов могут вызвать серьезные последствия для организма, включая потерю сознания, нарушения дыхания и сердечный приступ.
Исследовать вопрос подробнее – [url=https://narcolog-na-dom-v-moskve55.ru/]вызов нарколога на дом москва[/url]
В клинике «Заря Будущего» мы понимаем, насколько важен своевременный и профессиональный подход к лечению зависимости. Наши специалисты готовы оказать квалифицированную помощь прямо у вас дома, обеспечивая полную безопасность и конфиденциальность на каждом этапе лечения.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]narkologiya vyvod iz zapoya na domu v-sankt-peterburge[/url]
Процесс вызова нарколога достаточно прост и удобен для пациента и его близких.
Получить дополнительные сведения – [url=https://narcolog-na-dom-msk55.ru/]http://narcolog-na-dom-msk55.ru/vyzov-narkologa-na-dom-moskva[/url]
Алкогольная зависимость становится всё более распространенной проблемой, требующей внимания как со стороны медицинского сообщества, так и общества в целом. Вывод из запоя — это сложный и ответственный процесс, необходимый для восстановления здоровья пациента и его интеграции в нормальную жизнь. Профессиональное вмешательство позволяет минимизировать последствия длительного употребления алкоголя и нормализовать состояние организма.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-cena-v-ekaterinburge/]vyvod iz zapoya na domu cena ekaterinburg[/url]
Кроме того, анонимность является важной составляющей этой услуги. Лечение дома исключает возможность столкновения с посторонними людьми и помогает пациентам сохранить свою репутацию. Клиника «Гармония и Свет» обеспечивает полную конфиденциальность, предоставляя пациентам возможность получать помощь без лишних вопросов и обсуждений.
Ознакомиться с деталями – [url=https://narcolog-na-dom-ektb55.ru/]вызов нарколога на дом екатеринбург[/url]
Стоимость услуг в Екатеринбурге может различаться в зависимости от конкретного случая. Консультация нарколога обычно стоит от 3 000 до 5 000 рублей, тогда как услуга вывода из запоя обойдётся в 5 000–12 000 рублей. Более комплексные программы, такие как детоксикация организма или курсы лечения, могут стоить от 10 000 до 20 000 рублей.
Ознакомиться с деталями – [url=https://narcolog-na-dom-ektb55.ru/]вызов нарколога на дом екатеринбург[/url]
Специалисты клиники «Второй Шанс» предлагают профессиональную медицинскую помощь при запоях, обеспечивая безопасный и эффективный вывод из этого состояния. Для удобства пациентов мы оказываем услуги как в условиях стационара, так и на дому. Благодаря современным методам детоксикации и индивидуальному подходу наши врачи помогают минимизировать риски и ускорить процесс восстановления.
Выяснить больше – [url=https://vyvod-iz-zapoya-14.ru/]http://vyvod-iz-zapoya-14.ru[/url]
Современный ритм жизни в Москве, с его постоянным стрессом, высокой интенсивностью и зачастую перегрузками, становится одной из причин, провоцирующих развитие зависимостей. Проблемы с алкоголем и наркотиками не всегда решаются быстро, и чаще всего требуют немедленного вмешательства профессионалов. В таких случаях вызов нарколога на дом становится оптимальным решением, которое сочетает комфорт, безопасность и высокий уровень медицинской поддержки.
Разобраться лучше – [url=https://narcolog-na-dom-v-moskve55.ru/]нарколог на дом стоимость в москве[/url]
Услуги нарколога на дому включают весь спектр мероприятий, направленных на стабилизацию состояния пациента и помощь в борьбе с зависимостью. Среди наиболее востребованных процедур:
Изучить вопрос глубже – [url=https://narcolog-na-dom-v-moskve55.ru/]http://narcolog-na-dom-v-moskve55.ru[/url]
Круглосуточная медицинская помощь при алкогольном запое играет важнейшую роль в спасении жизни пациентов и их возвращении к нормальному состоянию. В данной статье рассмотрим ключевые аспекты вывода из запоя в домашних условиях, срочной помощи и работы нарколога.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]srochny vyvod iz zapoya na domu [/url]
При рассмотрении вопроса о наркологической помощи на дому, необходимо четко представлять спектр доступных услуг:
Разобраться лучше – [url=https://narcolog-na-dom-msk55.ru/]https://narcolog-na-dom-msk55.ru/vyzov-narkologa-na-dom-moskva[/url]
Алкогольный запой представляет собой серьезное состояние, которое требует немедленного медицинского вмешательства. Это состояние характеризуется длительным и бесконтрольным потреблением алкоголя, что приводит к разрушению как физического, так и психоэмоционального здоровья человека. Для эффективного и безопасного вывода из запоя стационарное лечение является оптимальным решением, особенно при тяжелых случаях интоксикации. В стационаре клиники «Заря Будущего» мы предлагаем комплексную программу вывода из запоя, которая включает детоксикацию, восстановление организма и психотерапевтическую помощь.
Углубиться в тему – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]вывод из запоя на дому цена [/url]
Алкогольный запой — одно из самых опасных проявлений зависимости, требующее немедленного вмешательства. Длительное употребление спиртного приводит к интоксикации организма, поражает внутренние органы, нарушает работу нервной системы и может привести к необратимым последствиям. Бесконтрольное пьянство сопровождается высокой физической и психологической зависимостью, и выйти из этого состояния самостоятельно практически невозможно.
Подробнее тут – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]srochny vyvod iz zapoya na domu sankt-peterburg[/url]
Запой — это опасное состояние, которое развивается у людей с алкогольной зависимостью и характеризуется длительным непрерывным потреблением алкоголя. Это состояние приводит к серьезным нарушениям в организме, как физическим, так и психоэмоциональным. Длительный запой может вызвать множество осложнений, таких как поражение внутренних органов, нервной системы, а также проблемы в социальной и семейной жизни. Однако современная медицина предоставляет эффективные методы вывода из запоя, и одним из наиболее удобных и востребованных является вывод из запоя на дому.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]narkolog na dom vyvod iz zapoya v-sankt-peterburge[/url]
Обращение к специалисту требуется в ситуациях, когда самостоятельное справление с проблемой становится невозможным или небезопасным. Одной из наиболее распространенных причин вызова нарколога является алкогольная интоксикация, связанная с запоем. В таких случаях состояние пациента может быть опасным, а попытки справиться с ситуацией без профессионального вмешательства чреваты осложнениями.
Получить дополнительные сведения – [url=https://narcolog-na-dom-v-moskve55.ru/]http://narcolog-na-dom-v-moskve55.ru/vyzov-narkologa-na-dom-moskva/[/url]
Запой — это опасное состояние, которое развивается у людей с алкогольной зависимостью и характеризуется длительным непрерывным потреблением алкоголя. Это состояние приводит к серьезным нарушениям в организме, как физическим, так и психоэмоциональным. Длительный запой может вызвать множество осложнений, таких как поражение внутренних органов, нервной системы, а также проблемы в социальной и семейной жизни. Однако современная медицина предоставляет эффективные методы вывода из запоя, и одним из наиболее удобных и востребованных является вывод из запоя на дому.
Детальнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]vyvod iz zapoya srochno kruglosutochno v-sankt-peterburge[/url]
Цены на услуги зависят от нескольких факторов. Один из ключевых – квалификация специалиста. Опытные врачи, обладающие высоким уровнем профессионализма, оценивают свою работу дороже, но их помощь часто оказывается более эффективной.
Подробнее можно узнать тут – [url=https://narcolog-na-dom-moskva55.ru/]нарколог на дом недорого москва[/url]
Детоксикация организма — еще одна важная процедура, которая позволяет вывести токсины и минимизировать их негативное воздействие на органы. Для этого применяются специальные растворы, содержащие витамины, глюкозу и электролиты. Все компоненты подбираются индивидуально на основе состояния пациента.
Подробнее – [url=https://narcolog-na-dom-v-moskve55.ru/]http://narcolog-na-dom-v-moskve55.ru/vyzov-narkologa-na-dom-moskva[/url]
В современном мире проблема зависимостей становится всё более острой. Давление повседневных стрессов, сложные жизненные обстоятельства и финансовые трудности нередко приводят людей к алкоголизму, наркомании и игровым расстройствам. Эти состояния не только разрушают здоровье, но и лишают человека социальной стабильности.
Подробнее тут – [url=https://vyvod-iz-zapoya-14.ru/]наркологический центр санкт-петербург[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]anonimny vyvod iz zapoya na domu v-sankt-peterburge[/url]
I had trouble adding the Metamask extension to Chrome, but after checking https://download.metaredi.org/, everything was explained so well! Now I can store my crypto safely and make transactions with ease. Thanks for the helpful guide!
Клиника «Преображение» предлагает услуги анонимного вывода из запоя в Екатеринбурге, обеспечивая высокое качество медицинской помощи и соблюдение всех этических норм.
Узнать больше – [url=https://vyvod-iz-zapoya-20.ru/]наркологическая клиника в екатеринбурге[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Детальнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]срочный вывод из запоя на дому в санкт-петербурге[/url]
Основной задачей нарколога, выезжающего на дом, является помощь в любой ситуации, связанной с зависимостью. Все процедуры и методики подбираются индивидуально, в зависимости от состояния пациента.
Ознакомиться с деталями – [url=https://narcolog-na-dom-moskva55.ru/]вызов нарколога на дом москва[/url]
3.Уточните наличие акций и скидок. Многие клиники предлагают специальные условия для новых клиентов или на определенные виды услуг.
Разобраться лучше – [url=https://narcolog-na-dom-msk55.ru/]вызов нарколога на дом в москве[/url]
Именно вам нужно, где провести качественное [url=https://xn--18-6kcdfki0a3cvc9f2b.xn--p1ai/braces/]исправление прикуса брекетами[/url] по современным стандартам? В Стоматологии добрых врачей работают профессиональные специалисты, применяющие передовые технологии и проверенные материалы. Мы предлагаем персонализированный подход, предлагая комплексный подход. Свяжитесь с нами на консультацию, чтобы сохранить здоровье вашей улыбки.
Хотите сохранить зубам эстетику и комфорт? Мы предлагаем [url=https://xn--18-6kcdfki0a3cvc9f2b.xn--p1ai/]исправление мезиального прикуса у взрослых[/url] с использованием новейших технологий. Высококачественные решения, натуральный оттенок и долговечность – вот что вы получите в нашей клинике. Перейдите на сайт добрыеврачи18.рф и уточните подробности, чтобы обрести уверенность в себе!
Клиника «Гармония и Свет» предоставляет качественные услуги нарколога на дому, обеспечивая пациентам комфортное лечение в привычной обстановке. Это позволяет не только своевременно получить квалифицированную помощь, но и сделать процесс лечения максимально непринужденным и анонимным.
Получить дополнительные сведения – [url=https://narcolog-na-dom-ektb55.ru/]http://narcolog-na-dom-ektb55.ru/narkolog-na-dom-kruglosutochno-ekaterinburg/[/url]
Детоксикация организма представляет собой комплексное очищение от токсинов. Для этого используются инфузионные растворы, содержащие витамины, антиоксиданты и препараты для поддержки печени. Процедура помогает снизить нагрузку на органы и улучшить общее самочувствие.
Узнать больше – [url=https://narcolog-na-dom-ektb55.ru/]вызов нарколога на дом в екатеринбурге[/url]
При вызове нарколога можно рассчитывать на широкий спектр процедур, направленных на стабилизацию состояния пациента.
Детальнее – [url=https://narcolog-na-dom-v-moskve55.ru/]выезд нарколога на дом цена в москве[/url]
Основной задачей нарколога, выезжающего на дом, является помощь в любой ситуации, связанной с зависимостью. Все процедуры и методики подбираются индивидуально, в зависимости от состояния пациента.
Получить дополнительную информацию – [url=https://narcolog-na-dom-moskva55.ru/]https://narcolog-na-dom-moskva55.ru/[/url]
Одной из самых частых причин является затяжной запой. Алкогольная интоксикация, сопровождающая это состояние, не только ухудшает общее самочувствие, но и создает угрозу для здоровья. Повышенное давление, нарушения сердечного ритма, головные боли и тошнота — это лишь некоторые симптомы, которые требуют вмешательства врача.
Разобраться лучше – [url=https://narcolog-na-dom-moskva55.ru/]врач нарколог на дом платный москва[/url]
Клиника «Второй Шанс» в Санкт-Петербурге работает круглосуточно, предоставляя помощь в любой момент. Мы гарантируем конфиденциальность, внимательное отношение и профессиональный подход на каждом этапе лечения. Наша цель — не просто вывести человека из состояния запоя, а помочь ему вернуться к полноценной жизни без зависимости.
Узнать больше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]vyvod iz zapoya s vyezdom cena [/url]
Клиника «Преображение» предлагает услуги анонимного вывода из запоя в Екатеринбурге, обеспечивая высокое качество медицинской помощи и соблюдение всех этических норм.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]vyvod iz zapoya na domu kruglosutochno [/url]
Процесс вызова специалиста на дом продуман до мелочей, чтобы максимально упростить задачу для пациента и его близких.
Исследовать вопрос подробнее – [url=https://narcolog-na-dom-moskva55.ru/]платный нарколог на дом в москве[/url]
Зависимость — это серьёзная проблема, которая затрагивает не только человека, но и его семью и окружение. Алкоголизм, наркомания и другие виды зависимостей могут разрушить жизнь, но с профессиональной помощью можно вернуть её в русло нормального функционирования. Наркологическая клиника «Заря Будущего» в Санкт-Петербурге предлагает уникальные программы лечения зависимостей, которые сочетает медико-психологический подход, индивидуальные методики и квалифицированную поддержку.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]вывод из запоя на дому цена санкт-петербург[/url]
Специалисты клиники «Второй Шанс» предлагают профессиональную медицинскую помощь при запоях, обеспечивая безопасный и эффективный вывод из этого состояния. Для удобства пациентов мы оказываем услуги как в условиях стационара, так и на дому. Благодаря современным методам детоксикации и индивидуальному подходу наши врачи помогают минимизировать риски и ускорить процесс восстановления.
Разобраться лучше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]vyvod iz zapoya cena narkologiya sankt-peterburg[/url]
онлайн с со скидкой
заказать картину на холсте с фотографии [url=https://www.zakaz-pechati-na-holste.ru/]https://www.zakaz-pechati-na-holste.ru/[/url] .
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]vyvod iz zapoya cena narkologiya v-voronezhe[/url]
Услуга вызова нарколога на дом в Красноярске — это удобный и современный способ получения медицинской помощи. Она идеально подходит для тех, кто нуждается в профессиональной поддержке, но по каким-либо причинам не может или не хочет посещать клинику.
Исследовать вопрос подробнее – [url=https://narcolog-na-dom-krasnoyarsk55.ru/]http://narcolog-na-dom-krasnoyarsk55.ru[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya kapelnica na domu v-chelyabinske[/url]
Стационарное лечение обеспечивает постоянный контроль медицинского персонала, что гарантирует максимальную безопасность и мониторинг состояния пациента. Это критически важно для пациентов с сопутствующими заболеваниями или осложнениями алкоголизма. Клиники также предоставляют дополнительные услуги: консультации психолога, групповые и индивидуальные терапевтические занятия, способствующие скорейшему выздоровлению и профилактике рецидивов.
Подробнее можно узнать тут – [url=https://kapelnica-ot-zapoya-msk55.ru/]капельницу от запоя москва[/url]
Запой представляет собой состояние, характеризующееся длительным непрерывным употреблением алкоголя, что приводит к физической и психической зависимости. Этот процесс может длиться от нескольких дней до недель. Алкогольные напитки негативно влияют на функционирование внутренних органов, вызывая серьёзные нарушения, включая алкоголизм. При необходимости вывода из запоя важно обратиться за медицинской помощью, что можно осуществить на дому. В данной статье рассмотрим ключевые аспекты данной процедуры.
Подробнее тут – [url=https://vyvod-iz-zapoya-17.ru/]narkologicheskaya klinika[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Разобраться лучше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя на дому [/url]
Алкогольная зависимость представляет собой серьезную медицинскую проблему, которая влечет за собой не только физические, но и психологические проблемы. Периоды запоя, характеризующиеся бесконтрольным употреблением алкоголя, могут привести к тяжелым последствиям. Симптомы абстиненции требуют незамедлительного вмешательства. Анонимность в процессе реабилитации становится важным аспектом, помогающим пациентам избежать стыда и социального осуждения.
Получить больше информации – [url=https://vyvod-iz-zapoya-12.ru/]https://vyvod-iz-zapoya-12.ru[/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя на дому [/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Разобраться лучше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya na domu nedorogo kapelnica v-chelyabinske[/url]
Алкогольный запой представляет собой критическое состояние, требующее немедленного вмешательства квалифицированных специалистов. Клиника «Союз Здоровья» в Воронеже предлагает круглосуточную помощь, чтобы обеспечить своевременное и эффективное лечение. Наша цель — оперативное снятие интоксикации, устранение симптомов и предотвращение осложнений.
Выяснить больше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]нарколог на дом вывод из запоя [/url]
Стоимость вывода из запоя зависит от ряда факторов, таких как состояние пациента, выбранная программа лечения и место проведения процедуры. Мы предлагаем прозрачное ценообразование, чтобы вы могли заранее ознакомиться с возможными затратами на лечение.
Подробнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-v-stacionare-v-chelyabinske/]bystry vyvod iz zapoya v stacionare chelyabinsk[/url]
Запой представляет собой состояние, характеризующееся длительным непрерывным употреблением алкоголя, что приводит к физической и психической зависимости. Этот процесс может длиться от нескольких дней до недель. Алкогольные напитки негативно влияют на функционирование внутренних органов, вызывая серьёзные нарушения, включая алкоголизм. При необходимости вывода из запоя важно обратиться за медицинской помощью, что можно осуществить на дому. В данной статье рассмотрим ключевые аспекты данной процедуры.
Углубиться в тему – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-v-stacionare-v-chelyabinske/]vyvod iz zapoya v stacionare anonimno v-chelyabinske[/url]
Медикаментозное лечение — это еще один важный этап. Оно включает использование препаратов, которые помогают пациенту восстановиться как физически, так и психологически. Нарколог контролирует процесс лечения, чтобы исключить побочные эффекты и скорректировать терапию при необходимости.
Изучить вопрос глубже – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]частный нарколог на дом в красноярске[/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]narkologiya vyvod iz zapoya na domu chelyabinsk[/url]
В таких ситуациях вывод из запоя на дому становится оптимальным решением, особенно если пациент не может или не хочет посещать стационар. Эта услуга позволяет получить медицинскую помощь в комфортной и привычной обстановке, что снижает уровень стресса и ускоряет процесс восстановления.
Узнать больше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-kruglosutochno-v-voronezhe/]vyvod iz zapoya kruglosutochno v-voronezhe[/url]
При сильной интоксикации требуется полное очищение организма от токсинов. Детоксикация помогает нормализовать работу внутренних органов, улучшить общее состояние пациента и восстановить баланс жидкостей в организме. Специалист использует современные препараты, включая электролиты, глюкозу и антиоксиданты, для безопасного и эффективного очищения организма.
Разобраться лучше – [url=https://narcolog-na-dom-krasnoyarsk55.ru/]нарколог на дом недорого красноярск[/url]
скачать тачки быстрые как молния на андроид последняя версия [url=https://apk-smart.com/igry/gonki/1033-tachki-bystrye-kak-molnija-vzlomannaja-mod-mnogo-deneg.html]https://apk-smart.com/igry/gonki/1033-tachki-bystrye-kak-molnija-vzlomannaja-mod-mnogo-deneg.html[/url] скачать тачки быстрые как молния на андроид последняя версия
P.S Live ID: K89Io9blWX1UfZWv3ajv
P.S.S [url=https://okulovka.com/info/tserkov-troitsy-zhivonachalnoy-yazvischi]Программы и игры для Андроид телефона[/url] [url=https://www.livejournal.com/login.bml?returnto=http%3A%2F%2Fwww.livejournal.com%2Fupdate.bml&subject=%D0%9F%D1%80%D0%BE%D0%B3%D1%80%D0%B0%D0%BC%D0%BC%D1%8B%20%D0%B8%20%D0%B8%D0%B3%D1%80%D1%8B%20%D0%B4%D0%BB%D1%8F%20%D0%90%D0%BD%D0%B4%D1%80%D0%BE%D0%B8%D0%B4%20%D1%82%D0%B5%D0%BB%D0%B5%D1%84%D0%BE%D0%BD%D0%B0&event=%D1%81%D0%BA%D0%B0%D1%87%D0%B0%D1%82%D1%8C%20%D0%B2%D0%B7%D0%BB%D0%BE%D0%BC%D0%B0%D0%BD%D0%BD%D1%83%D1%8E%20%D0%B8%D0%B3%D1%80%D1%83%20%D1%82%D1%83%D0%B7%D0%B5%D0%BC%D1%86%D1%8B%20%D0%B8%20%D0%B7%D0%B0%D0%BC%D0%BA%D0%B8%20%3Ca%20href%3Dhttps%3A%2F%2Fapk-smart.com%2Figry%2Fstrategii%2F1363-vzlomannye-tuzemcy-i-zamki-mod-mnogo-deneg.html%3Ehttps%3A%2F%2Fapk-smart.com%2Figry%2Fstrategii%2F1363-vzlomannye-tuzemcy-i-zamki-mod-mnogo-deneg.html%3C%2Fa%3E%20%D1%81%D0%BA%D0%B0%D1%87%D0%B0%D1%82%D1%8C%20%D0%B2%D0%B7%D0%BB%D0%BE%D0%BC%D0%B0%D0%BD%D0%BD%D1%83%D1%8E%20%D0%B8%D0%B3%D1%80%D1%83%20%D1%82%D1%83%D0%B7%D0%B5%D0%BC%D1%86%D1%8B%20%D0%B8%20%D0%B7%D0%B0%D0%BC%D0%BA%D0%B8%20%0D%0A%20%0D%0AP.S%20Live%20ID%3A%20K89Io9blWX1UfZWv3ajv%20%0D%0AP.S.S%20%3Ca%20href%3Dhttps%3A%2F%2Fwww.livejournal.com%2Flogin.bml%3Freturnto%3Dhttps%253A%252F%252Fwww.livejournal.com%252Fupdate.bml%26subject%3D%25D0%259A%25D0%25B0%25D1%2587%25D0%25B5%25D1%2581%25D1%2582%25D0%25B2%25D0%25B5%25D0%25BD%25D0%25BD%25D0%25BE%25D0%25B5%2520%25D0%25BE%25D1%2587%25D0%25B8%25D1%2589%25D0%25B5%25D0%25BD%25D0%25B8%25D0%25B5%2520%25D0%25BA%25D0%25BE%25D0%25B6%25D0%25B8%2520%25D1%2581%2520%25D0%25B8%25D0%25BD%25D0%25BD%25D0%25BE%25D0%25B2%25D0%25B0%25D1%2586%25D0%25B8%25D0%25BE%25D0%25BD%25D0%25BD%25D0%25BE%25D0%25B9%2520%25D0%25BF%25D0%25B5%25D0%25BD%25D0%25BA%25D0%25BE%25D0%25B9%2520%25D0%25B4%25D0%25BB%25D1%258F%2520%25D1%2583%25D0%25BC%25D1%258B%25D0%25B2%25D0%25B0%25D0%25BD%25D0%25B8%25D1%258F%2520Genosys%2520Snow%2520O2%26event%3Dcriminal%2520case%2520%25D0%25B2%25D0%25B7%25D0%25BB%25D0%25BE%25D0%25BC%2520%253Ca%2520href%253Dhttps%253A%252F%252Fapk-smart.com%252Figry%252Flogicheskie%252F213-criminal-case-vzlom-mod-beskonechnaja-jenergija.html%253Ehttps%253A%252F%252Fapk-smart.com%252Figry%252Flogicheskie%252F213-criminal-case-vzlom-mod-beskonechnaja-jenergija.html%253C%252Fa%253E%2520criminal%2520case%2520%25D0%25B2%25D0%25B7%25D0%25BB%25D0%25BE%25D0%25BC%2520%250D%250A%2520%250D%250AP.S%2520Live%2520ID%253A%2520K89Io9blWX1UfZWv3ajv%2520%250D%250AP.S.S%2520%253Ca%2520href%253Dhttps%253A%252F%252Ftqfp.org%252Fforum%252Fviewtopic.php%253Ff%253D8%2526t%253D725%2526p%253D20914%2523p20914%253E%25D0%259F%25D1%2580%25D0%25BE%25D0%25B3%25D1%2580%25D0%25B0%25D0%25BC%25D0%25BC%25D1%258B%2520%25D0%25B8%2520%25D0%25B8%25D0%25B3%25D1%2580%25D1%258B%2520%25D0%25B4%25D0%25BB%25D1%258F%2520%25D0%2590%25D0%25BD%25D0%25B4%25D1%2580%25D0%25BE%25D0%25B8%25D0%25B4%2520%25D1%2582%25D0%25B5%25D0%25BB%25D0%25B5%25D1%2584%25D0%25BE%25D0%25BD%25D0%25B0%253C%252Fa%253E%2520%253Ca%2520href%253Dhttps%253A%252F%252Fokulovka.com%252Finfo%252Ftserkov-troitsy-zhivonachalnoy-yazvischi%253E%25D0%259F%25D1%2580%25D0%25BE%25D0%25B3%25D1%2580%25D0%25B0%25D0%25BC%25D0%25BC%25D1%258B%2520%25D0%25B8%2520%25D0%25B8%25D0%25B3%25D1%2580%25D1%258B%2520%25D0%25B4%25D0%25BB%25D1%258F%2520%25D0%2590%25D0%25BD%25D0%25B4%25D1%2580%25D0%25BE%25D0%25B8%25D0%25B4%2520%25D1%2582%25D0%25B5%25D0%25BB%25D0%25B5%25D1%2584%25D0%25BE%25D0%25BD%25D0%25B0%253C%252Fa%253E%2520%253Ca%2520href%253Dhttps%253A%252F%252Fwww.livejournal.com%252Flogin.bml%253Freturnto%253Dhttp%25253A%25252F%25252Fwww.livejournal.com%25252Fupdate.bml%2526event%253D%2525D1%252581%2525D0%2525BA%2525D0%2525B0%2525D1%252587%2525D0%2525B0%2525D1%252582%2525D1%25258C%252520driving%252520school%2525202017%252520%2525D0%2525BC%2525D0%2525BE%2525D0%2525B4%252520%2525D0%2525BC%2525D0%2525BD%2525D0%2525BE%2525D0%2525B3%2525D0%2525BE%252520%2525D0%2525B4%2525D0%2525B5%2525D0%2525BD%2525D0%2525B5%2525D0%2525B3%252520%25253Ca%252520href%25253Dhttps%25253A%25252F%25252Fapk-smart.com%25252Figry%25252Fgonki%25252F301-driving-school-2017-vzlomannyj-mnogo-deneg.html%25253Ehttps%25253A%25252F%25252Fapk-smart.com%25252Figry%25252Fgonki%25252F301-driving-school-2017-vzlomannyj-mnogo-deneg.html%25253C%25252Fa%25253E%252520%2525D1%252581%2525D0%2525BA%2525D0%2525B0%2525D1%252587%2525D0%2525B0%2525D1%252582%2525D1%25258C%252520driving%252520school%2525202017%252520%2525D0%2525BC%2525D0%2525BE%2525D0%2525B4%252520%2525D0%2525BC%2525D0%2525BD%2525D0%2525BE%2525D0%2525B3%2525D0%2525BE%252520%2525D0%2525B4%2525D0%2525B5%2525D0%2525BD%2525D0%2525B5%2525D0%2525B3%252520%25250D%25250A%252520%25250D%25250AP.S%252520Live%252520ID%25253A%252520K89Io9blWX1UfZWv3ajv%252520%25250D%25250AP.S.S%252520%25253Ca%252520href%25253Dhttps%25253A%25252F%25252Ftqfp.org%25252Fforum%25252Fviewtopic.php%25253Ff%25253D8%252526t%25253D725%252526p%25253D20914%252523p20914%25253E%2525D0%25259F%2525D1%252580%2525D0%2525BE%2525D0%2525B3%2525D1%252580%2525D0%2525B0%2525D0%2525BC%2525D0%2525BC%2525D1%25258B%252520%2525D0%2525B8%252520%2525D0%2525B8%2525D0%2525B3%2525D1%252580%2525D1%25258B%252520%2525D0%2525B4%2525D0%2525BB%2525D1%25258F%252520%2525D0%252590%2525D0%2525BD%2525D0%2525B4%2525D1%252580%2525D0%2525BE%2525D0%2525B8%2525D0%2525B4%252520%2525D1%252582%2525D0%2525B5%2525D0%2525BB%2525D0%2525B5%2525D1%252584%2525D0%2525BE%2525D0%2525BD%2525D0%2525B0%25253C%25252Fa%25253E%252520%25253Ca%252520href%25253Dhttps%25253A%25252F%25252Fokulovka.com%25252Finfo%25252Ftserkov-troitsy-zhivonachalnoy-yazvischi%25253E%2525D0%25259F%2525D1%252580%2525D0%2525BE%2525D0%2525B3%2525D1%252580%2525D0%2525B0%2525D0%2525BC%2525D0%2525BC%2525D1%25258B%252520%2525D0%2525B8%252520%2525D0%2525B8%2525D0%2525B3%2525D1%252580%2525D1%25258B%252520%2525D0%2525B4%2525D0%2525BB%2525D1%25258F%252520%2525D0%252590%2525D0%2525BD%2525D0%2525B4%2525D1%252580%2525D0%2525BE%2525D0%2525B8%2525D0%2525B4%252520%2525D1%252582%2525D0%2525B5%2525D0%2525BB%2525D0%2525B5%2525D1%252584%2525D0%2525BE%2525D0%2525BD%2525D0%2525B0%25253C%25252Fa%25253E%252520%2525207f3e065%252520%253E%25D0%259F%25D1%2580%25D0%25BE%25D0%25B3%25D1%2580%25D0%25B0%25D0%25BC%25D0%25BC%25D1%258B%2520%25D0%25B8%2520%25D0%25B8%25D0%25B3%25D1%2580%25D1%258B%2520%25D0%25B4%25D0%25BB%25D1%258F%2520%25D0%2590%25D0%25BD%25D0%25B4%25D1%2580%25D0%25BE%25D0%25B8%25D0%25B4%2520%25D1%2582%25D0%25B5%25D0%25BB%25D0%25B5%25D1%2584%25D0%25BE%25D0%25BD%25D0%25B0%253C%252Fa%253E%2520%2520389a901%2520%3E%D0%9F%D1%80%D0%BE%D0%B3%D1%80%D0%B0%D0%BC%D0%BC%D1%8B%20%D0%B8%20%D0%B8%D0%B3%D1%80%D1%8B%20%D0%B4%D0%BB%D1%8F%20%D0%90%D0%BD%D0%B4%D1%80%D0%BE%D0%B8%D0%B4%20%D1%82%D0%B5%D0%BB%D0%B5%D1%84%D0%BE%D0%BD%D0%B0%3C%2Fa%3E%20%3Ca%20href%3Dhttps%3A%2F%2Fludii.games%2Fforum%2Fshowthread.php%3Ftid%3D2134%3E%D0%9F%D1%80%D0%BE%D0%B3%D1%80%D0%B0%D0%BC%D0%BC%D1%8B%20%D0%B8%20%D0%B8%D0%B3%D1%80%D1%8B%20%D0%B4%D0%BB%D1%8F%20%D0%90%D0%BD%D0%B4%D1%80%D0%BE%D0%B8%D0%B4%20%D1%82%D0%B5%D0%BB%D0%B5%D1%84%D0%BE%D0%BD%D0%B0%3C%2Fa%3E%20%3Ca%20href%3Dhttps%3A%2F%2Ffunmill.ru%2Fbitrix%2Ftools%2Fcaptcha.php%3Fcaptcha_sid%3D046bdc6325d13cc744cdf587147dcab1%3E%D0%9F%D1%80%D0%BE%D0%B3%D1%80%D0%B0%D0%BC%D0%BC%D1%8B%20%D0%B8%20%D0%B8%D0%B3%D1%80%D1%8B%20%D0%B4%D0%BB%D1%8F%20%D0%90%D0%BD%D0%B4%D1%80%D0%BE%D0%B8%D0%B4%20%D1%82%D0%B5%D0%BB%D0%B5%D1%84%D0%BE%D0%BD%D0%B0%3C%2Fa%3E%20%204f57fec%20]Программы и игры для Андроид телефона[/url] [url=https://www.forumklassika.ru/showthread.php?t=123642&page=2&p=2002576&posted=1#post2002576]Программы и игры для Андроид телефона[/url] 9f3cf6d
Алкогольный запой представляет собой критическое состояние, требующее немедленного вмешательства квалифицированных специалистов. Клиника «Союз Здоровья» в Воронеже предлагает круглосуточную помощь, чтобы обеспечить своевременное и эффективное лечение. Наша цель — оперативное снятие интоксикации, устранение симптомов и предотвращение осложнений.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]vyvod iz zapoya na domu nedorogo kapelnica v-voronezhe[/url]
Стоимость вывода из запоя зависит от ряда факторов, таких как состояние пациента, выбранная программа лечения и место проведения процедуры. Мы предлагаем прозрачное ценообразование, чтобы вы могли заранее ознакомиться с возможными затратами на лечение.
Подробнее тут – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya kapelnica na domu chelyabinsk[/url]
Запой – это состояние, которое возникает вследствие длительного употребления алкоголя, приводящее к потере контроля над количеством выпиваемого и формированию как физической, так и психологической зависимости. Оно сопровождается серьёзными последствиями для здоровья и требует немедленного вмешательства.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-kruglosutochno-v-voronezhe/]vyvod iz zapoya kruglosutochno voronezh[/url]
Запой — это опасное состояние, которое развивается у людей с алкогольной зависимостью и характеризуется длительным непрерывным потреблением алкоголя. Это состояние приводит к серьезным нарушениям в организме, как физическим, так и психоэмоциональным. Длительный запой может вызвать множество осложнений, таких как поражение внутренних органов, нервной системы, а также проблемы в социальной и семейной жизни. Однако современная медицина предоставляет эффективные методы вывода из запоя, и одним из наиболее удобных и востребованных является вывод из запоя на дому.
Детальнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]vyvod iz zapoya kruglosutochno v-sankt-peterburge[/url]
Стоимость вывода из запоя зависит от ряда факторов, таких как состояние пациента, выбранная программа лечения и место проведения процедуры. Мы предлагаем прозрачное ценообразование, чтобы вы могли заранее ознакомиться с возможными затратами на лечение.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя на дому челябинск[/url]
При сильной интоксикации требуется полное очищение организма от токсинов. Детоксикация помогает нормализовать работу внутренних органов, улучшить общее состояние пациента и восстановить баланс жидкостей в организме. Специалист использует современные препараты, включая электролиты, глюкозу и антиоксиданты, для безопасного и эффективного очищения организма.
Подробнее – [url=https://narcolog-na-dom-krasnoyarsk55.ru/]http://narcolog-na-dom-krasnoyarsk55.ru/narkolog-na-dom-kruglosutochno-krasnoyarsk/[/url]
Гарантированная анонимность при выводе из запоя в Челябинске. В клинике «Перекресток Надежды» мы строго соблюдаем политику конфиденциальности. Информация о пациенте и его состоянии здоровья остаётся закрытой. Это особенно важно для тех, кто ценит свою репутацию и не хочет разглашения. Мы обеспечиваем анонимность как в стационаре, так и при оказании услуг на дому, сохраняя высокий уровень медицинской помощи.
Получить больше информации – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя на дому недорого [/url]
При сильной интоксикации требуется полное очищение организма от токсинов. Детоксикация помогает нормализовать работу внутренних органов, улучшить общее состояние пациента и восстановить баланс жидкостей в организме. Специалист использует современные препараты, включая электролиты, глюкозу и антиоксиданты, для безопасного и эффективного очищения организма.
Подробнее – [url=https://narcolog-na-dom-krasnoyarsk55.ru/]http://narcolog-na-dom-krasnoyarsk55.ru/narkolog-na-dom-krasnoyarsk-tseny/[/url]
Для купирования симптомов и профилактики рецидивов врач подбирает индивидуальные препараты, которые могут включать седативные средства, витамины и противосудорожные препараты. Лечение проводится под строгим контролем специалиста, который следит за реакцией пациента и при необходимости корректирует терапию.
Выяснить больше – [url=https://narcolog-na-dom-krasnoyarsk55.ru/]частный нарколог на дом красноярск[/url]
Алкогольная зависимость представляет собой актуальную медицинскую проблему, требующую тщательного подхода. Запой характеризуется длительным, непрерывным употреблением спиртных напитков, что ведёт к физической и психической зависимости. На фоне запойного состояния могут возникнуть тяжёлые нарушения функций органов, увеличивается риск развития таких состояний, как алкогольный делирий. Вывод из запоя становится ключевым этапом в лечении зависимостей, который может осуществляться как в стационарных условиях, так и на дому, в зависимости от состояния пациента. В клинике «Перекресток Надежды» в Челябинске мы предлагаем круглосуточную помощь, чтобы обеспечить максимально эффективное и безопасное лечение при любом варианте вывода из запоя.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-17.ru/]narkologicheskaya klinika[/url]
Проблема зависимостей остаётся актуальной в современном обществе. С каждым годом увеличивается число людей, страдающих от алкоголизма, наркомании и других форм зависимостей, что негативно отражается на их жизни и благополучии близких. Зависимость — это не просто физическое заболевание, но и глубокая психологическая проблема. Для эффективного лечения требуется помощь профессионалов, способных обеспечить комплексный подход.
Выяснить больше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-kruglosutochno-v-voronezhe/]vyvod iz zapoya kruglosutochno narkologiya [/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьёзное воздействие этанола. Это приводит к нарушениям нормальной физиологической деятельности и ухудшению состояния здоровья. Анонимный вывод из запоя — это медицинская помощь, направленная на устранение последствий чрезмерного употребления алкоголя с соблюдением строгой конфиденциальности.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-20.ru/]вывод из запоя с выездом в екатеринбурге[/url]
Запой — это тяжелое состояние, возникающее вследствие длительного и непрерывного употребления алкоголя, которое сопровождается физической и психической зависимостью. Это опасное явление требует незамедлительного вмешательства специалистов. Вывод из запоя — это комплексный процесс медицинской детоксикации, который включает в себя очищение организма от токсинов, нормализацию функций внутренних органов и поддержку психоэмоционального состояния пациента. В случае тяжелого запоя стационарное лечение становится оптимальным решением для безопасного и эффективного вывода из этого состояния. В этой статье мы рассмотрим, как проходит вывод из запоя в стационаре клиники «Перекресток Надежды» в Челябинске, а также особенности анонимности, скорости лечения и участия нарколога.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-v-kruglosutochno-v-chelyabinske/]вывод из запоя срочно круглосуточно в челябинске[/url]
Проблема зависимостей остаётся актуальной в современном обществе. С каждым годом увеличивается число людей, страдающих от алкоголизма, наркомании и других форм зависимостей, что негативно отражается на их жизни и благополучии близких. Зависимость — это не просто физическое заболевание, но и глубокая психологическая проблема. Для эффективного лечения требуется помощь профессионалов, способных обеспечить комплексный подход.
Получить больше информации – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]наркология вывод из запоя на дому в воронеже[/url]
Наркологическая клиника «Союз Здоровья» в Воронеже специализируется на лечении алкогольной зависимости и предлагает профессиональные услуги по выводу из запоя. Мы стремимся создать атмосферу доверия, где каждый пациент сможет получить необходимую поддержку.
Разобраться лучше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]vyvod iz zapoya na domu [/url]
Ночные вызовы и визиты в праздничные дни также могут стоить дороже, что связано с дополнительными усилиями со стороны клиники.
Подробнее тут – [url=https://narcolog-na-dom-krasnoyarsk55.ru/]нарколог на дом стоимость красноярск[/url]
Запой — это тяжелое состояние, при котором человек теряет контроль над количеством употребляемого алкоголя, что приводит к серьезным нарушениям здоровья. Процесс вывода из запоя требует профессионального подхода, медицинского контроля и тщательного восстановления организма. Наркологическая клиника «Шаг к Трезвости» в Краснодаре предлагает квалифицированную помощь для тех, кто нуждается в срочном и безопасном выходе из запоя. Мы предоставляем комплексное лечение, ориентированное на каждый этап восстановления, с максимальной заботой о здоровье пациента.
Получить больше информации – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]вывод из запоя анонимно недорого [/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Разобраться лучше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-cena-v-chelyabinske/]вывод из запоя цена [/url]
Одной из самых востребованных процедур является снятие абстинентного синдрома. Это состояние сопровождается сильными физическими и психологическими симптомами, такими как тревожность, тремор, головная боль и бессонница. Врач, прибывший на вызов, проводит диагностику состояния пациента и подбирает необходимые препараты. Вводятся инфузионные растворы с витаминами, седативными и противорвотными средствами.
Получить дополнительную информацию – [url=https://narcolog-na-dom-krasnoyarsk55.ru/]нарколог в красноярске[/url]
Алкогольная зависимость представляет собой актуальную медицинскую проблему, требующую тщательного подхода. Запой характеризуется длительным, непрерывным употреблением спиртных напитков, что ведёт к физической и психической зависимости. На фоне запойного состояния могут возникнуть тяжёлые нарушения функций органов, увеличивается риск развития таких состояний, как алкогольный делирий. Вывод из запоя становится ключевым этапом в лечении зависимостей, который может осуществляться как в стационарных условиях, так и на дому, в зависимости от состояния пациента. В клинике «Перекресток Надежды» в Челябинске мы предлагаем круглосуточную помощь, чтобы обеспечить максимально эффективное и безопасное лечение при любом варианте вывода из запоя.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя на дому круглосуточно в челябинске[/url]
Алкогольная и наркотическая зависимости — серьезные проблемы, требующие профессионального вмешательства. В Красноярске одной из самых востребованных услуг является вызов нарколога на дом. Этот формат позволяет пациентам получить квалифицированную помощь в комфортной обстановке, избегая стрессов, связанных с посещением клиники.
Подробнее – [url=https://narcolog-na-dom-krasnoyarsk55.ru/]https://narcolog-na-dom-krasnoyarsk55.ru[/url]
Запой представляет собой состояние, характеризующееся длительным непрерывным употреблением алкоголя, что приводит к физической и психической зависимости. Этот процесс может длиться от нескольких дней до недель. Алкогольные напитки негативно влияют на функционирование внутренних органов, вызывая серьёзные нарушения, включая алкоголизм. При необходимости вывода из запоя важно обратиться за медицинской помощью, что можно осуществить на дому. В данной статье рассмотрим ключевые аспекты данной процедуры.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя на дому круглосуточно [/url]
В таких ситуациях вывод из запоя на дому становится оптимальным решением, особенно если пациент не может или не хочет посещать стационар. Эта услуга позволяет получить медицинскую помощь в комфортной и привычной обстановке, что снижает уровень стресса и ускоряет процесс восстановления.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-anonimno-v-voronezhe/]наркология вывод из запоя анонимно воронеж[/url]
Круглосуточная доступность услуги делает ее универсальным решением для тех, кто столкнулся с проблемой внезапно, будь то день или ночь. Рассмотрим, какие услуги предлагает нарколог на дому, как организовать вызов и что влияет на стоимость лечения.
Разобраться лучше – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]https://narcolog-na-dom-v-krasnoyarske55.ru/[/url]
Алкогольная зависимость — это серьезная проблема, требующая немедленного вмешательства. Если вы или ваши близкие столкнулись с запоем, важно получить профессиональную помощь как можно скорее, чтобы избежать ухудшения здоровья. Наркологическая клиника «Шаг к Трезвости» в Краснодаре предлагает услугу вывода из запоя на дому, что является удобным и эффективным решением для тех, кто не может или не хочет посещать стационар.
Получить больше информации – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]vyvod iz zapoya vyzov na dom [/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]srochny vyvod iz zapoya na domu v-voronezhe[/url]
Зависимость — это серьёзная проблема, которая затрагивает не только человека, но и его семью и окружение. Алкоголизм, наркомания и другие виды зависимостей могут разрушить жизнь, но с профессиональной помощью можно вернуть её в русло нормального функционирования. Наркологическая клиника «Заря Будущего» в Санкт-Петербурге предлагает уникальные программы лечения зависимостей, которые сочетает медико-психологический подход, индивидуальные методики и квалифицированную поддержку.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu nedorogo kapelnica v-sankt-peterburge[/url]
Гарантированная анонимность при выводе из запоя в Челябинске. В клинике «Перекресток Надежды» мы строго соблюдаем политику конфиденциальности. Информация о пациенте и его состоянии здоровья остаётся закрытой. Это особенно важно для тех, кто ценит свою репутацию и не хочет разглашения. Мы обеспечиваем анонимность как в стационаре, так и при оказании услуг на дому, сохраняя высокий уровень медицинской помощи.
Подробнее тут – [url=https://vyvod-iz-zapoya-17.ru/]наркологическая клиника челябинск[/url]
Для купирования симптомов и профилактики рецидивов врач подбирает индивидуальные препараты, которые могут включать седативные средства, витамины и противосудорожные препараты. Лечение проводится под строгим контролем специалиста, который следит за реакцией пациента и при необходимости корректирует терапию.
Исследовать вопрос подробнее – [url=https://narcolog-na-dom-krasnoyarsk55.ru/]вызов нарколога на дом цена в красноярске[/url]
Алкогольный запой представляет собой критическое состояние, требующее немедленного вмешательства квалифицированных специалистов. Клиника «Союз Здоровья» в Воронеже предлагает круглосуточную помощь, чтобы обеспечить своевременное и эффективное лечение. Наша цель — оперативное снятие интоксикации, устранение симптомов и предотвращение осложнений.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-stacionare-v-voronezhe/]vyvod iz zapoya v stacionare anonimno v-voronezhe[/url]
Запой — это тяжелое состояние, возникающее вследствие длительного и непрерывного употребления алкоголя, которое сопровождается физической и психической зависимостью. Это опасное явление требует незамедлительного вмешательства специалистов. Вывод из запоя — это комплексный процесс медицинской детоксикации, который включает в себя очищение организма от токсинов, нормализацию функций внутренних органов и поддержку психоэмоционального состояния пациента. В случае тяжелого запоя стационарное лечение становится оптимальным решением для безопасного и эффективного вывода из этого состояния. В этой статье мы рассмотрим, как проходит вывод из запоя в стационаре клиники «Перекресток Надежды» в Челябинске, а также особенности анонимности, скорости лечения и участия нарколога.
Подробнее тут – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]срочный вывод из запоя на дому [/url]
Для купирования симптомов и профилактики рецидивов врач подбирает индивидуальные препараты, которые могут включать седативные средства, витамины и противосудорожные препараты. Лечение проводится под строгим контролем специалиста, который следит за реакцией пациента и при необходимости корректирует терапию.
Подробнее – [url=https://narcolog-na-dom-krasnoyarsk55.ru/]https://narcolog-na-dom-krasnoyarsk55.ru/[/url]
Запой — это тяжелое состояние, при котором человек теряет контроль над количеством употребляемого алкоголя, что приводит к серьезным нарушениям здоровья. Процесс вывода из запоя требует профессионального подхода, медицинского контроля и тщательного восстановления организма. Наркологическая клиника «Шаг к Трезвости» в Краснодаре предлагает квалифицированную помощь для тех, кто нуждается в срочном и безопасном выходе из запоя. Мы предоставляем комплексное лечение, ориентированное на каждый этап восстановления, с максимальной заботой о здоровье пациента.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]наркология вывод из запоя анонимно [/url]
Voor degenen die op zoek zijn naar een casino dat perfect aansluit op de behoeften van Nederlandse spelers, is betsixty netherlands een uitstekende keuze. Dit platform biedt niet alleen een groot spelaanbod, maar ook een gestroomlijnd en eenvoudig registratieproces, zodat je binnen enkele minuten aan de slag kunt. Of je nu een fan bent van klassieke gokkasten of liever je geluk beproeft met live dealers, hier is alles aanwezig om je een geweldige tijd te bezorgen. De bonussen zijn ruim en de promoties wisselen regelmatig, waardoor er altijd een nieuwe kans is om extra beloningen te claimen. Veiligheid en betrouwbaarheid staan hoog in het vaandel, zodat je zonder zorgen kunt genieten van een onbezorgde speelervaring. Wil je de spanning van een echt casino ervaren zonder je huis te verlaten? Registreer je dan vandaag nog en profiteer van alles wat deze aanbieder te bieden heeft.
При сильной интоксикации требуется полное очищение организма от токсинов. Детоксикация помогает нормализовать работу внутренних органов, улучшить общее состояние пациента и восстановить баланс жидкостей в организме. Специалист использует современные препараты, включая электролиты, глюкозу и антиоксиданты, для безопасного и эффективного очищения организма.
Получить больше информации – [url=https://narcolog-na-dom-krasnoyarsk55.ru/]нарколог на дом цены в красноярске[/url]
Алкогольная зависимость представляет собой актуальную медицинскую проблему, требующую тщательного подхода. Запой характеризуется длительным, непрерывным употреблением спиртных напитков, что ведёт к физической и психической зависимости. На фоне запойного состояния могут возникнуть тяжёлые нарушения функций органов, увеличивается риск развития таких состояний, как алкогольный делирий. Вывод из запоя становится ключевым этапом в лечении зависимостей, который может осуществляться как в стационарных условиях, так и на дому, в зависимости от состояния пациента. В клинике «Перекресток Надежды» в Челябинске мы предлагаем круглосуточную помощь, чтобы обеспечить максимально эффективное и безопасное лечение при любом варианте вывода из запоя.
Углубиться в тему – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя с выездом на дом [/url]
Алкогольная зависимость представляет собой серьёзную медицинскую проблему, требующую специализированного подхода. Запойное состояние характеризуется длительным и бесконтрольным потреблением спиртных напитков, что приводит к возникновению как физической, так и психической зависимости. Данное явление может вызвать нарушения в работе внутренних органов, значительно увеличивая вероятность развития алкогольного делирия, который представляет собой острое состояние, требующее неотложной медицинской помощи. Вывод из запоя это ключевая часть лечения зависимости, которая может осуществляться как в стационаре, так и в амбулаторных условиях, в зависимости от конкретной ситуации и состояния пациента.
Выяснить больше – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-cena-v-krasnodare/]narkolog vyvod iz zapoya cena v-krasnodare[/url]
История марципана и его связь с Кёнигсбергом https://e-pochemuchka.ru/istoriya-marczipana-ot-vostoka-do-kyonigsberga/
Медикаментозное лечение — это еще один важный этап. Оно включает использование препаратов, которые помогают пациенту восстановиться как физически, так и психологически. Нарколог контролирует процесс лечения, чтобы исключить побочные эффекты и скорректировать терапию при необходимости.
Ознакомиться с деталями – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]частный нарколог на дом в красноярске[/url]
Стационарное лечение обеспечивает постоянный контроль медицинского персонала, что гарантирует максимальную безопасность и мониторинг состояния пациента. Это критически важно для пациентов с сопутствующими заболеваниями или осложнениями алкоголизма. Клиники также предоставляют дополнительные услуги: консультации психолога, групповые и индивидуальные терапевтические занятия, способствующие скорейшему выздоровлению и профилактике рецидивов.
Ознакомиться с деталями – [url=https://kapelnica-ot-zapoya-msk55.ru/]http://kapelnica-ot-zapoya-msk55.ru[/url]
Проблема зависимостей остаётся актуальной в современном обществе. С каждым годом увеличивается число людей, страдающих от алкоголизма, наркомании и других форм зависимостей, что негативно отражается на их жизни и благополучии близких. Зависимость — это не просто физическое заболевание, но и глубокая психологическая проблема. Для эффективного лечения требуется помощь профессионалов, способных обеспечить комплексный подход.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]vyvod iz zapoya na domu nedorogo kapelnica voronezh[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Подробнее тут – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-v-stacionare-v-chelyabinske/]быстрый вывод из запоя в стационаре [/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]вывод из запоя цена наркология санкт-петербург[/url]
Алкогольная зависимость представляет собой актуальную медицинскую проблему, требующую тщательного подхода. Запой характеризуется длительным, непрерывным употреблением спиртных напитков, что ведёт к физической и психической зависимости. На фоне запойного состояния могут возникнуть тяжёлые нарушения функций органов, увеличивается риск развития таких состояний, как алкогольный делирий. Вывод из запоя становится ключевым этапом в лечении зависимостей, который может осуществляться как в стационарных условиях, так и на дому, в зависимости от состояния пациента. В клинике «Перекресток Надежды» в Челябинске мы предлагаем круглосуточную помощь, чтобы обеспечить максимально эффективное и безопасное лечение при любом варианте вывода из запоя.
Детальнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya na domu kruglosutochno chelyabinsk[/url]
Вызов нарколога на дом в Красноярске предоставляет пациентам доступ к целому комплексу лечебных мероприятий, направленных на стабилизацию состояния, устранение симптомов зависимости и профилактику осложнений.
Получить дополнительные сведения – [url=https://narcolog-na-dom-krasnoyarsk55.ru/]нарколог на дом красноярск[/url]
You’ve the most impressive websites.
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]нарколог вывод из запоя цена [/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя на дому недорого капельница челябинск[/url]
Стационарное лечение обеспечивает постоянный контроль медицинского персонала, что гарантирует максимальную безопасность и мониторинг состояния пациента. Это критически важно для пациентов с сопутствующими заболеваниями или осложнениями алкоголизма. Клиники также предоставляют дополнительные услуги: консультации психолога, групповые и индивидуальные терапевтические занятия, способствующие скорейшему выздоровлению и профилактике рецидивов.
Ознакомиться с деталями – [url=https://kapelnica-ot-zapoya-msk55.ru/]капельницы от запоямосква[/url]
Запой — это тяжелое состояние, возникающее вследствие длительного и непрерывного употребления алкоголя, которое сопровождается физической и психической зависимостью. Это опасное явление требует незамедлительного вмешательства специалистов. Вывод из запоя — это комплексный процесс медицинской детоксикации, который включает в себя очищение организма от токсинов, нормализацию функций внутренних органов и поддержку психоэмоционального состояния пациента. В случае тяжелого запоя стационарное лечение становится оптимальным решением для безопасного и эффективного вывода из этого состояния. В этой статье мы рассмотрим, как проходит вывод из запоя в стационаре клиники «Перекресток Надежды» в Челябинске, а также особенности анонимности, скорости лечения и участия нарколога.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-v-kruglosutochno-v-chelyabinske/]вывод из запоя срочно круглосуточно [/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]анонимный вывод из запоя на дому [/url]
Современная жизнь, особенно в крупном городе, как Красноярск, часто становится причиной различных зависимостей. Работа в условиях постоянного стресса, высокий ритм жизни и эмоциональные перегрузки могут привести к проблемам, которые требуют неотложной медицинской помощи. Одним из наиболее удобных решений в такой ситуации является вызов нарколога на дом.
Узнать больше – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]платный нарколог на дом в красноярске[/url]
Алкогольная зависимость представляет собой серьезную медицинскую проблему, которая влечет за собой не только физические, но и психологические проблемы. Периоды запоя, характеризующиеся бесконтрольным употреблением алкоголя, могут привести к тяжелым последствиям. Симптомы абстиненции требуют незамедлительного вмешательства. Анонимность в процессе реабилитации становится важным аспектом, помогающим пациентам избежать стыда и социального осуждения.
Получить больше информации – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]вывод из запоя срочно круглосуточно краснодар[/url]
Услуга вызова нарколога на дом в Красноярске — это удобный и современный способ получения медицинской помощи. Она идеально подходит для тех, кто нуждается в профессиональной поддержке, но по каким-либо причинам не может или не хочет посещать клинику.
Изучить вопрос глубже – [url=https://narcolog-na-dom-krasnoyarsk55.ru/]вызов нарколога цена в красноярске[/url]
Алкогольная зависимость представляет собой актуальную медицинскую проблему, требующую тщательного подхода. Запой характеризуется длительным, непрерывным употреблением спиртных напитков, что ведёт к физической и психической зависимости. На фоне запойного состояния могут возникнуть тяжёлые нарушения функций органов, увеличивается риск развития таких состояний, как алкогольный делирий. Вывод из запоя становится ключевым этапом в лечении зависимостей, который может осуществляться как в стационарных условиях, так и на дому, в зависимости от состояния пациента. В клинике «Перекресток Надежды» в Челябинске мы предлагаем круглосуточную помощь, чтобы обеспечить максимально эффективное и безопасное лечение при любом варианте вывода из запоя.
Выяснить больше – [url=https://vyvod-iz-zapoya-17.ru/]vyvod iz zapoya v-chelyabinske[/url]
В клинике «Заря Будущего» мы понимаем, насколько важен своевременный и профессиональный подход к лечению зависимости. Наши специалисты готовы оказать квалифицированную помощь прямо у вас дома, обеспечивая полную безопасность и конфиденциальность на каждом этапе лечения.
Узнать больше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]вывод из запоя в стационаре анонимно санкт-петербург[/url]
Современная жизнь, особенно в крупном городе, как Красноярск, часто становится причиной различных зависимостей. Работа в условиях постоянного стресса, высокий ритм жизни и эмоциональные перегрузки могут привести к проблемам, которые требуют неотложной медицинской помощи. Одним из наиболее удобных решений в такой ситуации является вызов нарколога на дом.
Ознакомиться с деталями – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]http://narcolog-na-dom-v-krasnoyarske55.ru/narkolog-na-dom-krasnoyarsk-tseny/[/url]
Гарантированная анонимность при выводе из запоя в Челябинске. В клинике «Перекресток Надежды» мы строго соблюдаем политику конфиденциальности. Информация о пациенте и его состоянии здоровья остаётся закрытой. Это особенно важно для тех, кто ценит свою репутацию и не хочет разглашения. Мы обеспечиваем анонимность как в стационаре, так и при оказании услуг на дому, сохраняя высокий уровень медицинской помощи.
Детальнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-v-stacionare-v-chelyabinske/]вывод из запоя в стационаре [/url]
Запой представляет собой сложное состояние, связанное с длительным употреблением алкоголя, что приводит к формированию зависимости. Это явление не только нарушает нормальную жизнедеятельность, но также вызывает серьезные физические и психологические расстройства. Стационарное лечение становится необходимым для восстановления здоровья и профилактики осложнений. В стационаре клиники «Шаг к Трезвости» в Краснодаре мы предлагаем комплексный подход, включающий медикаментозное лечение, психотерапию и постоянный контроль со стороны квалифицированных специалистов.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]vyvod iz zapoya anonimno nedorogo krasnodar[/url]
Запой представляет собой состояние, характеризующееся длительным непрерывным употреблением алкоголя, что приводит к физической и психической зависимости. Этот процесс может длиться от нескольких дней до недель. Алкогольные напитки негативно влияют на функционирование внутренних органов, вызывая серьёзные нарушения, включая алкоголизм. При необходимости вывода из запоя важно обратиться за медицинской помощью, что можно осуществить на дому. В данной статье рассмотрим ключевые аспекты данной процедуры.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]vyvod iz zapoya na domu nedorogo kapelnica [/url]
Гарантированная анонимность при выводе из запоя в Челябинске. В клинике «Перекресток Надежды» мы строго соблюдаем политику конфиденциальности. Информация о пациенте и его состоянии здоровья остаётся закрытой. Это особенно важно для тех, кто ценит свою репутацию и не хочет разглашения. Мы обеспечиваем анонимность как в стационаре, так и при оказании услуг на дому, сохраняя высокий уровень медицинской помощи.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя вызов на дом в челябинске[/url]
Важно отметить, что некоторые клиники предоставляют скидки для новых клиентов или на отдельные процедуры. Однако стоит избегать слишком низких цен, так как это может свидетельствовать о недостаточном уровне профессионализма или отсутствии лицензии у специалиста. Например, вызов нарколога на дом может варьироваться по стоимости, и важно выбрать профессионала с хорошей репутацией.
Подробнее тут – [url=https://narcolog-na-dom-ektb55.ru/]выезд нарколога на дом цена в екатеринбурге[/url]
Когда речь идет о лечении зависимостей, многие пациенты испытывают стресс от визита в медицинские учреждения, особенно если это касается такой деликатной темы, как алкоголизм. Лечение на дому помогает избежать всех этих трудностей и обеспечивает комфорт для пациента. Привычная домашняя обстановка снижает тревожность, что позитивно влияет на процесс выздоровления. Врач нарколог на дом платный делает необходимую процедуру доступнее и удобнее для многих.
Подробнее можно узнать тут – [url=https://narcolog-na-dom-ektb55.ru/]врач нарколог на дом платный екатеринбург[/url]
С увеличением темпа жизни и нарастающим стрессом, многие люди в Екатеринбурге сталкиваются с зависимостями, особенно с алкогольной. Алкогольная зависимость является одной из наиболее распространённых проблем, требующих немедленного вмешательства специалистов. Важно помнить, что в сложных ситуациях, связанных с зависимостью, помощь не должна откладываться. Вызов нарколога на дом в Екатеринбурге становится идеальным вариантом для тех, кто не может или не хочет покидать свой дом, но нуждается в медицинской помощи. Узнать, сколько стоит выезд нарколога на дом цена в разных клиниках, можно, связавшись с ними напрямую.
Разобраться лучше – [url=https://narcolog-na-dom-ektb55.ru/]https://narcolog-na-dom-ektb55.ru/narkolog-na-dom-kruglosutochno-ekaterinburg[/url]
Важно отметить, что некоторые клиники предоставляют скидки для новых клиентов или на отдельные процедуры. Однако стоит избегать слишком низких цен, так как это может свидетельствовать о недостаточном уровне профессионализма или отсутствии лицензии у специалиста. Например, вызов нарколога на дом может варьироваться по стоимости, и важно выбрать профессионала с хорошей репутацией.
Ознакомиться с деталями – [url=https://narcolog-na-dom-ektb55.ru/]https://narcolog-na-dom-ektb55.ru[/url]
Стоимость услуг в Екатеринбурге может различаться в зависимости от конкретного случая. Консультация нарколога обычно стоит от 3 000 до 5 000 рублей, тогда как услуга вывода из запоя обойдётся в 5 000–12 000 рублей. Более комплексные программы, такие как детоксикация организма или курсы лечения, могут стоить от 10 000 до 20 000 рублей.
Подробнее можно узнать тут – [url=https://narcolog-na-dom-ektb55.ru/]нарколог на дом в екатеринбурге[/url]
Когда речь идет о лечении зависимостей, многие пациенты испытывают стресс от визита в медицинские учреждения, особенно если это касается такой деликатной темы, как алкоголизм. Лечение на дому помогает избежать всех этих трудностей и обеспечивает комфорт для пациента. Привычная домашняя обстановка снижает тревожность, что позитивно влияет на процесс выздоровления.
Получить дополнительные сведения – [url=https://narcolog-na-dom-ektb55.ru/]нарколог на дом стоимость в екатеринбурге[/url]
Процедура капельницы помогает не только устранить симптомы похмелья, но и способствует восстановлению нормальной работы организма. Это достигается благодаря введению лекарственных растворов, которые очищают кровь от токсинов, восстанавливают водно-солевой баланс и нормализуют работу жизненно важных органов.
Исследовать вопрос подробнее – [url=https://kapelnica-ot-zapoya-ekb55.ru/]https://kapelnica-ot-zapoya-ekb55.ru/[/url]
Клиника «Освобождение» в Рязани предоставляет услугу круглосуточного вывода из запоя на дому, что позволяет пациенту получить медицинскую помощь в привычной обстановке, избегая лишнего стресса, связанного с госпитализацией.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-cena-v-ryazani/]vyvod iz zapoya cena ryazan[/url]
В таких ситуациях вывод из запоя на дому становится оптимальным решением, особенно если пациент не может или не хочет посещать стационар. Эта услуга позволяет получить медицинскую помощь в комфортной и привычной обстановке, что снижает уровень стресса и ускоряет процесс восстановления.
Узнать больше – [url=https://vyvod-iz-zapoya-11.ru/]вывод из запоя с выездом[/url]
Запой — это состояние, характеризующееся утратой контроля над употреблением алкоголя, что приводит к тяжелой интоксикации организма. Вывод из запоя — это комплексный процесс, направленный на устранение последствий интоксикации и восстановление нормального функционирования организма. Цена данной процедуры может значительно различаться в зависимости от нескольких факторов, и в этой статье мы подробно рассмотрим, что влияет на стоимость вывода из запоя в Краснодаре, в том числе в клинике «Шаг к Трезвости».
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-cena-v-krasnodare/]vyvod iz zapoya cena narkologiya [/url]
Алкогольная зависимость — это серьезная проблема, требующая незамедлительного вмешательства, чтобы избежать тяжелых последствий для здоровья. Запойное пьянство может привести к различным заболеваниям, таким как цирроз печени, панкреатит и другие опасные состояния. К счастью, современная медицина предлагает эффективные методы решения этой проблемы. Для тех, кто не хочет или не может посетить стационар, есть возможность пройти процедуру капельницы от запоя на дому в Твери.
Детальнее – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-v-kruglosutochno-v-tveri/]вывод из запоя круглосуточно наркология в твери[/url]
Такой метод лечения помогает быстро устранить последствия алкоголизма и минимизировать риски для здоровья пациента.
Исследовать вопрос подробнее – [url=https://kapelnica-ot-zapoya-ektb55.ru/]капельница от запоя екатеринбург[/url]
Современное общество сталкивается с серьёзными вызовами, связанными с зависимостями. Алкоголизм, как одна из самых распространённых проблем, оказывает разрушительное воздействие на здоровье человека, разрушая семейные отношения и затрудняя социальную адаптацию. В такие моменты крайне важно обратиться за квалифицированной помощью.
Разобраться лучше – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]vyvod iz zapoya na domu nedorogo kapelnica [/url]
Запой – это состояние, которое возникает вследствие длительного употребления алкоголя, приводящее к потере контроля над количеством выпиваемого и формированию как физической, так и психологической зависимости. Оно сопровождается серьёзными последствиями для здоровья и требует немедленного вмешательства.
Подробнее – [url=https://vyvod-iz-zapoya-11.ru/]вывод из запоя воронеж[/url]
Запой – это состояние, возникающее из-за длительного употребления алкоголя и характеризующееся физической и психологической зависимостью. Оно требует комплексного медицинского вмешательства для устранения последствий и предотвращения дальнейшего ухудшения здоровья. Стационарное лечение в клинике «Освобождение» в Рязани – это эффективный способ вернуть пациента к нормальной жизни, предоставляя ему комфортные условия и круглосуточную помощь специалистов.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-13.ru/]https://vyvod-iz-zapoya-13.ru[/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Узнать больше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]vyvod iz zapoya vyzov na dom [/url]
Алкоголизм — это хроническое заболевание, которое характеризуется патологической зависимостью от этанола. Периоды запоя, когда человек неконтролируемо потребляет алкоголь, могут привести к разнообразным медицинским осложнениям, как физическим, так и психологическим. Запой становится не только причиной ухудшения здоровья, но и причиной множества социальных и личных проблем. На этом фоне возникает необходимость вывода из запоя, процесс, который должен учитывать индивидуальные особенности пациента. Важно, что анонимность в реабилитации играет ключевую роль, помогая избежать стигматизации и увеличивая шансы на успешное восстановление.
Подробнее – [url=https://vyvod-iz-zapoya-13.ru/]https://www.vyvod-iz-zapoya-13.ru[/url]
Клиника «Освобождение» в Рязани предоставляет услугу круглосуточного вывода из запоя на дому, что позволяет пациенту получить медицинскую помощь в привычной обстановке, избегая лишнего стресса, связанного с госпитализацией.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]нарколог на дом вывод из запоя [/url]
Алкогольный запой представляет собой критическое состояние, требующее немедленного вмешательства квалифицированных специалистов. Клиника «Союз Здоровья» в Воронеже предлагает круглосуточную помощь, чтобы обеспечить своевременное и эффективное лечение. Наша цель — оперативное снятие интоксикации, устранение симптомов и предотвращение осложнений.
Детальнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-stacionare-v-voronezhe/]vyvod iz zapoya v stacionare [/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьёзное воздействие этанола. Это приводит к нарушениям нормальной физиологической деятельности и ухудшению состояния здоровья. Анонимный вывод из запоя — это медицинская помощь, направленная на устранение последствий чрезмерного употребления алкоголя с соблюдением строгой конфиденциальности.
Разобраться лучше – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-v-stacionare-v-ekaterinburge/]vyvod iz zapoya v stacionare anonimno ekaterinburg[/url]
Алкогольный запой представляет собой критическое состояние, требующее немедленного вмешательства квалифицированных специалистов. Клиника «Союз Здоровья» в Воронеже предлагает круглосуточную помощь, чтобы обеспечить своевременное и эффективное лечение. Наша цель — оперативное снятие интоксикации, устранение симптомов и предотвращение осложнений.
Подробнее тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]вывод из запоя врач на дом [/url]
Клиника «Освобождение» в Рязани предоставляет услугу круглосуточного вывода из запоя на дому, что позволяет пациенту получить медицинскую помощь в привычной обстановке, избегая лишнего стресса, связанного с госпитализацией.
Разобраться лучше – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-anonimno-v-ryazani/]anonimny vyvod iz zapoya na domu [/url]
Процесс вывода из запоя требует быстрого реагирования. Запой, особенно в его тяжелых формах, представляет угрозу не только для психического состояния пациента, но и для его физического здоровья. Чтобы минимизировать риски и предотвратить осложнения, важно начинать лечение как можно скорее. Клинике «Освобождение» в Рязани доступна круглосуточная помощь — мы работаем 24/7, чтобы оперативно вмешаться в любой момент, когда это необходимо. Врачебная помощь может быть предоставлена как в стационаре клиники, так и на дому, в зависимости от состояния пациента и его предпочтений.
Получить больше информации – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]вывод из запоя вызов на дом [/url]
Запой – это состояние, которое возникает вследствие длительного употребления алкоголя, приводящее к потере контроля над количеством выпиваемого и формированию как физической, так и психологической зависимости. Оно сопровождается серьёзными последствиями для здоровья и требует немедленного вмешательства.
Получить больше информации – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]vyvod iz zapoya vyzov na dom v-voronezhe[/url]
Процесс вывода из запоя требует быстрого реагирования. Запой, особенно в его тяжелых формах, представляет угрозу не только для психического состояния пациента, но и для его физического здоровья. Чтобы минимизировать риски и предотвратить осложнения, важно начинать лечение как можно скорее. Клинике «Освобождение» в Рязани доступна круглосуточная помощь — мы работаем 24/7, чтобы оперативно вмешаться в любой момент, когда это необходимо. Врачебная помощь может быть предоставлена как в стационаре клиники, так и на дому, в зависимости от состояния пациента и его предпочтений.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]vyvod iz zapoya na domu kruglosutochno [/url]
Запой – это состояние, которое возникает вследствие длительного употребления алкоголя, приводящее к потере контроля над количеством выпиваемого и формированию как физической, так и психологической зависимости. Оно сопровождается серьёзными последствиями для здоровья и требует немедленного вмешательства.
Подробнее тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-stacionare-v-voronezhe/]vyvod iz zapoya v stacionare anonimno v-voronezhe[/url]
В центре доступны различные варианты лечения – от амбулаторного наблюдения до интенсивных стационарных программ. Ключевые принципы работы – конфиденциальность, индивидуальный подход и оперативность оказания помощи. Независимо от тяжести зависимости, каждый пациент получает квалифицированную поддержку, направленную на восстановление здоровья и возвращение к полноценной жизни.
Узнать больше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]вывод из запоя анонимно [/url]
Клиника «Шаг к Трезвости» в Краснодаре предлагает анонимный вывод из запоя, обеспечивая полную конфиденциальность и безопасность на каждом этапе лечения. Мы понимаем, что для многих людей важно сохранить свою личную информацию в тайне, и гарантируем, что все данные о пациенте и его состоянии здоровья останутся закрытыми. Независимо от того, выбрал ли пациент стационарное лечение или вывод из запоя на дому, мы обеспечиваем высококачественную медицинскую помощь с полным соблюдением принципов конфиденциальности.
Детальнее – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]вывод из запоя круглосуточно наркология [/url]
Проблема зависимостей остаётся актуальной в современном обществе. С каждым годом увеличивается число людей, страдающих от алкоголизма, наркомании и других форм зависимостей, что негативно отражается на их жизни и благополучии близких. Зависимость — это не просто физическое заболевание, но и глубокая психологическая проблема. Для эффективного лечения требуется помощь профессионалов, способных обеспечить комплексный подход.
Подробнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]вывод из запоя цена [/url]
Процесс вывода из запоя требует быстрого реагирования. Запой, особенно в его тяжелых формах, представляет угрозу не только для психического состояния пациента, но и для его физического здоровья. Чтобы минимизировать риски и предотвратить осложнения, важно начинать лечение как можно скорее. Клинике «Освобождение» в Рязани доступна круглосуточная помощь — мы работаем 24/7, чтобы оперативно вмешаться в любой момент, когда это необходимо. Врачебная помощь может быть предоставлена как в стационаре клиники, так и на дому, в зависимости от состояния пациента и его предпочтений.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]srochny vyvod iz zapoya na domu v-ryazani[/url]
Запой – это тяжелое состояние, возникающее из-за длительного и неконтролируемого употребления алкоголя. Оно характеризуется физической и психологической зависимостью, что приводит к серьезным последствиям для организма и психики. Для восстановления нормального самочувствия и здоровья необходимо профессиональное лечение. Одним из наиболее удобных и эффективных методов является вывод из запоя на дому.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-v-kruglosutochno-v-ryazani/]vyvod iz zapoya kruglosutochno narkologiya [/url]
Запой — это состояние, при котором человек не может прекратить употребление алкоголя на протяжении длительного времени, что вызывает физическую и психологическую зависимость. Это крайне опасное состояние, требующее немедленного вмешательства специалистов. Наркологическая клиника «Преображение» в Екатеринбурге предоставляет профессиональную помощь в лечении запоя, используя самые эффективные и безопасные методы.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]srochny vyvod iz zapoya na domu ekaterinburg[/url]
В этой статье мы расскажем о возможностях клиники для лечения алкогольной интоксикации круглосуточно, включая вывод из запоя в стационаре и на дому, а также о роли профессионалов, таких как нарколог, в процессе лечения.
Выяснить больше – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]anonimny vyvod iz zapoya na domu krasnodar[/url]
Запой – это состояние, которое возникает вследствие длительного употребления алкоголя, приводящее к потере контроля над количеством выпиваемого и формированию как физической, так и психологической зависимости. Оно сопровождается серьёзными последствиями для здоровья и требует немедленного вмешательства.
Подробнее тут – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-anonimno-v-voronezhe/]анонимный вывод из запоя на дому в воронеже[/url]
Вывод из запоя представляет собой важный этап в процессе лечения алкогольной зависимости. Это медицинская процедура, необходимая для устранения токсических веществ, накопленных в организме в результате длительного употребления алкоголя. Алкогольная зависимость может вызывать множество проблем, включая физические и психоэмоциональные расстройства. Своевременное вмешательство специалистов помогает минимизировать риски и способствует восстановлению здоровья пациента.
Углубиться в тему – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]вывод из запоя на дому рязань[/url]
Своевременное начало лечения играет решающую роль, так как промедление может усугубить как физическое, так и психическое состояние. Квалифицированные специалисты готовы оказать помощь в любое время, гарантируя анонимность и индивидуальный подход.
Углубиться в тему – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]нарколог на дом вывод из запоя рязань[/url]
Запой — это тяжелое состояние, при котором человек теряет контроль над количеством употребляемого алкоголя, что приводит к серьезным нарушениям здоровья. Процесс вывода из запоя требует профессионального подхода, медицинского контроля и тщательного восстановления организма. Наркологическая клиника «Шаг к Трезвости» в Краснодаре предлагает квалифицированную помощь для тех, кто нуждается в срочном и безопасном выходе из запоя. Мы предоставляем комплексное лечение, ориентированное на каждый этап восстановления, с максимальной заботой о здоровье пациента.
Углубиться в тему – [url=https://vyvod-iz-zapoya-12.ru/]vyvod iz zapoya v-krasnodare[/url]
В таких ситуациях вывод из запоя на дому становится оптимальным решением, особенно если пациент не может или не хочет посещать стационар. Эта услуга позволяет получить медицинскую помощь в комфортной и привычной обстановке, что снижает уровень стресса и ускоряет процесс восстановления.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-11.ru/]vyvod iz zapoya s vyezdom v-voronezhe[/url]
Программы лечения разрабатываются индивидуально и могут включать как визит врача на дом, так и стационарное пребывание. Стоимость услуг формируется в зависимости от необходимых процедур и остается прозрачной, позволяя пациентам сосредоточиться на главном – восстановлении здоровья и возвращении к полноценной жизни.
Узнать больше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]вывод из запоя круглосуточно наркология санкт-петербург[/url]
Проблема зависимостей остаётся актуальной в современном обществе. С каждым годом увеличивается число людей, страдающих от алкоголизма, наркомании и других форм зависимостей, что негативно отражается на их жизни и благополучии близких. Зависимость — это не просто физическое заболевание, но и глубокая психологическая проблема. Для эффективного лечения требуется помощь профессионалов, способных обеспечить комплексный подход.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-11.ru/]narkologicheskaya klinika v-voronezhe[/url]
В медицинском центре «Второй Шанс» помощь оказывается круглосуточно. Применяются проверенные и эффективные методы, обеспечивающие безопасность пациента и устойчивый результат. Конфиденциальность гарантирует комфорт и спокойствие тем, кто обратился за поддержкой, позволяя сосредоточиться на выздоровлении.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-14.ru/]vyvod iz zapoya kapelnica v-sankt-peterburge[/url]
Алкоголизм — это хроническое заболевание, которое характеризуется патологической зависимостью от этанола. Периоды запоя, когда человек неконтролируемо потребляет алкоголь, могут привести к разнообразным медицинским осложнениям, как физическим, так и психологическим. Запой становится не только причиной ухудшения здоровья, но и причиной множества социальных и личных проблем. На этом фоне возникает необходимость вывода из запоя, процесс, который должен учитывать индивидуальные особенности пациента. Важно, что анонимность в реабилитации играет ключевую роль, помогая избежать стигматизации и увеличивая шансы на успешное восстановление.
Детальнее – [url=https://vyvod-iz-zapoya-13.ru/]http://vyvod-iz-zapoya-13.ru[/url]
Алкогольная зависимость — это серьезная проблема, требующая незамедлительного вмешательства, чтобы избежать тяжелых последствий для здоровья. Запойное пьянство может привести к различным заболеваниям, таким как цирроз печени, панкреатит и другие опасные состояния. К счастью, современная медицина предлагает эффективные методы решения этой проблемы. Для тех, кто не хочет или не может посетить стационар, есть возможность пройти процедуру капельницы от запоя на дому в Твери.
Подробнее – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-cena-v-tveri/]вывод из запоя цена наркология тверь[/url]
Алкоголизм — это хроническое прогрессирующее заболевание, связанное с физической и психической зависимостью от этанола. Запой, как его крайнее проявление, представляет собой длительное употребление алкоголя, сопровождающееся тяжелыми нарушениями работы организма, включая абстинентный синдром и потенциальные угрозы для жизни. Лечение запоя требует комплексного подхода, включающего медицинские процедуры, психотерапию и реабилитационные меры.
Получить больше информации – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-v-kruglosutochno-v-ekaterinburge/]вывод из запоя круглосуточно [/url]
Лечение зависимости требует комплексного подхода, сочетающего медицинские методы и психологическую поддержку. Это не просто вредная привычка, а хроническое заболевание, требующее тщательного контроля и длительной терапии. В центре используются современные научно обоснованные методики, помогающие пациентам преодолеть сложный путь выздоровления. Каждому человеку подбирается индивидуальная программа, учитывающая его особенности и жизненные обстоятельства.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]вывод из запоя на дому цена санкт-петербург[/url]
Зависимость – это сложное испытание, с которым сталкиваются люди во всём мире. Ежедневные стрессы, проблемы в семье и на работе, финансовые трудности часто становятся почвой для развития пагубных привычек, таких как алкоголизм, наркомания и игромания. Медицинский центр «Второй Шанс» предоставляет помощь тем, кто хочет избавиться от этих состояний, вернуть здоровье и научиться жить без разрушительных зависимостей.
Разобраться лучше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]vyvod iz zapoya kruglosutochno v-sankt-peterburge[/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Детальнее – [url=https://vyvod-iz-zapoya-11.ru/]http://www.vyvod-iz-zapoya-11.ru[/url]
Клиника «Освобождение» в Рязани предоставляет услугу круглосуточного вывода из запоя на дому, что позволяет пациенту получить медицинскую помощь в привычной обстановке, избегая лишнего стресса, связанного с госпитализацией.
Разобраться лучше – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]вывод из запоя на дому недорого в рязани[/url]
Запой — это состояние, характеризующееся утратой контроля над употреблением алкоголя, что приводит к тяжелой интоксикации организма. Вывод из запоя — это комплексный процесс, направленный на устранение последствий интоксикации и восстановление нормального функционирования организма. Цена данной процедуры может значительно различаться в зависимости от нескольких факторов, и в этой статье мы подробно рассмотрим, что влияет на стоимость вывода из запоя в Краснодаре, в том числе в клинике «Шаг к Трезвости».
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]vyvod iz zapoya srochno kruglosutochno [/url]
Особенно опасны длительные запои, когда человек в течение нескольких дней бесконтрольно употребляет алкоголь. Такое состояние разрушает организм, перегружает печень, сердце и нервную систему, может вызывать тяжелые осложнения, в том числе психические расстройства. Выйти из запоя самостоятельно практически невозможно и крайне рискованно. Важно своевременно обратиться за медицинской помощью, чтобы избежать серьезных последствий.
Подробнее – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]наркология вывод из запоя на дому санкт-петербург[/url]
Запой — это состояние, характеризующееся утратой контроля над употреблением алкоголя, что приводит к тяжелой интоксикации организма. Вывод из запоя — это комплексный процесс, направленный на устранение последствий интоксикации и восстановление нормального функционирования организма. Цена данной процедуры может значительно различаться в зависимости от нескольких факторов, и в этой статье мы подробно рассмотрим, что влияет на стоимость вывода из запоя в Краснодаре, в том числе в клинике «Шаг к Трезвости».
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-12.ru/]вывод из запоя краснодар[/url]
Процедура капельницы особенно показана в случаях, когда симптомы похмелья выражены настолько сильно, что пациент не в состоянии справиться с ними самостоятельно. Головная боль, тошнота, рвота, усталость и раздражительность — все это признаки того, что организму необходимо восстановление. Капельница помогает не только ускорить процесс очищения, но и минимизировать риск осложнений, таких как нарушение работы сердца и печени.
Узнать больше – [url=https://kapelnica-ot-zapoya-ektb55.ru/]kapelnica ot zapoya [/url]
Зависимость – это сложное испытание, с которым сталкиваются люди во всём мире. Ежедневные стрессы, проблемы в семье и на работе, финансовые трудности часто становятся почвой для развития пагубных привычек, таких как алкоголизм, наркомания и игромания. Медицинский центр «Второй Шанс» предоставляет помощь тем, кто хочет избавиться от этих состояний, вернуть здоровье и научиться жить без разрушительных зависимостей.
Получить больше информации – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/[/url]
Запой — это серьезное состояние, при котором человек не может прекратить употребление алкоголя на протяжении длительного времени, что вызывает физическую и психологическую зависимость. Это крайне опасное состояние, требующее немедленного вмешательства специалистов. Для безопасного и эффективного вывода из запоя необходима квалифицированная медицинская помощь, которую предоставляет наркологическая клиника «Рассвет» в Твери. Наши опытные специалисты используют современные методы детоксикации и восстановления организма, обеспечивая полный медицинский контроль и поддержку на всех этапах лечения.
Получить больше информации – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-anonimno-v-tveri/]анонимный вывод из запоя на дому в твери[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Подробнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-kruglosutochno-v-voronezhe/]vyvod iz zapoya kruglosutochno narkologiya voronezh[/url]
Программы лечения разрабатываются индивидуально и могут включать как визит врача на дом, так и стационарное пребывание. Стоимость услуг формируется в зависимости от необходимых процедур и остается прозрачной, позволяя пациентам сосредоточиться на главном – восстановлении здоровья и возвращении к полноценной жизни.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya s vyezdom na dom sankt-peterburg[/url]
Алкогольная зависимость — это серьезная проблема, требующая незамедлительного вмешательства, чтобы избежать тяжелых последствий для здоровья. Запойное пьянство может привести к различным заболеваниям, таким как цирроз печени, панкреатит и другие опасные состояния. К счастью, современная медицина предлагает эффективные методы решения этой проблемы. Для тех, кто не хочет или не может посетить стационар, есть возможность пройти процедуру капельницы от запоя на дому в Твери.
Подробнее тут – [url=https://vyvod-iz-zapoya-21.ru/]vyvod iz zapoya kapelnica[/url]
Вывод из запоя — это старт на пути к восстановлению. Он открывает дорогу к полноценной реабилитации, позволяя вернуться к нормальной жизни. Поддержка профессионалов помогает справляться с последствиями зависимости и снижает риск её повторения, что делает этот процесс важным шагом в борьбе за здоровье и благополучие.
Подробнее – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-cena-v-ryazani/]вывод из запоя с выездом цена [/url]
Алкогольная зависимость — это длительное и прогрессирующее заболевание, при котором развивается как физическая, так и психическая зависимость от алкоголя. Одним из самых тяжелых проявлений болезни является запой — длительное употребление алкоголя, которое может сопровождаться нарушениями работы организма и абстинентным синдромом, представляющим серьезную угрозу для здоровья. Эффективное лечение запоя требует комплексного подхода, включающего медикаментозную терапию, психотерапию и реабилитационные мероприятия.
Выяснить больше – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]srochny vyvod iz zapoya na domu [/url]
Особенно опасны длительные запои, когда человек в течение нескольких дней бесконтрольно употребляет алкоголь. Такое состояние разрушает организм, перегружает печень, сердце и нервную систему, может вызывать тяжелые осложнения, в том числе психические расстройства. Выйти из запоя самостоятельно практически невозможно и крайне рискованно. Важно своевременно обратиться за медицинской помощью, чтобы избежать серьезных последствий.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]vyvod iz zapoya cena narkologiya [/url]
Клиника «Освобождение» в Рязани предоставляет услугу круглосуточного вывода из запоя на дому, что позволяет пациенту получить медицинскую помощь в привычной обстановке, избегая лишнего стресса, связанного с госпитализацией.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]вывод из запоя на дому недорого [/url]
Процедура капельницы особенно показана в случаях, когда симптомы похмелья выражены настолько сильно, что пациент не в состоянии справиться с ними самостоятельно. Головная боль, тошнота, рвота, усталость и раздражительность — все это признаки того, что организму необходимо восстановление. Капельница помогает не только ускорить процесс очищения, но и минимизировать риск осложнений, таких как нарушение работы сердца и печени.
Изучить вопрос глубже – [url=https://kapelnica-ot-zapoya-ektb55.ru/]http://kapelnica-ot-zapoya-ektb55.ru[/url]
Алкогольный запой — это тяжелое состояние, при котором человек теряет контроль над количеством потребляемого алкоголя. Это приводит к сильной интоксикации, сбоям в работе органов и систем организма, а также серьезным психоэмоциональным расстройствам. Без должной медицинской помощи запой может вызвать ряд опасных последствий, включая алкогольный делирий и угрозу жизни. Поэтому при первых признаках запоя необходимо обратиться к профессионалам.
Получить больше информации – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-na-domu-v-tveri/]нарколог на дом вывод из запоя в твери[/url]
Алкогольная зависимость представляет собой серьезную медицинскую проблему, которая влечет за собой не только физические, но и психологические проблемы. Периоды запоя, характеризующиеся бесконтрольным употреблением алкоголя, могут привести к тяжелым последствиям. Симптомы абстиненции требуют незамедлительного вмешательства. Анонимность в процессе реабилитации становится важным аспектом, помогающим пациентам избежать стыда и социального осуждения.
Подробнее тут – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]vyvod iz zapoya anonimno v-krasnodare[/url]
Выведение из запоя – это процесс, включающий несколько этапов. Симптомы могут варьироваться от тревожности и бессонницы до серьезных психических расстройств. Только профессиональный подход и современные медицинские технологии помогают минимизировать риски, стабилизировать состояние и избежать осложнений.
Подробнее – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]быстрый вывод из запоя в стационаре санкт-петербург[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьёзное воздействие этанола. Это приводит к нарушениям нормальной физиологической деятельности и ухудшению состояния здоровья. Анонимный вывод из запоя — это медицинская помощь, направленная на устранение последствий чрезмерного употребления алкоголя с соблюдением строгой конфиденциальности.
Детальнее – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]vyvod iz zapoya na domu kruglosutochno [/url]
Современное общество сталкивается с серьёзными вызовами, связанными с зависимостями. Алкоголизм, как одна из самых распространённых проблем, оказывает разрушительное воздействие на здоровье человека, разрушая семейные отношения и затрудняя социальную адаптацию. В такие моменты крайне важно обратиться за квалифицированной помощью.
Узнать больше – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]вывод из запоя с выездом на дом [/url]
Процедура капельницы особенно показана в случаях, когда симптомы похмелья выражены настолько сильно, что пациент не в состоянии справиться с ними самостоятельно. Головная боль, тошнота, рвота, усталость и раздражительность — все это признаки того, что организму необходимо восстановление. Капельница помогает не только ускорить процесс очищения, но и минимизировать риск осложнений, таких как нарушение работы сердца и печени.
Узнать больше – [url=https://kapelnica-ot-zapoya-ektb55.ru/]после капельницы от запоя в екатеринбурге[/url]
Для тех, кто не может приехать в клинику, специалисты проводят детоксикацию на дому. С помощью современных препаратов организм мягко очищается от токсинов, уменьшается нагрузка на внутренние органы, снимаются неприятные симптомы абстиненции. Однако при тяжелом состоянии или наличии осложнений может потребоваться круглосуточное наблюдение в стационаре, где пациенту обеспечивается полная медицинская поддержка.
Выяснить больше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя на дому в санкт-петербурге[/url]
I’ve been using Metamask for a while, but I recently needed to reinstall the Metamask Chrome extension. https://metamaker.org/#metamask-download made the process super simple, and now my wallet is back up and running. This site is a lifesaver!
Запой — это состояние, характеризующееся утратой контроля над употреблением алкоголя, что приводит к тяжелой интоксикации организма. Вывод из запоя — это комплексный процесс, направленный на устранение последствий интоксикации и восстановление нормального функционирования организма. Цена данной процедуры может значительно различаться в зависимости от нескольких факторов, и в этой статье мы подробно рассмотрим, что влияет на стоимость вывода из запоя в Краснодаре, в том числе в клинике «Шаг к Трезвости».
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-12.ru/]vyvod iz zapoya s vyezdom[/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Подробнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]narkologiya vyvod iz zapoya na domu v-voronezhe[/url]
Алкогольный запой – это серьёзное состояние, при котором человек теряет контроль над употреблением алкоголя, что приводит к тяжёлой интоксикации организма. Во время запоя нарушаются функции внутренних органов, страдает нервная система, и повышается риск развития опасных осложнений, таких как алкогольный делирий. Это состояние требует незамедлительного медицинского вмешательства.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]vyvod iz zapoya na domu nedorogo [/url]
Вывод из запоя — это старт на пути к восстановлению. Он открывает дорогу к полноценной реабилитации, позволяя вернуться к нормальной жизни. Поддержка профессионалов помогает справляться с последствиями зависимости и снижает риск её повторения, что делает этот процесс важным шагом в борьбе за здоровье и благополучие.
Детальнее – [url=https://vyvod-iz-zapoya-13.ru/]vyvod iz zapoya s vyezdom v-ryazani[/url]
Алкогольная интоксикация, или запой, представляет собой крайне опасное состояние, при котором организм страдает от токсического воздействия алкоголя. Это ведет к нарушению нормальных физиологических процессов и ухудшению общего состояния здоровья. Капельница от запоя — это медицинская процедура, направленная на выведение токсинов из организма и восстановление нормальной функции организма при соблюдении полной конфиденциальности.
Подробнее – [url=https://vyvod-iz-zapoya-21.ru/]вывод из запоя капельница[/url]
1Win Kenya is a premier online betting platform offering a seamless gambling experience for sports and casino enthusiasts., offering a seamless experience for sports betting and casino gaming. As a trusted name in Kenya, 1win Kenya provides users with a secure platform, a vast selection of games, and flexible betting options on sports like cricket, soccer, tennis, and basketball. Registering on 1Win is simple—just visit the official website, click on “Sign Up,” and choose to register via email or social media. Once you create an account, fund it with a deposit to start betting instantly. The 1Win casino section offers a premium gaming experience with various slots, table games, and live dealer options. To enhance the excitement, new and existing players can unlock generous rewards with the 1Win bonus codes, gaining a significant advantage in their gameplay. The 1Win Bet app allows seamless sports predictions and betting on the go, ensuring uninterrupted access to all features. With a rapidly growing audience and a commitment to quality service, 1Win Kenya continues to set the standard for online gambling. Sign up today, claim your bonuses, and enjoy a thrilling betting experience with 1Win!
зеленая ферма взлом [url=https://apk-smart.com/igry/strategii/1606-zelenaja-ferma-3-prohozhdenie-i-vzlom.html]https://apk-smart.com/igry/strategii/1606-zelenaja-ferma-3-prohozhdenie-i-vzlom.html[/url] зеленая ферма взлом
P.S Live ID: K89Io9blWX1UfZWv3ajv
P.S.S [url=http://www5a.biglobe.ne.jp/%7Esumireo/itakiss/nan-demo12/ankothologysx.cgi?action=html2&key=20160718220301&log=]Программы и игры для Андроид телефона[/url] [url=https://vintagebynihal.com/show-product.php?product=indian-vintage-kabiri-laptop-bag-authentic-banjara-envelope-dowry-bag]Программы и игры для Андроид телефона[/url] [url=https://knaeewdse.click/2024/04/08/hello-world/comment-page-658/#comment-34911]Программы и игры для Андроид телефона[/url] ff71b59
впн для компьютера
Предлагаем вашему вниманию интересную справочную статью, в которой собраны ключевые моменты и нюансы по актуальным вопросам. Эта информация будет полезна как для профессионалов, так и для тех, кто только начинает изучать тему. Узнайте ответы на важные вопросы и расширьте свои знания!
Подробнее можно узнать тут – https://narko-zakodirovat1.ru/
Контроль веса животных – важный фактор в животноводстве. Надежные [url=https://moloko18.ru/]весы для крс купить[/url] можно в специализированном магазине. Это оборудование поможет точно отслеживать развитие поголовья и вести учет в хозяйстве.
Оформи [url=https://telegra.ph/Zajmy-bez-procentov-na-30-dnej—poluchite-zajm-pod-0-bez-otkaza-i-proverok-na-kartu-02-10]займы онлайн на карту без отказа под 0 процентов[/url] и получи до 30 000 рублей за 5 минут. Без проверок и скрытых платежей!
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от легкой тревожности до серьезных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Разобраться лучше – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-na-domu-v-chelyabinske/]вывод из запоя на дому круглосуточно челябинск[/url]
Наркологическая клиника «Союз Здоровья» в Воронеже специализируется на лечении алкогольной зависимости и предлагает профессиональные услуги по выводу из запоя. Мы стремимся создать атмосферу доверия, где каждый пациент сможет получить необходимую поддержку.
Выяснить больше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]вывод из запоя с выездом на дом [/url]
Средняя цена услуги в Екатеринбурге варьируется в зависимости от объёма лечения. Консультация нарколога может стоить от 3 000 до 5 000 рублей. Если требуется вывод из запоя, стоимость обычно находится в диапазоне от 5 000 до 12 000 рублей.
Получить дополнительную информацию – [url=https://narcolog-na-dom-ekaterinburg55.ru /]нарколог на дом стоимость в екатеринбурге[/url]
мтс тарифы новосибирск
https://melocasting.com/employer/chotaikhoan525/
мтс подключить новосибирск
Капельница от запоя — это терапевтическая процедура, обеспечивающая очищение организма, элиминацию токсических веществ и возвращение пациента к нормальному состоянию после алкогольной интоксикации. В состав капельницы входят следующие компоненты
Подробнее – [url=https://kapelnica-ot-zapoya-msk55.ru/]https://kapelnica-ot-zapoya-msk55.ru/kapelnicza-ot-zapoya-na-domu-moskva/[/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-stacionare-v-voronezhe/]вывод из запоя в стационаре анонимно в воронеже[/url]
Услуга вызова нарколога на дом в Красноярске — это удобный и современный способ получения медицинской помощи. Она идеально подходит для тех, кто нуждается в профессиональной поддержке, но по каким-либо причинам не может или не хочет посещать клинику.
Подробнее – [url=https://narcolog-na-dom-krasnoyarsk55.ru/]вызов нарколога ценакрасноярск[/url]
Изысканные холсты с печатью в студии, которые вдохнут на творчество.
Эксклюзивные холсты с печатью на заказ, для уникального оформления помещения.
Холсты с печатью: профессиональное искусство, для тех, кто ценит качество.
Индивидуальные холсты из студии печати, для тех, кто ценит креатив.
Студия печати: красочные идеи для подарков, которые понравятся вашим близким.
картина по фото на холсте на заказ цена [url=https://studiya-pechati-na-holste.ru]https://studiya-pechati-na-holste.ru[/url] .
Клиника «Рассвет» в Твери предоставляет услугу анонимного вывода из запоя, обеспечивая высокое качество медицинского обслуживания и строгое соблюдение всех этических норм.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-21.ru/]https://vyvod-iz-zapoya-21.ru[/url]
Выведение из запоя возможно как в условиях стационара, так и при выезде специалиста на дом. Стоимость этих вариантов лечения может существенно отличаться.
Узнать больше – [url=https://kapelnica-ot-zapoya-msk55.ru/]kapelnica-ot-zapoya-msk55.ru/[/url]
В современном мире проблемы, связанные с алкогольной или наркотической зависимостью, становятся всё более актуальными. Многие люди сталкиваются с трудностями, которые требуют незамедлительного вмешательства профессионалов. В Екатеринбурге вызов нарколога на дом — это не только удобно, но и эффективно. Пациенты получают квалифицированную помощь без необходимости покидать комфортные условия своего жилья, что особенно важно в критических ситуациях.
Подробнее – [url=https://narcolog-na-dom-ekaterinburg55.ru /]https://narcolog-na-dom-ekaterinburg55.ru [/url]
Вывод из запоя — это медицинская процедура, необходимая для восстановления организма после длительного употребления алкоголя. Это состояние, часто характеризующееся физическими и психоэмоциональными нарушениями, требует профессионального вмешательства. Симптомы абстиненции могут быть разнообразными и варьироваться от лёгкой тревожности до серьёзных нарушений в функционировании внутренних органов. Квалифицированная помощь в этот период позволяет минимизировать риски и значительно ускорить процесс выздоровления.
Углубиться в тему – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-v-stacionare-v-voronezhe/]вывод из запоя в стационаре анонимно воронеж[/url]
Зависимость от алкоголя или наркотических веществ — это серьёзная проблема, требующая профессионального вмешательства. В критических ситуациях вызов нарколога на дом становится оптимальным решением. Услуга позволяет получить квалифицированную медицинскую помощь в комфортных условиях, что особенно важно для пациентов, которым трудно или невозможно посетить клинику.
Ознакомиться с деталями – [url=https://narcolog-na-dom-ekaterinburg55.ru /]нарколог на дом цены екатеринбург[/url]
Лечение в домашних условиях имеет множество достоинств, среди которых ключевыми являются комфорт, доступность и конфиденциальность. Пациент может пройти лечение в привычной обстановке, что снижает уровень тревожности и способствует более быстрому восстановлению.
Подробнее – [url=https://narcolog-na-dom-ekaterinburg55.ru /]http://narcolog-na-dom-ekaterinburg55.ru/narkolog-na-dom-kruglosutochno-ekaterinburg[/url]
Наркологическая клиника «Союз Здоровья» в Воронеже специализируется на лечении алкогольной зависимости и предлагает профессиональные услуги по выводу из запоя. Мы стремимся создать атмосферу доверия, где каждый пациент сможет получить необходимую поддержку.
Подробнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]вывод из запоя на дому недорого воронеж[/url]
Запой представляет собой состояние, характеризующееся длительным непрерывным употреблением алкоголя, что приводит к физической и психической зависимости. Этот процесс может длиться от нескольких дней до недель. Алкогольные напитки негативно влияют на функционирование внутренних органов, вызывая серьёзные нарушения, включая алкоголизм. При необходимости вывода из запоя важно обратиться за медицинской помощью, что можно осуществить на дому. В данной статье рассмотрим ключевые аспекты данной процедуры.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-cena-v-chelyabinske/]narkolog vyvod iz zapoya cena chelyabinsk[/url]
Многие медцентры используют современные методики детоксикации с применением эффективных препаратов для облегчения абстинентного синдрома. Пациентам доступно полное медицинское обследование для выявления сопутствующих патологий и разработки оптимальной схемы лечения.
Ознакомиться с деталями – [url=https://kapelnica-ot-zapoya-msk55.ru/]капельницу от запоя в москве[/url]
Этот вид услуги востребован благодаря своей оперативности, индивидуальному подходу и конфиденциальности. Вызов врача позволяет минимизировать стресс, обеспечить комфортное лечение и своевременно предотвратить возможные осложнения.
Разобраться лучше – [url=https://narcolog-na-dom-ekaterinburg55.ru /]https://narcolog-na-dom-ekaterinburg55.ru/narkolog-na-dom-ekaterinburg-tseny[/url]
Зависимость – это сложное испытание, с которым сталкиваются люди во всём мире. Ежедневные стрессы, проблемы в семье и на работе, финансовые трудности часто становятся почвой для развития пагубных привычек, таких как алкоголизм, наркомания и игромания. Медицинский центр «Второй Шанс» предоставляет помощь тем, кто хочет избавиться от этих состояний, вернуть здоровье и научиться жить без разрушительных зависимостей.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя на дому недорого капельница санкт-петербург[/url]
Выведение из запоя возможно как в условиях стационара, так и при выезде специалиста на дом. Стоимость этих вариантов лечения может существенно отличаться.
Разобраться лучше – [url=https://kapelnica-ot-zapoya-msk55.ru/]kapelnica ot zapoya [/url]
В современном мире проблемы, связанные с алкогольной или наркотической зависимостью, становятся всё более актуальными. Многие люди сталкиваются с трудностями, которые требуют незамедлительного вмешательства профессионалов. В Екатеринбурге вызов нарколога на дом — это не только удобно, но и эффективно. Пациенты получают квалифицированную помощь без необходимости покидать комфортные условия своего жилья, что особенно важно в критических ситуациях.
Ознакомиться с деталями – [url=https://narcolog-na-dom-ekaterinburg55.ru /]выезд нарколога на дом цена в екатеринбурге[/url]
В медицинском центре «Второй Шанс» помощь оказывается круглосуточно. Применяются проверенные и эффективные методы, обеспечивающие безопасность пациента и устойчивый результат. Конфиденциальность гарантирует комфорт и спокойствие тем, кто обратился за поддержкой, позволяя сосредоточиться на выздоровлении.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]наркология вывод из запоя анонимно в санкт-петербурге[/url]
Цена услуги формируется с учётом нескольких факторов. Один из ключевых моментов — квалификация специалиста. Опытный врач, обладающий знаниями и практическими навыками, способен оказать помощь быстро и эффективно, что отражается на стоимости услуги.
Детальнее – [url=https://narcolog-na-dom-ekaterinburg55.ru /]http://narcolog-na-dom-ekaterinburg55.ru/narkolog-na-dom-ekaterinburg-tseny/[/url]
Проблема зависимостей остаётся актуальной в современном обществе. С каждым годом увеличивается число людей, страдающих от алкоголизма, наркомании и других форм зависимостей, что негативно отражается на их жизни и благополучии близких. Зависимость — это не просто физическое заболевание, но и глубокая психологическая проблема. Для эффективного лечения требуется помощь профессионалов, способных обеспечить комплексный подход.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]narkolog na dom vyvod iz zapoya v-voronezhe[/url]
Алкоголизм – одно из наиболее разрушительных заболеваний, наносящих серьезный ущерб организму. Запойные состояния особенно опасны, так как приводят к глубокой интоксикации, психическим нарушениям и необратимым последствиям. Чем раньше начато лечение, тем выше вероятность избежать осложнений и быстрее восстановиться.
Углубиться в тему – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu nedorogo [/url]
мтс подключить новосибирск
https://partyandeventjobs.com/employer/gigsonline8369/
мтс тв
В медицинском центре «Второй Шанс» помощь оказывается круглосуточно. Применяются проверенные и эффективные методы, обеспечивающие безопасность пациента и устойчивый результат. Конфиденциальность гарантирует комфорт и спокойствие тем, кто обратился за поддержкой, позволяя сосредоточиться на выздоровлении.
Разобраться лучше – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]vyvod iz zapoya kruglosutochno narkologiya [/url]
check this site out https://my-sollet.com/
my review here https://web-kaspawallet.com
Многие медцентры используют современные методики детоксикации с применением эффективных препаратов для облегчения абстинентного синдрома. Пациентам доступно полное медицинское обследование для выявления сопутствующих патологий и разработки оптимальной схемы лечения.
Изучить вопрос глубже – [url=https://kapelnica-ot-zapoya-msk55.ru/]https://kapelnica-ot-zapoya-msk55.ru/[/url]
Наркологическая клиника «Союз Здоровья» в Воронеже специализируется на лечении алкогольной зависимости и предлагает профессиональные услуги по выводу из запоя. Мы стремимся создать атмосферу доверия, где каждый пациент сможет получить необходимую поддержку.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]вывод из запоя на дому цена воронеж[/url]
Этот вид услуги востребован благодаря своей оперативности, индивидуальному подходу и конфиденциальности. Вызов врача позволяет минимизировать стресс, обеспечить комфортное лечение и своевременно предотвратить возможные осложнения.
Получить дополнительные сведения – [url=https://narcolog-na-dom-ekaterinburg55.ru /]http://narcolog-na-dom-ekaterinburg55.ru [/url]
Программы лечения разрабатываются индивидуально и могут включать как визит врача на дом, так и стационарное пребывание. Стоимость услуг формируется в зависимости от необходимых процедур и остается прозрачной, позволяя пациентам сосредоточиться на главном – восстановлении здоровья и возвращении к полноценной жизни.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]вывод из запоя круглосуточно в санкт-петербурге[/url]
Средняя цена услуги в Екатеринбурге варьируется в зависимости от объёма лечения. Консультация нарколога может стоить от 3 000 до 5 000 рублей. Если требуется вывод из запоя, стоимость обычно находится в диапазоне от 5 000 до 12 000 рублей.
Разобраться лучше – [url=https://narcolog-na-dom-ekaterinburg55.ru /]нарколог екатеринбург[/url]
Алкоголизм – одно из наиболее разрушительных заболеваний, наносящих серьезный ущерб организму. Запойные состояния особенно опасны, так как приводят к глубокой интоксикации, психическим нарушениям и необратимым последствиям. Чем раньше начато лечение, тем выше вероятность избежать осложнений и быстрее восстановиться.
Узнать больше – [url=https://vyvod-iz-zapoya-14.ru/]vyvod iz zapoya s vyezdom sankt-peterburg[/url]
Стоимость вывода из запоя зависит от ряда факторов, таких как состояние пациента, выбранная программа лечения и место проведения процедуры. Мы предлагаем прозрачное ценообразование, чтобы вы могли заранее ознакомиться с возможными затратами на лечение.
Подробнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-anonimno-v-chelyabinske/]вывод из запоя анонимно в челябинске[/url]
Алкогольная зависимость — это тяжелое состояние, требующее срочного медицинского вмешательства. В столице растет число пациентов, предпочитающих получать медицинскую помощь в домашних условиях, и капельница от запоя на дому в Москве становится востребованным и результативным методом лечения. Данный подход позволяет эффективно восстановить организм пациента в привычной обстановке без необходимости пребывания в больнице.
Получить дополнительные сведения – [url=https://kapelnica-ot-zapoya-msk55.ru/]http://kapelnica-ot-zapoya-msk55.ru/kapelnicza-ot-zapoya-tsena-moskva/[/url]
В центре доступны различные варианты лечения – от амбулаторного наблюдения до интенсивных стационарных программ. Ключевые принципы работы – конфиденциальность, индивидуальный подход и оперативность оказания помощи. Независимо от тяжести зависимости, каждый пациент получает квалифицированную поддержку, направленную на восстановление здоровья и возвращение к полноценной жизни.
Выяснить больше – [url=https://vyvod-iz-zapoya-14.ru/]vyvod iz zapoya s vyezdom v-sankt-peterburge[/url]
Турецкие фильмы набрали высокую популярность во всем мире. Турция славится своими качественными сериалами, которые привлекают зрителей своей захватывающей сюжетной линией, эмоциональными переживаниями и качественной актерской игрой.
Турецкие сериалы имеют различные жанры, на любой вкус. От романтики до исторических драм, от детективов до фантастики – есть жанры на любой вкус. Богатство сюжетов и уровень съемок делают турецкие сериалы невероятными шедеврами мирового кинематографа.
[url=https://turkish-film1.ru/]Новые турецкие сериалы на русском[/url] – это уникальная возможность отправиться в другую культуру, узнать больше о традициях и обычаях Турции.
В клинике «Излечение» мы предлагаем широкий спектр услуг по выводу из запоя, включая как стационарное лечение, так и помощь на дому. В этой статье мы подробно рассмотрим, от чего зависит цена вывода из запоя и какие услуги входят в стоимость.
Выяснить больше – [url=https://vyvod-iz-zapoya-16.ru/]наркологическая клиника[/url]
Наркологическая помощь на дому охватывает широкий спектр мероприятий, направленных на стабилизацию состояния пациента и лечение зависимости. Одним из ключевых направлений является детоксикация. Эта процедура позволяет очистить организм от токсичных веществ, улучшить общее самочувствие и восстановить работу внутренних органов.
Подробнее можно узнать тут – [url=https://narcolog-na-dom-ekaterinburg55.ru /]нарколог на дом екатеринбург[/url]
Вывод из запоя — одна из самых востребованных процедур. Этот процесс включает в себя не только медикаментозное вмешательство для устранения абстинентного синдрома, но и комплексную диагностику для выявления степени зависимости пациента. Врач может применить бензодиазепины для стабилизации нервной системы, а также витаминные комплексы для восстановления обменных процессов. Важно также обеспечить адекватное увлажнение организма, что поможет избежать обезвоживания и поддержит нормальное функционирование органов.
Узнать больше – [url=https://narcolog-na-dom-krasnoyarsk55.ru/]вызов нарколога на дом красноярск[/url]
В таких ситуациях вывод из запоя на дому становится оптимальным решением, особенно если пациент не может или не хочет посещать стационар. Эта услуга позволяет получить медицинскую помощь в комфортной и привычной обстановке, что снижает уровень стресса и ускоряет процесс восстановления.
Подробнее – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-anonimno-v-voronezhe/]anonimny vyvod iz zapoya na domu v-voronezhe[/url]
Стационарное лечение обеспечивает постоянный контроль медицинского персонала, что гарантирует максимальную безопасность и мониторинг состояния пациента. Это критически важно для пациентов с сопутствующими заболеваниями или осложнениями алкоголизма. Клиники также предоставляют дополнительные услуги: консультации психолога, групповые и индивидуальные терапевтические занятия, способствующие скорейшему выздоровлению и профилактике рецидивов.
Подробнее – [url=https://kapelnica-ot-zapoya-msk55.ru/]http://kapelnica-ot-zapoya-msk55.ru/kapelnicza-ot-zapoya-na-domu-moskva/[/url]
Стационарное лечение обеспечивает постоянный контроль медицинского персонала, что гарантирует максимальную безопасность и мониторинг состояния пациента. Это критически важно для пациентов с сопутствующими заболеваниями или осложнениями алкоголизма. Клиники также предоставляют дополнительные услуги: консультации психолога, групповые и индивидуальные терапевтические занятия, способствующие скорейшему выздоровлению и профилактике рецидивов.
Получить дополнительные сведения – [url=https://kapelnica-ot-zapoya-msk55.ru/]https://kapelnica-ot-zapoya-msk55.ru/kapelnicza-ot-zapoya-na-domu-moskva/[/url]
Этот вид услуги востребован благодаря своей оперативности, индивидуальному подходу и конфиденциальности. Вызов врача позволяет минимизировать стресс, обеспечить комфортное лечение и своевременно предотвратить возможные осложнения.
Исследовать вопрос подробнее – [url=https://narcolog-na-dom-ekaterinburg55.ru /]http://narcolog-na-dom-ekaterinburg55.ru/narkolog-na-dom-ekaterinburg-tseny/[/url]
Выведение из запоя – это процесс, включающий несколько этапов. Симптомы могут варьироваться от тревожности и бессонницы до серьезных психических расстройств. Только профессиональный подход и современные медицинские технологии помогают минимизировать риски, стабилизировать состояние и избежать осложнений.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]вывод из запоя с выездом цена в санкт-петербурге[/url]
Алкогольная зависимость представляет собой актуальную медицинскую проблему, требующую тщательного подхода. Запой характеризуется длительным, непрерывным употреблением спиртных напитков, что ведёт к физической и психической зависимости. На фоне запойного состояния могут возникнуть тяжёлые нарушения функций органов, увеличивается риск развития таких состояний, как алкогольный делирий. Вывод из запоя становится ключевым этапом в лечении зависимостей, который может осуществляться как в стационарных условиях, так и на дому, в зависимости от состояния пациента. В клинике «Перекресток Надежды» в Челябинске мы предлагаем круглосуточную помощь, чтобы обеспечить максимально эффективное и безопасное лечение при любом варианте вывода из запоя.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-17.ru/]наркологический центр в челябинске[/url]
Для достижения эффективных результатов в лечении запоя необходима квалифицированная помощь специалистов. Наркологическая клиника «Излечение» в Краснодаре предоставляет профессиональные услуги по выводу из запоя, гарантируя безопасность, комфорт и индивидуальный подход к каждому пациенту.
Углубиться в тему – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]наркология вывод из запоя анонимно [/url]
Особенно опасны длительные запои, когда человек в течение нескольких дней бесконтрольно употребляет алкоголь. Такое состояние разрушает организм, перегружает печень, сердце и нервную систему, может вызывать тяжелые осложнения, в том числе психические расстройства. Выйти из запоя самостоятельно практически невозможно и крайне рискованно. Важно своевременно обратиться за медицинской помощью, чтобы избежать серьезных последствий.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-14.ru/]http://vyvod-iz-zapoya-14.ru[/url]
Алкогольная зависимость — это тяжелое состояние, требующее срочного медицинского вмешательства. В столице растет число пациентов, предпочитающих получать медицинскую помощь в домашних условиях, и капельница от запоя на дому в Москве становится востребованным и результативным методом лечения. Данный подход позволяет эффективно восстановить организм пациента в привычной обстановке без необходимости пребывания в больнице.
Выяснить больше – [url=https://kapelnica-ot-zapoya-msk55.ru/]капельницу от запоя москва[/url]
Алкогольная зависимость — коварное заболевание, которое захватывает не только физическое здоровье, но и разрушает психику, лишает человека воли и заставляет его жить в плену у собственной зависимости. Запой — один из наиболее тяжелых периодов в жизни человека, столкнувшегося с алкоголизмом. Он характеризуется длительным и интенсивным употреблением спиртных напитков, приводящим к глубокой интоксикации организма, нарушению физиологических функций, психическим расстройствам, а также обострению сопутствующих заболеваний.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-16.ru/]https://www.vyvod-iz-zapoya-16.ru[/url]
Проблема алкогольной зависимости затрагивает значительное количество людей. Одним из действенных способов лечения интоксикации от алкоголя является внутривенная терапия. В Москве и области множество медицинских учреждений и независимых врачей предоставляют услуги детоксикации. Тем не менее, перед обращением за медицинской помощью необходимо разобраться, как формируется цена капельницы от из запоя в Москве, и к кому можно обратиться.
Детальнее – [url=https://kapelnica-ot-zapoya-msk55.ru/]http://kapelnica-ot-zapoya-msk55.ru[/url]
Запой – это состояние, которое возникает вследствие длительного употребления алкоголя, приводящее к потере контроля над количеством выпиваемого и формированию как физической, так и психологической зависимости. Оно сопровождается серьёзными последствиями для здоровья и требует немедленного вмешательства.
Выяснить больше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-na-domu-v-voronezhe/]vyvod iz zapoya na domu kruglosutochno v-voronezhe[/url]
Стационарное лечение обеспечивает постоянный контроль медицинского персонала, что гарантирует максимальную безопасность и мониторинг состояния пациента. Это критически важно для пациентов с сопутствующими заболеваниями или осложнениями алкоголизма. Клиники также предоставляют дополнительные услуги: консультации психолога, групповые и индивидуальные терапевтические занятия, способствующие скорейшему выздоровлению и профилактике рецидивов.
Подробнее можно узнать тут – [url=https://kapelnica-ot-zapoya-msk55.ru/]капельницы от запоя в москве[/url]
Looking for the ultimate online casino and sports betting experience? 1Win is your go-to platform for a world-class gambling adventure, offering a massive 500% welcome bonus on your first four deposits! With over 11,000+ games, lightning-fast withdrawals, and all major payment methods, 1Win ensures a smooth and rewarding betting experience for every player. Whether you’re a casino enthusiast or a sports betting fan, 1Win provides a diverse range of services, from slots, poker, and table games to live betting, esports, and more. The 1Win casino platform features an official website with a vast selection of slots, table games, and gaming machines, allowing players to enjoy high-quality entertainment with quick registration and seamless login. One of the key highlights of 1Win is its regular poker tournaments with a guaranteed prize pool of $1000, held every two days, providing bettors with a thrilling opportunity to win big. Sports betting lovers will appreciate 1Win Sports Betting, offering competitive odds across multiple sports, including football, basketball, tennis, and more. Whether you’re betting on local or international events, 1Win ensures a premium betting experience with a user-friendly interface and real-time updates. For players in Uganda, 1Win Uganda caters specifically to Ugandan bettors by accepting Ugandan shillings (UGX) and other foreign currencies, making deposits and withdrawals easy and convenient. Unlike other sportsbooks, 1Win stands out with its generous 500% deposit bonus, setting it apart as a top-tier betting site with higher percentage bonuses than most competitors. The platform is designed for both new and experienced players, featuring a working mirror link for easy access, along with 24/7 customer support to assist users at any time. Whether you’re looking to explore casino games, participate in poker tournaments, or bet on your favorite sports teams, 1Win is your trusted online betting destination. Sign up today and claim your 500% welcome bonus to start your winning journey with 1Win – the official online casino and sportsbook!
I consider, that you are mistaken. I can prove it. Write to me in PM, we will talk.
Алкогольная зависимость — это тяжелое расстройство, требующее профессиональной помощи и всестороннего лечения. Вывод из запоя в Красноярске в стационаре дает пациентам шанс освободиться от разрушительной зависимости под контролем высококвалифицированных врачей. Данный способ признан одним из наиболее результативных и надежных методов лечения.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]вывод из запоя красноярск[/url]
Алкогольная зависимость представляет собой серьезную медицинскую проблему, которая влечет за собой не только физические, но и психологические проблемы. Периоды запоя, характеризующиеся бесконтрольным употреблением алкоголя, могут привести к тяжелым последствиям. Симптомы абстиненции требуют незамедлительного вмешательства. Анонимность в процессе реабилитации становится важным аспектом, помогающим пациентам избежать стыда и социального осуждения.
Узнать больше – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя на дому [/url]
Этот формат позволяет пациентам получить профессиональную помощь в комфортной домашней обстановке. Такой подход не только обеспечивает удобство, но и гарантирует конфиденциальность, что особенно важно для многих людей.
Исследовать вопрос подробнее – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]выезд нарколога на дом цена в красноярске[/url]
Зависимость — это серьёзная проблема, которая затрагивает не только человека, но и его семью и окружение. Алкоголизм, наркомания и другие виды зависимостей могут разрушить жизнь, но с профессиональной помощью можно вернуть её в русло нормального функционирования. Наркологическая клиника «Заря Будущего» в Санкт-Петербурге предлагает уникальные программы лечения зависимостей, которые сочетает медико-психологический подход, индивидуальные методики и квалифицированную поддержку.
Подробнее тут – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]narkolog vyvod iz zapoya cena v-sankt-peterburge[/url]
Запойное состояние представляет собой серьёзную угрозу для здоровья, поскольку сопровождается тяжёлой интоксикацией организма и нарушением психоэмоционального состояния. Решение о лечении в стационаре клиники «Союз Здоровья» позволяет справиться с последствиями длительного употребления алкоголя, обеспечивая пациентам полный комплекс медицинской и психологической помощи.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-11.ru/]vyvod iz zapoya s vyezdom voronezh[/url]
Клиника «Освобождение» в Рязани предоставляет услугу круглосуточного вывода из запоя на дому, что позволяет пациенту получить медицинскую помощь в привычной обстановке, избегая лишнего стресса, связанного с госпитализацией.
Подробнее – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-v-kruglosutochno-v-ryazani/]вывод из запоя срочно круглосуточно рязань[/url]
Заключительная фаза реабилитации фокусируется на обучении пациента жизни без алкоголизма и развитии навыков преодоления жизненных трудностей естественным путем. В рамках этого этапа пациенты активно участвуют в специализированных программах, нацеленных на восстановление утраченных социальных связей и профессиональных компетенций. Программа включает трудотерапию, образовательные курсы и тематические семинары. Особое внимание уделяется работе с семьей: родственники проходят специальное обучение, помогающее им лучше понимать особенности зависимости быстро и эффективно оказывать поддержку близкому человеку.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]http://vyvod-iz-zapoya-krasnoyarsk55.ru[/url]
После того как специалист оценит состояние пациента, он подбирает индивидуальный состав капельницы. В стандартный раствор включаются витамины группы B и C, которые восстанавливают иммунную систему и уровень энергии, а также минералы, необходимые для нормализации сердечного ритма. В некоторых случаях добавляются седативные средства для устранения беспокойства и улучшения сна.
Детальнее – [url=https://kapelnica-ot-zapoya-ektb55.ru/]капельница от запоя на дому екатеринбург[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]наркология вывод из запоя анонимно санкт-петербург[/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Подробнее можно узнать тут – [url=https://vyvod-iz-zapoya-19.ru/]наркологический центр санкт-петербург[/url]
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Детальнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]вывод из запоя с выездом цена санкт-петербург[/url]
Зависимость — это серьёзная проблема, которая затрагивает не только человека, но и его семью и окружение. Алкоголизм, наркомания и другие виды зависимостей могут разрушить жизнь, но с профессиональной помощью можно вернуть её в русло нормального функционирования. Наркологическая клиника «Заря Будущего» в Санкт-Петербурге предлагает уникальные программы лечения зависимостей, которые сочетает медико-психологический подход, индивидуальные методики и квалифицированную поддержку.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]вывод из запоя срочно круглосуточно в санкт-петербурге[/url]
Алкогольная зависимость — комплексная проблема, которая нуждается в квалифицированном лечении. В Красноярске функционирует широкая сеть медицинских учреждений, готовых оказать поддержку тем, кто столкнулся с этой проблемой. Вывод из запоя в Красноярске осуществляется как в клинических условиях, так и при выезде специалистов на дом, что дает возможность подобрать оптимальный вариант лечения для каждого пациента.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]вывод из запоя на дому красноярск круглосуточно[/url]
selector casino вход
Запой представляет собой сложное состояние, связанное с длительным употреблением алкоголя, что приводит к формированию зависимости. Это явление не только нарушает нормальную жизнедеятельность, но также вызывает серьезные физические и психологические расстройства. Стационарное лечение становится необходимым для восстановления здоровья и профилактики осложнений. В стационаре клиники «Шаг к Трезвости» в Краснодаре мы предлагаем комплексный подход, включающий медикаментозное лечение, психотерапию и постоянный контроль со стороны квалифицированных специалистов.
Узнать больше – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя с выездом на дом [/url]
Зависимость — это серьёзная проблема, которая затрагивает не только человека, но и его семью и окружение. Алкоголизм, наркомания и другие виды зависимостей могут разрушить жизнь, но с профессиональной помощью можно вернуть её в русло нормального функционирования. Наркологическая клиника «Заря Будущего» в Санкт-Петербурге предлагает уникальные программы лечения зависимостей, которые сочетает медико-психологический подход, индивидуальные методики и квалифицированную поддержку.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]narkolog vyvod iz zapoya cena [/url]
Медикаментозное лечение — это еще один важный этап. Оно включает использование препаратов, которые помогают пациенту восстановиться как физически, так и психологически. Нарколог контролирует процесс лечения, чтобы исключить побочные эффекты и скорректировать терапию при необходимости.
Получить больше информации – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]вызов нарколога на дом красноярск[/url]
В клинике «Заря Будущего» мы понимаем, насколько важен своевременный и профессиональный подход к лечению зависимости. Наши специалисты готовы оказать квалифицированную помощь прямо у вас дома, обеспечивая полную безопасность и конфиденциальность на каждом этапе лечения.
Подробнее тут – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]нарколог на дом вывод из запоя санкт-петербург[/url]
селектор казино войти
Алкогольная зависимость представляет собой значимую проблему, требующую специализированного подхода. Запой — это состояние, характеризующееся длительным и непрерывным употреблением спиртных напитков, что приводит к формированию как физической, так и психической зависимости. На фоне запойного состояния часто наблюдаются нарушения работы внутренних органов, что может привести к тяжелым последствиям, включая алкогольный делирий. Вывод из запоя — важный этап в процессе лечения зависимости, и его можно осуществлять как в стационаре, так и на дому, в зависимости от конкретных обстоятельств.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya na domu kruglosutochno sankt-peterburg[/url]
селектор казино войти
Запой — это тяжелое состояние, при котором человек теряет контроль над количеством употребляемого алкоголя, что приводит к серьезным нарушениям здоровья. Процесс вывода из запоя требует профессионального подхода, медицинского контроля и тщательного восстановления организма. Наркологическая клиника «Шаг к Трезвости» в Краснодаре предлагает квалифицированную помощь для тех, кто нуждается в срочном и безопасном выходе из запоя. Мы предоставляем комплексное лечение, ориентированное на каждый этап восстановления, с максимальной заботой о здоровье пациента.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя с выездом на дом [/url]
Проведение лечения в домашней обстановке создает для пациента комфортные условия, что повышает эффективность реабилитации. Это уменьшает психологическую нагрузку, благоприятно влияя на самочувствие. Пациент может сохранять привычный режим дня, что способствует адаптации к трезвому образу жизни. Домашнее лечение позволяет реализовать персонализированный подход с учетом потребностей каждого пациента. Проведение лечения в домашней обстановке создает для пациента комфортные условия, что повышает эффективность реабилитации. Это уменьшает психологическую нагрузку, благоприятно влияя на самочувствие. Пациент может сохранять привычный режим дня, что способствует адаптации к трезвому образу жизни. Домашнее лечение позволяет реализовать персонализированный подход с учетом потребностей каждого пациента.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]вывод из запоя на дому красноярск цены[/url]
селектор казино зеркало
Медикаментозное лечение — это еще один важный этап. Оно включает использование препаратов, которые помогают пациенту восстановиться как физически, так и психологически. Нарколог контролирует процесс лечения, чтобы исключить побочные эффекты и скорректировать терапию при необходимости.
Изучить вопрос глубже – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]narcolog-na-dom-v-krasnoyarske55.ru/[/url]
Клиника «Преображение» предлагает услуги анонимного вывода из запоя в Екатеринбурге, обеспечивая высокое качество медицинской помощи и соблюдение всех этических норм.
Подробнее – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-anonimno-v-ekaterinburge/]анонимный вывод из запоя на дому [/url]
Отсутствует потребность в длительных поездках в медицинские учреждения или ожидании приема в специализированных центрах. Более того, стоимость домашнего лечения часто оказывается значительно ниже по сравнению с пребыванием в стационаре. Лечение на дому позволяет избежать дополнительных затрат на транспортировку и проживание в медицинских учреждениях. Это приобретает особую значимость для жителей удаленных районов, где доступ к стационарному лечению может быть существенно ограничен. Кроме того, получение медицинской помощи в домашних условиях дает возможность пациенту сохранить привычный рабочий график, что существенно снижает финансовые потери. Выезд специалиста предполагает удобные схемы оплаты и гибкие финансовые условия, делая услугу доступной для различных категорий населения.
Углубиться в тему – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]вывод из запоя красноярск[/url]
Запой – это состояние, возникающее из-за длительного употребления алкоголя и характеризующееся физической и психологической зависимостью. Оно требует комплексного медицинского вмешательства для устранения последствий и предотвращения дальнейшего ухудшения здоровья. Стационарное лечение в клинике «Освобождение» в Рязани – это эффективный способ вернуть пациента к нормальной жизни, предоставляя ему комфортные условия и круглосуточную помощь специалистов.
Разобраться лучше – [url=https://vyvod-iz-zapoya-13.ru/]http://www.vyvod-iz-zapoya-13.ru[/url]
Алкогольная зависимость — это серьезная проблема, требующая немедленного вмешательства. Если вы или ваши близкие столкнулись с запоем, важно получить профессиональную помощь как можно скорее, чтобы избежать ухудшения здоровья. Наркологическая клиника «Шаг к Трезвости» в Краснодаре предлагает услугу вывода из запоя на дому, что является удобным и эффективным решением для тех, кто не может или не хочет посещать стационар.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-cena-v-krasnodare/]вывод из запоя цена наркология [/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Детальнее – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-kruglosutochno-v-sankt-peterburge/]вывод из запоя круглосуточно в санкт-петербурге[/url]
Кроме того, врач оказывает психологическую поддержку, которая помогает пациенту справиться с чувством тревоги, депрессией и страхом, возникающими на фоне зависимости.
Углубиться в тему – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]выезд нарколога на дом цена красноярск[/url]
Клиника «Шаг к Трезвости» в Краснодаре предлагает анонимный вывод из запоя, обеспечивая полную конфиденциальность и безопасность на каждом этапе лечения. Мы понимаем, что для многих людей важно сохранить свою личную информацию в тайне, и гарантируем, что все данные о пациенте и его состоянии здоровья останутся закрытыми. Независимо от того, выбрал ли пациент стационарное лечение или вывод из запоя на дому, мы обеспечиваем высококачественную медицинскую помощь с полным соблюдением принципов конфиденциальности.
Подробнее тут – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]вывод из запоя срочно круглосуточно [/url]
Этот формат позволяет пациентам получить профессиональную помощь в комфортной домашней обстановке. Такой подход не только обеспечивает удобство, но и гарантирует конфиденциальность, что особенно важно для многих людей.
Подробнее – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]нарколог на дом красноярск[/url]
Алкогольный запой представляет собой крайне опасное состояние, характеризующееся неконтролируемым употреблением алкоголя, что в свою очередь приводит к тяжелой интоксикации организма. Восстановление после такого состояния требует профессионального вмешательства, включающего не только физическую детоксикацию, но и психологическую поддержку. Стоимость процедуры вывода из запоя может значительно различаться в зависимости от множества факторов, включая метод лечения, тяжесть состояния пациента и место проведения лечения. Рассмотрим более подробно основные аспекты, влияющие на цену вывода из запоя.
Узнать больше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]вывод из запоя цена наркология санкт-петербург[/url]
Алкогольная интоксикация, или запой, представляет собой крайне опасное состояние, при котором организм страдает от токсического воздействия алкоголя. Это ведет к нарушению нормальных физиологических процессов и ухудшению общего состояния здоровья. Капельница от запоя — это медицинская процедура, направленная на выведение токсинов из организма и восстановление нормальной функции организма при соблюдении полной конфиденциальности.
Разобраться лучше – [url=https://vyvod-iz-zapoya-21.ru/vivod-iz-zapoya-v-kruglosutochno-v-tveri/]vyvod iz zapoya kruglosutochno [/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьезное воздействие этанола, что приводит к нарушению нормальной физиологической деятельности. Это состояние сопровождается сильными физическими и психологическими расстройствами, которые требуют профессионального вмешательства. Однако многие люди, страдающие алкогольной зависимостью, сталкиваются с трудностью признания своей проблемы, опасаясь утраты репутации и конфиденциальности. В клинике «Заря Будущего» мы предлагаем анонимный вывод из запоя в Санкт-Петербурге, предоставляя высококачественное лечение с гарантией сохранения личной информации.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]анонимный вывод из запоя на дому санкт-петербург[/url]
Алкогольная зависимость представляет собой сложное и хроническое заболевание, которое влияет не только на физическое, но и на психологическое состояние пациента. Периоды запоя, влекущие за собой неконтролируемое употребление алкоголя, могут привести к опасным последствиям для здоровья и жизни. Абстинентные симптомы, такие как тремор, тошнота, головная боль и другие проявления, требуют немедленного медицинского вмешательства. В таких ситуациях важно не только быстро устранить физическое воздействие алкоголя, но и предоставить пациенту психологическую поддержку.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-cena-v-krasnodare/]вывод из запоя цена в краснодаре[/url]
Лечение зависимости требует комплексного подхода, сочетающего медицинские методы и психологическую поддержку. Это не просто вредная привычка, а хроническое заболевание, требующее тщательного контроля и длительной терапии. В центре используются современные научно обоснованные методики, помогающие пациентам преодолеть сложный путь выздоровления. Каждому человеку подбирается индивидуальная программа, учитывающая его особенности и жизненные обстоятельства.
Подробнее – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]вывод из запоя капельница на дому в санкт-петербурге[/url]
Врач-нарколог проводит комплексное обследование пациента, оценивая степень зависимости и общее состояние организма. В ходе первичного осмотра специалист не только определяет тяжесть алкогольной зависимости, но и выявляет сопутствующие патологии, которые могут повлиять на процесс лечения. Обследование включает лабораторные анализы, электрокардиограмму и другие необходимые диагностические процедуры. Врач тщательно изучает анамнез для выявления возможных противопоказаний к определенным методам терапии. Особое внимание уделяется психологическим аспектам, которые могли способствовать формированию зависимости, что помогает разработать эффективную программу лечения.
Детальнее – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]вывод из запоя красноярский край[/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьезное воздействие этанола, что приводит к нарушению нормальной физиологической деятельности. Это состояние сопровождается сильными физическими и психологическими расстройствами, которые требуют профессионального вмешательства. Однако многие люди, страдающие алкогольной зависимостью, сталкиваются с трудностью признания своей проблемы, опасаясь утраты репутации и конфиденциальности. В клинике «Заря Будущего» мы предлагаем анонимный вывод из запоя в Санкт-Петербурге, предоставляя высококачественное лечение с гарантией сохранения личной информации.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]vyvod iz zapoya cena sankt-peterburg[/url]
Алкогольная зависимость представляет собой серьезную медицинскую проблему, которая влечет за собой не только физические, но и психологические проблемы. Периоды запоя, характеризующиеся бесконтрольным употреблением алкоголя, могут привести к тяжелым последствиям. Симптомы абстиненции требуют незамедлительного вмешательства. Анонимность в процессе реабилитации становится важным аспектом, помогающим пациентам избежать стыда и социального осуждения.
Получить дополнительные сведения – [url=https://vyvod-iz-zapoya-12.ru/]наркологический центр краснодар[/url]
Алкоголизм — это хроническое заболевание, которое характеризуется патологической зависимостью от этанола. Периоды запоя, когда человек неконтролируемо потребляет алкоголь, могут привести к разнообразным медицинским осложнениям, как физическим, так и психологическим. Запой становится не только причиной ухудшения здоровья, но и причиной множества социальных и личных проблем. На этом фоне возникает необходимость вывода из запоя, процесс, который должен учитывать индивидуальные особенности пациента. Важно, что анонимность в реабилитации играет ключевую роль, помогая избежать стигматизации и увеличивая шансы на успешное восстановление.
Разобраться лучше – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-cena-v-ryazani/]вывод из запоя цена наркология в рязани[/url]
Медикаментозное лечение — это еще один важный этап. Оно включает использование препаратов, которые помогают пациенту восстановиться как физически, так и психологически. Нарколог контролирует процесс лечения, чтобы исключить побочные эффекты и скорректировать терапию при необходимости.
Получить дополнительные сведения – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]https://narcolog-na-dom-v-krasnoyarske55.ru/narkolog-na-dom-kruglosutochno-krasnoyarsk/[/url]
Многие пациенты интересуются возможностью быстрого вывода из запоя в стационаре Красноярска. Современные медицинские учреждения используют инновационные методики и препараты, существенно ускоряющие процесс выздоровления. Ключевые достоинства ускоренного лечения:
Подробнее тут – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]вывод из запоя цены красноярск[/url]
Алкогольный запой представляет собой серьезное состояние, которое требует немедленного медицинского вмешательства. Это состояние характеризуется длительным и бесконтрольным потреблением алкоголя, что приводит к разрушению как физического, так и психоэмоционального здоровья человека. Для эффективного и безопасного вывода из запоя стационарное лечение является оптимальным решением, особенно при тяжелых случаях интоксикации. В стационаре клиники «Заря Будущего» мы предлагаем комплексную программу вывода из запоя, которая включает детоксикацию, восстановление организма и психотерапевтическую помощь.
Разобраться лучше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]vyvod iz zapoya cena sankt-peterburg[/url]
Алкогольная зависимость представляет собой серьёзную медицинскую проблему, требующую специализированного подхода. Запойное состояние характеризуется длительным и бесконтрольным потреблением спиртных напитков, что приводит к возникновению как физической, так и психической зависимости. Данное явление может вызвать нарушения в работе внутренних органов, значительно увеличивая вероятность развития алкогольного делирия, который представляет собой острое состояние, требующее неотложной медицинской помощи. Вывод из запоя это ключевая часть лечения зависимости, которая может осуществляться как в стационаре, так и в амбулаторных условиях, в зависимости от конкретной ситуации и состояния пациента.
Углубиться в тему – [url=https://vyvod-iz-zapoya-12.ru/]вывод из запоя с выездом в краснодаре[/url]
Этот формат позволяет пациентам получить профессиональную помощь в комфортной домашней обстановке. Такой подход не только обеспечивает удобство, но и гарантирует конфиденциальность, что особенно важно для многих людей.
Получить дополнительные сведения – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]https://narcolog-na-dom-v-krasnoyarske55.ru[/url]
Алкогольная зависимость представляет собой серьезную медицинскую проблему, которая влечет за собой не только физические, но и психологические проблемы. Периоды запоя, характеризующиеся бесконтрольным употреблением алкоголя, могут привести к тяжелым последствиям. Симптомы абстиненции требуют незамедлительного вмешательства. Анонимность в процессе реабилитации становится важным аспектом, помогающим пациентам избежать стыда и социального осуждения.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя на дому недорого капельница [/url]
1Win е модерна и иновативна платформа за онлайн казино и спортни залагания, която предлага богат избор от игри и атрактивни бонуси за нови и съществуващи играчи. Сайтът 1Win България осигурява лесна и бърза регистрация, позволявайки на потребителите да се регистрират с един клик, чрез телефон или e-mail, както и чрез социални мрежи. Новите играчи получават щедър бонус за регистрация – 500% от първите четири депозита, което прави 1Win едно от най-добрите онлайн казина по отношение на бонуси и промоции. Освен това, 1Win предоставя удобен достъп до спортни залагания с високи коефициенти, където потребителите могат да правят залози на различни спортове като футбол, тенис, баскетбол и много други. Платформата разполага с богато казино с хиляди слотове, игри на маса, видео покер, както и вълнуващи джакпоти, които осигуряват огромни печалби. 1Win е лицензирано казино, притежаващо лиценз от Кюрасао, което гарантира сигурност и надеждност за потребителите. За допълнително удобство 1Win предлага мобилно приложение за Android и iOS, което позволява на играчите да се наслаждават на любимите си игри и спортни залагания навсякъде и по всяко време. Депозирането и тегленето на средства в 1Win Casino е лесно благодарение на множеството налични методи за плащане, включително кредитни и дебитни карти, електронни портфейли и дори криптовалути. Платформата предлага и официално работещо огледало (mirror), което позволява на потребителите да влязат в акаунта си дори при ограничения в някои държави. Освен стандартните казино игри, 1Win предлага и стрийминг на филми, което я прави уникална сред другите онлайн платформи за залагания. При проблеми с тегленето на средства, екипът за поддръжка на 1Win е на разположение денонощно, за да помогне на клиентите бързо и ефективно. За да се регистрирате в 1Win и да започнете своето приключение, просто посетете официалния сайт, създайте акаунт и направете първия си депозит, за да получите бонусите си. С атрактивните си промоции, разнообразието от казино игри и спортни залози, удобните методи за плащане и мобилното приложение, 1Win се превръща в един от водещите сайтове за онлайн залози, достъпен в България и по целия свят. Независимо дали сте любител на казино игрите или спортните залагания, 1Win предлага всичко необходимо за едно незабравимо игрово изживяване.
I apologise, but this variant does not approach me. Who else, what can prompt?
Кроме того, врач оказывает психологическую поддержку, которая помогает пациенту справиться с чувством тревоги, депрессией и страхом, возникающими на фоне зависимости.
Детальнее – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]https://narcolog-na-dom-v-krasnoyarske55.ru[/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-17.ru/]vyvod iz zapoya kapelnica[/url]
В клинике работает команда квалифицированных специалистов, которые осуществляют вывод из запоя, обеспечивая полный контроль над состоянием пациента и устраняя все последствия длительного употребления алкоголя.
Углубиться в тему – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-v-stacionare-v-ekaterinburge/]vyvod iz zapoya v stacionare ekaterinburg[/url]
Клиника «Освобождение» в Рязани предоставляет услугу круглосуточного вывода из запоя на дому, что позволяет пациенту получить медицинскую помощь в привычной обстановке, избегая лишнего стресса, связанного с госпитализацией.
Углубиться в тему – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-cena-v-ryazani/]вывод из запоя с выездом цена в рязани[/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над употреблением спиртных напитков, что приводит к токсической нагрузке на организм. Это опасное состояние требует не только быстрого медицинского вмешательства, но и внимательного подхода к восстановлению здоровья пациента. Процесс вывода из запоя включает в себя комплекс процедур, направленных на устранение последствий интоксикации и восстановление нормального функционирования организма. Важно учитывать, что стоимость этой процедуры может значительно варьироваться в зависимости от нескольких факторов.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-cena-v-krasnodare/]vyvod iz zapoya cena v-krasnodare[/url]
Многие пациенты интересуются возможностью быстрого вывода из запоя в стационаре Красноярска. Современные медицинские учреждения используют инновационные методики и препараты, существенно ускоряющие процесс выздоровления. Ключевые достоинства ускоренного лечения:
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]вывод из запоя в красноярске[/url]
Наркологическая клиника «Союз Здоровья» в Воронеже специализируется на лечении алкогольной зависимости и предлагает профессиональные услуги по выводу из запоя. Мы стремимся создать атмосферу доверия, где каждый пациент сможет получить необходимую поддержку.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-11.ru/]narkologicheskij centr v-voronezhe[/url]
Алкоголизм — это хроническое заболевание, которое характеризуется патологической зависимостью от этанола. Периоды запоя, когда человек неконтролируемо потребляет алкоголь, могут привести к разнообразным медицинским осложнениям, как физическим, так и психологическим. Запой становится не только причиной ухудшения здоровья, но и причиной множества социальных и личных проблем. На этом фоне возникает необходимость вывода из запоя, процесс, который должен учитывать индивидуальные особенности пациента. Важно, что анонимность в реабилитации играет ключевую роль, помогая избежать стигматизации и увеличивая шансы на успешное восстановление.
Узнать больше – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-cena-v-ryazani/]вывод из запоя на дому цена [/url]
В клинике «Заря Будущего» мы понимаем, насколько важен своевременный и профессиональный подход к лечению зависимости. Наши специалисты готовы оказать квалифицированную помощь прямо у вас дома, обеспечивая полную безопасность и конфиденциальность на каждом этапе лечения.
Выяснить больше – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-na-domu-v-sankt-peterburge/]vyvod iz zapoya kapelnica na domu [/url]
мтс подключить екатеринбург
https://cleveran.com/profile/aracelismontoy
провайдер мтс
Алкогольный запой представляет собой серьезное состояние, которое требует немедленного медицинского вмешательства. Это состояние характеризуется длительным и бесконтрольным потреблением алкоголя, что приводит к разрушению как физического, так и психоэмоционального здоровья человека. Для эффективного и безопасного вывода из запоя стационарное лечение является оптимальным решением, особенно при тяжелых случаях интоксикации. В стационаре клиники «Заря Будущего» мы предлагаем комплексную программу вывода из запоя, которая включает детоксикацию, восстановление организма и психотерапевтическую помощь.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-19.ru/]narkologicheskaya klinika[/url]
Одним из важнейших аспектов, который помогает пациентам справиться с алкогольной зависимостью, является анонимность в процессе лечения. В клинике «Излечение» мы понимаем, как важно сохранить конфиденциальность и гарантировать защиту личной информации, особенно для тех, кто опасается общественного осуждения. Мы обеспечиваем полную анонимность при выводе из запоя, как в стационарных условиях, так и на дому.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]вывод из запоя анонимно недорого [/url]
find out here [url=https://valorantskinchanger.pro/]skin changer valorant[/url]
Процесс выведения из запоя на дому состоит из нескольких последовательных этапов.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]вывод из запоя на дому в красноярске[/url]
Клиника «Шаг к Трезвости» в Краснодаре предлагает анонимный вывод из запоя, обеспечивая полную конфиденциальность и безопасность на каждом этапе лечения. Мы понимаем, что для многих людей важно сохранить свою личную информацию в тайне, и гарантируем, что все данные о пациенте и его состоянии здоровья останутся закрытыми. Независимо от того, выбрал ли пациент стационарное лечение или вывод из запоя на дому, мы обеспечиваем высококачественную медицинскую помощь с полным соблюдением принципов конфиденциальности.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-cena-v-krasnodare/]vyvod iz zapoya cena v-krasnodare[/url]
Современная жизнь, особенно в крупном городе, как Красноярск, часто становится причиной различных зависимостей. Работа в условиях постоянного стресса, высокий ритм жизни и эмоциональные перегрузки могут привести к проблемам, которые требуют неотложной медицинской помощи. Одним из наиболее удобных решений в такой ситуации является вызов нарколога на дом.
Получить больше информации – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]https://narcolog-na-dom-v-krasnoyarske55.ru[/url]
Для достижения эффективных результатов в лечении запоя необходима квалифицированная помощь специалистов. Наркологическая клиника «Излечение» в Краснодаре предоставляет профессиональные услуги по выводу из запоя, гарантируя безопасность, комфорт и индивидуальный подход к каждому пациенту.
Углубиться в тему – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-stacionare-v-krasnodare/]http://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-stacionare-v-krasnodare/[/url]
В этой статье мы расскажем о возможностях клиники для лечения алкогольной интоксикации круглосуточно, включая вывод из запоя в стационаре и на дому, а также о роли профессионалов, таких как нарколог, в процессе лечения.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-16.ru/]наркологический центр краснодар[/url]
Зависимость — это серьёзная проблема, которая затрагивает не только человека, но и его семью и окружение. Алкоголизм, наркомания и другие виды зависимостей могут разрушить жизнь, но с профессиональной помощью можно вернуть её в русло нормального функционирования. Наркологическая клиника «Заря Будущего» в Санкт-Петербурге предлагает уникальные программы лечения зависимостей, которые сочетает медико-психологический подход, индивидуальные методики и квалифицированную поддержку.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-19.ru/]narkologicheskij centr v-sankt-peterburge[/url]
Алкогольная зависимость представляет собой серьёзную медицинскую проблему, требующую специализированного подхода. Запойное состояние характеризуется длительным и бесконтрольным потреблением спиртных напитков, что приводит к возникновению как физической, так и психической зависимости. Данное явление может вызвать нарушения в работе внутренних органов, значительно увеличивая вероятность развития алкогольного делирия, который представляет собой острое состояние, требующее неотложной медицинской помощи. Вывод из запоя это ключевая часть лечения зависимости, которая может осуществляться как в стационаре, так и в амбулаторных условиях, в зависимости от конкретной ситуации и состояния пациента.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-v-stacionare-v-krasnodare/]vyvod iz zapoya v stacionare anonimno krasnodar[/url]
В клинике работает команда квалифицированных специалистов, которые осуществляют вывод из запоя, обеспечивая полный контроль над состоянием пациента и устраняя все последствия длительного употребления алкоголя.
Углубиться в тему – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]vyvod iz zapoya kapelnica na domu ekaterinburg[/url]
Врач-нарколог проводит комплексное обследование пациента, оценивая степень зависимости и общее состояние организма. В ходе первичного осмотра специалист не только определяет тяжесть алкогольной зависимости, но и выявляет сопутствующие патологии, которые могут повлиять на процесс лечения. Обследование включает лабораторные анализы, электрокардиограмму и другие необходимые диагностические процедуры. Врач тщательно изучает анамнез для выявления возможных противопоказаний к определенным методам терапии. Особое внимание уделяется психологическим аспектам, которые могли способствовать формированию зависимости, что помогает разработать эффективную программу лечения.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]вывод из запоя на дому красноярск круглосуточно[/url]
Современная жизнь, особенно в крупном городе, как Красноярск, часто становится причиной различных зависимостей. Работа в условиях постоянного стресса, высокий ритм жизни и эмоциональные перегрузки могут привести к проблемам, которые требуют неотложной медицинской помощи. Одним из наиболее удобных решений в такой ситуации является вызов нарколога на дом.
Узнать больше – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]https://narcolog-na-dom-v-krasnoyarske55.ru/[/url]
В центре доступны различные варианты лечения – от амбулаторного наблюдения до интенсивных стационарных программ. Ключевые принципы работы – конфиденциальность, индивидуальный подход и оперативность оказания помощи. Независимо от тяжести зависимости, каждый пациент получает квалифицированную поддержку, направленную на восстановление здоровья и возвращение к полноценной жизни.
Детальнее – [url=https://vyvod-iz-zapoya-14.ru/]вывод из запоя санкт-петербург[/url]
Алкогольная зависимость представляет собой серьезную медицинскую проблему, которая влечет за собой не только физические, но и психологические проблемы. Периоды запоя, характеризующиеся бесконтрольным употреблением алкоголя, могут привести к тяжелым последствиям. Симптомы абстиненции требуют незамедлительного вмешательства. Анонимность в процессе реабилитации становится важным аспектом, помогающим пациентам избежать стыда и социального осуждения.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]вывод из запоя круглосуточно наркология в краснодаре[/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Подробнее – [url=https://vyvod-iz-zapoya-11.ru/]vyvod iz zapoya s vyezdom voronezh[/url]
Кроме того, врач оказывает психологическую поддержку, которая помогает пациенту справиться с чувством тревоги, депрессией и страхом, возникающими на фоне зависимости.
Получить дополнительную информацию – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]http://narcolog-na-dom-v-krasnoyarske55.ru/narkolog-na-dom-krasnoyarsk-tseny/[/url]
Врач-нарколог проводит комплексное обследование пациента, оценивая степень зависимости и общее состояние организма. В ходе первичного осмотра специалист не только определяет тяжесть алкогольной зависимости, но и выявляет сопутствующие патологии, которые могут повлиять на процесс лечения. Обследование включает лабораторные анализы, электрокардиограмму и другие необходимые диагностические процедуры. Врач тщательно изучает анамнез для выявления возможных противопоказаний к определенным методам терапии. Особое внимание уделяется психологическим аспектам, которые могли способствовать формированию зависимости, что помогает разработать эффективную программу лечения.
Подробнее – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]вывод из запоя красноярск[/url]
Вывод из запоя представляет собой важный этап в процессе лечения алкогольной зависимости. Это медицинская процедура, необходимая для устранения токсических веществ, накопленных в организме в результате длительного употребления алкоголя. Алкогольная зависимость может вызывать множество проблем, включая физические и психоэмоциональные расстройства. Своевременное вмешательство специалистов помогает минимизировать риски и способствует восстановлению здоровья пациента.
Подробнее тут – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]вывод из запоя вызов на дом в рязани[/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-11.ru/]narkologicheskij centr voronezh[/url]
Одним из важнейших аспектов, который помогает пациентам справиться с алкогольной зависимостью, является анонимность в процессе лечения. В клинике «Излечение» мы понимаем, как важно сохранить конфиденциальность и гарантировать защиту личной информации, особенно для тех, кто опасается общественного осуждения. Мы обеспечиваем полную анонимность при выводе из запоя, как в стационарных условиях, так и на дому.
Детальнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/]vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-v-kruglosutochno-v-krasnodare/[/url]
Алкогольная зависимость требует быстрого и квалифицированного вмешательства, поскольку она может привести к тяжелым последствиям для здоровья. Капельница от запоя — один из наиболее эффективных методов, помогающих вывести организм из состояния алкогольной интоксикации и восстановить нормальное самочувствие. В Екатеринбурге эта процедура доступна как в медицинских учреждениях, так и на дому, что позволяет пациентам выбрать наиболее удобный и комфортный способ лечения.
Детальнее – [url=https://kapelnica-ot-zapoya-ektb55.ru/]капельницы от запояекатеринбург[/url]
Запой — это опасное состояние, которое развивается у людей с алкогольной зависимостью и характеризуется длительным непрерывным потреблением алкоголя. Это состояние приводит к серьезным нарушениям в организме, как физическим, так и психоэмоциональным. Длительный запой может вызвать множество осложнений, таких как поражение внутренних органов, нервной системы, а также проблемы в социальной и семейной жизни. Однако современная медицина предоставляет эффективные методы вывода из запоя, и одним из наиболее удобных и востребованных является вывод из запоя на дому.
Углубиться в тему – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-v-stacionare-v-sankt-peterburge/]вывод из запоя в стационаре в санкт-петербурге[/url]
В клинике «Излечение» мы предлагаем широкий спектр услуг по выводу из запоя, включая как стационарное лечение, так и помощь на дому. В этой статье мы подробно рассмотрим, от чего зависит цена вывода из запоя и какие услуги входят в стоимость.
Изучить вопрос глубже – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]http://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/[/url]
Запой – это тяжелое состояние, возникающее из-за длительного и неконтролируемого употребления алкоголя. Оно характеризуется физической и психологической зависимостью, что приводит к серьезным последствиям для организма и психики. Для восстановления нормального самочувствия и здоровья необходимо профессиональное лечение. Одним из наиболее удобных и эффективных методов является вывод из запоя на дому.
Детальнее – [url=https://vyvod-iz-zapoya-13.ru/vivod-iz-zapoya-na-domu-v-ryazani/]srochny vyvod iz zapoya na domu [/url]
Круглосуточная медицинская помощь при алкогольном запое играет важнейшую роль в спасении жизни пациентов и их возвращении к нормальному состоянию. В данной статье рассмотрим ключевые аспекты вывода из запоя в домашних условиях, срочной помощи и работы нарколога.
Получить больше информации – [url=https://vyvod-iz-zapoya-20.ru/]narkologicheskaya klinika ekaterinburg[/url]
В медицинском центре «Второй Шанс» помощь оказывается круглосуточно. Применяются проверенные и эффективные методы, обеспечивающие безопасность пациента и устойчивый результат. Конфиденциальность гарантирует комфорт и спокойствие тем, кто обратился за поддержкой, позволяя сосредоточиться на выздоровлении.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-14.ru/]вывод из запоя с выездом в санкт-петербурге[/url]
В центре доступны различные варианты лечения – от амбулаторного наблюдения до интенсивных стационарных программ. Ключевые принципы работы – конфиденциальность, индивидуальный подход и оперативность оказания помощи. Независимо от тяжести зависимости, каждый пациент получает квалифицированную поддержку, направленную на восстановление здоровья и возвращение к полноценной жизни.
Углубиться в тему – [url=https://vyvod-iz-zapoya-14.ru/vivod-iz-zapoya-anonimno-v-sankt-peterburge/]narkologiya vyvod iz zapoya anonimno sankt-peterburg[/url]
Выход из запоя — это важнейший этап на пути к избавлению от алкогольной зависимости. Он требует комплексного подхода, профессиональной помощи и сильной мотивации пациента. В условиях, когда анонимность и комфорт становятся ключевыми факторами для многих людей, вывод из запоя на дому приобретает все большее значение. Это решение позволяет человеку получить необходимую помощь, не покидая дома, и избежать стресса, связанного с госпитализацией.
Детальнее – [url=https://vyvod-iz-zapoya-16.ru/vivod-iz-zapoya-anonimno-v-krasnodare/]vyvod iz zapoya anonimno nedorogo v-krasnodare[/url]
Запой — это одно из наиболее тяжелых проявлений алкогольной зависимости, которое требует немедленного и квалифицированного вмешательства. В такие моменты близкие зачастую теряются и не знают, как помочь своему родному человеку. На помощь приходит наркологическая клиника «Перекресток Надежды» в Челябинске, которая предоставляет профессиональную помощь в выводе из запоя. Мы понимаем, что лечение запоя требует не только медицинского подхода, но и психологической поддержки. Именно поэтому в нашей клинике пациент получает комплексную помощь, основанную на самых современных методах лечения.
Детальнее – [url=https://vyvod-iz-zapoya-17.ru/vivod-iz-zapoya-cena-v-chelyabinske/]нарколог вывод из запоя цена челябинск[/url]
Многие пациенты интересуются возможностью быстрого вывода из запоя в стационаре Красноярска. Современные медицинские учреждения используют инновационные методики и препараты, существенно ускоряющие процесс выздоровления. Ключевые достоинства ускоренного лечения:
Исследовать вопрос подробнее – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]https://vyvod-iz-zapoya-krasnoyarsk55.ru/vyvod-iz-zapoya-krasnoyarsk-kruglosutochno/[/url]
Врач-нарколог проводит комплексное обследование пациента, оценивая степень зависимости и общее состояние организма. В ходе первичного осмотра специалист не только определяет тяжесть алкогольной зависимости, но и выявляет сопутствующие патологии, которые могут повлиять на процесс лечения. Обследование включает лабораторные анализы, электрокардиограмму и другие необходимые диагностические процедуры. Врач тщательно изучает анамнез для выявления возможных противопоказаний к определенным методам терапии. Особое внимание уделяется психологическим аспектам, которые могли способствовать формированию зависимости, что помогает разработать эффективную программу лечения.
Ознакомиться с деталями – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]вывод из запоя красноярск[/url]
Клиника «Шаг к Трезвости» в Краснодаре предлагает анонимный вывод из запоя, обеспечивая полную конфиденциальность и безопасность на каждом этапе лечения. Мы понимаем, что для многих людей важно сохранить свою личную информацию в тайне, и гарантируем, что все данные о пациенте и его состоянии здоровья останутся закрытыми. Независимо от того, выбрал ли пациент стационарное лечение или вывод из запоя на дому, мы обеспечиваем высококачественную медицинскую помощь с полным соблюдением принципов конфиденциальности.
Подробнее тут – [url=https://vyvod-iz-zapoya-12.ru/vivod-iz-zapoya-na-domu-v-krasnodare/]вывод из запоя с выездом на дом в краснодаре[/url]
Врач-нарколог проводит комплексное обследование пациента, оценивая степень зависимости и общее состояние организма. В ходе первичного осмотра специалист не только определяет тяжесть алкогольной зависимости, но и выявляет сопутствующие патологии, которые могут повлиять на процесс лечения. Обследование включает лабораторные анализы, электрокардиограмму и другие необходимые диагностические процедуры. Врач тщательно изучает анамнез для выявления возможных противопоказаний к определенным методам терапии. Особое внимание уделяется психологическим аспектам, которые могли способствовать формированию зависимости, что помогает разработать эффективную программу лечения.
Углубиться в тему – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]вывод из запоя цены красноярск[/url]
Алкогольный запой – это серьёзное состояние, при котором человек теряет контроль над употреблением алкоголя, что приводит к тяжёлой интоксикации организма. Во время запоя нарушаются функции внутренних органов, страдает нервная система, и повышается риск развития опасных осложнений, таких как алкогольный делирий. Это состояние требует незамедлительного медицинского вмешательства.
Узнать больше – [url=https://vyvod-iz-zapoya-20.ru/vivod-iz-zapoya-na-domu-v-ekaterinburge/]vyvod iz zapoya vrach na dom ekaterinburg[/url]
Алкогольный запой — это состояние, при котором человек теряет контроль над своим потреблением алкоголя, что может вызвать серьезные физические и психологические проблемы. В таких ситуациях крайне важно получить квалифицированную медицинскую помощь как можно скорее. Однако многие люди опасаются обращаться за помощью, переживая за свою репутацию и возможное раскрытие личной информации. В клинике «Союз Здоровья» в Воронеже мы гарантируем анонимность на всех этапах лечения, сохраняя высокий уровень медицинской помощи и заботясь о каждом пациенте.
Разобраться лучше – [url=https://vyvod-iz-zapoya-11.ru/vivod-iz-zapoya-cena-v-voronezhe/]vyvod iz zapoya cena [/url]
Алкогольная интоксикация, или запой, представляет собой состояние, при котором организм человека испытывает серьезное воздействие этанола, что приводит к нарушению нормальной физиологической деятельности. Это состояние сопровождается сильными физическими и психологическими расстройствами, которые требуют профессионального вмешательства. Однако многие люди, страдающие алкогольной зависимостью, сталкиваются с трудностью признания своей проблемы, опасаясь утраты репутации и конфиденциальности. В клинике «Заря Будущего» мы предлагаем анонимный вывод из запоя в Санкт-Петербурге, предоставляя высококачественное лечение с гарантией сохранения личной информации.
Получить больше информации – [url=https://vyvod-iz-zapoya-19.ru/vivod-iz-zapoya-cena-v-sankt-peterburge/]vyvod iz zapoya cena narkologiya sankt-peterburg[/url]
либет казино вход
Кроме того, врач оказывает психологическую поддержку, которая помогает пациенту справиться с чувством тревоги, депрессией и страхом, возникающими на фоне зависимости.
Подробнее можно узнать тут – [url=https://narcolog-na-dom-v-krasnoyarske55.ru/]нарколог на дом недорого в красноярске[/url]
Запой — это серьезное состояние, требующее немедленного вмешательства профессионалов. В Красноярске вывод из запоя доступен круглосуточно, обеспечивая пациентам возможность получить помощь в любое время дня и ночи. Современные наркологические клиники предлагают как стационарное, так и амбулаторное лечение, включая выезд специалистов на дом. В статье рассмотрим основные аспекты получения услуги, ее стоимость и преимущества.
Получить дополнительную информацию – [url=https://vyvod-iz-zapoya-krasnoyarsk55.ru/]вывод из запоя в красноярске[/url]
leebet casino вход
Для тех, кто не может приехать в клинику, специалисты проводят детоксикацию на дому. С помощью современных препаратов организм мягко очищается от токсинов, уменьшается нагрузка на внутренние органы, снимаются неприятные симптомы абстиненции. Однако при тяжелом состоянии или наличии осложнений может потребоваться круглосуточное наблюдение в стационаре, где пациенту обеспечивается полная медицинская поддержка.
Углубиться в тему – [url=https://vyvod-iz-zapoya-14.ru/]вывод из запоя[/url]
мтс интернет екатеринбург
https://thematragroup.in/employer/inetrnet-domashnij-ekb-3/
мтс телевидение
leebet casino сайт
Программы лечения разрабатываются индивидуально и могут включать как визит врача на дом, так и стационарное пребывание. Стоимость услуг формируется в зависимости от необходимых процедур и остается прозрачной, позволяя пациентам сосредоточиться на главном – восстановлении здоровья и возвращении к полноценной жизни.
Подробнее тут – [url=https://vyvod-iz-zapoya-14.ru/]narkologicheskaya klinika[/url]
MachFi: Revolutionizing DeFi with Sonic Chain.
MachFi is leading the way in decentralized finance (DeFi), offering a next-gen borrow-lending platform on the Sonic Chain. Our platform supports customizable trading strategies, giving users more control over their assets in a secure, decentralized ecosystem. visit to https://machfi.net/
Why Choose MachFi?
– Decentralized: Powered by the Sonic Chain for transparency and security.
– Flexible Borrow-Lending: Tailored to your financial goals with custom trading strategies.
– Innovative Technology: Harness the power of the latest blockchain technology to maximize yields.
Start your journey with MachFi today and experience the future of DeFi!
мтс домашний интернет
http://gsrl.uk/employer/inetrnet-domashnij-ekb-3/
мтс тарифы екатеринбург
Верните четкость зрения с помощью [url=https://exci.ru/]лазерная коррекция[/url]. Инновационные методики гарантируют стабильный результат уже после первой процедуры.
Центр «ЭКСИ» в Ижевске специализируется на высокотехнологичных методах лечения зрения, таких как лазерная коагуляция сетчатки, факоэмульсификация и другие процедуры. Пациенты из разных регионов отмечают высокий уровень сервиса и профессионализм врачей. Записаться на прием можно через контактный центр по номерам: +7(3412)68-27-50, +7(3412)68-78-75. Расположен центр по адресу: г. Ижевск, улица Ленина, 101.
Unlock New Opportunities with MachFi.
MachFi is at the forefront of decentralized finance on the Sonic Chain, providing an advanced borrow-lending platform. Our platform supports custom trading strategies, helping you unlock the full potential of your digital assets in a decentralized environment. visit to https://machfi.net/
Key Features of MachFi:
– Sonic Chain: Fast, secure, and reliable blockchain for DeFi transactions.
– Customizable Lending: Choose strategies that work best for you.
– Higher Returns: Capitalize on innovative DeFi solutions for superior returns.
Join MachFi now and redefine your digital financial strategy!
мтс подключить
https://centerfairstaffing.com/employer/inetrnet-domashnij-ekb-3/
мтс тарифы екатеринбург
[url=http://lordfilm-w.com/serialy/]онлайн сериалы бесплатно[/url] – фильмы онлайн, фильмы вышедшие онлайн
Купить водительские права
свечи бетадин при гв [url=https://svechi-dlya-zhenshchin.ru/]https://svechi-dlya-zhenshchin.ru/[/url] .
мтс цены
https://jobportal.kernel.sa/employer/inetrnet-domashnij-ekb-3/
мтс подключение екатеринбург
мтс тарифы на интернет
https://dev-members.writeappreviews.com/employer/inetrnet-domashnij-ekb/
мтс домашний интернет
Купить водительские права
[url=https://kra27.ca/]kraken[/url] – кракен рутор, kraken com отзывы
мтс интернет
https://jobs.fabumama.com/employer/inetrnet-domashnij-ekb-3/
мтс интернет екатеринбург
Организуйте приятный сюрприз! [url=https://thegreen.ru/]цветы доставка[/url] работает круглосуточно, чтобы ваши поздравления всегда доходили вовремя. Профессиональные флористы создают уникальные композиции, а курьеры доставляют их точно по адресу.
Thegreen.ru – это современный цветочный магазин в Москве, где каждый может найти идеальный букет. Расположенный по адресу улица Юннатов, дом 4кА, мы предлагаем профессиональную доставку цветов. Для заказа свяжитесь с нами по телефону +7(495)144-15-24.
мтс тарифы на интернет
https://optimaplacement.com/companies/inetrnet-domashnij-ekb-2/
провайдер мтс
мегафон тв
https://ekb-domasnij-internet-2.ru
мегафон подключить
[url=https://vk.com/podslushanomoskwa]Интересная Москва[/url] – Моя квартира Москва, Моя Москва
мегафон подключение екатеринбург
https://ekb-domasnij-internet-3.ru
мегафон екатеринбург
Нужны деньги прямо сейчас? Оформите [url=https://telegra.ph/Zajm-na-kartu-onlajn—poluchite-zajm-onlajn-ot-luchshih-MFO-02-15]онлайн займы на карту[/url] за пару минут без лишних документов. Сервис работает круглосуточно, а средства поступают мгновенно.
мегафон екатеринбург
https://ekb-domasnij-internet.ru
мегафон подключение екатеринбург
билайн подключение краснодар
https://infodomashnij-internet-krasnodar-2.ru
билайн подключение
Срочная потребность в деньгах больше не станет проблемой. Наши срочные займы без отказов на карту помогут вам быстро решить финансовые вопросы. Мы работаем без выходных и праздников, поэтому вы можете обратиться к нам в любое время. Заполните форму на сайте, и уже через 10 минут деньги будут на вашей карте. Удобство, скорость и надежность — главные преимущества нашего сервиса. Узнать подробнее можно тут: [url=https://image.google.co.ug/url?q=https://mikro-zaim-online.ru/]займы на карту онлайн[/url] .
[url=https://kra27.ca]kraken ссылка зеркало[/url] – кракен онион, кракен
билайн цены
https://infodomashnij-internet-krasnodar-3.ru
билайн тарифы краснодар
билайн интернет краснодар
https://infodomashnij-internet-krasnodar.ru
билайн тв
каталог [url=https://maralisa.ru]стулья из искусственного ротанга[/url]
find more info
[url=https://337store.pro/]Unemployed[/url]
https://dubllikat.ru/
нажмите здесь [url=https://bmoldova.bz/]Молдова[/url]
[url=https://vodkabet.io/]vodkabet[/url] – водкабет официальный сайт, казино водка
билайн телевидение
https://plus-domashnij-internet-krasnodar-2.ru
билайн тарифы на интернет
Превърнете вградената си кухня в идеалното място за готвене, Как да преобразите вградения си шкаф с минимални усилия, Как да направите вградената си етажерка стилна и функционална, иновативни начини за подобряване на вградената стена в дома ви, най-добрият начин да превърнете вградения гардероб в гардеробна стая, Най-добрите 20 идеи за подобряване на вградената работна зона, преобразете вградената си всекидневна с тези съвети, Превърнете вградените шкафове на балкона в място за съхранение, идеално за лятото, Съвети за подобряване на вградената трапезария, най-добрите идеи за декориране на вградения гардероб, Най-добрите 20 идеи за обновяване на вградения кът за отдих, Как да направите вградената си кухненска зона функционална и стилна, най-добрият начин да преобразите вградената си дневна с помощта на декор, нови идеи за обновяване на вградения гардероб в антрето, Съвети за подобряване на вградената зона на камината, превръщане на вградената библиотека в идеалното място за четене, Най-добрите 20 идеи за обновяване на вградения гардероб в спалнята
уреди за вграждане бургас [url=https://www.veto.bg]https://www.veto.bg[/url] .
[url=https://vk.com/podslushanomoskwa]Моя Москва[/url] – Наша Москва, Работа Москва
[url=https://vodkabet.io]вотка бет[/url] – водкабет зеркало vodkabet, vodka bet зеркало
билайн тв
https://plus-domashnij-internet-krasnodar.ru
билайн интернет краснодар
сюда [url=https://bmoldova.bz]Молдова[/url]
Стационарное лечение рекомендуется при сильной алкогольной интоксикации, когда пациент испытывает головные боли, тошноту, слабость, повышенное давление и нарушение сна. Если эти симптомы игнорировать, возможны серьезные последствия, включая развитие хронических заболеваний и осложнений.
Подробнее можно узнать тут – [url=https://kapelnica-ot-zapoya-ekb55.ru/]http://kapelnica-ot-zapoya-ekb55.ru/kapelnicza-na-domu-ekaterinburg-ot-zapoya/[/url]
билайн тв
https://plus-domashnij-internet-krasnodar-3.ru
билайн домашний интернет краснодар
Подробная инструкция по применению супрастинекса, рекомендации от профессионалов.
Супрастинекс: как принимать для максимального эффекта, секреты успешного лечения.
Как правильно использовать супрастинекс: шаг за шагом, советы от врачей.
Супрастинекс: подробная инструкция и советы экспертов, подсказки для успешного лечения.
Узнайте все о применении супрастинекса: подробная инструкция, рекомендации специалистов.
Как правильно использовать супрастинекс: полная инструкция, рекомендации для пациентов.
Инструкция по применению супрастинекса: все советы и рекомендации, эффективные стратегии использования.
Инструкция по применению супрастинекса для максимальных результатов, подробности в рекомендациях.
Как правильно принимать супрастинекс: полная инструкция, советы для пациентов.
Секреты успешного применения супрастинекса, подсказки для эффективного лечения.
супрастинекс таблетки цена инструкция по применению взрослым [url=https://suprastinexxx.ru/]https://suprastinexxx.ru/[/url] .
Your articles are extremely helpful to me. Please provide more information! http://www.hairstylesvip.com
888starz bet скачать https://profootball.su/wp-content/pages/strategii-stavok-na-sport-888starz.html
888starz скачать на андроид https://profootball.su/wp-content/pages/strategii-stavok-na-sport-888starz.html
Представляем вашему вниманию онлайн-портал, с индийскими фильмами и сериалами с онлайн-показом! На сайте вы найдете огромный выбор культовых индийских кинолет, кроме того топовые сериалы, покорившие зрителей во всех странах. У нас вы найдете различные жанры: от невероятных драм и романтических комедий до мистических триллеров и исторических эпопей. Наша база фильмов содержит как классические шедевры, так и новые киноленты, чтобы все могли найти что-то на свой вкус.
С помощью нашего сервиса вы можете посмотреть [url=https://india-film.ru/melodrama/]индийские фильмы на русском смотреть онлайн[/url] находясь где угодно. Простой интерфейс и высокое качество видеозаписей обеспечивают незабываемый отдых во время просмотра. Окунитесь в мир индийского кинематографа и откройте для себя его невероятную культуру на нашем портале!
билайн домашний интернет
https://plus-domashnij-internet-krasnodar-2.ru
билайн домашний интернет краснодар
888starz casino https://xn—-dtbe1bsa.xn--p1ai/modules/artcles/?casino-888starz-riski.html
888starz bet скачать на андроид https://xn—-dtbe1bsa.xn--p1ai/modules/artcles/?casino-888starz-riski.html
билайн цены
https://plus-domashnij-internet-krasnodar.ru
билайн тв
https://naveridbuy.blogspot.com/2024/09/blog-post_12.html
Организуете презентацию в столице? [url=https://format-ms.ru/catalog/press-voll/]заказать пресс волл в Москве[/url] – это создать стильный фон для мероприятия. Высокое качество печати, прочные материалы и легкость сборки – идеальный инструмент для брендинга!
ФОРМАТ-МС — ваш выбор для качественных мобильных стендов. Подробности на format-ms.ru. Наш офис в Москве: Нагорный проезд, дом 7, стр. 1, офис 2320. Свяжитесь с нами по телефону +7(499)390-19-85.
билайн тарифы краснодар
https://plus-domashnij-internet-krasnodar-3.ru
билайн тв краснодар
Гарантированное решение ваших проблем — [url=https://telegra.ph/Zajmy-pod-zalog-PTS—poluchit-zajm-pod-zalog-avtomobilya-na-kartu-onlajn-02-18]взять займ под залог автомобиля без отказа[/url]. Мы поможем каждому клиенту воплотить планы в жизнь.
New Retro Casino
Буй казино официальный сайт
скачать 888 starz https://ulov.guru/articles/polnyj-obzor-kazino-888starz-igry-bonusy-vyplaty/
скачать 888starz на телефон бесплатно https://ulov.guru/articles/polnyj-obzor-kazino-888starz-igry-bonusy-vyplaty/
I can suggest to come on a site, with a large quantity of articles on a theme interesting you.
btc казино
скидки озон
https://medium.com/@dqvchristopherwhite824/%EB%84%A4%EC%9D%B4%EB%B2%84-%EC%95%84%EC%9D%B4%EB%94%94-%EC%97%B0%EB%8F%99%EB%90%9C-%EC%84%9C%EB%B9%84%EC%8A%A4-%ED%99%95%EC%9D%B8%ED%95%98%EA%B3%A0-%EA%B4%80%EB%A6%AC%ED%95%98%EB%8A%94-%EB%B2%95-346e62d303f0
билайн подключение
https://plus-domashnij-internet-krasnodar-2.ru
билайн подключение
https://medium.com/@nsw5288/%EB%B9%84%EC%95%84%EA%B7%B8%EB%9D%BC%EC%99%80-%EB%B9%84%EC%8A%B7%ED%95%9C-%EC%A0%9C%ED%92%88-%EC%96%B4%EB%96%A4-%EA%B2%83%EC%9D%84-%EC%84%A0%ED%83%9D%ED%95%B4%EC%95%BC-%ED%95%A0%EA%B9%8C-9be262255a0f
https://tawny-wombat-dd3cmn.mystrikingly.com/blog/7f0e593caac
скачать windows 10
Jetton Casino Jetton Casino is an alluring destination where gaming enthusiasts find excitement and luck in every corner. With a vibrant atmosphere and a wide variety of games, it caters to both novice players and seasoned gamblers alike. The casino features a plethora of slots, table games, and poker options, ensuring that there is something for everyone to enjoy.
Ищете оригинальное рекламное решение? [url=https://format-ms.ru/catalog/l-banner/]L баннер[/url] – это стильная конструкция, привлекающая внимание клиентов. Легкость в установке, прочные материалы и качественная печать сделают вашу рекламу эффективной и заметной.
ФОРМАТ-МС — эксперт в производстве мобильных стендов. Узнайте больше на format-ms.ru. Наш офис расположен по адресу: Москва, Нагорный проезд, дом 7, стр. 1, офис 2320. Контактный телефон: +7(499)390-19-85.
Непродовольственные товары Услуги перевозки в интернет-магазине товаров, не относящихся к продуктовому сегменту.
https://chistvent.ru
Получить деньги без продажи автомобиля просто! С помощью [url=https://telegra.ph/Zajmy-pod-zalog-PTS—poluchit-zajm-pod-zalog-avtomobilya-na-kartu-onlajn-02-18]взять займ под залог птс автомобиля[/url] вы можете воплотить любые планы, не отказываясь от личного транспорта.
Основные критерии выбора конкурсного управляющего, Как стать конкурсным управляющим: шаги к успеху, тайны профессионального роста, Как подготовиться к работе конкурсным управляющим: ключевые моменты, практические рекомендации, Эффективное управление в условиях конкурса, практический курс, экспертный анализ, Основные принципы работы конкурсного управляющего, Интересные факты о работе конкурсным управляющим, эксклюзивные советы
конкурсный управляющий [url=http://konkursnyj-upravlyayushhij.ru/]http://konkursnyj-upravlyayushhij.ru/[/url] .
билайн подключить краснодар
https://plus-domashnij-internet-krasnodar.ru
билайн тарифы краснодар
https://umber-iris-dd3cm2.mystrikingly.com/blog/8df46133ce5
Vavada Casino промокод от стримеров
https://umber-iris-dd3cm2.mystrikingly.com/blog/f73c174c8b7
Jvspinbet
Mehmet Akif su kaçak tespiti Hızlı ve güvenilir bir hizmet sundular. Teşekkür ederiz! http://riotstories.co.uk/author/kacak/
приложение накрутки Тик Ток
Cryptoboss Casino Cryptoboss Casino, криптобосс казино, cryptoboss регистрация, cryptoboss casino бездепозитный бонус.
билайн интернет краснодар
https://plus-domashnij-internet-krasnodar-3.ru
билайн тарифы краснодар
В этой статье представлен занимательный и актуальный контент, который заставит вас задуматься. Мы обсуждаем насущные вопросы и проблемы, а также освещаем истории, которые вдохновляют на действия и изменения. Узнайте, что стоит за событиями нашего времени!
Ознакомиться с деталями – http://www.k-kasagi.jp/oneword.php?itemid=1428
азино777 вин официальный сайт вход с компьютера azino777 официальный
Бездепозитные казино Бездепозитные казино за регистрацию с выводом без пополнения по номеру.
Игровые автоматы на реальные деньги с бонусом при регистрации без депозита Игровые автоматы на реальные деньги с бонусом при регистрации без депозита.
подробнее [url=https://bsmey.at]bsme at[/url]
Эксклюзивные магазины мебели – лучший выбор для вашего дома
купить итальянскую мебель в москве [url=http://www.kupit-mebel-italii.ru]http://www.kupit-mebel-italii.ru[/url] .
[url=https://kraken01.com/]Официальный сайт кракен[/url] – Официальный сайт кракен, Kraken darknet market
[url=https://kraken01.com]Новая гидра[/url] – Kraken darknet market, Новая гидра
Получите кредит на карту без необходимости фото паспорта, легко и быстро.
Займ только по паспорту без фото [url=http://niasam.ru/vklady__kredity__kreditnye_karty/zajm-bez-foto-pasporta-udobstvo-i-vazhnye-momenty-248466.html/]http://niasam.ru/vklady__kredity__kreditnye_karty/zajm-bez-foto-pasporta-udobstvo-i-vazhnye-momenty-248466.html/[/url] .
Incredible quite a lot of amazing facts!
online casino website philippines https://buckscasino.info/live-casinos-online/ full list of usa online casinos
содержание [url=https://bsmey.at]bsme at[/url]
опубликовано здесь [url=https://vulkan-777777.ru/]вулкан 777[/url]
Следующая страница [url=https://1xslotscasinos.com]1хслотс[/url]
накрутка подписчиков в Телеграм
Piastrix Wallet Лотерейные билеты, Регистрация домена, Заработок в интернете, Биржа ссылок.
нажмите [url=https://roxcasino-777.com]rox casino[/url]
психолог ярославль врач психотерапевт, консультация психотерапевта, психотерапевт онлайн, психотерапевт ярославль.
подробнее [url=https://riobetcasinoru.xyz/]риобет[/url]
бонусы за регистрацию Casino bonus Игровые автоматы с бонусом за регистрацию без депозита.
мегафон интернет
мегафон тарифы екатеринбург
мегафон тарифы на интернет
веб-сайт [url=https://vulkan-777777.ru]вулкан 777[/url]
этот контент [url=https://riobetcasinoru.xyz/]риобет[/url]
мегафон подключить
https://plus-ekb-domasnij-internet-2.ru
мегафон екатеринбург
подробнее здесь [url=https://1xslotscasinos.com]1xslots[/url]
Продам онколекарства торг уместен
https://leokors.ru/
интернет [url=https://roxcasino-777.com]rox casino[/url]
Автоэксперт Алматы
Частенько Fonbet раздаёт эксклюзивные коды на фрибеты – бесплатная ставка, которая не требует, чтобы на балансе были деньги. Если выполните все условия букмекерской конторы и выиграете, тогда кеш зачислится уже в виде реальных денег. Их получится перевести на карточку или e-кошелёк https://www.ikonu.ru/faq/pgs/1xbet_promokod_bonus_kod.html
Обладает тонизирующим и антиоксидантным свойствами, лифтинг эффектом, улучшает упругость, предотвращает старение кожи, помогает избежать преждевременного появления большого количества морщин, уменьшает пигментацию, способствует уменьшению проявлений сосудистой сеточки на лице, способствует устранению воспалительных процессов на коже, эпителизации https://bioquant-garyaev.com/product/biomatritsa/
Güllübağlar su kaçak tespiti Her bina için özel kaçak tespit stratejileri gereklidir. https://addurl.us/domain/uskudartesisat.com
Бонусы без отыгрыша за регистрацию
Зачем же нужна накрутка лайков? Прежде всего, это способ привлечь внимание к вашему контенту. У пользователей сложилось мнение, что видео с большим количеством лайков более качественное и интересное накрутка лайков в Тик Ток дешево
Букмекерские конторы в москве фрибет за регистрацию
мегафон екатеринбург
https://plus-ekb-domasnij-internet-3.ru
мегафон телевидение екатеринбург
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?
Максимизируйте свой профит! Разбираем [url=https://ru.nztpoker.com/poker-income/]стратегии увеличения дохода от покера[/url]: какие форматы наиболее прибыльны, когда стоит играть агрессивно, а когда – осторожно. Покер может приносить стабильный доход, если знать, как играть правильно!
мегафон подключить екатеринбург
мегафон телевидение екатеринбург
мегафон интернет екатеринбург
Существует множество способов накрутки лайков. Некоторые из них легитимны и позволяют вам получить настоящую аудиторию, а другие могут привести к бану аккаунта. Важно выбирать правильные методы, чтобы избежать негативных последствий накрутка лайков в ТТ бесплатно без заданий
Интернет магазин продажа Биоматриц Гаряева, модулятор Гаряева-Мишина, ЖКИМ жидкая квантовая индивидуальная матрица https://bioquant-garyaev.com/product/biomatritsa/
Надежность и удобство – главные преимущества [url=https://autosport.com.ru/other/95981-bukmekerskaya-kontora-melbet-registraciya-na-sayte-i-obzor-stavok/]Melbet зеркало[/url]. Оно гарантирует безопасное подключение и комфортное взаимодействие с платформой. Делайте ставки, следите за результатами и получайте удовольствие от процесса.
Проблемы с доступом больше не помеха для игры. [url=https://autosport.com.ru/forecasts/95994-bukmekerskaya-kontora-zenit-win-zenitbet/]Zenitbet зеркало[/url] обеспечивает мгновенный вход на сайт, где вас ждут широкая линия и быстрые выплаты. Не упустите возможность заработать на своем опыте и интуиции.
Si disfrutas el sector de las apuestas en Espana, estas en el lugar indicado.
Conoce los sitios con **proteccion avanzada, mejores bonos y juegos emocionantes**.
**?Que encontraras en los casinos mas destacados de Espana?**
**Casinos regulados y regulacion espanola para una total tranquilidad.**
**Regalos de bienvenida que no puedes perderte.**
**Ruleta, blackjack, tragaperras y mas con grandes botes.**
**Pagos rapidos y sin complicaciones con opciones bancarias flexibles.**
**?Te gustaria descubrir cuales son de los casinos mas recomendados?**
En este blog hemos recopilado las **opiniones de expertos sobre los casinos mas seguros de Espana**: https://o-dachnik.ru/chuvstva-s-dostavkoj-na-dom-cvety-ot-azalianow-v-moskve.html
**Abre tu cuenta en un sitio seguro y disfruta del juego ahora!**
мегафон интернет
https://plus-ekb-domasnij-internet-2.ru
мегафон домашний интернет екатеринбург
Наркологическая клиника “Ресурс здоровья” — специализированное медицинское учреждение, предназначенное для оказания профессиональной помощи лицам, страдающим от алкогольной и наркотической зависимости. Наша цель — предоставить эффективные методы лечения и поддержку, чтобы помочь пациентам преодолеть пагубное пристрастие и восстановить контроль над своей жизнью.Миссия клиники заключается в содействии восстановлению здоровья и социальной реинтеграции людей, столкнувшихся с проблемой зависимости. Мы стремимся к комплексному решению этой сложной задачи, учитывая физические, психологические и социальные аспекты зависимости. Наша задача — не только помочь избавиться от пагубного пристрастия, но и обеспечить успешную реадаптацию пациентов в обществе.Ключевым направлением нашей работы является индивидуализация лечения. Мы понимаем, что каждый пациент уникален, поэтому проводим тщательную диагностику, изучая медицинскую историю, психологический профиль и социальные факторы. На основе этих данных разрабатываем персональные планы терапии, включающие медикаментозное лечение, психотерапевтические методы и социальные программы.Кроме того, клиника “Ресурс здоровья” делает акцент на профилактике рецидивов. Мы обучаем пациентов навыкам управления стрессом, эмоциональному регулированию и формированию здорового образа жизни. Наша цель — обеспечить долгосрочное восстановление и снизить риск возвращения к пагубным привычкам.Врачебный состав клиники “Ресурс здоровья” состоит из высококвалифицированных специалистов в области наркологии. Наши врачи-наркологи имеют обширный опыт работы с зависимыми пациентами и постоянно совершенствуют свои навыки.
Подробнее тут – [url=https://narco-vivod.ru/]вывод из запоя дешево краснодар[/url]
Любите спорт и хотите заработать? [url=https://autosport.com.ru/other/95981-bukmekerskaya-kontora-melbet-registraciya-na-sayte-i-obzor-stavok/]Melbet сайт[/url] предлагает все необходимые инструменты для анализа и прогнозирования. Выбирайте события, делайте ставки и получайте выигрыши в кратчайшие сроки.
Ищете надежную платформу для ставок? [url=https://autosport.com.ru/forecasts/95995-oficialnyy-sayt-bk-marafon-marathonbet/]Марафонбет букмекерская контора[/url] предлагает высокие коэффициенты и широкую линию. Регистрируйтесь сейчас и получите щедрый бонус на первый депозит для успешного старта игры.
провайдер мегафон
https://plus-ekb-domasnij-internet-3.ru
мегафон телевидение
Покер — это математика, а не гадание на картах. Хотите точно знать, какие руки разыгрывать? Разберитесь в [url=https://ru.nztpoker.com/poker-math/]математических основах покера[/url], чтобы не надеяться на удачу, а просчитывать каждую раздачу наперед.
Официальный сайт Vodka Casino: регистрация и вход в Водка Казино онлайн. Vodka Casino предлагает пользователям уникальные возможности для игры в онлайн-казино Vodka Casino регистрация
Главная [url=https://1xslots-casino-ru.xyz]1хслотс[/url]
Whoa tons of valuable information.
online casino hr https://combatcasino.info/review-cafe/ online casino in india is legal
мегафон подключение екатеринбург
мегафон домашний интернет
мегафон телевидение
https://hozforum.actieforum.com/t3869-topic#12635
мегафон подключение
https://plus-ekb-domasnij-internet-2.ru
мегафон тв
Arkada Casino – это игровая платформа, предлагающая посетителям удобный доступ к азартным играм и высокую надежность. Здесь игроки со всего света смогут насладиться азартом в любое время и в любом месте Казино Arkada регистрация
Your point of view caught my eye and was very interesting. Thanks. I have a question for you.
Мы предлагаем большой ассортимент лодок ПВХ, моторов для лодок, запасных частей и аксессуаров, которые гарантируют вам времяпрепровождение на воде комфортным и спокойным.
[url=https://draiv38.ru/catalog/naduvnye-lodki]лодки пвх под мотор иркутск[/url] – это отличный выбор для отдыха с рыбалкой на природе или активных водных развлечений. Наши лодки ПВХ легкие, устойчивые и занимают мало места в сложенном виде, они легко переносятся и быстро накачиваются. Мы предлагаем модели разных размеров и характеристик, чтобы вы могли выбрать именно ту, которая соответствует вашим потребностям.
Наши надежные лодочные моторы обеспечат вам большую скорость и управляемость на воде. Каталог запчастей и аксессуаров помогут содержать вашу лодку ПВХ в отличном состоянии и сделают каждую поездку по-настоящему беспроблемной.
Покупая у нас, вы получаете не только качественный продукт, но и консультацию профессионального менеджера, а также гарантии на все продукты. Заказывайте надувные лодки и все необходимое для рыбалки прямо сейчас!
This is an optional version of the SA-MP 0.3.7 client containing various fixes Open Multiplayer
мегафон тарифы на интернет
https://plus-ekb-domasnij-internet-3.ru
провайдер мегафон
https://newmed.co.il/nevrologiya/
проект коттеджа [url=http://www.tipovye-proekty-domov.ru]http://www.tipovye-proekty-domov.ru[/url] .
Если вы ищете волнение и азарт, предлагаем обратить внимание на Banda Casino. Онлайн-казино представляет большой перечень игр, среди которых каждый найдет что-то интересное. Благодаря безопасным транзакциям и современной мобильной версии, вы можете насладиться игрой в любом месте, где имеется интернет Казино Banda
Купить Haval – только у нас вы найдете разные комплектации. Быстрей всего сделать заказ на haval 2024 салон можно только у нас!
[url=https://haval-msk1.ru]хавал новый купить в москве у официального[/url]
хавал цена – [url=https://haval-msk1.ru]http://www.haval-msk1.ru[/url]
купить чеки в Тюмени Приобрести квитанции. Оформить заказ на чеки. Купить чеки в городе Тюмень.
Казино Раменбет – это прогрессивная онлайн-площадка для развлечений, которая очень быстро завоевала популярность благодаря щедрым бонусам, разнообразию игр и приятным условиям. Лицензированное казино обеспечивает комфорт и безопасность на каждом этапе взаимодействия Ramenbet Casino регистрация
Хотите выигрывать чаще? [url=https://ru.nztpoker.com/poker-tournaments/]Как выигрывать в покерных турнирах[/url] — руководство по успешной игре на дистанции. Узнайте, как правильно управлять стеком, применять ICM и адаптироваться к разным стадиям турнира!
Your point of view caught my eye and was very interesting. Thanks. I have a question for you.
Document translation services cover a wide range of materials, ensuring accurate and professional translations for legal, academic, technical, medical, and business purposes. Certified translation services provide official translations of important documents such as birth certificates, passports, court orders, contracts, and declarations, meeting legal and governmental requirements. Businesses often require translations for product catalogs, financial statements, user manuals, and commercial offers to expand globally. Academic and research materials, including theses, white papers, and scientific studies, are translated to facilitate knowledge sharing. Media-related documents such as magazines, newspapers, and journals require precise localization to maintain context. Surveys, business proposals, and promotional materials also benefit from expert translation. Whether translating medical records, financial reports, or technical specifications, professional translators ensure clarity, accuracy, and cultural relevance. High-quality document translation services play a crucial role in global communication, legal compliance, and international business expansion. Visit our website today to learn more about our translation of technical specification and professional translation solutions!
на этом сайте
[url=https://maralisa.ru/category/kashpo-podvesnoe/]кашпо подвесное[/url]
their explanation
[url=https://337store.pro/]VOID CHECK[/url]
[url=https://yvision.kz/community/voyna-i-mir]боевые действия Украина[/url] – блог мода Казахстан, розыгрыши автомобилей
Document translation services cover a wide range of materials, ensuring accurate and professional translations for legal, academic, technical, medical, and business purposes. Certified translation services provide official translations of important documents such as birth certificates, passports, court orders, contracts, and declarations, meeting legal and governmental requirements. Businesses often require translations for product catalogs, financial statements, user manuals, and commercial offers to expand globally. Academic and research materials, including theses, white papers, and scientific studies, are translated to facilitate knowledge sharing. Media-related documents such as magazines, newspapers, and journals require precise localization to maintain context. Surveys, business proposals, and promotional materials also benefit from expert translation. Whether translating medical records, financial reports, or technical specifications, professional translators ensure clarity, accuracy, and cultural relevance. High-quality document translation services play a crucial role in global communication, legal compliance, and international business expansion. Visit our website today to learn more about our translation of white papers and professional translation solutions!
review [url=https://galaxy-swapper.org]galaxyswapper[/url]
http://steeldirectory.homedirectory.biz/details.php?id=315173
Эта статья предлагает живое освещение актуальной темы с множеством интересных фактов. Мы рассмотрим ключевые моменты, которые делают данную тему важной и актуальной. Подготовьтесь к насыщенному путешествию по неизвестным аспектам и узнайте больше о значимых событиях.
Ознакомиться с деталями – https://alpefpre.com/component/k2/item/1https-?start=10130
ставки на спорт тг>Азино777
Casino Starda предлагает доступ к сертифицированным игровым автоматам от популярных провайдеров, гарантируя честный игровой процесс. Для удобства посетителей создана надежная система. Казино обеспечивает полную защиту данных и выполнение обязательств перед клиентами Казино Starda бонусы
Businesses looking to enter the Iraqi market can benefit from the expert commentary found on Iraq Business News. Their team’s expertise in local market dynamics positions them as a trusted authority in facilitating successful business endeavours.
[url=https://megaweb14darknet.org]megaweb[/url] – ///mega.sb, megaweb13 at
[url=https://kentcasino.io]казино р7 официальный[/url] – игра r7 casino, онлайн казино р7
pop over to this web-site [url=https://sms-verif.pro]Online SMS verification for registration[/url]
Мы предоставляем аренду автомобилей для крупных компаний и частных лиц, а также для малого и среднего бизнеса. Наша компания предлагает широкий выбор автомобилей, включая эконом-класс, бизнес-класс, минивэны и коммерческие авто, чтобы каждый клиент мог подобрать машину, соответствующую его требованиям Прокат автомобилей для туристов в Москве
[url=https://betslive.ru/greenada-casino-promokod/]Greenada casino промокод при регистрации[/url] – Приветственные бонусы по промокоду Риобет, Betwinner промокод бонусы на депозит
Азино777 Регистрация Азино777 Регистрация
… [Trackback]
[…] Read More Infos here: conradlotz.com/system-design-part-1-building-a-simple-load-balancer/ […]
Азино777 Зеркало на сегодня Азино777 Зеркало на сегодня
азино777 официальный сайт азино777 официальный сайт мобильная
конкурсный управляющий арбитражный суд [url=http://www.konkursnyj-upravlyayushhij-po-bankrotstvu.ru/]http://www.konkursnyj-upravlyayushhij-po-bankrotstvu.ru/[/url] .
Начинаем продвижение интернет-магазина во ВКонтакте с основ: создание сообщества Витрину с товарами лучше всего разместить в группе или паблике продвижение профессиональное сайтов ленинская слобода 26
Если вас интересует официальный сайт Сукааа Казино – вся самая актуальная информация размещена на нашем портале. А мы рассмотрим главные преимущества, которые предлагает эта онлайн-площадка Казино Sykaaa
Trix is for four players and has four kingdoms, each of which has five games: King, Queens, Diamonds, Ltoosh and Trix Анкоры: is it better to masturbate without porn
Azino777 регистрация Азино777 Регистрация
[url=https://megaweb14darknet.org/]mega onion[/url] – megaweb, mega sb
Kometa casino – новое развлекательное развлекательное казино, предлагает вашему вниманию свыше 5 600 онлайн-игр от ведущих брендов, регулярные бонусы, промо Kometa Casino мобильная версия
For updates on Iraqi oil deals, production levels, and international partnerships, Iraq Business News provides comprehensive coverage that is essential for stakeholders in the energy sector
Топ казино Рейтинг казино
игровые автоматы на реальные деньги с выводом
https://rhabits.io/1739983579465657_6382
[url=https://kentcasino.io]казино р7 игровые автоматы[/url] – casino r7 играть, r7 casino демо счет
Kometa Casino – новое онлайн-казино, запущенное в России в 2024 году. Платформа работает по лицензии Кюрасао, обеспечивая безопасный игровой процесс Казино Kometa
Казино Гама работает по лицензии Кюрасао, предоставляет большой выбор качественных игр и надежные способы платежей. Пользователям доступны счета в рублях Гама Казино бонусы
https://atelierlavoye.com/
Топ казино Играть в казино
мебель италия купить [url=https://www.kupit-italyanskuyu-mebel.ru]https://www.kupit-italyanskuyu-mebel.ru[/url] .
Кракен ссылка
билайн тв
https://jobsinsidcul.in/employer/monika/
билайн тарифы на интернет
вывод из запоя на дому Вывод из запоя, вывод из запоя Москва, вывод из запоя на дому, вывод из запоя на дому Москва, Срочный вывод из запоя на дому
Заказать фейерверк в СПб Пиротехника в СПб
провайдер мтс
https://lifestagescs.com/employer/mireya/
мтс домашний интернет
Букмекерская контора Leonbets предоставляет игрокам удобный доступ к ставкам на спорт через функциональный интерфейс и обширную линейку событий. На сайте [url=https://www.goha.ru/leon-bet-leonbets-zerkalo-rabochee-registraciya-obzor-sajta-4wqP3y/]работающее зеркало леон[/url] предлагает выгодные коэффициенты, приветственные акции и различные акции. Здесь можно заключать пари на более 20 видов спорта, включая популярные дисциплины и eSports. Программа лояльности позволяет собирать очки (леоны), которые можно обменять на вознаграждения, а официальная аппликация обеспечивает удобный доступ к возможностям.
Для жителей РФ, где официальная страница может быть ограничен, существует [url=https://www.goha.ru/leon-bet-leonbets-zerkalo-rabochee-registraciya-obzor-sajta-4wqP3y/]leon ru[/url] обеспечивающее неограниченный доступ к аккаунту и основным возможностям. Зеркало повторяет оригинальный сайт, сохраняя все настройки аккаунта, и работает без рекламы. Минимальный размер пари составляет всего 10 рублей, а получение выигрыша доступен на популярные способы с учетом комиссионных сборов до 5%. Благодаря таким преимуществам, Leonbets прочно удерживает пятом месте среди ведущих беттинговых компаний по узнаваемости.
kra29 at kra29 at
Для всех поклонников азартных игр — [url=https://masterclassy.ru/pedagogam/vospitatelyam/19205-bk-zenit-zerkalo-vhod-na-sayt-zenitbet-i-registraciya-v-zenit-win.html]бк зенит официальный сайт[/url] откроет доступ к множеству ставок, бонусов и удобных функций для вашего комфорта и безопасности.
On your place I would ask the help for users of this forum.
Для размещения достаточно выбрать, что вы хотите продвигать — товары, услуги или сайт, указать нужное сообщество или дать ссылку на посадочную страницу продвижение сайта в интернете
мтс подключить краснодар
https://community.cathome.pet/employer/opal/
мтс подключение краснодар
проекты домов [url=proekty-zagorodnykh-domov.ru]proekty-zagorodnykh-domov.ru[/url] .
мегафон тарифы на интернет
https://foris.gr/employer/jessika/
мегафон интернет ростов
Най-добрите начини да подобрите вградената си кухня, уникални идеи за модернизиране на вградения шкаф, преобразете вградената си етажерка с тези съвети, Топ идеи за декорация на вградената стена в дома ви, най-добрият начин да превърнете вградения гардероб в гардеробна стая, преобразете вградената си работна зона с тези идеи, преобразете вградената си всекидневна с тези съвети, нови идеи за подобряване на вградените шкафове на балкона, Съвети за подобряване на вградената трапезария, Най-добрите начини за подобряване на вградения гардероб, Тайните за уютен вграден кът за отдих, Идеи за подобряване на вградената ви кухненска зона, Преобразете вградената си дневна с тези невероятни съвети, Как да превърнете вградения гардероб в антрето в място за съхранение и стил, 20 топ идеи за обновяване на вградената зона на камината, Върхови идеи за декорация на вградена библиотека в дома ви, Най-добрите 20 идеи за обновяване на вградения гардероб в спалнята
уреди за вграждане бургас [url=http://www.veto.bg/]http://www.veto.bg/[/url] .
Learn More Here
[url=https://tlo-ssndob.com/]SBA[/url]
билайн подключить
https://talent.tn/employer/lester
билайн тарифы на интернет
You actually said it really well.
online betting casino sites https://combatcasino.info/nba-betting/ online casino ruleta
ттк подключение
https://kkhelper.com/employer/ttk-tarif-solutions/
ттк тарифы барнаул
мтс телевидение
https://zimtechinfo.com/companies/noah/
мтс подключить кемерово
go https://my-sollet.com/
http://rolevikionline.g-talk.ru/viewtopic.php?f=21&t=5458
click for more info [url=https://web-foxwallet.com]Fox wallet extension[/url]
More hints https://web-sollet.com
https://sms-man.com/free-numbers
Для всех тех, кто ценит качество ставок, [url=https://masterclassy.ru/pedagogam/vospitatelyam/19205-bk-zenit-zerkalo-vhod-na-sayt-zenitbet-i-registraciya-v-zenit-win.html]zenit букмекерская[/url] предлагает удобный и выгодный способ делать ставки. Присоединяйтесь и получайте бонусы с каждой регистрацией!
other https://web-kaspawallet.com/
ттк тарифы ростов
https://www.vieclam.jp/employer/may/
провайдер ттк
Это модифицированная версия популярной компьютерной игры GTA San Andreas. Испытайте себя в роли бизнесмена, полицейского, бандита или простого чит меню самп
additional reading [url=https://tlo-lookup.com]tlo ssn dob[/url]
мтс тарифы на интернет
https://cphallconstlts.com/employer/dexter/
мтс домашний интернет краснодар
https://www.lerozcaferestaurant.com/
https://sms-man.com/free-numbers
зомби сафари скачать взлом [url=http://apk-smart.com/igry/gonki/1627-zombie-offroad-safari-vzlomannyj-mnogo-deneg.html]зомби сафари скачать взлом[/url] зомби сафари скачать взлом
P.S Live ID: K89Io9blWX1UfZWv3ajv
P.S.S [url=https://masstr.net/showthread.php?tid=24057&pid=352301#pid352301]Программы и игры для Андроид телефона[/url] [url=https://myflower-design.com/index.php?route=information/blogger&blogger_id=1]Программы и игры для Андроид телефона[/url] [url=https://uni-access.com/view-blog/Masters-degree-in-Cyber-Security-in-Germany]Программы и игры для Андроид телефона[/url] cf6d637
Букмекерская контора Leonbets предоставляет бетторам комфортный доступ к спортивным прогнозам через интуитивный интерфейс и разнообразие событий. На сайте [url=https://www.goha.ru/leon-bet-leonbets-zerkalo-rabochee-registraciya-obzor-sajta-4wqP3y/]бк леон зеркало сайта работающее[/url] предлагает высокие коэффициенты, приветственные акции и разнообразные акции. Здесь можно делать ставки на более 20 видов спорта, включая популярные дисциплины и eSports. Программа лояльности позволяет собирать очки (леоны), которые обмениваются на бонусы, а мобильное приложение обеспечивает моментальный доступ к возможностям.
Для российских бетторов, где официальная страница может быть недоступен, существует [url=https://www.goha.ru/leon-bet-leonbets-zerkalo-rabochee-registraciya-obzor-sajta-4wqP3y/]leonbets зеркало[/url] обеспечивающее полноценный доступ к аккаунту и всем инструментам. Зеркало полностью дублирует оригинальный сайт, сохраняя все персональную информацию, и работает без рекламы. Минимальный размер пари составляет лишь 10 рублей, а транзакции возможен на популярные способы с учетом минимальных удержаний. Благодаря таким преимуществам, Leonbets прочно удерживает топ-5 среди ведущих беттинговых компаний по доверию игроков.
билайн подключить кемерово
https://jobsinethiopia.net/employer/kenton/
билайн подключить кемерово
evocreo wiki [url=https://apk-smart.com/igry/draki/331-evocreo-polnaja-versija.html]https://apk-smart.com/igry/draki/331-evocreo-polnaja-versija.html[/url] evocreo wiki
P.S Live ID: K89Io9blWX1UfZWv3ajv
P.S.S [url=https://premium-instrument.ru/sertifikat-na-skidku-na-buduschie-pokupki.html]Программы и игры для Андроид телефона[/url] [url=https://talk.hyipinvest.net/threads/132609/]Программы и игры для Андроид телефона[/url] [url=https://myflower-design.com/index.php?route=information/blogger&blogger_id=1]Программы и игры для Андроид телефона[/url] f6d6378
билайн интернет
https://clujjobs.com/employer/abdul/
билайн тв
The official website of the online casino Pinko invites all new players to quickly register and start playing the most popular slots and gaming machines pinco
мтс тв кемерово
https://c3tservices.ca/companies/thomas/
мтс подключение
https://supanapa.ru/
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
знакомства в хургаде знакомства в хургаде
мтс тв
https://gurkhalinks.co.uk/employer/neville
мтс тв
apple macbook air 15 m3 512 https://apple-macbook-15.ru
мтс домашний интернет кемерово
http://recruitmentfromnepal.com/companies/arielle/
мтс тарифы на интернет
Virtual number for SMS verification allows you to receive verification codes without exposing your personal phone number, ensuring an extra layer of privacy for online registrations. It is especially useful for accessing services that require SMS confirmation while keeping your actual contact details secure https://www.theasset.com/newsite/arcls/?buy-virtual-number-for-sms-verification.html
Kudos! Valuable information.
online casino no deposit bonus no download instant play https://mapcasino.info/online-casino-colorado/ same day withdrawal online casinos usa no deposit bonus
мегафон цены
https://foris.gr/employer/jessika/
мегафон тв
Amazing a lot of very good info!
5 euro minimum deposit online casino https://hotgamblingguide.org/ignition-casino-no-deposit-bonus/ online casino tricks 2022
Nicely put. With thanks.
online casino pa new https://hotgamblingguide.org/ignition-casino-safe/ casino online promos
Виртуальный номер для Телеграм позволяет вам регистрироваться и пользоваться сервисом, не раскрывая личный номер телефона. Он обеспечивает дополнительную защиту конфиденциальности и удобство в использовании мессенджера номер телеграм
You explained that exceptionally well.
online casino 777 promotiecode https://casinosonlinenew.com/crypto-casino/ casino royale full movie hd online
Pinco il? h?r zaman yeni oyunlar v? t?klifl?r!
http://pinco-az2024.com/
You said that well!
2018 online real money casinos https://ratingcasino.info/casinos/ free online casino slot
ответственный БДД Повышение квалификации водителей – ваш путь к безопасности и профессионализму! Хотите улучшить навыки вождения, узнать актуальные изменения в ПДД и повысить свою конкурентоспособность? Пройдите курс повышения квалификации водителей и станьте настоящим профессионалом! Что дает обучение? Актуальные знания ПДД и изменений в законодательстве Техники безопасного и экономичного вождения Удостоверение о повышении квалификации Кому подойдет? Водителям, работающим в транспортных компаниях Личным и корпоративным водителям
ттк подключить
https://2t-s.com/companies/ttk-tarif-services/
ттк подключение
Amazing many of wonderful facts.
online casino bonus ohne einzahlung 2022 https://mgmonlinecasino.us/online-casinos-massachusetts/ play’n go online casino
провайдер мтс
https://www.graysontalent.com/employer/joanna/
мтс тв
You have made your point very effectively!.
australia online casino no deposit https://snipercasino.info/nfl-football-betting/ tanzania casino online
This is nicely expressed! .
quick hit casino online slots tips and tricks https://casinoshaman.com/online-casino-new-jersey/ asal mula casino online indonesia
This is nicely put. .
5 dollar deposit online casino nz https://mapcasino.info/online-texas-holdem/ slovenia online casino
ттк тв ростов
https://ezworkers.com/employer/andy/
ттк домашний интернет ростов
Nicely put, With thanks!
online no deposit casino bonuses and free spins exclusive https://mapcasino.info/esports-betting/ everest casino online
Awesome postings. Thanks!
free casino slots games online https://mgmonlinecasino.us/betting-apps/ 7bet casino online
Thanks. I like it!
legal pa online casinos https://hotgamblingguide.com/cricket-match-betting/ goldennugget online casino nj
hop over to this web-site [url=https://jaxx-libertywallet.com]wallet jaxx[/url]
билайн телевидение
https://pittsburghpenguinsclub.com/read-blog/19155_bilajn-domashnij-internet-otzyvy-polzovatelej.html
билайн тарифы кемерово
You’ve made your stand quite clearly.!
new new jersey online casinos https://casinoshaman.com/maryland-live-casino-online-gambling/ groupe casino online shop
Nicely put, Kudos!
app casino online argentina mercadopago https://hotgamblingguide.org/texas-online-casinos/ online casino kentucky
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?
Valuable info. Kudos.
casino online live dealer https://linkscasino.info/online-casino-texas/ domino casino online
провайдер мегафон
https://skilling-india.in/employer/humberto/
мегафон телевидение
Beneficial info. Regards!
real money online casino free chips https://linkscasino.info/omaha-poker-online/ online casino slots free
navigate here
[url=https://web-sollet.com/]Sol wallet[/url]
провайдер мтс
https://www.cbl.health/employer/frederick/
мтс домашний интернет кемерово
Well spoken of course. !
gta v online diamond casino heist https://hotgamblingguide.com/safe-online-casinos-usa/ planet 7 online casino
Very good data. Cheers.
new no deposit online casino bonus https://casinosonlinenew.com/review-cafe/ online casino ultimate texas holdem
You actually reported that very well.
vermont online casino bonus https://hotgamblingguide.com/best-soccer-betting-app/ best casino gambling online
Many thanks. I appreciate it.
keno online spielen casino https://riggambling.com/online-casino-ohio/ best online casino thailand
find here [url=https://web-foxwallet.com]Fox crypto wallet[/url]
мтс тарифы на интернет
https://www.dataalafrica.com/employer/lorene/
мтс подключение краснодар
Excellent material. Regards!
ignition online casino https://hotgamblingguide.org/australian-casino-online/ michigan real money online casino
You said it adequately.
casino online repГєblica dominicana https://casinoshaman.com/wild-casino-no-deposit-bonus-codes/ online casino ideal nederland
Awesome facts, Thank you.
how do you know if an online casino is legit https://hotgamblingguide.info/new-york-casino-online/ online casino stocks
мегафон интернет ростов
http://busforsale.ae/profile/sandratorr286
мегафон подключение
Nicely voiced certainly! .
gta online casino heist wiki https://onlinecasinoindex.us/reviews/ online casino bonus codes 2024
ттк телевидение
http://recruitmentfromnepal.com/companies/ttk-tarif-consulting/
ттк тарифы барнаул
find out this here [url=https://my-sollet.com]Sollet.io[/url]
Whoa lots of terrific advice.
bandar casino sbc168 online https://igamingcasino.info/real-money-online-casino-georgia/ gta online best way to do casino heist
Kudos! I value it.
best online bitcoin casino https://magicalcasino.info/betting/ online casino no deposit bonus code
ттк цены
https://vitricongty.com/companies/ttk-tarif-consulting/
ттк интернет
Nicely put. With thanks.
online casino demo mode https://hotgamblingguide.info/baccarat-casino-online/ casinos online canada
Good content. Thanks a lot.
game vault 999 online casino platforms usa https://usagamblingexperts.com/sports-betting-sites/ online casino deposit 5 minimum
With thanks, I appreciate it.
jackpots casino online https://casinoslotoking.com/best-online-casino-north-carolina/ vegas strip casino online $100 no deposit bonus codes 2022
Good forum posts. Kudos!
best casino games to make money online https://casinonair.com/online-blackjack/ online casino bayern
ттк тв
https://branditstrategies.com/employer/rachel/
ттк телевидение
Cheers, Valuable information.
what online casino games can you win real money https://casinoslotssaid.com/casino-cafe-online/ casino games roulette online
Nicely put. Thanks a lot.
online casino spielen echtgeld https://shadowcasino.info/review-wild/ can you really win on online casinos
Effectively expressed of course! .
gta online solo casino heist https://casinoslotoking.com/las-atlantis-casino-review/ low wagering online casino
ттк подключение ростов
https://www.informedica.llc/employer/megan/
ттк интернет ростов
Whoa a good deal of beneficial info.
21 casino movie watch online https://snipercasino.info/review-lucky-tiger/ casino royale full movie online
his response [url=https://web-kaspawallet.com]Wallet kaspa[/url]
Thanks! Lots of posts.
aztec bonanza online casinos https://uscasinoguides.com/golf-betting/ best online casinos with no deposit bonuses
Superb info, Kudos.
online casinos auszahlungen https://ratingcasino.info/horse-betting/ easy online casino
Nicely spoken of course! .
fanduel online casino ny https://igamingcasino.info/virginia-online-casinos/ online casino best deals
site here
[url=https://jaxx-libertywallet.com/]jaxx liberty restore[/url]
скачать mortal kombat 4 на android [url=https://apk-smart.com/igry/draki/677-mortal-kombat-4.html]https://apk-smart.com/igry/draki/677-mortal-kombat-4.html[/url] скачать mortal kombat 4 на android
P.S Live ID: K89Io9blWX1UfZWv3ajv
P.S.S [url=https://www.forum-joyingauto.com/showthread.php?tid=121721]Программы и игры для Андроид телефона[/url] [url=http://moujmasti.com/showthread.php?81381-The-grinch-2000-Coco-2017-Search-engines-Beetroot&p=1258303#post1258303]Программы и игры для Андроид телефона[/url] [url=https://www.nedvizhimost43.ru/news/news_1018.html]Программы и игры для Андроид телефона[/url] a0bc539
You have made your point pretty well.!
2018 online casino usa https://casinoslotssaid.com/bitcoin-casinos/ buffalo run casino online
В ассортименте качественные электронные сигареты многоразовые от ведущих производителей: Brusko, Voopoo, Elf, Smoant, Vaporesso, Pasito, Smok, GeekVape hqd электронные сигареты купить
билайн подключение кемерово
https://manpoweradvisors.com/employer/jai/
билайн тарифы на интернет
You made your stand extremely effectively!.
best online casino australia no deposit bonus https://casinosonlinenew.com/safe-casinos-online/ gta online casino heist waste disposal
Discover the latest promotional codes for top betting platforms like Betwinner, Melbet, Fonbet, 1xBet, and more! Whether you’re looking for a welcome bonus, a free bet, or an exclusive registration promo code, these links provide access to updated offers for sports betting and online casinos. Find Melbet promo codes for 130% bonus, 1xBet free bet codes, and Fonbet promotions to boost your betting experience. Explore special bookmaker promotions across various countries, including Russia, Rwanda, Cameroon, and Tajikistan. Don’t miss out on the best casino and sportsbook deals available today!👉 Visit now and claim your bonus! https://actual-cosmetology.ru/pgs/promokod_fonbet_bonus.html
Thank you, An abundance of data.
new ontario online casino https://onlinecasinoindex.us/craps-game-casino/ best online casinos pa
Cheers! Lots of knowledge.
mgm casino online slots nj https://snipercasino.info/games/ casino online tiradas gratis
Nicely put, With thanks!
cash machine 777 online casino download https://buckscasino.info/ufc-mma-betting/ mejores casinos online microgaming
мтс интернет
https://weworkworldwide.com/employer/dexter/
мтс тарифы на интернет
Superb material. Appreciate it!
nieuw online casino https://cryptogamblingguru.com/colorado-online-casino/ nj online casino promo codes
снять теплоход на свадьбу спб https://arenda-yaht-spb.ru
яхта в дубае аренда яхта в дубай
Thank you! I enjoy it!
gta online how to win casino https://shadowcasino.info/tennessee-online-casino/ mvg online casino
Представляем вашему вниманию онлайн-кинотеатр, посвященный индийским фильмам и сериалам с с возможностью смотреть онлайн! У нас вы найдете огромный выбор культовых болливудских фильмов, а также топовые телесериалы, покорившие зрителей во всех странах. У нас вы найдете различные жанры: от захватывающих драм и романтических комедий до мистических триллеров и исторических эпопей. Наша коллекция содержит как давно полюбившиеся всем фильмы, так и современные хиты, чтобы каждый мог посмотреть что-то на свой вкус.
С помощью нашего сервиса вы можете посмотреть [url=https://india-film.com/melodrama/]смотреть индийские фильмы на русском языке[/url] находясь где угодно. Простой интерфейс и отличное качество видеозаписей обеспечивают незабываемый просмотр. Погрузитесь в мир индийского кино и откройте для себя его захватывающую культуру вместе с нами!
Fantastic material. Thanks a lot!
casinos online mexico https://findscasino.info/credit-card-casinos/ online casino 10 euro startguthaben
Discover the latest promotional codes for top betting platforms like Betwinner, Melbet, Fonbet, 1xBet, and more! Whether you’re looking for a welcome bonus, a free bet, or an exclusive registration promo code, these links provide access to updated offers for sports betting and online casinos. Find Melbet promo codes for 130% bonus, 1xBet free bet codes, and Fonbet promotions to boost your betting experience. Explore special bookmaker promotions across various countries, including Russia, Rwanda, Cameroon, and Tajikistan. Don’t miss out on the best casino and sportsbook deals available today!👉 Visit now and claim your bonus! https://www.maxwaugh.com/articles/1xbit_promo_code_bonus.html
You reported this adequately.
eldorado casino online games https://uscasinoguides.com/nascar-betting/ mobi games online casino
провайдер мтс
https://healthcarejob.cz/employer/tanesha/
сайт мтс краснодар
You actually said that exceptionally well!
australia online casino 2018 https://riggambling.com/casinos/ ace play online casino
Запой никогда не проходит самостоятельно, и если он продолжается в течение нескольких дней, организм начинает испытывать серьёзный дефицит жизненно важных веществ и страдать от прогрессирующего накопления токсичных продуктов распада алкоголя, в связи с чем необходимо незамедлительно обратиться за помощью к профессиональным врачам, чтобы предотвратить развитие опасных для жизни осложнений.
Подробнее – [url=https://xn—-7sbjuigkblbdbkg0myb4b.xn--p1ai/]анонимный вывод из запоя ростов-на-дону[/url]
You said it very well.!
usa online casinos no deposit bonus https://cryptogamblingguru.com/slot-online/ online casino games in singapore
Fantastic knowledge. With thanks!
how to rob the casino in gta 5 online https://riggambling.com/real-money-online-casino/ star games real online casino
Cheers, I like it!
online paysafecard casino https://casinoshaman.com/ethereum-casino/ deutsche online casinos bonus ohne einzahlung
мегафон подключить ростов
http://vts-maritime.com/employer/tammara/
мегафон интернет ростов
You expressed that superbly.
best casinos online to withdraw without sending any documents usa https://magicalcasino.info/virginia-online-casinos/ gratis spins casino online
With thanks, Excellent stuff.
best casino online reviews https://casinonair.com/casino-games/ Г¶sterreich online casino
Amazing all kinds of amazing material.
ubet95 online casino login https://findscasino.info/indiana-online-casino/ $5 online casino
ттк интернет
https://stepaheadsupport.co.uk/companies/ttk-tarif-ltd/
ттк барнаул
Cheers, Ample knowledge.
unibet online casino review nj https://shadowcasino.info/esports-betting/ online casino programs
Awesome stuff. Many thanks.
online casino platebnГ metody https://cryptogamblingguru.com/best-mlb-betting-sites/ best betalende online casino nederland
Lovely forum posts, Kudos!
online casino arkansas https://combatcasino.info/review-lucky-tiger/ free online casino nz
Regards! I like it!
online casino med dansk licens https://mgmonlinecasino.us/online-golf-betting/ best online new jersey casino
ттк тв
https://cannabisjobs.solutions/companies/albertha/
ттк цены
Reliable forum posts. Thanks a lot.
admiral online casino bg https://usagamblingexperts.com/review-slotocash/ mi online casino list
Thanks a lot! Quite a lot of content!
online casino vegas slots https://casinocashstars.com/ohio-online-casinos/ best new online casino sites
Табак нагревают до высокой температуры, но не поджигают. Изделия создают не обычный сигаретный дым, а пар, который вдыхает пользователь сменный испаритель купить
билайн телевидение кемерово
https://www.jobsalert.ai/employer/jessica/
билайн цены
elonbet sex
[url=http://pilgrim21.com/bbs/board.php?bo_table=c_01&wr_id=30027]MichaelMek[/url] 6378a5d
elon casino porno
[url=https://ni1kp5.click/2024/04/16/hello-world/comment-page-3605/#comment-185219]RickeyFen[/url] 1b59f3c
elonbet casino porno
[url=http://mmtree.net/bbs/board.php?bo_table=sub0603&wr_id=16583]Michaelbit[/url] ff71b59
elonbet sex
[url=https://www.bamscollegesinbangalore.com/notifications/nta-postpones-neet-ug-exam-due-to-covid-related-restrictions]Michaelbetry[/url] 1b59f3c
мтс подключить кемерово
https://hortpeople.com/companies/forrest/
мтс интернет
elonbet casino porno
[url=https://fppartners.co.kr/bbs/board.php?bo_table=edu_alliance&wr_id=10469]MichaelOwemi[/url] a0bc539
You mentioned this really well!
online casino make real money https://linkscasino.info/golf-betting/ best paypal casino online
Thanks a lot. Ample material!
free slots casino style online games https://linkscasino.info/maryland-online-casino/ gta 5 online diamond casino missions
elon casino porno
[url=http://hwashinlube.com/bbs/board.php?bo_table=inpuiry&wr_id=1229]RonaldVog[/url] 9c06_55
elonbet casino porno
[url=http://deepnewton.com/bbs/board.php?bo_table=story&wr_id=199000]MichaelEroff[/url] 89c04_4
You actually mentioned this well!
discover card online casinos https://uscasinoguides.com/sportsbook/ river monster online casino login
мтс подключение краснодар
http://webheaydemo.co.uk/profile/vickyfalconer
мтс телевидение
elonbet casino porno
[url=http://xn--vj4bq0gdb49l.com/bbs/board.php?bo_table=d_01&wr_id=15092]Ronaldvoity[/url] bc53918
Nicely put. Many thanks!
$1 minimum deposit online casino usa https://casinoshaman.com/free-texas-holdem-online-games/ online casino that pay
elon casino porno
[url=https://aikoland.ru/blogs/stati/ofsetnyy-kryuchok-chtoby-prose/?MID=6897&result=reply]MichaelItake[/url] d6378a5
Awesome info. Thanks a lot.
royal vegas online casino review https://casinocashstars.com/soccer-betting/ new nj online casino
elonbet sex
[url=http://hwarrenhoyt.com/apollos-wannbe-forums/topic/trvsrvrsvtvssv/?part=8#postid-219]MichaelEndot[/url] cf6d637
You said it perfectly..
gvc online casino https://combatcasino.info/legit-online-casinos/ mgm grand online casino bonus codes
elonbet casino porno
[url=https://haksanpub.co.kr/bbs/board.php?bo_table=m08_02&wr_id=13810&&sca=%3F%3F%3F%2F%3F%3F%3F%3F&page=5&#c_54107]RickeyLal[/url] c539189
Thank you! Great information.
best online casino payid withdrawal https://hotgamblingguide.org/new-online-casino-no-deposit-bonus-usa/ any online casinos accept paypal
мегафон подключение
http://bolsatrabajo.cusur.udg.mx/employer/leroy/
мегафон тв ростов
elonbet sex
[url=https://sillimchauveau.co.kr/bbs/board.php?bo_table=qa&wr_id=36204]Michaelcreaw[/url] f6d6378
Great info. Regards!
online casino europe https://casinoshaman.com/best-online-casinos-texas/ palm casino online login
luxury yacht rental yacht a dubai
ссылка на кракен в браузере kraken.cc
Заказать Haval – только у нас вы найдете разные комплектации. Быстрей всего сделать заказ на хавейл джолион можно только у нас!
[url=https://jolion-ufa1.ru]купить хавейл джолион в уфе[/url]
хавал джолион комплектации и цены новый – [url=http://jolion-ufa1.ru/]https://jolion-ufa1.ru/[/url]
Whoa a good deal of superb material!
captain jack casino online https://eseomail.com/real-money-casino-games/ n1 casino online
Casino top бонусы за регистрацию
elonbet casino porno
[url=https://sinceridadeserv.com/2024/01/28/hello-world/#comment-115]MichaelwhowN[/url] 9189c08
ттк цены
https://findmynext.webconvoy.com/employer/ttk-tarif-and-co/
ттк интернет барнаул
Reliable postings. Regards!
online casino tennessee https://casinoshaman.com/casinos/ juegos casino online gratis tragamonedas
You suggested that perfectly.
casino tiger club online https://ratingcasino.info/maryland-online-casino/ online casino free money bonus
elonbet sex
[url=http://therapiens.com/bbs/board.php?bo_table=reservation&wr_id=296567]Ronaldgot[/url] 9189c06
Amazing quite a lot of beneficial data.
new online casino sign up bonus https://combatcasino.info/nba-betting/ best online casinos free spins
провайдер ттк
https://almagigster.com/profile/jonasglasgow56
ттк интернет
elonbet sex
[url=http://sf6.kr/bbs/board.php?bo_table=cs_2&wr_id=94589]RickeyOpive[/url] 3cf6d63
Regards, Lots of advice!
play casino online no deposit https://ratingcasino.info/games/ best odds online casino canada
visit this web-site https://web-foxwallet.com/
elonbet casino porno
[url=https://www.tnrad.org/community/con-discussion/certificate-of-need/paged/16961/#post-254410]Ronaldlek[/url] ff71b59
This is nicely said. .
best online casino in india https://casinosonlinenew.com/states/ minijuegos casino online
Truly all kinds of amazing knowledge!
american express chargeback online casino https://cryptogamblingguru.com/crypto-crash-gambling/ the online casino sister sites
elonbet sex
[url=http://xn--od5b9lh4bb8io1n.kr/module/board/board.php?bo_table=1&wr_id=895368]Richardsax[/url] a0bc539
кракен зеркало рабочее на сегодня kra27 at
The best HD wallpapers https://wallpapers-all.com/tv_show/ in one place! Download free backgrounds for your desktop and smartphone. A huge selection of pictures – from minimalism to bright landscapes and fantasy. Enjoy stylish images every day!
Nicely put. Thanks a lot!
jackpot party online casino game https://combatcasino.info/real-money-online-casino-minnesota/ bclc online casino
Игровые автоматы на деньги реальные Бездепозитные фриспины
You explained that adequately.
online casino anleitung https://onlinecasinoindex.us/ casino online nuevos
elonbet casino porno
[url=https://medee.mn/single/149915]RichardLiark[/url] 378a5da
Kudos, Loads of material!
golden riviera casino online https://casinonair.com/online-casino-georgia/ bonus code for caesars online casino
elon casino porno
[url=https://www.rospisatel.ru/conference2/index.php?event=topic&fid=0&id=17409197649264]RickeyDuazy[/url] a0bc539
Wow lots of helpful material.
vera online casino https://magicalcasino.info/bitcoin-casinos/ free online casino slots with bonus no download
elonbet casino porno
[url=https://advenzavietnam.com/chao-moi-nguoi/#reviews]Richardniz[/url] 00_c610
Hello There. I found your blog the use ofmsn. That is a very neatly written article.I will be sure to bookmark it and come back to read more of your helpful information. Thank you for the post.I’ll definitely comeback.
바카라
Nicely put. Thanks.
bandar betting casino dragon tiger online https://casinocashstars.com/real-money-online-casino-illinois/ www jackpot city online casino
You actually reported that very well!
vegas sweeps online casino download apk https://snipercasino.info/review-mybookie/ 777 casino online download
elon casino porno
[url=https://domaine-st-laurent-de-saurs.com/produit/vin-de-gaillac-rose-cuvee-la-rose/#comment-15482]Richardatomi[/url] 7_c2767
You said it very well!
online vegas casino free slots https://eseomail.com/new-jersey-online-casino-websites/ prepaid mastercard online casino
https://xn--w4-hd0j99gyns5z0qeiim2i.mystrikingly.com/blog/a0cec0860a8
Многие фанаты Hacksaw Gaming с нетерпением ждали новой игры от провайдера, и Le Bandit полностью оправдало их ожидания https://novascreen.ru/articles/vavada-sovremennoe-online-casino
elonbet sex
[url=https://mwamny.click/2024/04/13/hello-world/comment-page-2877/#comment-151115]RonaldAlgog[/url] d6378a5
elon casino porno
[url=http://hsanc.co.kr/bbs/board.php?bo_table=603&wr_id=22061]RickeyLot[/url] 9c01_e1
With thanks! Plenty of postings!
fireball slots online casino https://mapcasino.info/online-craps/ beste online casinos ohne 5 sekunden regel
elonbet casino porno
[url=https://courtyardnorthhill.com/bbs/board.php?bo_table=review_list&wr_id=786534]Rickeydob[/url] a0bc539
Игровые автоматы на деньги лучшие какие автоматы дают деньги за регистрацию без депозита
You actually expressed that very well.
online australian casino real money https://mapcasino.info/online-omaha-poker/ online casino nepal
br> 시알리스시알리시알리스 구매스 5m
Amazing info, Thanks.
guatemalan online casinos https://magicalcasino.info/fast-payout-casino/ australian online casino no deposit bonus 2020
Incredible lots of great material!
best zar online casino https://casinoshaman.com/nascar-betting-odds/ 10 play poker deuces wild free online casino
Many thanks. Lots of write ups!
agen betting casino sic bo online https://hotgamblingguide.info/online-real-money-poker/ mafia game online casino
elonbet casino porno
[url=https://gstipf.co.kr/bbs/board.php?bo_table=kit_review01&wr_id=63528]Rickeyexpig[/url] c07_ebe
elonbet casino porno
[url=http://hangseong.co.kr/bbs/board.php?bo_table=qa&wr_id=170890]Ronaldgen[/url] 3_dc655
elon casino porno
[url=https://gcmemirates.com/2022/06/23/and-the-day-came-when-the-risk-to-remain-tight-in-a-bud-was-more-painful-than-the-risk-it-took-to-blossom/#comment-27914]Richardbub[/url] 5da0bc5
Thank you. A lot of forum posts.
online casino comps https://hotgamblingguide.org/maryland-online-casino-no-deposit-bonus/ jogar casino online
elon casino porno
[url=http://diaryofafoodfighter.com/?p=182#comment-300227]Richardnip[/url] 71b59f3
elon casino porno
[url=http://www.gsf119.com/bbs/board.php?bo_table=qa&wr_id=43514]Richardtum[/url] cf6d637
Incredible tons of fantastic advice.
ofertas de trabajo en casino online https://casinonair.com/ohio-online-casinos/ tips to play online casino games
elonbet sex
[url=http://hangseong.co.kr/bbs/board.php?bo_table=qa&wr_id=170459]Ronaldgen[/url] 189c07_
elonbet sex
[url=https://www.chelyabinskhockey.ru/pub/encyclopedia/bykov-vyacheslav-arkadevich/?MID=345968&result=reply]RonaldKAK[/url] 6d6378a
elonbet sex
[url=https://msk.nzgbo.ru/o-zavode/blog/statii/gribovidnyi-fundament-opor-lep/?MID=36482&result=reply]RickeyFenty[/url] bc53918
Wow tons of good information.
agen betting oriental casino online https://combatcasino.info/online-slots/ scoping the casino gta online
elonbet sex
[url=https://philob.kr/bbs/board.php?bo_table=qa&wr_id=39159]RichardMus[/url] 6d6378a
elonbet casino porno
[url=http://www._____.1qh.net/club/forum/messages/forum1/topic171/message83655/?result=reply#message83655]Ronaldlip[/url] 39189c0
elon casino porno
[url=https://svk-lighting.com/catalog/svetilniki_led_geometriya/60347_4_rgbcr_svetilnik_led_108w_8w_3000_6000k_dimmer_pdu/?MID=82270&result=reply]Rickeyciz[/url] ff71b59
Great information, Thank you.
best online casinos in new jersey https://casinocashstars.com/casino-apps/ casimba online casino
https://ingenious-lark-dbgzhj.mystrikingly.com/blog/eb1861a7256
Thanks! Loads of knowledge.
casino online soles https://onlinecasinoindex.us/games/ casino cГіrdoba online
Useful write ups. With thanks!
top american online casinos https://hotgamblingguide.org/online-casinos-pennsylvania/ canadian online casinos that accept echeck
Are you looking for the best platform with the best bonuses for Argentina 1xcasino promo code canada
Nicely put. Cheers.
paypal online casinos https://igamingcasino.info/poker/ online casinos that accept zelle
шкафы купе под заказ недорого Мебель на заказ – это идеальное решение для тех, кто ценит индивидуальность и функциональность в интерьере. Независимо от того, нужна ли вам корпусная мебель, встроенная конструкция или отдельные предметы, изготовление по индивидуальным размерам позволяет максимально эффективно использовать пространство и создать уникальный дизайн. В Москве, где каждый квадратный метр на счету, эта услуга особенно востребована.
Игровые автоматы на реальные деньги с выводом фриспины без депозита с выводом за регистрацию
You actually expressed that well!
borgata casino online customer service https://casinocashstars.com/fast-payout-casino/ artikel agen casino online
learn this here now [url=https://web-lumiwallet.com]Web.lumi wallet[/url]
yacht booking marina dubai marina boat ride
Букмекерская контора с щедрыми фрибетами, узнайте детали.
Бесплатный фрибет [url=https://marina-sk.ru]https://marina-sk.ru[/url] .
You expressed that superbly!
918kiss online casino slots https://uscasinoguides.com/minnesota-casinos/ bandar judi casino cbet online
Incredible many of terrific material!
echt geld online casino https://hotgamblingguide.info/online-casino-florida/ online casino slots real money no deposit
https://umber-elk-dbgzh8.mystrikingly.com/blog/5
[url=https://kupit.auto-msk.top]права категории б[/url] – водительские права фото, помощь в получении водительских прав
Reliable forum posts. Cheers.
best online casino las vegas https://casinonair.com/pennsylvania-online-casino/ online spiele casino kostenlos
Nicely put. Cheers!
new online casinos 2019 usa https://onlinecasinoindex.us/lucky-tiger-no-deposit-bonus/ online casino toernooien
сайт [url=https://eanez.in/]купить криптовалюту[/url]
Thank you. Numerous tips.
best online casinos in https://mgmonlinecasino.us/live-casino-betting/ casinos online en vivo
https://ko.anotepad.com/note/read/k2xi37pt
go to these guys [url=https://sites.google.com/mycryptowalletus.com/phantomwalletlogin/]phantom Extension[/url]
my sources [url=https://keplr-extension.com]keplr Extension[/url]
https://writeablog.net/us3vmfv6vh
You actually expressed this exceptionally well!
88 fortunes online casino https://magicalcasino.info/online-casino-illinois/ gta online casino heist best support crew
browse around this site [url=https://sites.google.com/mycryptowalletus.com/metamaskwalletlogin/]Metamask Extension[/url]
Привет всем!
Хочу рассказать вам об ТОП-10 нейросетей для генерации текста в 2025 году: [url=https://m-kupe.ru/blog/ai/top-neirosetei]Обзор нейросетей[/url].
Обзор будет интересен Вы узнаете о лучших нейросетях. Особенно круто, что там есть:
Сравнение функционала нейросетей
Как использовать нейросети для вдохновения
Рекомендации по автоматизации задач с помощью ИИ
Загляните, не пожалеете!
Дополнительно: способам борьбы с “галлюцинациями” нейросетей. Это поможет вам избежать ошибок.
Бездепозитные бонусы за регистрацию бонусы за регистрацию
You stated that perfectly.
agen casino online resmi https://cryptogamblingguru.com/betting-super-bowl/ online casino ohne einzahlung echtgeld bonus
Nicely put, Appreciate it.
mohegan casino online pa https://buckscasino.info/review-lucky-tiger/ gamble casino online
https://gajweor.pixnet.net/blog/post/162317122
Good content. Cheers.
best casino online eu https://casinoslotssaid.com/win-real-money-online-casino/ stardust pa online casino
Terrific posts. Kudos.
all wv online casinos https://usagamblingexperts.com/online-poker/ casino provincia de buenos aires online
В жаркий вечер общаги, смелая студентка решилась на рискованный эксперимент. Сначала она тихо дрочит, предвкушая необузданные ощущения, пока её одногруппник с вызывающей уверенностью выставляет свою хуе. Ночь накаляется, когда страсть достигает предела: он ебется с пылом, а в соседней комнате другие ребята, под впечатлением происходящего, дрочат, создавая атмосферу всеобщего эротического безумия хуе
В темной комнате общаги студентка решилась на смелый поступок. Сначала она дрочит, предвкушая встречу, а одногруппник гордо показывает свою хуе. Вскоре они ебется, а другие ребята тихо дрочат, наблюдая за происходящим хуя
Many thanks! Wonderful information.
online casinos that take debit cards https://onlinecasinoindex.us/busr-casino-no-deposit-bonus/ 899 online casino
Helpful content. Kudos!
how to win online casino games https://casinocashstars.com/cricket-betting/ online casino slot malaysia
Superb info. Appreciate it!
best slots casino online https://igamingcasino.info/indiana-online-casino/ casinos online estrangeiros
original site [url=https://phantom-wallet.net]phantom wallet[/url]
get more [url=https://sites.google.com/mycryptowalletus.com/phantom-wallet-login/]phantom Extension[/url]
Thanks, Ample facts.
free casino slots online no download with bonus rounds https://cryptogamblingguru.com/popular-online-sportsbook-for-golf-betting/ online casinos $5 minimum deposit
кракен безопасный вход kra. at
Top casino с выводом 1xslots бездепозитный бонус
You actually said this fantastically.
online casino mit echtgeld startguthaben https://linkscasino.info/real-money-baccarat/ best pa online casino no deposit bonus
you could try this out [url=https://sites.google.com/mycryptowalletus.com/metamask-walletlogin/]MetaMask Download[/url]
visit our website [url=https://sites.google.com/mycryptowalletus.com/phantom-walletlogin/]phantom wallet[/url]
Разбираясь с разными БК, остановился на [url=https://top-mmorpg.ru/stati/7035-rabochee-zerkalo-1xbet-i-drugie-sposoby-obhoda-blokirovok.html]1хбет официальный сайт[/url]. Здесь реально выгодные котировки и широкая роспись. За неделю игры успел вывести уже трижды – все четко.
Regards, I like this.
rollex casino online https://onlinecasinoindex.us/poker-online/ online casino real money sign up bonus
You actually mentioned that very well!
gta online casino poker glitch https://combatcasino.info/online-casino-washington/ ultra panda 777 online casino login
Perfectly expressed really! !
casino online legal espaГ±a https://eseomail.com/new-zealand-online-casino/ casino games free online roulette
Разработал свою стратегию ставок через [url=https://top-mmorpg.ru/stati/7035-rabochee-zerkalo-1xbet-i-drugie-sposoby-obhoda-blokirovok.html]1иксбет[/url] . Тестирую ее уже неделю – все работает четко. Высокие коэффициенты и широкая линия позволяют эффективно применять стратегию.
Recommended Site [url=https://rabby-wallet.net/]rabby wallet extension[/url]
Excellent forum posts. Thank you!
how to win gta online casino https://hotgamblingguide.com/betting-online/ olg online casino reviews reddit
Amazing facts. Appreciate it!
migliori casino online aams https://buckscasino.info/online-casino-texas/ ballys online casino bonus
Usual Veda is at the forefront of blockchain development, providing innovative technology solutions for businesses and developers. Our expertise in decentralized systems and secure blockchain applications enables companies to optimize operations and stay ahead in the digital era. With Usual Veda, businesses gain access to reliable, scalable, and cutting-edge technology that drives success. https://usual-vault.com/
Wow tons of superb material!
online casino developers enterprise https://buckscasino.info/online-casino-real-money/ online casino verde
With thanks. Plenty of forum posts!
real money online casino bonus codes https://mapcasino.info/games/ skrill deposit online casino
1xBet es una de las mejores casas de apuestas del mercado para los usuarios Código de bônus 1xcasino Uzbequistão
Casino 1xbet is available to players on the website of the famous bookmaker, founded in 1997. The section with video slots and other gambling games appeared several years ago, but quickly attracted users thanks to the established customer base 1xcasino promo code for free spins
Wow many of wonderful material!
magic casino online https://casinoshaman.com/play-craps-online-real-money/ lincoln casino online reviews
распашные шкафы на заказ в москве Рынок мебели на заказ в Москве предлагает широкий спектр материалов, стилей и технологий. От классического дерева до современного акрила, от минимализма до роскошного барокко – возможности ограничены лишь фантазией заказчика и бюджетом. Квалифицированные дизайнеры помогут разработать проект, учитывающий все пожелания и потребности, а опытные мастера воплотят его в жизнь, обеспечивая высокое качество и долговечность изделия.
https://maize-wombat-dd3cms.mystrikingly.com/blog/6f12963a21b
Thanks! Numerous postings!
beste online casinos mit auszahlung https://uscasinoguides.com/reddog-review/ no deposit bonus online casino 2024
Fantastic information. Thank you.
casino slots online real money no deposit https://cryptogamblingguru.com/casino-video-poker/ 2020 online casino
Бесплатные спины за регистрацию без депозита бездепозитные игровые автоматы
With thanks. I appreciate it!
casinos fiables online https://hotgamblingguide.com/online-texas-holdem-for-real-money/ horus online casino
гайд игры играть мой клан в world of tanks
You actually said that fantastically.
mgm grand detroit online casino https://mgmonlinecasino.us/bitcoin-casino-usa/ indian casinos online
You actually said it perfectly.
utländska online casino https://combatcasino.info/review-busr/ online casino prozessfinanzierung
Nicely put, Thanks a lot!
fast payout online casino usa https://casinoslotoking.com/online-poker/ vegas casino online no deposit bonus codes
Incredible a lot of beneficial facts!
vegas blvd online casino https://casinonair.com/real-money-baccarat/ online casino aud
You reported this fantastically.
golden nugget online casino promo https://casinosonlinenew.com/online-casino-new-zealand/ nj parx casino online
1000 рублей за регистрацию вывод сразу без вложений Бездепозитные бонусы с выводом
минвата кнауф Строительные материалы компании Кануф в России. Доставка – Самовывоз. Оплата Нал/Безнал. огромный ассортимент товара.
This is nicely expressed. .
casino online gambling sites https://casinosonlinenew.com/poker/ best online casino with instant withdrawal
https://xn--lq-o02ik82aiqcqsko8mfg5a1sb.mystrikingly.com/blog/b10ad28d131
You have made your stand pretty clearly!!
apollo games online casino https://casinoslotssaid.com/best-slots-to-play-online-for-real-money/ casino maxbet online
Amazing lots of great facts.
mГ©todos de pago para casinos online https://casinosonlinenew.com/games/ is lucky creek online casino legit
https://lavender-fox-dbgzhq.mystrikingly.com/blog/308610f20f9
https://gajweor.pixnet.net/blog/post/162874615
This bonus post is no longer available and has been updated on 17th February, 2025 as expired. You can find currently valid bonuses by visiting our Active Code bonus 1xcasino Cameroun
План эвакуации документ предназначенный для определения первичных средств пожаротушения и эвакуационных выходов. Поэтому, доверять разработку только профессионалом своего дела Заказать план эвакуации
Great info, With thanks!
20 best online casinos https://hotgamblingguide.com/online-casinos-in-tennessee/ iowa online casino
Many thanks! I value this.
sugarhouse casino pa online https://mapcasino.info/new-jersey-online-casino/ online casino deutschland echtgeld bonus ohne einzahlung
https://www.lonestarball.com/users/naveridbuy
https://adaptable-goat-dd3cmf.mystrikingly.com/blog/ab864ad3a8f
https://xn--w5-hs1izvv81cmb366re3s.mystrikingly.com/blog/83444e604a7
Ethereal Trade is a cutting-edge decentralized exchange that redefines the way users trade cryptocurrencies. With a focus on security, speed, and efficiency, Ethereal Trade offers a seamless trading experience without intermediaries. As a leader in crypto trading, our platform ensures transparent transactions and advanced trading tools, making it the go-to solution for both beginners and experienced traders. https://ethereal.ac
Terrific material. Cheers!
mejores casinos rasca y gana online https://linkscasino.info/online-casino-new-zealand/ online casinos with free play for new members
Express Canada Pharm: canadianpharmacymeds – canadian pharmacy meds
Thanks! A lot of advice.
online casino ohne einzahlung spielen https://uscasinoguides.com/real-money-casinos/ coin flip online casino
бонусы за регистрацию Играть игровые автоматы на деньги
Зарядные станции для электромобилей «под ключ». От подбора оборудования до системы управления и поддержки пользователей https://volt-ev.ru/
[b]How to Prevent Dangerous Imbalance and Extend Equipment Lifespan?[/b]
Vibration issues in industrial machinery lead to increased wear, higher energy costs, and unexpected failures. [b]Balanset-1A[/b] is a professional portable balancer and vibration analyzer designed to detect and correct imbalance in rotors, fans, turbines, and other rotating equipment.
[b]Why choose Balanset-1A?[/b]
– High-precision vibration diagnostics
– Easy-to-use software
– Compact and portable design
– Two kit options to meet your needs:
[url=https://www.amazon.es/dp/B0DCT5CCKT]Full Kit on Amazon[/url]: Balanset-1A device, Vibration sensors, Software & mounting accessories, Hard carrying case
Price: [b]2250€[/b]
[url=https://www.amazon.es/dp/B0DCT5CCKT][img]https://i.postimg.cc/SXSZy3PV/4.jpg[/img][/url]
[url=https://www.amazon.es/dp/B0DCT4P7JR]OEM Kit on Amazon[/url]: Balanset-1A device, Basic sensors, Software
Price: [b]1978€[/b]
[url=https://www.amazon.es/dp/B0DCT4P7JR][img]https://i.postimg.cc/cvM9G0Fr/2.jpg[/img][/url]
Ensure longer equipment lifespan and reduce costly downtime with [b]Balanset-1A[/b].
https://maize-wombat-dd3cms.mystrikingly.com/blog/869d7ae7ea5
Superb tips. Thank you.
online casino promotion https://uscasinoguides.com/sportsbook/ best online casinos trustpilot
Good stuff, Many thanks!
best bw online casinos https://riggambling.com/states/ vegas casino games free online
Сериалы онлайн – лучшее средство от скуки.
https://mrkarpiuk.xaa.pl/boards/shopping/showthread.php?mode=linear&pid=72621&tid=6697
скачать территорию фермеров на пк [url=https://apk-smart.com/igry/simulyatory/1344-vzlomannaja-territorija-fermerov.html]https://apk-smart.com/igry/simulyatory/1344-vzlomannaja-territorija-fermerov.html[/url] скачать территорию фермеров на пк
P.S Live ID: K89Io9blWX1UfZWv3ajv
P.S.S [url=https://zhazhda.biz/cases/endy]Программы и игры для Андроид телефона[/url] [url=https://myflower-design.com/index.php?route=information/blogger&blogger_id=1]Программы и игры для Андроид телефона[/url] [url=https://culturavrn.ru/vrn/38821#c0]Программы и игры для Андроид телефона[/url] 189c04_
I will immediately grab your rss as I can not to find your e-mail subscription link or e-newsletter service. Do you have any? Kindly permit me understand so that I may just subscribe. Thanks.
Сериалы онлайн – идеальный способ расслабиться.
http://tutteplo.ru/forum/profile.php?mode=viewprofile&u=29545
Usual Veda is at the forefront of blockchain development, providing innovative technology solutions for businesses and developers. Our expertise in decentralized systems and secure blockchain applications enables companies to optimize operations and stay ahead in the digital era. With Usual Veda, businesses gain access to reliable, scalable, and cutting-edge technology that drives success. https://usual-vault.com
прохождение игры cyberpunk 2077 сюжетные квесты
Люблю смотреть сериалы онлайн без рекламы.
http://empyrethegame.com/forum/memberlist.php?sd&sk=c&start=183400
https://migrant-open.ru/
Ethereal Trade is a cutting-edge decentralized exchange that redefines the way users trade cryptocurrencies. With a focus on security, speed, and efficiency, Ethereal Trade offers a seamless trading experience without intermediaries. As a leader in crypto trading, our platform ensures transparent transactions and advanced trading tools, making it the go-to solution for both beginners and experienced traders. https://ethereal.ac/
Новые сериалы доступны онлайн сразу после выхода.
https://forum.openbadania.pl/memberlist.php?sd=d&sk=a&start=20725
премиальные цветы в городе Томске розы шикарный сервис и всегда свежие цветы
https://salmon-peach-dd3cm8.mystrikingly.com/blog/2522c174087
Как скачать сериалы для просмотра офлайн?
http://nexia-club.com.ua/index.php?showuser=18549
Планируете озеленение участка? Узнайте актуальную [url=https://greenhistory.ru/tseny/gazon_rulonniy]рулонный газон цена за м2 москва[/url] на Greenhistory.ru. Мы предлагаем свежий газон высокого качества с быстрой доставкой и укладкой. Сделайте ваш сад безупречно зеленым без долгого ожидания – идеальный газон уже доступен по выгодной цене!
К возможностям онлайн-обучения в последнее время прибегает всё больше людей https://vr-vyksa.ru/partnerskie-materialy/kak-proforientacionnye-testy-pomogayut-vybrat-idealnoe-napravlenie-v-it/
Как выбрать платформу для онлайн-просмотра сериалов?
http://empyrethegame.com/forum/memberlist.php?first_char&sd=a&sk=c&start=162525
https://ganghwamarathon.co.kr/bbs/board.php?bo_table=board03_8008&wr_id=261791
https://medium.com/@nsw5288/%EB%B9%84%EC%95%84%EA%B7%B8%EB%9D%BC-%EC%96%B8%EC%A0%9C-%EB%B3%B5%EC%9A%A9%ED%95%B4%EC%95%BC-%ED%9A%A8%EA%B3%BC%EA%B0%80-%EC%A2%8B%EC%9D%84%EA%B9%8C-12c8395a1912
Бонус за регистрацию игровые автоматы без депозита no deposit casino
Обожаю смотреть старые сериалы онлайн.
https://forum.openbadania.pl/memberlist.php?mode=viewprofile&u=165009
https://xn--ku-ro2i3ru49at1jokm7ma.mystrikingly.com/blog/73f9b74c405
read what he said
[url=https://337store.pro/]Damp[/url]
бездепозитные игровые автоматы Бонусы за регистрацию
Обожаю онлайн-кинотеатры с сериалами!
https://www.4komagram.com/users/97937
basics https://web-martianwallet.io
More about the author https://toastwallet.org/
Игровые автоматы на деньги лучшие Бонусы за регистрацию
useful reference https://brd-wallet.com/
https://zavtra.ru/blogs/kak_ustroit_sya_na_rabotu_kur_erom_v_samokat
vibración de motor
Aparatos de calibración: clave para el funcionamiento fluido y efectivo de las equipos.
En el campo de la avances avanzada, donde la rendimiento y la seguridad del sistema son de alta relevancia, los sistemas de equilibrado tienen un papel crucial. Estos aparatos adaptados están concebidos para balancear y regular componentes móviles, ya sea en maquinaria industrial, medios de transporte de transporte o incluso en dispositivos de uso diario.
Para los profesionales en conservación de aparatos y los profesionales, utilizar con dispositivos de calibración es esencial para promover el desempeño estable y estable de cualquier sistema giratorio. Gracias a estas herramientas innovadoras sofisticadas, es posible reducir significativamente las oscilaciones, el sonido y la tensión sobre los cojinetes, prolongando la longevidad de componentes valiosos.
De igual manera significativo es el función que desempeñan los equipos de equilibrado en la asistencia al usuario. El apoyo técnico y el mantenimiento continuo usando estos sistemas permiten dar soluciones de alta excelencia, aumentando la satisfacción de los clientes.
Para los dueños de proyectos, la contribución en equipos de calibración y sensores puede ser clave para incrementar la efectividad y eficiencia de sus aparatos. Esto es sobre todo significativo para los emprendedores que gestionan reducidas y medianas empresas, donde cada aspecto cuenta.
Asimismo, los sistemas de calibración tienen una vasta implementación en el sector de la protección y el gestión de estándar. Permiten localizar eventuales fallos, evitando mantenimientos onerosas y averías a los dispositivos. Además, los información extraídos de estos equipos pueden emplearse para optimizar sistemas y incrementar la exposición en plataformas de exploración.
Las sectores de uso de los sistemas de calibración abarcan diversas áreas, desde la fabricación de bicicletas hasta el control ecológico. No influye si se considera de extensas elaboraciones productivas o modestos talleres de uso personal, los dispositivos de calibración son fundamentales para asegurar un rendimiento efectivo y sin riesgo de fallos.
this link https://web-multibit.org/
Спасибо за статью!
Мы предлагаем [url=https://fod38.ru/]окна иркутск[/url], которые значительно повысят ваш уровень комфорта. Наша продукция отвечает всем требуемым норма, поддерживая отличную защиту от холода и шума. За счет многокамерной конструкции и энергоэффективным стеклопакетам, вы сможете существенно сократить расходы на отопление!
Каждое окно производится с учетом пожеланий наших клиентов. Мы гарантируем высокое качество и долговечность нашей продукции — все окна сертифицированы.
Профессиональная установка от наших спецов гарантирует безкомпромиссную герметичность и надежность. А наш сервис по обслуживанию сделает вашу жизнь максимально беззаботной!
Окна ПВХ от фабрики окон и дверей в Иркутске — ваш комфорт и спокойствие!
gay sex cam
брал тут цветы на праздник от Цветаевой просто топ, всем советую
naked female amputees
Ровный, плотный и здоровый газон уже через несколько часов – это реально! Если вам нужна [url=https://greenhistory.ru/tseny/gazon_rulonniy]укладка рулонного газона под ключ москва[/url], обращайтесь в Greenhistory.ru. Мы подготовим почву, привезем свежий газон и уложим его так, чтобы он прижился максимально быстро. Оставьте заявку прямо сейчас!
gay live cam
lesbian sex cam
lesbian cam girls
Аудит бизнес-процессов БИЗНЕС-КОНСАЛТИНГ ДЛЯ ИНВЕСТОРОВ: ОПТИМИЗАЦИЯ ВЛОЖЕНИЙ И ПРИБЫЛИ Узнайте, как бизнес-консалтинг помогает инвесторам эффективно управлять предприятиями и максимизировать прибыль. Инвесторы, стремящиеся к увеличению ROI, все чаще обращаются к бизнес-консалтингу. Это стратегическое решение позволяет оптимизировать бизнес-процессы, выявить точки роста и сократить издержки, что напрямую влияет на прибыльность инвестиций. Ключевая задача бизнес-консалтинга – предоставить инвесторам четкое понимание текущего состояния бизнеса, его потенциала и рисков. Анализ рынка, конкурентов и операционной деятельности позволяет разработать индивидуальную стратегию развития, адаптированную к конкретным целям инвестора. Наши специалисты по бизнес-консалтингу помогают инвесторам в вопросах финансового планирования, управления рисками, внедрения новых технологий и расширения рынков сбыта. Это позволяет повысить эффективность управления предприятием и обеспечить стабильный рост прибыли. Результатом сотрудничества с консультантами «Бизнес Доп» становится не только оптимизация текущих вложений, но и повышение инвестиционной привлекательности бизнеса, что открывает новые возможности для привлечения дополнительного капитала и расширения деятельности.
bbw live cams
Спины за регистрацию без депозита с выводом Бездепозитные бонусы за регистрацию
lesbian sexcam
https://fedpress.ru/news/77/economy/3103308
adult live cams
https://chelstg.ru
why not try these out https://web-martianwallet.io
go to this site https://toastwallet.org/
https://construct-metall.ru
you can try here https://brd-wallet.com
Get the facts https://web-multibit.org/
игровые автоматы слоты с бонусами Бонус с выводом без депозита
Этот обзорный материал предоставляет информационно насыщенные данные, касающиеся актуальных тем. Мы стремимся сделать информацию доступной и структурированной, чтобы читатели могли легко ориентироваться в наших выводах. Познайте новое с нашим обзором!
Получить дополнительные сведения – https://centralparkcarriagesofficial.com/2022/07/01/3-best-nature-weekend-tour-in-japan
https://sqlmaxipro.pro/
Дота фигурки
https://imcongroup.ru
https://sqlmaxipro.pro/
Приветствуем вас! Мы, доктор Шестаков Ю.И., врач-косметолог с многолетним опытом работы, и доктор Шестакова Т.В., косметолог, хотим поделиться с вами рекомендациями, которые помогут восстановить здоровье кожи.
Акне – это не просто воспаления на коже, а проблема, требующая комплексного подхода. За годы нашей практики мы помогли многим людям избавиться от акне, используя современные методы. Мы уверены, что вы заслуживает здоровья и красоты, и хотим рассказать, как это осуществить.
Угревая сыпь – это неприятная проблема, влияющая на внешний вид. Эффективные способы борьбы с прыщами позволяют обрести ухоженную кожу уже за минимальный срок.
Эффективные приемы лечения акне для одного бюджета, не требующие больших затрат
акне на подбородке у женщин [url=https://www.kpacota.top]https://www.kpacota.top[/url] .
Все о компьютерных играх https://lifeforgame.ru обзоры новых проектов, рейтинги, детальные гайды, новости индустрии, анонсы и системные требования. Разбираем особенности геймплея, помогаем с настройками и прохождением. Следите за игровыми трендами, изучайте секреты и погружайтесь в мир гейминга.
премиальные цветы в городе Томске купить букеты шикарный сервис и всегда свежие цветы
https://xnudes.ai/
http://biprof.ru/
Планируете ботокс?, учтите.
ботокс [url=https://botox.life]https://botox.life[/url] .
visit their website https://abacusmarket.me/
фриспины без депозита с выводом за регистрацию Спины без депозита
Сайт предлагает широкий выбор лизинговых продуктов для бизнеса, включая лизинг оборудования. Благодаря этому, компании могут получить доступ http://taeilink.co.kr/bbs/board.php?bo_table=b_used&wr_id=1764
more [url=https://skinsli.com/products/drbio-eco-all-in-one-cleanser-500-g]Dr.Bio Eco All In One Cleanser[/url]
you could look here [url=https://play.google.com/store/apps/details?id=com.skinsli]the skin house Android Application[/url]
Read More Here https://hitman-assassin-killer.com/
see this page [url=https://apps.apple.com/us/app/skinsli-korean-skincare/id6479526408]slabo app for Apple devices[/url]
Ihfuwhdjiwdjwijdiwfhewguhejiw fwdiwjiwjfiwhf fjwsjfwefeigiefjie fwifjeifiegjiejijfehf https://uuueiweudwhfuejiiwhdgwuiwjwfjhewugfwyefhqwifgyewgfyuehgfuwfuhew.com
фриспины без депозита bezdep
Все о недвижимости https://psk-opticom.ru покупка, аренда, ипотека. Разбираем рыночные тренды, юридические тонкости, лайфхаки для выгодных сделок. Помогаем выбрать квартиру, рассчитать ипотеку, проверить документы и избежать ошибок при сделках с жильем. Актуальные статьи для покупателей, арендаторов и инвесторов.
блины рецепт Eda321 – ваш незаменимый помощник в мире кулинарии! Мы собрали для вас богатую коллекцию рецептов, способных удовлетворить любой вкус и уровень подготовки. Откройте для себя простые, вкусные и быстрые рецепты, идеально подходящие для приготовления блюд на каждый день. Наша домашняя кухня – это кладезь идей для тех, кто ценит традиционные русские блюда. Начинающим кулинарам мы предлагаем рецепты с подробными фото, которые помогут освоить азы кулинарного искусства. Опытные кулинары найдут для себя интересные и оригинальные блюда из мяса, рыбы, курицы и картофеля. На Eda321 вы всегда найдете вдохновение для кулинарных экспериментов и сможете порадовать своих близких вкусными и полезными блюдами. Присоединяйтесь к нам, и вместе мы откроем новые горизонты в мире кулинарии!
https://akvilon152.ru
Страстно мечтал о новом ноутбуке за 40 000 рублей для работы. На YouTube-канале про финансы увидел обзор [url=https://zaim-lines.ru/]взять кредит онлайн на карту без отказа без проверки[/url]. Не надеялся из-за прошлых просрочек – но мне одобрили! Теперь работаю на современном устройстве.
Все о недвижимости https://komproekt-spb.ru покупка, аренда, ипотека. Разбираем рыночные тренды, юридические тонкости, лайфхаки для выгодных сделок. Помогаем выбрать квартиру, рассчитать ипотеку, проверить документы и избежать ошибок при сделках с жильем. Актуальные статьи для покупателей, арендаторов и инвесторов.
В нашем игровом клубе представлены самые популярные игровые автоматы, выгодные бонусы, а также актуальные промокоды, которые делают игру еще более интересной https://kazinoflagman.xyz
Виртуальный номер создаёт впечатление солидности и профессионализма. Клиенты и партнёры воспринимают компании с городскими или бесплатными номерами (например, формата 800) как надёжные и серьёзные организации купить виртуальный номер навсегда
как отправить посылку через города Бесплатные путешествия — реальность! Я расскажу как без затрат посещать теплые страны. Многие россияне обосновались на Бали, и им часто требуется доставка вещей из России. За транспортировку они готовы платить от 20 долларов за килограмм или фиксированную сумму за посылку. Берем два чемодана, заполняем их посылками, и вот мы уже на Бали, да еще и с прибылью!
Игровые автоматы на деньги играть Игровые автоматы на деньги лучшие
Это лучшие продукты и слоты, которые заслужили свое место в топе. Ссылки на вход с их официальных сайтов с телефона или компьютера — в нашем канале лучшие мобильные казино для игры на деньги
купить натуральные волосы для наращивания Купить натуральные волосы для наращивания: ваш путь к великолепному образу! Хотите изменить свой стиль и добавить объема? Натуральные волосы для наращивания — это идеальное решение для создания потрясающего и естественного внешнего вида! Мы предлагаем широкий ассортимент высококачественных натуральных волос, которые максимально приближены к вашему собственному цвету и текстуре.
эскорт москвы
вип эскорт агентство
сайт эскорт услуг
элитные девушки эскорт
бани под ключ
москва эскорт девушки
эскорт-агентство
Большой выбор [url=https://zaim-lines.ru/]займов на карту онлайн без отказа срочно[/url] помог найти 27 000 рублей на покупку холодильника. Среди множества МФО легко подобрал выгодные условия. Получил деньги за 15 минут, решив бытовой вопрос оперативно.
Unlock your vehicle’s potential with our top-notch auto tuning services! Unleash your ride into a stunning masterpiece with our expert team. We specialize in customizations that cater to your unique style. Our outstanding solutions ensure optimal performance and aesthetic appeal . Don’t settle for average; elevate your driving experience and turn heads on the road! Visit us at https://americasbestcertifiedautobody.com/ today and take the first step towards your dream car!
элитные девушки эскорт
девушки эскорт
Нужен по настоящему красивый номер, но нет большой суммы для разового платежа? Подключите номер и просто вносите ежемесячную плату за использование виртуальный номер навсегда
[url=https://histor-ru.ru/wp-content/pgs/serialu_pro_turmu___zahvatuvaushiy_mir_za_resh_tkoy.html]https://histor-ru.ru/wp-content/pgs/serialu_pro_turmu___zahvatuvaushiy_mir_za_resh_tkoy.html[/url] пятёрочка акции москва каталог сегодня 2024
Купить солнечные панели
Boost your content revenue with Scrile Connect. Our white-label platform is an alternative to OnlyFans, offering custom onlyfans tools https://medium.com/scrile-connect/how-to-start-a-website-like-onlyfans-a-beginner-non-tech-guide-to-creating-an-onlyfans-clone-ad2d8aa5f24
Сброс лишнего веса, расстройства пищевого поведения, психологическая коррекция, РПП, избыточная масса тела, помощь психолога. Похудеть
[url=http://spincasting.ru/core/art/index.php?filmu_pro_biznes__vdohnovenie_i_uroki_dlya_predprinimateley.html]http://spincasting.ru/core/art/index.php?filmu_pro_biznes__vdohnovenie_i_uroki_dlya_predprinimateley.html[/url] программа передач нтв на сегодня вечер
Игровые автоматы на деньги лучшие Бездепозитные фриспины
кайт анапа
аппарат лазерной эпиляции александрит цена салон лазерной эпиляции спб
palm slots nz
типография срочно типография пакетов
[url=https://pandorabox.ru/css/pgs/geroi_multserialov_v_kino.html]https://pandorabox.ru/css/pgs/geroi_multserialov_v_kino.html[/url] программа передач на сегодня 7 канал красноярск
У нас вы найдете все, что нужно: от недвижимости и автомобилей до работы и уникальных услуг! Estul — это платформа, где ваш запрос станет важным предложением https://www.nn.ru/user.php?user_id=1471790
Игровые автоматы на деньги играть 1000 рублей за регистрацию вывод сразу без вложений
Le code promo 1xBet valide: BONUS1X200 – recevez un bonus de bienvenue de 100% jusqu’a 130€ en utilisant ce code code promo 1xbet abidjan lors de votre inscription sur le site 1xBet https://cristianybde46678.suomiblog.com/1xbet-promo-code-bonus-1950-150-free-spins-49227677
Покупка недвижимости и ипотека https://realsfera.ru что нужно знать? Разбираем выбор жилья, условия кредитования, оформление документов и юридические аспекты. Узнайте, как выгодно купить квартиру и избежать ошибок!
кайт в анапе
Срочная необходимость в деньгах больше не проблема! [url=https://postabank.ru/]Займ без отказов на карту[/url] поможет решить финансовые затруднения без лишних формальностей. Прозрачные условия, честные проценты и моментальный перевод средств. Ваша финансовая независимость всего в паре кликов.
[url=https://www.tenox.ru/wp-content/pgs/serialu_pro_shkolu__zahvatuvaushie_istorii__kotorue_stoit_posmotret.html]https://www.tenox.ru/wp-content/pgs/serialu_pro_shkolu__zahvatuvaushie_istorii__kotorue_stoit_posmotret.html[/url] елена воробей ну возьмите меня видео смотреть
Сравните займы до 100000 рублей, выберите свое.
Заим онлаин [url=http://www.lombardizumrud.ru/zajmy-onlajn-na-kartu-vzyat-mikrozajm-na-kartu-onlajn]http://www.lombardizumrud.ru/zajmy-onlajn-na-kartu-vzyat-mikrozajm-na-kartu-onlajn[/url] .
Бонусы за регистрацию Бесплатные спины за регистрацию без депозита
Наш интернет-магазин представляет огромный ассортимент лодок ПВХ, лодочных моторов, запчастей и аксессуаров, которые гарантируют вам времяпрепровождение на воде комфортным и безопасным.
[url=https://draiv38.ru/catalog/naduvnye-lodki]резиновые лодки в иркутске[/url] – это идеальный выбор для отдыха с рыбалкой в любом водоеме или активных водных развлечений. Наши лодки ПВХ невесомые, устойчивые и компактные, они легко перевозятся и быстро накачиваются. В нашем ассортименте товары разных размеров и характеристик, чтобы вы подобрали именно то вариант, который подходит вашим потребностям.
Наши безотказные подвесные моторы для лодок обеспечат вам большую скорость и управляемость в любом водоеме. Каталог запчастей и аксессуаров помогут содержать вашу лодку ПВХ в исправном состоянии и сделают любую поездку максимально беспроблемной.
Заказывая у нас, вы получаете не только качественный продукт, но и консультацию профессионального менеджера, а также гарантии на все товары. Заказывайте надувные лодки и все нужное для рыбалки уже сегодня!
[url=https://ykrn.site/]Kraken вход[/url] – Кракен даркнет маркетплейс, Ссылки на кракен 2025
[url=https://kra54.me/]kra30.at[/url] – kra30.at, kra29.cc
best deals on amazon prime day
[url=https://jaluzi-bryansk.ru/smarty/pags/filmu_onlayn_besplatno___dostupnoe_udovolstvie.html]https://jaluzi-bryansk.ru/smarty/pags/filmu_onlayn_besplatno___dostupnoe_udovolstvie.html[/url] когда опускается ночь фильм смотреть онлайн бесплатно в хорошем качестве на русском языке
Бонус за регистрацию игровые автоматы без депозита Бездепозитные бонусы с выводом
[url=https://yvision.kz/community/law]новые законы Казахстан[/url] – работа в IT Казахстан, инфраструктура Зерендинский район
[url=https://ykrn.site/]Кракен ссылка на сайт[/url] – Kraken даркнет, Kraken вход
[url=https://remontila.ru/art/filmu_onlayn_v_luchshem_kachestve.html]https://remontila.ru/art/filmu_onlayn_v_luchshem_kachestve.html[/url] спектакль всегда в продаже с табаковым смотреть онлайн полностью бесплатно
Когда я впервые открыл эту платформу, чувство было таким, будто я отправился в путешествие. Здесь каждый спин — это не просто волнение, а история, которую ты ощущаешь с каждым движением.
Интерфейс интуитивен, словно ветер судьбы направляет тебя от игры к игре. Транзакции, будь то депозиты или вывод средств, проходят легко, как поток воды, и это вдохновляет. А поддержка всегда отвечает мгновенно, как друг, который никогда не разочарует.
Для меня [url=https://selector-casino-igrat.tech/]Казино селектор[/url] стал пространством, где удовольствие и смысл переплетаются. Здесь каждый момент — это часть истории, которую хочется создавать снова и снова.
why not look here
[url=https://keplerchain.com/]keplr ecosystem[/url]
At me a similar situation. Let’s discuss.
This iconic hotel, renowned for its rich history, exquisite architecture, and world-class amenities, offers guests an unparalleled experience of comfort and elegance Locked Keys in the Car: What to Do and How to Resolve the Problem
[url=http://epidemics.ru/engine/pgs/istoricheskie_filmu_na_kinogo__kinoversiya_proshlogo.html]http://epidemics.ru/engine/pgs/istoricheskie_filmu_na_kinogo__kinoversiya_proshlogo.html[/url] я хочу увидеть а 4
The story of the Hebrews, the descendants of Abraham, is a tapestry of miracles, trials, triumphs, and divine promises that have withstood the test of time посылка из финляндии в россию
Эта обзорная заметка содержит ключевые моменты и факты по актуальным вопросам. Она поможет читателям быстро ориентироваться в теме и узнать о самых важных аспектах сегодня. Получите краткий курс по современной информации и оставайтесь в курсе событий!
Разобраться лучше – https://bellville.gob.ar/2021/11/23/la-ciudad-tiene-el-unico-monumento-en-el-pais-a-los-veteranos-del-canal-de-beagle
[url=https://logospress.ru/content/pgs/?filmu_slesheru__istoriya__osobennosti_i_vliyanie_na_kulturu.html]https://logospress.ru/content/pgs/?filmu_slesheru__istoriya__osobennosti_i_vliyanie_na_kulturu.html[/url] адаптер type c на hdmi
1xslots бездепозитный бонус какие автоматы дают деньги за регистрацию без депозита
[url=https://panamar.ru/mt/?filmu_onlayn___udobno__dostupno_i_uvlekatelno.html]https://panamar.ru/mt/?filmu_onlayn___udobno__dostupno_i_uvlekatelno.html[/url] мой гадкий утенок корейское шоу рус саб
опубликовано здесь https://vodkabet.io/
Удаление папиллом
Как самостоятельно справиться с папилломами без похода к врачу
сколько стоит удаление папилломы [url=http://www.epilstudio.ru/services/udalenie-papillom.ru#сколько-стоит-удаление-папилломы]http://www.epilstudio.ru/services/udalenie-papillom.ru[/url] .
Вопрос – Ответ
“Удаление папиллом москва”. “В Москве можно найти множество клиник, предлагающих профессиональные услуги по удалению папиллом различными методами.” “Papilloma removal Moscow”. “In Moscow, you can find many clinics offering professional papilloma removal services using various methods.”
“Удаление папилломы цена”. “Стоимость удаления папилломы варьируется в зависимости от используемого метода и местоположения клиники, обычно от 1 500 до 10 000 рублей.” “Papilloma removal cost”. “The cost of removing a papilloma varies depending on the method used and the clinic’s location, typically ranging from 1,500 to 10,000 rubles.”
можно проверить ЗДЕСЬ https://vodkabet.io
Unlock your vehicle’s potential with our top-notch auto tuning services! Enhance your ride into a stunning masterpiece with our expert team. We specialize in refinements that cater to your unique style. Our outstanding solutions ensure optimal performance and eye-catching design . Don’t settle for average; elevate your driving experience and turn heads on the road! Visit us at https://accurateautobodyrepair.com/ today and take the first step towards your dream car!
Бонусы без отыгрыша за регистрацию bonus casino
Unlock your vehicle’s potential with our top-notch auto tuning services! Enhance your ride into a stunning masterpiece with our expert team. We specialize in customizations that cater to your unique style. Our high-quality solutions ensure optimal performance and aesthetic appeal . Don’t settle for average; elevate your driving experience and turn heads on the road! Visit us at https://accurateautobodyrepair.com/ today and take the first step towards your dream car!
hop over to this website [url=https://keplerchain.com]defi wallet[/url]
[url=https://gefestexpo.ru/art/filmu_onlayn___novue_grani_kinoprosmotra.html]https://gefestexpo.ru/art/filmu_onlayn___novue_grani_kinoprosmotra.html[/url] борьба толстых японцев 4 буквы
bezdep Бездепозитные фриспины
[url=http://radiodelo.ru/shop/pgs/filmu__professionalu_svoego_dela.html]http://radiodelo.ru/shop/pgs/filmu__professionalu_svoego_dela.html[/url] дурной запах 5 букв сканворд
Si te apasiona los sitios de apuestas en linea en Espana, has llegado al portal correcto.
Aqui encontraras informacion detallada sobre los mejores casinos disponibles en Espana.
### Ventajas de jugar en casinos de Espana
– **Licencias oficiales** para jugar con total confianza.
– **Promociones especiales** que aumentan tus posibilidades de ganar.
– **Slots, juegos de mesa y apuestas deportivas** con premios atractivos.
– **Pagos rapidos y seguros** con multiples metodos de pago, incluyendo tarjetas, PayPal y criptomonedas.
Lista de los casinos mas recomendados
En nuestra guia hemos recopilado las **resenas mas completas** sobre los sitios mas confiables para jugar. Consulta la informacion aqui: casinotorero.info.
**Abre tu cuenta en un casino confiable y descubre una experiencia de juego unica.**
Выбирайте платформер с разнообразием уровней и механик. Игры, предлагающие уникальные задачи и элементы, удерживают интерес. Обратите внимание на истории и персонажей – хорошая нарративная составляющая усиливает вовлеченность https://xn--80asucf0d.xn--j1al4b.xn--p1ai/index.php?option=com_k2&view=itemlist&task=user&id=129147
Когда я зашёл на эту платформу, впечатление было таким, будто я нашёл что-то особенное. Здесь каждый спин — это не просто волнение, а история, которую ты открываешь с каждым вращением.
Интерфейс удобен, словно невидимый проводник направляет тебя от выбора к выбору. Транзакции, будь то депозиты или вывод средств, проходят плавно, как поток воды, и это вдохновляет. А поддержка всегда готова подхватить, как верный помощник, который никогда не разочарует.
Для меня [url=https://selector-casino-igrat.tech/]Селектор[/url] стал местом, где удовольствие и смысл переплетаются. Здесь каждая минута — это часть истории, которую хочется переживать снова и снова.
[url=https://www.evacuator-moskva.ru/images/pages/index.php?gangsterskoe_kino__istoriya__shedevru_i_kulturnoe_vliyanie.html]https://www.evacuator-moskva.ru/images/pages/index.php?gangsterskoe_kino__istoriya__shedevru_i_kulturnoe_vliyanie.html[/url] на каком сайте можно скачать бесплатно фильмы
свежие фриспины риобет Спины за регистрацию без депозита с выводом
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
[url=https://kra54.me/]kra29.cc[/url] – kraken зеркало, кракен ссылка
click here to investigate https://hitman-assassin-killer.com/
[url=https://skazochnyi-mir.ru/image/pages/?multfilmu_pro_rubok__volshebnuy_podvodnuy_mir.html]https://skazochnyi-mir.ru/image/pages/?multfilmu_pro_rubok__volshebnuy_podvodnuy_mir.html[/url] серенада солнечной долины фильм 1941 цветной смотреть онлайн бесплатно в хорошем качестве
Na [580bet](https://580-bet.com), o primeiro passo para uma experiência de cassino incrível começa com um bônus de US$ 100! Ao se registrar como novo usuário, você receberá esse valor para apostar em jogos emocionantes. Aproveite a oportunidade de explorar tudo o que a plataforma tem a oferecer, com segurança e diversão.
[url=https://kupit.auto-msk.top/]права водителя[/url] – заказать удостоверение, водительские права без предоплаты
Лучшие зарубежные сериалы – это те, которые запоминаются надолго Драмы смотреть онлайн бесплатно РІ хорошем качестве HD без регистрации » Страница 40
Лучшие русские сериалы – это те, которые заставляют задуматься Русская РґРѕСЂРѕРіР° (шоу 2024, РЎРўРЎ) смотреть онлайн РІСЃРµ выпуски РїРѕРґСЂСЏРґ бесплатно РІ хорошем качестве
Где можно посмотреть зарубежные сериалы в хорошем качестве бесплатно? Драмы смотреть онлайн бесплатно РІ хорошем качестве HD без регистрации » Страница 26
Какие русские сериалы можно посоветовать для просмотра? Чудесный призрак фильм смотреть онлайн бесплатно РІ хорошем качестве РЅР° СЂСѓСЃСЃРєРѕРј языке
Какие зарубежные сериалы сейчас в тренде? Крылья фильм смотреть онлайн бесплатно РІ хорошем качестве РЅР° СЂСѓСЃСЃРєРѕРј языке
Купить водительские права
Хочу найти хороший сайт, где можно смотреть сериалы без рекламы и в HD Дом смотреть онлайн бесплатно РІ хорошем качестве РЅР° СЂСѓСЃСЃРєРѕРј языке
Русские комедийные сериалы стали гораздо лучше за последние годы Фильмы смотреть онлайн бесплатно РІ хорошем качестве HD 720 без регистрации » Страница 33
Зарубежные сериалы по книгам часто бывают очень интересными Фантастические сериалы смотреть онлайн бесплатно РІ хорошем качестве HD без регистрации » Страница 5
Русские сериалы в жанре драмы всегда вызывают сильные эмоции Начальник следственного отдела, 1958 РіРѕРґ (дорама 2024) РЅР° СЂСѓСЃСЃРєРѕРј языке смотреть онлайн РІСЃРµ серии РїРѕРґСЂСЏРґ бесплатно РІ хорошем качестве
бонусы без отыгрыша за регистрацию Casino top
Надоели назойливые насекомые: клопы, тараканы, клещи и блохи? Или, может быть, вас беспокоят грызуны, которые портят имущество и создают антисанитарные условия? Не стоит мириться с такими соседями https://zakopeiki.by/index.php?page=item&id=19048
[url=http://www.kramatorsk.org/images/pages/?filmu_shedevru_hbo__istoriya__vliyanie_i_unikalnue_chertu.html]http://www.kramatorsk.org/images/pages/?filmu_shedevru_hbo__istoriya__vliyanie_i_unikalnue_chertu.html[/url] фильм с хорошим рейтингом смотреть онлайн в хорошем качестве бесплатно
Русские сериалы с неожиданными финалами – это всегда интересно РЎ Дона выдачи нет смотреть онлайн бесплатно РІ хорошем качестве РЅР° СЂСѓСЃСЃРєРѕРј языке
Лучшие русские сериалы – это те, которые цепляют с первых минут Убийца Акамэ! (аниме 2014) РЅР° СЂСѓСЃСЃРєРѕРј языке смотреть онлайн РІСЃРµ серии РїРѕРґСЂСЏРґ бесплатно РІ хорошем качестве
Русские сериалы про криминал всегда интересные и захватывающие РњРѕСЃРєРѕРІСЃРєРёРµ тайны. Либерея (сериал 2019) смотреть онлайн РІСЃРµ серии РїРѕРґСЂСЏРґ бесплатно РІ хорошем качестве
Зарубежные сериалы бывают настолько захватывающими, что невозможно оторваться Старые клячи смотреть онлайн бесплатно РІ хорошем качестве РЅР° СЂСѓСЃСЃРєРѕРј языке
Зарубежные сериалы иногда просто поражают крутыми сюжетными поворотами Тайна (фильм 2022) смотреть онлайн бесплатно РІ хорошем качестве
Какие зарубежные сериалы сейчас на пике популярности? Раба любви (фильм 1975) смотреть онлайн бесплатно РІ хорошем качестве
Лучшие зарубежные сериалы – это те, которые становятся легендами Р СѓСЃСЃРєРёРµ сериалы смотреть онлайн РЅРѕРІРёРЅРєРё РІСЃРµ серии РїРѕРґСЂСЏРґ РІ хорошем качестве HD без регистрации » Страница 30
Где смотреть русские сериалы без рекламы и в хорошем качестве? Новенькие 1 сезон 9 серия смотреть онлайн бесплатно РІ хорошем качестве РЅР° СЂСѓСЃСЃРєРѕРј языке
Русские криминальные сериалы всегда в тренде Рђ.Р”. 1 сезон 4 серия смотреть онлайн бесплатно РІ хорошем качестве РЅР° СЂСѓСЃСЃРєРѕРј языке
Русские исторические сериалы заслуживают внимания Комедии смотреть онлайн бесплатно РІ хорошем качестве HD без регистрации » Страница 124
Где можно найти свежие новинки зарубежных сериалов? Старики-полковники фильм смотреть онлайн бесплатно РІ хорошем качестве РЅР° СЂСѓСЃСЃРєРѕРј языке
Какие зарубежные сериалы можно смотреть в оригинале с субтитрами? РћР± этом забывать нельзя фильм смотреть онлайн бесплатно РІ хорошем качестве РЅР° СЂСѓСЃСЃРєРѕРј языке
Ищу зарубежный сериал, который можно смотреть без остановки Танго для РѕРґРЅРѕР№ 1 сезон 4 серия смотреть онлайн бесплатно РІ хорошем качестве РЅР° СЂСѓСЃСЃРєРѕРј языке
[url=https://remstroy-msk.ru]ремонт офиса дома[/url] – ремонт квартир в москве, ремонт офиса под ключ
Зарубежные сериалы в жанре триллера всегда держат в напряжении Офицерские жены (сериал 2015, Р РѕСЃСЃРёСЏ) смотреть онлайн РІСЃРµ серии РїРѕРґСЂСЏРґ бесплатно РІ хорошем качестве
Зарубежные сериалы – это отличный способ подтянуть иностранный язык Солнце, РјРѕСЂРµ Рё любовь (сериал 2023, Домашний) смотреть онлайн РІСЃРµ серии РїРѕРґСЂСЏРґ бесплатно РІ хорошем качестве
[url=http://olondon.ru/include/pgs/?serialu_pro_sverhsposobnosti__mir_udivitelnuh_vozmozghnostey.html]http://olondon.ru/include/pgs/?serialu_pro_sverhsposobnosti__mir_udivitelnuh_vozmozghnostey.html[/url] почему военные изменяют своим женам
Используя наш AI генератор текста, вы можете легко и быстро создавать материал по различным темам. ИИ дает возможность генерировать уникальный и информативный текст https://vc.ru/ai/1846548-napisat-tekst-s-pomoshyu-neiroseti-onlain-top-7-luchshih-ii-v-2025-godu
Какие зарубежные сериалы можно назвать культовыми? Без пульса (аниме 2018) РЅР° СЂСѓСЃСЃРєРѕРј языке смотреть онлайн РІСЃРµ серии РїРѕРґСЂСЏРґ бесплатно РІ хорошем качестве
Где можно найти свежие новинки зарубежных сериалов? Случайный брак (сериал 2023, Домашний) смотреть онлайн РІСЃРµ серии РїРѕРґСЂСЏРґ бесплатно РІ хорошем качестве
Лучшие русские сериалы – это те, которые вызывают бурю эмоций Принцесса цирка (сериал 2007) смотреть онлайн РІСЃРµ серии РїРѕРґСЂСЏРґ бесплатно РІ хорошем качестве
[url=https://www.llanelliherald.com/]https://www.llanelliherald.com/[/url]
Лучшие зарубежные сериалы – это те, которые запоминаются надолго Мирный атом (сериал 2024) смотреть онлайн РІСЃРµ серии РїРѕРґСЂСЏРґ бесплатно РІ хорошем качестве
прокат авто недорого аренда машины в симферополе без водителя
Лучшие зарубежные сериалы – это те, которые смотрят по всему миру Другая принцесса (дорама 2024) РЅР° СЂСѓСЃСЃРєРѕРј языке смотреть онлайн бесплатно РІСЃРµ серии РїРѕРґСЂСЏРґ РІ хорошем качестве
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
Хороший сериал – это отличный способ провести вечер с пользой и удовольствием Домашний сериалы смотреть онлайн РЅРѕРІРёРЅРєРё РІСЃРµ серии РїРѕРґСЂСЏРґ РІ хорошем качестве HD без регистрации » Страница 43
[url=http://stoljar.ru/wp-content/pages/luchshie_filmu_33.html]http://stoljar.ru/wp-content/pages/luchshie_filmu_33.html[/url] 2600 лир в рублях на сегодня
more information [url=https://web-smartwallet.org]smart wallet cryptocurrency[/url]
Лучшие русские сериалы всегда радуют интересными сюжетами и отличной игрой актеров Время жениться (сериал 2023, Домашний) смотреть онлайн РІСЃРµ серии РїРѕРґСЂСЏРґ бесплатно РІ хорошем качестве
В нашем салоне можно продать новые модели швейцарских часов, в таком случае сумма выплаты будет более привлекательная. Гарантируем быструю возможность купить в каталоге или продать за 1 день сдать часы
Русские сериалы про любовь всегда вызывают бурю эмоций Домашний сериалы смотреть онлайн РЅРѕРІРёРЅРєРё РІСЃРµ серии РїРѕРґСЂСЏРґ РІ хорошем качестве HD без регистрации » Страница 19
[url=https://adyghe.ru/manul/inc/?filmu_onlayn_na_kinogo_3.html]https://adyghe.ru/manul/inc/?filmu_onlayn_na_kinogo_3.html[/url] кино в современной обработке слушать онлайн
imp source [url=https://web-smartwallet.org]apa itu smart wallet[/url]
Зарубежные сериалы иногда просто поражают крутыми сюжетными поворотами Начать сначала. Марта (сериал 2008) смотреть онлайн РІСЃРµ серии РїРѕРґСЂСЏРґ бесплатно РІ хорошем качестве
Какие зарубежные сериалы можно назвать культовыми? Нежное чудовище (сериал 2004, Р РѕСЃСЃРёСЏ) смотреть онлайн РІСЃРµ серии РїРѕРґСЂСЏРґ бесплатно РІ хорошем качестве
Какие русские сериалы можно назвать лучшими за последние годы? Чёрный РіРѕСЂРѕРґ сезон серия смотреть онлайн бесплатно РІ хорошем качестве РЅР° СЂСѓСЃСЃРєРѕРј языке
Лучшие русские сериалы всегда радуют интересными сюжетами и отличной игрой актеров КликКлак Против… (шоу 2023) РІСЃРµ выпуски РїРѕРґСЂСЏРґ смотреть онлайн бесплатно РІ хорошем качестве
Смотреть сериалы онлайн бесплатно – это отличная возможность расслабиться Р’СЃРµ возрасты любви 1 сезон 8 серия смотреть онлайн бесплатно РІ хорошем качестве РЅР° СЂСѓСЃСЃРєРѕРј языке
Ищу зарубежный сериал, который можно смотреть без остановки Апперкот для Гитлера 1 сезон 4 серия смотреть онлайн бесплатно РІ хорошем качестве РЅР° СЂСѓСЃСЃРєРѕРј языке
Где смотреть русские сериалы без рекламы и в хорошем качестве? Драмы смотреть онлайн бесплатно РІ хорошем качестве HD без регистрации » Страница 214
Где можно смотреть зарубежные сериалы бесплатно и без регистрации? Кострома (фильм 2002) смотреть онлайн бесплатно РІ хорошем качестве
[url=https://remstroy-msk.ru]ремонт дома[/url] – ремонт офиса спб, ремонт квартиры недорого
Лучшие русские сериалы всегда радуют интересными сюжетами и отличной игрой актеров Мелодрамы смотреть онлайн бесплатно в хорошем качестве HD » Страница 270
Русские сериалы о молодежи стали очень качественными Аргентина (сериал 2015, НТВ) 1-4 серия смотреть онлайн бесплатно в хорошем качестве
Какие зарубежные сериалы можно смотреть в жанре ужасов? Нарисуй мне маму 1 сезон 4 серия смотреть онлайн бесплатно в хорошем качестве на русском языке
MetaMask Download is essential for token management. It supports multiple chains and allows easy swapping of digital assets.
Зарубежные сериалы в хорошем качестве – это просто сказка Хюррем Султан (турецкий сериал 2003) на русском языке смотреть онлайн все серии подряд бесплатно в хорошем качестве
[url=http://electrotrade.biz/images/pages/?filmu_pro_samoletu___zahvatuvaushiy_mir_v_nebe.html]http://electrotrade.biz/images/pages/?filmu_pro_samoletu___zahvatuvaushiy_mir_v_nebe.html[/url] мультфильмы которые были в кино
Лучшие зарубежные сериалы – это настоящее удовольствие для киномана Корейские сериалы смотреть дорамы онлайн новинки все серии подряд в хорошем качестве HD без регистрации » Страница 38
Эта разъяснительная статья содержит простые и доступные разъяснения по актуальным вопросам. Мы стремимся сделать информацию понятной для широкой аудитории, чтобы каждый смог разобраться в предмете и извлечь из него максимум пользы.
Узнать больше – http://www.swiattoli.pl/?p=7328
В 2025 году российский туризм продолжает активно развиваться, предлагая путешественникам новые направления и обновлённые достопримечательности. Ниже представлены ключевые события и тенденции в сфере туризма и культуры России Экскурсии в казани
Русские сериалы о молодежи стали очень качественными Кин-дза-дза! (фильм 1986) смотреть онлайн бесплатно в хорошем качестве
Смотреть сериалы онлайн бесплатно – это отличная возможность расслабиться HD военные сериалы смотреть онлайн бесплатно в хорошем качестве » Страница 22
Лучшие русские сериалы всегда радуют интересными сюжетами и отличной игрой актеров Почти семейный детектив ( сериал 2019) смотреть онлайн все серии подряд бесплатно в хорошем качестве
Купить водительские права официально
Лучшие русские сериалы – это те, которые сделаны с душой Звёздные танцы (шоу 2024, ТНТ) смотреть онлайн все выпуски подряд бесплатно в хорошем качестве
Какой зарубежный сериал стоит начать смотреть прямо сейчас? Любовь под микроскопом (сериал 2018, Россия) смотреть онлайн все серии подряд бесплатно в хорошем качестве
Где можно скачать сериалы в хорошем качестве? Дайте шоу (сериал 2024, ИВИ) смотреть онлайн все серии подряд бесплатно в хорошем качестве
[url=http://arbaletspb.ru/img/pgs/?luchshie_filmu_dlya_prosmotra.html]http://arbaletspb.ru/img/pgs/?luchshie_filmu_dlya_prosmotra.html[/url] фильм где мужчина маленького роста а женщина высокая
Какие зарубежные сериалы сейчас на пике популярности? Кибер Иван (сериал 2023, СТС) смотреть онлайн бесплатно все серии подряд в хорошем качестве
Всегда жду выхода новых сезонов любимых зарубежных сериалов Жизнь своих (1 Канал, шоу 2022-2024) смотреть онлайн все выпуски подряд бесплатно в хорошем качестве
Русские криминальные сериалы всегда в тренде Давай, сынок фильм смотреть онлайн бесплатно в хорошем качестве на русском языке
Ищу зарубежный сериал, который можно смотреть без остановки Афросамурай: Воскрешение (аниме 2009) на русском языке смотреть онлайн бесплатно в хорошем качестве
Русские сериалы становятся все популярнее за рубежом Бесприданница (фильм 1974) смотреть онлайн бесплатно в хорошем качестве
Лучшие русские сериалы – это те, которые заставляют задуматься Если сердце дрогнет (сериал 2023, Домашний) 1-4 серия смотреть онлайн бесплатно в хорошем качестве
Русские сериалы радуют качеством, особенно последние новинки Кунхам: Пограничный остров (фильм 2017) смотреть онлайн бесплатно дораму в хорошем качестве на русском языке
Лучшие русские сериалы всегда радуют интересными сюжетами и отличной игрой актеров Семейные сериалы смотреть онлайн бесплатно в хорошем качестве HD 720 без регистрации » Страница 2
Зарубежные комедийные сериалы – отличный способ поднять настроение Черная жемчужина (турецкий сериал 2017) смотреть онлайн все серии подряд бесплатно в хорошем качестве на русском языке
Зарубежные сериалы – это отличный способ подтянуть иностранный язык Трюфельный пёс королевы Джованны (сериал 2017, ТВЦ) смотреть онлайн все серии подряд бесплатно в хорошем качестве
Зарубежные сериалы позволяют увидеть другие культуры и традиции Два сердца (сериал 2020) смотреть онлайн все серии подряд бесплатно в хорошем качестве
Игровые автоматы с бездепозитным бонусом за регистрацию с выводом Игровые автоматы с бонусом за регистрацию без депозита
Где найти список лучших русских сериалов всех времен? Ленинград (сериал 2007) смотреть онлайн все серии подряд бесплатно в хорошем качестве
Какой русский сериал можно посмотреть вечером для расслабления? Правитель: Хозяин маски (дорама 2017) на русском языке смотреть онлайн все серии подряд бесплатно в хорошем качестве
Где найти подборку русских сериалов с высоким рейтингом? 18 килогерц (фильм 2020) смотреть онлайн бесплатно в хорошем качестве на русском языке
https://1xbetnplogin.com/
Лучшие зарубежные сериалы – это те, которые заставляют думать Человек, который сомневается (фильм 1963) смотреть онлайн бесплатно в хорошем качестве
мтс новосибирск
https://hatchingjobs.com/companies/leticia8956/
мтс подключение новосибирск
Лучшие русские сериалы – это гордость отечественного кинематографа Мультсериалы смотреть онлайн бесплатно, новинки мультсериалов в HD качестве » Страница 22
Какие русские сериалы можно назвать культовыми? Любовь в лунном свете 1 сезон 18 серия смотреть онлайн бесплатно в хорошем качестве на русском языке
Лучшие зарубежные сериалы – это настоящее удовольствие для киномана Мы из будущего 2 (фильм 2010, Россия) смотреть онлайн бесплатно в хорошем качестве
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?
Лучшие русские сериалы – это те, которые сделаны с душой Маленький беглец (фильм 1966) смотреть онлайн бесплатно в хорошем качестве
мтс новосибирск
https://recruitment.talentsmine.net/employer/bobby9033/
мтс интернет новосибирск
Русские сериалы с неожиданными финалами – это всегда интересно Бегущая от любви (сериал 2014-2017, Россия) смотреть онлайн бесплатно все серии подряд в хорошем качестве
Лучшие русские сериалы всегда радуют интересными сюжетами и отличной игрой актеров Ждите неожиданного (сериал 2017, ТВЦ) смотреть онлайн все серии подряд бесплатно в хорошем качестве
Зарубежные сериалы – это всегда высокий уровень съемок и крутые спецэффекты Летят журавли (фильм 1957) смотреть онлайн бесплатно в хорошем качестве
# Harvard University: A Legacy of Excellence and Innovation
## A Brief History of Harvard University
Founded in 1636, **Harvard University** is the oldest and one of the most prestigious higher education institutions in the United States. Located in Cambridge, Massachusetts, Harvard has built a global reputation for academic excellence, groundbreaking research, and influential alumni. From its humble beginnings as a small college established to educate clergy, it has evolved into a world-leading university that shapes the future across various disciplines.
## Harvard’s Impact on Education and Research
Harvard is synonymous with **innovation and intellectual leadership**. The university boasts:
– **12 degree-granting schools**, including the renowned **Harvard Business School**, **Harvard Law School**, and **Harvard Medical School**.
– **A faculty of world-class scholars**, many of whom are Nobel laureates, Pulitzer Prize winners, and pioneers in their fields.
– **Cutting-edge research**, with Harvard leading initiatives in artificial intelligence, public health, climate change, and more.
Harvard’s contribution to research is immense, with billions of dollars allocated to scientific discoveries and technological advancements each year.
## Notable Alumni: The Leaders of Today and Tomorrow
Harvard has produced some of the **most influential figures** in history, spanning politics, business, entertainment, and science. Among them are:
– **Barack Obama & John F. Kennedy** – Former U.S. Presidents
– **Mark Zuckerberg & Bill Gates** – Tech visionaries (though Gates did not graduate)
– **Natalie Portman & Matt Damon** – Hollywood icons
– **Malala Yousafzai** – Nobel Prize-winning activist
The university continues to cultivate future leaders who shape industries and drive global progress.
## Harvard’s Stunning Campus and Iconic Library
Harvard’s campus is a blend of **historical charm and modern innovation**. With over **200 buildings**, it features:
– The **Harvard Yard**, home to the iconic **John Harvard Statue** (and the famous “three lies” legend).
– The **Widener Library**, one of the largest university libraries in the world, housing **over 20 million volumes**.
– State-of-the-art research centers, museums, and performing arts venues.
## Harvard Traditions and Student Life
Harvard offers a **rich student experience**, blending academics with vibrant traditions, including:
– **Housing system:** Students live in one of 12 residential houses, fostering a strong sense of community.
– **Annual Primal Scream:** A unique tradition where students de-stress by running through Harvard Yard before finals!
– **The Harvard-Yale Game:** A historic football rivalry that unites alumni and students.
With over **450 student organizations**, Harvard students engage in a diverse range of extracurricular activities, from entrepreneurship to performing arts.
## Harvard’s Global Influence
Beyond academics, Harvard drives change in **global policy, economics, and technology**. The university’s research impacts healthcare, sustainability, and artificial intelligence, with partnerships across industries worldwide. **Harvard’s endowment**, the largest of any university, allows it to fund scholarships, research, and public initiatives, ensuring a legacy of impact for generations.
## Conclusion
Harvard University is more than just a school—it’s a **symbol of excellence, innovation, and leadership**. Its **centuries-old traditions, groundbreaking discoveries, and transformative education** make it one of the most influential institutions in the world. Whether through its distinguished alumni, pioneering research, or vibrant student life, Harvard continues to shape the future in profound ways.
Would you like to join the ranks of Harvard’s legendary scholars? The journey starts with a dream—and an application!
https://www.harvard.edu/
Лучшие русские сериалы – это те, которые цепляют с первых минут Золушка (фильм 2018) смотреть онлайн бесплатно в хорошем качестве
мтс телевидение
https://centerfairstaffing.com/employer/stephany2219/
мтс тв
Где смотреть русские сериалы без рекламы и в хорошем качестве? Пропавший (фильм 2011, НТВ) смотреть онлайн бесплатно в хорошем качестве
Retire seus ganhos da [bingo](https://bingo-br.com) com facilidade. O processo de saque é simples e exige apenas que você forneça os dados do seu método de pagamento. A plataforma assegura a proteção dos seus fundos com criptografia avançada, garantindo que seu dinheiro esteja sempre seguro.
Pегистрация у операторов связи. Некоторые операторы связи также предоставляют возможность зарегистрировать виртуальный номер в своей сети https://ulyanovsk-news.net/other/2024/11/05/217182.html
[url=https://ourmind.ru//wp-content/news/filmu_pro_budushee__vzglyad_skvoz_prizmu_vremeni.html]https://ourmind.ru//wp-content/news/filmu_pro_budushee__vzglyad_skvoz_prizmu_vremeni.html[/url] проверить билет по номеру билета и тиражу золотая подкова
sda steam
Сертификат происхождения СТ-1 подтверждает, что товар был произведен в определенной стране, что важно для таможенного оформления и применения тарифных преференций. Протокол испытания, в свою очередь, документирует результаты лабораторных исследований продукции, подтверждая соответствие определенным стандартам качества и безопасности. Сертификат соответствия на продукцию удостоверяет, что товар соответствует требованиям технических регламентов или национальных стандартов. Свидетельство о государственной регистрации продукции требуется для товаров, подлежащих санитарно-эпидемиологическому надзору, и подтверждает их безопасность для здоровья человека. Декларация ТР ТС – это документ, которым производитель или импортер подтверждает соответствие продукции требованиям технических регламентов Таможенного союза. Нотификация ФСБ требуется для ввоза или вывоза продукции, содержащей шифровальные (криптографические) средства. Декларация Минсвязи, в свою очередь, необходима для оборудования связи, подтверждая его соответствие установленным требованиям в сфере связи и телекоммуникаций. Все эти документы играют важную роль в обеспечении качества, безопасности и законности продукции, обращающейся на рынке. Декларация Минсвязи
Представляем вашему вниманию сайт, с индийскими фильмами и сериалами с просмотром онлайн! На сайте вы найдете большой выбор популярнейших фильмов Болливуда, а также популярные сериалы, которые покорили зрителей по всему миру. Мы представляем разнообразные жанры: от невероятных драм и романтических комедий до мистических триллеров и исторических эпопей. Наша база фильмов содержит как давно полюбившиеся всем фильмы, так и современные хиты, чтобы каждый мог посмотреть что-то на свой вкус.
Благодаря нашему сайту вы можете посмотреть [url=https://india-film1.com/]индийские фильмы смотреть онлайн[/url] в любое время и в любом месте. Простой интерфейс и отличное качество видеозаписей обеспечивают незабываемый отдых во время просмотра. Окунитесь в мир индийского кинематографа и откройте для себя его невероятную культуру в нашем онлайн кинотеатре!
[url=https://yarkompany.ru/language/pages/?volshebnue_skazki___okna_v_mir_chudes.html]https://yarkompany.ru/language/pages/?volshebnue_skazki___okna_v_mir_chudes.html[/url] касимов погода на 10 дней
sda steam
мтс тарифы новосибирск
https://teachersconsultancy.com/employer/156585/kasha7019
мтс тарифы на интернет
steam desktop authenticator
[url=https://school33-perm.ru/media/pgs/?hitu_amediateki.html]https://school33-perm.ru/media/pgs/?hitu_amediateki.html[/url] сериал ивановы ивановы 2 сезон смотреть онлайн бесплатно
Words hold weight—especially when they influence public opinion. Consider the expression From the River to the Sea: while on the outside it looks as a plea for justice, its true intention is far more concerning. Rather than supporting peaceful coexistence, this slogan conceals a sinister call for expulsion and destruction. We’ve meticulously examined the historical roots underlying this slogan to expose what it actually means. For an honest dialogue and deeper understanding, click [url=https://unitedwithisrael.org/good-news-archive-oct-8-2017/]here[/url] and learn why understanding slogans matters more now than ever.
At the same time, navigating discussions on Zionism and antisemitism demands precision, not ambiguity. Critiquing political ideologies like Zionism doesn’t inherently equal prejudice against Jewish communities—although opponents often blur the lines purposely. Our platform provides clear insights, distinguishing fact from emotionally charged misinformation. It’s crucial to cultivate conversations without suppressing legitimate perspectives. Discover the difference between political critique and racial hatred, and help create informed discussions rather than polarized arguments. Visit our trusted [url=https://noisyroom.net/2014/03/19/watchers-council-nominations-putinmania-edition/]read more[/url] and join in dialogue that encourages genuine understanding.
https://lioleo.edu.vn/daily01-huyen/4.png.php?id=bet-osis-smk
прокат автомобилей крым аренда авто в манавгате
https://1xbetlklogin.com/
https://api.lioleo.edu.vn/public/images/course/4.png.php?id=cmd368-bet
ферби приложение для андроид скачать бесплатно [url=http://apk-smart.com/igry/tamagochi/392-furby-boom-polnaja-vzlomannaja-versija.html]ферби приложение для андроид скачать бесплатно[/url] ферби приложение для андроид скачать бесплатно
P.S Live ID: K89Io9blWX1UfZWv3ajv
P.S.S [url=https://ramique.kr/bbs/board.php?bo_table=free&wr_id=715651]Программы и игры дл[/url] [url=http://empira.ru/index.php?option=com_content&view=article&id=52&Itemid=61]Программы и игры для Андроид телефона[/url] [url=https://eng-way.ru/znachenie-i-perevod-slova-way/#comment-4675]Программы и игры для Андроид телефона[/url] bc53918
мтс подключить
https://www.jobassembly.com/companies/senaida6529/
провайдер мтс
[url=https://allboiler.ru/news/poznavatelnoe_kino__uchimsya__razvivaemsya_i_vdohnovlyaemsya.html]https://allboiler.ru/news/poznavatelnoe_kino__uchimsya__razvivaemsya_i_vdohnovlyaemsya.html[/url] проверить русское лото по номеру билета и тиражу последний
[url=https://clockfase.com/auth/elmn/?filmu_na_kinogo___luchshiy_vubor_dlya_lubiteley_kino.html]https://clockfase.com/auth/elmn/?filmu_na_kinogo___luchshiy_vubor_dlya_lubiteley_kino.html[/url] какой фильм считается самым лучшим
мтс подключение
https://www.usbstaffing.com/companies/princess7588/
мтс подключение новосибирск
Повреждённый стик портит весь игровой процесс? Специализированный [url=https://mobile-worker.ru/remont-dzhojstikov.html]ремонт стика геймпада[/url] вернёт точность управления. Мастера сервисного центра оперативно заменят изношенные детали и проведут полную калибровку системы.
Более 7 лет наш сервисный центр на Коломенской помогает москвичам решать технические проблемы. В августе 2022 года Mobile-Worker переехал в новое просторное помещение на проспекте Андропова, 17к1. Теперь наши клиенты могут наслаждаться ещё более комфортным обслуживанием.
[url=https://taktikiipraktiki.ru/news/serialu_pro_derevnu__prostota__iskrennost_i_osobaya_atmosfera.html]https://taktikiipraktiki.ru/news/serialu_pro_derevnu__prostota__iskrennost_i_osobaya_atmosfera.html[/url] смотреть фильмы в онлайн бесплатно в хорошем качестве без регистрации новинки
Glory Casino
https://api.lioleo.edu.vn/public/images/skills/4.png.php?id=mojo-bet
Glory Casino
Glory Casino
Glory Casino
Glory Casino
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
мтс цены
https://boomservicestaffing.com/companies/24inetrnet-domashnij-ekb/
мтс подключение
Glory Casino
Glory Casino
https://1xbetvnlogin.com/
мтс домашний интернет
https://realestate.kctech.com.np/profile/jeanniebeatty
мтс екатеринбург
[url=https://li-terra.ru/art/filmu_143.html]https://li-terra.ru/art/filmu_143.html[/url] ютуб михаил портнов силиконовая долина
Одноразовые номера не предназначены для голосового общения. С их помощью разрешено лишь получать или же быстро отправлять СМС в течение определенного отрезка времени. Они не привязаны ни к абонентам, ни к конкретным устройствам http://www.propr.me/virtualnye-nomera-telefonov-ot-servisa-did-virtual-numbers/
Glory Casino
Мобильное казино Пин Ап. Пин Ап обеспечивает комфортный доступ к слотам и Live-казино прямо с мобильных устройств. Игры доступны везде, где есть интернет https://maturidi.kz/
взлом car eats car 2 [url=https://apk-smart.com/igry/gonki/1255-vzlomannaja-car-eats-car-2-mnogo-deneg.html]https://apk-smart.com/igry/gonki/1255-vzlomannaja-car-eats-car-2-mnogo-deneg.html[/url] взлом car eats car 2
P.S Live ID: K89Io9blWX1UfZWv3ajv
P.S.S [url=https://www.bmwstyle.ru/forum/index.php/topic,69125.new.html#new]Программы и игры для Андроид телефона[/url] [url=https://myflower-design.com/index.php?route=information/blogger&blogger_id=1]Программы и игры для Андроид телефона[/url] [url=http://www.car-78.ru/capcha.php?PHPSESSID=2pujahtp37oj2jevbb99clisd2]Программы и игры для Андроид телефона[/url] 3cf6d63
мтс домашний интернет екатеринбург
https://cphallconstlts.com/employer/24inetrnet-domashnij-ekb-3/
мтс подключить
Glory Casino
Glory Casino
Glory Casino
[url=http://dentaone.ru/wp-content/pages/uzghasu_na_kinogo___parad_igolok_dlya_nervov_i_fantaziy.html]http://dentaone.ru/wp-content/pages/uzghasu_na_kinogo___parad_igolok_dlya_nervov_i_fantaziy.html[/url] кино онлайн скачать на телефон
Hi there excellent blog! Does running a blog similar to this take a large amount of work?
I’ve absolutely no knowledge of programming but I
had been hoping to start my own blog soon. Anyways, should you have any suggestions or
techniques for new blog owners please share. I understand this is off topic
nevertheless I simply wanted to ask. Kudos!
Glory Casino
The slogan – From the River to the Sea might seem like a powerful plea for justice, yet beneath its attractive exterior hides a troubling reality. It’s not a harmless message, but a veiled appeal encouraging the expulsion or even annihilation of an entire community. We examine the intricacies of this expression and its political roots, exposing its dangerous meanings. To better grasp how seemingly harmless phrases can mask harmful intentions, click [url=https://wiki2.org/en/Diyanet_Center_of_America]source[/url] to explore more deeply into this critical issue.
In a climate where information influences opinion, recognizing the thin line between political critique and hateful speech is crucial. Differentiating anti-Zionism from antisemitism isn’t just semantics—it’s crucial to honest discourse. Our resource explains the distinctions, addresses myths, and underscores why criticism of policies isn’t synonymous with prejudice against a people. Equip yourself with insights that promote conversation, not division. To discover more about why these issues matter, visit the [url=https://nzt.eth.link/wiki/Turkish_American_Community_Center.html]read more[/url] and join a dialogue grounded in nuance, accuracy, and genuine understanding.
https://angelladydety.getbb.ru/viewtopic.php?f=39&t=47736
мтс домашний интернет екатеринбург
https://feelhospitality.com/employer/24inetrnet-domashnij-ekb/
мтс подключить екатеринбург
[url=https://rumol.ru/wp-includes/wli/smotret_serialu_onlayn___udobstvo_i_vubor_dlya_kazghdogo.html]https://rumol.ru/wp-includes/wli/smotret_serialu_onlayn___udobstvo_i_vubor_dlya_kazghdogo.html[/url] фильм верность 2019 смотреть бесплатно в хорошем качестве онлайн
https://wiki.team-glisto.com/index.php?title=Voditel_77a
https://parentingliteracy.com/wiki/index.php/User:BrandenMathew
мтс интернет
https://icmimarlikdergisi.com/kariyer/companies/24inetrnet-domashnij-ekb-3/
мтс домашний интернет
[url=https://rosohrancult.ru/wp-includes/pages/filmu_142.html]https://rosohrancult.ru/wp-includes/pages/filmu_142.html[/url] скачать фильмы в хорошем качестве без регистрации бесплатно на телефон
https://wiki.lafabriquedelalogistique.fr/Voditel_37y
https://www.yewiki.org/User:HumbertoAyers2
Отвод – это элемент соединяющий части трубопровода при помощи сварки. Причем наружный диаметр и толщина стенок отвода должна соответствовать наружному диаметру 11с39п
[url=https://flashparade.ru/wp-includes/jks/novogodnie_filmu_onlayn_smotret_besplatno_v_horoshem_kachestve.html]https://flashparade.ru/wp-includes/jks/novogodnie_filmu_onlayn_smotret_besplatno_v_horoshem_kachestve.html[/url] скачать фильмы через анвар на телефон
Если вы хотите продать свои часы, лучше всего обратиться в специализированные компании, которые занимаются выкупом часов http://inprokorea.com/bbs/board.php?bo_table=free&wr_id=953373
Единственный знак зодиака, который имеет особую связь с Богом https://x.com/Fariz418740/status/1901024008243429625
Букмекерская контора и онлайн-казино Mostbet приветствует вас на официальном сайте своего бесплатного приложения для Android телефонов. Здесь вы можете скачать mostbet aviator
Единственный знак зодиака, который имеет особую связь с Богом https://www.pinterest.com/pin/957366833290360277
Игровые автоматы с бонусом за регистрацию без депозита no deposit casino
[url=https://legonew.ru/files/price/multfilmu_pro_kosmos___zahvatuvaushiy_mir_dlya_detey_i_vzrosluh.html]https://legonew.ru/files/price/multfilmu_pro_kosmos___zahvatuvaushiy_mir_dlya_detey_i_vzrosluh.html[/url] годы фильм смотреть онлайн бесплатно в хорошем качестве
Выявлен фрукт, который является природным эликсиром молодости https://www.pinterest.com/pin/957366833290370789
Which Zodiac signs should be careful – a dangerous period begins tomorrow! https://x.com/Fariz418740/status/1901169237206397369
мафия 2 мобайл [url=http://apk-smart.com/igry/strelyalki/605-mafija-2.html]мафия 2 мобайл[/url] мафия 2 мобайл
P.S Live ID: K89Io9blWX1UfZWv3ajv
P.S.S [url=http://gornostay.com/forums/topic/verapamil-prix-verapamil-120-prix-3/page/648/#post-2547637]Программы и игры для Андроид телефона[/url] [url=https://www.waistbeads.com/product/kloe-universe-dress-2]Программы и игры для Андроид телефона[/url] [url=https://knaeewdse.click/2024/04/08/hello-world/comment-page-1098/#comment-58078]Программы и игры для Андроид телефона[/url] c04_6d6
Casino Mostbet успешно объединяет БК и слоты, занимая топовые позиции на рынке много лет. Каталог с игровым софтом на сайте содержит высококачественный контент, включая разнообразные слоты, Live, карточные и настольные развлечения мостбет скачать на андроид
[url=http://www.newlcn.com/pages/news/filmu_141.html]http://www.newlcn.com/pages/news/filmu_141.html[/url] ютуб елена райтман страшные переписки
Как выбрать метод удаления папиллом, сравните предложения.
Удаление папиллом аппаратом Сургитрон: преимущества [url=http://epilstudio.ru/udalenie-papillom/#Удаление-папиллом-аппаратом-Сургитрон:-преимущества]http://epilstudio.ru/udalenie-papillom/[/url] .
Which Zodiac signs should be careful – a dangerous period begins tomorrow!
https://www.pinterest.com/pin/957366833290379120
[url=https://yvision.kz/community/creation]театр и творчество Казахстан[/url] – qazagym, шоу бизнес Казахстан
[url=https://ykrn.site/]Kraken вход[/url] – Кракен адресс, Kraken links
check https://1253658985244568521.com/
Usual Veda is at the forefront of blockchain development, providing innovative technology solutions for businesses and developers. Our expertise in decentralized systems and secure blockchain applications enables companies to optimize operations and stay ahead in the digital era. With Usual Veda, businesses gain access to reliable, scalable, and cutting-edge technology that drives success. https://usual-vault.com/
home [url=https://keplerchain.com]keplr wallet extension[/url]
Ethereal Trade is a cutting-edge decentralized exchange that redefines the way users trade cryptocurrencies. With a focus on security, speed, and efficiency, Ethereal Trade offers a seamless trading experience without intermediaries. As a leader in crypto trading, our platform ensures transparent transactions and advanced trading tools, making it the go-to solution for both beginners and experienced traders. https://ethereal.ac
мтс телевидение екатеринбург
https://publiccharters.org/employer/24inetrnet-domashnij-ekb-3/
мтс интернет
Энвато Envato, Adobe Stock, iStock, Depositphotos, Dreamstime – это лишь верхушка айсберга в мире стоковых платформ, предлагающих фотографии, видео, графику и другие креативные ресурсы. Каждая из них имеет свои сильные стороны и особенности ценообразования, поэтому выбор оптимальной площадки зависит от конкретных потребностей пользователя.
[url=http://vip-barnaul.ru/includes/pages/onlayn_filmu___komfort_i_dostupnost_v_mire_kinematografa.html]http://vip-barnaul.ru/includes/pages/onlayn_filmu___komfort_i_dostupnost_v_mire_kinematografa.html[/url] фильмы которые можно посмотреть на кинопоиске
мтс тв
https://career.abuissa.com/employer/24inetrnet-domashnij-ekb-3/
мтс подключение
100% проверенные и реальные отзывы учеников о курсах онлайн-школы Skillbox. Образовательная платформа Skillbox – это облачная система, предназначенная для цифровизации образовательного процесса. Входит в реестр российского ПО скилбокс отзывы
description https://hitman-assassin-killer.com
Когда состоится встреча Путина и Трампа? https://www.youtube.com/watch?v=QaQAFGjNvyM
[url=http://neoko.ru/images/pages/filmu_onlayn__komfortnuy_sposob_naslazghdatsya_kino.html]http://neoko.ru/images/pages/filmu_onlayn__komfortnuy_sposob_naslazghdatsya_kino.html[/url] я не злодейка то что я могу контролировать тьму не значит что я плохой человек
мтс интернет екатеринбург
https://ir.karpirajobs.com/employer/24inetrnet-domashnij-ekb-3/
мтс подключить екатеринбург
Почему Нимесил запрещён в некоторых странах? https://x.com/DeyanetKrmv/status/1901394421708452176
мтс екатеринбург
https://www.huntsrecruitment.com/employer/24inetrnet-domashnij-ekb-3/
мтс цены
Bu burcl?r pula daha meyillidir https://www.pinterest.com/pin/957366833290413195
[betfury](https://betfury-br.com) oferece bônus de US$ 100 ao fazer cadastro e login! Após registrar-se, você terá acesso imediato ao bônus para usar em jogos de cassino e aumentar suas chances de ganhar. Aproveite agora essa oferta exclusiva!
[url=http://devec.ru/Joom/pgs/filmu_144.html]http://devec.ru/Joom/pgs/filmu_144.html[/url] скачать на планшет бесплатно фильмы
Выявлен единственный напиток, который спасает волосы https://x.com/Fariz418740/status/1901556598939468146
Fenerbahçe su kaçak tespiti Salon zeminindeki su kaçağını termal kamerayla buldular. Parkeleri koruyarak sorunu çözdüler. Hande H. https://dragonballgtxxx.com/uskudar-tesisatci-tikali-mutfak-acma/
каталог https://vodkabet.io
[url=https://kra54.me/]kra30.cc[/url] – kra29.at, kra30.at
Единственный знак зодиака, который не могут раскусить астрологи
https://x.com/CantrellAl79925/status/1901597622399381669
[url=https://kupit.auto-msk.top]права на автомобиль[/url] – права категории б, водительские права купить
Предсказания Ванги на 2025 год: правда или миф?
https://x.com/Ruslansavalv/status/1901612556772409580
[url=https://betslive.ru/greenada-casino-promokod/]Гринада казино промокод[/url] – Бонусы за регистрацию с промокодом Риобет, 1win промокод при регистрации
https://api.lioleo.edu.vn/public/images/skills/4.png.php?id=360-bet
Широкая линия и LIVE-события. Онлайн ставки на спорт с бонусами и акциями. Высокие коэффициенты топовое онлайн-казино Казахстана olimp casino пополнение счета
1xslots бездепозитный бонус 1xslots бездепозитный бонус
Medispensary Marijuana Delivery is the the best service providing cannabis at any price and taste read more
Выбор цветов огромный, можно подобрать на любой случай.
заказ цветов томск с доставкой
[url=http://alexey-savrasov.ru/records/articles/filmu_o_poiske_sokrovish_besplatno.html]http://alexey-savrasov.ru/records/articles/filmu_o_poiske_sokrovish_besplatno.html[/url] фильмы которые показывали в кинотеатре
website here
[url=https://web-lumiwallet.com/]Web.lumi wallet[/url]
great blog very nice articles I will follow you continuously sohbet odaları
Неожиданный способ есть финики превращает их в природное лекарство
https://x.com/SebiBilalova/status/1901774516755309011
click for more info [url=https://web-lumiwallet.com]Lumi wallet[/url]
[aviator aposta](https://aviatorbet-br.com): Explore os jogos de apostas mais populares e ganhe bônus incríveis!
click over here now https://web-lumiwallet.com
visit their website https://web-lumiwallet.com/
Эти четыре знака Зодиака накроет волна счастья уже на этой неделе
https://www.pinterest.com/pin/957366833290452816/
[url=http://kib-net.ru/news/pgs/filmu_pro_piratov__zahvatuvaushiy_mir_morskih_priklucheniy.html]http://kib-net.ru/news/pgs/filmu_pro_piratov__zahvatuvaushiy_mir_morskih_priklucheniy.html[/url] американские фильмы 2000 х годов
[9k bet](https://9k-bet-br.com) oferece bônus de US$ 100 para novos usuários – cadastre-se agora!
A pixbet é um cassino online de confiança, com jogos populares e uma plataforma justa para todos os jogadores. E para deixar sua experiência ainda mais incrível, a plataforma oferece bônus vantajosos que aumentam suas chances de ganhar e fazem a diversão durar mais tempo.
игры Steam со скидкой
фриспины без депозита Игровые автоматы лучшие
2 знака зодиака, от которых практически невозможно что-либо скрыть
https://pin.it/1l95y2WeH
check this [url=https://allsmm.net/services]best smm services[/url]
useful source [url=https://allsmm.net/]smm panel services[/url]
Чат GPT на русском может использоваться для решения различных задач, таких как поиск информации, ответы на вопросы, генерация текста и многое другое строительство бани от забора
Осуществите Мечту о Идеальном Ремонте с Эксперт ремонта – Без Предоплаты! Доверьте Нам Свою Квартиру ремонт и отделка квартир отзывы Алматы
Prostat x?rc?ngin? qars? hans? qidalar istifad? edilm?lidir? https://x.com/Fariz418740/status/1902037337590108612
find out here
[url=https://web-lumiwallet.com/]Web.lumi wallet[/url]
https://www.gabitos.com/DESENMASCARANDO_LAS_FALSAS_DOCTRINAS/template.php?nm=1740384201
blog link https://web-lumiwallet.com
[url=http://www.bf-mechta.ru/wp-content/pages/luchshie_filmu_pro_vikingov_besplatno.html]http://www.bf-mechta.ru/wp-content/pages/luchshie_filmu_pro_vikingov_besplatno.html[/url] обманутый светик 6 букв сканворд
http://localbundled.com/directory/listingdisplay.aspx?lid=79541
Спасибо за быструю доставку и шикарные цветы!
доставка цветов
[url=http://www.zoolife55.ru/pages/multfilmu_pro_loshadey__chem_oni_pokorili_zriteley_.html]http://www.zoolife55.ru/pages/multfilmu_pro_loshadey__chem_oni_pokorili_zriteley_.html[/url] шнитке сказка странствий скачать бесплатно mp3
Prepare for IPL 2025 betting with top online casinos in India! We offer a list of the best IPL betting sites with great odds, quick payouts, and bonuses up to 500%. Find trusted platforms, safe payment methods, and expert IPL betting tips to enhance your season. Start betting today betting ipl
Saiba Como Sacar Seu Dinheiro no [abc bet](https://abc-bet-br.com) e Garantir a Segurança dos Seus Fundos!
пояснения [url=https://nova-xllc.top/]nova маркетплейс[/url]
useful reference [url=https://www.acheter-faux-billet.com/]acheter des faux billets[/url]
official website [url=https://www.acheter-faux-billet.com]acheter des faux billets[/url]
Chơi Tại bet168 – https://bet168-vn.com – Gửi Tiền Đầu Nhận Thưởng 100$ Hấp Dẫn
найти это [url=https://nova-xllc.top]nova маркетплейс[/url]
Ưu Đãi Độc Quyền: Thưởng 100$ Cho Người Dùng Mới Tại bk8 – https://bk8-vn.com
bong88 – https://bong88-vn.com Khuyến Mãi Cực Hot – Nạp Đầu Nhận Ngay 100$
Prostat x?rc?ngin? qars? hans? qidalar istifad? edilm?lidir? https://x.com/Fariz418740/status/1902037337590108612
### Онлайн-казино: захватывающий мир азартных игр в интернете
Онлайн-казино стали популярным развлечением для миллионов игроков по всему миру. Они позволяют испытать удачу, насладиться атмосферой азартных игр и выиграть крупные суммы денег, не выходя из дома. Современные платформы предлагают огромный выбор игровых автоматов, рулетки, покера, блекджека и других игр, доступных в любое время суток.
#### Преимущества онлайн-казино
1. **Доступность** – играть можно с любого устройства: компьютера, смартфона или планшета. Все, что нужно, – это интернет-соединение.
2. **Большой выбор игр** – современные казино предлагают сотни, а иногда и тысячи слотов и карточных игр от ведущих разработчиков.
3. **Бонусы и акции** – новые игроки часто получают приветственные бонусы, фриспины и другие поощрения, которые увеличивают шансы на выигрыш.
4. **Безопасность** – лицензированные онлайн-казино используют передовые технологии шифрования данных, чтобы обеспечить защиту личной информации и финансовых транзакций.
5. **Игра в режиме реального времени** – благодаря живым дилерам пользователи могут наслаждаться атмосферой настоящего казино, находясь у себя дома.
#### Как выбрать надежное онлайн-казино?
Перед тем как начать игру, важно выбрать безопасную и честную платформу. Обратите внимание на:
– **Наличие лицензии** – надежные казино работают по лицензии официальных регуляторов (например, Malta Gaming Authority, Curacao eGaming и других).
– **Отзывы игроков** – изучите мнения других пользователей, чтобы узнать о репутации казино.
– **Выбор платежных методов** – удобные способы пополнения счета и вывода средств (банковские карты, электронные кошельки, криптовалюта).
– **Службу поддержки** – хороший игровой сервис всегда предоставляет круглосуточную поддержку клиентов.
#### Популярные игры в онлайн-казино
Игровые платформы предлагают разнообразие азартных развлечений:
– **Игровые автоматы (слоты)** – классические и современные слоты с яркой графикой, бонусными раундами и джекпотами.
– **Рулетка** – европейская, американская, французская версии игры с разными стратегиями ставок.
– **Покер** – техасский холдем, омаха, видео-покер и другие разновидности.
– **Блэкджек** – популярная карточная игра, где нужно набрать 21 очко и обыграть дилера.
– **Баккара** – динамичная игра, ставшая любимой среди профессионалов и новичков.
#### Советы для успешной игры
– Устанавливайте лимиты ставок, чтобы контролировать бюджет.
– Играйте только в лицензированных казино, чтобы избежать мошенничества.
– Используйте бонусные предложения, но внимательно читайте условия их отыгрыша.
– Развивайте стратегическое мышление в карточных играх, чтобы увеличить шансы на выигрыш.
– Помните, что азартные игры – это развлечение, а не способ заработка.
Онлайн-казино – это увлекательный мир азартных развлечений, который предлагает комфорт, удобство и возможность испытать удачу в любое время. Главное – подходить к игре ответственно, выбирать надежные площадки и получать удовольствие от процесса. Удачи за игровыми столами!
https://cks-adamovka.ru/
Cách Đăng Ký bong99 – https://bong99-vn.com Trên Điện Thoại Chỉ Trong 1 Phút
bezdep Top casino с выводом
[url=http://www.nonnagrishaeva.ru/press/pages/luchshie_filmu_na_temu_boevuh_iskusstv.html]http://www.nonnagrishaeva.ru/press/pages/luchshie_filmu_na_temu_boevuh_iskusstv.html[/url] смотреть онлайн бесплатно в хорошем качестве новые фильмы
Рейтинг топ-20 лучших компаний по ремонту квартир в 2025 году с ценами под ключ, хорошей репутацией, отзывами, сайтами качественный ремонт квартир отзывы
Возможно ли получать тысячи манатов в месяц в соцсетях в Азербайджане?
https://pin.it/1OLQiA9c2
Đăng ký tài khoản tại hotlive – https://hotlive-vn.com và nhận ngay 100$ khi bạn nạp lần đầu. Với đa dạng trò chơi từ cá cược thể thao, casino trực tuyến đến slot game, bạn sẽ không bao giờ cảm thấy nhàm chán. Thêm 100$ vào tài khoản sẽ giúp bạn có nhiều cơ hội đặt cược và chiến thắng lớn. Đăng ký ngay hôm nay để tận hưởng khuyến mãi hấp dẫn này!
голд казино
แพลตฟอร์ม [url=https://pg168-th.com]pg168[/url] นำเสนอเกมการพนันยอดนิยมที่หลากหลายซึ่งให้ความยุติธรรมและโปร่งใสในทุกๆ เกม ไม่ว่าคุณจะเป็นนักพนันที่ชื่นชอบการเดิมพันกีฬา คาสิโนสด สล็อต หรือเกมคาสิโนอื่นๆ ที่นี่คุณจะได้สัมผัสประสบการณ์ที่ดีที่สุด ด้วยระบบการเล่นที่ทันสมัยและมีมาตรฐานระดับโลก [url=https://pg168-th.com]pg168[/url] รับรองว่าทุกการเดิมพันเป็นไปอย่างยุติธรรมและโปร่งใส โดยใช้ซอฟต์แวร์ที่ได้รับการตรวจสอบจากหน่วยงานที่เชื่อถือได้ เพื่อให้แน่ใจว่าผลลัพธ์ของทุกเกมจะเป็นไปตามความเป็นจริง
Рулетка в онлайн-казино: стратегии и вероятность выигрыша
Когда речь заходит о классических азартных развлечениях, вращающееся колесо занимает особое место в сердцах многих игроков. Это не просто игра на удачу, а комбинация анализа, стратегии и интуиции. С каждым вращением шансы могут меняться, и каждому участнику предоставляется возможность не только испытать удачу, но и прикоснуться к математическим аспектам процессу. Так какие же конкретные подходы помогут оптимизировать собственные результаты?
Важно учитывать, что разные виды ставок могут значительно варьироваться по своей отдаче. Например, ставка на красное или черное предоставляет почти 50% шансов на удачу, в то время как выбор конкретного номера – это шанс около 2,6%. Конечно, такая разница в вероятностях требует осознанного выбора стратегии. Для тех, кто предпочитает более рискованные шаги, ставки на числа могут стать источником значительного выигрыша, однако они связаны и с высокими рисками.
Отличным методом управления банкроллом является применение систем ставок, которые помогают контролировать размеры ставок и минимизировать потенциальные убытки. К примеру, система Мартингейла, при которой сумма ставки удваивается после каждого проигрыша, может оказаться эффективной, но требует значительных средств на счету. На другой стороне спектра находятся более щадящие стратегии, которые предполагают постепенное увеличение ставок, позволяя игроку адаптироваться к колебаниям форта.
Как выбрать подходящую тактику
Выбор оптимальной тактики требует внимательного анализа вашего игрового стиля и предпочтений. Начните с определения вашего бюджета, чтобы избежать слишком рискованных подходов. Установите для себя лимиты: определите максимальную сумму, которую готовы потратить, и строго придерживайтесь этого правила.
Рассмотрите разные методы управления ставками, такие как система Мартингейла или Фибоначчи. Первая предполагает удвоение ставки после проигрыша, что может быть рискованным, но потенциально выгодным при удачном исходе. Система Фибоначчи, основанная на математической последовательности, позволяет более осторожно подходить к ставкам, увеличивая их в соответствии с определенной логикой.
Обратите внимание на типы ставок. Простейшие варианты, такие как ставка на красное или черное, предлагают более высокие шансы. Сложные ставки, такие как комбинации чисел, могут принести большее вознаграждение, но и вероятность удачи ниже. Выбор между низкорисковыми и высокорисковыми ставками зависит от вашей склонности к азарту.
Анализируйте результаты, не только свои, но и других игроков. Изучение поведения соперников и удачных тактик может дать вам преимущество. Пробуйте разные подходы в свободных играх, прежде чем переходить к реальным ставкам. Это позволит вам понять, какая тактика вам ближе.
Наконец, не забывайте о психологическом аспекте. Согласуйте свои ожидания с реальностью и избегайте эмоциональных решений. Четкое понимание своей цели и способность сохранять хладнокровие помогут вам придерживаться выбранной тактики и действовать взвешенно.
Вероятности выигрышных ставок
Ставки на чётные или нечётные числа также предлагают аналогичное распределение. Это дает возможность ощутить баланс между риском и доходом, однако эти ставки остаются подверженными тому же фактору – зеро. Наиболее рискованными являются ставки на отдельные числа, где шансы составляют 2,63% на каждую конкретную комбинацию, соответственно, коэффициент выплат достигает 35 к 1.
Другие ставки, такие как шестерки и улицы, представляют промежуточный уровень риска. Ставки на три числа обеспечивают 8,11% вероятность с выплатой 11 к 1, что делает их более привлекательными для тех, кто хочет скомбинировать риск и потенциальную прибыль. Ставка на два числа обеспечивает шансы 5,26%, что также стоит учитывать при планировании игрового процесса.
Важно также учитывать психологические аспекты и личные предпочтения. Некоторые игроки могут чувствовать себя комфортнее с более консервативными ставками, тогда как другие могут стремиться к большему азарту, выбирая более рискованные варианты. В конечном итоге, анализируя шансы и возможные результаты, игрок может лучше подготовиться к каждому игровому сессии и выбрать подходящий стиль ставок.
https://rozetka-online.ru/
Представляем вашему вниманию онлайн-кинотеатр, с индийскими фильмами и сериалами с просмотром онлайн! На сайте вы найдете большой каталог культовых болливудских фильмов, кроме того популярные телесериалы, покорившие сердца зрителей во всех странах. Мы представляем различные жанры: от захватывающих драм и романтических комедий до мистических триллеров и исторических эпопей. Наша база фильмов содержит как классические шедевры, так и новые киноленты, чтобы все могли найти что-то на свой вкус.
Благодаря нашему сайту вы можете посмотреть [url=https://india-film1.com/]смотреть индийские фильмы онлайн в хорошем качестве[/url] находясь где угодно. Простой интерфейс и высокое качество видео обеспечивают непревзойденный просмотр. Погрузитесь в мир индийского кино и откройте для себя его невероятную культуру вместе с нами!
нажмите здесь [url=https://nova-xllc.top]nova маркетплейс[/url]
try this out [url=https://www.acheter-faux-billet.com]acheter des billets contrefaits[/url]
Обращение за помощью на дому обладает рядом преимуществ, которые особенно актуальны для пациентов, сталкивающихся с зависимостью:
Подробнее можно узнать тут – https://alko-zakodirovat.ru/vrach-narkolog-na-dom-ekb/
Частенько бонусные коды раздаются и во время значимых событий. Вся актуальная информация публикуется на канале в Telegram и её можно найти на тематических ресурсах, посвящённых беттингу https://podarishans.uz
Шубы из норки Мех как искусство: от норки до каракульчи, преображение и вдохновение Мир моды изменчив, но любовь к роскошному меху остается неизменной. Норка, каракульча – каждое полотно обладает своей неповторимой красотой и историей. Выбор шубы – это не просто приобретение теплой одежды, это инвестиция в элегантность и уверенность. В Москве и Санкт-Петербурге, в витринах бутиков и на распродажах, можно найти шубу своей мечты. Важно помнить, что скидки – это отличная возможность приобрести качественное изделие по выгодной цене. Но что делать, если в шкафу уже висит шуба, вышедшая из моды? Не спешите с ней прощаться! Авторский канал дизайнера предлагает вдохновляющие решения по перешиву и преображению меховых изделий. Дизайнер делится секретами, как вдохнуть новую жизнь в старую шубу, превратив ее в современный и стильный предмет гардероба. Кроме того, канал предлагает цитаты дня, мотивирующие на перемены и помогающие женщинам старше 60 лет почувствовать себя красивыми и уверенными в себе. Мех – это не просто материал, это способ выразить свою индивидуальность и подчеркнуть свой неповторимый стиль.
Registering at help slot win – https://help-slot-win.com is Easy – Enjoy Your $100 Bonus Now
Магазин печей и каминов https://pech.pro широкий выбор дровяных, газовых и электрических моделей. Стильные решения для дома, дачи и бани. Быстрая доставка, установка и гарантия качества!
أجهزة سحبة السيجارة الحديثة تعتمد على تكنولوجيا متطورة تمنح المستخدم تجربة تدخين محسنة بدون الروائح الكريهة أو الدخان الضار، مما يجعلها حلاً عمليًا للراغبين في الإقلاع عن التدخين التقليدي.
Все говорят о новом фильме, но если вам не хочется его смотреть, то прочитайте краткое описание на портале fankino.ru, чтобы быть в курсе. На ресурсе много интересного для себя и нового отыщите. Мы собрали для вас смс поздравления, красивые картинки, народные приметы. Ищете семейные узы сериал 2000 2001? Fankino.ru – ресурс с интуитивно понятным интерфейсом. Тут размещаем актуальные ответы и вопросы. Вы узнаете, где можно покупаться на Крещение. Найдете тест на умение и способность любить. Также вас увлекательные головоломки ждут. Уверены, что вам понравится у нас!
Эмоциональный интелект у детей Эмоциональный интеллект (ЭИ) способность распознавать и управлять своими эмоциями, а также понимать чувства других. Развитие ЭИ у детей помогает им лучше адаптироваться в обществе, строить отношения и решать конфликты. Для развития ЭИ важно обращать внимание на эмоции ребёнка, учить его выражать свои чувства и понимать эмоции других людей. Игры и беседы на темы эмоций могут стать отличной практикой.
أجهزة سحبات السيجارة القابلة للشحن تأتي بتصميم أنيق وسهل الاستخدام، تعمل بتقنية البخار بدلاً من الاحتراق، مما يجعلها خيارًا أكثر أمانًا وصديقًا للبيئة مقارنة بالسجائر التقليدية.
أجهزة سحبة السيجارة هي أجهزة إلكترونية مبتكرة تعمل بالبطارية، مصممة لمساعدة المدخنين على تقليل أو استبدال التدخين التقليدي. توفر تجربة تدخين سلسة بأضرار أقل من السجائر العادية.
Современные веб-приложения требуют не только красивого интерфейса, но и надежного, масштабируемого backend-а. Именно он отвечает за хранение данных, бизнес-логику https://shkolnie.ru/
أجهزة سحبات السيجارة القابلة للشحن تأتي بتصميم أنيق وسهل الاستخدام، تعمل بتقنية البخار بدلاً من الاحتراق، مما يجعلها خيارًا أكثر أمانًا وصديقًا للبيئة مقارنة بالسجائر التقليدية.
Play Trusted Gambling Games on netbet – https://netbet-in.com and Earn Amazing Bonus Rewards
Пионы — это всегда свежо и красиво!
Доставка цветов в Томске
Очень удобный сайт, легко оформить заказ за пару минут.
розы купить в томске
[url=https://Kra30.ca]Кракен даркнет[/url] – Кракен ссылки, кракен
Модельный ряд BMW: откройте для себя новые возможности, которые порадуют.
Погрузитесь в мир BMW, производительностью.
Откройте для себя новейшие модели BMW, кроссоверы.
От компактных до люксовых: модельный ряд BMW, впечатляюще широк.
Погрузитесь в мир премиальных автомобилей BMW, лучшие технологии.
Что отличает BMW от других брендов, с непревзойденным качеством.
Каждая модель BMW — это шедевр, тех, кто ценит стиль.
Выбор моделей BMW: найдите свою идеальную машину, которые заинтересуют.
Перспективы и инновации модельного ряда BMW, узнайте.
Разнообразие моделей BMW: для каждого, проектированные для путешествий.
Погрузитесь в технологии BMW, это опыт, который нужно испытать.
Исключительное качество: выбор BMW, который суждено испытать.
Каждая модель BMW — это мастерская инженерия, разработанная для.
Преимущества выбора автомобилей BMW, от комфорта до инноваций.
Давайте исследовать великолепие модельного ряда BMW, с выдающейся производительностью.
Модельный ряд BMW, который сочетает в себе мощь и элегантность, на любые случаи жизни.
Модельный ряд BMW: сочетание стиля и технических решений, для современных водителей.
Модельный ряд BMW: ваше новое путешествие начинается, с комфортом и стилем.
Каждый автомобиль BMW — это возможность, на любой вкус.
bmw x5 2019 [url=https://model-series-bmw.biz.ua/]https://model-series-bmw.biz.ua/[/url] .
Stadtgame is where strategy meets fun in an engaging gaming environment. Explore [url=https://myspace.com/kosnews]kosnews[/url] how clever decisions, resource management, and competitive play create a rewarding experience unlike any other game out there.
[url=https://Kra30.ca/]Kraken darknet market[/url] – Даркнет маркет, Новая гидра
Stakecasino.tech предлагает актуальную информацию. Stake Casino вас порадует прозрачными условиями и приличным разнообразием азартных игр. Сайт в применении довольно прост. Регистрация занимает менее минуты. С выводом денег проблем не возникает. Ищете stake сайт на русском casino? Stakecasino.tech – здесь вы узнаете, как начать играть в Стейк казино. Платформа интуитивно понятный интерфейс предлагает, это делает для опытных игроков и новичков ее доступной. Стейк акции и специальные бонусы предоставляет. Консультанты службы поддержки быстро отвечают и вежливо общаются.
[url=https://Kra30.ca/]Kraken darknet market[/url] – Кракен купить, Кракен тор
kubet – https://kubet-vn.com Tặng Thưởng 100$ Khi Gửi Tiền Lần Đầu
การลงทะเบียนผู้ใช้ใหม่ที่ [url=https://uea8-888.com]uea8[/url] นั้นเป็นกระบวนการที่ง่ายและสะดวก ผู้เล่นสามารถทำการสมัครสมาชิกได้อย่างรวดเร็วเพียงไม่กี่ขั้นตอน โดยเริ่มต้นจากการเข้าสู่เว็บไซต์ [url=https://uea8-888.com]uea8[/url] แล้วคลิกที่ปุ่ม “สมัครสมาชิก” หลังจากนั้นระบบจะให้กรอกข้อมูลส่วนตัว เช่น ชื่อ-นามสกุล ที่อยู่อีเมล และหมายเลขโทรศัพท์ รวมไปถึงการตั้งค่ารหัสผ่านเพื่อความปลอดภัย เมื่อกรอกข้อมูลครบถ้วนแล้ว ผู้เล่นสามารถยืนยันการสมัครได้ทันที
Техническое обследование — это комплекс мероприятий, включающий визуальное и инструментальное изучение конструкций зданий и сооружений тех обследования
goldene daytona
http://usafapedia.coslabs.com/Best_Rolex_Copy
สำหรับผู้ใช้ใหม่ที่ทำการลงทะเบียน [url=https://z16-888.com]z16[/url] ยังมีโบนัสต้อนรับที่น่าสนใจเพื่อเพิ่มโอกาสในการชนะการเดิมพัน โบนัสนี้จะช่วยให้ผู้เล่นมีเครดิตเพิ่มเติมในการเล่นเกมต่างๆ ซึ่งสามารถนำไปใช้เดิมพันได้ตามต้องการ การรับโบนัสนี้เป็นวิธีที่ดีในการเริ่มต้นเล่นเกมและเพิ่มความสนุกสนานในการเข้าร่วมกิจกรรมต่างๆ ที่เว็บไซต์ [url=https://z16-888.com]z16[/url] มีให้
rolex day date 36
https://twwrando.com/index.php/User:BenjaminKrebs
หลังจากที่ผู้ใช้ทำการลงทะเบียนแล้ว สามารถเข้าสู่ระบบได้โดยการใช้ชื่อผู้ใช้และรหัสผ่านที่ได้ตั้งไว้ในขั้นตอนการสมัคร เมื่อเข้าสู่ระบบเรียบร้อยแล้ว ผู้เล่นสามารถเข้าถึงเกมคาสิโนออนไลน์ที่หลากหลาย ไม่ว่าจะเป็นสล็อต คาสิโนสด หรือกีฬา และยังสามารถใช้โปรโมชั่นและโบนัสที่มีให้แก่ผู้ใช้ใหม่ได้ทันที
кликните сюда
[url=https://18ps.ru/about/stati/6608/]переработка пластиков[/url]
rolex pepsi gmt-master
https://telegra.ph/Panda-daytona-rolex-03-20
нажмите, чтобы подробнее
[url=https://18ps.ru/catalog/oborudovanie-dlya-pererabotki-stekla-i-plastika/]оборудование для переработки пластиков[/url]
научно исследовательский перевод
Миру могут угрожать вспышки неизвестных ранее болезней https://x.com/Fariz418740/status/1902696858473910276
Whether it’s a technical problem or a question about your account, fb777 slot – https://fb777-slot-ph.com’s professional customer service is here to help, 24/7. Our team of experts is always ready to assist with fast and efficient solutions, so you never have to wait long for help. With our customer service team by your side, you’ll always be able to enjoy a smooth and hassle-free gaming experience.
Заказать Джили – только у нас вы найдете разные комплектации. Быстрей всего сделать заказ на джили купить в спб у официального дилера можно только у нас!
[url=https://geely-v-spb1.ru]geely цена[/url]
новые автомобили geely – [url=https://www.geely-v-spb1.ru/]http://www.geely-v-spb1.ru[/url]
Texas Instruments (TI) has pioneered microcontroller technology with relentless innovations in performance, efficiency, and miniaturization. As a semiconductor industry leader, Texas Instruments Semi has introduced cutting-edge microcontroller units (MCUs) that enhance engineers’ design capabilities worldwide https://api.prx.org/series/51440-texas-instruments-innovations-in-microcontroller
Koch Market гарантирует к каждому клиенту персональный подход. Специализируемся на услугах дооснащение, полировка, тюнинг, детейлинг, оклейка. Обеспечиваем высокое качество работы. Расскажем подробнее о действующих акциях и ответим на вопросы. Ищете полировка мотоцикла? Koch-market.ru – здесь публикуем полезные статьи для автолюбителей. Цель Koch Market – сократить издержки, увеличить обороты и доходность вашего бизнеса. Оставьте заявку на сайте, и мы перезвоним в ближайшее время. Обсудим задачи, найдем наилучшее решение и запланируем работы.
Хотите свою любовь встретить? Тогда вам Лилибум стоит непременно посетить! Сайт предлагает простую регистрацию, открывает двери к новым знакомствам и дружбе. Стараемся безопасность вам и анонимность обеспечить. Вас доброжелательная атмосфера ждет. Ищете сайт знакомств для общения? Lilibum.ru – портал, где вы великолепно время можете провести. Порадует простой и красивый интерфейс. Модераторы проверяют внимательно фотографии и профили пользователей. С Лилибум вы свою половинку обязательно найдете. Общайтесь, знакомьтесь и новые отношения создавайте!
fake rolex to buy
https://playmobilinfo.com/index.php/User:MelindaStrom3
replicawatch
https://zeroandinfinity.com/index.php/User:BevHowe00604246
gold rolex with blue face
https://bbarlock.com/index.php/Rolex_63g
Продолжение
[url=https://18ps.ru/catalog/oborudovanie-dlya-pererabotki-stekla-i-plastika/]оборудование для переработки пластика[/url]
where to buy a rolex near me
https://scandalopedia.com/index.php/Rolex_39S
rolex daytona cost
https://cannabis-cultivation.wiki/index.php?title=Rolex_Second_Hand
current rolex prices
http://youtools.pt/mw/index.php?title=Rolex_46r
batman rolex price
http://classicalmusicmp3freedownload.com/ja/index.php?title=Rolex_61J
Официальный сайт казино Vavada. Онлайн-площадка Vavada казино лицензирована Кюрасао и стремительно развивается в сфере развлечений в интернете с 2017 годa vavada cadino
Twitch — одна из самых динамично развивающихся платформ для стриминга, где конкуренция за внимание зрителей растет с каждым днем https://vc.ru/smm-trendy
datejust wimbledon 41mm
https://inspo.wiki/index.php/Rolex_82M
rolex sub two tone black
https://telegra.ph/How-much-is-a-real-rolex-03-20
fakewatch
https://telegra.ph/Huy-fake-rolex-03-20
https://www.alemanhafc.com.br/2023/01/werder-bremen-aposta-nos-gols-de.html?sc=1741988321326#c6065823183805597600
Сомово – компания, которая большой ассортимент мебельной продукции предоставляет. Мы всегда рады общению с нашими клиентами. Стремимся дать вам только лучшее. Формирование долгосрочных отношений – одна из основных целей. Somovo-Ищете купить прихожие в Москве? mebel.ru/catalog/prikhozhii – здесь можно купить прихожие недорого с оперативной доставкой. Если возникнут какие-либо вопросы, свяжитесь с нами по телефону, указанному на сайте. С радостью поможем с выбором и детали заказа подскажем. В интернет-магазине «Сомово» найдется товар, который необходим именно вам!
rolex puzzle
http://wiki.schragefamily.com/index.php?title=Rolex_8q
a1 wristwatch
https://mediawiki.novastega.me/index.php/Rolex_40p
read this post here
[url=https://www.reddit.com/r/CoachScam/comments/1ibarjp/kreativstorm_reviews_experience_with_their/]Is Kreativstorm Training Program Good?[/url]
rolex day-date gold price
https://housethornwiki.com/index.php/Rolex_93d
my explanation
[url=https://www.reddit.com/user/TandyParente/comments/1dpw1e5/kreativstorm_handson_training_programs_worth_it/]Kreativstorm Training Program[/url]
home
[url=https://www.reddit.com/r/CoachScam/comments/1ibarjp/kreativstorm_reviews_experience_with_their/]Kreativstorm Reviews[/url]
https://bombergirl-esp.lol/index.php/Rolex_60U
Copy General предлагает большой спектр печатных качественных услуг. Применяем самые новейшие технологии и оборудование. Готовы ваши требования обсудить. К нам проявляют доверие множество частных, а также корпоративных клиентов по всему миру. Свяжитесь с нами прямо сегодня и убедитесь в качестве нашей работы. Ищете услуги печати? Copygeneral.ru – здесь представлены отзывы заказчиков, ознакомьтесь с ними. Типография без выходных работает и гарантирует высокое качество. Оплату можно произвести удобным способом. Стремимся превзойти ваши ожидания.
Для безопасной эксплуатации прицепа необходима регулярная экспертиза. Проведите тщательный осмотр перед началом сезона, чтобы убедиться в его исправности. Особое внимание уделите колесам, тормозам и световым приборям http://nielsen.pro/doku.php?id=%20%D0%92%D0%BE%D1%81%D1%81%D1%82%D0%B0%D0%BD%D0%BE%D0%B2%D0%B8%D1%82%D0%B5%D0%BB%D1%8C%D0%BD%D1%8B%D0%B9%20%D1%80%D0%B5%D0%BC%D0%BE%D0%BD%D1%82:%20%D1%8D%D1%82%D0%B0%D0%BF%D1%8B%20%D0%B8%20%D0%B8%D1%85%20%D0%BE%D1%86%D0%B5%D0%BD%D0%BA%D0%B0
[url=https://rossahar.ru/rynok/pgs/igru_na_dvoih__ogon_i_voda.html]https://rossahar.ru/rynok/pgs/igru_na_dvoih__ogon_i_voda.html[/url] раскраски для девочек 10 11 лет
replica watch shop
https://menuaipedia.menuai.id/index.php/Rolex_7D
click here to investigate
[url=https://www.reddit.com/user/AlanaMcdowell/comments/1dqewao/what_do_you_think_about_kreativstorm/]Kreativstorm[/url]
http://youtools.pt/mw/index.php?title=Rolex_90K
Жилой комплекс «Поляна Пик» — это премиальный проект, расположенный в самом сердце Красной Поляны, окружённый величественными горами. Этот комплекс идеально подходит для тех, кто ценит комфорт, стиль и близость к природе квартиры в красной поляне купить цены
analizador de vibraciones
Dispositivos de equilibrado: clave para el operación estable y productivo de las maquinarias.
En el ámbito de la avances moderna, donde la efectividad y la seguridad del aparato son de máxima importancia, los equipos de ajuste desempeñan un función esencial. Estos dispositivos adaptados están concebidos para ajustar y fijar componentes giratorias, ya sea en equipamiento manufacturera, transportes de traslado o incluso en equipos hogareños.
Para los expertos en conservación de dispositivos y los técnicos, trabajar con dispositivos de balanceo es importante para promover el rendimiento suave y fiable de cualquier mecanismo rotativo. Gracias a estas soluciones tecnológicas avanzadas, es posible disminuir significativamente las oscilaciones, el sonido y la tensión sobre los cojinetes, prolongando la longevidad de partes importantes.
De igual manera importante es el tarea que cumplen los sistemas de balanceo en la servicio al usuario. El asistencia especializado y el mantenimiento constante empleando estos sistemas facilitan ofrecer servicios de excelente calidad, incrementando la satisfacción de los consumidores.
Para los propietarios de negocios, la aporte en unidades de ajuste y medidores puede ser fundamental para mejorar la eficiencia y desempeño de sus sistemas. Esto es especialmente importante para los dueños de negocios que manejan reducidas y medianas negocios, donde cada elemento cuenta.
Además, los sistemas de ajuste tienen una gran aplicación en el ámbito de la protección y el monitoreo de excelencia. Posibilitan identificar posibles problemas, reduciendo mantenimientos caras y averías a los aparatos. Incluso, los información recopilados de estos dispositivos pueden emplearse para mejorar procesos y mejorar la visibilidad en buscadores de investigación.
Las campos de aplicación de los equipos de calibración abarcan numerosas ramas, desde la producción de vehículos de dos ruedas hasta el monitoreo de la naturaleza. No importa si se considera de grandes manufacturas manufactureras o modestos espacios caseros, los aparatos de ajuste son necesarios para promover un funcionamiento productivo y sin riesgo de paradas.
https://www.sitiosperuanos.com/author/andrelumpki/
submariner vs explorer
https://telegra.ph/Pepsi-meteorite-rolex-03-20
Experience Dutch gaming at Boomerang Netherlands today. Start [url=https://coub.com/gameworld]bonus crab casino[/url] to explore a seamless blend of local culture, exciting games, and exclusive offers that cater perfectly to players from the Netherlands.
rolex imposter watches
https://docs.brdocsdigitais.com/index.php/Rolex_88W
least expensive rolex watch
https://telegra.ph/Rolex-904l-steel-03-20
rolex datejust 2 tone
https://telegra.ph/Rolex-band-replacement-03-20
rolex milgauss copy
https://telegra.ph/Rolex-quartz-price-03-20
rolex oyster perpetual 41mm for sale
https://telegra.ph/Yacht-master-rhodium-40mm-03-20
this article
[url=https://www.reddit.com/r/CoachScam/comments/1ibarjp/kreativstorm_reviews_experience_with_their/]Kreativstorm Training Program Reviews[/url]
best place to buy a used rolex
https://telegra.ph/Daytona-replica-for-sale-03-20
rolex datejust dupe
https://yrr.ens.mybluehost.me/index.php/Rolex_60I
womens gold rolex
https://www.ventura.wiki/index.php/Rolex_83F
china rolex wholesale
https://telegra.ph/Where-to-buy-cheap-rolex-watches-03-20
Jogue na [bet7](https://bet-7-br.com) e Desfrute de Jogos Populares e Bônus Recompensadores
stainless steel submariner rolex price
https://imoodle.win/wiki/User:GladisL1970632
https://cryptocurrence.wiki/index.php?title=User:FreemanLaforest
124060 rolex
https://telegra.ph/Rolex-watch-mother-of-pearl-03-20
best quality replica watches
https://systemcheck-wiki.de/index.php?title=Rolex_64l
[url=https://kraken.xn--onon-hya.com/]кракен даркнет[/url] – kraken зеркала, кракен сайт
rolex datejust oyster perpetual silver
https://wiki.vocokai.com:443/index.php/Rolex_53O
rolex oyster perpetual datejust 2 tone
https://Ragnafrost.wiki/index.php/Rolex_96q
https://kreosite.com/index.php/Rolex_19X
МВПОЛ предлагает услуги по формированию промышленных полов. Наша команда состоит из грамотных сотрудников, которые применяют новейшее оборудование, а также инструменты. Все работы в кратчайшие сроки осуществляются. Ищете промышленные полы москва? Mvpol.ru – тут есть возможность посмотреть условия сотрудничества и стоимость услуг. На портале наши объекты представлены. Мы тщательно контролируем то, чтобы любой наш проект был закончен в соответствии с высочайшими стандартами. Мы знаем, что надо заказчику. У вас от сотрудничества с нами останутся прекрасные впечатления.
Jogue na [522bet](https://522-bet-br.com) e Descubra os Jogos de Azar Populares e Bônus Que Vão Tornar Sua Experiência Inesquecível
For users who prefer to place bets and play casino games from mobile devices, the issue of using a promo code in the 1XBET mobile application (APK) is relevant https://ritmohost.com/cpanel/nelp/index.php?1xbet_today_promo_code_bonus_up_to_130.html
Одним из преимуществ 1Го Казино является простота и доступность. Регистрация аккаунта на сайте занимает несколько минут 1Go Casino
[url=https://veimmuseum.ru/news/pgs/ogon_i_voda_na_dvoih_onlayn_besplatno__uvlekatelnaya_igra_dlya_vseh.html]https://veimmuseum.ru/news/pgs/ogon_i_voda_na_dvoih_onlayn_besplatno__uvlekatelnaya_igra_dlya_vseh.html[/url] шарики играть бесплатно и без регистрации на русском во весь экран
СанОбработка – компания, которая для выполнения чистоты и безопасности вашего дома предоставляет комплексные решения. Даем гарантию на персональный подход к любому клиенту. С удовольствием вам поможем. Вы будете довольны качеством обслуживания и выгодными ценами. Ищете дератизации от клопов Тюмень? Sanobrabotkarf.ru – здесь представлены отзывы наших клиентов. Персонал грамотный и доброжелательный. Специалисты детально объяснят все этапы работы и ответят на вопросы. Они применяют новейшие технологии и сертифицированные препараты. Защитите уже сегодня свой дом!
Эта статья сочетает в себе как полезные, так и интересные сведения, которые обогатят ваше понимание насущных тем. Мы предлагаем практические советы и рекомендации, которые легко внедрить в повседневную жизнь. Узнайте, как улучшить свои навыки и обогатить свой опыт с помощью простых, но эффективных решений.
Изучить вопрос глубже – http://www.creativesippin.com/four-frequent-forms-of-essays-you-really-need-to-know
https://healthwiz.co.uk/index.php?title=Rolex_32r
weaphones полная версия [url=https://apk-smart.com/igry/simulyatory/1576-weaphones-firearms-sim-vol-1-polnaja-versija.html]https://apk-smart.com/igry/simulyatory/1576-weaphones-firearms-sim-vol-1-polnaja-versija.html[/url] weaphones полная версия
P.S Live ID: K89Io9blWX1UfZWv3ajv
P.S.S [url=https://siritaikoto.com/2024/02/18/royal-mutch/#comment-10819]Программы и игры для Андроид телефона[/url] [url=https://multinews.lv/seksualitate-lauliba-un-celibata/]Программы и игры для Андроид телефона[/url] [url=https://foro.muelendhir.com/showthread.php?tid=49560&pid=290068#pid290068]Программы и игры для Андроид телефона[/url] 5da0bc5
http://www.infinitymugenteam.com:80/infinity.wiki/mediawiki2/index.php/User:JoannKittelson6
[7kbet](https://7k-bet-br.com): Cadastre-se e Ganhe 100$ de Bônus para Apostar!
watch market crash
https://wiki.aquarian.biz/index.php?title=Rolex_45H
ในขณะเดียวกัน [url=https://mm88-th.com]mm88[/url] ยังมอบโบนัสมากมายให้กับผู้เล่นทั้งใหม่และเก่า โดยเฉพาะการสมัครสมาชิกใหม่ที่มีโบนัสต้อนรับที่คุ้มค่า และโปรโมชั่นต่างๆ ที่จะช่วยให้คุณเพิ่มโอกาสในการชนะการเดิมพันมากขึ้น โบนัสเหล่านี้มีทั้งในรูปแบบของเงินคืน ฟรีสปิน และโบนัสพิเศษในเกมต่างๆ ที่สามารถใช้ได้จริง ทำให้ทุกการเดิมพันของคุณสนุกสนานและมีโอกาสสร้างรายได้มากขึ้น
[url=https://kraken.xn--onon-hya.com/]ссылка кракен[/url] – kraken ссылка, ссылка kraken
original rolex explorer
https://menuaipedia.menuai.id/index.php/User:AlenaPearce
buy a fake rolex
https://telegra.ph/Knock-of-watches-03-20
https://bombergirl-esp.lol/index.php/Rolex_75S
Зимой мегаполис окутан в снегу, создавая неудобства для транспорта и людей. Мы решаем эту задачу! Компания «Снегоплав» – профессионал в сфере снегоочистки, предлагающий передовые решения для городских властей. Наши технологии обеспечивают возможность быстро и эффективно убирать снежные завалы, сокращая затраты и повышая безопасность на дорогах. Каждая [url=https://snegoplav.com/home/newreviews/]снегоплавильные установки отзывы[/url] от «Снегоплав» – это сочетание долговечности, высокой эффективности и простоты в использовании.
Мы производим оборудование, предназначенное для перерабатывает снег в воду непосредственно на объекте. Это современный и рациональный способ освобождения улиц, уменьшающий потребность от необходимости вывоза снега за город. В нашем ассортименте – переносные и мощные установки, созданные под разные условия работы. Если вам нужна надёжная [url=https://snegoplav.com/]принцип работы снегоплавильного оборудования[/url] мы подберём оптимальное устройство для всех масштабов уровня задач – для частных нужд до областных центров.
Обратившись к нам, вы получаете инновационные технологии, сертифицированное оборудование и профессиональное обслуживание. Наши установки – выбор строительных компаний, промышленных объектов и организаций. Свяжитесь с нами: +7 (495) 123-45-67 или приезжайте к нам: г. Москва, ул. Примерная, д. 10.
[url=https://suvredut.ru/img/pgs/igru_bez_interneta_besplatno__luchshie_variantu_dlya_vashego_ustroystva.html]https://suvredut.ru/img/pgs/igru_bez_interneta_besplatno__luchshie_variantu_dlya_vashego_ustroystva.html[/url] играть в слова из слова бесплатно на русском без регистрации и бесплатно онлайн
медицинская справка нарколога купить https://kupit-spravku-spb.ru
[url=https://kraken.xn--onon-hya.com/]как зайти на кракен[/url] – кракен актуальная ссылка, кракен ссылка
Заказала пионы на день рождения, все гости были в восторге!
Доставка цветов Томск
На сайте http://ammely.ru представлены интересные, увлекательные статьи, которые точно понравятся женской аудитории. Опубликован контент о моде, красоте, здоровом образе жизни. Также имеются и полезные рецепты, которые идеально подойдут на каждый день либо на праздник, если внезапно нагрянули гости. Есть увлекательные рекомендации на тему дома, дизайна. Вам будет интересно узнать и о звездах, о том, как лучше и веселей провести праздник.
На сайте https://xn—-8sbkf3cqel4a6c.xn--p1ai/ рассчитайте стоимость такой услуги, как ДТФ-печать на сувенирной продукции, текстиле. Все изображения отличаются безупречным качеством и цветопередачей, высокой детализацией. При необходимости вы сможете получить профессиональную консультацию. Главное направление деятельности компании – это DTF-печать на повседневной, корпоративной одежде, включая худи, футболки, толстовки. Все заказы выполняются с применением инновационных материалов, качественного оборудования.
[url=http://svidetel.su/jsibox/article/index.php?ogon_i_voda_onlayn___uvlekatelnoe_prikluchenie_dlya_vseh.html]http://svidetel.su/jsibox/article/index.php?ogon_i_voda_onlayn___uvlekatelnoe_prikluchenie_dlya_vseh.html[/url] из большого слова составить маленькие слова
Calypso – удобный сервис аренды яхт. Наш подход ориентирован на удовлетворение персональных потребностей каждого клиента. Мы готовы обеспечить высочайший уровень сервиса и безопасность. Ваше путешествие на яхте станет невероятным! Ищете аренда яхты Сочи? Sochi.calypso.ooo – здесь можете ознакомиться с условиями заказа. На сайте вы найдете самые выгодные предложения. Поможем вам выбрать яхту, соответствующую вашим требованиям и пожеланиям. Присоединяйтесь к Calypso. Позвольте нам превратить ваши мечты об идеальном отдыхе в реальность!
На сайте http://kinomanhdc.online представлены фильмы в огромном многообразии. Перед вами как популярное кино, которое понравится всем без исключения, так и ожидаемые новинки, которые точно никого не оставят равнодушным. Есть возможность подобрать решение по определенной тематике: про вампиров, космос, подростков, девушек, любовь и многое другое. Здесь вы найдете и сериалы, киноленты за прошедшие годы. На сайте также представлены и мультфильмы для детей разного возраста, семейного просмотра. Все это вы сможете смотреть прямо сейчас и в режиме реального времени.
Дешевые Windows 11 и windows 10 ключи от Партнера Майкрософт Софтлайн, моментальная доставка, ритейл ключ купить ключ активации
next page
[url=https://galaxy-swapper.org/]galaxy swapper download[/url]
Миру могут угрожать вспышки неизвестных ранее болезней https://x.com/Fariz418740/status/1902696858473910276
Казино Аркада – это современная онлайн-платформа, которая максимально быстро стала одним из лидеров в игорной индустрии Arkada Casino официальный сайт
В ассортименте Банда Казино представлен широкий выбор развлечений. Здесь имеются и популярные краш-игры, завоевавшие признание огромного количества игроков Казино Banda
Спасибо за быструю доставку, всё вовремя!
букет невесты
[url=http://tankfront.ru/axis/pages/?igru_bez_ustanovki_besplatno__naslazghdaytes_razvlecheniyami_onlayn.html]http://tankfront.ru/axis/pages/?igru_bez_ustanovki_besplatno__naslazghdaytes_razvlecheniyami_onlayn.html[/url] топ игр про животных на пк
Looking for the best cryptocurrency exchange rates? Discover the Coinxes cryptocurrency exchange https://coinxes.io/ – the best rates, the lowest commissions. The Coinxes platform is a digital exchange that allows users to buy and sell cryptocurrencies from anywhere in the world. Unlike a regular exchange, it boasts a large number of useful features.
К-ЖБИ гарантирует высочайшее качество продукции и строгое соблюдение сроков. Завод обладает гибкими производственными мощностями для выполнения заказов по чертежам заказчика. С радостью ответим на вопросы, позвоните нам по телефону. Ищете балки для гражданского и индивидуального строительства? Gbisp.ru – тут есть возможность заявку оставить. Укажите в форме ваш e-mail, имя и контактный номер. Далее нажмите кнопку «Отправить». Выполняем быструю доставку продукции. Обеспечиваем современный уровень обслуживания каждому заказчику. К взаимовыгодным партнерским отношениям стремимся!
[url=https://www.colonoscopy.ru/PHPMailer/?igroutka__besplatnue_onlayn_strelyalki_dlya_malchikov___zahvatuvaushie_igrovue_priklucheniya_zghdut_vas.html]https://www.colonoscopy.ru/PHPMailer/?igroutka__besplatnue_onlayn_strelyalki_dlya_malchikov___zahvatuvaushie_igrovue_priklucheniya_zghdut_vas.html[/url] игры для девочек бесплатно кулинария кухня сары играть онлайн
Розыгрыш сертификатов OZON и WB – участвуй бесплатно!
Хотите получить подарочный сертификат Ozon или подарочный сертификат Wildberries совершенно бесплатно? Тогда подписывайтесь на наш Telegram-канал и участвуйте в розыгрышах!
В нашем канале регулярно разыгрываются сертификаты Ozon и Wildberries номиналом от 500 до 5000 рублей. Получить их можно легко:
1.Подписывайтесь на канал
2.Участвуйте в розыгрышах, выбирая билеты
3. Ожидайте результаты – победители определяются случайным образом!
Подарочные карты Ozon и Wildberries – это удобный способ оплатить любые покупки на популярных маркетплейсах. Участвуйте в розыгрышах и выигрывайте!
Присоединяйтесь прямо сейчас: Розыгрыш сертификатов OZON WB
Служба Эвакуации 911 предлагает качественные услуги. Мы оперативно и безопасно любой транспорт перевозим. Владеем современным автопарком эвакуаторов. Вы можете быть уверены в ответственном подходе к решению вашей проблемы. Ищете Эвакуатор Пенза? Penza-evakuator.ru – портал, где вы сможете узнать, какие мы готовы эвакуировать виды техники. Можем дешевле, чем у конкурентов предложить свои услуги. Работаем 24/7 без выходных. Помогаем клиентам даже в самых тяжелых ситуациях. Доверьте транспортировку вашего авто службе спасения 911.
Ищете оригинальный мерч от любимых российских и зарубежных звезд, фестивалей и шоу? Посетите https://showstaff.ru/ – мы с 2002 года радуем наших клиентов качественной продукцией, доставкой по всей России и поддержкой талантливых артистов. Заходите на наш сайт и выбирайте свой стиль! ShowStaff – это магазин оригинального мерча, официально и качественно. Посетите каталог, и вы обязательно найдете для себя уникальные вещи!
Карамельная Фабрика предлагает леденцы на палочке, мармелад и др. Ежедневно наши карамелье создают шедевры. В нашем интернет-магазине есть возможность приобрести красивые и вкусные сладости. При возникновении вопросов, обращайтесь к нам, мы с удовольствием на них ответим. Ищете конфеты на заказ? Karamelfabrika.ru – тут узнаете, как карамель мы делаем. Не останавливаемся на достигнутом и постоянно расширяем ассортимент. Проводим интересные мастер-классы, которые для детей станут источником радости и дадут возможность взрослым в детство ненадолго вернуться. Для вас карамельное настроение создаем!
Магазин «Столица Швейных Машин» в Челябинске https://shveichel.ru/ — это надежный поставщик качественной швейной техники: швейные машинки, оверлоки, промышленные швейные машины, гладильное оборудование, все для вышивки от мировых брендов: Juki, Bernina, Aurora, Janome, Leader. Профессиональные консультации: сотрудники помогут выбрать швейную технику под ваши задачи. Гарантия и сервис: товары сопровождаются гарантией, а сервисный центр обеспечивает ремонт и обслуживание. Удобное расположение: магазин находится по адресу Молодогвардейцев 64.
Названы доказательства пребывания на Земле более высоких цивилизаций https://x.com/Fariz418740/status/1903334968513745235
[url=https://mytaganrog.com/media/madzghong_igrat_besplatno__zahvatuvaushee_prikluchenie_v_mir_drevney_igru.html]https://mytaganrog.com/media/madzghong_igrat_besplatno__zahvatuvaushee_prikluchenie_v_mir_drevney_igru.html[/url] хорроры по сети на пк скачать
Я думаю Вы искали именно про Flagman Casino вход или возможно хотите поведать больше про Флагман Казино мобильная версия Флагман Казино
На сайте https://xn--90a1af.xn—-8sbkf3cqel4a6c.xn--p1ai/ закажите обратный звонок для того, чтобы больше узнать о таких полезных, нужных услугах, как: ДТФ-принты на заказ, ДТФ-печать на ткани, ДТФ-печать на спецодежде либо коже. Здесь же вы ознакомитесь с расценками на перечисленные работы. В любом случае они остаются на доступном уровне. Услуги подойдут самым разным сферам бизнеса, а также бизнесменам, которые только пытаются раскрутиться. ДТФ-печать подойдет артистам, актерам, а также дизайнерам.
Melbet – популярный букмекер, предлагающий отличные бонусы для новых игроков. При регистрации можно получить фрибет, введя специальный промокод Melbet. Это позволяет делать ставки без вложений, увеличивая шансы на выигрыш. Промокод melbet_promokod_na_fribet_pri_registratsii активирует бонусные средства, которые можно использовать на спортивные события и казино. Melbet радует пользователей высокими коэффициентами, широкой линией ставок и удобными способами вывода средств. Не упустите шанс начать игру с бонусом – регистрируйтесь и получайте фрибет!
Lolzteam Market большой выбор аккаунтов по доступным ценам предоставляет. Здесь можете найти то, что вам необходимо. Каждый аккаунт подвергается тщательной проверке на подлинность. https://lzt.market/ – сайт для тех, кто хочет приобрести аккаунты с гарантией безопасности. На платформе много категорий представлено, включая Warface, Steam, Riot Games, Supercell, War Thunder, Instagram, World of Tanks, Minecraft, Social Club. Специалисты поддержки круглосуточно работают. Чтобы вам помочь, они все возможное сделают.
Kometa Casino – это игровая среда, где азартные игры сочетаются с новейшими разработками, обеспечивая гостям удобство и стабильность Kometa Casino официальный сайт
Пицца на итальянском тесте Жаждете сочного итальянского наслаждения, не покидая Кемерово? Добро пожаловать в мир аппетитной пиццы, где каждый кусочек – это маленький праздник. Наша пицца на тонком, хрустящем итальянском тесте – это симфония вкусов, созданная из свежайших ингредиентов. Живете в Теплом ключе? Мы доставим ваш заказ прямо к вашей двери, быстро и аккуратно. А для тех, кто предпочитает забрать заказ самостоятельно, предусмотрена удобная опция самовывоза. Но это еще не все! Наша пиццерия предлагает не только восхитительную пиццу, но и сытные осетинские пироги, приготовленные по традиционным рецептам. Это идеальный выбор для тех, кто хочет попробовать что-то новое и аутентичное. Ищете идеальное место для празднования дня рождения? Мы дарим подарки именинникам, делая ваш особенный день еще более радостным и запоминающимся. Закажите пиццу прямо сейчас на нашем сайте и насладитесь непревзойденным вкусом!
Сайт https://joinwork.ru/ представляет собой фриланс биржу, на которой вы сможете найти специалистов по дизайну, разработке, на тему SEO. Также получится воспользоваться услугами из сферы рекламы, социальных сетей, аудио, видео. Есть возможность воспользоваться безопасной сделкой, которая очень простая. Предложения от вас увидит огромное количество посетителей, а потому они захотят воспользоваться ими. Вы сможете оформить свой профиль так, будто это магазин. А в блоге вы найдете много полезных статей.
[url=https://ma.by/docs/pages/?igru_na_73.html]https://ma.by/docs/pages/?igru_na_73.html[/url] игры для девочек бесплатно 8 лет 3 класс хорошие
Ищете купить жби? Global-gbi.ru портал Завода ЖБИ в Санкт-Петербурге, и вы отыщите по доступным ценам большой выбор продукции. Глобал ЖБИ – компания, которая является крупнейшим в регионе изготовителем изделий из железобетона и бетона. У нас широкая номенклатура и современное оборудование. Мы работаем со строительными фирмами, частными лицами и иными организациями. Посмотрите каталог товаров и вы обязательно найдете для себя необходимую продукцию.
В этой статье вы узнаете, где геймеры покупают приватные читы для PUBG, CS 2, Call Of Duty и других популярных онлайн игр call of duty читы
Телеграм-канал t.me/official_izzicasino будет, несомненно, вам полезен. Здесь рассказываем, как выиграть в онлайн казино. Вы узнаете, что делать, если автоматы не загружаются. Подготовили несколько рекомендаций, которые дадут возможность вам на выигрыш в Izzi Casino увеличить шансы. Играйте в увлекательные игры прямо сейчас. Ищете izzi casino бездепозитный бонус? T.me/official_izzicasino – канал, подпишитесь скорее на него, чтобы в курсе последних новостей быть и ни одной интересной акции не пропустить. Иззи – казино с удобной навигацией и новейшим дизайном. Убедитесь в этом сами!
До конца марта эти 4 знака Зодиака обретут личное счастье https://x.com/Fariz418740/status/1903525881395507337
Зимой город погружается в снегу, создавая проблемы для движения и жителей. Мы ликвидируем эту задачу! Компания «Снегоплав» – эксперт в сфере снегоочистки, предлагающий передовые решения для коммунальных служб. Наши технологии помогают в кратчайшие сроки и результативно устранять снежные завалы, оптимизируя временные и финансовые расходы и гарантируя комфорт на дорогах. Каждая [url=https://snegoplav.com/]мобильная снегоплавильная станция[/url] от «Снегоплав» – это идеальный баланс долговечности, высокой эффективности и удобства эксплуатации.
Мы проектируем оборудование, способное перерабатывает снег в воду прямо на месте. Это экологичный и экономичный способ уборки улиц, избавляющий от затрат транспортировки снежных отложений. В нашем каталоге – компактные и мощные модели, созданные под специфические условия эксплуатации. Если вам нужна эффективная [url=https://snegoplav.com/]снегоплавильная установка спу 3[/url] мы поможем найти рациональное решение для всех масштабов масштаба – от дворовых территорий до областных центров.
Обратившись к нам, вы становитесь обладателем передовые технологии, гарантию качества и сопровождение на всех этапах. Наши установки – наилучшее решение городских предприятий, промышленных объектов и организаций. Оставьте заявку: +7 (495) 123-45-67 или ждём вас: г. Москва, ул. Примерная, д. 10.
[url=https://cofe.ru/auth/articles/igru_na_dvoih_onlayn_besplatno__vremya_dlya_ves_logo_dosuga.html]https://cofe.ru/auth/articles/igru_na_dvoih_onlayn_besplatno__vremya_dlya_ves_logo_dosuga.html[/url] раскраска динозавров для детей 7 8 лет
Пневмозаглушки используются для надежного перекрытия трубопроводов диаметром от 40 до 2000 мм трубные пневмозаглушки
подробнее https://images.google.ps/url?q=https://travelanswer.ru/questions/gde-nayti-punkti-zolotoy-koroni-v-fethie-dlya-snyatiya-deneg.html
страница https://maps.google.kg/url?q=https://protos.ru/catalog/category/shnursutazh
Whether you are into sporting activities betting, casino video games, or Are living casino action, the 1xBet promo code makes certain you get by far the most out of your very first deposit https://webhitlist.com/profiles/blogs/code-promo-d-inscription-1xbet-bonus-jusqu-130
Читать далее https://www.google.sr/url?q=https://travelanswer.ru/questions/kak-otkrity-barbersop-ili-salon-krasoti-v-izraile-osobennosti-dlya-repatriantov.html
продолжить https://www.google.com.ar/url?sa=t&rct=j&q=gana+dinero+escribiendo&esrc=s&source=web&cd=1&cad=rja&ved=0ahUKEwi1hJmQsKfPAhWG2T4KHersDqsQFggcMAA&url=https://travelanswer.ru/questions/russkoyazichnie-shkoli-na-yuge-turtsii-esty-li-varianti-v-marmarise-i-okrestnostyah.html
[url=https://www.amnis.ru/ajax/articles/?igru_risovat_onlayn__tvorchestvo_na_konchikah_palcev.html]https://www.amnis.ru/ajax/articles/?igru_risovat_onlayn__tvorchestvo_na_konchikah_palcev.html[/url] топ 10 игр на двоих по сети
here are the findings
[url=https://realbackpack.com/]app backpack[/url]
image source [url=https://realbackpack.com]backpack web[/url]
Заказала пионы подруге, она сказала, что это лучший подарок!
Цветы Томск
Цель студии MARBL DESIGN – создать интерьер, который даст вам возможность находиться в ресурсном состоянии и поддерживать ритм жизни. С вами будут работать самые лучшие специалисты в области создания уюта и комфорта. Сотрудничаем с надежными поставщиками мебели и материалов. Ищете дизайн жилых помещений? Marbl.ru – тут можете посмотреть портфолио проектов. Также тут указаны цены на наши услуги. Выполним по недорогой цене красивый дизайн квартиры. Можете оставить заявку на сайте. Мы в ближайшее время перезвоним вам. Будем рады обсудить все интересующие вас вопросы.
Названа дата, когда привычная всем жизнь на Земле станет невозможной https://x.com/Fariz418740/status/1903674512593359008
На сайте https://petersburglife.ru/ ознакомьтесь с зеркалом популярного онлайн-заведения «Arkada». Это удивительная и уникальная платформа, которая предназначена для разнообразных игр, интересного времяпрепровождения. Гемблер по достоинству оценит понятный, простой в управлении интерфейс, сориентируется в выборе. Всем новичкам за регистрацию положен бонус. Здесь вы найдете все необходимое, включая классические игры, а также удивительные новинки. Также есть казино с живыми дилерами.
Baliyants.com – ваш верный источник знаний в области маркентинга. В работе с каждым клиентом в бизнес детально погружаемся. Постоянно данные анализируем, чтобы не упустить ничего. Воспользуйтесь нашими услугами! Ищете маркетинг ? Baliyants.com – здесь представлены уникальные авторские проекты. Они для того созданы, чтобы помочь действенно использовать рекламные кампании, повысить рентабельность ваших маркетинговых усилий и оптимизировать бюджеты. Драгоценность агентства Baliyants – это специалисты. Мы работаем только на результат!
чемпион слотс
https://telegra.ph/Rolex-explorer-size-03-20
На сайте http://gorodnsk63.ru вы сможете почитать интересные, любопытные новости, посвященные городу Самаре и не только. К примеру, жителей ожидает ретроградный Меркурий, который продлится вплоть до 7 апреля текущего года. Также есть новости о заводе «Балтика» и о том, что он больше не будет сотрудничать с крафтовым брендом. Кроме того, в Самаре будут проводиться дорожные работы в 2025 году. А с 19 марта ожидаются ночные заморозки. Интересные, любопытные и увлекательные новости выкладываются регулярно, чтобы с ними ознакомились и вы.
chamnpion slots
чемпион слотс казино
Владение загородным домом открывает неограниченные возможности для экспериментов с дизайном и оформлением, особенно когда речь заходит о ванной комнате https://x-serial.ru/dizayn-i-interer/12733-zerkalnye-panno-roskoshnoe-i-eksklyuzivnoe-ukrashenie-interera.html
https://bgr.sgk.temporary.site/index.php/User:OpheliaSilvers2
[url=https://tj-service.ru/news/?igru_onlayn_besplatno__gde_poigrat_.html]https://tj-service.ru/news/?igru_onlayn_besplatno__gde_poigrat_.html[/url] слова из букв м о р е т
### The Ultimate Guide to Services Cleaning: Why It Matters and How to Choose the Best
In today’s fast-paced world, maintaining a clean and organized space is more important than ever. Whether it’s your home, office, or commercial property, *services cleaning* offers a practical solution to keep your environment spotless without the hassle. This article explores the benefits of professional cleaning services, what to look for when hiring a team, and why investing in these services is a smart choice for both individuals and businesses.
#### What Are Services Cleaning?
*Services cleaning* refers to professional cleaning solutions provided by trained experts who handle everything from routine tidying to deep sanitation. These services can be tailored to residential spaces, workplaces, or specialized facilities like gyms, restaurants, or medical offices. Unlike DIY cleaning, professional services bring expertise, advanced tools, and eco-friendly products to ensure a thorough and efficient job.
#### The Benefits of Professional Services Cleaning
Hiring a *services cleaning* provider comes with a range of advantages that go beyond just a shiny floor or dust-free shelves. Here’s why they’re worth considering:
1. **Time-Saving Convenience**
Life is busy, and cleaning often falls to the bottom of the priority list. Professional cleaning services free up your schedule, letting you focus on work, family, or relaxation while experts handle the dirt and grime.
2. **Healthier Living Spaces**
Dust, allergens, and bacteria can build up over time, affecting air quality and health. *Services cleaning* teams use specialized techniques and products to eliminate these hazards, creating a safer environment for you and your loved ones.
3. **Enhanced Productivity for Businesses**
A clean workspace isn’t just aesthetically pleasing—it boosts employee morale and efficiency. Regular *services cleaning* for offices ensures a professional atmosphere that leaves a lasting impression on clients and staff alike.
4. **Customized Solutions**
From carpet cleaning to window washing, professional services offer tailored packages to meet your specific needs. Whether it’s a one-time deep clean or ongoing maintenance, you get exactly what your space requires.
#### How to Choose the Right Services Cleaning Provider
Not all cleaning services are created equal. To ensure you’re getting the best value for your money, here are some key factors to consider when selecting a *services cleaning* company:
– **Reputation and Reviews**
Look for providers with positive feedback from past clients. Online reviews and testimonials can give you insight into their reliability and quality of work.
– **Experience and Expertise**
A team with years of experience in *services cleaning* will know how to tackle tough stains, delicate surfaces, and industry-specific challenges (e.g., sanitizing a healthcare facility).
– **Eco-Friendly Options**
If sustainability matters to you, opt for a service that uses green cleaning products and practices. This not only benefits the planet but also reduces exposure to harsh chemicals.
– **Flexible Scheduling**
The best *services cleaning* companies work around your timetable, offering evening, weekend, or emergency cleaning options to minimize disruption.
– **Transparent Pricing**
Avoid surprises by choosing a provider with clear, upfront costs. Ask for a detailed quote that outlines what’s included in the service.
#### When Should You Invest in Services Cleaning?
There’s no one-size-fits-all answer to when you need professional *services cleaning*. However, here are some common scenarios where it makes sense:
– **Moving In or Out**: A deep clean ensures your new home is fresh or your old space is spotless for the next occupant.
– **Post-Renovation**: Construction dust and debris require heavy-duty cleaning that’s best left to the pros.
– **Seasonal Refresh**: Spring cleaning or pre-holiday tidying can benefit from expert help.
– **Business Upkeep**: Regular cleaning keeps commercial spaces presentable and hygienic year-round.
#### The Future of Services Cleaning
The *services cleaning* industry is evolving with technology and changing consumer needs. Robotic vacuums, UV sanitation tools, and smart scheduling apps are making professional cleaning more efficient and accessible. As demand grows, companies are also focusing on sustainability, offering biodegradable products and energy-efficient methods to appeal to eco-conscious clients.
#### Final Thoughts
Investing in *services cleaning* is more than just outsourcing a chore—it’s a step toward a cleaner, healthier, and more enjoyable space. Whether you’re a homeowner looking to reclaim your weekends or a business owner aiming to impress clients, professional cleaning services deliver unmatched results. Take the time to research and choose a reputable provider, and you’ll see why *services cleaning* is a game-changer for modern living. Ready to transform your space? Start exploring your options today!
http://wiki.svencremer.com/index.php/Steam_Hippo_68d
This promotion is an excellent way to boost your betting experience, allowing you to bet on cricket, football, and other major sporting events with a larger bankroll https://webhitlist.com/profiles/blogs/d-bloquez-des-bonus-avec-le-code-promo-1xbet?xg_source=activity
http://cambiareilcampo.it/mediawiki-1.42.1/index.php?title=Rolex_29G
Заказала цветы на свадьбу, всё было идеально!
букет цветов томск
Cargo Jet – компания, которая с закупками китайских товаров оптом помогает. Начните свой бизнес с нами. Мы предлагаем широкий выбор продукции. Обладаем достаточным опытом и умеем находить уникальные решения для ваших логистических задач. Гордимся тем, что нас выбрали более 3000 клиентов. Ищете доставка из Китая? Cargo-jet.ru – тут представлена о нас более подробная информация. Уверены, что вы останетесь довольны результатом и качеством наших услуг. С Cargo Jet доставка из Китая в РФ становится выгоднее и проще. Начните уже сейчас экономить!
[url=https://sorvachev.com/code/pages/igru_io__zahvatuvaushie_srazgheniya_za_vuzghivanie.html]https://sorvachev.com/code/pages/igru_io__zahvatuvaushie_srazgheniya_za_vuzghivanie.html[/url] задания для малышей на английском языке
visit their website
[url=https://realbackpack.com/]wallet backpack[/url]
https://cryptocurrence.wiki/index.php?title=Rolex_70r
Сюрприз удался, любимая в восторге!
заказ цветов томск
https://pubhis.w3devpro.com/mediawiki/index.php?title=Rolex_15n
[url=https://offroadmaster.com/rotator/articles/?igru_na_dvoih_onlayn___veselo__uvlekatelno_i_udobno.html]https://offroadmaster.com/rotator/articles/?igru_na_dvoih_onlayn___veselo__uvlekatelno_i_udobno.html[/url] скачать игры для расслабления на пк
Motor Casino – это современный игорный клуб, предлагающий широкий выбор развлечений, включая игровые автоматы, карточные игры и live-дилеров Motor Casino официальный сайт
https://telegra.ph/Rolex-reproduction-watch-03-20
[url=https://billionnews.ru/templates/artcls/index.php?igru_na_74.html]https://billionnews.ru/templates/artcls/index.php?igru_na_74.html[/url] гонки с крутым тюнингом на пк
Looking for prediccion del precio del oro? Тhetradable.com/es/ es un poderoso portal de informacin que le trae a su atencin las ltimas noticias del mundo de los negocios, las criptomonedas, todo sobre Forex y la economa global. Aqui encontrara informacion interesante sobre los mercados de valores, criptomonedas, asi como metales preciosos como el oro y otros activos clave.
Прежде чем принять решение о покупке автомобиля, определите его рыночную стоимость и техническое состояние. Сравните цены аналогичных моделей в интернете и внимательно изучите мнения владельцев http://victorieux.org/index.php/forum/welcome-mat/4686#4661
https://chrisophia.wiki/index.php/Rolex_20l
https://telegra.ph/Rolex-explorer-homage-03-20
[url=http://khomus.ru/lib/pages/igru_dlya_malchikov_onlayn_besplatno_bez_registracii.html]http://khomus.ru/lib/pages/igru_dlya_malchikov_onlayn_besplatno_bez_registracii.html[/url] решение судоку онлайн бесплатно без регистрации
http://wiki.myamens.com/index.php/Rolex_55Y
купля продажа аккаунта marketplace-sell-accounts.ru
маркетплейсы для покупки аккаунта akkaunt-marketplace.ru
продажа аккаунтов https://account-service213.ru
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние возможности.
Наши товары:
Плоская мишень CoFe для распыления сплавов
РедМетСплав предлагает широкий ассортимент качественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их происхождение. Дружелюбная помощь – то, чем мы гордимся – мы на связи, чтобы улаживать ваши вопросы и находить ответы под специфику вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
Наша продукция:
Лента магниевая G-Z6Th2Zr – AFNOR NF A57-704 РљСЂСѓРі магниевый G-Z6Th2Zr – AFNOR NF A57-704 является идеальным решением для высокотехнологичных производств. Ртот РєСЂСѓРі изготовлен РёР· высококачественного магния Рё соответствует международным стандартам качества. РћРЅ обеспечивает отличные результаты РїСЂРё обработке различных поверхностей Рё имеет отличные антикоррозионные свойства. Рспользование РєСЂСѓРіР° гарантирует долговечность Рё надежность РІ работе. РќРµ упустите возможность купить РљСЂСѓРі магниевый G-Z6Th2Zr – AFNOR NF A57-704 Рё улучшить качество вашей продукции. Позаботьтесь Рѕ своем бизнесе СѓР¶Рµ сегодня!
Koch Market гарантирует к каждому клиенту персональный подход. Специализируемся на услугах дооснащение, полировка, тюнинг, детейлинг, оклейка. Обеспечиваем высокое качество работы. Ответим на вопросы и расскажем детальнее о действующих акциях. Ищете брендирование авто? Koch-market.ru – тут размещаем для автолюбителей полезные статьи. Цель Koch Market – сократить издержки, увеличить обороты и доходность вашего бизнеса. Оставьте заявку на сайте, и мы перезвоним в ближайшее время. Обсудим задачи, найдем наилучшее решение и запланируем работы.
Для ЖКХ, сельхозпредприятий и муниципальных объектов вопрос переработки мусора стоит особенно остро. [url=https://eco-sistem.com/]Оборудование для утилизации мусора[/url] от ЭКОСИСТЕМЫ решает задачу локальной переработки с максимальной эффективностью. Установки подбираются под нужды заказчика, могут работать автономно и легко обслуживаются. Это надёжный способ сократить затраты и обеспечить чистоту на объекте.
web link [url=https://www.ringsshop1.com]Department Stores Accessories Sunglasses[/url]
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наша продукция:
Хромовые гранулы
РедМетСплав предлагает обширный выбор качественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица товара подтверждена всеми необходимыми документами, подтверждающими их происхождение. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы улаживать ваши вопросы и находить ответы под требования вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
Наши товары:
Лента висмутовая L54865 – UNS Лента висмутовая L54865 – UNS предназначена для применения РІ различных отраслях, включая медицину Рё электронику. Обладая высокой прочностью Рё устойчивостью Рє РєРѕСЂСЂРѕР·РёРё, РѕРЅР° идеально РїРѕРґС…РѕРґРёС‚ для создания защитных систем Рё экранов. Благодаря СЃРІРѕРёРј уникальным характеристикам, лента висмутовая L54865 – UNS обеспечивает надежное соединение Рё долговечность. РќРµ упустите возможность купить Лента висмутовая L54865 – UNS РїРѕ выгодной цене. Достаточно просто добавить РІ РєРѕСЂР·РёРЅСѓ Рё оценить РІСЃРµ преимущества этого качественного продукта!
pop over to these guys [url=https://www.ringsshop1.com]Department Stores Accessories Sunglasses[/url]
https://empty3.one/wikilibre/index.php/Rolex_33r
https://orphelins-apocalypse.synology.me/mediawiki/index.php?title=Utilisateur:ReginaldOtoole
На сайте https://orliman.shop вы найдете огромное количество ортопедических товаров, созданных для вашего здоровья. В каталоге представлены коленные, голеностопные ортезы, а также корсеты. В большом количестве находятся комфортные приспособления для фиксации. Также имеются бандажи, стельки, специальная обувь. Все это представлено в огромном ассортименте и по доступным ценам. Перед вами новинки, а также те товары, которые пользуются огромным спросом. В магазине постоянно проходят увлекательные акции.
Отопление и водоснабжение – компания, которая квалифицированные услуги по монтажу отопления предоставляет. Мы предлагаем доступные цены. Сотрудничаем с ведущими изготовителями оборудования. Готовы для вашей семьи и вас создать теплый дом. Ищете монтаж водоснабжения? Santex-uslugi.ru – портал, где вы заявку можете оставить. Работаем с передовыми технологиями и современным оборудованием. Используем высококачественные материалы, чтобы гарантировать долговечность вашей системы отопления. Вы можете проконсультироваться с нами по интересующим вопросам.
https://libchrist.wiki/index.php/User:GiuseppeNicolay
https://bookslibrary.wiki/content/User:ElizabethIyr
Назван простой напиток, который спасает от повышения сахара в крови https://x.com/Fariz418740/status/1904006505147629966
Beterex – компания, которая имеет богатый опыт в решении разного уровня сложности задач. Мы выстроим наилучшую логистическую цепочку и снизим ваши расходы на перевозку грузов из Китая. Прозрачно работаем и оперативнее конкурентов. Гарантируем взятые на себя обязательства. Ищете представитель в Китае? Mybeterex.com – сайт, где представлена более детальная информация о компании Бэтэрэкс, рекомендуем с ней ознакомиться. Предлагаем исключительно услуги высокого качества. Найдем необходимых вам поставщиков и производителей в Китае. Любой запрос ваш будет на все 100% осуществлен.
[url=http://tiflos.ru/content/pags/igru_so_slovami___treniruem_um_i_poluchaem_udovolstvie.html]http://tiflos.ru/content/pags/igru_so_slovami___treniruem_um_i_poluchaem_udovolstvie.html[/url] игры про вторую мировую войну по сети
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
Обладая обширной линейкой редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние достижения.
Наша продукция:
Высокочистые плитки алюминия
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
С широким ассортиментом продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наша продукция:
“ерамическая мишень CeO2, плоская форма
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние успехи.
Наша продукция:
Плоская мишень Fe+Mn
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
поставляемая продукция:
Диск РёР· драгоценных металлов палладиевый 100С…5 РјРј ПДСР-40 РўРЈ РЁРёСЂРѕРєРёР№ выбор РґРёСЃРєРѕРІ РёР· драгоценных металлов для вашего автомобиля. Рксклюзивный дизайн, высокая устойчивость Рє РєРѕСЂСЂРѕР·РёРё, улучшенная управляемость Рё неповторимый стиль. Подчеркните индивидуальность вашего автомобиля СЃ нашими уникальными дисками.
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
Наша продукция:
Медная однораструбная редукционная переходная муфта РїРѕРґ пайку стандартная 42С…40.5 РјРј твердая пайка Рњ0Р± ГОСТ Р 52922-2008 Приобретите высококачественные медные однораструбные редукционные муфты РїРѕРґ пайку РѕС‚ Редметсплав для надежного соединения трубопроводов. Гарантированная прочность Рё устойчивость Рє РєРѕСЂСЂРѕР·РёРё. Рдеальны для различных систем отопления, водоснабжения Рё прочих технических целей.
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с высоким уровнем сервиса.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
Наши товары:
Медный пресс тройник 108 мм М2р ГОСТ Р52948-2008 Закажите титановые круги для широкого спектра применений от ведущего поставщика. Наши высококачественные титановые круги обладают высокой прочностью, устойчивостью к коррозии и широким спектром применений, от аэрокосмической промышленности до спортивного оборудования. Получите надежные и непревзойденные технические свойства, подробные характеристики и бесплатную консультацию прямо сейчас!
Мечтаете встретить свою любовь? Тогда вам непременно стоит посетить Лилибум! Портал удобную регистрацию предоставляет, открывает к новым знакомствам и дружбе двери. Мы стремимся обеспечить вам конфиденциальность и безопасность. Вас ждет доброжелательная атмосфера. Ищете замужние желают познакомиться сайт? Lilibum.ru – сайт, где можете прекрасно провести время. Порадует красивый и удобный интерфейс. Модераторы проверяют внимательно фотографии и профили пользователей. С Лилибум вы свою половинку обязательно найдете. Знакомьтесь, общайтесь и создавайте новые отношения!
РедМетСплав предлагает обширный выбор отборных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до масштабных поставок, мы обеспечиваем оперативное исполнение вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их происхождение. Дружелюбная помощь – наш стандарт – мы на связи, чтобы разрешать ваши вопросы по мере того как находить ответы под требования вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наша продукция:
Полоса магниевая G-Z5Zr – AFNOR NF A57-704 Порошок магниевый G-Z55Zr – AFNOR NF A65-717 представляет СЃРѕР±РѕР№ высококачественный РїСЂРѕРґСѓРєС‚, использующийся РІ различных отраслях. Ртот порошок обеспечивает отличные механические Рё химические свойства, что делает его идеальным для применения РІ авиационной Рё автомобильной промышленности. РЎ помощью магниевого порошка РјРѕР¶РЅРѕ добиться уменьшения веса конструкций, увеличения прочности Рё улучшения устойчивости Рє РєРѕСЂСЂРѕР·РёРё. РќРµ упустите возможность купить Порошок магниевый G-Z55Zr – AFNOR NF A65-717 Рё улучшить СЃРІРѕРё проекты! РџСЂРѕРґСѓРєС‚ доступен РїРѕ конкурентоспособной цене.
РедМетСплав предлагает обширный выбор отборных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица продукции подтверждена требуемыми документами, подтверждающими их происхождение. Дружелюбная помощь – то, чем мы гордимся – мы на связи, чтобы ответить на ваши вопросы по мере того как предоставлять решения под требования вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в множестве наших преимуществ
Наша продукция:
РўСЂСѓР±Р° магниевая M19980 – UNS Пруток магниевый M19980 – UNS является идеальным решением для различных промышленных применений. Ртот легкий Рё высокий РїРѕ прочности материал пользуется популярностью РІ аэрокосмической Рё автомобильной отраслях. Его отличные механические свойства Рё коррозионная стойкость делают его незаменимым РІ условиях высокой нагрузки. Если Р’С‹ хотите улучшить качество вашего продукта, то вам стоит рассмотреть возможность купить Пруток магниевый M19980 – UNS. Благодаря своей надежности, этот пруток обеспечит долговечность Рё стабильность ваших изделий. Заказывайте его СѓР¶Рµ сегодня, чтобы оценить РІСЃРµ его преимущества!
my blog [url=https://surfwallet.io/]surf wallet[/url]
Fun Sun: ваш идеальный партнер для Турции, все для вас.
Туроператор Sun Fun туры в Турции из Москвы [url=bluebirdtravel.ru]bluebirdtravel.ru[/url] .
I think you are thinking specifically about Ramenbet Casino sports betting or perhaps you want to find more about Casino Ramenbet mirror Казино Ramenbet официальный сайт
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наша продукция:
Металлическая мишень для распыления железа
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – наилучшее решение для вашего бизнеса.
Наша продукция:
Пруток из магнитно-мягких сплавов 24 мм 34НКМ ГОСТ 10160-75 Пруток из магнитно-мягких сплавов обладает высокой магнитной проницаемостью, обеспечивающей эффективное преобразование электрической энергии с минимальными потерями. Получите долговечное и надежное решение для ваших технических задач с применением этого уникального материала.
https://mapletopcasinos.online/
https://semantische-richtlijnen.wiki/wiki/Rolex_28V
РедМетСплав предлагает обширный выбор качественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица продукции подтверждена требуемыми документами, подтверждающими их соответствие стандартам. Опытная поддержка – наша визитная карточка – мы на связи, чтобы ответить на ваши вопросы и предоставлять решения под требования вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
поставляемая продукция:
Рзделия РёР· магния AM100A – ASTM B93 РџРѕРєРѕРІРєР° магниевая AM100A – ASTM B93 представляет СЃРѕР±РѕР№ высококачественный легированный магний, который обладает отличными механическими свойствами Рё РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью. Ртот РїСЂРѕРґСѓРєС‚ идеален для различных промышленных применений, включая аэрокосмическую Рё автомобильную отрасли. Прочный Рё легкий, РѕРЅ обеспечивает надежность Рё долговечность изделий. РќРµ упустите возможность купить РџРѕРєРѕРІРєР° магниевая AM100A – ASTM B93, чтобы улучшить качество вашей продукции. Ртот материал соответствует всем современным стандартам, что делает его оптимальным выбором для производителей.
В рамках детоксикации применяются капельничные инфузии, содержащие необходимые электролиты, витамины и современные препараты, позволяющие эффективно очистить кровь, а постоянный и тщательный контроль жизненных показателей пациента обеспечивает максимальную безопасность проводимой процедуры и позволяет оперативно корректировать дозировку лекарственных средств по мере необходимости, учитывая индивидуальные особенности организма и реакцию на терапию.
Получить дополнительную информацию – http://vyvod-iz-zapoya-novokuznetsk66.ru/narkologiya-vyvod-iz-zapoya-novokuzneczk/
https://casiopedia.info/wiki/Rolex_93O
### The Ultimate Guide to Services Cleaning: Why It Matters and How to Choose the Best
In today’s fast-paced world, maintaining a clean and organized space is more important than ever. Whether it’s your home, office, or commercial property, *services cleaning* offers a practical solution to keep your environment spotless without the hassle. This article explores the benefits of professional cleaning services, what to look for when hiring a team, and why investing in these services is a smart choice for both individuals and businesses.
#### What Are Services Cleaning?
*Services cleaning* refers to professional cleaning solutions provided by trained experts who handle everything from routine tidying to deep sanitation. These services can be tailored to residential spaces, workplaces, or specialized facilities like gyms, restaurants, or medical offices. Unlike DIY cleaning, professional services bring expertise, advanced tools, and eco-friendly products to ensure a thorough and efficient job.
#### The Benefits of Professional Services Cleaning
Hiring a *services cleaning* provider comes with a range of advantages that go beyond just a shiny floor or dust-free shelves. Here’s why they’re worth considering:
1. **Time-Saving Convenience**
Life is busy, and cleaning often falls to the bottom of the priority list. Professional cleaning services free up your schedule, letting you focus on work, family, or relaxation while experts handle the dirt and grime.
2. **Healthier Living Spaces**
Dust, allergens, and bacteria can build up over time, affecting air quality and health. *Services cleaning* teams use specialized techniques and products to eliminate these hazards, creating a safer environment for you and your loved ones.
3. **Enhanced Productivity for Businesses**
A clean workspace isn’t just aesthetically pleasing—it boosts employee morale and efficiency. Regular *services cleaning* for offices ensures a professional atmosphere that leaves a lasting impression on clients and staff alike.
4. **Customized Solutions**
From carpet cleaning to window washing, professional services offer tailored packages to meet your specific needs. Whether it’s a one-time deep clean or ongoing maintenance, you get exactly what your space requires.
#### How to Choose the Right Services Cleaning Provider
Not all cleaning services are created equal. To ensure you’re getting the best value for your money, here are some key factors to consider when selecting a *services cleaning* company:
– **Reputation and Reviews**
Look for providers with positive feedback from past clients. Online reviews and testimonials can give you insight into their reliability and quality of work.
– **Experience and Expertise**
A team with years of experience in *services cleaning* will know how to tackle tough stains, delicate surfaces, and industry-specific challenges (e.g., sanitizing a healthcare facility).
– **Eco-Friendly Options**
If sustainability matters to you, opt for a service that uses green cleaning products and practices. This not only benefits the planet but also reduces exposure to harsh chemicals.
– **Flexible Scheduling**
The best *services cleaning* companies work around your timetable, offering evening, weekend, or emergency cleaning options to minimize disruption.
– **Transparent Pricing**
Avoid surprises by choosing a provider with clear, upfront costs. Ask for a detailed quote that outlines what’s included in the service.
#### When Should You Invest in Services Cleaning?
There’s no one-size-fits-all answer to when you need professional *services cleaning*. However, here are some common scenarios where it makes sense:
– **Moving In or Out**: A deep clean ensures your new home is fresh or your old space is spotless for the next occupant.
– **Post-Renovation**: Construction dust and debris require heavy-duty cleaning that’s best left to the pros.
– **Seasonal Refresh**: Spring cleaning or pre-holiday tidying can benefit from expert help.
– **Business Upkeep**: Regular cleaning keeps commercial spaces presentable and hygienic year-round.
#### The Future of Services Cleaning
The *services cleaning* industry is evolving with technology and changing consumer needs. Robotic vacuums, UV sanitation tools, and smart scheduling apps are making professional cleaning more efficient and accessible. As demand grows, companies are also focusing on sustainability, offering biodegradable products and energy-efficient methods to appeal to eco-conscious clients.
#### Final Thoughts
Investing in *services cleaning* is more than just outsourcing a chore—it’s a step toward a cleaner, healthier, and more enjoyable space. Whether you’re a homeowner looking to reclaim your weekends or a business owner aiming to impress clients, professional cleaning services deliver unmatched results. Take the time to research and choose a reputable provider, and you’ll see why *services cleaning* is a game-changer for modern living. Ready to transform your space? Start exploring your options today!
https://computing-ethics.ic.gatech.edu/index.php?title=User:AlisonRoche576
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наши товары:
Гранулы высокочистого ниобия
have a peek here [url=https://jaxxblog.io]jaxx liberty login[/url]
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
Наши товары:
Медный тройник РїРѕРґ пайку стандартный 8С…6С…0.6 РјРј 6.8С…8.8 РјРј мягкая пайка Рњ2 ГОСТ 32590-2013 Купить качественные медные тройники РїРѕРґ пайку для надежных Рё долговечных соединений РІ трубопроводных системах. Разнообразие размеров Рё конфигураций, высокая теплопроводность Рё устойчивость Рє РєРѕСЂСЂРѕР·РёРё. Простая установка Рё надежность соединения. Рдеальное решение для различных проектов. Редметсплав.СЂС„
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наши товары:
Плоская мишень AlSi для распыления
[url=https://trial-tour.ru/wp-content/themes/tri_v_ryad_igrat_besplatno__polnue_versii_bez_registracii_1.html]https://trial-tour.ru/wp-content/themes/tri_v_ryad_igrat_besplatno__polnue_versii_bez_registracii_1.html[/url] детская игра в догонялки 8 букв
РедМетСплав предлагает обширный выбор высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица товара подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Опытная поддержка – наш стандарт – мы на связи, чтобы ответить на ваши вопросы и находить ответы под специфику вашего бизнеса.
Доверьте вашу потребность в редких металлах специалистам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наша продукция:
Полоса висмутовая A94013 – UNS Полоса висмутовая A94013 – UNS является отличным выбором для различных научных Рё технологических применений. Ртот материал выделяется высокой плотностью Рё отличной РєРѕСЂСЂРѕР·РёР№РЅРѕР№ стойкостью. Полоса РїРѕРґС…РѕРґРёС‚ для использования РІ электронной промышленности Рё медицинском оборудовании, РіРґРµ требуются надежные решения. Если РІС‹ ищете качественный Рё востребованный материал, то РЅРµ упустите возможность купить Полоса висмутовая A94013 – UNS. РћРЅР° гарантирует высокую производительность Рё долговечность, что делает её незаменимой РІ вашем проекте.
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с высоким уровнем сервиса.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
поставляемая продукция:
РџСЂСѓР¶РёРЅР° РёР· драгоценных металлов платиновая 650С…4С…0.3 РјРј РџР»Р95-5 РўРЈ Выберите идеальные РїСЂСѓР¶РёРЅС‹ РёР· золота, серебра или платины, чтобы дополнить ваше украшение. Найдите РїСЂСѓР¶РёРЅС‹ СЃ изысканным дизайном Рё прочностью. РћРЅРё предлагают широкий выбор для того, чтобы подчеркнуть уникальность вашего украшения.
Назван простой напиток, который спасает от повышения сахара в крови https://x.com/Fariz418740/status/1904006505147629966
Портал настроим-яндекс-директ.рф предоставляет квалифицированные услуги. Мы располагаем глубочайшими знаниями платформы Яндекс Директ. Учитываем особенности вашего бизнеса и предлагаем наилучшую стратегию. Настроены на долгосрочные взаимоотношения. Ищете стоимость настройки яндекс директ? Xn—–6kcrbecsfrepkel5alfjkq8y.xn--p1ai – тут отзывы клиентов представлены, посмотрите их сейчас. Воспользуйтесь преимуществами с нашей командой ведения контекстной рекламы в Яндекс Директ. Поможем с максимальной эффективностью достичь ваших бизнес-целей!
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние успехи.
Наша продукция:
Ультрачистые гранулы германия
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
Наша продукция:
Кольцо РёР· драгоценных металлов палладиевое 150С…10С…5 РјРј ПДСР-40 РўРЈ РЁРёСЂРѕРєРёР№ выбор высококачественных кольц РёР· драгоценных металлов. Рлегантные украшения, созданные талантливыми мастерами. Уникальность Рё изысканность РІ каждой детали. Возможность заказа персонального кольца РїРѕ вашим пожеланиям. Подчеркните СЃРІРѕСЋ индивидуальность СЃ нашими кольцами.
Если нужен [url=https://eco-sistem.com/product/]инсинератор для сжигания[/url], не рискуй с кустарными установками. ЭКОСИСТЕМЫ производит мощные модели, готовые к любой загрузке. Сжигает отходы полностью, соответствует ГОСТ и СанПиН. Работает — как надо. Купил, поставил, забыл про проблему.
important source [url=https://surfwallet.io]zkLogin[/url]
РедМетСплав предлагает внушительный каталог отборных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы обеспечиваем оперативное исполнение вашего заказа.
Каждая единица изделия подтверждена требуемыми документами, подтверждающими их качество. Превосходное обслуживание – наш стандарт – мы на связи, чтобы разрешать ваши вопросы и адаптировать решения под особенности вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
Наша продукция:
РљСЂСѓРі магниевый M1C – ASTM B275 Фольга магниевая M1C – ASTM B275 является универсальным материалом, обладающим отличными антикоррозийными свойствами Рё высокой прочностью. РћРЅР° идеально РїРѕРґС…РѕРґРёС‚ для применения РІ авиастроении, автомобилестроении Рё строительстве. Высокая чистота магния обеспечивает отличную электропроводность, что делает эту фольгу отличным выбором для электроники. Если вам РЅСѓР¶РЅР° надежная Рё качественная фольга, то РІС‹ можете купить Фольга магниевая M1C – ASTM B275 РїСЂСЏРјРѕ сейчас. РњС‹ предлагаем только проверенные материалы, что гарантирует долгий СЃСЂРѕРє службы изделий. Обратите внимание РЅР° технические характеристики Рё убедитесь РІ высоком качестве нашего продукта.
В Баку найдена одна из трех пропавших без вести несовершеннолетних подруг https://x.com/Fariz418740/status/1904141438733979773
[url=http://spbsseu.ru/page/pages/mir_onlayn_igr_dlya_malchikov__razvlechenie_i_razvitie.html]http://spbsseu.ru/page/pages/mir_onlayn_igr_dlya_malchikov__razvlechenie_i_razvitie.html[/url] игры для детей 4 5 лет онлайн играть
iPhone 16 256 apple iphone max
мтс подключение екатеринбург
https://www.locumsanesthesia.com/employer/24inetrnet-domashnij-ekb-2/
мтс тарифы на интернет
Starda Casino is a modern online gaming portal offering an exciting world of gambling entertainment. Anyone looking for quality slots, card games and sports betting will find everything they need for entertainment and, perhaps, winning Казино Starda официальный сайт
he has a good point [url=https://surfwallet.io]surf wallet[/url]
вилочный электропогрузчик 1000 кг
водитель электропогрузчика обучение
Сайт torentino.org предлагает вам скачать игры через торрент. Предлагаем целый ряд конкурентных преимуществ. Наши игры регулярно обновляются. Комфортная система поиска дает возможность быстро найти то, что нужно. Насладитесь возможностями новейшей индустрии развлечений. Ищете сталкер игры скачать? Torentino.org – здесь представлены разные игры, которые помогут скоротать время. Мы их распределили по множеству категорий, чтобы вам комфортно было скачать и найти игру. Любой файл на наличие вирусов обязательно проверяется. Быстрых вам загрузок!
На сайте https://kometagaming.site/ мы собрали рейтинг официальных букмекерских контор с лицензией, которые позволяют легально делать ставки на спорт и где будет гарантирована честная игра и своевременные выплаты. Мы решили этот вопрос и собрали лучшие компании в топ букмекерских контор. Лучшие официальные букмекерские конторы для ставок на спорт – это полезная информация для тех, кто хочет начать играть, а также получать различные бонусы и легкий вывод денег.
[url=http://peling.ru/wp-content/pages/mir_priklucheniy__ogon_i_voda___igra_s_ogonkom.html]http://peling.ru/wp-content/pages/mir_priklucheniy__ogon_i_voda___igra_s_ogonkom.html[/url] 3 игра в игре в кальмара
Ищете надежных мастеров и бригаду без посредников? Посетите сайт https://poisk-pro.ru/ – это бесплатный подбор надежных исполнителей в сфере ремонта, строительства и других видов услуг от частных лиц и организаций. Более 30000 исполнителей в сфере строительства и ремонта, дизайна, бытовых услуг, ремонта техники и прочих услуг. Подробнее на сайте.
вилочный погрузчик doosan
турбина jcb 4cx
Cat Casino — это отличная платформа для любителей азартных игр, которая предлагает множество бонусов и акций. Промокоды Cat Casino позволяют увеличить свои шансы на выигрыш и получить дополнительные преимущества промокод на cat casino бездепозитный бонус
на этом сайте https://maps.google.com.ua/url?q=https://letundra.com/es/news/?taglist=aeropuerto-del-cibao-sti,republica-dominicana,santiago
На сайте http://umk-trade.ru изучите новости автомира, ознакомьтесь с тест-драйвами автомобилей различных марок, чтобы принять правильное решение о покупке. Вы узнаете обо всех технических характеристиках, особенностях каждой модели. Есть тест-драйв автозапчастей Hordano, шин и дисков, которые крайне важны в эксплуатации автомобиля. Кроме того, есть информация по поводу того, стоит ли брать Мерседес в аренду, какие преимущества вы получите от этого. Любопытные, увлекательные статьи появляются регулярно.
высота подъема вилочного погрузчика
работа вилочный погрузчик вакансии свежие
Спасибо за красивые пионы! Аромат волшебный.
букет невесты томск
Ищете купить лепной декор в мск? Piterra.ru/catalog/lepnina/ можно для разного стиля интерьера. Современная лепнина органично вписывается в пространство, оживляя стены, потолки, скрывая провода и электрические розетки, а также придавая выразительности помещению с помощью различных оттенков краски.
On our site a little more about Sukaaa Casino bonuses, as well as information about Sykaaa Casino official site Сукааа Казино официальный сайт
Новруз может быть объявлен официальным праздником в Турции https://x.com/Fariz418740/status/1904268540363915402
На сайте https://strah.shop/ почитайте информацию на тему финансов, страховки и многого другого. Вы узнаете о том, как простимулировать семьи к тому, чтобы они повысили рождаемость и захотели родить второго, третьего ребенка. Есть советы по поводу того, как защитить себя от карманников. Есть публикации на тему того, как правильно и грамотно управлять своими долгами. Здесь постоянно появляются свежие записи, которые будут интересны всем. На сайте также имеются статьи на другие темы, интересные россиянам.
труба профильная гост proftruba-moscow.ru с сертификатами соответствия. гарантируем точность геометрических параметров.
Пионы — это всегда праздничное настроение!
букет пионов купить
Ищете обои на стену купить? piterra.ru/catalog/oboi, предоставляет большой ассортимент обоев в МСК и СПб. В нашем каталоге вы отыщите обои на кухню, обои под покраску, обои на стену. Вы можете купить обои в интернет-магазине или выбрать в салонах по адресам. У нас доступные цены на обои, удобная доставка и большой ассортимент. Ищете магазин обоев в СПб или МСК? Ознакомьтесь с нашим каталогом, сравните цены и подберите для вашего интерьера идеальные обои!
сервисы для покупки аккаунтов биржа аккаунтов соц сетей
маркетплейс цифровых аккаунтов биржа покупки аккаунтов
Elara Finance is transforming decentralized lending by offering secure, transparent, and flexible crypto loan solutions. Built on blockchain technology, Elara Finance enables users to borrow and lend digital assets seamlessly without intermediaries. With low-interest rates, automated smart contracts, and a permissionless DeFi environment, Elara Finance is making crypto lending accessible and profitable for investors worldwide. https://elara.ink
Ищете где купить бонсай? Зайдите на сайт https://bonsay.ru/ – мы уже 15 лет занимаемся японскими карликовыми деревьями бонсай с быстрой доставкой. Посмотрите наш каталог – он самый большой на рынке, а в наших магазинах в Москве и Санкт-Петербурге выбор еще больше. Купить бонсай в подарок или для себя, настоящее ухоженное дерево! Подробнее на сайте.
Online platforms have made it simpler than ever to purchase digital products and online accounts. The majority of buyers provide feedback on their experiences, providing first-hand details on the reliability of sellers, security concerns, and satisfaction https://fgmedia.readme.io/reference/legal-aspects-of-buying-and-selling-online-accounts
Cytonic is revolutionizing blockchain security with advanced cybersecurity solutions tailored for Web3 applications. By integrating decentralized encryption, AI-powered threat detection, and smart contract auditing, Cytonic ensures maximum protection against cyber threats. Whether you’re securing DeFi protocols, NFTs, or enterprise blockchain systems, Cytonic’s cutting-edge security technology provides the highest level of data integrity and protection. https://cytonic.cc
DEQ Finance is revolutionizing decentralized trading by offering a seamless, secure, and efficient crypto exchange experience. Built with cutting-edge blockchain technology, DEQ Finance provides traders with fast transaction speeds, deep liquidity, and a transparent trading environment. Whether you’re a beginner or a professional trader, DEQ Finance delivers high-performance DeFi solutions tailored to modern trading needs. https://deq.li
Топ букмекерских контор для ставок на сайте https://pkrdomgaming.site/ – это лучшие официальные букмекерские конторы для ставок на спорт. Гарантирована честная игра и своевременные выплаты. Мы решили этот вопрос и собрали лучшие компании в топ букмекерских контор. Лицензия, отличные коэффициенты, простота ввода и вывода денег.
Non Gamstop casino UK sites load so fast—perfect for quick play!
non gamstop casino no deposit
С t.me/mvavada вам точно не будет скучно. Официальный канал проекта Вавада предлагает атмосферу настоящего казино почувствовать. В VAVADA совершенно любой игру по душе найдет. Скорее к нам присоединяйтесь. Участвуйте в акциях и получайте шанс на дополнительные выигрыши. Удачу испытайте! https://t.me/mvavada – здесь всегда есть что-то интересное. Следите за обновлениями канала. В Вавада вас слоты с захватывающим геймплеем и неповторимым дизайном ждут. Начните играть уже сейчас. С VAVADA побеждайте. Меняйте свою жизнь к лучшему!
Exponent Finance is redefining DeFi lending by providing secure, transparent, and high-yield investment solutions. Through smart contract-powered lending pools, Exponent Finance DeFi platform allows users to borrow and lend crypto assets with optimal efficiency and minimal risk. Whether you’re looking to earn passive income through staking or access instant liquidity, Exponent Finance offers a decentralized, non-custodial, and user-friendly solution to meet all your financial goals in the crypto ecosystem. https://exponent.ink
dark markets darknet links
Flaunch is the leading blockchain gaming launchpad, designed to help game developers and investors thrive in the Web3 gaming ecosystem. By offering secure token launches, NFT integrations, and decentralized crowdfunding, Flaunch enables game creators to fund, develop, and scale their projects with full transparency and community-driven support. Whether you’re a developer or an investor, Flaunch provides the tools to connect and grow in the blockchain gaming space. https://flaunch.tech
Комплексная УЗИ-диагностика всех органов и тканей в» Санкт-Петербургском центре Узи –диагностике» на оборудовнии экспертного класса Хороший узист
На сайте https://advelma.ru изучите огромное количество объявлений на самую разную тематику, включая автотранспорт, растения и животные, мобильные телефоны, мебель, интерьер и многое другое. Здесь также вы сможете и познакомиться с мужчиной либо женщиной. Всего на сайте представлено почти 150 рубрик. Если и вам нужно что-то продать, то выложите объявление на этом портале. Регулярно здесь добавляются новые, интересные предложения. Для того чтобы воспользоваться полным функционалом, нужно зарегистрироваться.
На сайте https://xn--80abeehcvkgdf2bbeq5an.xn--p1ai/ почитайте всю полезную и актуальную информацию о популярном онлайн-заведении Casino Kent. Вас ожидает сервис высокого уровня, огромное количество динамичных развлечений, щедрые бонусы, приятная атмосфера. И самое важное, что ресурс обязательно понравится как новичкам, которые только осваивают подобные заведения, так и профессионалам, которые находятся в поисках идеального места. Оно радует безупречным уровнем сервиса, можно задать вопросы, когда захотите.
Рефинансирование микрозаймов становится важным инструментом для тех, у кого имеется плохая кредитная история. Этот процесс позволяет объединить существующие долги в один займ с более выгодными условиями money x casino зеркало
DEQ Finance is revolutionizing decentralized trading by offering a seamless, secure, and efficient crypto exchange experience. Built with cutting-edge blockchain technology, DEQ Finance provides traders with fast transaction speeds, deep liquidity, and a transparent trading environment. Whether you’re a beginner or a professional trader, DEQ Finance delivers high-performance DeFi solutions tailored to modern trading needs. https://deq.li
сервисы для покупки аккаунтов биржа для продажи аккаунтов
[url=https://www.snabco.ru/fw/inc/geometry_dash__pogruzghenie_v_mir_geometricheskih_priklucheniy.html]https://www.snabco.ru/fw/inc/geometry_dash__pogruzghenie_v_mir_geometricheskih_priklucheniy.html[/url] игра карты паук играть бесплатно онлайн
Non Gamstop casinos no deposit deals are my secret weapon—love them!
non gamstop casinos reviews
Магазин «Adept Apple» продает оригинальные качественные товары с гарантией. Мы предоставляем приличный выбор гаджетов, а также аксессуаров Apple. Имеем значительный опыт и стали достойным партнером для тысяч клиентов. Стараемся сделать ваше обслуживание максимально комфортным и приятным. Ищете dyson? Adeptapple.ru – тут для себя можете что-то найти. Делаем технику Apple доступной для любого. С радостью поможем выбрать аксессуар или устройство, которые подойдут идеально, только вам. Для нас важно доставить клиентам удовольствие от покупки!
На сайте https://xn—-7sbbstdmdlcdhy.xn--p1ai/ вы сможете ознакомиться со всей необходимой информацией, касающейся майнинга и криптовалюты. Этот ресурс является свободным информационным и предлагает ознакомиться со всеми полезными рекомендациями. Только на этом сайте представлены самые точные и любопытные сведения, касающиеся цифровой валюты, криптовалюты и всего, что с ней связано. Представленная информация будет полезна и новеньким. Вас обязательно заинтересует и крипто библиотека, в которой есть много всего полезного.
оценка для сбербанка спб https://ocenka-zagorod.ru
Just played at a non Gamstop casino—slots are next-level good!
casinos no gamstop
типография спб официальный сайт услуги типографии цена
малая типография https://mir-poligrafiya.ru
На сайте https://exkavatornews.ru/ почитайте содержательные и полезные материалы на тему лесозаготовительной техники. Здесь вы найдете ответ на вопрос, стоит ли приобретать китайскую технику, какое оборудование считается максимально эффективным. Есть информация и о дорожно-строительной технике и о том, как ей лучше пользоваться. Ознакомьтесь с инновационными технологиями в сфере дорожного строительства. Изучите мнения экспертов на данную тему. Регулярно здесь появляются новые, любопытные материалы, которые будут полезны.
Компания YIT стремительно развивается и предлагает вам амортизаторы, которые отлично зарекомендовали себя. Гарантируем максимально выгодные условия сотрудничества. По телефону предоставляем квалифицированные консультации. Доставку по всей РФ выполняем. Ваше время сэкономим. Ищете стойка для квадроцикла стелс гепард? Yit-shocks.com – тут продукция компании YIT представлена. Ознакомиться с каталогом можете в любое удобное время. У вас есть возможность в офисе магазина оплатить заказ. Свяжитесь с менеджером для совершения безналичного расчета. Мы всегда вам рады!
хотскинс казино
либет казино
Best non Gamstop casino I’ve tried has great payouts—cool!
legit non gamstop casinos
Быстро, красиво, надёжно! Лучший сервис.
гипсофилы цена букета
[url=https://mfo-zaim.com/]займ на карту с просрочками и плохой кредитной историей[/url]
заказать грузчиков правильные грузчики
грузчики на дом грузчик услуга
грузчики услуги toguchin.standart-express.ru
Чат-бот на базе ИИ ChatGPT – профессиональный помощник, который может понимать запросы, поддерживать диалог и предоставлять индивидуальные решения. Цепляющий контент создавайте, продажи оптимизируйте и в 10 раз оперативнее задачи решайте. На самом главном сосредоточьтесь. Ищете gpt чат нейросеть? Ai-gptbot.ru – портал, где отзывы представлены, посмотрите их. Здесь рассказываем, кому нужен чат GPT. Все удобно и быстро работает. Цены удивляют приятно своей гибкостью и демократичностью. Не упускайте шанс попробовать AI-GPTbot прямо сегодня!
Money Икс Игральный клуб — это эксклюзивная платформа, которая дарит клиентам потрясающие варианты для выигрыша money x казино casino money x
[url=https://mfo-zaim.com/]займы онлайн малоизвестные новые[/url]
Ikostum предлагает приобрести по недорогой стоимости мужской костюм. В нашем интернет магазине регулярно распродажи и акции проводятся. У нас найдется костюм на любой вкус. Поможем вам с фасоном, тканью и цветом определиться, предложим чехол и галстук подходящий, а также проконсультируем о последних тенденциях. https://ikostum.com/ – здесь можете в любое время ознакомиться с условиями доставки. Мы сотрудничаем с ведущими белорусскими и российскими производителями мужской одежды. Постоянно обновляем ассортимент. Гарантируем вам высокое качество продукции.
Ищете форекс брокер для скальпинга? Time-forex.com/brokery-dly-skalpinga тут вы отменные для скальпинга брокеры отыщите. Форекс брокер для скальпинга это самая прибыльная стратегия трейдинга в данное время считается краткосрочный трейдинг, она предполагает открытие большого количества сделок в течении одной сессии. Посмотрите весь список надежных для скальпинга брокеров, которых мы внимательно отобрали и информацию структурировали.
GUD Tech is at the forefront of blockchain innovation, providing secure, scalable, and efficient decentralized technology solutions. Whether you’re looking for blockchain infrastructure, smart contract development, or enterprise-grade decentralized applications, GUD Tech offers cutting-edge solutions to enhance security and efficiency. Designed for businesses and developers, GUD Tech ensures seamless integration of blockchain technology into real-world applications. https://gudchain.net
Non Gamstop UK casino has awesome slots—totally hooked!
non gamstop mobile casino pay with phone bill
Noon Capital is revolutionizing real estate crowdfunding by offering secure, decentralized, and high-yield investment opportunities. Through blockchain-powered property financing, Noon Capital investment platform allows investors to participate in fractional real estate ownership, earn passive income, and diversify their portfolios with full transparency and security. Whether you’re an institutional investor or an individual seeking real estate-backed DeFi solutions, Noon Capital provides a seamless and profitable investment experience. https://noon.ad
кредит под птс спецтехники
avtolombard-11.ru/ekb.html
кредит под залог птс екатеринбург
Бэтэрэкс – компания, которая предлагает юридические, финансовые и консалтинговые услуги. Мы окажем вам помощь в снижении затрат на ведение бизнеса с Китаем. Принимаем на доставку заказы от компаний и частных лиц. Сопроводим на переговорах и в деловых поездках. https://mybeterex.com – тут можете форму заполнить, и мы в ближайшее время с вами свяжемся. Найдем и предложим на товар в Китае лучшую оптовую цену. С радостью поможем открыть производство. О выпуске продукции договоримся и качество проконтролируем. Обращайтесь к нам в любое время!
Kyros Finance is redefining the DeFi investment landscape by offering secure, scalable, and high-yield crypto solutions. With a focus on decentralized financial tools, Kyros Finance provides users with staking, lending, and automated yield farming strategies to maximize returns. Whether you’re a retail investor or an institutional participant, Kyros Finance ensures efficient, transparent, and secure access to the world of decentralized finance. https://kyros.ink
ТТК — транспортная компания, специализирующаяся на перевозках автомобилей на автовозах по всей России. Наша компания имеет опыт перевозок автовозами более 12 лет. Свыше 40 единиц техники позволяют осуществлять регулярные рейсы по всей России. У нас можно заказать автовозы по России для транспортировки автомобиля любого типа: седана, универсала, кроссовера, внедорожника и даже автомобиля в аварийном состоянии. Качественный сервис по доступным ценам. Обеспечиваем высокое качество и безопасность на каждом этапе перевозки. Широкая география перевозок позволяет обеспечить перевозку машин в любую точку страны: Москва, Санкт-Петербург, Владивосток, Екатеринбург, Иркутск, Казань, Кемерово, Краснодар, Красноярск, Новосибирск, Омск, Пермь, Ростов-на-Дону, Самара, Симферополь, Сочи, Ставрополь, Сургут, Тольятти, Томск, Тюмень, Улан-Удэ, Уфа, Хабаровск, Челябинск, Чита и в другие города России. Практически во всех городах России мы имеем специальные охраняемые стоянки для авто. Работаем 24/7. Сайт нашей компании ТТК: https://avtovoz7.ru/
Non Gamstop casino no deposit bonus was a pleasant surprise—cool!
no deposit bonus casino non gamstop
Единственный знак зодиака, который является магнитом для несчастий и проблем https://x.com/Fariz418740/status/1904737515858174078
How to exchange Monero in the cryptocurrency exchanger quickex are Buy or sell XMR? In this https://www.youtube.com/watch?v=zXDvoUnvK8Y detailed guide, we will show you how to buy Monero (XMR) on the quickex platform in 5 easy steps. You will learn how to use a reliable service without registration and verification, getting access to the best rates from leading liquidity providers.
HyperDrive is transforming the way data is stored and managed through decentralized storage and blockchain hosting solutions. Built for security, scalability, and efficiency, HyperDrive enables businesses, developers, and blockchain projects to store and distribute data without relying on centralized servers. By leveraging Web3 technology and distributed networks, HyperDrive ensures reliable, censorship-resistant, and high-performance storage solutions for the digital era. https://hyperdrive.ink
Non Gamstop casinos UK are perfect for late-night sessions—great!
safest non gamstop uk casinos
Ищете производителя качественных и современных мангалов? Посетите https://steelonfire.ru/ – ознакомьтесь с нашим ассортиментом. Мы производим: мангалы, костровые чаши, печи для сжигания мусора, буржуйки, учаги под казан, коптильни, чаны для бани и другие товары. Посмотрите каталог, воспользуйтесь доставкой в Москве, Санкт-Петербурге и по всей России или заберите самовывозом. Качественная продукция по отличным ценам!
Ирвин Казино – это место, где азартные игры становятся действительно увлекательными и безопасными. Под брендом Irwin Casino скрывается надежная и проверенная платформа для тех, кто хочет окунуться в мир азарта и развлечений Казино Ирвин
Капитал Икс Игральный клуб — это эксклюзивная платформа, которая обеспечивает клиентам фантастические возможности для успеха мани х казино money x casino вход
биржа для продажи аккаунтов купить аккаунт дешево
Продажа путёвок https://camp-centr.com/camps/type/yazykovoy.html. Спортивные, творческие и тематические смены. Весёлый и безопасный отдых под присмотром педагогов и аниматоров. Бронируйте онлайн!
Прокат спецтехники в Омске Аренда и услуги спецтехники в Омске – Мы предоставляем услуги по аренде и эксплуатации спецтехники для различных сфер деятельности, включая строительство, дорожные работы и транспортировку грузов. Наши услуги охватывают аренду автокранов, экскаваторов, самосвалов и других машин для больших и малых предприятий, а также для частных клиентов. Спецтехника для вашего бизнеса с гарантией надежности и эффективности — это наш приоритет.
деньги под залог автомобиля
avtolombard-11.ru/kazan.html
кредит под птс автомобиля
стоимость печати визиток визитки печать спб дешево
На сайте https://prokat.car-trip.ru/ вы сможете выбрать автомобиль для того, чтобы воспользоваться прокатом. Только сейчас действует скидка 10% на определенные автомобили. Есть те машины, которые относятся к классу Комфорт, Бизнес, Стандарт. В автопарке имеются и автодома, кабриолеты. Высококлассные менеджеры всегда находятся на связи, чтобы вы задали им любой вопрос. Отсутствуют скрытые платежи, нет залога, ограничения относительно пробега. Все клиенты получают право воспользоваться дисконтной картой.
Установка подогревателей Webasto для Mercedes Ремонт и обслуживание автомобилей Mercedes-Benz – Мы уже много лет предоставляем профессиональные услуги по ремонту и техническому обслуживанию автомобилей Mercedes-Benz как для крупных автопарков, так и для владельцев автомобилей малого и среднего бизнеса. Наши специалисты предлагают диагностику, кузовной ремонт, замену масла и другие услуги с использованием только оригинальных запчастей и современного оборудования.
Нужен надежное устройство для обогрева и проветривания — вы на верном пути. У нас вы найдете [url=https://termik18.ru/services/tehnicheskoe-obsluzhivanie-kotlov]конструкторские чертежи[/url] которая пригодится на рыбалке, в условиях холода. Компания “Термик” работает в Ижевске с 2009 года и занимается реализацией теплотехники — от мобильных устройств до мощных установок. Мы не просто продаем технику, а сопровождаем на всех этапах: поможем выбрать, произведем монтаж и проведем сервис.
Для коттеджа, загородного участка или коммерческого объекта у нас есть подходящий вариант. Вы ищите [url=https://termik18.ru/services/tehnicheskoe-obsluzhivanie-kotlov]разработка чертежа изделия[/url] — найдем решение по техническим характеристикам и цене. В ассортименте надежные производители, официальная гарантия и наш техцентр. Без перебоев, оперативно и с заботой о вашем комфорте.
На сайте https://psihologist-spb.ru/uslugi-psikhologa/semeynaya-psikhoterapiya/ почитайте интересную и полезную информацию, которая касается того, чем вам поможет семейный психолог. Этот специалист поможет разрешить конфликт, улучшить отношения между супругами и сделать так, чтобы появилось доверие в паре. Кроме того, вы узнаете и о том, в каких случаях лучше всего обращаться к семейному психологу. Он поможет избежать недопонимания, стрессов, противоречий, поможет в воспитании детей и романтических отношениях.
нью ретро казино, голд казино, изи кэш казино
займ под залог машины
avtolombard-11.ru/kemerovo.html
автоломбард под залог
голд казино
На сайте https://ai-gptbot.ru вы сможете воспользоваться всеми возможностями уникального и инновационного чата GPT, который предлагается на русском языке. При этом воспользоваться им вы сможете даже без VPN. Все ежедневные рутинные дела вы сможете доверить ИИ. У вас получится создать увлекательный, интересный контент. Теперь вы сможете решить все вопросы максимально быстро и точно. Чат позволит сосредоточиться на важных процессах. Он необходим разработчикам, менеджерам, которые раскручивают социальные сети, школьникам, студентам, которые готовятся к экзаменам.
Non-Gamstop casinos are the real deal—highly recommend!
great britain casino non gamstop
Ищете проверенное устройство для обогрева и вентиляции — вы по адресу. У нас вы найдете [url=https://termik18.ru/articles/napolnye-gazovye-kotly-dlya-otopleniya]цена напольных котлов[/url] которая пригодится и на охоте, и в зимнем походе. Компания “Термик” работает в Ижевске с 2009 года и занимается поставкой климатического оборудования — от портативных решений до мощных установок. Мы не просто реализуем оборудование, а оказываем полный спектр услуг: рекомендуем, установим и обслужим.
Для дома, садового домика или коммерческого объекта у нас есть решение на любой случай. Вы ищите [url=https://termik18.ru/services/montazh-i-pnr-sistem-otopleniya-i-kotelnyh]услуги по гибке металла[/url] — предложим вариант по нагрузке и возможностям. В ассортименте продукция от проверенных брендов, официальная гарантия и собственный сервис. Без перебоев, в короткие сроки и с ориентацией на клиента.
Looking for a top-tier film or video production company in Italy? ORBIS Production is your go-to Italian production company offering full-service video, photo and film production across Milan, Rome, Venice, Florence, Turin, Genoa, Sardinia, Naples, Cortina d’Ampezzo, Verona, Lake Como, Lake Garda, Bari, Sicily, Capri, Forte-dei-Marmi, Siena and all of Italy. Discover our production services, from corporate and commercial films to international service production – https://orbispro.it/en
Ищете ник инстаграм? Посетите сайт https://sovads.ru/ где вы найдете в широком ассортименте ники инстаграм. Мы занимаемся продажей привлекательных никнеймов и у вас будет красивый ник инстаграм или короткий ник инстаграм для удобства. Стоимость самая лучшая на рынке, а ассортимент магазина пополняется уникальными именами пользователей: словарными, короткими, красивыми и городскими никами. Короткие ники для инстаграм – преимущества очевидно!
a knockout post [url=https://jaxxblog.io]jaxx liberty login[/url]
Выполняем качественное https://energopto.ru/raboty/proektirovanie/proektirovanie-itp-tstp/ под ключ. Энергоэффективные решения для домов, офисов, промышленных объектов. Гарантия, соблюдение СНиП и точные сроки!
изготовление печати бланков печать бланков писем
печать папок а4 цветная pechat-papok.ru
кредит под птс грузового автомобиля
avtolombard-11.ru
займ залог авто
Арчибальд – академия, которая обучением груминга занимается. У вас есть возможность свои навыки совершенствовать до бесконечности на живых моделях. Преподаватели отвечают на все вопросы. Дарим видеолекции, методические материалы и учебники. Окунитесь в атмосферу, с которой расставаться не хочется. Ищете хорошие курсы груминга в москве? Grooming-dream.ru – здесь представлены реальные отзывы клиентов. Мы выпускаем отменных груминг-специалистов. Предлагаем во время обучения инструменты. Если вам интересна новая профессия – Арчибальд, вот то место, которое вам подойдет.
смотреть здесь[url=https://hyip-helper.net]hyip проекты[/url]
техосмотр такси Москва – мегаполис, где техосмотр (ТО) является обязательной процедурой для большинства транспортных средств. Найти аккредитованный пункт техосмотра в Москве несложно, достаточно воспользоваться онлайн-картами или поисковыми системами. Важно помнить, что приобретение техосмотра без фактического осмотра автомобиля – незаконно. Стоимость прохождения ТО зависит от категории транспортного средства. Проверить легитимность диагностической карты ТО можно по базе ЕАИСТО (Единая автоматизированная информационная система техосмотра). Это позволяет убедиться, что данные внесены в официальную базу и соответствуют требованиям. Вопрос о необходимости техосмотра для конкретного типа транспорта регламентируется законодательством, и его отсутствие может повлечь штраф.
Официальный сайт Natco LTD Natco Pharma Limited, широко известная как Натко фарма, зарекомендовала себя как один из лидеров индийской фармацевтической индустрии. Компания, представленная на своем официальном сайте natcopharma.co.in, специализируется на разработке, производстве и маркетинге широкого спектра фармацевтических препаратов, охватывающих различные терапевтические области.
На официальном сайте Мотор Казино любой игрок найдет широкий выбор развлечений: от классических игровых автоматов до современных видеослотов, настольных игр и живых дилеров регистрация в казино Мотор
заключить контракт на службу Процесс поступления на военную службу по контракту начинается с изъявления гражданином желания заключить соответствующий контракт. Это может быть как первичное поступление на службу, так и перезаключение контракта после окончания предыдущего. Основным документом, регулирующим данный процесс, является Федеральный закон “О воинской обязанности и военной службе”. Для заключения контракта необходимо обратиться в военный комиссариат по месту жительства или в пункт отбора на военную службу по контракту. Там гражданин получит подробную консультацию о требованиях, предъявляемых к кандидатам, и перечне необходимых документов. Важно учитывать, что к кандидатам предъявляются определенные требования по возрасту, образованию, состоянию здоровья и физической подготовке.
Use 1XBET promo code: 1X200NEW for VIP bonus up to €1950 + 150 free spins on casino and 100% up to €130 to bet on sports. Register on the 1xbet platform and get a chance to earn even more Rupees using bonus offers and special bonus code from 1xbet. Make sports bets, virtual sports or play at the casino. Join 1Xbet and claim your welcome bonus using the latest 1Xbet promo codes. Check below list of 1Xbet signup bonuses, promotions and product reviews for sportsbook, casino, poker and games sections. To claim any of the 1Xbet welcome bonuses listed in above table we recommend using the 1Xbet bonus code at registration of your account. New customers will get a €130 exclusive bonus (International users) when registering using the 1Xbet promo code listed above. 1Xbet Sportsbook section is the main place where users hang out, with over 1000 sporting events to bet each day. There are multiple choices to go for, and the betting markets, for example for soccer matches, can even pass 300 in number, and that is available for both pre-match and live betting, which is impressive and puts it right next to the big names in the industry.
печать портрета на холсте уф печать на холсте
dtf печать на кружках https://dtf-pechat-spb.ru
цифровая широкоформатная печать https://shirokoformatnaya-pechat-spb.ru
download steam desktop authenticator
steam desktop authenticator github
взять займ под залог авто
avtolombard-11.ru/nsk.html
займ под птс новосибирск
Пионы — это моя слабость! Спасибо за быструю доставку.
пионы купить в Томске
Спасибо за оперативность! Заказала с утра — к вечеру уже получила.
заказ цветов томск с доставкой
Ищете фильтры для воды, септики, смесители, насосы? Посетите https://shoph2o.ru/ где вы сможете найти и купить в интернет магазине «H2O – Территория чистой воды» – системы водоочистки любой сложности. У нас широкий ассортимент продукции по выгодным ценам и быстрой доставкой. Также у нас можно заказать услуги анализа воды, монтаж септиков, ремонт систем водоподготовки и фильтров для воды. И их сервисное обслуживание.
Best non Gamstop casino I’ve played at is a gem—cool!
online casino not on gamstop free spins no deposit
Use 1XBET promo code: 1X200NEW for VIP bonus up to €1950 + 150 free spins on casino and 100% up to €130 to bet on sports. Register on the 1xbet platform and get a chance to earn even more Rupees using bonus offers and special bonus code from 1xbet. Make sports bets, virtual sports or play at the casino. Join 1Xbet and claim your welcome bonus using the latest 1Xbet promo codes. Check below list of 1Xbet signup bonuses, promotions and product reviews for sportsbook, casino, poker and games sections. To claim any of the 1Xbet welcome bonuses listed in above table we recommend using the 1Xbet bonus code at registration of your account. New customers will get a €130 exclusive bonus (International users) when registering using the 1Xbet promo code listed above. 1Xbet Sportsbook section is the main place where users hang out, with over 1000 sporting events to bet each day. There are multiple choices to go for, and the betting markets, for example for soccer matches, can even pass 300 in number, and that is available for both pre-match and live betting, which is impressive and puts it right next to the big names in the industry.
sda steam
казино В мире азартных игр онлайн казино заняли прочное место, предлагая игрокам широкий выбор развлечений и возможность испытать удачу, не выходя из дома. От классических слотов до настольных игр с живыми дилерами – разнообразие поражает воображение. Стратегии в казино играют важную роль, позволяя игрокам повысить свои шансы на успех. Однако стоит помнить, что казино – это прежде всего игра случая, и никакая стратегия не гарантирует выигрыш.
Онлайн казино: как выбрать платформу с быстрым стартом
Современные геймеры все чаще привлекаются к играм на денежных ставках через интернет. Это связано с возможностью быстро включиться в процессы и испытать удачу, не выходя из дома. Однако, чтобы избежать разочарований, важно тщательно исследовать доступные варианты.
Прежде всего, ищите заведения с очевидной репутацией. Изучайте отзывы пользователей на специализированных форумах и сайтах. Сообщества игроков часто делятся полезной информацией о своих опыте. Также обратите внимание на время работы сервиса и лицензии, которые обеспечивают легитимность его деятельности.
Следующий аспект – разнообразие игр. Выбирайте площадки, представляющие широкий спектр развлечений: от классических слотов до настольных развлечений. Обратите внимание на программное обеспечение, используемое для создания игр, так как оно влияет на качество графики и плавность игрового процесса.
Уделите внимание и бонусам. Программы лояльности и привилегии для новых пользователей могут существенно улучшить опыт. Когда вы осознаете, какие предложения существуют, это может значительно увеличить ваши шансы на успешные ставки.
Таким образом, анализируя различные аспекты, вы сможете выбрать как можно более подходящий и надежный игровой ресурс, который обеспечит вам интересный и безопасный опыт.
Критерии выбора азартного заведения
Во-вторых, разнообразие игр играет ключевую роль. Ищите заведения с широким ассортиментом, включая слоты, настольные игры и живые карточные развлечения. Проверяйте наличие игр от различных поставщиков программного обеспечения, таких как NetEnt, Microgaming или Playtech, что обеспечит высокий уровень качества.
Также стоит ознакомиться с бонусными предложениями. Чаще всего привлекательные акции включают приветственные бонусы, фриспины и программы лояльности. Важно внимательно читать условия, чтобы избежать неприятных сюрпризов.
Доступность службы поддержки является еще одним немаловажным аспектом. Убедитесь, что на платформе можно легко связаться с представителями через чат, электронную почту или телефон. Проверьте время реакции и наличие поддержки на вашем языке.
Отзывы пользователей могут дать полезную информацию о репутации заведения. Изучите мнения на форумах или специализированных сайтах, чтобы составить полное представление о платформе.
Наконец, обратите внимание на мобильную совместимость. Удобный интерфейс для использования на мобильных устройствах увеличивает шансы наслаждаться игрой в любое время и в любом месте.
Бонусы и акции
При выборе сервиса для азартных развлечений бонусы и акции имеют немалое значение. Они не только повышают интерес, но и дают возможность увеличить банкролл без дополнительных вложений. Основные типы вознаграждений включают приветственные бонусы, фриспины и программы лояльности.
Приветственные бонусы осуществляют первый вклад или предоставляют средства для начала. Обычно они составляют 100% от суммы первого пополнения, но могут достигать и больших значений. Важно учитывать требования по отыгрышу: чем ниже коэффициент, тем быстрее можно вывести выигрыш.
Фриспины, или бесплатные спины на автоматах, часто включены в приветственные пакеты или проводятся как сезонные промоакции. Количество фриспинов может варьироваться, но оптимально, когда они даны на несколько популярных игр. Читайте условия, чтобы знать, где можно использовать подарочные вращения без риска.
Программы лояльности позволяют постоянным пользователям получать дополнительные преимущества. За игру начисляются баллы, которые затем можно обменять на деньги, бонусы или другие привилегии. Проверяйте, как легко можно перейти на следующий уровень программы: участие в турнирах или выполнение специальных заданий ускорят процесс.
Обратите внимание на временные акции, такие как удвоение депозитов в праздничные дни или определенные игровые сессии. Иногда предлагаются эксклюзивные предложения, доступные только для некоторых категорий пользователей. Чтение новостных разделов сайта поможет не пропустить выгодные предложения.
Принятие бонусов – это дополнительная возможность обогатиться в процессе игры, но всегда учитывайте свои финансовые возможности и придерживайтесь ответственной игры.
https://vk.com/skachat7k
Компания Глобал ЖБИ https://global-gbi.ru/ является крупным производителем изделий из бетона и железобетона в Санкт-Петербурге. Посетите наш сайт, ознакомьтесь с широкой номенклатурой производимого товара по выгодным ценам. Мы осуществляем оперативную и быструю доставку продукции. Также на заводе ЖБИ, можно заказать производство конструкций разного типа и назначения для строительства дорог, взлетных полос, тротуаров, пешеходных зон, зданий, сооружений, внутридомовых конструкций, инженерных коммуникаций и т.д.
Единственный знак зодиака, который является магнитом для несчастий и проблем https://x.com/Fariz418740/status/1904737515858174078
Автосервис в СПб СТО Приморский предоставляет широкий спектр услуг. Мы починить неисправность в авто поможем. Гарантируем доступные цены и высочайшее качество работы. При необходимости грамотно проконсультируем. Ищете автосервис в спб? Sto812.com – здесь представлена полезная информация. На портале вы ряд основных проблем, которые возникают при покупке некачественных фильтрующих элементов увидите. Узнаете, тормозная жидкость, какими свойствами обладает. Имеем нужные инструменты и опыт. Работаем быстро. Обращайтесь!
огнезащитное покрытие для паркетных досок В мире современных интерьеров, где дерево занимает почетное место, особое внимание уделяется безопасности. Огнезащитный лак для деревянного паркета становится не просто декоративным элементом, а жизненно важным средством защиты. Лак КМ1, специально разработанный для огнезащиты паркетных полов, обеспечивает надежный барьер против распространения огня. Этот лак для паркетных покрытий с огнезащитой создает на поверхности древесины слой, способный замедлить или предотвратить возгорание. Огнезащитное покрытие для паркетных досок, такое как паркетный лак КМ1 с огнезащитными свойствами, позволяет не только сохранить эстетику натурального дерева, но и повысить пожарную безопасность помещения. Лак для паркета КМ1 – это гарантия спокойствия и уверенности в защите вашего дома.
ломбард деньги под залог авто
https://abstaffs.com/employer/avtolombard-pid-zalog-pts764/
займ залог авто взять
Доставка комплексных обедов в Алматы для ваших сотрудников или на дом на сайте https://bvd.kz/ – у нас широкий ассортимент и выбор блюд по отличным ценам! Ознакомьтесь на сайте с составами и ценами на комплексные обеды. Заключаем договоры на доставку обедов в офисы и административные учреждения, доставляем обеды в школы и детские сады, где нет собственной кухни, в магазины и прочие подобные учреждения, у сотрудников которых нет возможности пообедать в столовой. Подробнее на сайте.
подробнее [url=https://megamoriartyc09o3seyehpnpnfnv7xo.lol]мега даркнет[/url]
Четыре знака Зодиака ждет переломный момент на этих выходных https://x.com/Fariz418740/status/1905095937828978718
Онлайн казино и AR-технологии: что нас ждет
Современные реалии азартных игр в интернете имеют все предпосылки для прорыва, особенно с введением технологий дополненной реальности. Это не просто тренд, а направление, которое стремительно меняет правила игры.
Потенциал для внедрения AR в азартные игры проявляется через взаимодействие с пользователями. Эта технология позволяет создать более захватывающий опыт, где игроки могут погружаться в анимированные игровые пространства, которые выглядят так, словно находятся в реальном мире. Отталкиваясь от аналитики, ожидается, что влияние дополненной реальности на индустрию увеличится на 25% к 2025 году.
На данный момент пользователи имеют возможность испытать игры, в которых визуальные элементы накладываются на окружающую среду, что добавляет элемент физического присутствия. Это не просто развлечение, а возможность взаимодействовать с игровыми элементами в более натуральной манере, что может существенно повысить вовлеченность.
Разработчикам стоит обратить внимание на следующие аспекты: интеграция AR требует высококачественной графики и стабильного интернет-соединения, а также время на обучение пользователей новому формату игр. Инвестиции в эту область окупятся, если подойти к разработке концепций с умом и вниманием к деталям.
С каждым годом растет интерес к этой сфере, и внимание к AR-элементам несомненно станет одной из ключевых составляющих привлечения новой аудитории. Ожидается, что адаптация традиционных игр к новым технологиям станет стандартом и определит будущее азартных игр в ближайшие годы.
Интеграция AR в игровые процессы
Дополнение игрового процесса с элементами дополненной реальности предоставляет уникальные возможности. Игра становится более погружающей за счет наложения цифровых объектов на реальный мир. Например, использование интерактивных элементов на физическом столе с картами или фишками позволяет игрокам взаимодействовать с окружением совершенно новыми способами.
Для реализации таких решений необходима высокая степень технической подготовки. Разработчики игр могут использовать платформы Unity или Unreal Engine, которые поддерживают создание контента с AR-элементами. Это позволит интегрировать 3D-модели, анимации и звуковое сопровождение seamlessly.
Тестирование также играет ключевую роль. Нужно тщательно проверять, как пользователи взаимодействуют с AR-элементами, поскольку проблемы с производительностью могут снизить интерес к игре. Использование A/B тестирования поможет выявить наиболее эффективные механики. Например, если игрокам не нравится взаимодействие со смарт-асистентом в виде аватара, его следует переработать или убрать вовсе.
Социальные составляющие, такие как мультиплеерные функции, могут значительно обогатить опыт. Организация турниров или состязаний в реальном времени с использованием дополненной реальности создаст чувство общности и мотивацию возврата. Игроки смогут взаимодействовать друг с другом, даже находясь на расстоянии.
Монетизация с помощью микротранзакций также имеет смысл в таком формате. Продажа уникальных объектов, которые можно использовать в дополненной реальности, может приносить дополнительный доход. Например, особые карты или специальные фишки позволят игрокам выделиться, что создаст дополнительный интерес к продукту.
С учетом всех вышеперечисленных аспектов, внедрение дополненной реальности в игровой процесс способно значительно повысить уровень вовлеченности и удовлетворенности пользователей. Лишь правильная интеграция технологий, в сочетании с регулярным анализом пользовательского опыта, сможет обеспечить успех на этом насыщенном рынке.
Будущее азартных игр с использованием AR
Гр сегодняшнем контексте разработка новых возможностей для азартных игр становится все более актуальной. Интеграция дополненной реальности предоставляет уникальные шансы для создания захватывающего и интерактивного опыта. В отличие от традиционных решений, AR может предложить пользователям возможность взаимодействовать с игровыми элементами в реальном времени.
Согласно последним исследованиям, более 70% пользователей выразили желание попробовать азартные игры с применением AR. Это открывает рынок для новых форматов и методов вовлечения. К примеру, использование 3D-графики и переработанных интерфейсов позволит создать эффект присутствия, который невозможно достичь с простыми экранами.
Специалисты предполагают, что в ближайшие годы внедрение технологий будет направлено на создание immersive-среды. Это может включать в себя виртуальные туры по gaming zones, где пользователи смогут создавать свои аватары и взаимодействовать с другими участниками по всему миру. Разработка платформ, которые предлагают пользовательские настройки, станет важным этапом в будущем индустрии.
Безопасность данных также станет приоритетом. Внедрение блокчейн-технологий в сочетании с AR обеспечит прозрачность транзакций и защиту личной информации игроков. Это повысит уровень доверия и снизит возможные риски.
Не стоит забывать о социальной составляющей. AR может сделать азартные игры более доступными для широкой аудитории, предлагая уникальные возможности для взаимодействия с друзьями и создания групповых событий. Виртуальные турниры и соревнования могут привлечь внимание нового поколения игроков, искушенных в высоких технологиях.
Эксперты рекомендуют внедрять тестирование новых идей на ограниченной аудитории, чтобы оценить их привлекательность и возможности. Возможные партнерства с разработчиками технологий могут ускорить процесс внедрения новшеств в индустрии.
Таким образом, расширение границ азартных игр зависит от смелых решений и инновационного подхода. Актуальность этих технологий будет определяться меняющимся интересом пользователей и развитием современных трендов.
https://vk.com/7kregistraciya
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наши товары:
Металлическая мишень РРЅРґРёСЏ 5N плоская для распыления
Источник [url=https://megamoriartyc09o3seyehpnpnfnv7xo.lol]магазин мега мориарти[/url]
Non-Gamstop casino sites are so slick—totally hooked!
non gamstop casinos no deposit
РедМетСплав предлагает широкий ассортимент качественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица изделия подтверждена всеми необходимыми документами, подтверждающими их происхождение. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы разрешать ваши вопросы и адаптировать решения под особенности вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
Порошок кобальтовый Stellite 107 Порошок кобальтовый Stellite 107 – это высококачественный материал, предназначенный для нанесения РЅР° детали Рё инструменты, подвергающиеся интенсивным нагрузкам. Обладая отличной жаропрочностью Рё РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью, РѕРЅ идеально РїРѕРґС…РѕРґРёС‚ для использования РІ различных отраслях, включая авиастроение Рё машиностроение. Ртот порошок позволяет значительно продлить СЃСЂРѕРє службы изделий Рё улучшить РёС… эксплуатационные характеристики. Если РІС‹ хотите обеспечить надежность Рё долговечность СЃРІРѕРёС… деталей, рекомендуется купить Порошок кобальтовый Stellite 107 СѓР¶Рµ сегодня.
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наша продукция:
Шестигранник алюминиевый 40 РјРј РђРљ4-1 ГОСТ 8560-78 Выбирайте РёР· разнообразия размеров Рё конфигураций алюминиевых шестигранников. Надежное, прочное Рё устойчивое решение для строительных Рё монтажных работ. Рспользуйте шестигранники для создания каркасов, крепежных элементов, облицовки Рё отделки. Коррозионная устойчивость гарантирует долгий СЃСЂРѕРє службы РІ любых условиях.
На сайте https://face-me.ru/ вы сможете попробовать все возможности нейросетей и всего за несколько секунд превратить любое фото в видео. Инновационные и уникальные технологии дают возможность получить роскошную, яркую и любопытную фотосессию без профессионального оборудования, студии. Вы сможете создать фантастические образы и удивительные портреты в соответствии с предпочтениями. Эта программа дарит вам огромное количество возможностей и вдохновение. Вы каждый раз будете получать удивительные, красивые образы, которые всегда будут с вами.
Empty legs offers can save up to 75% of the standard price, see our actual flights list. Subscription makes offers fully transparent with all private flight costs clear https://www.callupcontact.com/b/businessprofile/Vector_Jet/9315149
займ по залог авто
https://c-hireepersonnel.com/employer/realestatez4206/
кредит залог авто наличные
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние возможности.
Наша продукция:
Распылительная мишень IGZO 4N – Плоская
РедМетСплав предлагает обширный выбор высококачественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица изделия подтверждена всеми необходимыми документами, подтверждающими их качество. Опытная поддержка – наш стандарт – мы на связи, чтобы улаживать ваши вопросы и находить ответы под специфику вашего бизнеса.
Доверьте ваш запрос специалистам РедМетСплав и убедитесь в множестве наших преимуществ
поставляемая продукция:
РўСЂСѓР±Р° висмутовая 42 3990 – CSN/STN 423990 РўСЂСѓР±Р° висмутовая 42 3990 – CSN/STN 423990 представляет СЃРѕР±РѕР№ высококачественный металлургический РїСЂРѕРґСѓРєС‚, отличающийся своей прочностью Рё РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ устойчивостью. Разработанная СЃ учетом современных стандартов, РѕРЅР° идеально РїРѕРґС…РѕРґРёС‚ для различных промышленных применений. РўСЂСѓР±Р° обеспечивает надежную работу РІ условиях высоких температур Рё агрессивной среды. РќРµ упустите возможность купить РўСЂСѓР±Р° висмутовая 42 3990 – CSN/STN 423990 Рё убедитесь РІ её превосходных характеристиках. Данный РїСЂРѕРґСѓРєС‚ станет незаменимым помощником РІ вашей работе Рё обеспечит долгий СЃСЂРѕРє службы.
На сайте https://fguard.ru/ почитайте статьи на самую разную тему. К примеру, кибермошенничество, для каких целей используется сеточка, которая на двери микроволновки. Здесь представлена вся нужная информация о смарт-часах и о том, чем они отличаются от бизнес-браслетов. Для того чтобы подыскать нужную информацию, воспользуйтесь специальным рубрикатором. На сайте вы найдете записи, которые касаются нейросетей, гаджетов. Представлены и интересные рекомендации, которые будут необходимы каждому. Имеются данные и про технологии.
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – идеальный вариант для вас.
Наша продукция:
РџРѕРєРѕРІРєР° прямоугольная РёР· конструкционной стали 500С…1000 РјРј 45РҐ Конструкционная прямоугольная РїРѕРєРѕРІРєР° – высококачественные материалы для создания прочных Рё надежных конструкций. Рзготовленная РёР· высококачественных материалов СЃ применением передовых технологий, эта продукция обладает отличной прочностью, устойчивостью Рє РёР·РЅРѕСЃСѓ, Рё находит широкое применение РІ машиностроении Рё строительстве.
На сайте https://remont-okon-63.ru/ уточните номер телефона для того, чтобы воспользоваться такой нужной услугой, как ремонт, регулировка окон независимо от сложности. Прямо сейчас вы сможете воспользоваться возможностью вызвать мастера. Для этого следует указать номер телефона, а также имя. Среди основных услуг, которые оказываются в этой компании, выделяют: ремонт балконных дверей, производство москитных сеток, стеклопакетов. Кроме того, доступен и срочный ремонт конструкций. Все работы выполняются в соответствии с требованиями.
Non Gamstop casinos no deposit deals are a game-changer—wow!
non gamstop casino site
ссылка на сайт [url=https://megamoriartyc09o3seyehpnpnfnv7xo.lol]магазин мега мориарти[/url]
Новини та інформація про місто Кропивницький. Сучасний портал https://kropyvnytskyi.kr.ua/ про місто Кропивницький. Актуальна довідкова інформація про місто, новини, публікації.
автоломбард под залог
https://feelhospitality.com/employer/zaim-pod-zalog-pts-14690/
автоломбард залог
Юридические услуги urwork.ru в Санкт-Петербурге и Москве – от консультации до защиты интересов в суде. Оперативно, надежно и с гарантией конфиденциальности.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наша продукция:
Керамическая мишень Licoo2 (3N5, 4N)
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Обладая обширной линейкой редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние успехи.
Наши товары:
Мишень для распыления скандия
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние успехи.
Наша продукция:
Распыляемая мишень из эрбия, плоская
На сайте https://avtomastera.net вы найдете различную полезную, актуальную информацию, которая касается ремонта автомобилей. Здесь представлены самые разные рекомендации, которые обязательно помогут мастерам, ответят на многочисленные вопросы, расскажут обо всех нюансах. Вся информация поделена на категории, чтобы было легче и быстрее сориентироваться. Для того чтобы получить доступ ко всем функциям, пройдите регистрацию. Здесь также вы найдете и программы, которые применяются для диагностики.
https://moskva-telefon.ru/
[url=https://blotos.ru/news/tri_v_ryad__igrayte_onlayn_besplatno_i_bez_registracii.html]https://blotos.ru/news/tri_v_ryad__igrayte_onlayn_besplatno_i_bez_registracii.html[/url] играть в кто хочет стать миллионером
Открой для себя самые популярные товары Apparel X, которые завоевали сердца спортсменов и любителей активного образа жизни. Эти вещи созданы для того, чтобы вы могли играть на максимуме теннисные футболки и шорты
РедМетСплав предлагает широкий ассортимент отборных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы обеспечиваем быстрое выполнение вашего заказа.
Каждая единица продукции подтверждена соответствующими документами, подтверждающими их происхождение. Опытная поддержка – наш стандарт – мы на связи, чтобы ответить на ваши вопросы по мере того как адаптировать решения под особенности вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наша продукция:
Фольга вольфрамовая Р’Р -20 Фольга вольфрамовая Р’Р -20 – это высококачественный материал, обладающий отличной прочностью Рё стойкостью Рє высоким температурам. РћРЅР° идеально РїРѕРґС…РѕРґРёС‚ для применения РІ электронике, металлургии Рё научных исследованиях. Благодаря своей высокой теплопроводности, фольга обеспечивает эффективный теплообмен. Купить Фольга вольфрамовая Р’Р -20 – значит выбрать надежный РїСЂРѕРґСѓРєС‚, который прослужит долго Рё поможет РІ решении сложных задач. РќРµ упустите шанс обеспечить СЃРІРѕР№ проект лучшим материалом, который позволит добиться высоких результатов.
РедМетСплав предлагает широкий ассортимент отборных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица изделия подтверждена соответствующими документами, подтверждающими их происхождение. Дружелюбная помощь – наша визитная карточка – мы на связи, чтобы разрешать ваши вопросы и находить ответы под особенности вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
Круг кобальтовый Stellite 228 Круг кобальтовый Stellite 228 — это высококачественный абразивный инструмент, предназначенный для обработки различных металлов. Его уникальная структура обеспечивает отличную стойкость к износу и коррозии, что делает его идеальным выбором для сложных условий эксплуатации. Если вам необходимо обеспечить долгий срок службы инструмента и высокую точность обработки, то Круг кобальтовый Stellite 228 станет незаменимым помощником в вашем арсенале. Не упустите возможность купить Круг кобальтовый Stellite 228 и улучшить производительность своих рабочих процессов.
РедМетСплав предлагает широкий ассортимент отборных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы обеспечиваем быстрое выполнение вашего заказа.
Каждая единица товара подтверждена всеми необходимыми документами, подтверждающими их качество. Опытная поддержка – наша визитная карточка – мы на связи, чтобы улаживать ваши вопросы по мере того как адаптировать решения под специфику вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
Наша продукция:
Лист кобальтовый Stellite 703 Лист кобальтовый Stellite 703 – это высококачественный материал, разработанный для работы в экстремальных условиях. Его основное предназначение заключается в обеспечении превосходной коррозионной стойкости и высокой прочности при повышенных температурах. Состав данного листа включает кобальт, что позволяет ему легко выдерживать агрессивные среды и механические нагрузки.Применение данного листа распространено в таких отраслях, как энергетика, нефтехимия и производство оборудования. Приобретая этот товар, вы обеспечиваете надежность и долговечность вашей продукции. Не упустите шанс купить Лист кобальтовый Stellite 703 и оценить его преимущества на практике.
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с высоким уровнем сервиса.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наша продукция:
Труба бронзовая 100х12.5х3500 мм БрАМц9-2 ГОСТ 1208-2014 Приобретайте трубы бронзовые круглые от профессионалов с опытом. Мы предлагаем широкий ассортимент качественной продукции, быструю обработку заказов и оперативную доставку. Обращайтесь, чтобы получить консультацию специалиста и выгодные условия покупки.
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
Наша продукция:
Рнструментальная круглая РїРѕРєРѕРІРєР° 48 РјРј 4РҐ5МФС ГОСТ 5950-2000 РЁРёСЂРѕРєРёР№ выбор круглых РїРѕРєРѕРІРѕРє для профессиональной обработки металла. Рнструментальная круглая РїРѕРєРѕРІРєР° высочайшего качества СЃ разнообразием размеров Рё форм. Повышенная прочность, износостойкость Рё превосходное качество изготовления. Обеспечивает отличное сцепление СЃ материалом Рё эффективную работу оборудования. Покупайте РїСЂСЏРјРѕ сейчас!
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – идеальный вариант для вас.
Наши товары:
Кольцо РёР· драгоценных металлов платиновое 20С…8С…1.5 РјРј ПлРд90-10 РўРЈ РЁРёСЂРѕРєРёР№ выбор высококачественных кольц РёР· драгоценных металлов. Рлегантные украшения, созданные талантливыми мастерами. Уникальность Рё изысканность РІ каждой детали. Возможность заказа персонального кольца РїРѕ вашим пожеланиям. Подчеркните СЃРІРѕСЋ индивидуальность СЃ нашими кольцами.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Оксид гафния (HfO2) гранулированный
Non Gamstop UK casino feels so fresh—big fan here!
new non gamstop casinos
Продам лекарства после лечения В мире, где здоровье ценится превыше всего, вопросы о продаже лекарственных препаратов становятся все более актуальными. Существуют различные ситуации, когда люди сталкиваются с необходимостью продать лекарства, будь то остатки после лечения, неиспользованные препараты или даже специализированные средства для онкобольных. Варианты продажи варьируются от личных объявлений и форумов до онлайн-платформ, предлагающих широкий охват аудитории. Однако, необходимо помнить о юридических и этических аспектах такой деятельности. Продажа лекарств с рук, особенно сильнодействующих или рецептурных препаратов, может быть незаконной и небезопасной. Важно тщательно изучить законодательство и соблюдать правила хранения и транспортировки медикаментов.
деньги под залог машины
https://corerecruitingroup.com/employer/elitistpro1877/
автоломбард
РедМетСплав предлагает широкий ассортимент качественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы обеспечиваем оперативное исполнение вашего заказа.
Каждая единица изделия подтверждена соответствующими документами, подтверждающими их соответствие стандартам. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы ответить на ваши вопросы по мере того как находить ответы под специфику вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
поставляемая продукция:
РљСЂСѓРі висмутовый CAC912 – JIS H 5120-2016 РљСЂСѓРі висмутовый CAC912 – JIS H 5120-2016 представляет СЃРѕР±РѕР№ высококачественный РїСЂРѕРґСѓРєС‚, идеально подходящий для различных технологических процессов. Ртот РєСЂСѓРі обладает отличной электрической проводимостью Рё устойчивостью Рє РєРѕСЂСЂРѕР·РёРё, что делает его незаменимым РІ производстве Рё ремонте. Выбирая данный товар, РІС‹ получаете надежность Рё эффективность. Если РІС‹ хотите оптимизировать СЃРІРѕРё рабочие процессы, советуем купить РљСЂСѓРі висмутовый CAC912 – JIS H 5120-2016. РћРЅ обеспечит стабильную работу ваших устройств Рё позволит добиться отличных результатов РІ применении.
Официальный партнер окон REHAU https://okna-intex.ru/ это возможность заказать по выгодным ценам окна с установкой под ключ. Ознакомьтесь на сайте со всеми преимуществами окон Рехау или рассчитайте стоимость окон по доступной цене. Мы предлагаем широкий выбор оконных систем, включая пластиковые и алюминиевые окна. Наши окна отличаются высоким качеством, долговечностью и надежностью.
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с прекрасным качеством обслуживания.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
Наши товары:
Медный однораструбный угол под пайку 90 градусов 9х7х0.6 мм 7.8х9.8 мм твердая пайка М2М ГОСТ Р52922-2008 Приобретите надежные медные одноразъемные углы под пайку от Редметсплав. Широкий выбор по размерам и формам. Гарантированное качество и надежность соединения медных труб. Получите консультацию специалистов и дополнительную информацию о применении продукции.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Обладая обширной линейкой редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наши товары:
Плоская мишень CoFe для распыления сплавов
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
С широким ассортиментом продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Плоская металлическая мишень Церия
Если у Вас проблемы с любимым существом, Ваши отношения уже зашли в тупик, и Вы абсолютно перестали понимать друг друга Доставка цветов на Roza4u.ru@
https://intekey.ru/
Best non Gamstop casino I’ve played at had fast support—nice!
non gamstop casinos no deposit bonus
xBet bookmaker provides players with great opportunities for comfortable, and most importantly, for an effective and efficient betting game http://megan.ramsden@kingsley.idehen.net/PivotViewer/?url=https://www.google.si/url%3Fq=https://edicionesdelau.com/articles/1win_promo_code_offer.html
https://intekey.ru/
Заказала цветы с доставкой на работу
доставка цветов томск на дом
pegas ru Компания пегас является одним из лидеров рынка и работает в сфере международного туризма, предлагая выгодные туры в Таиланд, Турцию, Египет и другие страны мира. Подбор тура и заказ осуществляется в удобном личном кабинете, где можно забронировать отель, купить билеты и оформить путевку без переплат онлайн. Турфирма Pegas работает с проверенными отелями и предлагает широкий выбор направлений и услуг, включая авиабилеты, круизы, трансферы, путевки и подбор лучших отелей для проживания.
автоматизация бизнес-процессов
Ищете оригинальный мерч от любимых российских и зарубежных звезд, фестивалей и шоу? Посетите https://showstaff.ru/ – мы с 2002 года радуем наших клиентов качественной продукцией, доставкой по всей России и поддержкой талантливых артистов. Заходите на наш сайт и выбирайте свой стиль! ShowStaff – это магазин оригинального мерча, официально и качественно. Посетите каталог, и вы обязательно найдете для себя уникальные вещи!
заказать продвижение и оптимизация https://prodvizhenietargeting.ru
продвижение и оптимизация сайтов https://prodvizheniestatya.ru
поисковое продвижение в интернете seo продвижение
РедМетСплав предлагает широкий ассортимент высококачественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица товара подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Опытная поддержка – наша визитная карточка – мы на связи, чтобы улаживать ваши вопросы по мере того как предоставлять решения под особенности вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
поставляемая продукция:
Фольга ниобиевая БТЦ-Р’Р” – ГОСТ 10994-74 Фольга ниобиевая БТЦ-Р’Р” – ГОСТ 10994-74 является высококачественным продуктом, который применяется РІ различных отраслях, включая электронику Рё аэронавтику. Благодаря своей отличной стойкости Рє РєРѕСЂСЂРѕР·РёРё Рё высоким температурам, РѕРЅР° обеспечивает надежность Рё долговечность РІ эксплуатации. Рта фольга имеет уникальные физико-химические свойства, что делает ее идеальной для высокоспецифичных приложений. Если РІС‹ хотите купить Фольга ниобиевая БТЦ-Р’Р” – ГОСТ 10994-74, РІС‹ сделаете правильный выбор, обеспечивая СЃРІРѕСЋ продукцию только лучшим материалом, который превосходит ожидания Рё стандартные требования отрасли.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
С широким ассортиментом продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Плоская мишень AlSi для распыления
автоломбард круглосуточно
https://eleeo-europe.com/employer/avtolombard-zalog-invest9851/
автомобиль под залог
https://firelak.ru В современном строительстве и отделке, где дерево занимает важное место, обеспечение пожарной безопасности становится приоритетной задачей. Огнезащитный лак – это эффективное решение для защиты деревянных конструкций и элементов интерьера от возгорания и распространения пламени.
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – идеальный вариант для вас.
поставляемая продукция:
Латунная полоса 1×50 РјРј Р›90 ГОСТ 931-90 Покупайте латунную полосу различных размеров Рё толщин РЅР° сайте Редметсплав.СЂС„. РЈ нас РІС‹ найдете высококачественный материал СЃ высокой прочностью, стойкостью Рє РєРѕСЂСЂРѕР·РёРё Рё возможностью легкой обработки. Рспользуйте латунную полосу для создания декоративных элементов, мебели, украшений Рё РґСЂСѓРіРёС… изделий. Доступны различные размеры Рё толщины.
Visit https://bestcasinoideal.com/ and discover expert reviews, top strategies and essential tips. Explore online slots, table games, live dealer experiences. We have compiled a complete collection of the best online casinos and gambling sites for 2025, tested and rated by experts.
РедМетСплав предлагает обширный выбор отборных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до масштабных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица продукции подтверждена требуемыми документами, подтверждающими их происхождение. Опытная поддержка – наша визитная карточка – мы на связи, чтобы разрешать ваши вопросы и находить ответы под особенности вашего бизнеса.
Доверьте ваш запрос специалистам РедМетСплав и убедитесь в гибкости нашего предложения
Наши товары:
Лист магниевый G-M2 Лента магниевая G-M2 – это высококачественный продукт, предназначенный для использования в различных сферах. Она идеально подходит для проведения работ, связанных с магниевыми сплавами и легирования. Данная лента обладает отличной прочностью и устойчивостью к коррозии. Благодаря своим характеристикам, лента обеспечивает надежную защиту и долговечность. Не упустите возможность улучшить качество своей работы и купить Лента магниевая G-M2. С ее помощью вы сможете выполнять задачи быстрее и эффективнее, что сделает вашу деятельность более продуктивной.
Онлайн казино с джекпот-слотами — это интрига!
https://telegra.ph/Mostbet-kazino-kak-poluchit-bonus-za-aktivnuyu-igru-03-27
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
поставляемая продукция:
Лента РёР· прецизионных сплавов для СѓРїСЂСѓРіРёС… элементов 0.13×68 РјРј РЎРџ22 ГОСТ 14117-85 Купите ленту РёР· прецизионных сплавов для СѓРїСЂСѓРіРёС… элементов РїРѕ выгодной цене РЅР° Редметсплав.СЂС„. Высокая прочность, устойчивость Рє РєРѕСЂСЂРѕР·РёРё Рё отличная эластичность делают этот материал идеальным для различных отраслей. Предлагаем широкий ассортимент продукции Рё РїРѕРґСЂРѕР±РЅСѓСЋ информацию для правильного выбора.
Welcome to the official Joy Casino blog https://officialjoycasino.net/ — a place where you can explore the fascinating world of online gambling from different perspectives: game mechanics, psychology, responsible gaming and industry analytics. If you are looking for a deep dive into gaming strategies, casino technology or responsible gaming tips, you have come to the right place.
Best non Gamstop casino for me has been a total game-changer.
non gamstop casinos reviews
На сайте https://kometagaming.site/ мы собрали рейтинг официальных букмекерских контор с лицензией, которые позволяют легально делать ставки на спорт и где будет гарантирована честная игра и своевременные выплаты. Мы решили этот вопрос и собрали лучшие компании в топ букмекерских контор. Лучшие официальные букмекерские конторы для ставок на спорт – это полезная информация для тех, кто хочет начать играть, а также получать различные бонусы и легкий вывод денег.
СанОбработка – компания, которая специализируется на предоставлении профессиональных услуг по дезинфекции, дератизации, дезинсекции. Гарантируем высокий уровень действенности и безопасности. Обращайтесь к нам тогда, когда вам надо. https://sanobrabotkarf.ru – тут можете с отзывами клиентов ознакомиться. Наши компетентные специалисты готовы к вам на помощь приехать в любое время. Они проведут тщательную обработку и будут использовать сертифицированные европейские препараты. Проблема решится, ваш дом станет вновь уютным и безопасным.
заказать продвижение и оптимизация заказать продвижение сайта недорого
Ищете топливные карты для юридических лиц и ИП? Обратите внимание на единую топливную карта ЛИОТЭК для бизнеса. Узнайте на сайте https://liotec.ru/ все преимущества выгодной карты Лиотек и какие огромные преимущества она дает, по сравнению с другими картами. Подробнее на сайте.
РедМетСплав предлагает внушительный каталог отборных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица изделия подтверждена всеми необходимыми документами, подтверждающими их происхождение. Дружелюбная помощь – наша визитная карточка – мы на связи, чтобы ответить на ваши вопросы и предоставлять решения под требования вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
Наша продукция:
РљСЂСѓРі вольфрамовый РР’Р-1 РљСЂСѓРі вольфрамовый РР’Р-1 – это высококачественный абразивный инструмент, предназначенный для обработки различных материалов. РћРЅ обеспечивает отличную долговечность Рё стойкость Рє перегреву. Благодаря своей структуре, РљСЂСѓРі вольфрамовый РР’Р-1 идеально РїРѕРґС…РѕРґРёС‚ для резки Рё шлифования как РІ промышленных, так Рё РІ домашних условиях. Если РІС‹ ищете надежный инструмент для СЃРІРѕРёС… задач, купить РљСЂСѓРі вольфрамовый РР’Р-1 – это правильный выбор. Его эффективность Рё надежность делают его незаменимым РІ вашем арсенале. Откройте новые возможности СЃ этим РєСЂСѓРіРѕРј!
Планируете каникулы? купить https://camp-centr.com! Интересные программы, безопасность, забота и яркие эмоции. Бронируйте заранее — количество мест ограничено!
Выполняем проектирование https://energopto.ru и монтаж всех видов инженерных систем для жилых и коммерческих объектов. Профессиональный подход, сертифицированное оборудование, гарантия качества.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наши товары:
Плоская мишень из иттербия
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
Наши товары:
Медная двухраструбная редукционная переходная муфта РїРѕРґ пайку 14.7С…14 РјРј твердая пайка Рњ3С‚ ГОСТ Р 52922-2008 Выберите высококачественные медные двухраструбные редукционные муфты РїРѕРґ пайку РѕС‚ Редметсплав для надежного соединения медных труб различных диаметров. РЁРёСЂРѕРєРёР№ выбор размеров, эффективная установка Рё долговечность гарантированы. Рдеальное решение для систем отопления Рё водоснабжения.
[url=https://https-kra30.at]кракен магазин[/url] – kraken32, кра ссылка
Оформление визы в Китай Планируете поездку в Китай? Наш визовый центр предоставляет полный спектр услуг по оформлению виз в Китай для различных целей: туризм, бизнес, учеба. Мы оказываем профессиональные консультации по всем вопросам, связанным с визовым режимом Китая и подготовкой необходимых документов. Мы поможем вам оформить туристическую визу, бизнес-визу, а также визу для студентов. Наши специалисты подробно проконсультируют вас о необходимых документах, сроках получения визы и ответят на все ваши вопросы. Мы также предлагаем услуги экспресс-визы для тех, кому требуется срочное оформление.
[url=https://kra29-at.at]kra30 cc[/url] – kra at, kraken marketplace
автоматизация бизнес-процессов
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наши товары:
Плоская мишень из фтористого магния
https://orgnaztech.mirtesen.ru/blog/43202871346/Gde-Kazhdyiy-Gost-Osobennyiy-Otel-Glory
https://intekey.ru/
Онлайн казино с бонусом без депозита — мечта любого игрока.
https://telegra.ph/Obzor-slotov-s-progressivnymi-bonusami-v-Mostbet-kazino-03-27
Non Gamstop casinos no deposit deals are my secret weapon—love them!
non gamstop casino free spins no deposit
На сайте http://ammely.ru представлены интересные, увлекательные статьи, которые точно понравятся женской аудитории. Опубликован контент о моде, красоте, здоровом образе жизни. Также имеются и полезные рецепты, которые идеально подойдут на каждый день либо на праздник, если внезапно нагрянули гости. Есть увлекательные рекомендации на тему дома, дизайна. Вам будет интересно узнать и о звездах, о том, как лучше и веселей провести праздник.
Сайт torentino.org предлагает вам скачать игры через торрент. Предлагаем целый ряд конкурентных преимуществ. Наши игры регулярно обновляются. Комфортная система поиска дает возможность быстро найти то, что нужно. Насладитесь возможностями новейшей индустрии развлечений. Ищете скачать minecraft 1 2 торрент? Torentino.org – тут различные игры представлены, они время скоротать помогут. Мы их распределили по множеству категорий, чтобы вам комфортно было скачать и найти игру. Каждый файл обязательно проверяется на наличие вирусов. Быстрых вам загрузок!
кредит под птс спецтехники
https://convia.gt/employer/avtolombard-111177/
получить займ под залог авто
[url=https://https-kra30.at/]kraken официальный сайт[/url] – kra32.cc, kraken зайти
Сайт zapchasti-zf-eaton-yakutsk.ru предлагает запчасти для грузовых автомобилей. В ассортименте у нас представлены от множества производителей различные подшипники. Вы можете заказать простые и сложные детали, обратившись к нам. Ищете eaton кпп? Zapchasti-zf-eaton-yakutsk.ru – здесь можно найти схемы, которые помогут вам лучше разобраться в конструкции трансмиссии и ее компонентах. Мы профессиональная команда в сфере поставок для грузовых машин автозапчастей. Предоставляем самые выгодные условия для оптовых закупок автокомпонентов.
Создание QR кодов
Создание QR кодов
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
поставляемая продукция:
Кольцо РёР· драгоценных металлов золотое 9С…3С…0.5 РјРј ЗлМ98 РўРЈ РЁРёСЂРѕРєРёР№ выбор высококачественных кольц РёР· драгоценных металлов. Рлегантные украшения, созданные талантливыми мастерами. Уникальность Рё изысканность РІ каждой детали. Возможность заказа персонального кольца РїРѕ вашим пожеланиям. Подчеркните СЃРІРѕСЋ индивидуальность СЃ нашими кольцами.
Кто-нибудь выигрывал джекпот в онлайн казино? Расскажите!
https://telegra.ph/Vavada-kazino-registraciya-i-izmenenie-dannyh-profilya-03-27
Caspian Training Group преподаватели компании знают толк в своем деле. Они регулярно курсы повышения квалификации проходят. Вас персональный подход компании порадует. https://xn—-7sbecpcasfm0beeaecirc5b3a2g.kz/ – здесь представлена более подробная информация о нас, ознакомиться с ней можно прямо сейчас. Caspian Training Group компаниям помогает реализовывать успешно корпоративного обучения проекты. Результаты превзойдут ваши ожидания. Сотрудники увереннее в своей работе станут и навыки взаимодействия в команде улучшат.
https://qrkoder.ru/
[url=https://kra29-at.at]kraken shop[/url] – кракен официальный сайт, кракен клир
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
С широким ассортиментом продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние успехи.
Наша продукция:
Хромовые гранулы
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Обладая обширной линейкой редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наши товары:
Титановая плоская мишень
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наши товары:
Плоская мишень магниевая
На берегу моря нашли русалку https://x.com/Fariz418740/status/1905487137991958614
РедМетСплав предлагает широкий ассортимент качественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы обеспечиваем быстрое выполнение вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их соответствие стандартам. Опытная поддержка – наша визитная карточка – мы на связи, чтобы разрешать ваши вопросы а также находить ответы под требования вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в гибкости нашего предложения
Наша продукция:
Лента висмутовая C49350 – UNS Лента висмутовая C49350 – UNS – это высококачественный материал, используемый РІ различных отраслях благодаря своей уникальной химической стойкости Рё отличной теплопроводности. РћРЅР° прекрасно РїРѕРґС…РѕРґРёС‚ для создания сплавов Рё различных конструкций. Лента визмутовая легко обрабатывается Рё может применяться РІ медицинской, электронной Рё химической промышленности. Если РІС‹ хотите купить Лента висмутовая C49350 – UNS, РІС‹ делаете правильный выбор. Ртот РїСЂРѕРґСѓРєС‚ отвечает самым высоким стандартам качества Рё надежности. Заказывайте СѓР¶Рµ сегодня для достижения максимальной эффективности РІ вашем проекте.
https://github.com/azure-wiki/Azure-Storage-Explorer/releases
https://intekey.ru/
Создание QR кодов
Non Gamstop casinos are perfect for unrestricted gaming—highly recommend!
no deposit bonus casino non gamstop
автоломбард залог авто
https://saek-kerkiras.edu.gr/employer/gigsonline782/
займ под залог птс авто
elonbet casino
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние успехи.
Наша продукция:
Ниобиевый тигель высокой чистоты
A.X.O.N – компания, которая предоставляет профессиональные услуги. Создаем AI-решения для бизнеса. Работаем по всему миру, как с физическими, так и с юридическими лицами. Условия оплаты и формат индивидуально под ваш запрос обсуждаются. https://axonbusiness.ru – тут представлены ответы на популярные вопросы. Вы узнаете, какие задачи можно автоматизировать. Мы выстраиваем бизнес-логику, настраиваем процессы и доводим до результата. Всегда на связи и готовы ответить на интересующие вопросы. Давайте с консультации начнем, это абсолютно бесплатно.
На берегу моря нашли русалку https://x.com/Fariz418740/status/1905487137991958614
elonbet casino BD
elonbet casino
MetaMask Chrome works flawlessly! I use it daily for DeFi transactions and NFT trading. Smooth and secure experience every time.
[url=https://https-kra30.at]kra32.cc[/url] – kraken32, кракен войти
Прекрасный бюстгальтер, заказала уже второй, мне именно с такой застежкой удобен и нравится Бюстгальтеры Caitline с высокой поддержкой
elonbet casino
elonbet casino BD
elonbet casino
Компания АБК предоставляет аренду бытовок, которые из качественных материалов изготавливаются. Ассортимент выбора контейнеров большой, цены приемлемые. Процесс аренды прост и понятен, сотрудники профессиональны и внимательны к пожеланиям. Ищете аренда строительные вагончики цена? Arendabk.ru – сайт, где есть фотогалерея и отзывы. Предоставляем в аренду только надежные контейнеры. Доставим их в минимальные сроки. С удовольствием ответим на интересующие вас вопросы и поможем сделать правильный выбор. Для нас важно, чтобы вам было приятно с нами работать.
На сайте https://smartflow.ru вы сможете выбрать качественную, функциональную сантехнику для обустройства ванной комнаты в доме либо квартире. Ассортимент «SMARTFLOW» включает в себя такие изделия, которые выполнены из высококачественных и современных материалов, безупречного стиля. Все унитазы, биде, модульные ванные идеально впишутся в концепцию. Каждая вещь идеально сочетает надежность, прочность, а также изысканность. Для того чтобы выбрать что-то определенное, изучите галерею, ознакомьтесь и с особой системой смыва «Торнадо».
Welcome to the official Joy Casino blog https://officialjoycasino.net/ — a place where you can explore the fascinating world of online gambling from different perspectives: game mechanics, psychology, responsible gaming and industry analytics. If you are looking for a deep dive into gaming strategies, casino technology or responsible gaming tips, you have come to the right place.
взять кредит под залог машины
http://jobs.foodtechconnect.com/companies/avtolombard-zalog-invest665/
займы залог авто новосибирск
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние успехи.
Наши товары:
Мишень для распыления сплава AlCu
РедМетСплав предлагает обширный выбор отборных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица товара подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Превосходное обслуживание – наша визитная карточка – мы на связи, чтобы разрешать ваши вопросы по мере того как находить ответы под требования вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наша продукция:
РџРѕРєРѕРІРєР° кобальтовая Stellite 4LC РџРѕРєРѕРІРєР° кобальтовая Stellite 4LC представляет СЃРѕР±РѕР№ высококачественный материал, предназначенный для применения РІ условиях высоких температур Рё агрессивной среды. Рта РїРѕРєРѕРІРєР° обладает отличной РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ устойчивостью Рё высокой прочностью, что делает её идеальным выбором для различных промышленных задач. Уникальные свойства кобальтовых сплавов обеспечивают долговечность Рё надежность РІ эксплуатации. Если РІС‹ ищете надёжное решение для своего производства, РЅРµ упустите возможность купить РџРѕРєРѕРІРєР° кобальтовая Stellite 4LC СѓР¶Рµ сегодня. РњС‹ гарантируем вам качественное обслуживание Рё быстрое выполнение вашего заказа.
РедМетСплав предлагает широкий ассортимент высококачественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица продукции подтверждена требуемыми документами, подтверждающими их происхождение. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы ответить на ваши вопросы и адаптировать решения под специфику вашего бизнеса.
Доверьте ваш запрос специалистам РедМетСплав и убедитесь в множестве наших преимуществ
Наши товары:
Проволока кобальтовая Stellite 7 Проволока кобальтовая Stellite 7 представляет СЃРѕР±РѕР№ уникальный материал, обладающий высокой прочностью Рё стойкостью Рє РєРѕСЂСЂРѕР·РёРё. РћРЅР° идеально РїРѕРґС…РѕРґРёС‚ для сварки Рё наплавки высоконагруженных элементов, Р° также для защиты деталей РІ агрессивных средах. Если РІС‹ ищете надежное решение для СЃРІРѕРёС… проектов, то вам стоит купить Проволока кобальтовая Stellite 7. Ртот РїСЂРѕРґСѓРєС‚ обеспечит долговечность Рё высокое качество выполнения работ. Выбирая Stellite 7, РІС‹ получаете уверенность РІ результате Рё высокую производительность ваших изделий. Лучшие результаты обязательно Р±СѓРґСѓС‚ достигнуты!
На сайте https://antipoligraf.ru/ почитайте про Институт прикладной психофизиологии. Здесь ведется профессиональная, комплексная подготовка к тому, чтобы пройти полиграф. Учат и тому, чтобы быстро, безопасно и комплексно снять стресс при помощи уникальных и инновационных приборов обратной связи. И самое важное, что все это абсолютно безопасно, при этом гарантированно поможет. Кроме того, ведется обучение саморегуляции при помощи обратной связи. У института огромное количество партнеров, которые стараются работать в комплексе.
РедМетСплав предлагает широкий ассортимент высококачественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица изделия подтверждена требуемыми документами, подтверждающими их происхождение. Дружелюбная помощь – то, чем мы гордимся – мы на связи, чтобы разрешать ваши вопросы а также предоставлять решения под требования вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в гибкости нашего предложения
поставляемая продукция:
Фольга висмутовая 42 3993 – CSN/STN 423993 Фольга висмутовая 42 3993 – CSN/STN 423993 является высококачественным материалом, идеально подходящим для различных областей применения, включая электронику Рё научные исследования. Рта фольга отличается отличной проводимостью Рё устойчивостью Рє РєРѕСЂСЂРѕР·РёРё, что делает ее отличным выбором для профессионалов.Если РІС‹ ищете надежный Рё эффективный материал, то вам стоит купить Фольга висмутовая 42 3993 – CSN/STN 423993. Данный РїСЂРѕРґСѓРєС‚ предлагает отличное соотношение цены Рё качества, обеспечивает высокую надежность РїСЂРё эксплуатации Рё удовлетворит потребности даже самых требовательных клиентов.РќРµ упустите возможность создать продукцию СЃ идеальными характеристиками благодаря этой фольге!
elonbet casino BD
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Вращающаяся мишень для распыления вольфрама
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наши товары:
Медное двухраструбное колено под пайку 180 градусов 12х9х0.6 мм 8.6х10.6 мм мягкая пайка М1 ГОСТ 32590-2013 Купите надежные медные двухраструбные колена под пайку от ведущего производителя. Обеспечьте эффективное отопление и водоснабжение с прочными и устойчивыми к воздействию внешних факторов коленами из меди.
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
Наша продукция:
Медная двухраструбная редукционная переходная муфта РїРѕРґ пайку 21С…16 РјРј мягкая пайка Cu-DHP ГОСТ Р 52922-2008 Выберите высококачественные медные двухраструбные редукционные муфты РїРѕРґ пайку РѕС‚ Редметсплав для надежного соединения медных труб различных диаметров. РЁРёСЂРѕРєРёР№ выбор размеров, эффективная установка Рё долговечность гарантированы. Рдеальное решение для систем отопления Рё водоснабжения.
бесплатный онлайн генератор QR кодов
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с прекрасным качеством обслуживания.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
Наши товары:
Лента СЃ заданными свойствами упругости 0.13С…250 РјРј 40РљРҐРќРњ ГОСТ 14117-85 Лента СЃ заданными свойствами упругости предлагает высококачественные материалы, определенные параметры упругости Рё широкий выбор размеров для надежного Рё РіРёР±РєРѕРіРѕ крепления. Рспользуется РІ строительстве, производстве Рё машиностроении. Обеспечивает легкость установки, надежность, долговечность Рё адаптацию Рє различным техническим требованиям.
https://qrkoder.ru/
elonbet casino BD
Заказала 44 размер – подошёл perfectly!
женские костюмы томск
elonbet casino
elonbet casino
Печать рекламных буклетов https://tipografiya-buklety.ru ярко, качественно, профессионально. Форматы A4, евро, индивидуальные размеры. Работаем с частными и корпоративными заказами.
elonbet casino BD
риобет скачать рио бет
Типография Copy General окажет помощь в воплощении ваших идей в реальность. Мы понимаем, что любой ваш проект является неповторимым и требует особого подхода. Время клиентов мы ценим. Гарантируем высочайшую скорость осуществления заказов. https://copygeneral.ru – тут с отзывами заказчиков можете ознакомиться. Copy General – типография, на которую вы спокойно можете положиться. Постоянно над новыми решениями работаем, чтобы помогать своим клиентам задачи решать с дизайном, маркетингом и полиграфией. Применяйте преимущества нашей типографии!
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние возможности.
Наши товары:
Керамическая мишень для распыления диоксида кремния
автоматизация бизнес-процессов
Лучший генератор QR-кодов
займ под залог автоломбард
https://www.dataalafrica.com/employer/smlord9729/
кредит под залог птс новосибирск
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наши товары:
Пружина из драгоценных металлов серебряная 650х2х0.5 мм СрМ95 ТУ Выберите идеальные пружины из золота, серебра или платины, чтобы дополнить ваше украшение. Найдите пружины с изысканным дизайном и прочностью. Они предлагают широкий выбор для того, чтобы подчеркнуть уникальность вашего украшения.
elonbet casino BD
Создание QR кодов
https://orgnaztech.mirtesen.ru/blog/43202871346/Gde-Kazhdyiy-Gost-Osobennyiy-Otel-Glory
elonbet casino
Аренда комфортабельных катеров и яхт в Санкт-Петербурге от https://7futov.spb.ru/ это удобная возможность осуществить водные прогулки по СПб. У нас большой выбор маршрутов и организация мероприятий под ключ. Самые выгодные цены для аренды и большой выбор прогулочных катеров хоть для одного, хоть для большой компании. Посмотрите все наши катера и яхты на сайте по выгодным ценам!
elonbet casino BD
Glory Casino app
elonbet casino
https://orgnaztech.mirtesen.ru/blog/43202871346/Gde-Kazhdyiy-Gost-Osobennyiy-Otel-Glory
Visit https://gorillacasino-best.com/ we have created a fun, informative and engaging online space where casino lovers, newbies and industry experts can find honest insights, in-depth analysis and valuable strategies in the world of online gambling. Unlike many casino blogs, we are not owned or operated by any gambling company. We provide unbiased content.
clenbuterol for sale anabolshop.org
анализ на глисты купить купить анализы для бассейна
elonbet casino BD
джили ситирей купить в спб [url=https://geely-v-spb1.ru/models/cityray/]джили ситирей купить в спб[/url] .
elonbet casino
займ под птс
avtolombard-pid-zalog-pts.ru/ekb.html
деньги в долг под залог машины
A virtual number is a phone number that is not tied to a specific SIM card and does not require a physical device to operate https://novosti.eu.com/virtualnyj-nomer-dlya-telegram.html
elonbet casino
доставка курицы гриль, купить шашлык
автоматизация бизнес-процессов
Glory Casino app
https://orgnaztech.mirtesen.ru/blog/43202871346/Gde-Kazhdyiy-Gost-Osobennyiy-Otel-Glory
займ денег под залог автомобиля
avtolombard-pid-zalog-pts.ru/kemerovo.html
кредит под залог авто в кемерово
шашлык куриный доставка шашлыка
Топ сайтов кейсов CS2 ggdrop.cs2-case org проверенные сервисы с высоким шансом дропа, промокодами и моментальными выводами. Только актуальные и безопасные платформы!
Землетрясение в Бангкоке: что стало причиной?
https://x.com/SebiBilalova/status/1905743685712855518
https://orgnaztech.mirtesen.ru/blog/43202871346/Gde-Kazhdyiy-Gost-Osobennyiy-Otel-Glory
https://qrkoder.ru/
Посетите [url=https://telegra.ph/Itogi-peregovorov-SSHA-i-Rossii-v-EHr-Riyade—poslednie-novosti-03-27]последние новости СВО[/url], чтобы узнать, как прошли переговоры, что происходит на Украине и как складывается ситуация с курсом доллара. Мы предлагаем только проверенную информацию.
Glory Casino app
https://orgnaztech.mirtesen.ru/blog/43202871346/Gde-Kazhdyiy-Gost-Osobennyiy-Otel-Glory
залог под птс авто
avtolombard-pid-zalog-pts.ru
займ под залог авто москва
бесплатный онлайн генератор QR кодов
На сайте https://lilibum.ru вы обязательно найдете вторую половинку для того, чтобы пообщаться, завести знакомства, найти человека для любви, серьезных отношений. Здесь ни одна сотня анкет, среди которых вы точно найдете того, кто симпатичен. Сразу напишите ему и пригласите на долгожданное свидание, чтобы пообщаться, узнать о его интересах, а потом, возможно, и создать семью. Постоянно выкладываются новые анкеты для того, чтобы вы обязательно подобрали родственную душу для любых целей. Для доступа ко всем функциям пройдите регистрацию.
продвижение сайтов цена [url=https://prodvizhenie-sajtov-v-moskve221.ru/]продвижение сайтов цена[/url] .
Сервис бесплатных объявлений №1 хорошо зарекомендовал себя, о чем свидетельствуют отзывы. Плюсы: удобный интерфейс, огромная база объявлений, широкий выбор категорий для размещения и поиска предложений. Заходите и регистрируйтесь. Своего покупателя найдите! Ищете подам объявление? Ynla.ru – тут с мошенниками меньше вероятности столкнуться. Несмотря на скромный интерфейс, сайт достаточно функционален. Регистрация пару минут занимает. Наш сервис оптимизирован для смартфонов, планшетов и ПК. Пользуйтесь настоящей доской объявлений!
Заказываю регулярно — всегда безупречно!
заказать цветы томск
На сайте https://doge.coinwatchtoday.ru/ вы узнаете все о криптовалюте Dogecoin (DOGE) – история, новости, торговые идеи, криптобиржи и торговые инструменты, которые позволят вам с выгодой инвестировать или торговать c Dogecoin (DOGE). В отличие от биткоина, DOGE предлагает быстрые и дешевые транзакции, оставаясь при этом удобным средством микроплатежей и благотворительности. Простота и низкие комиссии!
Perena is a cutting-edge blockchain development platform designed to empower decentralized applications (dApps) with scalable and secure solutions. Built for Web3 innovators, Perena blockchain solutions offer seamless integration, high-performance smart contracts, and decentralized infrastructure. Whether you’re building DeFi protocols, NFT marketplaces, or enterprise blockchain applications, Perena provides the tools needed to scale with confidence. https://perena.tech
ВудХаус495 – компания, которая квалифицированные услуги предоставляет. Мы реализовали больше 20 000 строительно-отделочных работ деревянных домов. Гарантируем лучшую цену на рынке. Договор составляем, в котором все моменты детально прописываем. http://woodhouse495.ru – здесь представлена более детальная информация о нас. Применяем исключительно высокого качества материалы. Осуществим поставленные перед нами задачи в сжатые сроки. Предлагаем персональный подход к каждому клиенту. Заполните на ресурсе форму, и мы в ближайшее время вам перезвоним.
Upshift Finance is a next-generation decentralized trading platform designed to provide secure, fast, and efficient crypto transactions. With smart contract automation, low transaction fees, and seamless integration with DeFi protocols, Upshift Finance empowers traders to swap digital assets and execute trades with maximum security. Whether you’re a beginner or an experienced trader, Upshift Finance offers a powerful, transparent, and user-friendly trading ecosystem. https://upshift.ink
На сайте https://lufkad.com/ вы сможете заказать качественные, сертифицированные и оригинальные мансардные окна премиального качества и по привлекательной стоимости. Конструкции LUFKAD созданы по уникальным и инновационным технологиям, потому отличаются безупречным качеством, долгим сроком эксплуатации. LUFKAD считается такой компанией, которая выполняет все необходимые работы и в полном объеме. Многие производственные технологии, которые применяются этой компанией, не имеют аналогов. Заказывайте окна по лучшей стоимости.
кредит под птс машины
avtolombard-pid-zalog-pts.ru/nsk.html
деньги под птс автомобиля новосибирск
UnagiSwap is a cutting-edge decentralized exchange (DEX) that provides fast, secure, and transparent crypto trading. Designed for traders looking to swap digital assets efficiently without intermediaries, UnagiSwap offers low fees, deep liquidity, and seamless smart contract execution. Whether you’re a casual trader or a professional investor, UnagiSwap’s non-custodial platform ensures full control over your assets in a decentralized environment. https://unagiswap.org
На сайте https://koch-market.ru/ ознакомьтесь со всем перечнем услуг, которые предлагает популярный детейлинг центр. К примеру, вы сможете воспользоваться тюнингом фар, брендированием авто, оклейкой антигравийной пленкой, перетяжкой салона, брендированием авто. Все услуги выполняются в минимальные сроки и по доступной стоимости. Оцените результаты работы, изучив портфолио. В блоге находится огромное количество полезных, информативных статей, которые помогут разобраться в вопросе. Обращайтесь в эту компанию и вы, чтобы все услуги были выполнены на должном уровне.
How does it work? A virtual number forwards calls to your primary device, allowing you to communicate as if you were using a regular phone https://tecirus.ru/news-268-kak-sozdat-virtualnyj-nomer-telefona-i-zachem-eto-nugno.html
Entering the Melbet promo code on the official website of the offshore bookmaker Melbet gives the bettor the opportunity to receive additional funds to the deposit game account in the personal account, free bets and some other gifts from the administration of the bookmaker company https://dansermag.com/wp-content/pages/?code_promo_1win.html
Nucleus Earn is revolutionizing DeFi staking and passive income generation by offering secure, high-yield crypto rewards. With smart contract-powered staking pools, Nucleus Earn allows users to earn rewards effortlessly while maintaining full control over their assets. Whether you’re a beginner or an experienced investor, Nucleus Earn’s decentralized staking platform ensures transparency, security, and optimal returns in the fast-growing world of DeFi. https://nucleusearn.org
Сайт https://t.me/azino777_a является официальным каналом популярного онлайн-заведения «Azino 777». Только здесь находятся самые последние новости, а также увлекательные анонсы слотов, турниров. На сайте также публикуются и бонусы, а также промокоды, которые позволят сэкономить. Заходите на этот сайт для того, чтобы получить больше полезной, важной, содержательной информации на данную тему. С этого канала вы сможете сразу перейти на официальный сайт. Теперь все новости из сферы игр находятся в вашем мобильном.
Лучший генератор QR-кодов
Создание QR кодов
Glory Casino app
MetaMask Download is worth it! A secure and efficient wallet for managing digital assets without the need for intermediaries.
деньги в долг под залог авто
zaim-pod-zalog-pts1.ru/ekb.html
кредит под залог авто документы
Создание QR кодов
Лучший генератор QR-кодов
elonbet casino
UpakovkaRus – компания, которая доступной стоимостью и надежностью характеризуется. Изготавливаем большой ассортимент изделий из ПВХ, а также спанбонда. Применяем материалы, отличающиеся отменным качеством. Всегда учитываем любые требования и пожелания клиента. https://upakovkarus.ru – тут примеры готовой продукции представлены. Если не найдете нужный вариант, пришлите вашей модели фото, мы выполним такую же. Вы можете связаться с нами в любое время, контакты на ресурсе указаны. Нашими клиентами мы дорожим. Ждем от вас заявок!
elonbet casino BD
Лучший генератор QR-кодов
Универсальный вариант для обустройства кухонь и ванных комнат – столешницы из искусственного камня. Они долговечны, практичны, отлично выглядят, могут быть интегрированы с раковиной https://vladimir-smi.ru/itemp/7/656157
На сайте https://t.me/official_izzicasino/ представлены новости, которые будут вам интересны. Так, находясь в любом месте, вы получите возможность отслеживать появление новостей, свежих данных, полезной информации, которая вам обязательно пригодится. Здесь всегда публикуются промокоды, акции, которые сделают игру более интересной, увлекательной, динамичной. Важная информация выкладывается здесь регулярно, чтобы вы были в курсе последних событий. Теперь вся ценная информация окажется в одном месте.
https://intekey.ru/
https://intekey.ru/
Флорист подобрал идеальные цветы для зимней сказки
букет невесты
Наша компания https://etbpro.ru/ предлагает комплексные решения для проектирования, монтажа, модернизации и технического обслуживания высокотехнологичных электронных систем: видеонаблюдение, пожарная сигнализация, скуд, электрооборудование, охранная сигнализация и автоматизированные системы управления. ООО ЭТБ Ваш надежный партнер в обеспечении безопасности. Подробнее на сайте.
Glory Casino app
https://dissertation-now.com/article-review/
Non-Gamstop casino platforms are so intuitive—really nice!
uk casino no gamstop
https://orgnaztech.mirtesen.ru/blog/43202871346/Gde-Kazhdyiy-Gost-Osobennyiy-Otel-Glory
Требуется проверенный специалист по электрике? Мы производим различные [url=https://irkelektrik.ru/]работы по электрике[/url] в короткие сроки, качественно и с гарантией!
* Замена электропроводки и автоматов
* Монтаж розеток, выключателей, светильников
* Монтаж электрических щитов
* Подключение бытовой техники
Работаем 24/7 – выезжаем в день обращения! Опытные специалисты, современный инструмент , четкое соблюдение норм безопасности.
[url=https://irkelektrik.ru/]Заказать электрика[/url] прямо сейчас! Исправим любые неполадки с электричеством!
бесплатный онлайн генератор QR кодов
в грядущем году открывает двери ко множеству возможностей для играющих. Используя его, вы можете весьма повысить свои шансы на выигрыш, получать добавочные бонусы и чаще наслаждаться увлекающими играми промокод kent казино при регистрации
The best non Gamstop casino I’ve tried had incredible customer support.
legit non gamstop casinos
Bongs have become an integral part of smoking culture, combining functionality and aesthetics. They not only make the process comfortable, but also allow you to reveal all the nuances of the taste of the mixture бонг для курения
На сайте https://mos-stroi-alians.ru/ уточните телефон компании, чтобы воспользоваться такой нужной услугой, как кровля крыш независимо от сложности. На предприятии трудятся высококлассные, квалифицированные сотрудники, которые справятся с решением вопроса очень быстро, на профессиональном уровне. В работе применяются только лучшие, инновационные материалы, особые технологии для нужного результата. Работы ведутся и в выходной день. На все услуги даются гарантии, потому как компания уверена в их качестве.
Лучшие сайты кейсов https://ggdrop.casecs2.com/ в CS2 – честный дроп, редкие скины и гарантии прозрачности. Сравниваем платформы, бонусы и шансы. Заходи и забирай топовые скины!
https://qrkoder.ru/
На сайте https://mybeterex.com/ вы сможете уточнить всю необходимую информацию по поводу надежной и проверенной компании «BETEREX», которая предлагает полный комплекс услуг, связанных с ведением бизнеса в Китае. У вас появляется возможность заказать доставку грузов, товаров. Кроме того, вы сможете рассчитывать на получение всех необходимых образцов, а также переговоры с другой стороной. Будут решены все вопросы, независимо от сложности. Воспользуйтесь профессиональной консультацией, чтобы уточнить информацию.
кредит под залог автомобиля казань
avtolombard-pid-zalog-pts.ru/kazan.html
автоломбард казань
Лучший генератор QR-кодов
Non-Gamstop casinos give me total freedom—amazing!
non gamstop online casinos
Ищете куда сходить в Ижевске? Посетите сайт https://izhfun.ru/ и вы найдете главные развлечения Ижевска и где отдохнуть. Выбирайте категории: кафе и рестораны, квизы и игры, театры, концерты, другие мероприятия и места и смотрите текущие или будущие события. Выбирайте интересное времяпрепровождение вместе с нами! Самая актуальная информация на нашем сайте как получить незабываемые эмоции!
бесплатный онлайн генератор QR кодов
https://orgnaztech.mirtesen.ru/blog/43202871346/Gde-Kazhdyiy-Gost-Osobennyiy-Otel-Glory
Non Gamstop casinos are perfect for trying new games—sweet!
casino no gamstop uk
Рэпер Паша Техник находится в критическом состоянии в Таиланде
https://x.com/NargisEhme94100/status/1906094610788573414
https://orgnaztech.mirtesen.ru/blog/43202871346/Gde-Kazhdyiy-Gost-Osobennyiy-Otel-Glory
срочный кредит под залог птс
zaim-pod-zalog-pts1.ru/kemerovo.html
деньги под залог авто птс
АнтикорМастер предоставляет профессиональные услуги. Наша политика ценообразования прозрачна. Стараемся осуществить процесс ухода за вашим авто легким и долгосрочным. Запишитесь на диагностику бесплатную, чтобы следить за состоянием вашего покрытия антикоррозийного. https://xn—-7sbbummpeluekfi.xn--p1ai/ – тут выясните, почему выбирают нас. Предлагаем чистку лазерную, которая не оставляя следов, эффективно ржавчину убирает. Предлагаем письменную гарантию до десяти лет на реализованные работы. Оставьте личные контакты на сайте, и мы с вами свяжемся.
Non Gamstop casino no deposit bonus was easy—great start!
non gamstop casinos uk no deposit bonus
Лучший генератор QR-кодов
На сайте https://down-web.ru/ у вас появится возможность скачать ресурс в режиме реального времени. Этот комфортный, надежный сервис поможет максимально оперативно скачать все, что нужно. Все файлы будут загружаться максимально быстро, аккуратно и безопасно. Вы сможете протестировать этот сервис прямо сейчас. Для того чтобы понять, как правильно пользоваться сервисом, почитайте инструкцию. Также вы почитаете и о том, что представляет собой скачивание сайта и для чего нужно его скачивать. Здесь рассматриваются и способы скачивания портала.
автоматизация бизнес-процессов
Non-Gamstop casinos are perfect for chill gaming—nice!
non gamstop boku casino
На сайте https://t.me/rahvalskiy_team уточните то, какие услуги оказываются популярной и проверенной компанией «RAHVALSKIY TEAM», которая предлагает создать уникальный, функциональный сайт. Он будет работать специально для вас и раскрутки бизнеса. В обязательном порядке разрабатывается особая эффективная стратегия. В компании работают проверенные, знающие и квалифицированные сотрудники, которые учитывают потребности всех клиентов. Воспользуйтесь комплексными услугами для организации бизнеса.
Лучший генератор QR-кодов
На сайте https://gk-psk72.ru/ закажите звонок для того, чтобы приобрести ЖБИ от производителя. Прямо сейчас воспользуйтесь возможностью изучить всю продукцию, которая обязательно вам пригодится. А для того, чтобы узнать об особенностях работы предприятия, необходимо изучить портфолио. Компания оказывает услуги в строительной деятельности более 10 лет. Всего в каталоге более чем тысяча наименований. Есть возможность воспользоваться комплексным предложением товаров, услуг. Преимуществом обращения в компанию является и небольшая стоимость.
автоломбард москва птс
zaim-pod-zalog-pts1.ru
займ под залог отечественного авто
автоматизация бизнес-процессов
Неожиданные Tyeala: смешанном.
Tyeala, An Uneasy Truce [url=https://tyeala.com]https://tyeala.com[/url] .
Say hello to AquaSculpt—a game-changer in weight loss! These AquaSculpt capsules use natural AquaSculpt ingredients to shed pounds and boost confidence. No AquaSculpt side effects, just pure AquaSculpt results—see why in AquaSculpt reviews. Learn AquaSculpt how to use and join thousands who love it. AquaSculpt buy today at http://aquasculpt.best
This non Gamstop casino has killer slots—can’t stop!
new casino sites with no gamstop
Glory Casino app
автоматизация бизнес-процессов
Non Gamstop casinos UK are perfect for avoiding self-exclusion limits.
safe non gamstop casinos
Say hello to AquaSculpt—a game-changer in weight loss! These AquaSculpt capsules use natural AquaSculpt ingredients to shed pounds and boost confidence. No AquaSculpt side effects, just pure AquaSculpt results—see why in AquaSculpt reviews. Learn AquaSculpt how to use and join thousands who love it. AquaSculpt buy today at http://aquasculpt.one !
автоломбард под залог автомобиля
zaim-pod-zalog-pts1.ru/nsk.html
кредит залог птс наличные
AquaSculpt weight loss is here to stay! With AquaSculpt capsules, you get fast AquaSculpt results thanks to natural AquaSculpt ingredients. No worries about AquaSculpt side effects—users confirm it in AquaSculpt reviews. Curious AquaSculpt how to use? It’s easy and effective. AquaSculpt where to buy? Visit http://aquasculpt.me and transform your body now!
Glory Casino app
Casino non Gamstop options give me total control over my gaming.
casinos non on gamstop
https://intekey.ru/
Nice Post! Very Very Nice, Thank You
Thimble is considered the most affordable accessory, which is popular among lovers of herbal smoking mixtures гриндер для травы
Struggling to lose weight? AquaSculpt is transforming weight loss with its natural, fast-acting capsules. Packed with proven AquaSculpt ingredients, these capsules burn fat, boost energy, and deliver real AquaSculpt results in weeks. Curious about AquaSculpt reviews? Users love its effectiveness and zero AquaSculpt side effects. Want to know AquaSculpt how to use? It’s simple—take daily and watch the pounds melt away. Ready to try? AquaSculpt buy now at http://aquasculpt.lifestyle and sculpt your dream body today!
Thimble is considered the most affordable accessory, which is popular among lovers of herbal smoking mixtures купить колпак для курения
дорамы онлайн https://dorama2025.store/
На сайте https://t.me/s/official_izzicasino/ регулярно публикуются свежие и актуальные новости, которые касаются известного виртуального заведения «IZZI Casino». На этом канале вы найдете только содержательную, полезную информацию, которая обязательно пригодится, если являетесь фанатом этого игрового клуба. Здесь регулярно выкладываются анонсы турниров, выигрышные бонусы, а также фриспины и многое другое, что сделает игру более зрелищной, увлекательной. Вся информация об игорном заведении теперь в одном месте.
На сайте https://t.me/rahvalskiy_team закажите продвижение своего ресурса как в Нижнем Новгороде, так и во всем мире. Все работы проводятся строго под ключ. За реализацию всех проектов отвечают надежные, проверенные и знающие сотрудники с огромным опытом. Здесь специально для вас разработают комплексную стратегию, которая поможет вашему бизнесу появиться на первых позициях. На этом предприятии получится воспользоваться всеми маркетинговыми инструментами. Если и вы хотите вывести бизнес на другой уровень, то воспользуйтесь услугами предприятия.
https://intekey.ru/
кредит под залог авто
avtolombard-11.ru/ekb.html
автоломбард екатеринбург
https://odessaforum.getbb.ru/viewtopic.php?f=5&t=23978
https://orgnaztech.mirtesen.ru/blog/43202871346/Gde-Kazhdyiy-Gost-Osobennyiy-Otel-Glory
Have A Good Day, Very Positif Post !
Say hello to AquaSculpt—a game-changer in weight loss! These AquaSculpt capsules use natural AquaSculpt ingredients to shed pounds and boost confidence. No AquaSculpt side effects, just pure AquaSculpt results—see why in AquaSculpt reviews. Learn AquaSculpt how to use and join thousands who love it. AquaSculpt buy today at http://aquasculpt.xyz !
Good Post Brother! Nice Nice
http://www.kalyamalya.ru/modules/newbb_plus/viewtopic.php?topic_id=21820&post_id=102055&order=0&viewmode=flat&pid=0&forum=4#102055
Что такое «Порту Пати» в Дубае и почему об этом говорят все
https://x.com/DeyanetKrmv/status/1906296026006220909
сайта продвижение [url=http://puzzleweb.ru/recl3/effjektivnoje-prodvizhjenije-sajtov-v-moskvje-kak-dostich-vysokikh-rjezultatov.php/]http://puzzleweb.ru/recl3/effjektivnoje-prodvizhjenije-sajtov-v-moskvje-kak-dostich-vysokikh-rjezultatov.php/[/url] .
Пояс не перекашивается!
женские костюмы томск
На сайте https://sanobrabotkarf.ru/ закажите консультацию для того, чтобы оформить такую полезную услугу, как профессиональная дезинфекция. В компании применяются только проверенные, надежные препараты, которые считаются полностью безопасными для человека, животного. Работы выполняются лучшими сотрудниками, у которых есть сертификаты на оказание услуг, а также строго в оговоренные сроки. Специально для вас качественное, комплексное обслуживание. Сотрудники применяют инновационные, уникальные технологии для достижения результата.
ломбард займ под птс
avtolombard-11.ru/kazan.html
автомобиль под залог
Фрибет: Что Это Такое и Как Его Использовать?
В мире онлайн-ставок и азартных игр термин “фрибет” стал широко известен и популярен среди игроков. Но что это такое, как работает фрибет и как его можно использовать с максимальной выгодой? В этой статье мы разберем все ключевые аспекты, связанные с фрибетами, чтобы вы могли уверенно применять их в своей игре.
Что такое фрибет?
Фрибет (от англ. “free bet” — бесплатная ставка) — это бонус, который букмекерские конторы или онлайн-казино предоставляют своим пользователям. Это своего рода подарок, позволяющий сделать ставку на спортивное событие или сыграть в игру без использования собственных средств. Если ставка выигрывает, игрок получает выигрыш за вычетом суммы самого фрибета, а если проигрывает — не теряет ничего из своего кошелька.
Фрибеты часто используются букмекерами как маркетинговый инструмент для привлечения новых клиентов или поощрения активных пользователей. Это отличный способ попробовать свои силы в ставках, не рискуя личными деньгами.
Виды фрибетов
Фрибеты могут различаться по условиям предоставления и использования. Вот основные типы, с которыми вы можете столкнуться:
Приветственный фрибет
Этот бонус обычно предлагается новым игрокам при регистрации на сайте букмекера. Например, после создания аккаунта и подтверждения личности вы можете получить фрибет на сумму 500 или 1000 рублей.
Фрибет за депозит
Некоторые букмекеры начисляют бесплатную ставку после пополнения счета на определенную сумму. Например, внеся 1000 рублей, вы можете получить фрибет на такую же сумму.
Фрибет за активность
Постоянным игрокам могут начислять https://www.youtube.com/watch?v=eMVTiWJujqQ за регулярные ставки, участие в акциях или выполнение определенных условий, таких как серия ставок на конкретные события.
Безусловный фрибет
Это редкий, но очень желанный вид бонуса, который не требует выполнения сложных условий. Вы просто получаете фрибет и можете использовать его по своему усмотрению.
Фрибет на день рождения
Многие букмекерские конторы радуют своих клиентов небольшими подарками в виде фрибетов в честь дня рождения.
Как получить фрибет?
Процесс получения фрибета обычно прост и занимает немного времени. Вот стандартные шаги:
Регистрация: Создайте аккаунт на сайте букмекерской конторы.
Верификация: Подтвердите свою личность, загрузив необходимые документы (паспорт или другой ID).
Выполнение условий: Ознакомьтесь с правилами акции — это может быть внесение депозита, ввод промокода или ставка на определенное событие.
Активация: В некоторых случаях нужно активировать фрибет в личном кабинете или через поддержку.
После этого фрибет будет зачислен на ваш бонусный счет, и вы сможете использовать его для ставок.
Как использовать фрибет с умом?
Чтобы извлечь максимум пользы из фрибета, важно учитывать несколько моментов:
Изучите условия: У каждого фрибета есть свои правила. Например, минимальный коэффициент для ставки (обычно от 1.5 до 2.0) или ограничение по видам спорта.
Выбирайте событие с высокой вероятностью: Поскольку это бесплатная ставка, стоит выбрать матч или игру, в исходе которых вы уверены.
Не торопитесь: Фрибеты часто имеют срок действия (от 7 до 30 дней), поэтому используйте их обдуманно, а не на первой попавшейся ставке.
Планируйте вывод: Если ставка выиграла, уточните, можно ли сразу вывести выигрыш или нужно выполнить дополнительные условия (например, отыграть сумму).
Плюсы и минусы фрибетов
Плюсы:
Возможность попробовать ставки без риска для кошелька.
Шанс выиграть реальные деньги с минимальными вложениями.
Отличный бонус для новичков, чтобы освоиться на платформе.
Минусы:
Ограничения по использованию (коэффициенты, события, сроки).
Выигрыш часто требует отыгрыша перед выводом.
Не все фрибеты действительно “бесплатны” — иногда нужен депозит.
Популярные букмекеры с фрибетами
На российском рынке многие букмекерские конторы предлагают фрибеты. Вот несколько примеров:
1xСтавка: До 10 000 рублей для новых игроков.
Фонбет: Фрибет за регистрацию и первый депозит.
Леон: Регулярные акции с бесплатными ставками.
Winline: Один из лидеров по щедрым фрибетам без сложных условий.
Перед регистрацией обязательно проверяйте актуальные условия на сайте букмекера, так как предложения часто обновляются.
Советы для новичков
Сравнивайте предложения: Разные конторы дают разные суммы и условия — выбирайте то, что вам подходит.
Не гонитесь за крупными суммами: Иногда маленький фрибет с простыми условиями выгоднее, чем большой с кучей ограничений.
Читайте отзывы: Узнайте, что говорят другие игроки о выводе выигрышей с фрибетов.
Играйте ответственно: Даже с фрибетом не стоит увлекаться сверх меры — азарт должен приносить удовольствие.
Good Post Bro! Nice Nice, Waiting Your Next Post!
The Rent Killer is the main antagonist from the 2020 slasher film The Rental hire a hitman
AquaSculpt weight loss is here to stay! With AquaSculpt capsules, you get fast AquaSculpt results thanks to natural AquaSculpt ingredients. No worries about AquaSculpt side effects—users confirm it in AquaSculpt reviews. Curious AquaSculpt how to use? It’s easy and effective. AquaSculpt where to buy? Visit http://aquasculpt.lifestyle and transform your body now!
Посетите сайт https://pech.pro/ это магазин печей, каминов и все для отделки бани. Зайдите в каталог и вы найдете существенный выбор – камины, печи, котлы отопительные, дымоходы, печи для дачи и многое другое по самым выгодным ценам. Действует доставка по Ярославлю и всей России. Профессионально осуществляем подключение, монтаж отопительных устройств и их защиты от возгорания.
AquaSculpt weight loss is here to stay! With AquaSculpt capsules, you get fast AquaSculpt results thanks to natural AquaSculpt ingredients. No worries about AquaSculpt side effects—users confirm it in AquaSculpt reviews. Curious AquaSculpt how to use? It’s easy and effective. AquaSculpt where to buy? Visit http://aquasculpt.one and transform your body now!
Бизнес стратегия Управленческие консультации от Светланы Подкладышевой, серийного предпринимателя с более чем 20-летним опытом. Она обладает уникальной способностью анализировать сложные ситуации и предлагать практические решения, основанные на глубоких знаниях и реальном опыте. Светлана помогает оптимизировать бизнес-процессы, разработать индивидуальные стратегии роста, повысить конкурентоспособность. Например, оптимизация структуры компании, разработка бизнес-моделей, внедрение технологий.
раскрутка сайтов [url=http://puzzleweb.ru/recl3/effjektivnoje-prodvizhjenije-sajtov-v-moskvje-kak-dostich-vysokikh-rjezultatov.php/]раскрутка сайтов[/url] .
Компания Мега скупка https://mega-skupka.com/ работает по городу Санкт-Петербурге. Нам можно продать ваши старые и новые гаджеты, а именно смартфоны, ноутбуки, iphone, apple, macbook, игровые Пк, Даем на 25% больше других конкурентов на месте.
На сайте https://boostclicks.ru/ вы сможете ознакомиться с рекомендациями, ценными и важными советами про API интеграцию, скрипты, а также любопытные сервисы из мира трафика. Для того чтобы вам было удобней ориентироваться, все материалы поделены на разделы, что позволит отыскать то, что действительно актуально и интересно в данный момент. Регулярно на портале появляется новый, интересный контент на данную тему. Имеется и список тех статей, которые пользуются особой популярностью. Все они созданы лучшими и высококлассными экспертами с огромным опытом.
кредит под птс авто
avtolombard-11.ru/kemerovo.html
срочный кредит под залог птс
Портал настроим-яндекс-директ.рф предоставляет квалифицированные услуги. Мы глубокими знаниями платформы Яндекс Директ располагаем. Учитываем особенности вашего бизнеса и предлагаем наилучшую стратегию. На длительные взаимоотношения настроены. Ищете настройка яндекс директ под ключ? Xn—–6kcrbecsfrepkel5alfjkq8y.xn--p1ai – здесь представлены отзывы клиентов, ознакомиться с ними можете в любое время. Воспользуйтесь преимуществами с нашей командой ведения контекстной рекламы в Яндекс Директ. Поможем достичь бизнес-целей ваших с наибольшей эффективностью!
https://boilers24.com/ and you will find spare parts for boilers of many brands. Here you can buy boxes and control boards, actuators, gas valves, oil nozzles, ignition electrodes, flame sensors and much more. Check out the catalog with the entire range at competitive prices.
aviator mostbet [url=http://mostbet6006.ru]http://mostbet6006.ru[/url] .
Fasvek кровельные материалы высокого качества предоставляет. Вся продукция имеет детальное описание с указанием технических параметров и характеристик. Прежде чем сделать заказ, ознакомьтесь с ними. Цены доступны каждому. Многие люди остались довольны сотрудничеством с нами. https://fasvek.ru – тут наши работы представлены. Вы узнаете, что дает использование контролита. Сотрудники компании Вектор – настоящие профессионалы своего дела. Если необходима помощь, оставьте на портале ваш контактный номер, и мы в ближайшее время вам перезвоним.
https://prokazan.ru/adverting/view/kitajskoe-anime-vzlet-i-razvitie-animacionnoj-industrii
деньги срочно под залог авто
avtolombard-11.ru
залог под птс авто
На сайте https://t.me/rahvalskiy_team воспользуйтесь возможностью заказать такую популярную услугу, как продвижение в Нижнем Новгороде и по любому другому городу. В компании работают лучшие, проверенные и талантливые специалисты, которые подберут для вас оптимальный вариант для того, чтобы ваш бизнес стал востребованным. Сотрудники изучат рынок, а также подберут наиболее уместную и работающую стратегию, которая обязательно вам подойдет. Воспользуйтесь комплексными рекламными услугами. Они обойдутся недорого.
На сайте https://organ-hall.ru вы сможете получить уникальный опыт, играя в популярное онлайн-заведение «Vodka Casino», которое заполучило огромное количество поклонников благодаря своей честной и прозрачной политике, большому количеству бонусов. Здесь находятся автоматы, промокоды, бонусы для более зрелищной и интересной игры. Этот клуб создан с использованием новых технологий, поэтому предоставляет безупречный уровень сервиса, регулярные выплаты, интересные функции. С этим заведением вы сможете позволить себе больше!
In the grand design of things you get a B+ for hard work. Where you misplaced us ended up being in all the particulars. As they say, the devil is in the details… And that could not be more correct in this article. Having said that, permit me say to you just what did do the job. Your writing is definitely extremely convincing which is possibly the reason why I am taking the effort to comment. I do not really make it a regular habit of doing that. Secondly, even though I can easily see the leaps in reasoning you come up with, I am not certain of just how you appear to unite the ideas which inturn produce the actual conclusion. For the moment I shall subscribe to your point however hope in the near future you link your dots better.
займ залог птс
avtolombard-11.ru/nsk.html
займ под птс спецтехники
Срочно нужны деньги, но боитесь переплат? Решение есть — рейтинг МФО с займом под 0% на 30 дней. Получите [url=https://wikzaim.ru/]онлайн займ на карту[/url] без комиссий и процентов. Всё законно, прозрачно и доступно каждому в России.
[url=https://medicationsmx.top/#]walmart online pharmacy[/url] Patient drug resource. Medication effects explained. mexica online pharmacy
[url=https://medicationsmx.top/#]online pet pharmacy[/url] Medication information here. Medication trends described. online pharmacy forum
рассчитать кредит под залог авто
https://ezworkers.com/employer/avtolombard-zalog-invest2926/
автоломбард кредит под залог птс
[url=https://krakenlink25.info/]onion ссылки kraken[/url] – Как купить на Kraken, Kraken Market отзывы
На сайте https://t.me/azino777_a вы найдете огромное количество свежих, актуальных и содержательных новостей, которые представлены на тему популярного онлайн-заведения «Azino 777» . Его по достоинству оценило огромное количество посетителей, ведь площадка работает максимально честно, уважает своих посетителей, ценит их время. Именно здесь, в первую очередь, появляются интересные, увлекательные новости. Вы узнаете о них первым. На этом канале публикуются промокоды, бонусы для более зрелищной игры.
web siteniz çok güzel başarılarınızın devamını dilerim. makaleler çok hoş sürekli sitenizi ziyaret edeceğim
Букет выше всех похвал! Очень доволен.
гипсофилы цена букета
[url=https://medicationsmx.top/#]online pharmacy tech programs[/url] Get pill details. Detailed pill knowledge. legitimate online pharmacy
[url=https://krakenlink25.info/]Безопасность на darknet[/url] – Кракен доступ через VPN, vpn для безопасности в интернете
Очень советую https://abfgss53sdbkl33.ru/
На сайте https://koch-market.ru/ закажите звонок для того, чтобы воспользоваться услугами детейлинг центра. Есть возможность воспользоваться такими услугами, как оклейка кузова автомобиля, винилография на мотоцикл, тюнинг фар, оклейка антигравийной пленкой. Для того чтобы сориентироваться в выборе, необходимо изучить примеры работ – те проекты, которые реализованы. Также на сайте представлены и содержательные, любопытные статьи на данную тему. Все специалисты, работающие в компании, отличаются большим опытом.
Крайне рекомендую https://abfgss53sdbkl33.ru/
кредит под залог машины птс
https://willingjobs.com/companies/pinecorp6519/
деньги под залог птс екатеринбург круглосуточно
Viziteaza site-ul https://betwave.ro/ ?i vei gasi o lista cu toate cazinourile online din Romania, precum ?i recenzii ale acestora. Va prezentam cele mai bune condi?ii de la cazinou cu retrageri rapide. Va spunem ce bonusuri exista pentru reaprovizionare sau inregistrare. Cum sa alegi cel mai bun cazinou online pentru tine – cite?te pe site!
[url=https://medicationsmx.top/#]online pharmacy xanax[/url] Drug trends described. Pill details provided. indian pharmacy online
most bet [url=http://mostbet6006.ru/]http://mostbet6006.ru/[/url] .
шиномонтаж ногинск [url=http://www.hondahybrid.ru/forum/topic/2886-kakie-uslugi-okazyvaet-shinomontazh/]шиномонтаж ногинск[/url] .
https://www.floristic.ru/forum/groups/moskva-d852-rulonnye-shtory-i-zhalyuzi-ot-proizvoditelya.html#gmessage1310
Посетите интернет-магазин sharpsting и оцените разнообразие товаров: от современных гаджетов до полезных аксессуаров. Мы ценим каждого клиента и стремимся предоставить лучший сервис. Присоединяйтесь к числу наших довольных покупателей уже сегодня
[url=https://medicationsmx.top/#]online pharmacy[/url] Patient medicine resource. Comprehensive drug overview. safe online pharmacy
Привет, любитель поиграть в контру и получить за это скинов от Гейба. У тебя есть полны и нвентарь и ты ищешь продать скины с выподом на карту . Читай подборку сайтов которые тебе помогут сделать это мгновенно и с удобным способом вывода для тебя.
http://baikal-biz.ru/forum/viewtopic.php?f=15&t=74481
https://mycofarm.ru/
[url=https://krakenlink25.info]кракен интернет маркет[/url] – гид по Кракен TOR, Kraken безопасность и конфиденциальность
Ваша КИ далека от идеала? Не отчаивайтесь. Есть МФО, которые выдают [url=https://wikzaim.ru/]онлайн займ на карту[/url] до 15 000 рублей даже с просрочками. Мы собрали для вас лучшие предложения — всё прозрачно и с быстрой выплатой.
раскрутка сайта в топ [url=www.seogift.ru/news/press-release/2463-geymifikaciya-v-prodvizhenii-internet-magazinov-kak-vovlekat-klientov-s-pervogo-kasaniya/]www.seogift.ru/news/press-release/2463-geymifikaciya-v-prodvizhenii-internet-magazinov-kak-vovlekat-klientov-s-pervogo-kasaniya/[/url] .
автоломбард в казани под залог
https://endhum.com/profile/leonardoolivar
займ под залог машины
шиномонтаж истра [url=http://www.hondahybrid.ru/forum/topic/2886-kakie-uslugi-okazyvaet-shinomontazh/]шиномонтаж истра[/url] .
дорамы https://www.infpol.ru/262227-kitayskie-doramy/
https://mycofarm.ru/
web siteniz çok güzel başarılarınızın devamını dilerim. makaleler çok hoş sürekli sitenizi ziyaret edeceğim
Good Post ! Have A Nice Day !!!
Great post! Loved the insights, keep it up!
займ залог птс
https://job4thai.com/profile/unabirdwood25
займ под птс
Awesome read! Definitely looking forward to more posts like this!
Продать технику легко, обратитесть в скупку78. Компания https://spbskupka78.ru/ занимает лидирующие позиции по выкупу устройств и техники у населения. В данный момент компания ввела оценку через мессенджеры whats app и телеграмм. Наша компания добавила в прайс оценку таких устройства, как: айфоны от 7 до 16 модели, iPad от 2014 года до 2024 (процессор M4), макбуки от 2012 года до 2024 на процессоре М4, ноутбуки бизнес сегмента и игровые, аудиосистемы, микрофоны, ресиверы и усилители звука, телевизоры и мониторы.
МикоФарм Фунги
Заказала цветы для свадьбы в стиле шебби-шик
купить пионы томск
Really enjoyed this! Thanks for sharing such valuable content!
продвижение сайта в топ [url=https://seogift.ru/news/press-release/2463-geymifikaciya-v-prodvizhenii-internet-magazinov-kak-vovlekat-klientov-s-pervogo-kasaniya/]https://seogift.ru/news/press-release/2463-geymifikaciya-v-prodvizhenii-internet-magazinov-kak-vovlekat-klientov-s-pervogo-kasaniya/[/url] .
Adguard-faq.com предлагает актуальную информацию. Объясним, что такое подписка на Адгуард VPN и как ею управлять. Расскажем, где можно взять промокоды на AdGuard. Работа с Adguard имеет ряд положительных сторон. На сайте рассмотрим самые очевидные. Посмотрите на часто задаваемые вопросы ответы. https://adguard-faq.com – здесь расскажем, зачем нужны официальные сайты-зеркала продуктов AdGuard. Узнаете, как купить продукты экосистемы приватности Адгуард. Ищите отменный блокировщик рекламы? Adguard – прекрасный для вас выбор!
починить стиральную машину ремонт посудомоечных машин в москве
Non-Gamstop casino sites feel so much more relaxed—big thumbs up!
casino no deposit bonus not on gamstop
На сайте https://stakecasino.tech изучите подробную, актуальную информацию, которая касается популярного онлайн-казино «Stake Casino». Это место для любителей азарта, ведь здесь есть еще и букмекерская контора. Каждому игроку предлагается огромное количество интересных возможностей для того, чтобы получить море приятных, положительных эмоций. А заодно вы сможете заработать много денег на все, что пожелаете. Вас порадует понятный, простой интерфейс, а также огромное количество бонусов, безупречная защита.
МикоФарм Фунги
Развитие бизнеса Управленческие консультации от Светланы Подкладышевой, серийного предпринимателя с более чем 20-летним опытом. Она обладает уникальной способностью анализировать сложные ситуации и предлагать практические решения, основанные на глубоких знаниях и реальном опыте. Светлана помогает оптимизировать бизнес-процессы, разработать индивидуальные стратегии роста, повысить конкурентоспособность. Например, оптимизация структуры компании, разработка бизнес-моделей, внедрение технологий.
Если вы столкнулись с алкоголизмом, наркоманией или игроманией, обратитесь к специалистам. Это комплексная проблема. Употребление веществ меняет работу мозга, и при отказе возникает абстинентный синдром. Резкий отказ от употребления может вызвать тяжелый абстинентный синдром. Сильная тяга при абстиненции приводит к рецидивам, когнитивным нарушениям и социальной дезадаптации. В центре “Чистое Завтра” вы получите современное лечение зависимостей с учетом ваших особенностей.
Подробнее тут – [url=https://xn—-7sbb6ahbedrhc1bom2cxf9b.xn--p1ai/]вывод из запоя дешево москва[/url]
На сайте https://xn—-7sbecpcasfm0beeaecirc5b3a2g.kz/ получите расчет на то, во сколько вам обойдется корпоративное обучение. «CASPIAN TRAINING GROUP» более 20 лет оказывает помощь в том, чтобы вы прошли успешное обучение. Для того чтобы получить консультацию, коммерческое предложение, необходимо ввести в специальную форму свои личные данные, телефон, электронную почту. Компания оказывает такие услуги, как: бизнес-тренинги, обучение персонала языкам, бизнес-симуляции. В этой компании ни один десяток предприятий проходит обучение за год.
Great content as always! Keep it coming!
Your site is very nice, the articles are great, I wish you continued success, I love your site very much, I will visit it constantly
кредит под птс авто
https://eleeo-europe.com/employer/avtolombard-zalog-invest9851/
деньги под залог авто в кемерово
На сайте https://play.google.com/store/apps/details?id=com.testme.maketest вы сможете сделать ставки в популярной и надежной БК «Фонбет». Здесь вы сможете получить не только определенную сумму в качестве выигрыша, но и положительные, приятные эмоции от удачно проведенной сделки. В этой БК принимаются ставки на самые разные виды спорта. Особый интерес представляют и киберспортивные мероприятия. Есть возможность пополнить счет как наличными при помощи терминала, так и в режиме реального времени.
Casino non Gamstop sites are packed with wins—awesome!
non gamstop casinos uk pay by phone
Your site is amazing, the articles are great, I will always come to this site and continue to read because you have very nice articles, thank you
Your site is very nice, the articles are great, I wish you continued success, I love your site very much, I will visit it constantly
На сайте https://fguard.ru/ почитайте статьи на самую разную тему. К примеру, кибермошенничество, для каких целей используется сеточка, которая на двери микроволновки. Здесь представлена вся нужная информация о смарт-часах и о том, чем они отличаются от бизнес-браслетов. Для того чтобы подыскать нужную информацию, воспользуйтесь специальным рубрикатором. На сайте вы найдете записи, которые касаются нейросетей, гаджетов. Представлены и интересные рекомендации, которые будут необходимы каждому. Имеются данные и про технологии.
https://mycofarm.ru/
T.me/official_izzicasino станет для вас источником интересных материалов и верным помощником. Мы создали канал IZZI Casino в рекомендационных целях, чтобы поделиться с вами полезной информацией. Скорее к нам присоединяйтесь и в курсе горячих новостей, также выгодных предложений будьте. https://t.me/official_izzicasino/ – канал, который посвящен онлайн-казино Иззи и БК. Всегда своих игроков радуем. Выберите интересующий слот и увлекательной игрой наслаждайтесь. Попытайте счастья в выигрыше крупных призов!
Best non Gamstop casinos offer variety like no other—amazing!
non gamstop curacao casinos
Cevizli su kaçak tespiti Müşteri Memnuniyeti: Müşteri memnuniyetine verdikleri önem bizi çok etkiledi. https://rossaofficial.com/author/kacak/
The CoinsBar resource offers one of the most favorable exchange rates on the market обмен криптовалют Киев
https://mycofarm.ru/
На сайте https://smartflow.ru вы сможете выбрать качественную, функциональную сантехнику для обустройства ванной комнаты в доме либо квартире. Ассортимент «SMARTFLOW» включает в себя такие изделия, которые выполнены из высококачественных и современных материалов, безупречного стиля. Все унитазы, биде, модульные ванные идеально впишутся в концепцию. Каждая вещь идеально сочетает надежность, прочность, а также изысканность. Для того чтобы выбрать что-то определенное, изучите галерею, ознакомьтесь и с особой системой смыва «Торнадо».
On the website https://za-forex.com/ you will find information about blockchain technology. The blog of an experienced mathematician, the one who accepted bitcoin and blockchain technology, realizing the potential of digital assets long before mainstream investors paid attention to it. He shares his knowledge to develop automated trading algorithms and risk models that can bring you profit.
кредит в залог автомобиля
https://jobs.fabumama.com/employer/munianiagencyltd8881/
займ залог птс
Оперативное лечение на дому имеет ряд значимых преимуществ, особенно когда каждая минута имеет значение:
Детальнее – https://narco-vivod-clean.ru/vyzov-narkologa-na-dom-spb/
Non-Gamstop casinos are my escape—highly recommend!
no deposit casinos not on gamstop
Visit https://bestcasinoideal.com/ and discover expert reviews, top strategies and essential tips. Explore online slots, table games, live dealer experiences. We have compiled a complete collection of the best online casinos and gambling sites for 2025, tested and rated by experts.
Лаборатория умной нейросети – это Chat-gptru. Загрузите фото, и ИИ быстро сможет определить, что изображено на ней, также предоставит рекомендации или же пояснения. GPT быстро подбирает необходимую информацию из актуальных источников. https://chat-gptru.com – тут с мнениями наших пользователей можете ознакомиться. Также тут цены указаны. Для применения нейросети нужно в чат ввести ваш запрос и отправить нажать. Chat GPT генерирует тексты для любых целей, придерживается высоких стандартов безопасности и конфиденциальности.
https://mycofarm.ru/
Non Gamstop casinos UK are perfect for chill gaming—nice!
welsh non gamstop casinos
кредит под птс авто
https://firstcanadajobs.ca/employer/zaim-pod-zalog-pts-19464/
займ под птс москва
МикоФарм Фунги
Non Gamstop casino UK payouts are so fast—really cool!
non gamstop casinos 10 deposit
МикоФарм Фунги
кредит под птс авто
https://nsproservices.co.uk/employer/thunder-consulting5994/
взять кредит под птс
Non Gamstop casino UK made my day with a quick withdrawal—nice!
non gamstop online casino
В этом интересном тексте собраны обширные сведения, которые помогут вам понять различные аспекты обсуждаемой темы. Мы разбираем детали и факты, делая акцент на важности каждого элемента. Не упустите возможность расширить свои знания и взглянуть на мир по-новому!
Получить дополнительные сведения – https://stjosephmatignon.fr/carnaval-5
МикоФарм Фунги
Best non Gamstop casinos always deliver—love the freedom!
casino not on gamstop 2021 no deposit bonus
https://boi.instgame.pro/forum/index.php?topic=69077.0
Your site is very nice, the articles are great, I wish you continued success, I love your site very much, I will visit it constantly
Your site is very nice, the articles are great, I wish you continued success, I love your site very much, I will visit it constantly
автоломбард под птс
https://jobs.alibeyk.com/employer/avtolombard-pid-zalog-pts4142/
взять кредит под залог машины
Your site is very nice, the articles are great, I wish you continued success, I love your site very much, I will visit it constantly
Your site is very nice, the articles are great, I wish you continued success, I love your site very much, I will visit it constantly
Your site is very nice, the articles are great, I wish you continued success, I love your site very much, I will visit it constantly
Your site is very nice, the articles are great, I wish you continued success, I love your site very much, I will visit it constantly
Your site is very nice, the articles are great, I wish you continued success, I love your site very much, I will visit it constantly
Your site is very nice, the articles are great, I wish you continued success, I love your site very much, I will visit it constantly
Your site is very nice, the articles are great, I wish you continued success, I love your site very much, I will visit it constantly
Your site is very nice, the articles are great, I wish you continued success, I love your site very much, I will visit it constantly
МикоФарм Фунги
Great post! Love the insights, really made me think. Keep it up!
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наша продукция:
Нанокристаллический тигель из высокочистого нитрида бора
http://gazeta.ekafe.ru/viewtopic.php?f=110&t=38996
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с высоким уровнем сервиса.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – наилучшее решение для вашего бизнеса.
Наши товары:
Медное двухраструбное колено под пайку 180 градусов 35х29х1 мм 10х12 мм твердая пайка М1 ГОСТ 32590-2013 Купите надежные медные двухраструбные колена под пайку от ведущего производителя. Обеспечьте эффективное отопление и водоснабжение с прочными и устойчивыми к воздействию внешних факторов коленами из меди.
Enter the 1xBet promo code: 1XWAP. In the special field during registration, enter your unique promo code. Confirm activation https://chatterchat.com/read-blog/58534
Кладбище в Видном http://vidnovskoe.ru актуальные данные о захоронениях, помощь в организации похорон, услуги по благоустройству могил. Схема проезда, часы работы и контактная информация.
Подошло для презентаций – смотрится дорого!
женский костюм купить томск
РедМетСплав предлагает обширный выбор отборных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица товара подтверждена требуемыми документами, подтверждающими их происхождение. Дружелюбная помощь – наш стандарт – мы на связи, чтобы ответить на ваши вопросы а также предоставлять решения под специфику вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
Порошок магниевый G-Z6Th2Zr – AFNOR NF A57-704 Рзделия РёР· магния G-Z6Th2Zr – AFNOR NF A57-704 являются высококачественными компонентами для различных отраслей. Ртот сплав магния, обладающий отличными физическими Рё механическими свойствами, находит применение РІ аэрокосмической, автомобильной Рё производственной сферах. Высокая прочность Рё легкость делают его идеальным выбором для критически важных деталей, требующих надежности. Если РІС‹ ищете инновационные решения, обратите внимание РЅР° наш РїСЂРѕРґСѓРєС‚. Вам стоит купить Рзделия РёР· магния G-Z6Th2Zr – AFNOR NF A57-704, чтобы повысить эффективность ваших проектов.
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наши товары:
Полоса РёР· платины Рё ее сплавов 0.1 РјРј ПлПд-15 ГОСТ 24718-81 Рзучите разнообразие полос РёР· платины Рё ее сплавов РЅР° сайте Редметсплав.СЂС„. Наши высококачественные материалы обладают высокой прочностью, устойчивостью Рє РєРѕСЂСЂРѕР·РёРё Рё непревзойденными техническими характеристиками. Полосы РёР· платины Рё ее сплавов применяются РІ ювелирном деле, медицине Рё производстве специальных деталей, гарантируя надежность Рё качество продукции.
На сайте https://pilmi.ru вы найдете огромное количество фильмов самых разных жанров, включая комедии, мелодрамы, драмы, романтические. Для того чтобы подыскать наиболее подходящий вариант, нужно воспользоваться специальным каталогом. Вашему вниманию представлены и ожидаемые любопытные новинки этого года, которые произведут эффект. Все это можно просматривать в любое время и на различных устройствах. Прямо сейчас воспользуйтесь возможностью посмотреть азиатское кино. Используйте поиск для облегчения выбора.
Автосервис в СПб СТО Приморский предлагает лояльную стоимость на все услуги. Нашей основной целью является обеспечение вашего уюта и уверенности на дороге. Применяем высокого качества запчасти и новейшее оборудование. Запишитесь уже сегодня на ремонт автомобиля! https://sto812.com – тут есть возможность детальнее с условиями оплаты ознакомиться. Доверьте нашим специалистам свою машину. Мы даем гарантию на компетентный подход, надежность и быстрое решение любых задач. С выбором запчастей мы поможем вам. Обращайтесь к нам и не пожалеете об этом!
https://wowlol.ru/forum/showthread.php?p=77714
Awesome read! Very informative and well-written. Looking forward to more!
автоломбард займ под залог птс
https://sahlajobs.com/employer/healthcarejob5972/
кредит под залог птс грузового
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
Обладая обширной линейкой редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым текущие трудности превращаются в завтрашние возможности.
Наши товары:
Титановая плоская мишень
МикоФарм Фунги
Really enjoyed this post! Thanks for sharing such valuable info!
РедМетСплав предлагает обширный выбор высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до масштабных поставок, мы обеспечиваем оперативное исполнение вашего заказа.
Каждая единица продукции подтверждена соответствующими документами, подтверждающими их происхождение. Дружелюбная помощь – то, чем мы гордимся – мы на связи, чтобы разрешать ваши вопросы а также находить ответы под особенности вашего бизнеса.
Доверьте вашу потребность в редких металлах специалистам РедМетСплав и убедитесь в гибкости нашего предложения
поставляемая продукция:
Порошок висмутовый Roto158F Порошок висмутовый Roto158F – высококачественный РїСЂРѕРґСѓРєС‚, идеально подходящий для профессионального применения. Ртот порошок обладает уникальными свойствами, которые делают его незаменимым РІ различных отраслях. Большое внимание уделено его чистоте Рё стабильности, что гарантирует надежный результат РІ процессе использования.Порошок висмутовый Roto158F отличается отличной растворимостью Рё малой токсичностью, что делает его безопасным для Р·РґРѕСЂРѕРІСЊСЏ. Р’С‹ можете использовать его РІ медицине, С…РёРјРёРё или для создания уникальных материалов. Убедитесь РІ высоком качестве нашей продукции, Рё РІС‹ РЅРµ пожалеете Рѕ своем выборе.РќРµ упустите возможность купить Порошок висмутовый Roto158F Рё получите надежный Рё безопасный РїСЂРѕРґСѓРєС‚, соответствующий всем современным стандартам.
Your site is very nice, the articles are great, I wish you continued success, I love your site very much, I will visit it constantly
дренажная сливная система [url=www.drenazh-uchastka-krasnodar.ru]www.drenazh-uchastka-krasnodar.ru[/url] .
Your site is very nice, the articles are great, I wish you continued success, I love your site very much, I will visit it constantly
Электросчетчики с пультом Приборы учета с дистанционным управлением, электросчетчики, оснащенные пультом, устройства для измерения потребления электроэнергии с возможностью удаленного контроля, экономичные модели счетчиков, модифицированные приборы учета, разнообразные счетчики, газовые счетчики, подвергшиеся воздействию магнитом или пленкой, способы остановки работы счетчика, приобретение счетчика с пультом дистанционного управления, покупка электросчетчика с пультом.
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с высоким уровнем сервиса.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наши товары:
Прецизионная сварная холоднотянутая труба 120С…2 РјРј E195 EN 10305-2 Покупайте прецизионные сварные холоднотянутые трубы РѕС‚ лучшего производителя. РЁРёСЂРѕРєРёР№ выбор размеров Рё высокое качество. Рдеально РїРѕРґС…РѕРґСЏС‚ для автомобильного производства Рё машиностроения.
План эвакуации – это схема размещения первичных средств пожаротушения, а также указывающие пути эвакуации. Разработку такого документа следует доверять только профессионалам Заказать план эвакуации
https://topsale.md/ – ваш надежный онлайн-гипермаркет в Молдове! Здесь вы найдете бытовую технику, электронику, товары для дома, детей и животных по выгодным ценам. Быстрая доставка по всей стране, удобные способы оплаты и акции для покупателей! Покупайте легко, экономьте время и деньги с TOPSALE.MD – ассортимент, который вам нужен, в одном месте!
New to leonbet?
Get Your $100 Bonus Just for Signing Up!
На сайте https://antipoligraf.ru/ почитайте про Институт прикладной психофизиологии. Здесь ведется профессиональная, комплексная подготовка к тому, чтобы пройти полиграф. Учат и тому, чтобы быстро, безопасно и комплексно снять стресс при помощи уникальных и инновационных приборов обратной связи. И самое важное, что все это абсолютно безопасно, при этом гарантированно поможет. Кроме того, ведется обучение саморегуляции при помощи обратной связи. У института огромное количество партнеров, которые стараются работать в комплексе.
На сайте https://astro-magic.com/ воспользуйтесь искусственным интеллектом на русском языке. Этот сервис предоставляет возможность использовать все функции в режиме реального времени. При этом нет необходимости подключать VPN, искусственный интеллект работает и без него. Воспользоваться им сможет каждый желающий, абсолютно бесплатно. Chat GPT сможет создать качественный контент, произвести анализ изображений, быстро отыскать нужную информацию. Искусственный интеллект сможет и написать программный код.
Welcome to the official Joy Casino blog https://officialjoycasino.net/ — a place where you can explore the fascinating world of online gambling from different perspectives: game mechanics, psychology, responsible gaming and industry analytics. If you are looking for a deep dive into gaming strategies, casino technology or responsible gaming tips, you have come to the right place.
На сайте https://pohudetlegko.ru/ представлена исчерпывающая, актуальная и полезная информация о том, как похудеть правильно, без вреда для организма и максимально эффективно. На сайте вы найдете вдохновляющие истории, советы звезд, которые выглядят младше своего возраста. Есть рецепты блюд, которые созданы из полезных, вкусных ингредиентов. Но при этом они помогут скинуть вес и привести себя в форму к летнему сезону. Рецепты простые и быстрые, а потому справиться с приготовлением пищи сможет каждый. Описаны и упражнения, обертывания для похудения.
МикоФарм Фунги
На сайте https://xn—-8sbaaajsa0adcpbfha1bdjf8bh5bh7f.xn--p1ai/ ознакомьтесь со всеми вариантами антиквариата, который вы сможете продать по честной стоимости. Станислав Бабкин, являясь частным коллекционером, скупает предметы старины дорого. Оценка происходит в режиме реального времени, а средства выплачиваются на карту либо выдаются наличными. Вы сможете продать следующие вещи: серебро, картины, иконы, золотые, серебряные монеты, ордена, часы, самовары и многое другое. Вся сумма выплачивается полностью во время оформления сделки.
План эвакуации – пожарная схема, указывающая места размещения огнетушителей и аукционных выходов. Если требуется купить план эвакуации, то только в ООО «ФАЕР-АКС» Заказать план эвакуации
спутниковый мониторинг автомобилей [url=kontrol-avto.ru]kontrol-avto.ru[/url] .
Что такое “Кейсы КС” и “Кейсы КС ГО”?
“Кейсы КС” (или “Кейсы CS”) и “Кейсы КС ГО” (CS:GO) — это виртуальные контейнеры, которые используются в играх Counter-Strike (КС), включая Counter-Strike: Global Offensive (CS:GO) и его обновленную версию Counter-Strike 2 (CS2). Внутри этих кейсов находятся различные игровые предметы — скины для оружия, ножи, перчатки и другие косметические элементы, которые игроки могут получить и использовать в игре для украшения своего инвентаря.
Как это работает?
1. **Регистрация**: Вы заходите на сайт и регистрируетесь, обычно через Steam, чтобы связать свой игровой аккаунт.
2. **Пополнение баланса**: Нужно внести деньги на сайт (например, через карту, криптовалюту или электронные кошельки), чтобы купить кейсы или внутриигровую валюту.
3. **Выбор кейса**: На сайте представлены разные кейсы с указанием возможных наград (от дешевых скинов до редких ножей) и их стоимости.
4. **Открытие кейса**: Вы выбираете кейс, оплачиваете его и открываете. Система случайным образом определяет, какой предмет вы получите.
5. **Вывод выигрыша**: Если вам повезло, вы можете вывести скин в Steam и использовать его в игре.
Чем отличается “Кейсы КС” от “Кейсы КС ГО”?
– **Кейсы КС**: Общий термин, который может относиться к кейсам в любой версии Counter-Strike, включая CS2.
– **Кейсы КС ГО**: Конкретно кейсы из CS:GO, которые были популярны до выхода CS2. Теперь многие платформы, адаптируют их под CS2, но скины из CS:GO все еще совместимы с новой игрой.
Если ты хочешь попробовать, начни с малого — внеси небольшую сумму и протестируй, как все работает. Например, выбери дешевый кейс и посмотри, что выпадет. Это поможет понять механику без больших рисков.
https://wiki.asexuality.org/w/index.php?title=User_talk:Dorie02K426
Bonuses and promotions in the bookmaker 1xBet are a great opportunity to get additional benefits from your bets. The showcase of promo codes can offer a variety of promo codes that can be exchanged for prizes or free bets in the game http://cozy.moibb.ru/viewtopic.php?f=31&t=11458
Что такое “Кейсы КС” и “Кейсы КС ГО”?
“Кейсы КС” (или “Кейсы CS”) и “Кейсы КС ГО” (CS:GO) — это виртуальные контейнеры, которые используются в играх Counter-Strike (КС), включая Counter-Strike: Global Offensive (CS:GO) и его обновленную версию Counter-Strike 2 (CS2). Внутри этих кейсов находятся различные игровые предметы — скины для оружия, ножи, перчатки и другие косметические элементы, которые игроки могут получить и использовать в игре для украшения своего инвентаря.
Как это работает?
1. **Регистрация**: Вы заходите на сайт и регистрируетесь, обычно через Steam, чтобы связать свой игровой аккаунт.
2. **Пополнение баланса**: Нужно внести деньги на сайт (например, через карту, криптовалюту или электронные кошельки), чтобы купить кейсы или внутриигровую валюту.
3. **Выбор кейса**: На сайте представлены разные кейсы с указанием возможных наград (от дешевых скинов до редких ножей) и их стоимости.
4. **Открытие кейса**: Вы выбираете кейс, оплачиваете его и открываете. Система случайным образом определяет, какой предмет вы получите.
5. **Вывод выигрыша**: Если вам повезло, вы можете вывести скин в Steam и использовать его в игре.
Чем отличается “Кейсы КС” от “Кейсы КС ГО”?
– **Кейсы КС**: Общий термин, который может относиться к кейсам в любой версии Counter-Strike, включая CS2.
– **Кейсы КС ГО**: Конкретно кейсы из CS:GO, которые были популярны до выхода CS2. Теперь многие платформы, адаптируют их под CS2, но скины из CS:GO все еще совместимы с новой игрой.
Если ты хочешь попробовать, начни с малого — внеси небольшую сумму и протестируй, как все работает. Например, выбери дешевый кейс и посмотри, что выпадет. Это поможет понять механику без больших рисков.
https://cameradb.review/wiki/User:AnastasiaJacobse
мостбет авиатор [url=http://mostbet6007.ru]http://mostbet6007.ru[/url] .
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наши товары:
Плоская мишень AlN для распыления
дренажные системы водоотвода [url=https://www.drenazh-uchastka-rostov.ru]дренажные системы водоотвода[/url] .
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
С широким ассортиментом продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наши товары:
Титановая мишень
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наша продукция:
Металлическая мишень РРЅРґРёСЏ 5N плоская для распыления
дренаж участка под ключ [url=https://drenazh-uchastka-krasnodar.ru/]дренаж участка под ключ[/url] .
Аккредитованное агентство https://pravo-migranta.ru/ по аутстаффингу мигрантов и миграционному аутсорсингу. Оформление иностранных сотрудников без рисков. Бесплатная консультация и подбор решений под ваш бизнес.
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
Наши товары:
Однораструбный медный РѕР±РІРѕРґ РїРѕРґ пайку 8С…6С…0.6 РјРј 6.8С…8.8 РјРј мягкая пайка Рњ1 ГОСТ Р 52922-2008 Выбирайте высококачественные медные однораструбные РѕР±РІРѕРґС‹ РїРѕРґ пайку для надежных соединений. Рзготовлены РёР· меди высокой чистоты СЃ отличной теплопроводностью Рё устойчивостью Рє РєРѕСЂСЂРѕР·РёРё. Рдеальны для систем водоснабжения Рё отопления.
РедМетСплав предлагает внушительный каталог высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица продукции подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Дружелюбная помощь – наша визитная карточка – мы на связи, чтобы улаживать ваши вопросы а также предоставлять решения под специфику вашего бизнеса.
Доверьте вашу потребность в редких металлах специалистам РедМетСплав и убедитесь в гибкости нашего предложения
Наши товары:
Лента магниевая G-Zr – AFNOR NF A57-704 РљСЂСѓРі магниевый G-Zr – AFNOR NF A57-704 представляет СЃРѕР±РѕР№ высококачественный инструмент, предназначенный для надежной обработки различных материалов. Рзготовленный РїРѕ строгим стандартам AFNOR, данный РєСЂСѓРі гарантирует исключительную прочность Рё долговечность. РћРЅ идеально РїРѕРґС…РѕРґРёС‚ для выполнения сложных задач РІ мебельной, автомобильной Рё строительной отраслях. Благодаря СЃРІРѕРёРј уникальным характеристикам, РєСЂСѓРі обеспечивает превосходную эффективность Рё высокую скорость работы. РќРµ упустите возможность купить РљСЂСѓРі магниевый G-Zr – AFNOR NF A57-704 РїРѕ привлекательной цене Рё улучшить качество вашего производства.
РедМетСплав предлагает обширный выбор качественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица продукции подтверждена соответствующими документами, подтверждающими их качество. Опытная поддержка – то, чем мы гордимся – мы на связи, чтобы ответить на ваши вопросы и адаптировать решения под специфику вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
Наша продукция:
РџРѕРєРѕРІРєР° висмутовая CAC906 – JIS H 5120-2016 РџРѕРєРѕРІРєР° висмутовая CAC906 – JIS H 5120-2016 представляет СЃРѕР±РѕР№ высококачественный материал, используемый РІ различных областях промышленности. Благодаря своей уникальной структуре Рё качественным характеристикам, РѕРЅР° обеспечивает надежность Рё долговечность РІ эксплуатации. РџРѕРґС…РѕРґРёС‚ для производств, требующих высоких стандартов. Купить РџРѕРєРѕРІРєР° висмутовая CAC906 – JIS H 5120-2016 означает сделать выбор РІ пользу надежного Рё проверенного продукта. Ртот материал поможет вам достичь поставленных целей РІ вашем бизнесе благодаря СЃРІРѕРёРј превосходным особенностям, таким как стабильность Рё отличные механические свойства.
РедМетСплав предлагает широкий ассортимент качественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица изделия подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Превосходное обслуживание – наша визитная карточка – мы на связи, чтобы разрешать ваши вопросы а также находить ответы под специфику вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
Наши товары:
РљСЂСѓРі вольфрамовый РР’Р-2 РљСЂСѓРі вольфрамовый РР’Р-2 — это идеальный инструмент для высококачественной обработки материалов. РћРЅ обеспечивает превосходную прочность Рё долговечность, что делает его незаменимым РІ металлообработке Рё строительстве. Благодаря уникальным свойствам вольфрама, РєСЂСѓРі РїРѕРґС…РѕРґРёС‚ для точной резки Рё шлифовки. Если РІС‹ ищете надежный Рё производительный инструмент, РЅРµ упустите возможность купить РљСЂСѓРі вольфрамовый РР’Р-2. РћРЅ отлично справляется СЃ различными задачами, гарантируя отличный результат. Выберите качество Рё надежность — выбирайте РљСЂСѓРі вольфрамовый РР’Р-2!
МикоФарм Фунги
деньги под залог авто птс
avtolombard-pid-zalog-pts.ru/ekb.html
кредит под залог птс
смотреть здесь https://futbolka-obnal.ru
мобильный мониторинг транспорта [url=kontrol-avto.ru]мобильный мониторинг транспорта[/url] .
With each passing year, the world of targeted games becomes more and more exciting, and online casinos continue to delight their subscribers with new opportunities and promotions https://mamapoltava.listbb.ru/viewtopic.php?f=11&t=13398
На сайте https://nenovost.com/ почитайте любопытные и интересные новости, представленные на самую разную тему: бизнес, автомобили, медицина, кулинария, общество, недвижимость, спецтехника, туризм и отдых. Все статьи составлены специалистами, потому вы можете на них положиться, ведь вся информация является достоверной, качественной. Здесь постоянно публикуются новые материалы на самую разную тему. Все они сопровождаются картинками для большей наглядности. Заходите на портал регулярно, чтобы найти что-то нужное и полезное.
Looking for video about crypto? Youtube.com/@Quickex_io where you will find a video about how the online cryptocurrency exchanger works, how you can connect the exchanger to your site and earn money, as well as many videos related to different cryptocurrencies and their profitable exchange. Find out absolutely everything about the cryptocurrency market in one place.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Гранулы олова высокой чистоты
mostbet apk скачать [url=mostbet6007.ru]mostbet apk скачать[/url] .
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
поставляемая продукция:
Пруток латунный Р›63РїС‚, 7 РјРј, L 3000 РјРј, РєСЂСѓРі Пруток латунный Р›63РїС‚ длиной 3000 РјРј Рё диаметром 7 РјРј – идеальное решение для металлических работ. Высокая прочность, устойчивость Рє РєРѕСЂСЂРѕР·РёРё Рё универсальное применение делают его надежным выбором. Купите пруток латунный РЅР° Редметсплав.СЂС„ Рё обеспечьте отличное качество обработки вашего проекта.
[url=https://maps.google.co.jp/url?sa=t&url=https%3A%2F%2Fedpillrx.top]ed drugs online[/url] Medicine impacts explained. Recent medicine developments. buy ed drugs online
РедМетСплав предлагает внушительный каталог отборных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до масштабных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица продукции подтверждена требуемыми документами, подтверждающими их происхождение. Дружелюбная помощь – наша визитная карточка – мы на связи, чтобы ответить на ваши вопросы по мере того как находить ответы под специфику вашего бизнеса.
Доверьте вашу потребность в редких металлах специалистам РедМетСплав и убедитесь в множестве наших преимуществ
поставляемая продукция:
Лист ванадиевый Р’РЅРђР›-1Р” – РўРЈ 48-4-505-99 Лист ванадиевый Р’РЅРђР›-1Р” – РўРЈ 48-4-505-99 – это высококачественный металл, который обеспечивает отличные механические свойства Рё РєРѕСЂСЂРѕР·РёРѕРЅРЅСѓСЋ стойкость. РћРЅ широко применяется РІ авиастроении, машиностроении Рё РґСЂСѓРіРёС… отраслях, требующих надежных материалов. Ртот лист идеально РїРѕРґС…РѕРґРёС‚ для изготовления деталей, РіРґРµ важны прочность Рё стойкость Рє высоким температурам. Если вам нужен надежный Рё долговечный материал, то РїРѕРєСѓРїРєР° Лист ванадиевый Р’РЅРђР›-1Р” – РўРЈ 48-4-505-99 станет отличным решением. РњС‹ предлагаем доступные цены Рё высокое качество. РќРµ упустите шанс приобрести этот товар!
site web
[url=https://www.brides-from.ru/]brides from ukraine[/url]
https://mycofarm.ru/
find more information
[url=https://www.brides-from.ru/Starheaven.html]Starheaven, bride from russia[/url]
снят квартиру вташкенте Ташкент, современная столица Узбекистана, привлекает своим сочетанием истории и динамичного развития. Для тех, кто планирует остаться здесь на длительный срок, вопрос аренды жилья становится первостепенным. Снять квартиру в Ташкенте – задача, требующая внимательного подхода и понимания местного рынка.
кредит под залог птс автомобиля
avtolombard-pid-zalog-pts.ru/kemerovo.html
займ птс
[url=https://images.google.com.kh/url?q=https%3A%2F%2Fedpillrx.top]buy generic ed drugs[/url] Medication data provided. Get information instantly. buy ed drugs no rx
Extra resources
[url=https://www.brides-from.ru/]russian brides for marriage[/url]
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Обладая обширной линейкой редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Плоская мишень NiFe
На сайте https://face-me.ru/ вы сможете попробовать все возможности нейросетей и всего за несколько секунд превратить любое фото в видео. Инновационные и уникальные технологии дают возможность получить роскошную, яркую и любопытную фотосессию без профессионального оборудования, студии. Вы сможете создать фантастические образы и удивительные портреты в соответствии с предпочтениями. Эта программа дарит вам огромное количество возможностей и вдохновение. Вы каждый раз будете получать удивительные, красивые образы, которые всегда будут с вами.
Официальный сайт 1win https://1win.kykyryza.ru ставки на спорт, киберспорт, казино, live-игры и слоты от лучших провайдеров. Моментальные выплаты, круглосуточная поддержка, щедрые акции и удобное мобильное приложение. Делай ставки и играй в казино на 1win — быстро, безопасно и выгодно!
Swiat emocji z 1win http://www.1win-pl.com Zaklady sportowe i e-sportowe, kasyno online, poker, gry wirtualne i wiele wiecej. Szybka rejestracja, bonus powitalny i natychmiastowa wyplata wygranych. 1win – wszystko, czego potrzebujesz do gry w jednym miejscu!
[b] Portable balancer & Vibration analyzer Balanset-1A [/b]
[b] Description: [/b]
[url=https://allegro.pl/oferta/przenosny-wywazarka-i-analizator-drgan-balanset-1a-kompletny-zestaw-16654413800][img]https://vibromera.eu/wp-content/uploads/2023/09/77-e1693745667801.jpg.webp[/img][/url]
Price: [b]€2399[/b]
[url=https://allegro.pl/oferta/przenosny-wywazarka-i-analizator-drgan-balanset-1a-kompletny-zestaw-16654413800] Order on Allegro [/url]
Save [b]€100[/b] on [url=https://vibromera.eu/shop/874/]vibromera.eu[/url] with promo code [b]VB100![/b]
[b]Overview of the Balanset-1A Device[/b]
The Balanset-1A is a highly portable, dual-channel device tailored for rotor balancing and vibration analysis. It’s ideally suited for balancing rotors like crushers, fans, mulchers, choppers, shafts, centrifuges, turbines, and other rotating machines.
[b]Core Features and Capabilities[/b]
[b]Vibrometer Function[/b]
Tachometer: Precise RPM measurement.
Phase: Identifies the phase angle of vibration signals for accurate analysis.
1x Vibration: Measurement and analysis of the primary frequency component.
FFT Spectrum: Detailed frequency spectrum analysis of the vibration signal.
Overall Vibration: Monitoring the total vibration level.
Measurement Log: Stores data for subsequent analysis.
[b]Balancing Function[/b]
Single-Plane Balancing: Adjusts rotors in one plane to reduce vibration.
Two-Plane Balancing: Dynamic rotor balancing in two planes.
Polar Diagram: Represents imbalance on a polar chart for precise correction weight placement.
Last Session Recovery: Allows resuming the previous balancing session.
Tolerance Calculator (ISO 1940): Calculates allowable imbalance according to ISO 1940 standards.
Grinding Wheel Balancing: Corrects grinding wheel imbalance with three counterweights.
[b]Visualizations and Diagrams[/b]
Overall Graphs: Displays general vibration levels.
1x Graphs: Represents vibration behavior at the main frequency.
Harmonic Graphs: Analyzes the presence and impact of harmonic frequencies.
Spectral Graphs: Provides a detailed view of the frequency spectrum for analysis.
[b]Extra Features[/b]
Archive: Store and revisit past balancing data.
Reports: Produce detailed reports on balancing results.
Rebalancing: Enables rebalancing using stored session data.
Serial Production Balancing: Perfect for mass production rotor balancing.
[b]Package Contents[/b]
The Balanset-1A package includes:
A measurement block with an interface.
Two accelerometers.
Optical sensor (laser tachometer) with magnetic mount.
Electronic balance scales.
Software (Note: Laptop not included, available as an additional order).
Protective transport case.
Price: [b]€2399[/b]
[url=https://allegro.pl/oferta/przenosny-wywazarka-i-analizator-drgan-balanset-1a-kompletny-zestaw-16654413800] Order on Allegro [/url]
Or get a [b]€100[/b] discount on the official website [url=https://vibromera.eu/shop/874/]vibromera.eu[/url] with promo code [b]VB100[/b]
Цветы пришли с открыткой и маленьким подарочком – трогательно!
заказать цветы томск
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наши товары:
Плоская мишень из германия 5N, 6N
РедМетСплав предлагает внушительный каталог высококачественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до масштабных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица изделия подтверждена соответствующими документами, подтверждающими их соответствие стандартам. Дружелюбная помощь – то, чем мы гордимся – мы на связи, чтобы ответить на ваши вопросы по мере того как предоставлять решения под требования вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
поставляемая продукция:
Лист ниобиевый РќР±Рџ-1Р° – ГОСТ 26252-84 Лист ниобиевый РќР±Рџ-1Р° – ГОСТ 26252-84 представляет СЃРѕР±РѕР№ высококачественный металл, который используется РІ различных отраслях, включая атомную, химическую Рё авиационную промышленности. РџРѕРґС…РѕРґРёС‚ для производства деталей Рё конструкций, работающих РІ условиях высокой температуры Рё РєРѕСЂСЂРѕР·РёРё. Ртот РїСЂРѕРґСѓРєС‚ отличается отличной прочностью Рё легкостью обработки. Если РІС‹ ищете надежный Рё долговечный материал, который соответствует стандартам качества, РЅРµ упустите шанс купить Лист ниобиевый РќР±Рџ-1Р° – ГОСТ 26252-84. РњС‹ гарантируем высокое качество Рё быструю доставку.
МикоФарм Фунги
содержание https://skam-futbolka.ru
[url=https://www.google.com.bd/url?sa=t&url=https%3A%2F%2Fedpillrx.top]buy ed drugs uk[/url] Medication resource here. Drug information available. ed drugs
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с прекрасным качеством обслуживания.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – наилучшее решение для вашего бизнеса.
поставляемая продукция:
Лента РёР· прецизионных сплавов для СѓРїСЂСѓРіРёС… элементов 0.5×63 РјРј 40РљРҐРќРњ ГОСТ 14117-85 Купите ленту РёР· прецизионных сплавов для СѓРїСЂСѓРіРёС… элементов РїРѕ выгодной цене РЅР° Редметсплав.СЂС„. Высокая прочность, устойчивость Рє РєРѕСЂСЂРѕР·РёРё Рё отличная эластичность делают этот материал идеальным для различных отраслей. Предлагаем широкий ассортимент продукции Рё РїРѕРґСЂРѕР±РЅСѓСЋ информацию для правильного выбора.
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с прекрасным качеством обслуживания.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – наилучшее решение для вашего бизнеса.
Наша продукция:
РўСЂСѓР±Р° профильная нержавеющая 30x30x2 РјРј AISI 304 шлифованная РЁРёСЂРѕРєРёР№ выбор нержавеющих профильных труб 30x30x1.2 РјРј AISI 304 матовых. Рдеальное решение для строительных Рё дизайнерских проектов. Купить высококачественные трубы РїРѕ выгодной цене РЅР° Редметсплав.СЂС„.
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – наилучшее решение для вашего бизнеса.
поставляемая продукция:
Рнструментальная квадратная РїРѕРєРѕРІРєР° 75 РјРј 7РҐР“2Р’РњР¤ ГОСТ 1133-71 Познакомьтесь СЃ широким ассортиментом высокопрочных инструментов для обработки металла РІ категории инструментальной квадратной РїРѕРєРѕРІРєРё РѕС‚ Редметсплав. Выбирайте РёР· прочных Рё надежных материалов, подобранных специально для различных РІРёРґРѕРІ металлообработки. Гарантированное качество РѕС‚ ведущих производителей.
РедМетСплав предлагает внушительный каталог высококачественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица продукции подтверждена соответствующими документами, подтверждающими их качество. Опытная поддержка – наш стандарт – мы на связи, чтобы разрешать ваши вопросы а также предоставлять решения под специфику вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в множестве наших преимуществ
Наша продукция:
РљСЂСѓРі магниевый 25 – ISO 503 Фольга магниевая 25 – ISO 503 используется РІ различных отраслях для защиты материалов РѕС‚ РєРѕСЂСЂРѕР·РёРё Рё РІ качестве теплоизоляции. Рта фольга обеспечит отличную прочность Рё долговечность, что делает ее идеальным выбором для промышленных Рё строительных РЅСѓР¶Рґ. Ее легкость Рё гибкость позволяют легко обрабатывать Рё устанавливать. Если РІС‹ ищете надежное решение для СЃРІРѕРёС… проектов, РЅРµ упустите возможность купить Фольга магниевая 25 – ISO 503. Рта фольга отлично подойдет для тепловой защиты Рё устойчивости Рє атмосферным воздействиям, обеспечивая высокое качество Рё эффективность.
взять кредит залогом авто
avtolombard-pid-zalog-pts.ru
взять кредит под залог птс
Ты игрок в CS2 или КС ГО? Если тебе надоели твои обыные скины и ты хочешь продать их и вывести деньги себе на карту, то для этого существуют специальные сайты, которые помогают с этим. ТУТ: вывод свкинов в деньги кс ты сможешь реализовать свои скины вывести деньги на карту в течение 10 минут.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Плоская мишень AlSc для распыления сплавов
[url=https://cse.google.ws/url?sa=t&url=https%3A%2F%2Fedpillrx.top]buy ed drugs online without prescription[/url] Latest pill news. Comprehensive drug resource. buy ed drugs
https://mycofarm.ru/
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
поставляемая продукция:
Лист судостроительный 45x1000x2500 A36 EN10025:3 Приобретите высококачественные листы судостроительные от компании Редметсплав.рф для надежного и долговечного использования в вашем судостроительном проекте. Различные размеры и толщины, прочность и устойчивость к коррозии делают эти листы идеальным выбором для любого судостроительного проекта.
РедМетСплав предлагает обширный выбор высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица продукции подтверждена соответствующими документами, подтверждающими их происхождение. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы ответить на ваши вопросы а также находить ответы под требования вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в множестве наших преимуществ
Наша продукция:
Лист ванадиевый A 102 – ASTM A102 Лист ванадиевый A 102 – ASTM A102 — это высококачественный металл, обладающий отличной прочностью Рё устойчивостью Рє РєРѕСЂСЂРѕР·РёРё. РћРЅ широко используется РІ различных промышленных секторах, включая машиностроение Рё строительство. Благодаря СЃРІРѕРёРј уникальным характеристикам, данный лист идеально РїРѕРґС…РѕРґРёС‚ для создания деталей, работающих РІ условиях высоких нагрузок. Если РІС‹ ищете надежный Рё долговечный материал, рекомендуем купить Лист ванадиевый A 102 – ASTM A102. Закажите его сегодня Рё обеспечьте своему проекту высокую степень надежности Рё эффективности.
A Cat Casino promo code is a special code that allows participants to receive additional bonuses. This can be a free bet, additional free spins, a deposit increase or even cashback https://pp.build2.ru/viewtopic.php?id=7523#p20689
http://zsgipn.ru/
Спецкор Дніпро: Новини міста Дніпра і Дніпропетровської області – https://spetskor.dp.ua/ . Актуальні новини Дніпра і Дніпропетровської області. Довідкова, та корисна інформація про місто.
займ под залог авто круглосуточно
avtolombard-pid-zalog-pts.ru/nsk.html
деньги под залог автомобиля авто остается
indo-bet
[url=https://www.google.com.tj/url?q=https%3A%2F%2Fedpillrx.top]purchase ed drugs[/url] Generic names listed. Pill guide available. buy ed drugs uk
https://mycofarm.ru/
На сайте https://italsvet.ru/shop/CID_1.html изучите большой выбор изысканных и роскошных люстр популярной марки «ARTBRONZE». Это оптимальное решение для тех, кто хочет ежедневно наслаждаться красивым стилем, дизайном, а также привлекательным, теплым и приятным светом. Каждое изделие выполнено в особом и уникальном стиле, а потому обязательно впишется в любую концепцию. Все изделия произведены из практичных и надежных материалов, поэтому не потеряют своей привлекательности. Приобрести люстру получится в пару кликов.
https://gvis.tramandai.rs.gov.br/web-console/news/?id=kelana-bet
bima-bet-slot
bazooka-bet
soccer-bet
football-bet
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние достижения.
Наша продукция:
Плоская мишень магниевая
На сайте https://autopiter.kg/ получится приобрести как оригинальные, так и неоригинальные запчасти, комплектующие для ТО, автохимию и автомасла, аккумуляторы, все для спецтехники. Представлен и каталог ГАЗ, ВАЗ, КАМАЗ. Есть возможность подобрать фильтр для автомобиля. Также представлены и автолампочки. Изучите раздел с рекомендуемыми товарами. Для того чтобы подобрать необходимую деталь, нужно воспользоваться поиском – в строке указать название детали. Все запчасти хранятся на складе и в нужном количестве.
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с высоким уровнем сервиса.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наши товары:
Заготовка из конструкционной стали круглая 20 мм 12Х2Н4А ОСТ 3-1686-90 Выбирайте конструкционные заготовки высокого качества для вашего проекта на Редметсплав.рф. Наш ассортимент включает прочные и долговечные материалы, идеально подходящие для различных областей применения, включая строительство и машиностроение.
кредит под залог птс
zaim-pod-zalog-pts1.ru/ekb.html
кредит под залог авто без авто
РедМетСплав предлагает обширный выбор отборных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица изделия подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Превосходное обслуживание – наша визитная карточка – мы на связи, чтобы ответить на ваши вопросы а также находить ответы под особенности вашего бизнеса.
Доверьте ваш запрос специалистам РедМетСплав и убедитесь в гибкости нашего предложения
Наша продукция:
Проволока кобальтовая Stellite 21 Проволока кобальтовая Stellite 21 – это высококачественный материал, предназначенный для сварки Рё напыления. РћРЅР° обладает уникальной устойчивостью Рє РєРѕСЂСЂРѕР·РёРё Рё РёР·РЅРѕСЃСѓ, что делает ее идеальной для применения РІ условиях высокой температуры Рё агрессивных сред. Рспользуется РІ различных отраслях, включая машиностроение Рё медицинскую технику. Кобальтовая проволока обеспечит надежность Рё долговечность ваших изделий, что существенно увеличит СЃСЂРѕРє РёС… службы. РќРµ упустите возможность купить Проволока кобальтовая Stellite 21 Рё обеспечьте своему производству высокую надежность!
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наша продукция:
Медный переходной пресс тройник 70х27.4 мм М2т ГОСТ Р52948-2008 Приобретайте надежные медные переходные тройники для соединения труб с разным диаметром. Устойчивы к коррозии, легки в монтаже и обеспечивают надежное соединение. Широкий выбор размеров. Гарантированное качество и долговечность.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наша продукция:
Керамическая мишень Al2O3, чистота 4N, плоская форма.
Before announcing a list of our exclusive products and services with an indication of their features and benefits, we urge you not to even try to write to us, begging to give you these products or provide services for free how to commit suicide
Oxys Crema es un tratamiento facial avanzado disponible en varios paises de Latinoamerica como Mexico, Uruguay, Paraguay, Chile y Peru; no se vende en farmacias tradicionales y solo se puede adquirir de forma segura a traves de su sitio web oficial https://oxyscrema.org/
Компания «КАРДЕКС» https://card-oil.ru/ — надежный партнер на рынке топливных решений, работающий более 12 лет. Специализируется на выпуске и обслуживании топливных карт для юридических лиц и индивидуальных предпринимателей. Преимущество КАРДЕКС — доступ к обширной сети из более чем 13000+ АЗС по всей России, включая федеральные и региональные заправочные станции. Это позволяет клиентам заправляться без привязки к конкретному бренду, оптимизируя расходы и логистику. Топливные карты КАРДЕКС обеспечивают удобный контроль затрат, предоставляют детальную аналитику и помогают экономить за счет персонализированных условий и скидок. Компания зарекомендовала себя как стабильный и технологичный игрок, предлагающий инновационные решения для бизнеса.
РедМетСплав предлагает обширный выбор отборных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица товара подтверждена требуемыми документами, подтверждающими их соответствие стандартам. Превосходное обслуживание – наша визитная карточка – мы на связи, чтобы улаживать ваши вопросы по мере того как предоставлять решения под специфику вашего бизнеса.
Доверьте ваш запрос специалистам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
поставляемая продукция:
Круг кобальтовый Stellite 1 Круг кобальтовый Stellite 1 – это высококачественный инструмент, разработанный для обработки металлов и сплавов. Он отличается высокой прочностью и устойчивостью к износу, что делает его идеальным выбором для профессионалов в сфере машиностроения и металлообработки. Состав кобальта обеспечивает отличные механические свойства, позволяя работать с различными материалами без потери качества. Не упустите возможность купить Круг кобальтовый Stellite 1 и повысить эффективность своих работ. Отличные характеристики и надежность помогут вам достичь лучших результатов в вашем бизнесе.
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с высоким уровнем сервиса.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
поставляемая продукция:
Круг 125 мм 10Х11Н23Т3МРКупить круги нержавеющие специальные ведущих производителей по выгодным ценам. Широкий выбор размеров и диаметров. Гарантия качества и надежности. Применение в различных отраслях и производствах. Надежная защита от коррозии и воздействия внешних факторов.
На сайте https://bitovayatechnika.blogspot.com/2025/03/blog-post.html изучите полезную, актуальную информацию, которая касается сплит-систем и того, как правильно их выбрать домой либо в офис. Эти функциональные и умные устройства нацелены на то, чтобы поддержать приятную температуру в помещении. Но для того, чтобы воспользоваться всеми функциями, необходимо правильно выбрать устройство. К важным преимуществам такой техники относят то, что она является энергоэффективной, работает практически бесшумно.
На сайте http://officepro54.ru представлено огромное количество мебели, которая идеально подходит для организации офисного пространства. Вся она выполнена из инновационных, уникальных материалов, а потому прослужит очень долго, не синтезирует в воздух опасных веществ. В разделе вы найдете кабинет руководителя, функциональные и вместительные столы для переговоров, шкафы-купе, сейфы, акустические системы, ученическую мебель и многое другое. Постоянно действуют акции. На продукцию установлены привлекательные цены.
деньги под залог авто в казани
avtolombard-pid-zalog-pts.ru/kazan.html
деньги под залог авто
нажмите здесь https://futbolka-alt.ru
Despite the fact that this advertisement is written in your native language, we communicate in international, English language hitman for hire
Find the Perfect Clock clocks top for Any Space! Looking for high-quality clocks? At Top Clocks, we offer a wide selection, from alarm clocks to wall clocks, mantel clocks, and more. Whether you prefer modern, vintage, or smart clocks, we have the best options to enhance your home. Explore our collection and find the perfect timepiece today!
селектор казино зеркало
[url=https://cse.google.gg/url?sa=t&url=https%3A%2F%2Fedpillrx.top]order ed drugs[/url] Drug effects explained. Pill trends described. buy ed drugs uk
check here
[url=https://sms-verif.pro/]Virtual numbers for service activation[/url]
Добро пожаловать на vavadaukr.kiev.ua, интересные материалы.
На vavadaukr.kiev.ua вы сможете, для того чтобы.
Не упустите шанс посетить vavadaukr.kiev.ua, вы найдете.
углубленное понимание.
С vavadaukr.kiev.ua.
Погрузитесь в контент vavadaukr.kiev.ua.
Развивайтесь вместе с vavadaukr.kiev.ua, что.
На vavadaukr.kiev.ua вы найдете, помогут вам.
На сайте vavadaukr.kiev.ua вы увидите, знать.
обширный контент.
Узнайте больше о vavadaukr.kiev.ua, где вам предлагается.
vavadaukr.kiev.ua предлагает, которые.
vavadaukr.kiev.ua выделяется среди других, поскольку.
На vavadaukr.kiev.ua вы найдёте поддержку в.
Делитесь своим мнением на vavadaukr.kiev.ua, где создаются.
На vavadaukr.kiev.ua мы предлагаем, которые.
Как vavadaukr.kiev.ua может помочь вам, придавая уверенность.
https://vavadaukr.kiev.ua/ [url=https://vavadaukr.kiev.ua/]https://vavadaukr.kiev.ua/[/url] .
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наши товары:
Гранулы гафния Hf 3N5
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние возможности.
Наши товары:
Плоская мишень CoTaZr для распыления сплава
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наши товары:
Мишень для распыления молибдена
Экскурсия на куршскую косу из калининграда kurshskaya-kosa-ekskursii.ru
кредит в залог автомобиля
zaim-pod-zalog-pts1.ru/kemerovo.html
кредит под птс
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – наилучшее решение для вашего бизнеса.
Наши товары:
Кольцо РёР· драгоценных металлов серебряное 40С…8С…4.5 РјРј РЎСЂ99.99 РўРЈ РЁРёСЂРѕРєРёР№ выбор высококачественных кольц РёР· драгоценных металлов. Рлегантные украшения, созданные талантливыми мастерами. Уникальность Рё изысканность РІ каждой детали. Возможность заказа персонального кольца РїРѕ вашим пожеланиям. Подчеркните СЃРІРѕСЋ индивидуальность СЃ нашими кольцами.
Сделать мероприятие незабываемым – это главная цель компании АРЕНДА КОМФОРТА. Предлагаем доступные цены и лояльные условия аренды шатров. Готовы помочь с выбором подходящей конструкции. Позвоните нам уже сейчас! xn--https://xn--80aaaaaaxhx2a2ai2af3a8grd.xn--p1ai/ – тут опубликованы отзывы наших клиентов, посмотрите их в удобное для вас время. Если ищете компанию, которая предоставляет высококачественные шатры, то АРЕНДА КОМФОРТА является одним из наилучших выборов. Мы гарантируем отменный уровень обслуживания, обращайтесь.
Looking for the best welcome bonus in online betting? 1xBet, a global gambling leader, gives new players an unbeatable 100% first deposit bonus (up to $100) when using exclusive promo code 1XRUN200 https://kasinoinsta.online/1xbet-promo-code-100-welcome-bonus-up-to-e130/
Looking for flower delivery in Ukraine? Visit https://ukraineflora.com/ where you will find the largest selection of fresh flowers and exclusive gifts. Look through the catalog and you will definitely find not only luxurious flowers and bouquets, but also gift baskets, perfumes, balloons and even cakes.
Looking for prediccion del precio de btc? Тhetradable.com/es/ es un poderoso portal de informacin que le trae a su atencin las ltimas noticias del mundo de los negocios, las criptomonedas, todo sobre Forex y la economa global. Aqui encontrara informacion interesante sobre los mercados de valores, criptomonedas, asi como metales preciosos como el oro y otros activos clave.
РедМетСплав предлагает широкий ассортимент отборных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица изделия подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы ответить на ваши вопросы а также находить ответы под требования вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в множестве наших преимуществ
Наша продукция:
РўСЂСѓР±Р° магниевая B 199 (EZ33A) – ASTM B199 Пруток магниевый B 199 (EZ33A) – ASTM B199 представляет СЃРѕР±РѕР№ высококачественный магниевый сплав, отличающийся легкостью Рё прочностью. Ртот материал идеально РїРѕРґС…РѕРґРёС‚ для использования РІ аэрокосмической промышленности, автомобильной отрасли Рё РґСЂСѓРіРёС… областях, требующих надежных Рё легких решений. Благодаря СЃРІРѕРёРј отличным механическим свойствам, пруток обеспечивает эффективную обработку Рё высокую РєРѕСЂСЂРѕР·РёРѕРЅРЅСѓСЋ стойкость. Выбирая данный РїСЂРѕРґСѓРєС‚, РІС‹ получаете гарантированное качество Рё долговечность. РќРµ упустите возможность купить Пруток магниевый B 199 (EZ33A) – ASTM B199 Рё улучшить эффективность СЃРІРѕРёС… проектов!
РедМетСплав предлагает обширный выбор отборных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до масштабных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица продукции подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Дружелюбная помощь – наш стандарт – мы на связи, чтобы улаживать ваши вопросы и адаптировать решения под особенности вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
Наши товары:
Фольга висмутовая CAC904 Фольга висмутовая CAC904 – отличное решение для различных промышленных и декоративных задач. Она отличается высокой прочностью, устойчивостью к коррозии и способен обеспечить надежную защиту от высоких температур. Применяется в электронике, медицине и для создания уникальных декоративных элементов. Благодаря своим качествам, фольга легко обрабатывается и поддается формовке. Не упустите возможность купить Фольга висмутовая CAC904 и обеспечьте свою продукцию высококачественными материалами, которые соответствуют современным требованиям.
[url=https://images.google.co.nz/url?sa=t&url=http%3A%2F%2Fedpillrx.top]buy ed drugs usa[/url] Comprehensive pill resource. Medication resource here. ed drugs
In the online gambling environment, promo codes have become an integral part, offering insiders unique opportunities to receive prizes and promotions https://oboyan.7bb.ru/viewtopic.php?id=1811#p17802
Цветы простояли больше недели – рекорд!
заказать цветы томск
Willkommen in einer Welt voller spannender Casino-Unterhaltung! Entdecke eine gro?e Auswahl an Online Slots, klassischen Tischspielen und Live-Casino-Erlebnissen Yaacasino
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние возможности.
Наши товары:
Тигель тантала для источников испарения
The new TR Energy https://www.zillow.com/profile/jreliford8 service aims to optimize both energy and bandwidth usage, solving the problem of high transaction fees that have previously hampered TRON’s scalability.
fruit cocktail
1xBet is a well-known online betting platform that is famous for its generous bonuses and attractive offers. If you are looking for a unique gaming experience and additional chances of success, then 1xBet bonuses will be an excellent choice for you https://thesleepinghusband.rolka.me/viewtopic.php?id=25085#p62445
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – идеальный вариант для вас.
Наша продукция:
Заготовка из конструкционной стали квадратная 10 мм 20ХМ ОСТ 3-1686-90 Выбирайте конструкционные заготовки высокого качества для вашего проекта на Редметсплав.рф. Наш ассортимент включает прочные и долговечные материалы, идеально подходящие для различных областей применения, включая строительство и машиностроение.
займ на неделю без процентов
РедМетСплав предлагает внушительный каталог высококачественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы обеспечиваем оперативное исполнение вашего заказа.
Каждая единица продукции подтверждена соответствующими документами, подтверждающими их соответствие стандартам. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы ответить на ваши вопросы и находить ответы под особенности вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
поставляемая продукция:
РљСЂСѓРі магниевый ZE10A Фольга магниевая ZE10A – это высококачественный РїСЂРѕРґСѓРєС‚, который идеально РїРѕРґС…РѕРґРёС‚ для использования РІ различных отраслях, включая авиацию Рё автомобилестроение. РћРЅР° обладает отличной прочностью Рё легкостью, что делает ее незаменимой РІ производственных процессах. Рта фольга устойчива Рє РєРѕСЂСЂРѕР·РёРё Рё обеспечивает надежную защиту РїСЂРё длительном использовании. РќРµ упустите возможность улучшить СЃРІРѕРё проекты СЃ помощью этого материала! Р’С‹ можете купить Фольга магниевая ZE10A РїСЂСЏРјРѕ сейчас Рё воспользоваться всеми преимуществами, которые РѕРЅР° предлагает. Данное изделие соответствует современным требованиям Рё стандартам качества.
Заказать Tank – только у нас вы найдете цены ниже рынка. Быстрей всего сделать заказ на официальный дилер tank в спб можно только у нас!
[url=https://tankautospb.ru]купить автомобиль марки танк[/url]
автомобиль танк стоимость – [url=http://www.tankautospb.ru]https://www.tankautospb.ru/[/url]
[url=https://cse.google.hn/url?sa=t&url=https%3A%2F%2Fedpillrx.top]buy ed drugs[/url] Latest drug developments. Get pill facts. buy ed drugs medication
https://mycofarm.ru/
На сайте https://ooo-rodnik.ru/ уточните телефон популярной компании «Родник», основной сферой деятельности которой является чистка, ремонт скважин по всей Московский области. Предприятие оказывает услуги в своей сфере уже более 5 лет. Работа происходит без выходных, пенсионеры смогут рассчитывать на приятные скидки, бонусы. На все работы дается гарантия в один год. Компания занимается обслуживанием дач, коттеджей, домов, производств, поселков городского типа. Со всеми направлениями деятельности ознакомьтесь на сайте.
teen patti
Non Gamstop casino UK has great support—big thumbs up!
safe non gamstop casinos
[url=https://maps.google.com.ly/url?sa=t&url=https%3A%2F%2Fcanadianrxp.top]buy ed drugs with no prescription[/url] Patient pill resource. Current medication trends. buy ed drugs online
fishin frenzy
plinko
mines game
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние достижения.
Наша продукция:
Керамическая мишень HfO2
razor returns
Ищете оборудование из Китая по ценам производителя? Посетите сайт https://totem28.ru/ где вы найдете широкий ассортимент не только оборудования, но и спец техники. Наш каталог постоянно пополняется, мы тщательно отбираем новинки китайского машиностроения. Посмотрите наш каталог и узнайте подробнее на сайте.
подключить интернет тарифы екатеринбург
domashij-internet-ekaterinburg001.ru
домашний интернет
plinko
ВДГБ помогает клиентам управлять финансами, складским учетом и производственными процессами. Кроме этого мы предлагаем для ваших сотрудников обучение и поддержку системы на всех этапах ее эксплуатации обеспечиваем. Вы сможете узнать на сайте, какие преимущества бизнесу автоматизация предоставляет. https://erp.vdgb.ru – сайт, где бесплатно можно заказать экспертизу и получить массу достоинств. На вопросы ответьте, и мы вам подготовим лучшее предложение. Заполните уже сейчас форму. На портале вы детальнее с этапами проекта внедрения ЕРП можете ознакомиться.
МикоФарм Фунги
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наша продукция:
Плоская мишень CoTaZr для распыления сплава
my latest blog post [url=https://Keplr.at]keplr Download[/url]
РедМетСплав предлагает внушительный каталог высококачественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица изделия подтверждена требуемыми документами, подтверждающими их соответствие стандартам. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы разрешать ваши вопросы и адаптировать решения под требования вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
Наши товары:
Лента магниевая MgAl6Zn – DIN 1729 Part 2 РљСЂСѓРі магниевый MgAl6Zn – DIN 1729 Part 2 – это высококачественный материал, предназначенный для применения РІ различных отраслях. РћРЅ изготовлен РёР· сплава, который обладает отличными антикоррозионными свойствами Рё высокой прочностью. Рспользуется РІ производстве деталей Рё конструкций, РіРґРµ важна легкость Рё долговечность. Благодаря СЃРІРѕРёРј характеристикам, этот РєСЂСѓРі идеально РїРѕРґС…РѕРґРёС‚ для применения РІ авиации, автомобилестроении Рё РґСЂСѓРіРёС… высокотехнологичных сферах. Если РІС‹ хотите повысить надежность СЃРІРѕРёС… изделий, купите РљСЂСѓРі магниевый MgAl6Zn – DIN 1729 Part 2 сегодня!
Эксперт-Техника – компания, которая осуществляет полный спектр услуг – продажа, поставки запчасти и комплектующих, сервисное обслуживание. Наши специалисты повышение квалификации и техническое обучение проходят. Мы разработали гибкую и надежную систему доставки. https://extehno.ru – здесь можете ознакомиться с условиями оплаты. Предлагает широкий выбор спецтехники. Стремимся к клиентам ближе быть. При возникновении вопросов, созвонитесь с нами по телефону, который на портале указан. Отменный уровень сервиса мы вам гарантируем.
book of ra
plinko
[url=https://cse.google.com.pk/url?sa=t&url=https%3A%2F%2Fcanadianrxp.top]buy ed drugs[/url] Medicine leaflet here. Pill facts available. purchase ed drugs online no prescription
aviator game
The best non Gamstop casinos keep me coming back—pure fun!
legit non gamstop casinos
скачать mostbet на телефон [url=http://svstrazh.forum24.ru/?1-18-0-00000136-000-0-0-1743260517]скачать mostbet на телефон[/url] .
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
поставляемая продукция:
Меднаядвухраструбная подвижнаямуфта под пайку76.1х2.6ммCu-DHPГОСТ 32590-2013 Выберите медные двухраструбные муфты под пайку от производителя RedmetSplav. Прочные и герметичные соединения для систем водоснабжения, отопления и газоснабжения. Долговечные и надежные муфты для любых видов работ. Подходят для использования в домашних условиях, коммерческих и промышленных объектах.
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наши товары:
Труба бронзовая 45х7.5х2500 мм БрАЖН9-4-4 ГОСТ 1208-2014 Приобретайте трубы бронзовые круглые от профессионалов с опытом. Мы предлагаем широкий ассортимент качественной продукции, быструю обработку заказов и оперативную доставку. Обращайтесь, чтобы получить консультацию специалиста и выгодные условия покупки.
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
Наши товары:
Бронзовый шестигранник 12 мм БрОЦ4-3 ТУ 48-21-867-89 Приобретите бронзовый шестигранник диаметром 12 мм, марки БрОЦ4-3 по ТУ 48-21-867-89 на Редметсплав.рф. Прочный и износостойкий продукт, идеальный для точных монтажных работ и использования в различных отраслях. Гарантия долгого срока службы и высокой устойчивости к коррозии.
Вместе с t.me/azino777_a у вас есть возможность в увлекательное путешествие по миру азартных игр отправиться. Предлагаем персонализированные предложения и уникальные бонусы. Расскажем, как начать играть в Азино. Вас ждет возможность выиграть крупный приз и невероятные эмоции. Испытайте свою удачу уже сейчас! Ищете azino777 мобильная версия? – тут имеется множество актуальной информации. Собрали самые интересующие вопросы от игроков. Всегда готовы помочь вам. Приготовьтесь к увлекательным турнирам в Азино. Участвуйте и боритесь за призы с другими игроками!
a knockout post [url=https://sites.google.com/mycryptowalletus.com/metamask-wallet-login/]Metamask Extension[/url]
РедМетСплав предлагает широкий ассортимент отборных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до масштабных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица товара подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Опытная поддержка – то, чем мы гордимся – мы на связи, чтобы улаживать ваши вопросы и предоставлять решения под особенности вашего бизнеса.
Доверьте ваш запрос специалистам РедМетСплав и убедитесь в множестве наших преимуществ
Наша продукция:
РљСЂСѓРі висмутовый CACIn904 – JIS H 2202-2016 РљСЂСѓРі висмутовый CACIn904 – JIS H 2202-2016 является высококачественным материалом, предназначенным для различных промышленных применений. Его уникальные свойства обеспечивают отличную прочность Рё долговечность, что делает его идеальным выбором для специалистов РІ области металлообработки. РЎ помощью этого РєСЂСѓРіР° РІС‹ сможете добиться высокой точности Рё качества обработки. РќРµ упустите возможность купить РљСЂСѓРі висмутовый CACIn904 – JIS H 2202-2016 Рё улучшить СЃРІРѕРё производственные процессы. Выберите надежность Рё эффективность РІ РѕРґРЅРѕРј продукте!
plinko
turn photo into augmented reality [url=www.augmented-reality-platform.ru/]www.augmented-reality-platform.ru/[/url] .
одежда с надписями брендов [url=www.dbkids.ru/]www.dbkids.ru/[/url] .
tome of madness
andar bahar
address [url=https://phantom-wallet.at/]phantom Download[/url]
провайдер по адресу
domashij-internet-ekaterinburg002.ru
провайдеры интернета в екатеринбурге по адресу проверить
аренда лофт [url=https://snyat-loft-v-spb.ru/]аренда лофт[/url] .
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым текущие трудности превращаются в завтрашние достижения.
Наша продукция:
Плоская мишень Nb2O5 4N
[url=http://www.city-fs.de/url?q=https%3A%2F%2Fcanadianrxp.top]buy ed drugs[/url] Find medication information. Pill information here. buy ed drugs online without prescription
Use 1XBET promo code: 1XTAX200 for VIP bonus up to €1950 + 150 free spins on casino and 100% up to €130 to bet on sports. Register on the 1xbet platform and get a chance to earn even more Rupees using bonus offers and special bonus code from 1xbet. Make sports bets, virtual sports or play at the casino 1xbet promo code today bd
МикоФарм Фунги
In the world of online betting and gambling, promo codes have become an integral part of the strategy of players looking for maximum benefit from their bets https://nastyapoleva.ru/templates/pages/promokod_melbet_bonus_do_15_000_rub.html
Приобрести документ о получении высшего образования можно у нас в Москве. [url=http://diplomgorkiy.com/kupit-diplom-bryansk/]diplomgorkiy.com/kupit-diplom-bryansk[/url]
Где заказать диплом специалиста?
Наши специалисты предлагают выгодно и быстро купить диплом, который выполнен на оригинальном бланке и заверен мокрыми печатями, штампами, подписями официальных лиц. Данный диплом способен пройти лубую проверку, даже с применением профессионального оборудования. Решите свои задачи быстро и просто с нашими дипломами.
Приобрести диплом университета [url=http://diplomt-v-chelyabinske.ru/kupit-diplom-v-tambove-11/]diplomt-v-chelyabinske.ru/kupit-diplom-v-tambove-11/[/url]
купить диплом вгу
Топ фирм для квартирного переезда в Петербурге в 2025 году Шокирующий разбор ценников: почему одни берут 9000 ?, а другие 25 000 ? за одинаковый переезд? Мы расшифровали прайсы 30 компаний и нашли подвох — в 70% случаев переплата идет за ненужные услуги. В материале — конкретные названия трех фирм с честной фиксированной стоимостью и реальными отзывами от клиентов.
Где купить диплом по актуальной специальности?
[b]Наша компания предлагает[/b] выгодно и быстро заказать диплом, который выполняется на оригинальном бланке и заверен мокрыми печатями, водяными знаками, подписями. Данный диплом пройдет лубую проверку, даже при использовании профессиональных приборов. Достигайте своих целей быстро и просто с нашим сервисом. Купить диплом о высшем образовании! [url=http://forum.rivnefish.com/viewtopic.php?t=701188/]forum.rivnefish.com/viewtopic.php?t=701188[/url]
Vinci Spin
Vinci Spin
ganesha gold
РедМетСплав предлагает внушительный каталог отборных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица продукции подтверждена соответствующими документами, подтверждающими их происхождение. Превосходное обслуживание – наш стандарт – мы на связи, чтобы улаживать ваши вопросы а также адаптировать решения под особенности вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
Пруток магниевый G-A6Z3 – AFNOR NF A57-704 Проволока магниевая G-A6Z3 – AFNOR NF A57-704 представляет СЃРѕР±РѕР№ высококачественный материал, который широко применяется РІ различных отраслях. Благодаря СЃРІРѕРёРј уникальным свойствам, таким как высокая прочность Рё устойчивость Рє РєРѕСЂСЂРѕР·РёРё, этот РїСЂРѕРґСѓРєС‚ идеально РїРѕРґС…РѕРґРёС‚ для изготовления легких конструкций. Рспользование магниевых сплавов помогает значительно снизить вес изделий без ущерба для РёС… прочности. Если вам РЅСѓР¶РЅР° надежная проволока для СЃРІРѕРёС… проектов, РЅРµ упустите возможность купить Проволока магниевая G-A6Z3 – AFNOR NF A57-704. Ртот товар станет отличным решением для профессионалов.
Судоходная компания Навигатор https://teplohod-restoran.ru/ это возможность организовать праздник на воде в Санкт-Петербурге. Свадьбы, дни рождения, корпоративы, выпускные, а также банкеты и фуршеты. Мы комплексно подходим к организации любого события. Посетите сайт, посмотрите наш флот и стоимость аренды судов, яхт, катеров, теплоходов.
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
поставляемая продукция:
Медный переходной пресс тройник 66.7х14 мм М1р ГОСТ Р52948-2008 Приобретайте надежные медные переходные тройники для соединения труб с разным диаметром. Устойчивы к коррозии, легки в монтаже и обеспечивают надежное соединение. Широкий выбор размеров. Гарантированное качество и долговечность.
На сайте https://ooo-rodnik.ru/ уточните телефон популярной компании «Родник», основной сферой деятельности которой является чистка, ремонт скважин по всей Московский области. Предприятие оказывает услуги в своей сфере уже более 5 лет. Работа происходит без выходных, пенсионеры смогут рассчитывать на приятные скидки, бонусы. На все работы дается гарантия в один год. Компания занимается обслуживанием дач, коттеджей, домов, производств, поселков городского типа. Со всеми направлениями деятельности ознакомьтесь на сайте.
Вместе с t.me/azino777_a можете отправиться в захватывающее путешествие по миру азартных игр. Предлагаем персонализированные предложения и уникальные бонусы. Расскажем, как в Азино начать играть. Вас ждет возможность выиграть крупный приз и невероятные эмоции. Не упустите шанс испытать свою удачу! Ищете azino777 песня? – здесь представлено много интересной информации. Собрали самые интересующие вопросы от игроков. Всегда готовы помочь вам. Приготовьтесь к завлекательным в Азино турнирам. Участвуйте и с другими игроками боритесь за призы!
Доставка комплексных обедов в Алматы для ваших сотрудников или на дом на сайте https://bvd.kz/ – у нас широкий ассортимент и выбор блюд по отличным ценам! Ознакомьтесь на сайте с составами и ценами на комплексные обеды. Заключаем договоры на доставку обедов в офисы и административные учреждения, доставляем обеды в школы и детские сады, где нет собственной кухни, в магазины и прочие подобные учреждения, у сотрудников которых нет возможности пообедать в столовой. Подробнее на сайте.
детская одежда с надписями [url=http://dbkids.ru]http://dbkids.ru[/url] .
Где приобрести диплом специалиста?
Мы изготавливаем дипломы любых профессий по приятным тарифам. Мы предлагаем документы ВУЗов, расположенных на территории всей Российской Федерации. Можно приобрести качественный диплом от любого заведения, за любой год, включая сюда документы СССР. Документы печатаются на “правильной” бумаге самого высокого качества. Это позволяет делать настоящие дипломы, которые невозможно отличить от оригиналов. Они будут заверены всеми обязательными печатями и подписями. Стараемся поддерживать для заказчиков адекватную ценовую политику. Важно, чтобы документы были доступны для подавляющей массы граждан. [url=http://diplom-city24.ru/kupit-diplom-v-velikom-novgorode-4/]diplom-city24.ru/kupit-diplom-v-velikom-novgorode-4[/url]
Non Gamstop casinos have unreal bonuses—super cool!
non gamstop casinos uk no deposit bonus
I won $4500 online—dream night! dragon tiger
[url=https://images.google.lu/url?q=https%3A%2F%2Fcanadianrxp.top]buy generic ed drugs[/url] Detailed drug knowledge. Medicine effects explained. buy ed drugs online
подключить интернет в екатеринбурге в квартире
domashij-internet-ekaterinburg003.ru
провайдер по адресу екатеринбург
На сайте https://miners-store.ru/ вы сможете связаться с представителями компании для того, чтобы выбрать и приобрести асики для майнинга. Перед вами функциональное и качественное оборудование, которое требуется для того, чтобы добыть криптовалюту. Изучите всех производителей такого оборудования, которое можно заказать по лучшей стоимости. Есть и самые популярные модели, которые приобретают чаще остальных. Здесь также осуществляется продажа видеокарт. Специалисты соберут специальную ферму для майнинга с нуля.
mines
[b]Eliminate Vibration Issues and Improve Equipment Performance[/b]
Vibration is a silent killer of industrial machines. Imbalance leads to worn-out bearings, misalignment, and costly breakdowns. [b]Balanset-1A[/b] is the ultimate tool for detecting and correcting vibration problems in electric motors, pumps, and turbines.
[b]What Makes Balanset-1A Stand Out?[/b]
– Precise vibration measurement & balancing
– Compact, lightweight, and easy to use
– Two kit options:
[url=https://www.amazon.es/dp/B0DCT5CCKT]Full Kit on Amazon[/url] – Advanced sensors & accessories, Software for real-time data analysis, Hard carrying case
Price: [b]2250 EUR[/b]
[url=https://www.amazon.es/dp/B0DCT5CCKT][img]https://i.postimg.cc/SXSZy3PV/4.jpg[/img][/url]
[url=https://www.amazon.es/dp/B0DCT4P7JR]OEM Kit on Amazon[/url] – Includes core balancing components, Same high-quality device
Price: [b]1978 EUR[/b]
[url=https://www.amazon.es/dp/B0DCT4P7JR][img]https://i.postimg.cc/cvM9G0Fr/2.jpg[/img][/url]
Prevent unexpected breakdowns – Invest in [b]Balanset-1A[/b] today!
How to exchange BITCOIN in the cryptocurrency exchanger quickex are Buy or sell BTC? In this https://www.youtube.com/watch?v=0MupM-q6I3A detailed guide, we will show you how to buy BTC on the quickex platform in 5 easy steps. You will learn how to use a reliable service without registration and verification, getting access to the best rates from leading liquidity providers.
create ar online [url=www.augmented-reality-platform.ru]create ar online[/url] .
Are you sure you’re ready to see this?
Access restricted to 18+
[url=https://ify.ac/1cMg]Penetrate[/url]
На сайте https://rodinasportsclub.com/ вы найдете информацию, которая касается спортивно-развлекательного клуба «Родина». Каждый желающий получает возможность записаться на тренировку по боксу, которая подходит как новичкам, так и более опытным спортсменам. Вас заинтересуют тренировки по рукопашному бою. Перед вами расписание предстоящих матчей на апрель. Ознакомьтесь с ними сейчас, чтобы построить планы. Также имеются и отчеты по прошедшим соревнованиям. Опубликованы разные новости из жизни клуба.
аренда лофта спб [url=http://snyat-loft-v-spb.ru/]аренда лофта спб[/url] .
Купить диплом об образовании!
Мы готовы предложить дипломы любой профессии по приятным ценам— [url=http://diplomskiy.com/kupit-diplom-vracha-po-lechebnomu-delu-vigodno/]diplomskiy.com/kupit-diplom-vracha-po-lechebnomu-delu-vigodno/[/url]
Мы изготавливаем дипломы психологов, юристов, экономистов и других профессий по приятным ценам. Всегда стараемся поддерживать для заказчиков адекватную ценовую политику. Для нас важно, чтобы документы были доступны для подавляющей массы граждан.
Заказ документа, подтверждающего окончание института, – это грамотное решение. Купить диплом любого университета: [url=http://diplomdoc.ru/gde-kupit-diplom-texnikuma-5/]diplomdoc.ru/gde-kupit-diplom-texnikuma-5/[/url]
sugar rush
МикоФарм Фунги
Başakşehir su sızıntısı tespiti Mutfak tezgahı altındaki su kaçağını özel kameralarla buldular. Dolaplara hiç zarar gelmedi. Aysun Q. https://www.ypchina.org/?p=180239
Компания «Эксперт-Техника» реализовывает весь спектр услуг – продажа, сервисное обслуживание, поставки запчасти, также комплектующих. Наши специалисты повышение квалификации и техническое обучение проходят. Мы разработали гибкую и надежную систему доставки. https://extehno.ru – тут с условиями оплаты можете ознакомиться. Предлагает широкий выбор спецтехники. Стремимся к клиентам ближе быть. Если у вас возникли вопросы, обратитесь к менеджеру по телефону, указанному на сайте. Отменный уровень сервиса мы вам гарантируем.
Moderni nabytek satni skrin bila do kazdeho interieru – od minimalismu po klasiku. Vice nez 1000 modelu skladem. Online objednavka, pohodlna platba, pomoc navrhare. Zaridte svuj domov pohodlim!
plinko
Используйте AstoMagic – бесплатную онлайн-нейросеть. Задайте вопрос – и получите необходимый ответ за секунды. ИИ анализирует ваш стиль общения и под него подстраивается. Получайте в написании кода помощь и ошибок исправлении. https://astomagic.com – здесь собрали ответы на часто задаваемые вопросы. На ресурсе тарифы и отзывы пользователей представлены. Общайтесь голосом – система распознает речь и выдает ответы в текстовом виде. ИИ информацию анализирует и точные данные предоставляет. Не упустите возможность опробовать нейросеть онлайн!
Casino non Gamstop sites load so quick—awesome!
no gamstop casinos
[url=https://cse.google.md/url?sa=t&url=https%3A%2F%2Fcanadianrxp.top]buy ed drugs with no prescription[/url] Patient drug facts. Pill impacts described. ed drugs
На сайте https://remont-okon-63.ru/ уточните номер телефона для того, чтобы воспользоваться такой нужной услугой, как ремонт, регулировка окон независимо от сложности. Прямо сейчас вы сможете воспользоваться возможностью вызвать мастера. Для этого следует указать номер телефона, а также имя. Среди основных услуг, которые оказываются в этой компании, выделяют: ремонт балконных дверей, производство москитных сеток, стеклопакетов. Кроме того, доступен и срочный ремонт конструкций. Все работы выполняются в соответствии с требованиями.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
С широким ассортиментом продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние успехи.
Наши товары:
Плоская мишень распыления Cegd сплавов
Etsitko hyodyllista tietoa lainoista? Vieraile osoitteessa https://esimerkkilinkki.fi/ – josta loydat kattavaa tietoa asuntolainasta, vinkkeja lainan hakemiseen, lainojen vertailuun ja paikkaan, kuinka saada laina heti ilman luottotietoja tai luottohistoriaa ja paljon muuta. Hyodyllista tietoa sinulle verkkosivuillamme.
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
поставляемая продукция:
Проволока вольфрамовая 1.5 мм ВТ-15-А ГОСТ 18903-73 Купить вольфрамовую проволоку у производителя. Широкий выбор диаметров и нарезка по размеру. Высокая теплопроводность и стойкость к коррозии. Доставка по России.
мостбет скачать казино [url=https://www.mostbet6008.ru]https://www.mostbet6008.ru[/url] .
МикоФарм Фунги
The live dealers online rule—true vibe! legacy of dead
РедМетСплав предлагает обширный выбор высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их качество. Дружелюбная помощь – то, чем мы гордимся – мы на связи, чтобы ответить на ваши вопросы а также адаптировать решения под особенности вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
поставляемая продукция:
Лента магниевая РњРђ12 – ГОСТ 14957-76 РљСЂСѓРі магниевый РњРђ12 – ГОСТ 14957-76 представляет СЃРѕР±РѕР№ высококачественный металлургический РїСЂРѕРґСѓРєС‚, используемый РІ различных сферах. РћРЅ обладает отличной прочностью Рё легкостью, что делает его идеальным для применения РІ строительстве, авиации Рё машиностроении. Сравнительно низкая плотность магния позволяет сократить вес конструкций РїСЂРё неизменной прочности. Если РІС‹ ищете надежное решение для СЃРІРѕРёС… проектов, рекомендуем купить РљСЂСѓРі магниевый РњРђ12 – ГОСТ 14957-76. Ртот РїСЂРѕРґСѓРєС‚ сочетает РІ себе высокие эксплуатационные характеристики Рё доступную цену.
I wish online kept classics—old love! plinko
The live games online shine—big fan! book of dead
Посетите сайт https://4px.ru/ – 4 Пикселя – это Digital агентство интернет-маркетинга полного цикла в Москве, с комплексным подходом к созданию рекламы в интернете. Ознакомьтесь с нашими выгодными услугами в SEO, Яндекс.Директ, SMM, Serm, разработкой и созданием сайтов и многими другими профессиональными услугами. Ознакомьтесь с нашими кейсами – мы будем рады видеть вас в числе наших клиентов!
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с прекрасным качеством обслуживания.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
поставляемая продукция:
РџСЂСѓР¶РёРЅР° РёР· драгоценных металлов палладиевая 650С…5С…0.2 РјРј РџРґР90-10 РўРЈ Выберите идеальные РїСЂСѓР¶РёРЅС‹ РёР· золота, серебра или платины, чтобы дополнить ваше украшение. Найдите РїСЂСѓР¶РёРЅС‹ СЃ изысканным дизайном Рё прочностью. РћРЅРё предлагают широкий выбор для того, чтобы подчеркнуть уникальность вашего украшения.
https://ruera.ru/ero/10594-izyaschnye-sputnicy-dlya-samyh-izyskannyh-mgnoveniy.html
На сайте https://miners-store.ru/ вы сможете связаться с представителями компании для того, чтобы выбрать и приобрести асики для майнинга. Перед вами функциональное и качественное оборудование, которое требуется для того, чтобы добыть криптовалюту. Изучите всех производителей такого оборудования, которое можно заказать по лучшей стоимости. Есть и самые популярные модели, которые приобретают чаще остальных. Здесь также осуществляется продажа видеокарт. Специалисты соберут специальную ферму для майнинга с нуля.
Are online casino wins legit, or just a lure? aviator
Visit https://reviewspoty.com/ and you can easily collect reviews by integrating with your existing technology and e-commerce platforms. Increase sales and reduce marketing costs with reviews. Learn more about us and what we offer, as well as pricing and a free trial.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наши товары:
Гранулы магниевого фторида 4N
Online casinos should have stricter age verification; kids could easily sneak in. plinko
АВАНГАРД – компания, которая на продаже и доставке в Краснодарском крае инертных материалов специализируется. Предлагаем конкурентные цены и гарантируем высочайшее качество поставляемого плодородного грунта. Мы нарастили большую базу клиентов. https://avangard-kuban.ru/chernozjom-i-grunty/ – здесь можете подробнее ознакомиться с условиями оплаты и доставки. На чернозем действуют скидки. Если ищете надежную компанию доставки инертных материалов, обратитесь к нам. С радостью поможем вам в решении любых вопросов. Звоните нам!
Online casinos should show—odds up! fruit cocktail
казино Мир казино – это захватывающая вселенная, где переплетаются азарт, стратегия и технологические инновации. Онлайн казино предлагают широкий спектр игр, от классических слотов до захватывающих настольных игр, предоставляя игрокам возможность испытать удачу и применить свои навыки. Стратегии играют ключевую роль в успехе. Будь то блэкджек, покер или рулетка, понимание правил и умение анализировать ситуацию могут значительно повысить шансы на выигрыш. Важно помнить о разумном управлении банкроллом и осознанном подходе к игре.
Мы предлагаем дипломы психологов, юристов, экономистов и любых других профессий по приятным ценам. Стоимость зависит от той или иной специальности, года получения и ВУЗа. Стараемся поддерживать для заказчиков адекватную ценовую политику. Важно, чтобы документы были доступны для большого количества наших граждан. [url=http://vacshidiplom.com/kupit-diplom-spb-gosudarstvennoj-akademii-teatralnogo-iskusstva/]диплом кемерово купить[/url]
Где заказать диплом специалиста?
Наша компания предлагает выгодно и быстро заказать диплом, который выполняется на оригинальном бланке и заверен печатями, водяными знаками, подписями должностных лиц. Документ пройдет лубую проверку, даже с применением специально предназначенного оборудования. Достигайте своих целей максимально быстро с нашей компанией.
Заказать диплом любого ВУЗа [url=http://diploml-174.ru/kupit-diplom-texnologa-5/]diploml-174.ru/kupit-diplom-texnologa-5/[/url]
купить диплом в москве недорого
РедМетСплав предлагает внушительный каталог качественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица товара подтверждена всеми необходимыми документами, подтверждающими их происхождение. Опытная поддержка – то, чем мы гордимся – мы на связи, чтобы улаживать ваши вопросы а также адаптировать решения под особенности вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
РџРѕРєРѕРІРєР° магниевая AZ-91B – РўРЈ 1714-001-00545484-96 Лист магниевый AZ-91B – РўРЈ 1714-001-00545484-96 представляет СЃРѕР±РѕР№ легкий Рё надежный материал, который широко используется РІ различных отраслях, включая авиастроение Рё автомобилестроение. Обладая высокой прочностью Рё РєРѕСЂСЂРѕР·РёР№РЅРѕР№ стойкостью, данный магниевый лист идеально РїРѕРґС…РѕРґРёС‚ для изготовления деталей, требующих легкости Рё долговечности. Приобретая этот лист, РІС‹ получаете высококачественный РїСЂРѕРґСѓРєС‚, который отвечает современным требованиям. РќРµ упустите возможность купить Лист магниевый AZ-91B – РўРЈ 1714-001-00545484-96 для СЃРІРѕРёС… проектов Рё получите конкурентные преимущества!
Где купить диплом по актуальной специальности?
[b]Мы предлагаем[/b] выгодно приобрести диплом, который выполняется на оригинальном бланке и заверен печатями, водяными знаками, подписями официальных лиц. Документ пройдет лубую проверку, даже при использовании специально предназначенного оборудования. Достигайте свои цели максимально быстро с нашим сервисом. Приобрести диплом ВУЗа! [url=http://chatclik.com/read-blog/7120_kupit-svidetelstvo-o-zaklyuchenii-braka.html/]chatclik.com/read-blog/7120_kupit-svidetelstvo-o-zaklyuchenii-braka.html[/url]
mosbet [url=www.mostbet6008.ru]www.mostbet6008.ru[/url] .
Ищете, через какой сервис моментально оформить заём, не проходя долгие проверки на неодобрения? На сайте [url=https://all-credit.ru/]малоизвестные новые займы на карту онлайн без отказа с плохой кредитной историей и просрочками[/url] собраны актуальные МФО, которые реально одобряют заявки всем — от 18 лет и всего по удостоверению личности. Даже если у вас испорченная кредитная история или были задержки платежей, вы найдёте каталог займов, созданную именно под это. Всё просто, быстро и без неожиданностей.
Актуальное обновление 2025 года — обновлённый рейтинг займов под залог ПТС, и при этом автомобиль остаётся у вас. А ещё мы собрали советы и рекомендации, которые помогут упорядочить ваше финансовое положение без рисков. Выбирайте [url=https://all-credit.ru/]займ под зарплату срочно без проверок реальный сайт[/url] с моментальным одобрением, онлайн-доступом 24/7 и высокой вероятностью на получение денег уже через несколько минут.
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
Наши товары:
Нержавеющая прямоугольная РїРѕРєРѕРІРєР° 200С…470 РјРј 04РҐ19МАФТ Рзучите широкий ассортимент нержавеющих прямоугольных РїРѕРєРѕРІРѕРє РЅР° RedmetSplav СЃ гарантией качества Рё надежности. Устойчивость Рє РєРѕСЂСЂРѕР·РёРё Рё разнообразие размеров для всевозможных проектов.
Olymp Casino Online feels legit, but I’m still cautious about depositing too much. olymp casino
The poker visuals online are insane—cool!
my webpage – https://rapidapi.com/MartinRichards/api/hermi2/discussions/148450
I’ve had a streak of bad luck at Olymp Casino Online lately—hoping it turns around.
olymp casino
Online casinos should guide—too hard! plinko
Купить диплом университета!
Мы предлагаем документы институтов, которые находятся на территории всей России.
[url=http://diplomus-spb.ru/kupit-diplom-s-zaneseniem-bistro-i-bez-problem/]diplomus-spb.ru/kupit-diplom-s-zaneseniem-bistro-i-bez-problem/[/url]
Где купить диплом специалиста?
Мы предлагаем дипломы любой профессии по доступным тарифам. Мы можем предложить документы техникумов, которые находятся в любом регионе РФ. Вы можете заказать качественный диплом от любого учебного заведения, за любой год, указав необходимую специальность и оценки за все дисциплины. Дипломы и аттестаты выпускаются на “правильной” бумаге самого высшего качества. Это дает возможности делать государственные дипломы, не отличимые от оригиналов. Они будут заверены необходимыми печатями и штампами. Стараемся поддерживать для покупателей адекватную политику тарифов. Важно, чтобы дипломы были доступными для большинства граждан. [url=http://diplomoz-197.com/kupit-attestat-za-9-klassov-2-3/]diplomoz-197.com/kupit-attestat-za-9-klassov-2-3[/url]
Официальный сайт 1win https://1win.onedivision.ru спортивные ставки, киберспорт, казино, покер, рулетка и многое другое. Надежный сервис, поддержка 24/7, удобный интерфейс. Забирай бонус за регистрацию уже сейчас!
[url=https://images.google.gr/url?sa=t&url=https%3A%2F%2Fcanadianrxp.top]order ed drugs[/url] Access drug details. Patient medicine guide. buy ed drugs online without prescription
Online casinos should warn you when you’ve been playing too long. aviator game
Olymp Casino Online’s promotions keep me coming back for more.
olymp casino
Заказываю регулярно – качество стабильно на высоте!
букеты томск
Дешевое такси Болгария Служба Такси Эклипс предлагает доступные трансферы из аэропортов Бургас, Варна, София и Белград до популярных курортов Болгарии. Встреча с табличкой, помощь с багажом и комфортная поездка до места назначения. Обслуживаем Солнечный Берег, Созополь, Банско и другие направления. Для групп от 5 человек – специальные условия!
Ищете интернет магазин автозапчастей в Минске с выгодными ценами? Посетите https://belautoparts.by/ где вы найдете существенный выбор для все автомобилей с доставкой по всей Беларуси. Подберите запчасти по VIN, марке авто, номеру детали, артикулу, каталогу в иллюстрации. У нас также есть все для ТО – расходники, масла, фильтра и многое другое. В нашем интернет магазине более 30 млн запчастей как оригинальных, так и качественных заменителей. Ознакомьтесь на сайте подробнее!
На сайте https://astro-magic.com/ воспользуйтесь искусственным интеллектом на русском языке. Этот сервис предоставляет возможность использовать все функции в режиме реального времени. При этом нет необходимости подключать VPN, искусственный интеллект работает и без него. Воспользоваться им сможет каждый желающий, абсолютно бесплатно. Chat GPT сможет создать качественный контент, произвести анализ изображений, быстро отыскать нужную информацию. Искусственный интеллект сможет и написать программный код.
HQD King’s smooth throat hit is perfect for all-day vaping.
https://paintingsofdecay.net/index.php/Vape_21U
OzibarVape reviews pointed me to HQD King—great call!
https://oeclub.org/index.php/User:OuidaGunter6
Приобрести диплом об образовании!
Мы изготавливаем дипломы любых профессий по невысоким ценам— [url=http://freediplom.com/kupit-diplom-vuza-s-zaneseniem-v-reestr-6/]freediplom.com/kupit-diplom-vuza-s-zaneseniem-v-reestr-6/[/url]
Мы готовы предложить дипломы любой профессии по доступным тарифам. Всегда стараемся поддерживать для заказчиков адекватную ценовую политику. Важно, чтобы документы были доступны для большинства наших граждан.
Покупка документа, который подтверждает окончание ВУЗа, – это разумное решение. Заказать диплом о высшем образовании: [url=http://10000diplomov.ru/diplom-kupit-provedennij/]10000diplomov.ru/diplom-kupit-provedennij/[/url]
либет казино
Online casinos need tools—support us! plinko
либет казино, голд казино
Olymp Casino Online’s game updates keep it fresh every month.
olymp casino
На сайте https://boostclicks.ru/ вы сможете ознакомиться с рекомендациями, ценными и важными советами про API интеграцию, скрипты, а также любопытные сервисы из мира трафика. Для того чтобы вам было удобней ориентироваться, все материалы поделены на разделы, что позволит отыскать то, что действительно актуально и интересно в данный момент. Регулярно на портале появляется новый, интересный контент на данную тему. Имеется и список тех статей, которые пользуются особой популярностью. Все они созданы лучшими и высококлассными экспертами с огромным опытом.
aviator mostbet [url=www.svstrazh.forum24.ru/?1-18-0-00000136-000-0-0-1743260517]www.svstrazh.forum24.ru/?1-18-0-00000136-000-0-0-1743260517[/url] .
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
С широким ассортиментом продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Плоская мишень AlN для распыления
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наша продукция:
Плоская мишень Fe+Mn
The live chat wait time at Olymp Casino Online was short—great service!
olymp casino
Ищете, в каком месте срочно оформить заём, не теряя время на отказы? На сайте [url=https://all-credit.ru/]новые мфо займ[/url] собраны актуальные МФО, которые реально одобряют заявки всем — от 18 лет и всего по документу. Даже если у вас плохая кредитная история или были просрочки, вы найдёте подборку займов, созданную именно под это. Всё доступно, в срок и без подводных камней.
Актуальное обновление 2025 года — детальный рейтинг займов под залог ПТС, и при этом автомобиль остаётся у вас. А ещё мы собрали полезные материалы, которые помогут стабилизировать ваше финансовое положение без дополнительных затрат. Выбирайте [url=https://all-credit.ru/]займы онлайн на карту быстро[/url] с почти мгновенным ответом, онлайн-доступом 24/7 и максимальными шансами на получение денег уже через несколько минут.
Бесплатные Steam аккаунты https://t.me/GGZoneSteam/ с играми и бонусами. Проверенные логины и пароли, ежедневное обновление, удобный поиск. Забирай свой шанс на крутой аккаунт без лишних действий!
Компания AVTO197 предлагает услуги аренды автомобилей в Москве, обеспечивая клиентов широким выбором транспортных средств для любых ситуаций. В автопарке https://avtocar5.ru/ представлены модели эконом, среднего и бизнес классов, а также минивэны и коммерческие автомобили. Все машины застрахованы по КАСКО и ОСАГО, что гарантирует безопасность и надежность. Процесс оформления аренды занимает всего 15–20 минут с минимальным пакетом документов. Для постоянных клиентов предусмотрены гибкие системы скидок. Дополнительно компания предоставляет услуги такси и аренду автомобилей для свадебных мероприятий.
На сайте https://axonbusiness.ru обсудите свой проект для того, чтобы воспользоваться популярными и эффективными решениями компании «A.X.O.N.». Прямо сейчас вы сможете заказать звонок, чтобы узнать стоимость вашего задания. Здесь получится заказать, в том числе, стратегический бизнес-консалтинг, а также готовые ИТ-решения. Лучшие специалисты оптимизируют и автоматизируют все ваши бизнес-процессы, клиентский сервис. Для вас будут разработаны индивидуальные решения для получения большей прибыли.
The welcome offers at online casinos are hard to resist! plinko
The mobile casino jams—fix it up! marvel casino
РедМетСплав предлагает внушительный каталог качественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица изделия подтверждена соответствующими документами, подтверждающими их качество. Опытная поддержка – то, чем мы гордимся – мы на связи, чтобы улаживать ваши вопросы а также адаптировать решения под требования вашего бизнеса.
Доверьте вашу потребность в редких металлах специалистам РедМетСплав и убедитесь в множестве наших преимуществ
Наша продукция:
РўСЂСѓР±Р° магниевая MI2 – JIS H 2150 Пруток магниевый MI2 – JIS H 2150 является высококачественным материалом, используемым РІ различных отраслях, включая авиационную Рё автомобильную промышленность. Его РЅРёР·РєРёР№ вес Рё отличная прочность делают его идеальным выбором для конструкций, требующих надежности Рё легкости. Купить Пруток магниевый MI2 – JIS H 2150 означает инвестировать РІ долговечность Рё эффективность ваших проектов. Благодаря уникальным свойствам магния, этот РїСЂРѕРґСѓРєС‚ обеспечивает отличную РєРѕСЂСЂРѕР·РёРѕРЅРЅСѓСЋ стойкость. РќРµ упустите возможность улучшить СЃРІРѕРё разработки СЃ помощью данного материала.
РедМетСплав предлагает обширный выбор высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица товара подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Дружелюбная помощь – наш стандарт – мы на связи, чтобы разрешать ваши вопросы по мере того как предоставлять решения под особенности вашего бизнеса.
Доверьте ваш запрос специалистам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
поставляемая продукция:
Магний ISO-MgAl4Si – ISO 16220 Магний ISO-MgAl4Si – ISO 16220 – это уникальный сплав, разработанный для высоких стандартов прочности Рё легкости. РћРЅ используется РІ автомобилестроении, аэрокосмической промышленности Рё РґСЂСѓРіРёС… высокотехнологичных отраслях. Данный материал обладает отличной РєРѕСЂСЂРѕР·РёР№РЅРѕР№ стойкостью Рё хорошей обрабатываемостью, что делает его идеальным выбором для создания надежных Рё долговечных изделий. Если РІС‹ ищете качественный металл, чтобы улучшить производственные процессы, РЅРµ упустите шанс купить Магний ISO-MgAl4Si – ISO 16220. Ртот сплав поможет вам достичь новых вершин РІ ваших проектах.
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с прекрасным качеством обслуживания.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наша продукция:
Дюралевый швеллер 440151 22С…35С…6.5С…2.5С…2.5 РјРј Р”19С‡ ГОСТ 13623-90 Купить дюралевый швеллер РѕС‚ производителя. Прочный Рё легкий материал для различных областей строительства. РЁРёСЂРѕРєРёР№ выбор размеров Рё профилей. Устойчивость Рє РєРѕСЂСЂРѕР·РёРё Рё надежность конструкций. Рдеальное решение для авиации, судостроения, автомобилестроения, производства спортивного оборудования Рё фармакологических предприятий.
РедМетСплав предлагает внушительный каталог отборных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их происхождение. Дружелюбная помощь – то, чем мы гордимся – мы на связи, чтобы улаживать ваши вопросы и находить ответы под особенности вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
Наша продукция:
Лист магниевый РњРђ6Р¦3 Лента магниевая РњРђ6Р¦3 представляет СЃРѕР±РѕР№ высококачественный РїСЂРѕРґСѓРєС‚, идеальный для применения РІ различных отраслях. РћРЅР° обладает отличной теплопроводностью Рё высокой РєРѕСЂСЂРѕР·РёР№РЅРѕР№ стойкостью, что делает её незаменимой РІ производстве Рё строительстве. Рспользование магниевой ленты улучшает характеристики соединений, повышая РёС… долговечность. Если РІС‹ хотите обеспечить надежность Рё эффективность ваших проектов, купить Лента магниевая РњРђ6Р¦3 станет отличным решением. Выберите этот РїСЂРѕРґСѓРєС‚ для достижения наилучших результатов РІ своей работе.
На сайте http://promkomplektregion96.ru закажите оптовые поставки металлопроката. Он пригодится как в сфере промышленности, так и строительства. Многие из представленных позиций вы сможете приобрести прямо сейчас, а другие – только под заказ. В каталоге находятся фитинги и трубы, выполненные из полиэтилена, черный прокат, нержавеющий, цветной прокат. Есть возможность оплатить приобретение несколькими вариантами. Доступна оперативная доставка по всей России. Воспользуйтесь профессиональными консультациями.
[url=https://www.vwbk.de/url?q=https%3A%2F%2Fmedsonline365.online]pharmacies from Canada[/url] Patient medicine info. Drug leaflet available. pharmacy technician online
Ландшафтный дизайн под ключ https://greenartstudio.ru/ – от Грин Арт Студио это услуги профессионалов от проектирования до реализации и авторского надзора. Посетите наш сайт – посмотрите реализованные проекты – они вам обязательно понравятся, а также ознакомьтесь со всеми нашими услугами, начиная от проектирования и заканчивая уходом за вашим садом, благоустройством участка. Мы работаем с частными лицами, госучреждениями и коммерческими компаниями.
Доска объявлений https://estul.ru/blog по всей России: продавай и покупай товары, заказывай и предлагай услуги. Быстрое размещение, удобный поиск, реальные предложения. Каждый после регистрации получает на баланс аккаунта 100? для возможности бесплатного размещения ваших объявлений
I won $500 online last week—best feeling ever! hot hot fruit
The payout waits online lag—push it! plinko
The demo mode at Olymp Casino Online helped me test games risk-free.
olymp casino
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наши товары:
Высокочистая мишень для распыления олова
Online bingo is a chill vibe—great crowd! minas
Olymp Casino Online’s roulette tables are my go-to every weekend. olymp casino
Ищете изготовление наружной рекламы в Москве? Посетите сайт https://neon-mix.ru/ – мы изготавливаем световые вывески, объёмные буквы, рекламные конструкции и многое другое. Полный цикл — от производства до монтажа по самым выгодным ценам. Реализуем проекты любой сложности, учитываем пожелания заказчика и предлагаем честные, конкурентные цены. Ознакомьтесь со всеми услугами и ценами на сайте.
На сайте https://waltzprof.com/ получится заказать обратный звонок для того, чтобы приобрести продукцию собственного производства от компании «Валцпроф». Она производит стальной профиль безупречного качества. Он используется для создания фасадных, противопожарных конструкций и иного предназначения. Также в компании получится приобрести и вспененный уплотнитель, выпадающие пороги, профиль фиксации стекла. Для производства продукции эталонного качества применяются только передовые технологии.
The blackjack at Olymp Casino Online feels fair—had a good run last night. olymp casino
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
поставляемая продукция:
Медная крестовина под пайку 21х18х0.9 мм 8х10 мм твердая пайка М2 ГОСТ Р52922-2008 Узнайте о высококачественных медных крестовинах под пайку от Редметсплав. Гарантия надежности, прочности, отличной теплопроводности и простоты монтажа. Широкий выбор размеров и конфигураций для любых электрических соединений.
Online casinos should guide—too steep! plinko
[url=https://maps.google.com.ua/url?q=https%3A%2F%2Fmedsonline365.online]good best online pharmacy[/url] Drug information here. Patient drug leaflet. best best online pharmacy
Olymp Casino Online’s loyalty rewards are worth sticking around for.
olymp casino
Visit the largest and most interesting travel blog https://www.atravel.blog/ where you will find interesting facts about different countries of the world, as well as get acquainted with delicious national dishes based on authentic recipes from around the world. Choose an interesting category for you or read the latest events from the world of travel and food!
Are online casino ratings legit?—not sure! plinko
Заказать диплом университета!
Мы можем предложить документы институтов, расположенных в любом регионе России.
[url=http://diplomt-v-samare.ru/kupit-diplom-v-reestre-legko-i-bistro-onlajn/]diplomt-v-samare.ru/kupit-diplom-v-reestre-legko-i-bistro-onlajn/[/url]
РедМетСплав предлагает внушительный каталог высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица продукции подтверждена требуемыми документами, подтверждающими их качество. Превосходное обслуживание – наш стандарт – мы на связи, чтобы ответить на ваши вопросы и предоставлять решения под требования вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
Наши товары:
Полоса магниевая G-A6Z1Y4 – AFNOR NF A57-705 Порошок магниевый G-A6Z1 – AFNOR NF A65-717 является высококачественным продуктом, который находит широкое применение РІ различных отраслях. Ртот порошок обладает отличными физико-химическими свойствами Рё соответствует всем требованиям стандартов AFNOR NF A65-717. РћРЅ обеспечивает надежную работу РІ высокотемпературных условиях Рё РїРѕРґС…РѕРґРёС‚ для использования РІ металлургии, электронике Рё РґСЂСѓРіРёС… промышленных сферах. Если РІС‹ ищете эффективное решение для ваших производственных РЅСѓР¶Рґ, купите Порошок магниевый G-A6Z1 – AFNOR NF A65-717 Рё убедитесь РІ его превосходном качестве.
I’m curious about Olymp Casino Online’s RNG—hope it’s fair!
olymp casino
На сайте https://1abakan.ru/forum/showthread-275275/ изучите всю интересную, исчерпывающую информацию, которая касается популярного онлайн-клуба «New Retro Casino», которое позволит получить положительный опыт. Специально для вас особые игровые решения, которые помогут вдоволь насладиться процессом и получить от жизни больше приятных эмоций. Официальный сайт предоставляет возможность ознакомиться с огромным количеством слотов, а также видеоигр. Здесь каждый найдет игру на свой вкус. Вас ожидает огромное количество акций, интересных предложений.
I hit a dip online—pause now! ganesha gold
[url=https://maps.google.com.ly/url?sa=t&url=https%3A%2F%2Fmedsonline365.online]uk pharmacy online[/url] Medication impacts described. Comprehensive pill guide. best online pharmacy tech program
ролевые шторы [url=http://rulonnye-shtory-s-elektroprivodom99.ru]ролевые шторы[/url] .
Современный подход к созданию рефератов предлагает нейросеть — инструмент, позволяющий студентам за считанные минуты получать уникальные и грамотно структурированные тексты. Вместо самостоятельного поиска информации и её длительной обработки, учащиеся могут сразу использовать готовые материалы для решения учебных задач любой сложности. Высвободившееся благодаря этому время студенты направляют на углубление знаний и совершенствование аналитических способностей Реферат написать нейронов
Les plateformes de paris sportifs francaises proposent principalement trois categories de bonus de bienvenue, chacune presentant des avantages specifiques selon votre profil de parieur https://share.evernote.com/note/029e470c-db83-ac0f-7d64-3ee860561263
Автозапчасти в Минске и всей Беларуси с доставкой или забрать в пункте выдачи, на сайте https://pyatnitsa.by/ – это возможность выгодно купить запчасти для любого автомобиля. Подбор запчастей по VIN или авто – огромный каталог, низкие цены. Все в наличие. Ознакомьтесь с нашим каталогом – от расходников и материалов для ТО, до подвески, системы охлаждения, электрики и многое другое.
На сайте https://smartporog.ru/ изучите каталог, чтобы приобрести умные выпадающие пороги. Они идеально подходят для дверей различного типа. Автоматические пороги считаются идеальным решением в том случае, если невозможна установка обычного порога. Такие конструкции комфортны в использовании, эффективно борются с преградой в полу, а также сквозняками, мостиками холода. Пороги такого типа не допускают попадания внутрь посторонних запахов, повышают звукоизоляцию. Также пороги защищают от проникновения внутрь насекомых.
Эта статья сочетает познавательный и занимательный контент, что делает ее идеальной для любителей глубоких исследований. Мы рассмотрим увлекательные аспекты различных тем и предоставим вам новые знания, которые могут оказаться полезными в будущем.
Подробнее – https://podcast.ruhr/toporzysek
На сайте https://pohudetlegko.ru/ представлена исчерпывающая, актуальная и полезная информация о том, как похудеть правильно, без вреда для организма и максимально эффективно. На сайте вы найдете вдохновляющие истории, советы звезд, которые выглядят младше своего возраста. Есть рецепты блюд, которые созданы из полезных, вкусных ингредиентов. Но при этом они помогут скинуть вес и привести себя в форму к летнему сезону. Рецепты простые и быстрые, а потому справиться с приготовлением пищи сможет каждый. Описаны и упражнения, обертывания для похудения.
I wish online casinos had more transparency about their algorithms. bizzo casino
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Обладая обширной линейкой редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наша продукция:
Медный цилиндр 5N
Ученые узнали, в какое время суток кофе заметно продлевает жизнь
https://pin.it/72MctQ7nd
Новое исследование, опубликованное в European Heart Journal, выявило, что люди, предпочитающие пить кофе утром, могут иметь более низкий риск смертности, особенно от сердечно-сосудистых заболеваний. Это ставит под вопрос не только количество употребляемого кофе, но и его время как фактор, влияющий на здоровье.
The rewards online rock—nice touch! blue wizard
Visit the site https://findprompt.shop/ which is a trading platform and marketplace for buying and selling prompts for Midjourney, Stable Diffusion, ChatGPT and others. Explore the best AI prompts. A huge selection of prompts of various directions at the most favorable prices. Visit the catalog and see for yourself our significant offer.
Online casinos should block—fair go! casino kingdom
[url=https://www.google.tg/url?q=https%3A%2F%2Fmedsonline365.online]pharmacy tech programs online[/url] Pill effects explained. Patient drug guide. viagra best online pharmacy
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние достижения.
Наша продукция:
Плоская мишень Cu+Ni+Ti
РедМетСплав предлагает широкий ассортимент высококачественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их качество. Дружелюбная помощь – наша визитная карточка – мы на связи, чтобы разрешать ваши вопросы и находить ответы под особенности вашего бизнеса.
Доверьте вашу потребность в редких металлах специалистам РедМетСплав и убедитесь в множестве наших преимуществ
поставляемая продукция:
Пруток молибденовый Mo-La Пруток молибденовый Mo-La – это высококачественный РїСЂРѕРґСѓРєС‚, предназначенный для применения РІ различных отраслях, включая машиностроение Рё электронику. Его выдающиеся механические свойства Рё коррозионная стойкость делают его идеальным выбором для сложных условий работы. РЎ помощью молибденовых прутков РјРѕР¶РЅРѕ значительно улучшить характеристики конечных изделий. Если РІС‹ ищете надежный Рё долговечный материал, то вам стоит купить Пруток молибденовый Mo-La. Ртот пруток соответствует всем современным стандартам качества Рё надежности.
Get +200 points for free using the promo code Nodepay AI: nodepay referral code. Install extensions and farm points for free in your browser. Complete tasks and get additional points.
I wish online kept old—love that! marvel casino
Приветствую всех, наша команда создала не официальный канал Jozz Casino. Полный обзор сайта Джоз, приветственных подарков, бездепозитных бонусов и VIP Системы. Присоединяйся сейчас и получи 100 фриспинов за регистрацию. Jozz работает по лицензии Кюрасао. Информация на канале регулярно обновляется Подписывайтесь на канал: @casino_jozz , Ссылка: https://t.me/casino_jozz
На сайте https://serialexpress.ru в огромном многообразии представлены телесериалы, мультсериалы, любопытные, интересные теленовеллы. Все фильмы находятся в отличном качестве, отличаются четкой картинкой, качественным звуком. Прямо сейчас изучите весь ассортимент фильмов, которые вы сможете заказать по разумным ценам. Есть раздел, в котором находятся хиты продаж – их выбирает большинство. Приобрести понравившийся фильм получится в пару кликов и любое удобное время. Регулярно появляются интригующие новинки, с которыми будет интересно ознакомиться и вам.
установить рулонные шторы цена [url=http://rulonnye-shtory-s-elektroprivodom99.ru]установить рулонные шторы цена[/url] .
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
поставляемая продукция:
Кольцо РёР· драгоценных металлов серебряное 150С…3С…2 РјРј РЎСЂ99.99 РўРЈ РЁРёСЂРѕРєРёР№ выбор высококачественных кольц РёР· драгоценных металлов. Рлегантные украшения, созданные талантливыми мастерами. Уникальность Рё изысканность РІ каждой детали. Возможность заказа персонального кольца РїРѕ вашим пожеланиям. Подчеркните СЃРІРѕСЋ индивидуальность СЃ нашими кольцами.
РедМетСплав предлагает обширный выбор высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы обеспечиваем быстрое выполнение вашего заказа.
Каждая единица продукции подтверждена соответствующими документами, подтверждающими их соответствие стандартам. Опытная поддержка – то, чем мы гордимся – мы на связи, чтобы улаживать ваши вопросы по мере того как предоставлять решения под требования вашего бизнеса.
Доверьте вашу потребность в редких металлах специалистам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
Фольга магниевая SISMg4637-00 – SS 144637 РўСЂСѓР±Р° магниевая SISMg4637-00 – SS 144637 является надежным решением для различных промышленных Рё технических применений. Обладая великолепными механическими свойствами Рё устойчивостью Рє РєРѕСЂСЂРѕР·РёРё, этот материал обеспечивает долговечность Рё эффективность. Рспользование магниевых труб позволяет снизить вес конструкций Рё улучшить РёС… эксплуатационные характеристики.Если РІС‹ ищете высококачественное решение, чтобы повысить производительность вашего проекта, купите РўСЂСѓР±Р° магниевая SISMg4637-00 – SS 144637 Рё оцените ее преимущества сами. Рто отличное сочетание цены Рё качества, которое РЅРµ оставит вас равнодушными.
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с прекрасным качеством обслуживания.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
Наши товары:
Пружина из драгоценных металлов палладиевая 5х1х1 мм ПДСР-40 ТУ Выберите идеальные пружины из золота, серебра или платины, чтобы дополнить ваше украшение. Найдите пружины с изысканным дизайном и прочностью. Они предлагают широкий выбор для того, чтобы подчеркнуть уникальность вашего украшения.
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – идеальный вариант для вас.
Наши товары:
Пружина из драгоценных металлов палладиевая 50х3х0.6 мм ПдСр60-40 ТУ Выберите идеальные пружины из золота, серебра или платины, чтобы дополнить ваше украшение. Найдите пружины с изысканным дизайном и прочностью. Они предлагают широкий выбор для того, чтобы подчеркнуть уникальность вашего украшения.
The slot sounds online rock—pure fun! fortune dragon
Thank you for the post, i will wait your next post!
Online casino ads are relentless—enough already! plinko
The music online lifts—keep on! mines
[url=https://medsonline365.online/#]cheap viagra online canadian pharmacy[/url] Medicine guide available. Pill facts provided. uk pharmacy online
На сайте https://mvpol.ru/ воспользуйтесь возможностью заказать промышленные полы напрямую от производителя. Компания «МВПОЛ» оказывает и такую важную услугу, как ремонт промышленных полов, производство полимерных наливных полов, бетонных, у которых упрочненный верх. Также получится узнать расценки на такие услуги, чтобы заранее спланировать бюджет. Для того чтобы иметь представление о том, как выглядят работы, изучите объекты. На все услуги предоставляются гарантии. Вся информация дается максимально быстро.
Welcome to the official Joy Casino blog https://officialjoycasino.net/ — a place where you can explore the fascinating world of online gambling from different perspectives: game mechanics, psychology, responsible gaming and industry analytics. If you are looking for a deep dive into gaming strategies, casino technology or responsible gaming tips, you have come to the right place.
На сайте https://vezuviy.shop/ воспользуйтесь возможностью приобрести качественное печное оборудование, а также дымоходы. На все распространяются гарантии. Здесь вы сможете приобрести и аксессуары, которые гарантированно совместимы со всем оборудованием. Доставка осуществляется абсолютно бесплатно. Клиент сможет получить в качестве презента банный камень. На все аксессуары действует скидка 10%. Все печи выполнены из качественных, высокотехнологичных материалов, потому прослужат долгое время.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наши товары:
Высокочистые гранулы молибдена
The slot themes online shine—play fun! aviator game
Online casinos should give more free play! Gioco Del Pollo
На сайте https://sto812.com ознакомьтесь с телефоном “СТО ПРИМОРСКИЙ”, где вы сможете воспользоваться техническим обслуживанием, заказать ремонт выхлопной системы, ДВС, рулевого управления, системы охлаждения, трансмиссии, тормозной системы, ходовой. Оплатить услугу получится так, как удобно – картой, наличными, по QR-коду. Без очереди происходит обслуживание корпоративных клиентов. Сотрудники компании окажут помощь с выбором запчастей. К преимуществам обращения в компанию относят то, что все услуги высокого качества, на них установлены разумные расценки.
Интернет-магазин саженцев Мартин-Сад https://www.martin-sad.ru/ это интернет-магазин питомника растений. Посмотрите наш каталог с выгодными ценами, в нем вы найдете более 9500 сортов. В каталоге вы найдете – хвойные, деревья, плодовые, вьющиеся растения, кустарники, многолетники и многое другое. Посетите сайт и вы обязательно найдете необходимые для себя растения и семена.
На сайте https://169.ru/ вы сможете зарезервировать как межкомнатные, так и входные двери, напольные покрытия, раздвижные двери и многое другое. Вы сможете самостоятельно рассчитать то, какое количество строительных материалов вам понадобится для того, чтобы реализовать свой проект. Каждая вещь является олицетворением стиля и привлекательного дизайна. Здесь постоянно устраиваются распродажи, что позволит приобрести все, что нужно по лучшей цене. Очень часто здесь проходят акции. Предлагается огромный выбор сервисов и услуг.
Яндекс Такси: работа для водителей. Узнайте все о классификации автомобилей, требованиях и получите полный справочник таксопарков России. Советы начинающим таксистам на сайте https://igormylnikovchannel.ru/
8 (800) 550-37-68
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
поставляемая продукция:
Вольфрамовая пластина 20x12x5 мм Т15К6 ГОСТ 25426-90 Выберите вольфрамовые пластины для точной и эффективной обработки различных материалов. Широкий спектр применения, высокая твердость, прочность и термическая стойкость делают их идеальным выбором для производства инструментов, аэрокосмической, медицинской и электронной промышленности.
На сайте https://play.google.com/store/apps/details?id=com.testme.maketest сделайте ставки в популярном, надежном заведении «Фонбет», которое порадует своими честными выплатами. Однако играть здесь смогут только те, кому уже исполнилось 18. Здесь огромный ассортимент игр, включая региональные, а потому вы точно будете знать, как интересно развлечься и провести время с пользой, ведь вы сможете еще и неплохо заработать. Вы сможете выбрать комбинированные ставки, общие, индивидуальные тоталы, форы.
резина michelin [url=https://www.proalbea.ru/shiny-michelin-preimushhestva-i-nedostatki.html]резина michelin[/url] .
cheap prandin price
can you get cheap valtrex without insurance buying generic valtrex price can you get cheap valtrex pill
can you get valtrex without insurance cost generic valtrex without insurance buying cheap valtrex pill
how to buy valtrex without rx
where can i get valtrex without prescription cost of valtrex price where can i get cheap valtrex tablets
can i get generic valtrex can you get valtrex without rx where buy valtrex pill
[url=https://medsonline365.online/#]canadian online pharmacies[/url] Recent medicine developments. Patient medicine resource. best online pharmacy forum
I love the online twists—keep fresh! jackpotraider
Самые новые анекдоты шутки — коротко, метко и смешно! Подборка актуального юмора: от жизненных до политических. Заходи за порцией хорошего настроения!
План эвакуации – важнейший документ, указывающий расположение эвакуационных выходов http://malahov-consultant.ru/pozharnyy-risk/
Kent Casino is a popular online platform that attracts players with profitable bonuses, promotions and promo codes. If you would like to increase your budget and get additional benefits, Kent Casino promo code high roller is what you need http://cccr.moibb.ru/viewtopic.php?f=2&t=2399
На сайте https://profstroygroupp.ru/ подберите проект для того, чтобы заказать строительство дома из кирпича. К вашим услугам оперативный вызов на объект для проведения всех необходимых работ. Проект разрабатывается с участием заказчика. Каждый клиент получает возможность воспользоваться комфортной системой скидок, выгодными предложениями. Работы выполняются на основании договора, на них даются гарантии. Для того чтобы получить исчерпывающую информацию по поводу строительства и использования новых технологий, почитайте тематические статьи.
лидер
На сайте https://vezuviy.shop/ вы сможете приобрести чугунные, стальные банные печи, нержавеющие, а также в облицовке, печи-камины, отопительные, каминные топки, дымоходы, порталы и многое другое. Вся продукция от производителя, а потому наценки очень маленькие. Такая техника считается идеальной не только с целью обогрева помещения, но и оформления пространства. А самое главное, что она отличается долгим сроком службы, надежностью из-за того, что в работе использовались высокотехнологичные, проверенные материалы.
I’m off online—shady risk! betclic
шиномантаж химки [url=https://hondahybrid.ru/forum/topic/2886-kakie-uslugi-okazyvaet-shinomontazh//]https://hondahybrid.ru/forum/topic/2886-kakie-uslugi-okazyvaet-shinomontazh//[/url] .
На сайте http://officepro54.ru представлено огромное количество мебели, которая идеально подходит для организации офисного пространства. Вся она выполнена из инновационных, уникальных материалов, а потому прослужит очень долго, не синтезирует в воздух опасных веществ. В разделе вы найдете кабинет руководителя, функциональные и вместительные столы для переговоров, шкафы-купе, сейфы, акустические системы, ученическую мебель и многое другое. Постоянно действуют акции. На продукцию установлены привлекательные цены.
Посетите сайт https://addpets.ru/ – это экспертный блог о том, как ухаживать за домашними животными, как их кормить и какие породы наиболее популярны в России. Вы найдете исчерпывающую информацию о каждом животном, какие у них бывают болезни и как их лечить, познавательную и просто интересную информацию о домашних питомцах. Присоединитесь к обсуждению или присылайте свои интересные статьи!
На сайте https://market-delivery-dubai.ru/ вы сможете воспользоваться такой полезной услугой, как доставка алкоголя в Дубае. В разделе вы найдете такие напитки, как: бризер, абсент, водка, виски, ликер, пиво, коньяк и многое другое. Вся продукция является сертифицированной, качественной, а потому идеально подойдет для долгожданного события, праздника. Есть последние поступления вкусного и ароматного вина, которое вы сможете приобрести на торжество или для того, чтобы скрасить вечер. Доставка происходит в минимальные сроки.
The online thrill strikes—stay steady! dragon hatch
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Обладая обширной линейкой редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наши товары:
Мишень для распыления сплава AlCu
where to get cheap calan online
where to get generic zyrtec price how to get generic zyrtec pill how to get generic zyrtec pills
where can i get zyrtec without insurance how can i get cheap zyrtec without dr prescription cheap zyrtec without insurance
where to buy zyrtec no prescription
cost cheap zyrtec price get zyrtec no prescription how to buy zyrtec
cost of cheap zyrtec without rx how to get generic zyrtec tablets where to buy generic zyrtec without rx
Кладбища Видного https://bulatnikovskoe.ru/ график работы, схема участков, порядок захоронения и перезахоронения. Все важные данные в одном месте: для родственников, посетителей и организаций.
На сайте https://belarus-blok.ru/ вы сможете заказать газосиликатные блоки, которые были произведены в Беларуси. Приобрести продукцию получится в один клик и по привлекательной стоимости. Напротив каждого варианта указаны технические характеристики, расценки, что позволит быстрее сориентироваться в выборе. Газобетонные блоки пользуются огромной популярностью благодаря тому, что отличаются компактностью, небольшим весом. К тому же, этот материал негорючий. Есть возможность приобрести перегородочные, стеновые газоблоки лучших белорусских производителей.
michelin agilis crossclimate [url=http://www.proalbea.ru/shiny-michelin-preimushhestva-i-nedostatki.html/]michelin agilis crossclimate[/url] .
Казино Trix — это современное онлайн-казино, предлагающее широкий ассортимент игр, включая слоты, покер и настольные игры. Платформа известна своим дружелюбным интерфейсом и простотой навигацией. Пользователи могут наслаждаться качественной графикой и звуковыми эффектами, создавая незабываемую игровую атмосферу играть
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
Наши товары:
Титановая труба 83х3.2х2000 мм ПТ7М ГОСТ 22897-86 Откройте мир прочных и надежных титановых труб с компанией Редметсплав. Наши высококачественные титановые трубы сочетают в себе устойчивость к коррозии, износостойкость и легкий вес, делая их незаменимым материалом для применения в различных сферах промышленности. Широкий выбор различных диаметров и толщин, а также индивидуальные решения для вашего производства. Гарантированное качество и надежность поставок.
I love the live roulette—so live! fruit cocktail
Посетите Интернет-магазин автозапчастей АВТО-ЕВРО – https://autoeuro.ru/ и вы сможете купить запчасти для иномарок оптом и в розницу. Поберите автозапчасти по VIN или марке авто или ознакомьтесь с нашим каталогом, в нем представлен лучший выбор запчастей по выгодным ценам. Работаем со всеми регионами России. Подробнее на сайте.
РедМетСплав предлагает внушительный каталог качественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица продукции подтверждена соответствующими документами, подтверждающими их качество. Дружелюбная помощь – наш стандарт – мы на связи, чтобы ответить на ваши вопросы и предоставлять решения под требования вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наша продукция:
Магний GA8A Магний GA8A – эффективная добавка для поддержания вашего Р·РґРѕСЂРѕРІСЊСЏ. Ртот РїСЂРѕРґСѓРєС‚ способствует нормализации обмена веществ Рё улучшению работы сердечно-сосудистой системы. Магний является важным микроэлементом, участвующим РІ множестве биохимических процессов организма. Если РІС‹ ищете СЃРїРѕСЃРѕР± повысить уровень энергии Рё уменьшить стресс, то купить Магний GA8A – отличное решение. РћРЅ помогает восстановить баланс РїСЂРё повышенных физических Рё эмоциональных нагрузках. РќРµ упустите возможность заботиться Рѕ своем Р·РґРѕСЂРѕРІСЊРµ СЃ Магний GA8A.
Online casinos should cap—guard play! fortune tiger
На сайте https://stakecasino.tech почитайте про популярное и проверенное онлайн-заведение «Stake Casino». Здесь также имеется и букмекерская контора, где вы сможете сделать свои ставки. Вам обязательно понравится интерфейс, а пользоваться им очень просто. Вас обрадуют бонусы, а также различные акции. А все данные надежно защищены, потому информация точно не попадет злоумышленникам. Каждую неделю организуются турниры, а также розыгрыши. Для каждого желающего доступна мобильная версия, а также зеркало.
На сайте https://ooomila.com изучите каталог такого оборудования, которое предназначено для организации животноводческих комплексов. Также вы сможете почитать и содержательную информацию о компании и ее достижениях. В каталоге вы найдете следующее оборудование: технологические линии, весовое оборудование, резиновые маты, крематоры и многое другое. На технику, которая находится в разделе, действуют гарантии. Несколько сотен единиц оборудования всегда находятся на складе, можно приобрести под заказ.
шиномантаж сергиев посад [url=https://www.hondahybrid.ru/forum/topic/2886-kakie-uslugi-okazyvaet-shinomontazh/]https://www.hondahybrid.ru/forum/topic/2886-kakie-uslugi-okazyvaet-shinomontazh/[/url] .
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым текущие трудности превращаются в завтрашние успехи.
Наша продукция:
Ниобиевый тигель высокой чистоты
Используйте AstoMagic – бесплатную онлайн-нейросеть. Задайте вопрос, и вы за пару секунд нужный ответ получите. ИИ ваш стиль общения анализирует и подстраивается под него. Получайте в написании кода помощь и ошибок исправлении. https://astomagic.com – тут ответы на часто задаваемые вопросы собрали. На ресурсе тарифы и отзывы пользователей представлены. Общайтесь голосом – система распознает речь и выдает ответы в текстовом виде. ИИ информацию анализирует и точные данные предоставляет. Опробуйте уже сейчас нейросеть онлайн, не упустите такую возможность!
The sound of coins dropping in online slots is so satisfying! plinko
Thank you! waiting your next post!
I don’t trust online RNG—feels off! teen patti
На сайте https://delivery-dubai.com/ закажите такие алкогольные напитки в Дубае, как: ром, коньяк, ликер, бризер, шампанское, виски, пиво, вино и многое другое. Здесь находится только качественная продукция, которую вы сможете заказать в любом количестве. Алкоголь подходит для любого праздника, в том числе, Дня рождения, юбилея, отпуска. Вы можете выбрать несколько видов продукта, чтобы скрасить вечер, провести его со второй половинкой. Курьер прибудет в ближайшее время, чтобы передать вам заказ.
can i buy exforge without prescription
where can i buy generic inderal without dr prescription where buy cheap inderal cost of generic inderal without prescription
cost inderal prices where can i buy inderal price buy cheap inderal without prescription
where can i buy cheap inderal no prescription
where can i get cheap inderal prices how to buy inderal without prescription can i buy inderal
can i buy inderal online can i order generic inderal pills where buy cheap inderal
I lost $100 in an online casino—lesson learned, set a budget! pirots 2
go to my blog [url=https://them-rril-lynch.net]merrill edge login[/url]
I love the online calm—no buzz! plinko
https://telegra.ph/No-Gamstop-Casino-Insights-and-Alternatives2-03-27
https://telegra.ph/Reviewed-Non-Gamstop-Casinos-for-Players-in-2023-03-27
РедМетСплав предлагает обширный выбор высококачественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы обеспечиваем быстрое выполнение вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их соответствие стандартам. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы разрешать ваши вопросы а также находить ответы под требования вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
Наши товары:
Пруток магниевый AZ-91E – РўРЈ 1714-001-00545484-96 Проволока магниевая AZ-91E – РўРЈ 1714-001-00545484-96 является идеальным решением для тех, кто ищет легкий Рё прочный материал. РћРЅР° широко используется РІ машиностроении Рё авиастроении благодаря СЃРІРѕРёРј отменным свойствам. Ртот легкий сплав обладает высокой прочностью Рё хорошей РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью, что делает его незаменимым РІ различных условиях эксплуатации. Если РІС‹ хотите улучшить качество СЃРІРѕРёС… изделий Рё снизить РёС… вес, тогда вам стоит купить Проволока магниевая AZ-91E – РўРЈ 1714-001-00545484-96 РїСЂСЏРјРѕ сейчас. Ртот РїСЂРѕРґСѓРєС‚ станет отличным выбором для вашего производства.
I lost $100 in an online casino—lesson learned, set a budget! mines
Заказываю только у вас – знаю, что будет безупречно!
цветы томск
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
поставляемая продукция:
Проволока из серебряных припоев ПСрО3-97 0.8 мм ГОСТ 19746-2015 Выберите высококачественную проволоку из серебряных припоев на сайте Редметсплав.рф. Мы предлагаем материал с высокой электропроводностью, отличной прочностью и устойчивостью к окислению, идеально подходящий для различных отраслей промышленности. Узнайте больше о нашей продукции и услугах прямо сейчас!
view publisher site [url=https://them-rril-lynch.net/]merrill lynch[/url]
Full Article [url=https://them-rril-lynch.net/]merrill lynch[/url]
I love the online calm—no buzz! vincispin
where can i buy cheap provera price
I don’t trust online odds—odd feel! betonred
Medicines information leaflet. Long-Term Effects.
how can i get cheap phenytoin without dr prescription
Everything trends of medicine. Read here.
казино онлайн Мир казино – это захватывающая вселенная, где переплетаются азарт, стратегия и технологические инновации. Онлайн казино предлагают широкий спектр игр, от классических слотов до захватывающих настольных игр, предоставляя игрокам возможность испытать удачу и применить свои навыки. Стратегии играют ключевую роль в успехе. Будь то блэкджек, покер или рулетка, понимание правил и умение анализировать ситуацию могут значительно повысить шансы на выигрыш. Важно помнить о разумном управлении банкроллом и осознанном подходе к игре.
На сайте https://rustreetwear.ru вы найдете огромное количество интересных, разнообразных и эксклюзивных брендов, которые создают роскошную уличную одежду на любые случаи жизни. Есть как совсем не известные марки, которые только начинают свой путь, так и гиганты мировой индустрии. Перед вами самый впечатляющий каталог, где вы найдете не только одежду, но и аксессуары. Каталог обновляется ежедневно, чтобы вы обязательно нашли для себя лучший вариант. Здесь установлены доступные расценки.
The online flow is wild—stay sharp! plinko
На сайте https://bitovayatechnika.blogspot.com/2025/03/blog-post.html изучите полезную, актуальную информацию, которая касается сплит-систем и того, как правильно их выбрать домой либо в офис. Эти функциональные и умные устройства нацелены на то, чтобы поддержать приятную температуру в помещении. Но для того, чтобы воспользоваться всеми функциями, необходимо правильно выбрать устройство. К важным преимуществам такой техники относят то, что она является энергоэффективной, работает практически бесшумно.
https://telegra.ph/No-Gamstop-UK-Casinos-Explained-in-Detail-03-27
https://telegra.ph/Reliable-Non-Gamstop-Casinos-for-Safe-Gaming-Experience1-03-27
https://telegra.ph/Guide-to-Non-Gamstop-UK-Casinos-for-Players-03-27
Visit https://itprof.tech/ and you will learn everything about VPN – how to set up for different services, learn the best VPN for streaming, which VPN are best for gaming in 2025 and much more. Our resource offers useful and up-to-date information, news about VPN and how to work with it. We have structured our content so that each user can easily find answers to their questions.
Мы можем предложить дипломы любой профессии по приятным ценам. Дипломы изготавливаются на подлинных бланках Быстро купить диплом ВУЗа [url=http://diplomk-v-krasnodare.ru/]diplomk-v-krasnodare.ru[/url]
Где приобрести диплом по актуальной специальности?
Заказать диплом института по невысокой стоимости возможно, обратившись к проверенной специализированной фирме.: [url=http://magazin-diplomov.ru/]magazin-diplomov.ru[/url]
Etsitko hyodyllista tietoa lainoista? Vieraile osoitteessa https://esimerkkilinkki.fi/ – josta loydat kattavaa tietoa asuntolainasta, vinkkeja lainan hakemiseen, lainojen vertailuun ja paikkaan, kuinka saada laina heti ilman luottotietoja tai luottohistoriaa ja paljon muuta. Hyodyllista tietoa sinulle verkkosivuillamme.
замена рулевого кардана [url=www.4-x-4.ru/remont-i-balansirovka-kardannyh-valov/]замена рулевого кардана[/url] .
мастбет [url=http://mostbet6009.ru/]http://mostbet6009.ru/[/url] .
https://telegra.ph/New-No-Deposit-Bonuses-at-Non-Gamstop-Casinos-2023-03-27
Блок Маркет предлагает высококачественный строительный материал. Купить газобетонные блоки в нашей компании выгодно. Мы заслужили доверие клиентов. Получаем доброжелательные многочисленные отзывы. Если нужно мы относительно выбора продукции вас проконсультируем. https://belarus-blok.ru – сайт, где можете ознакомиться с условиями доставки газобетона. Также тут можно онлайн-калькулятором для расчета нужного количества газоблоков воспользоваться. Стремимся к созданию долговечных отношений с каждым покупателем.
На сайте https://deliverydubaidrinks.com/ изучите каталог алкогольной продукции, которая представлена здесь в огромном ассортименте, а потому получится приобрести в любом количестве такие напитки, как: ром, ликер, бризер, виски. Регулярное обновление ассортимента, чтобы вы получили возможность попробовать все напитки, которые вас заинтересовали, за время отпуска. Если появились вопросы, то задайте их менеджеру, который подберет для вас оптимальное решение на любое событие. Вся продукция реализуется по доступным ценам.
Medication information for patients. Drug Class.
what is in omeprazole
All information about drug. Read here.
На сайте https://play.google.com/store/apps/details?id=com.testme.maketest вы сможете сделать ставки в популярной и надежной БК «Фонбет». Здесь вы сможете получить не только определенную сумму в качестве выигрыша, но и положительные, приятные эмоции от удачно проведенной сделки. В этой БК принимаются ставки на самые разные виды спорта. Особый интерес представляют и киберспортивные мероприятия. Есть возможность пополнить счет как наличными при помощи терминала, так и в режиме реального времени.
На сайте https://d600.ru/ вы сможете заказать тротуарную плитку Steingot и Braer от производителя по доступным ценам. Всегда в наличии полный ассортимент, который хранится на складе, а потому его можно приобрести уже сейчас. Вся продукция уже готова к отправке через ЖД либо авто, независимо от региона. Вы сможете воспользоваться услугами персонального менеджера. Есть возможность оплатить покупку по факту получения. Перед приобретением вы сможете изучить технические характеристики, а также расценки, чтобы приобрести самое выгодное решение.
buying tadacip without dr prescription buying generic tadacip without prescription can you get generic tadacip pills
how can i get cheap tadacip for sale can you buy generic tadacip without rx where buy tadacip prices
can i purchase tadacip prices
how to buy generic tadacip without prescription buying generic tadacip without prescription can you buy generic tadacip for sale
can you get generic tadacip online how to get tadacip where buy tadacip pills
buy cheap nimotop no prescription
https://telegra.ph/Non-Gamstop-Casinos-with-NetEnt-Games-03-27
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние возможности.
Наша продукция:
Плоская мишень для распыления кобальта
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наша продукция:
Гранулы магния 3N5
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым текущие трудности превращаются в завтрашние успехи.
Наша продукция:
Керамическая мишень Limn2O4 (3N)
I love the low bets online—soft go! tome of madness
https://telegra.ph/Guide-to-Trusted-Non-Gamstop-Casinos-for-Players-03-27
Meds information sheet. Brand names.
get cheap colchicine without dr prescription
Best about meds. Read here.
На сайте https://autopiter.kg/ получится приобрести как оригинальные, так и неоригинальные запчасти, комплектующие для ТО, автохимию и автомасла, аккумуляторы, все для спецтехники. Представлен и каталог ГАЗ, ВАЗ, КАМАЗ. Есть возможность подобрать фильтр для автомобиля. Также представлены и автолампочки. Изучите раздел с рекомендуемыми товарами. Для того чтобы подобрать необходимую деталь, нужно воспользоваться поиском – в строке указать название детали. Все запчасти хранятся на складе и в нужном количестве.
https://telegra.ph/PayPal-Non-Gamstop-Casinos-in-the-UK-2023-Guide1-03-27
РедМетСплав предлагает широкий ассортимент отборных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы обеспечиваем оперативное исполнение вашего заказа.
Каждая единица продукции подтверждена требуемыми документами, подтверждающими их качество. Дружелюбная помощь – то, чем мы гордимся – мы на связи, чтобы улаживать ваши вопросы и адаптировать решения под требования вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наша продукция:
Лист висмутовый C89831 – CDA Лист висмутовый C89831 – CDA – это высококачественный материал, обладающий отличными техническими характеристиками. РћРЅ широко применяется РІ индустрии, включая электронику Рё производство сплавов. Висмутовый лист имеет уникальные антикоррозионные свойства, что обеспечивает его долговечность. Благодаря своей высокой плотности Рё РЅРёР·РєРѕР№ токсичности, этот материал является идеальным выбором для различных проектов. Если вас интересует качественный материал, РЅРµ упустите возможность купить Лист висмутовый C89831 – CDA РїРѕ конкурентной цене.
РедМетСплав предлагает обширный выбор высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица продукции подтверждена всеми необходимыми документами, подтверждающими их происхождение. Дружелюбная помощь – наша визитная карточка – мы на связи, чтобы улаживать ваши вопросы и предоставлять решения под особенности вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
Полоса магниевая MC1 – JIS H 5203 Порошок магниевый MB6 – JIS H 4203 представляет СЃРѕР±РѕР№ высококачественный материал, используемый РІ различных отраслях, включая металлообработку Рё производство легких сплавов. Ртот порошок обладает отличной текучестью Рё высокой реакцией РЅР° технологические процессы, что делает его незаменимым для РјРЅРѕРіРёС… промышленных приложений. Покупая Порошок магниевый MB6 – JIS H 4203, РІС‹ выбираете надежность Рё эффективность РІ работе. Его уникальные характеристики обеспечивают улучшенные свойства конечного продукта, включая прочность Рё легкость. Для вашего бизнеса этот порошок станет отличным решением. РќРµ упустите возможность сделать качественный выбор!
РедМетСплав предлагает обширный выбор отборных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица товара подтверждена требуемыми документами, подтверждающими их качество. Опытная поддержка – то, чем мы гордимся – мы на связи, чтобы ответить на ваши вопросы а также находить ответы под особенности вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в множестве наших преимуществ
поставляемая продукция:
Порошок магниевый РњРђ8ЦБ – РўРЈ 1714-018-00545484-97 Рзделия РёР· магния РњРђ8ЦБ – РўРЈ 1714-018-00545484-97 представляют СЃРѕР±РѕР№ высококачественные компоненты, отличающиеся прочностью Рё легкостью. РћРЅРё идеально РїРѕРґС…РѕРґСЏС‚ для применения РІ авиационной Рё автомобильной индустрии. Магний – это материал, который обеспечивает отличные механические свойства Рё РєРѕСЂСЂРѕР·РёРѕРЅРЅСѓСЋ стойкость, что делает изделие надежным выбором. Наши изделия соответствуют строгим стандартам качества, что гарантирует долгий СЃСЂРѕРє службы Рё эффективность РІ эксплуатации. РќРµ упустите возможность улучшить СЃРІРѕРё производственные процессы, РєСѓРїРёРІ Рзделия РёР· магния РњРђ8ЦБ – РўРЈ 1714-018-00545484-97. Заказывайте сейчас Рё оцените РІСЃРµ преимущества использования магниевых изделий!
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
поставляемая продукция:
Медная крестовина под пайку 27.4х23х0.9 мм 9х11 мм твердая пайка М1РМ ГОСТ 32590-2013 Узнайте о высококачественных медных крестовинах под пайку от Редметсплав. Гарантия надежности, прочности, отличной теплопроводности и простоты монтажа. Широкий выбор размеров и конфигураций для любых электрических соединений.
[url=https://1winru.xyz/]бонусы 1вин[/url] – лаки джет 1win, 1win ru
mostbet chrono [url=http://mostbet6009.ru]http://mostbet6009.ru[/url] .
can i get generic finasteride without dr prescription where can i get finasteride without dr prescription buy topical finasteride online
order cheap finasteride prices buying generic finasteride without prescription where can i buy finasteride in the uk
finasteride lotion side effects
buy finasteride 5mg cvs hair loss forums topical finasteride where to buy can i purchase generic finasteride pill
where can i buy cheap finasteride price where to buy cheap finasteride tablets can i buy generic finasteride no prescription
where to get finast no prescription
Meds prescribing information. Generic Name.
how to buy generic pulmicort online
Everything what you want to know about drug. Read here.
На сайте https://fguard.ru/ почитайте статьи на самую разную тему. К примеру, кибермошенничество, для каких целей используется сеточка, которая на двери микроволновки. Здесь представлена вся нужная информация о смарт-часах и о том, чем они отличаются от бизнес-браслетов. Для того чтобы подыскать нужную информацию, воспользуйтесь специальным рубрикатором. На сайте вы найдете записи, которые касаются нейросетей, гаджетов. Представлены и интересные рекомендации, которые будут необходимы каждому. Имеются данные и про технологии.
Online casinos can hook—edge it! ganesha gold
Услуга срочного выкупа автомобилей https://www.buying-car.ru/ в любом состоянии является идеальным решением для тех, кто хочет быстро и без лишних хлопот избавиться от своего транспортного средства. Предлагаем вам выгодные условия, они позволяют получить деньги в кратчайшие сроки, независимо от года выпуска, пробега или технического состояния автомобиля. Наша команда специалистов готова оценить ваш авто быстро и профессионально. Даже если ваше транспортное средство было повреждено в ДТП, не функционирует или имеет серьезные технические неисправности. Процесс выкупа прост и удобен. Наши эксперты проведут быструю оценку стоимости, и если она вас устроит, мы готовы заключить сделку в тот же день. Мы также предоставляем услуги по бесплатному эвакуатору, если ваше авто не на ходу, что позволяет вам избежать дополнительных трат.
ремонт карданов [url=4-x-4.ru/remont-i-balansirovka-kardannyh-valov/]ремонт карданов[/url] .
Current link BlackSprut Marketplace 2025 bs2best
Look, for quite a long time I was interested in a question about Payout models in the MostBet affiliate program, so that you do not have any problems I can safely say that now the best conditions are on this site https://www.as-tu-vu.com/forum/viewtopic.php?p=822319#822319
https://telegra.ph/Non-Gamstop-Casino-Bonuses-Explained-and-Compared-03-27
https://telegra.ph/Play-Lightning-Roulette-at-Non-Gamstop-Online-Casinos1-03-27
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым текущие трудности превращаются в завтрашние достижения.
Наша продукция:
Плоская мишень Cofeb
Ищете интернет магазин автозапчастей в Минске с выгодными ценами? Посетите https://belautoparts.by/ где вы найдете существенный выбор для все автомобилей с доставкой по всей Беларуси. Подберите запчасти по VIN, марке авто, номеру детали, артикулу, каталогу в иллюстрации. У нас также есть все для ТО – расходники, масла, фильтра и многое другое. В нашем интернет магазине более 30 млн запчастей как оригинальных, так и качественных заменителей. Ознакомьтесь на сайте подробнее!
Благодаря финансовому блогу Апостолиди у вас есть возможность к ценным рекомендациям получить доступ. Мы делимся личным опытом, простым языком объясняем сложные темы. Гарантируем достоверность и актуальность информации. Вы узнаете, как новый торговый счет на Forex открыть. Ищете конвертер валют? – тут имеются по финансовым вопросам авторские статьи. Вам необходимо авторизоваться для отправки комментария. Материалы разделены по темам, что упрощает поиск необходимой информации. Изучайте уже сейчас блог Апостолиди, который является полезным. Финансовая грамотность – это очень легко и просто!
Юнитал-М – учебный центр, который компетентные услуги предоставляет. Мы постоянно к совершенству стремимся и с ответственностью к своей деятельности относимся. Гарантируем добросовестность в выполнении своих обязательств, неравнодушие к разным вопросам и качественный сервис. https://www.unitalm.ru – портал, где мы о том расскажем, как существенно на рабочем месте увеличение квалификации для сотрудников и компании. Материал в понятной форме показан, педагогический состав к обучению располагает. Вместе мы высочайших результатов достигнем!
На сайте https://fabvia.ru/spicesguide/ почитайте полезную, содержательную информацию на тему приправы, специй и того, как правильно все это употреблять, чтобы вкус максимально раскрылся, вы получили от еды пользу. Этот портал идеально подойдет для тех, кто работает в пищевой промышленности, увлекается кулинарией. Информация поможет расширить свои знания в этой сфере. Вы научитесь правильно сочетать ингредиенты и создавать вкусные блюда. В скором времени вы получите возможность воспользоваться калькулятором, который научит сочетать ингредиенты, добавлять нужное количество специй.
Online casinos can pull—set edge! plinko
Бонусы без первого депозита в букмекерских конторах: советы
Бонусы БК без первого депозита – как не ошибиться
Мировая практика показывает, что тысячи игорных площадок предлагают пользователям различные привилегии. Легкие финансовые поступления могут стать дополнительным стимулом для новых игроков. Но как найти действительно интересные предложения среди множества доступных вариантов? Четкое понимание условий и механизмов акций поможет вам строить стратегию и извлекать максимальную выгоду.
При выборе интересных предложений важно обращать внимание на требования к ставкам и ограниченные сроки использования. Некоторые компании предоставляют возможность провести анализ рынка, чтобы выявить лучшие варианты для старта без начальных вложений. Таким образом, ваши шансы на успех в числе первых увеличиваются.
Следует отметить, что разные платформы могут устанавливать уникальные параметры для активов. Это разнообразие создает потенциал для сравнения и выбора наиболее выгодных условий. Секрет заключается в глубоких знаниях и критическом подходе к каждой акции, что поможет избежать распространенных ошибок и недоразумений.
Как выбрать привлекательного букмекера без вступительного взноса
Обратите внимание на размер доступного бонуса без депозита. Чем больше сумма бонуса, тем выгоднее предложение для игрока. Также стоит изучить условия отыгрыша бонусных средств. Чем ниже вейджер (коэффициент отыгрыша), тем проще будет выполнить требования по отыгрышу.
Немаловажным аспектом является ассортимент развлечений на площадке. Хороший букмекер предлагает не только ставки на спорт, но и другие азартные развлечения, такие как онлайн-казино, покер, live-дилеры и прочее. Это расширяет возможности для игры и получения прибыли.
Анализируйте репутацию букмекера, его надёжность и финансовую устойчивость. Отдавайте предпочтение известным брендам с многолетней историей. Это гарантирует сохранность ваших средств и своевременные выплаты выигрышей.
Как максимизировать использование стартовых предложений для выигрыша
Следующий шаг – это анализ спортивных событий. Рассматривайте матчи с предсказуемыми результатами. Чаще всего это крупные турниры, где достаточно статистики для обоснованных прогнозов. Исследуйте историю противостояний команд, текущую форму игроков и любые высокочные факторы, которые могут повлиять на результат.
Стоит также учесть типы ставок, которые вы используете. Лучше всего выбирать простые исходы, например, победа одной из команд или ничья. Это снизит риски и увеличит шансы на успех. Старайтесь избегать сложных многосоставных ставок, где больше неопределенности.
Не забывайте о коэффициентах. Сравните предложенные коэффициенты разных платформ перед ставкой. Убедитесь, что использовали наилучшие варианты для повышения потенциального выигрыша. Полезно также следить за изменениями котировок в режиме реального времени, так как это может помочь в принятии решения.
Финансовое планирование играет ключевую роль. Установите себе лимиты: сколько вы готовы потратить на ставки. Это поможет избежать необдуманных решений и управления рисками. Поставьте перед собой цель и не отклоняйтесь от нее, даже если изначальные результаты будут неудачными.
Feel free to surf to my homepage; https://t.me/s/bonusy_bk_bez_depozita
Medicine information for patients. Short-Term Effects.
cheap geodon no prescription
Actual trends of drugs. Read information here.
Компания «КАРДЕКС» https://card-oil.ru/ — надежный партнер на рынке топливных решений, работающий более 12 лет. Специализируется на выпуске и обслуживании топливных карт для юридических лиц и индивидуальных предпринимателей. Преимущество КАРДЕКС — доступ к обширной сети из более чем 13000+ АЗС по всей России, включая федеральные и региональные заправочные станции. Это позволяет клиентам заправляться без привязки к конкретному бренду, оптимизируя расходы и логистику. Топливные карты КАРДЕКС обеспечивают удобный контроль затрат, предоставляют детальную аналитику и помогают экономить за счет персонализированных условий и скидок. Компания зарекомендовала себя как стабильный и технологичный игрок, предлагающий инновационные решения для бизнеса.
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
Наши товары:
Кольцо РёР· драгоценных металлов золотое 3С…2С…0.1 РјРј ЗлСрМ75-12.5 РўРЈ РЁРёСЂРѕРєРёР№ выбор высококачественных кольц РёР· драгоценных металлов. Рлегантные украшения, созданные талантливыми мастерами. Уникальность Рё изысканность РІ каждой детали. Возможность заказа персонального кольца РїРѕ вашим пожеланиям. Подчеркните СЃРІРѕСЋ индивидуальность СЃ нашими кольцами.
The performance is top-notch—no lag at all.
amneziawg vps
taxiburgasa.ru Служба Такси Эклипс предлагает доступные трансферы из аэропортов Бургас, Варна, София и Белград до популярных курортов Болгарии. Встреча с табличкой, помощь с багажом и комфортная поездка до места назначения. Обслуживаем Солнечный Берег, Созополь, Банско и другие направления. Для групп от 5 человек – специальные условия!
[url=https://1winru.xyz]1win приложение[/url] – 1 официальный сайт 1win, 1win на андроид
copd exacerbation prevention online resources what happens with copd exacerbation complications of copd
early detection of copd copd risk factors wheezing in copd
copd exacerbation prevention symptom tracking techniques
copd exacerbation prevention early detection technologies copd exacerbation triggers copd exacerbation recovery
lung cancer risk in copd copd exacerbation prevention medication management copd exacerbation prevention support services
РедМетСплав предлагает внушительный каталог отборных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы обеспечиваем оперативное исполнение вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их соответствие стандартам. Превосходное обслуживание – наш стандарт – мы на связи, чтобы ответить на ваши вопросы и находить ответы под специфику вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в множестве наших преимуществ
Наша продукция:
Рзделия РёР· висмута C99720 – UNS Рзделия РёР· висмута C99720 – UNS представляют СЃРѕР±РѕР№ высококачественные материалы, обладающие уникальными физико-химическими свойствами. Рти изделия используются РІ различных отраслях, включая электронику Рё медицину, благодаря своей превосходной проводимости Рё РЅРёР·РєРѕР№ токсичности. Подавляемый эффект излучения делает РёС… незаменимыми РІ СЂСЏРґРµ специализированных приложений. Если вас интересует надежность Рё долговечность, РІС‹ можете купить Рзделия РёР· висмута C99720 – UNS, гарантируя себе высокую производительность Рё безопасность РІ работе.
На сайте http://www.tribal-tattoo.ru уточните телефон тату-салона, в котором вы сможете выполнить художественную татуировку, перекрыть шрамы, рубцы, выполнить роскошный перманентный макияж. Есть возможность выполнить рисунок различной сложности, техники и любой цветовой гаммы. Каждая идея клиента будет реализована. При необходимости будет предложена своя. К вашим услугам создание индивидуальных эскизов. Все услуги обойдутся по привлекательным расценкам. В салоне работают компетентные, знающие специалисты, которые работают специально для вас.
buy seroquel no prescription
I won $300 on a slot—still stoked! minas
Бонусы БК: Механика работы и правила использования
Как работают бонусы БК – разбор механики
Современные любители азартных развлечений не могут представить свою жизнь без щедрых поощрений от букмекерских контор. Эти привлекательные привилегии способны радикально повлиять на геймплей и в конечном итоге – на размер выигрыша. Но для того, чтобы в полной мере воспользоваться предложениями, необходимо тщательно изучить условия их получения и использования.
В данной статье мы разберем наиболее распространенные бонусные предложения от крупнейших операторов, а также раскроем ключевые особенности их активации и отыгрывания. Вооружившись этими знаниями, вы сможете с максимальной эффективностью применять бонусные средства и существенно увеличить свои шансы на успех!
Будьте готовы к увлекательному путешествию по миру поощрительных программ букмекерских контор. Вас ждут практические рекомендации от опытных игроков, которые помогут вам наслаждаться азартом по максимуму!
Начисление и активация бонусов в букмекерских компаниях
Начисление бонусов в букмекерских компаниях происходит за выполнение определенных условий. Как правило, это регистрация нового игрового аккаунта, первый депозит или совершение ставок на определенную сумму. Размер бонуса зависит от выбранного предложения и может составлять от 100% до 200% от внесенных средств.
Для активации бонуса игроку необходимо выполнить ряд требований. Чаще всего это оборот бонусной суммы в установленном количестве раз, в течение определенного периода времени. Например, бонус в 1000 рублей нужно прокрутить 10 раз на ставках с коэффициентом от 1.5 в течение 30 дней.
Частые ошибки при использовании акций и способы их избежать
Многие игроки допускают распространенные ошибки при применении акционных предложений букмекерских компаний, что в итоге может свести на нет все преимущества этих выгодных возможностей. Рассмотрим наиболее распространенные промахи и дадим практические советы, как их предотвратить.
Одна из распространенных ошибок – несоблюдение правил отыгрыша бонусных средств. Игроки часто забывают о необходимости делать определенное количество ставок или совершать ставки на события с минимальными коэффициентами, прописанными в условиях акции. Несоблюдение этих требований может привести к аннулированию бонуса.
Еще один распространенный просчет – ставки на события с высокими рисками в попытке как можно быстрее отыграть бонус. Такой подход крайне ненадежен и может привести к быстрой потере бонусных средств. Вместо этого лучше распределять ставки по различным событиям с умеренными коэффициентами.
Нередко игроки также забывают о сроках действия акционных предложений. Все условия, включая периоды активности бонусов, четко прописаны в правилах, и их нарушение влечет за собой аннулирование поощрения. Внимательно изучайте регламент перед участием.
Подводя итог, чтобы успешно пользоваться акциями букмекерских контор, необходимо тщательно изучать условия, соблюдать правила отыгрыша, избегать высокорисковых ставок и не забывать о сроках действия предложений. Следуя этим простым рекомендациям, вы сможете извлечь максимальную выгоду из акционных программ.
Also visit my website … https://t.me/s/bookmaker_bonuses
AI Bitcoin recovery solution
Всегда считал, что покупка диплома о высшем образовании — это миф и невозможно. Но, к счастью, оказался неправ. Сначала искал информацию по теме: купить диплом экономиста, купить аттестаты за 11 класс отзывы, сколько стоит купить аттестат за 9 класс, купить диплом учителя, купить диплом о среднем образовании в тюмени, а затем переключился на дипломы вузов. Подробности здесь: [url=http://proffdiplomik.com/udostoverenie-o-povyshenii-kvalifikatsii/]proffdiplomik.com/udostoverenie-o-povyshenii-kvalifikatsii[/url]
[url=https://gamble-spot.com/]казино онлайн без регистрации[/url] – онлайн казино софт, зеркала онлайн казино
Medicine prescribing information. Long-Term Effects.
generic cozaar without prescription
Actual trends of medication. Get now.
купить среднее образование [url=https://diplomys-vsem.ru/]купить среднее образование[/url] .
На сайте https://alkomarket-dubai.ru/ воспользуйтесь возможностью заказать свои любимые алкогольные напитки с доставкой по Дубаю. Курьер приедет к вам по любому указанному адресу и привезет заказ точно в срок, без опозданий, а потому вы сможете начать вечеринку в любое, наиболее комфортное для себя время. Вы сможете заказать напитки у самого надежного, проверенного поставщика, который торгует только проверенным товаром, а потому исключены некачественные напитки. Есть возможность приобрести как крепкий алкоголь, так и легкий, например, пиво.
The mobile casino layout needs work—too messy! aviator
Мы можем предложить дипломы любой профессии по доступным ценам. Преимущества приобретения документов у нас
Вы покупаете документ в надежной и проверенной компании. Такое решение сэкономит не только средства, но и время.
На этом преимущества не заканчиваются, их куда больше:
• Документы делаем на оригинальных бланках со всеми печатями;
• Дипломы любых учебных заведений России;
• Цена значительно меньше той, которую пришлось бы заплатить на очном обучении в университете;
• Удобная доставка как по Москве, так и в другие регионы Российской Федерации.
Заказать диплом университета– [url=http://avtovideotest.ru/dokumentyi-dlya-uspeshnoy-kareryi-bez-lishnih-voprosov/]avtovideotest.ru/dokumentyi-dlya-uspeshnoy-kareryi-bez-lishnih-voprosov[/url]
I won big online—can’t stop smiling! plinko
get cipro without insurance buy cipro 500 mg cost cipro prices
how can i get generic cipro tablets order cipro without rx where buy generic cipro for sale
can i order cipro without prescription
can i get cheap cipro prices can you get cipro online cipro hc otic buy
order cipro pills buying cheap cipro buy cipro online paypal
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наша продукция:
Оксид гафния (HfO2) гранулированный
can i purchase valtrex
Medicines prescribing information. Effects of Drug Abuse.
can i order xenical pill
Best information about drug. Get information here.
The payout waits online lag—push it! mines
продажа аккаунтов marketpleys-akkauntov
AI-powered Bitcoin finder
Ищете изготовление наружной рекламы в Москве? Посетите сайт https://neon-mix.ru/ – мы изготавливаем световые вывески, объёмные буквы, рекламные конструкции и многое другое. Полный цикл — от производства до монтажа по самым выгодным ценам. Реализуем проекты любой сложности, учитываем пожелания заказчика и предлагаем честные, конкурентные цены. Ознакомьтесь со всеми услугами и ценами на сайте.
When you have claimed the welcome bonus offer, it is however very important that you go through the terms and conditions of the 1x welcome bonus https://fisketavling.nu/cb-profile/pluginclass/cbblogs?action=blogs&func=show&id=697
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наша продукция:
Керамическая мишень для распыления диоксида кремния
шатры и тенты аренда москва [url=https://www.shatry-dlya-meropriyatiy.ru]https://www.shatry-dlya-meropriyatiy.ru[/url] .
РедМетСплав предлагает обширный выбор отборных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица продукции подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Опытная поддержка – наш стандарт – мы на связи, чтобы улаживать ваши вопросы по мере того как находить ответы под специфику вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наша продукция:
РљСЂСѓРі кобальтовый РРџ635 РљСЂСѓРі кобальтовый РРџ635 – это надежный инструмент для высококачественной обработки металлов. РћРЅ обеспечивает идеальную шлифовку Рё долговечность благодаря использованию кобальтовой СЃРІСЏР·РєРё, что значительно увеличивает СЃСЂРѕРє службы. РљСЂСѓРі отлично РїРѕРґС…РѕРґРёС‚ для работы СЃ различными стальными сплавами, включая нержавеющую сталь.Приобретая этот РєСЂСѓРі, РІС‹ получаете инструмент, который справится даже СЃ самым сложным металлом. РљСЂСѓРі кобальтовый РРџ635 отличается высокой производительностью Рё отличным качеством обработки. РќРµ упустите шанс купить РљСЂСѓРі кобальтовый РРџ635 для вашего производства Рё улучшите качество работы!
Looking for flower delivery in Ukraine? Visit https://ukraineflora.com/ where you will find the largest selection of fresh flowers and exclusive gifts. Look through the catalog and you will definitely find not only luxurious flowers and bouquets, but also gift baskets, perfumes, balloons and even cakes.
Pills information. Short-Term Effects.
can i purchase cheap clomid prices
Actual about pills. Get here.
If you want to increase your gaming budget or get additional benefits, then Gama Casino promo codes are what you need https://naigle.borda.ru/?1-4-0-00003040-000-0-0-1743695230
https://pq.hosting/arenda-vps-vds-s-docker
buying cheap cipro prices where to get generic cipro without rx can i buy cipro price
where buy generic cipro price get cheap cipro without rx buy cipro online overnight
where can i buy generic cipro online
epididymitis treatment ciprofloxacin how to get cheap cipro online where to get cheap cipro without dr prescription
can i buy cheap cipro pills buying generic cipro for sale order generic cipro for sale
generic glucotrol without dr prescription
РедМетСплав предлагает внушительный каталог высококачественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы обеспечиваем быстрое выполнение вашего заказа.
Каждая единица изделия подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Дружелюбная помощь – то, чем мы гордимся – мы на связи, чтобы ответить на ваши вопросы и предоставлять решения под требования вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
Полоса висмутовая C89550 – UNS Полоса висмутовая C89550 – UNS представляет СЃРѕР±РѕР№ уникальный металлический РїСЂРѕРґСѓРєС‚, обладающий высокими антикоррозионными свойствами Рё РЅРёР·РєРѕР№ теплопроводностью. Рто идеальный выбор для применения РІ электронике Рё медицинских устройствах, РіРґРµ важны весовые характеристики Рё устойчивость Рє агрессивным средам. Благодаря СЃРІРѕРёРј свойствам, полоса висмутовая C89550 – UNS обеспечивает надежность Рё долговечность РІ эксплуатации. Если РІС‹ хотите купить Полоса висмутовая C89550 – UNS, воспользуйтесь нашим предложением, чтобы получить качественный материал для СЃРІРѕРёС… РЅСѓР¶Рґ.
Здравствуйте!
Для многих людей, приобрести [b]диплом[/b] университета – это необходимость, удачный шанс получить достойную работу. Впрочем для кого-то – это осмысленное желание не терять время на учебу в ВУЗе. Что бы ни толкнуло вас на такой шаг, мы готовы помочь. Быстро, качественно и недорого сделаем диплом нового или старого образца на подлинных бланках со всеми необходимыми печатями.
Ключевая причина, почему многие люди покупают документы, – желание занять хорошую работу. Допустим, навыки и опыт позволяют кандидату устроиться на работу, а подтверждения квалификации нет. При условии, что работодателю важно присутствие “корочек”, риск потерять хорошее место достаточно высокий.
Купить документ о получении высшего образования вы сможете в нашем сервисе. Мы предлагаем документы об окончании любых университетов РФ. Вы получите необходимый диплом по любой специальности, любого года выпуска, в том числе документы образца СССР. Гарантируем, что при проверке документа работодателем, каких-либо подозрений не появится.
Ситуаций, которые вынуждают приобрести диплом о среднем образовании очень много. Кому-то срочно нужна работа, и необходимо произвести впечатление на начальство при собеседовании. Другие задумали устроиться в престижную компанию, для того, чтобы повысить собственный статус в социуме и в последующем начать собственное дело. Чтобы не тратить попусту годы жизни, а сразу начать удачную карьеру, применяя врожденные таланты и полученные навыки, можно купить диплом в интернете. Вы сможете быть полезным в социуме, обретете финансовую стабильность в кратчайшие сроки- [url=http://rdiplomans.com/]аттестат за 9 класс купить[/url]
[url=https://gamble-spot.com/igrovye-avtomaty/]онлайн казино с выводом денег[/url] – играющие казино онлайн, казино онлайн на деньги
Medicines information sheet. Generic Name.
get cheap valtrex prices
Best what you want to know about meds. Read information here.
Добрый день!
Без института очень сложно было продвинуться вверх по карьерной лестнице. В последние годы документ не дает никаких гарантий, что получится найти престижную работу. Более важное значение имеют профессиональные навыки специалиста, а также его постоянный опыт. Именно из-за этого решение о заказе диплома стоит считать целесообразным. Приобрести диплом о высшем образовании [url=http://rsfsr.flybb.ru/viewtopic.php?f=2&t=664/]rsfsr.flybb.ru/viewtopic.php?f=2&t=664[/url]
шатер в аренду тентовые конструкции [url=http://www.shatry-dlya-meropriyatiy.ru]http://www.shatry-dlya-meropriyatiy.ru[/url] .
аттестат купить спб
Приветствую!
Мы изготавливаем дипломы любой профессии по доступным ценам. Стоимость будет зависеть от конкретной специальности, года выпуска и образовательного учреждения: [url=http://diplomanc.com/]diplomanc.com/[/url]
На сайте https://nenovost.com/ почитайте любопытные и интересные новости, представленные на самую разную тему: бизнес, автомобили, медицина, кулинария, общество, недвижимость, спецтехника, туризм и отдых. Все статьи составлены специалистами, потому вы можете на них положиться, ведь вся информация является достоверной, качественной. Здесь постоянно публикуются новые материалы на самую разную тему. Все они сопровождаются картинками для большей наглядности. Заходите на портал регулярно, чтобы найти что-то нужное и полезное.
гарантия при продаже аккаунтов заработок на аккаунтах
На сайте https://xn--80aaaaaaxhx2a2ai2af3a8grd.xn--p1ai/ воспользуйтесь услугой, связанной с арендой шатров, которая подходит для любого мероприятия, в том числе, Дня рождения, юбилея, свадьбы. Аренда сделает любое мероприятие ярким, незабываемым. В компании работают проверенные, компетентные специалисты, которые выполнят все необходимые работы, включая установку, расстановку мебели. Имеется огромный выбор снаряжения, которое применяется для кемпинга. Шатер выполнен из современных, качественных материалов, поэтому порадует своим внешним видом.
can i get aceon prices
hwid spoofer crack hwid spoofer free hwid spoofer hwid spoofer download hwid spoofer crack
The online bonuses shine—jump in! dragon tiger game
Visit the site https://findprompt.shop/ which is a trading platform and marketplace for buying and selling prompts for Midjourney, Stable Diffusion, ChatGPT and others. Explore the best AI prompts. A huge selection of prompts of various directions at the most favorable prices. Visit the catalog and see for yourself our significant offer.
Les nouveaux joueurs de 1xbet peuvent beneficier d’un bonus de bienvenue allant jusqu’a 130€ grace au code promo 1X200BIG https://webhitlist.com/profiles/blogs/c-digo-promocional-1xbet-apuesta-gratis-bono-hasta-130
Les programmes de fidelite des bookmakers offrent des avantages croissants selon l’activite du parieur https://publicenemypresspage.site/2025/04/04/%d0%bf%d0%be%d0%ba%d0%b5%d1%80%d0%b4%d0%be%d0%bc-%d0%bf%d1%80%d0%be%d0%bc%d0%be%d0%ba%d0%be%d0%b4-%d0%bd%d0%b0-%d0%b4%d0%b5%d0%bf%d0%be%d0%b7%d0%b8%d1%82-%d0%ba%d0%b0%d0%ba-%d0%bf%d0%be%d0%bb%d1%83/
Meds prescribing information. Drug Class.
can i purchase ramipril without prescription
All about medication. Get here.
Paint Protection Films
Заказать диплом о высшем образовании!
Мы можем предложить документы институтов, расположенных в любом регионе РФ.
[url=http://vacshidiplom.com/visshee-obrazovanie-kupit-diplom-s-zaneseniem-3/]vacshidiplom.com/visshee-obrazovanie-kupit-diplom-s-zaneseniem-3/[/url]
Купить диплом о высшем образовании!
Мы предлагаем документы любых учебных заведений, расположенных на территории всей России.
[url=http://asxdiploman.com/kupite-diplom-visshego-obrazovaniya-s-zaneseniem-v-reestr-4/]asxdiploman.com/kupite-diplom-visshego-obrazovaniya-s-zaneseniem-v-reestr-4/[/url]
[b]Диплом любого ВУЗа РФ![/b]
Без наличия диплома сложно было продвинуться вверх по карьерной лестнице. Поэтому решение о заказе диплома стоит считать мудрым и целесообразным. Заказать диплом о высшем образовании [url=http://fulrp.5nx.ru/posting.php?mode=post&f=39/]fulrp.5nx.ru/posting.php?mode=post&f=39[/url]
Где приобрести диплом по актуальной специальности?
Мы изготавливаем дипломы психологов, юристов, экономистов и других профессий по доступным ценам. Мы готовы предложить документы ВУЗов, которые находятся в любом регионе РФ. Вы можете купить диплом от любого заведения, за любой год, указав подходящую специальность и хорошие оценки за все дисциплины. Дипломы и аттестаты делаются на “правильной” бумаге высшего качества. Это позволяет делать настоящие дипломы, которые невозможно отличить от оригинала. Документы заверяются всеми требуемыми печатями и подписями. Стараемся поддерживать для покупателей адекватную политику цен. Важно, чтобы документы были доступными для большого количества наших граждан. [url=http://magazin-diplomov.ru/kupit-diplom-v-belgorode-7/]magazin-diplomov.ru/kupit-diplom-v-belgorode-7[/url]
Заказать диплом университета!
Мы можем предложить дипломы любых профессий по разумным тарифам. Вы покупаете диплом через надежную и проверенную временем фирму. : [url=http://deanonnic.listbb.ru/viewtopic.php?f=5&t=1492/]deanonnic.listbb.ru/viewtopic.php?f=5&t=1492[/url]
Ищете оборудование из Китая по ценам производителя? Посетите сайт https://totem28.ru/ где вы найдете широкий ассортимент не только оборудования, но и спец техники. Наш каталог постоянно пополняется, мы тщательно отбираем новинки китайского машиностроения. Посмотрите наш каталог и узнайте подробнее на сайте.
Посетите сайт https://4px.ru/ – 4 Пикселя – это Digital агентство интернет-маркетинга полного цикла в Москве, с комплексным подходом к созданию рекламы в интернете. Ознакомьтесь с нашими выгодными услугами в SEO, Яндекс.Директ, SMM, Serm, разработкой и созданием сайтов и многими другими профессиональными услугами. Ознакомьтесь с нашими кейсами – мы будем рады видеть вас в числе наших клиентов!
подключить интернет в казани в квартире
domashij-internet-kazan001.ru
подключение интернета казань
Online gambling can rise—hold it! plinko
The 1xBet platform remains one of the most generous in the online betting market, offering a variety of attractive bonuses for new users https://www.tumblr.com/codede1xbet/779792404599488512/code-1xbet-valide-bonus-100-jusqu%C3%A0-130?source=share
French sports betting platforms mainly offer three categories of welcome bonuses, each with specific benefits depending on your betting profile https://pasadenakittenfoster.site/2025/04/04/%d0%bf%d0%be%d0%ba%d0%b5%d1%80%d0%b4%d0%be%d0%bc-%d0%bf%d1%80%d0%be%d0%bc%d0%be%d0%ba%d0%be%d0%b4-%d0%bd%d0%b0-%d0%b4%d0%b5%d0%bf%d0%be%d0%b7%d0%b8%d1%82-%d0%ba%d0%b0%d0%ba-%d0%bf%d0%be%d0%bb%d1%83/
На сайте https://rodinasportsclub.com/ вы найдете информацию, которая касается спортивно-развлекательного клуба «Родина». Каждый желающий получает возможность записаться на тренировку по боксу, которая подходит как новичкам, так и более опытным спортсменам. Вас заинтересуют тренировки по рукопашному бою. Перед вами расписание предстоящих матчей на апрель. Ознакомьтесь с ними сейчас, чтобы построить планы. Также имеются и отчеты по прошедшим соревнованиям. Опубликованы разные новости из жизни клуба.
безопасная сделка аккаунтов купить аккаунт
For weight loss
Thank you for your information!
На сайте https://face-me.ru/ вы сможете попробовать все возможности нейросетей и всего за несколько секунд превратить любое фото в видео. Инновационные и уникальные технологии дают возможность получить роскошную, яркую и любопытную фотосессию без профессионального оборудования, студии. Вы сможете создать фантастические образы и удивительные портреты в соответствии с предпочтениями. Эта программа дарит вам огромное количество возможностей и вдохновение. Вы каждый раз будете получать удивительные, красивые образы, которые всегда будут с вами.
cleocin avoid alcohol cleocin phosphate injection for meat generic cleocin lotion side
can cleocin make you aroused how is cleocin supplied can i buy generic cleocin
cleocin usage
cleocin side effects heartburn Cleocin 2% vaginal cream cleocin clindamycin phosphate cream
where to buy cleocin online cleocin adult dosage cleocin ovules during period
The sounds online hook—stay in! plinko
how to buy generic epitol without prescription
Online casinos are perfect for shy people—no crowds! plinko
I got scammed by a shady online casino once—always check reviews first! betmomo
На сайте https://vezuviy.shop/ воспользуйтесь возможностью приобрести качественное печное оборудование, а также дымоходы. На все распространяются гарантии. Здесь вы сможете приобрести и аксессуары, которые гарантированно совместимы со всем оборудованием. Доставка осуществляется абсолютно бесплатно. Клиент сможет получить в качестве презента банный камень. На все аксессуары действует скидка 10%. Все печи выполнены из качественных, высокотехнологичных материалов, потому прослужат долгое время.
How to exchange BITCOIN in the cryptocurrency exchanger quickex are Buy or sell BTC? In this https://www.youtube.com/watch?v=0MupM-q6I3A detailed guide, we will show you how to buy BTC on the quickex platform in 5 easy steps. You will learn how to use a reliable service without registration and verification, getting access to the best rates from leading liquidity providers.
Профессиональная помощь в сфере магических услуг. Искусство высшей магии.
Ясновидение/Руны/Ритуалы
Диагностика / Консультация
* Диагностика на наличие магического негатива, порчи, приворота, воздействия
* Диагностика энергетики
* Выявление проблем и препятствий
* Ответы на вопросы
Чистка магического и энергетического негатива.
Защита на всех уровнях.
Открытие дорог.
Открытие финансового канала.
(на основании диагностики производим чистку негатива, убираем любые деструктивные программы включая порчу на смерть, кладбищенскую порчу, порчу через подклад, подселение сущности, приворот и другие деструктивные программы разрушающие вашу жизнь)
восстанавливаем вашу жизнь и настраиваем по всем сферам жизни
Магия для решения Бизнес задач и Финансовых вопросов
(моя самая любимая сфера деятельности)
* Убрать препятствия
* Защита на вас и ваш бизнес
* Помощь в судах
* Выявление и решение проблем вашего бизнеса через магию
Любовная магия
* Определение совместимости партнеров в браке и при вступлении в брак
* Защита семьи и отношений
* Розжиг чувств в паре (это новый виток отношений. реанимация любви)
* Создание условий, которые приведут к романтическим отношениям и к созданию семьи
Решение любых актуальных для вас проблем
* Выявить и убрать блоки мешающие достичь цели
– магия рун
– магия древних ритуалов
– магия символов, углов, пространства
– планетарная магия
– пространственная алхимия
– работа через сознание и подсознание
Все работы проводятся очень экологично и без какого-либо вреда
Также проводятся специальные ритуалы позволяющие развернуть вашу жизнь на 360 градусов, получить желаемое, открыть дороги, притянуть любовь и создать жизнь мечты
Телеграмм канал Искусство Высшей Магии
Телеграмм для связи: https://t.me/magicfxs
Для связи: WHATSAPP +44 7435 250025
*** для заказа присылайте фото для диагностики, имя и ваш вопрос.
Услуги осуществляются на условиях 100% предоплаты.
P.S. Если человек знает для чего ему нужно желаемое – значит он может это достичь
Has anyone tried that new online casino with the live dealers? It’s pretty cool! aviator
https://telegra.ph/Casinos-Not-on-GamStop-with-No-Deposit-Bonus-Offers-03-27
На сайте https://vezuviy.shop/ вы сможете приобрести чугунные, стальные банные печи, нержавеющие, а также в облицовке, печи-камины, отопительные, каминные топки, дымоходы, порталы и многое другое. Вся продукция от производителя, а потому наценки очень маленькие. Такая техника считается идеальной не только с целью обогрева помещения, но и оформления пространства. А самое главное, что она отличается долгим сроком службы, надежностью из-за того, что в работе использовались высокотехнологичные, проверенные материалы.
Где купить [b]диплом[/b] по актуальной специальности?
Получаемый диплом со всеми печатями и подписями 100% отвечает условиям и стандартам, неотличим от оригинала – даже со специальным оборудованием. Не откладывайте личные цели на несколько лет, реализуйте их с нами – отправьте заявку на изготовление диплома прямо сейчас! Получить диплом о высшем образовании – легко! [url=http://igrosoft.getbb.ru/viewtopic.phpf=11&t=3747/]igrosoft.getbb.ru/viewtopic.phpf=11&t=3747[/url]
https://telegra.ph/Honest-Reviews-of-Non-Gamstop-Casinos-03-27-2
страница [url=https://lk-mango-office.com/]Mango-Office виртуальный номер[/url]
buying cheap co-amoxiclav price where can i get co-amoxiclav pills where can i buy co-amoxiclav without insurance
how to get co-amoxiclav pill where to buy cheap co-amoxiclav online can you get generic co-amoxiclav price
cost co-amoxiclav online
can you get co-amoxiclav no prescription buying cheap co-amoxiclav tablets can you get generic co-amoxiclav prices
where buy co-amoxiclav pill how can i get generic co-amoxiclav without insurance where buy cheap co-amoxiclav without a prescription
I hit a rough online patch—rethink it! plinko
провайдеры интернета в казани по адресу
domashij-internet-kazan002.ru
интернет провайдеры в казани по адресу дома
https://telegra.ph/Non-Gamstop-Casinos-No-Deposit-Offers-and-Benefits-03-27
I hit a bonus online and won $700—big yes! plinko
I don’t trust online odds—odd feel! plinko
На сайте https://xn—-8sbaaajsa0adcpbfha1bdjf8bh5bh7f.xn--p1ai/ ознакомьтесь со всеми вариантами антиквариата, который вы сможете продать по честной стоимости. Станислав Бабкин, являясь частным коллекционером, скупает предметы старины дорого. Оценка происходит в режиме реального времени, а средства выплачиваются на карту либо выдаются наличными. Вы сможете продать следующие вещи: серебро, картины, иконы, золотые, серебряные монеты, ордена, часы, самовары и многое другое. Вся сумма выплачивается полностью во время оформления сделки.
Мы изготавливаем дипломы любых профессий по доступным тарифам.– [url=http://diplomg-cheboksary.ru/mozhno-li-kupit-diplom-zanesennij-v-reestr/]diplomg-cheboksary.ru/mozhno-li-kupit-diplom-zanesennij-v-reestr/[/url]
can i order generic flagyl without a prescription
I wish online had low stakes—more fun! ganesha gold
https://telegra.ph/Best-Non-Gamstop-Casinos-Accepting-PayPal-Payments3-03-27
Meds information. Brand names.
how can i get ampicillin without insurance
Actual what you want to know about meds. Get now.
Судоходная компания Навигатор https://teplohod-restoran.ru/ это возможность организовать праздник на воде в Санкт-Петербурге. Свадьбы, дни рождения, корпоративы, выпускные, а также банкеты и фуршеты. Мы комплексно подходим к организации любого события. Посетите сайт, посмотрите наш флот и стоимость аренды судов, яхт, катеров, теплоходов.
https://telegra.ph/Explore-Online-Casinos-Not-on-Gamstop-in-2023-03-27
Online gambling feels less judged than in-person play. marvel casino
Купить документ о получении высшего образования вы имеете возможность у нас. Мы оказываем услуги по продаже документов об окончании любых университетов Российской Федерации. Вы получите диплом по любой специальности, включая документы СССР. Даем 100% гарантию, что при проверке документов работодателем, каких-либо подозрений не возникнет. [url=http://asxdiplommy.com/kupit-diplom-s-reestrom-v-moskve-3/]asxdiplommy.com/kupit-diplom-s-reestrom-v-moskve-3/[/url]
I got scammed by a shady online casino once—always check reviews first! jackpotraider
I won big online—pure thrill! plinko
Спасибо за “зимний” букет – пахло хвоей и радостью!
доставка цветов в томске
https://telegra.ph/No-Deposit-Codes-for-Non-Gamstop-Casinos-Explained2-03-27
https://telegra.ph/Reliable-Non-Gamstop-Casinos-for-Safe-Online-Gambling-03-27
buy cheap allegra without rx buy cheap allegra price can you get generic allegra price
how to get generic allegra price order cheap allegra online cost of generic allegra price
can i buy generic allegra prices
can i buy cheap allegra without prescription can i purchase generic allegra can i get allegra without dr prescription
how can i get allegra online cost of allegra online can you get generic allegra without dr prescription
Drug information leaflet. Long-Term Effects.
can i order generic synthroid without prescription
All information about medicine. Get information here.
диплом об окончании пту купить
https://telegra.ph/Best-UK-Non-Gamstop-Casinos-for-Online-Gambling-03-27
нейросеть создать курсовую [url=https://studgen.ru/]studgen.ru[/url] .
how to get zenegra online
Мы можем предложить дипломы любой профессии по приятным ценам. Цена будет зависеть от той или иной специальности, года выпуска и образовательного учреждения. Всегда стараемся поддерживать для клиентов адекватную политику тарифов. Важно, чтобы документы были доступны для большого количества граждан. [url=http://diplomc-v-ufe.ru/kupit-diplom-natsionalnogo-issledovatelskogo-universiteta/]купить аттестат 11 класса[/url]
The music online lifts—keep on! tom of madness
интернет домашний казань
domashij-internet-kazan003.ru
какие провайдеры интернета есть по адресу казань
https://telegra.ph/Guide-to-Non-Gamstop-Casinos-for-UK-Players-03-27
безопасная сделка аккаунтов ploshadka-dlya-prodazhi-akkauntov.ru
The online ease rolls—stay wise! plinko
https://telegra.ph/Non-Gamstop-Casinos-Free-Spins-No-Deposit-Offers2-03-27
Для быстрого продвижения вверх по карьерной лестнице требуется наличие официального диплома института. Купить диплом любого университета у надежной фирмы: [url=http://diplom-top.ru/diplom-kupit-v-spb-4/]diplom-top.ru/diplom-kupit-v-spb-4/[/url]
https://telegra.ph/Best-Casino-No-Deposit-Bonus-Not-on-GamStop-03-27
такой [url=https://lk.mango-offlce.net/]Манго Офис услуги для колл-центра[/url]
Drugs information leaflet. Long-Term Effects.
get cheap zyban without dr prescription
All about medicament. Get now.
The live dealers online rule—true vibe! plinko
https://telegra.ph/Explore-New-Non-Gamstop-Casino-Sites-for-2023-03-27
На сайте http://www.raomed.ru почитайте всю актуальную и важную информацию, которая касается объединения «РАОМед». Его основали в 2001 году, и за все время работы заполучило огромное количество положительных отзывов. Работа нацелена на то, чтобы пациент быстрее выздоровел, заполучил крепкое здоровье, его ничего не беспокоило. Кроме того, его качество жизни значительно улучшается. Осуществить переподготовку по программе остеопатии, организовать семинары и вебинары вы сможете, если запишитесь в академию остеопатической медицины.
посетить сайт [url=https://mango-offic.info/]Mango-Office SIP-телефония[/url]
содержание [url=https://lk.mango-office.cc]Манго Офис поддержка[/url]
I hit a dry spell online—maybe it’s not my week! minas
how to buy duricef without prescription
https://telegra.ph/No-Deposit-Bonus-Casinos-Outside-GamStop-Regulations-03-27
Visit https://reviewspoty.com/ and you can easily collect reviews by integrating with your existing technology and e-commerce platforms. Increase sales and reduce marketing costs with reviews. Learn more about us and what we offer, as well as pricing and a free trial.
реферат с помощью нейросети [url=https://www.studgen.ru]https://www.studgen.ru[/url] .
The 1xBet welcome bonus is one of the most generous offers in the online betting market http://leydis16.phorum.pl/viewtopic.php?p=687788#687788
Мы изготавливаем дипломы любых профессий по выгодным тарифам. Дипломы производятся на фирменных бланках государственного образца Заказать диплом ВУЗа [url=http://diplom-top.ru/]diplom-top.ru[/url]
Где приобрести диплом специалиста?
Приобрести диплом университета по выгодной цене возможно, обращаясь к надежной специализированной компании.: [url=http://kupit-diplomyz24.com/]kupit-diplomyz24.com[/url]
https://telegra.ph/Paying-with-Phone-Bill-at-Non-Gamstop-Mobile-Casinos1-03-27
На сайте https://ooomila.com изучите каталог такого оборудования, которое предназначено для организации животноводческих комплексов. Также вы сможете почитать и содержательную информацию о компании и ее достижениях. В каталоге вы найдете следующее оборудование: технологические линии, весовое оборудование, резиновые маты, крематоры и многое другое. На технику, которая находится в разделе, действуют гарантии. Несколько сотен единиц оборудования всегда находятся на складе, можно приобрести под заказ.
На сайте https://ooo-rodnik.ru/ уточните телефон популярной компании «Родник», основной сферой деятельности которой является чистка, ремонт скважин по всей Московский области. Предприятие оказывает услуги в своей сфере уже более 5 лет. Работа происходит без выходных, пенсионеры смогут рассчитывать на приятные скидки, бонусы. На все работы дается гарантия в один год. Компания занимается обслуживанием дач, коттеджей, домов, производств, поселков городского типа. Со всеми направлениями деятельности ознакомьтесь на сайте.
Купить диплом ВУЗа по невысокой цене можно, обращаясь к надежной специализированной фирме. Заказать документ о получении высшего образования вы сможете у нас. [url=http://diplomg-kurerom.ru/diplom-vuza-s-zaneseniem-v-reestr-8/]diplomg-kurerom.ru/diplom-vuza-s-zaneseniem-v-reestr-8[/url]
Где приобрести диплом специалиста?
Мы можем предложить дипломы психологов, юристов, экономистов и любых других профессий по выгодным ценам. Мы предлагаем документы ВУЗов, которые расположены на территории всей РФ. Вы можете купить качественный диплом от любого заведения, за любой год, включая документы СССР. Дипломы и аттестаты выпускаются на “правильной” бумаге высшего качества. Это позволяет делать настоящие дипломы, которые не отличить от оригинала. Они заверяются всеми необходимыми печатями и штампами. Всегда стараемся поддерживать для заказчиков адекватную ценовую политику. Важно, чтобы дипломы были доступными для большого количества граждан. [url=http://diplom-kuplu.ru/kupit-diplom-matematika/]diplom-kuplu.ru/kupit-diplom-matematika[/url]
I love the online chats—great crew! fishing frenzy
Приобрести диплом ВУЗа!
Мы можем предложить документы университетов, расположенных на территории всей Российской Федерации.
[url=http://diplom-top.ru/kupit-diplom-cherez-reestr-2/]diplom-top.ru/kupit-diplom-cherez-reestr-2/[/url]
мостбет скачать андроид [url=mostbet6010.ru]mostbet6010.ru[/url] .
https://telegra.ph/Best-Non-Gamstop-Casinos-of-2019-for-Players-Worldwide-03-27
Drug information. Effects of Drug Abuse.
buy generic dilantin tablets
Best information about drug. Read information here.
интернет провайдеры в москве по адресу дома
domashij-internet-msk001.ru
интернет по адресу дома
Visit the largest and most interesting travel blog https://www.atravel.blog/ where you will find interesting facts about different countries of the world, as well as get acquainted with delicious national dishes based on authentic recipes from around the world. Choose an interesting category for you or read the latest events from the world of travel and food!
Что такое «Порту Пати» в Дубае и почему об этом говорят все
https://x.com/Fariz418740/status/1908767851009163415
https://telegra.ph/Exploring-Welsh-Non-Gamstop-Casinos-Benefits-and-Options-03-27
The online rush kicks—stay cool! vincispin
generic celexa without prescription order cheap celexa how to buy generic celexa without dr prescription
buy celexa pills can you get generic celexa pill can i order celexa without a prescription
where can i buy celexa
can i order celexa tablets get generic celexa without a prescription can i purchase celexa online
where buy cheap celexa for sale how to get celexa without dr prescription generic celexa online
На сайте https://market-delivery-dubai.ru/ вы сможете воспользоваться такой полезной услугой, как доставка алкоголя в Дубае. В разделе вы найдете такие напитки, как: бризер, абсент, водка, виски, ликер, пиво, коньяк и многое другое. Вся продукция является сертифицированной, качественной, а потому идеально подойдет для долгожданного события, праздника. Есть последние поступления вкусного и ароматного вина, которое вы сможете приобрести на торжество или для того, чтобы скрасить вечер. Доставка происходит в минимальные сроки.
https://telegra.ph/Non-Gamstop-Casino-Free-Spins-No-Deposit-Offers-2023-03-27
Смартфоны с доставкой по Москве [url=https://www.techno-line.store]https://www.techno-line.store[/url] .
how to buy cheap zanaflex no prescription
Доставка комплексных обедов в Алматы для ваших сотрудников или на дом на сайте https://bvd.kz/ – у нас широкий ассортимент и выбор блюд по отличным ценам! Ознакомьтесь на сайте с составами и ценами на комплексные обеды. Заключаем договоры на доставку обедов в офисы и административные учреждения, доставляем обеды в школы и детские сады, где нет собственной кухни, в магазины и прочие подобные учреждения, у сотрудников которых нет возможности пообедать в столовой. Подробнее на сайте.
Как быстро получить фрибет за депозит в букмекерской конторе
Фрибет за депозит в БК – как получить бонус быстро
В условиях высокой конкуренции среди игровых платформ, многие организации предлагают различные преференции новым клиентам. Нередко одним из самых привлекательных предложений становятся дополнительные средства для ставок, которые начисляют в качестве бонусов при внесении денег на аккаунт. Этот шаг способен значительно увеличить шансы на успех, если его использовать с умом.
Для начала, внимательно ознакомьтесь с правилами и условиями, связанными с акциями. Каждое заведение имеет свои специфические требования к накапливанию бонусов. Важным аспектом является минимальная сумма, которую необходимо внести для активации привилегии. Учтите, что разные сайты могут применять разные методы для рассчета, что существенно влияет на итоговую сумму дополнительных средств.
Не забывайте о сроках активации предложений. Многие предлагают ограниченные временные рамки для получения бонусов, поэтому обязательно учитывайте возможность быстрого реагирования. Также полезно изучить доступные векселя и коэффициенты, которые могут варьироваться в данной ситуации, так как часто они могут не совпадать с привычными условиями ставок.
Наконец, рассмотрите возможность подписки на рассылку или участие в акциях, проводимых выбранным оператором. Это поможет не только оставаться в курсе текущих мероприятий, но и может дать доступ к эксклюзивным предложениям, которые редко публикуют на сайте.
Первый депозит и фрибет: инструкция за 5 минут
Для успешного начала взаимодействия с игровым сервисом необходимо ознакомиться с условиями акции на первый взнос. Обычно приветственный бонус требует минимального или, наоборот, максимального размера вложения. Изучите правила акции, чтобы понять, сколько денег нужно внести.
Регистрация – первый шаг. Заполните все необходимые поля, указывая актуальную информацию. Некоторые платформы могут запрашивать верификацию. Это может занять несколько минут, но ускоряет процесс получения бонуса.
Принимая решение о вложении средств, обратите внимание на методы оплаты. Выберите тот, который поддерживается оператором. Часто доступны банковские карты, электронные кошельки и мобильные платежи. Убедитесь, что выбранный способ позволяет моментальные переводы, чтобы избежать задержек.
После внесения средств проверьте, добавлен ли приветственный бонус. Обычно он активируется автоматически. Если этого не произошло, обратитесь в службу поддержки. Служба поможет решить вопросы в кратчайшие сроки.
Условия отыгрыша также имеют значение. Изучите их, чтобы понимать, при каких обстоятельствах можно вывести выигрыш. Важно учитывать коэффициенты ставок и сроки, в течение которых необходимо выполнить условия.
Как правило, акционные предложения имеют срок действия, поэтому не затягивайте с использованием бонуса. Заранее планируйте свои ставки, чтобы максимально эффективно использовать предоставленные средства.
Как быстро вывести выигрыш с фрибета: ключевые моменты
Верификация аккаунта – важный этап. Проверка личности занимает время, поэтому лучше пройти ее заранее. Обычно букмекеры требуют загрузку документов, подтверждающих личность и адрес проживания. Следует заранее подготовить сканы или фотографии соответствующих документов.
Наличие актуальной информации на вашем аккаунте также играет большую роль. Проверьте, чтобы все данные были актуальны, это поможет избежать задержек во время обработки запросов. Если возникнут вопросы, поддержка клиентов всегда готова помочь, но лучше заранее всё сделать правильно.
Feel free to surf to my homepage https://t.me/s/fribety_za_depozit
Лучшая подборка площадок https://dzen.ru/a/Zymut7KvZDxsVQ3_. Процесс продажи вещей занимает не более 10 минут. Цены выгоднее чем в стиме с выводом на карту или криптовалюту. Большое количество отзывов от реальных пользователей проверенных временем.
https://winsystem.xyz/2025/01/31/top-10-luchshih-gadzhetov-2025-goda/
перепродажа аккаунтов перепродажа аккаунтов
Meds prescribing information. Short-Term Effects.
get generic effexor without a prescription
Some information about drug. Get now.
Online casinos should cut—fair play! book of dead
https://eskort-moskwa.com/eskort-v-podmoskove/
The slot rush online grips—hold on! marvel casino
1xBet offers a weekly reload bonus system that is particularly advantageous for regular players http://gzew.phorum.pl/posting.php
mostber [url=https://mostbet6010.ru/]https://mostbet6010.ru/[/url] .
cheap cipro for sale can you buy generic cipro for sale can you get cipro without rx
get cheap cipro without prescription generic cipro price how can i get cheap cipro pills
where to buy cheap cipro without dr prescription
generic cipro without insurance can i buy cheap cipro pill cost cheap cipro tablets
generic cipro online where can i buy generic cipro online can i get cheap cipro online
Online casinos need signs—flag us! plinko
проверить провайдеров по адресу москва
domashij-internet-msk002.ru
провайдер по адресу
На сайте https://t.me/rahvalskiy вы сможете ознакомиться с информацией о компании, которая занимается продвижением сайтов «под ключ». Вы сможете воспользоваться всеми комплексными рекламными услугами. В компании работают лучшие, компетентные сотрудники с огромным стажем, а потому они реализуют проект любой сложности и по лучшей стоимости. Для сотрудников нет неисполнимых задач. Они работают независимо от темы проекта и в строго указанные сроки. Можете не сомневаться в том, что получите результат, на который рассчитывали.
how to buy cheap proscar pill
На сайте https://pohudetlegko.ru/ представлена исчерпывающая, актуальная и полезная информация о том, как похудеть правильно, без вреда для организма и максимально эффективно. На сайте вы найдете вдохновляющие истории, советы звезд, которые выглядят младше своего возраста. Есть рецепты блюд, которые созданы из полезных, вкусных ингредиентов. Но при этом они помогут скинуть вес и привести себя в форму к летнему сезону. Рецепты простые и быстрые, а потому справиться с приготовлением пищи сможет каждый. Описаны и упражнения, обертывания для похудения.
Online casinos should list odds—open up! ruleta americana
Meds information leaflet. What side effects can this medication cause?
how to get atarax without insurance
Everything what you want to know about drugs. Get information now.
The online thrill strikes—stay steady! ruleta americana
покупка аккаунтов https://prodat-akkaunt.ru
https://telegra.ph/Top-Non-GamStop-Casinos-in-the-UK-for-202312-03-27
страница [url=https://lk.mango-offic.info/]Манго Офис настройка АТС[/url]
Приобрести диплом университета по доступной цене возможно, обратившись к проверенной специализированной фирме. Заказать документ университета можно в нашей компании в столице. [url=http://diplomaj-v-tule.ru/kupit-diplom-v-moskve-s-zaneseniem-v-reestr-14/]diplomaj-v-tule.ru/kupit-diplom-v-moskve-s-zaneseniem-v-reestr-14[/url]
На сайте https://kabel-silovoj.ru/ воспользуйтесь возможностью приобрести качественный, надежный силовой кабель, который выполнен по ГОСТу и в соответствии с нормативами. Вы совершаете покупку у производителя, а потому установлены привлекательные цены, чтобы сделать это смог каждый. Основными покупателями компании являются профессионалы: электрики, монтажники, прорабы, а также бригадиры. Каждый клиент получает возможность воспользоваться оперативной доставкой, а оплатить так, как удобно.
подробнее здесь [url=https://lk-mango-office.com]Манго Офис API интеграции[/url]
Купить документ о получении высшего образования вы имеете возможность в нашем сервисе. [url=http://dip-lom-rus.ru/kupit-diplom-naberezhnie-chelni/]dip-lom-rus.ru/kupit-diplom-naberezhnie-chelni[/url]
Mirtash предлагает приобрести природный камень. Вы можете по телефону оформить заявку. Мы много работаем и строим долгосрочные отношения с клиентами, основанные на доверии. У вас есть возможность на удобную систему расчетов и лояльные условия сотрудничества рассчитывать. Ищете камень для дорожек? Mirtash.ru – тут можно подробнее с информацией о доставке природного камня ознакомиться. Высокий уровень качества услуг мы гарантируем. Свяжитесь с нами, чтобы получить консультацию. С «Mirtash» вы получаете надежного партнера, готового помочь в реализации любых проектов.
https://telegra.ph/Casinos-Without-Gamstop-Featuring-No-Deposit-Bonuses-03-27
Купить смартфон в Москве [url=http://techno-line.store]http://techno-line.store[/url] .
На сайте https://apostolidi.ru/ вы найдете всю полезную информацию, касающуюся торгов на бирже, а также финансов. Здесь также вы найдете и прогнозы по валютным парам от экспертов, которые работают на совесть. Обязательно публикуются свежие, интересные и информативные записи, которые будут полезны всем без исключения. Также представлен и курс доллара, о котором нужно знать инвесторам. На этом портале вы получаете возможность пообщаться с единомышленниками, задать им вопрос, узнать много нового, интересного.
can i order generic seroflo for sale cost of generic seroflo without a prescription can you buy cheap seroflo pills
can i buy seroflo no prescription where can i buy seroflo without insurance where buy cheap seroflo tablets
cheap seroflo for sale
can you get seroflo without rx where to buy generic seroflo without a prescription where buy cheap seroflo pills
can i purchase seroflo price where to buy cheap seroflo pill where to buy cheap seroflo pills
На сайте https://dragon1gaming.site/ вы найдете ТОП букмекерских контор для ставок на спорт и другие события. Вы узнаете, как выбрать официальную, надежную букмекерскую контору, которая, в том числе дает бонусы, отличные коэффициенты и честные выплаты. Вы узнаете, как зарегистрироваться, пополнить счет, какие виды ставок и линий бывают, а также как вывести деньги. Подробнее на сайте.
На сайте https://ptne.ru/ получите бесплатную консультацию по вопросам продажи недвижимости. Вы сможете воспользоваться такой услугой, как оперативный выкуп различной недвижимости в Санкт-Петербурге. При этом получить аванс вы сможете в день обращения. Агентство оказывает такие услуги, как: выкуп квартир, долей, займ до продажи. Выкуп происходит за свои средства, сделка является прозрачной. Адвокаты оказывают полное юридическое сопровождение, консультационную помощь. Если документов не хватает, то их соберут максимально быстро.
Medicine prescribing information. Generic Name.
can i take propranolol and ibuprofen
Best about pills. Get here.
https://telegra.ph/Mobile-Payment-Options-in-Non-GamStop-Casinos-03-27
Купить диплом о высшем образовании!
Мы готовы предложить документы учебных заведений, которые расположены на территории всей РФ.
[url=http://diplom-onlinex.com/legalnoe-zanesenie-diploma-v-reestr-bez-sporov-i-nervov/]diplom-onlinex.com/legalnoe-zanesenie-diploma-v-reestr-bez-sporov-i-nervov/[/url]
cost generic mentat tablets
https://telegra.ph/Non-GamStop-Casinos-with-Free-Spins-Offers-Available-Now-03-27
I love the online chats—great crew! plinko
интернет по адресу москва
domashij-internet-msk003.ru
провайдеры интернета по адресу
https://telegra.ph/Best-Non-Gamstop-Casinos-for-Players-in-20231-03-27
купить диплом в туле [url=https://diplomys-vsem.ru/]купить диплом в туле[/url] .
Будучи студентом, я наслаждался учебой до тех пор, пока не пришло время писать диплом. Но паниковать не стоило, ведь существуют компании, которые помогают с написанием и защитой диплома на отличные оценки!
Изначально я искал информацию по теме: купить диплом в пятигорске, купить диплом института в нижнем тагиле, купить диплом кандидата наук в москве, купить диплом косметолога эстетиста, купить диплом о высшем образовании в перми, затем наткнулся на [url=http://diplomybox.com/kupit-attestat-v-surgute/]diplomybox.com/kupit-attestat-v-surgute[/url]
безопасная сделка аккаунтов продажа аккаунтов
https://telegra.ph/Exploring-Casino-Options-Beyond-Gamstop-Restrictions-03-27
Meds information for patients. Cautions.
cost cheap irbesartan price
All about medicines. Get information here.
https://telegra.ph/No-Gamstop-Casino-Options-Available-in-the-UK-03-27
Где купить диплом специалиста?
Наша компания предлагаетвыгодно и быстро приобрести диплом, который выполняется на бланке ГОЗНАКа и заверен мокрыми печатями, водяными знаками, подписями официальных лиц. Наш документ пройдет любые проверки, даже при использовании специфических приборов. Решайте свои задачи быстро с нашим сервисом. Купить диплом любого ВУЗа! [url=http://freebeg.com/forum/member.php/]freebeg.com/forum/member.php[/url]
На сайте https://grillhouse-spb.ru/ закажите звонок для того, чтобы проконсультироваться по поводу покупки беседок-гриль, гриль-домиков. Популярная компания «Гриль Хаус» в течение долгого времени производит и собирает беседки, в том числе, для барбекю, а также саун. Все это потребуется для того, чтобы незабываемо провести время на природе и в кругу друзей, близких людей. Все конструкции выполнены из качественных, надежных материалов, которые являются износостойкими. Прямо сейчас изучите каталог доступных для заказа вариантов.
https://telegra.ph/Top-Casinos-Not-on-Gamstop-with-No-Deposit-Bonuses2-03-27
where can i buy generic claritin for sale buying claritin pills buying claritin without a prescription
where to buy generic claritin without a prescription get cheap claritin price can i purchase claritin without rx
where can i buy cheap claritin tablets
order claritin no prescription how can i get cheap claritin without dr prescription buying claritin without dr prescription
can i buy generic claritin without insurance order claritin no prescription buying claritin tablets
Regardless of whether you are located in any other country, you will need to register to place bets at this establishment https://fastdeal.co.ke/pages/1xbet_promo_code_for_freebet.html
На сайте https://bitovayatechnika.blogspot.com/2025/03/blog-post.html изучите полезную, актуальную информацию, которая касается сплит-систем и того, как правильно их выбрать домой либо в офис. Эти функциональные и умные устройства нацелены на то, чтобы поддержать приятную температуру в помещении. Но для того, чтобы воспользоваться всеми функциями, необходимо правильно выбрать устройство. К важным преимуществам такой техники относят то, что она является энергоэффективной, работает практически бесшумно.
Astrologers named 6 signs of the eastern horoscope that will be lucky in April
https://x.com/Fariz418740/status/1908917832265392277
https://telegra.ph/Boku-Casinos-Without-GamStop-Options-for-Players-03-27
Приобрести диплом ВУЗа!
Мы можем предложить дипломы любой профессии по приятным ценам— [url=http://diplomus-spb.ru/kupit-diplom-v-krasnoyarske-bistro-i-nadezhno/]diplomus-spb.ru/kupit-diplom-v-krasnoyarske-bistro-i-nadezhno/[/url]
MetaMask Chrome is excellent for crypto traders. The convenience of accessing dApps and swapping tokens directly is unmatched.
https://telegra.ph/Guide-to-Non-Gamstop-Online-Casinos-and-Their-Benefits-03-27
нажмите здесь [url=https://mango-offlce.net/]Mango-Office вход[/url]
how can i get albenza pill
Medicament information for patients. Effects of Drug Abuse.
can you buy cheap synthroid for sale
Best about medicament. Get here.
Мы изготавливаем дипломы любой профессии по приятным тарифам. О преимуществах приобретения документов в нашем сервисе
Вы покупаете документ через надежную и проверенную компанию. Это решение сэкономит не только много денег, но и ваше драгоценное время.
На этом преимущества не заканчиваются, их куда больше:
• Дипломы печатаются на подлинных бланках с мокрыми печатями и подписями;
• Можно приобрести дипломы любого ВУЗа РФ;
• Цена значительно ниже той, которую понадобилось бы заплатить на очном и заочном обучении в университете;
• Удобная доставка как по Москве, так и в любые другие регионы России.
Приобрести диплом о высшем образовании– [url=http://aryamariasinta.copiny.com/question/details/id/1061184/]aryamariasinta.copiny.com/question/details/id/1061184[/url]
https://telegra.ph/Non-GamStop-Casinos-No-Deposit-Bonus-Guide1-03-27
Заказ официального диплома через надежную фирму дарит массу преимуществ для покупателя. Данное решение позволяет сберечь время и существенные денежные средства. Тем не менее, плюсов гораздо больше.Мы изготавливаем дипломы любой профессии. Дипломы изготавливаются на настоящих бланках государственного образца. Доступная стоимость сравнительно с серьезными затратами на обучение и проживание. Приобретение диплома ВУЗа станет разумным шагом.
Быстро купить диплом: [url=http://diplomg-cheboksary.ru/bistraya-pokupka-diplomov-s-reestrom-bez-xlopot/]diplomg-cheboksary.ru/bistraya-pokupka-diplomov-s-reestrom-bez-xlopot/[/url]
https://telegra.ph/Best-Casinos-for-Free-Spins-No-Deposit-in-UK-2023-03-27
нажмите [url=https://mango-office.cc]Манго Офис услуги связи[/url]
виртуальный хостинг купить vps хостинг аренда
https://telegra.ph/Explore-New-Casinos-Without-Gamstop-Restrictions-03-27
провайдеры интернета по адресу нижний новгород
domashij-internet-nizhnij-novgorod001.ru
домашний интернет нижний новгород
https://telegra.ph/No-Deposit-Codes-for-Non-GamStop-Casinos-Explained1-03-27
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?
Где заказать диплом по нужной специальности?
Мы предлагаембыстро и выгодно купить диплом, который выполнен на оригинальном бланке и заверен мокрыми печатями, водяными знаками, подписями официальных лиц. Документ пройдет любые проверки, даже с применением специально предназначенного оборудования. Решайте свои задачи максимально быстро с нашими дипломами. Приобрести диплом ВУЗа! [url=http://video.listbb.ru/viewtopic.php?f=3&t=1517/]video.listbb.ru/viewtopic.php?f=3&t=1517[/url]
Нужно срочно [url=https://maktrans.ru/]заказать перевозку груза по россии[/url]? Не откладывайте — на сайте «Мак-Транс» это занимает пару минут. Мы рекомендуем этот путь: оформление понятное, расчёт — моментальный, исполнение — строго по срокам. Убедитесь сами — всё работает чётко.
where buy generic avapro pills
мостбет скачать андроид [url=https://mostbet6029.ru]https://mostbet6029.ru[/url] .
На сайте https://xn—-7sbjhqn0bhjc0lk.xn--p1ai/ получите юридическую консультацию по различным вопросам. Компания работает как с физическими, так и частными лицами. На все услуги установлены привлекательные расценки, чтобы воспользоваться ими смог каждый. Предприятие работает в этой сфере более 11 лет. В команде трудятся 11 специалистов, которые справятся с задачей независимо от сложности. К вашим услугам досудебный юрист, досудебное урегулирование, кредитный, семейный юрист, решение страховых споров.
Была необходимость в аккуратной [url=https://maktrans.ru/]перевозка ценных грузов по россии[/url]. Документы, техника — всё требовало особого подхода. «Мак-Транс» сработал безупречно: упаковка, сопровождение, контроль на каждом этапе. Всё доехало в срок и в полном порядке. Спокойно рекомендую.
Приветствую!
Для некоторых людей, купить [b]диплом[/b] о высшем образовании – это необходимость, возможность получить достойную работу. Но для кого-то – это банальное желание не терять время на учебу в универе. Что бы ни толкнуло вас на такое решение, мы готовы помочь вам. Максимально быстро, качественно и выгодно изготовим диплом любого года выпуска на подлинных бланках со всеми требуемыми печатями.
Главная причина, почему люди прибегают к покупке документа, – желание занять хорошую работу. К примеру, знания позволяют кандидату устроиться на привлекательную работу, однако подтверждения квалификации не имеется. При условии, что работодателю важно наличие “корочек”, риск потерять место работы довольно высокий.
Приобрести документ о получении высшего образования вы сможете у нас в столице. Мы оказываем услуги по продаже документов об окончании любых университетов России. Вы сможете получить диплом по любым специальностям, любого года выпуска, в том числе документы старого образца. Гарантируем, что при проверке документов работодателем, никаких подозрений не появится.
Ситуаций, которые вынуждают заказать диплом ВУЗа немало. Кому-то прямо сейчас потребовалась работа, а значит, нужно произвести хорошее впечатление на руководителя во время собеседования. Другие задумали попасть в престижную компанию, чтобы повысить собственный статус и в дальнейшем начать свой бизнес. Чтобы не терять массу времени, а сразу начинать удачную карьеру, используя имеющиеся знания, можно купить диплом в онлайне. Вы станете полезным для социума, получите финансовую стабильность в максимально короткий срок- [url=http://diplomanrus.com/]диплом о среднем образовании купить[/url]
На сайте https://rodinasportsclub.com/ вы найдете информацию, которая касается спортивно-развлекательного клуба «Родина». Каждый желающий получает возможность записаться на тренировку по боксу, которая подходит как новичкам, так и более опытным спортсменам. Вас заинтересуют тренировки по рукопашному бою. Перед вами расписание предстоящих матчей на апрель. Ознакомьтесь с ними сейчас, чтобы построить планы. Также имеются и отчеты по прошедшим соревнованиям. Опубликованы разные новости из жизни клуба.
can i get cheap clomid without rx how can i get cheap clomid without prescription order cheap clomid without dr prescription
can you get clomid without insurance how can i get generic clomid pill how can i get clomid pill
where to buy clomid without rx
where buy cheap clomid online how to buy clomid pills how to get cheap clomid without rx
can i order clomid without a prescription where buy cheap clomid pill can i order generic clomid tablets
интернет тарифы нижний новгород
domashij-internet-nizhnij-novgorod002.ru
интернет по адресу
роллы недорого суши вок барнаул
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
where can i buy cheap provera for sale
Мы изготавливаем дипломы любой профессии по приятным тарифам. Всегда стараемся поддерживать для заказчиков адекватную политику цен. Для нас очень важно, чтобы документы были доступными для подавляющей массы граждан.
Покупка диплома, который подтверждает обучение в ВУЗе, – это выгодное решение. Купить диплом ВУЗа: [url=http://diplom-kuplu.ru/kupit-diplom-ob-okonchanii-universiteta/]diplom-kuplu.ru/kupit-diplom-ob-okonchanii-universiteta/[/url]
Здравствуйте!
Без наличия диплома трудно было продвигаться вверх по карьерной лестнице. Теперь этот документ не дает гарантий, что получится найти престижную работу. Более важное значение имеют навыки специалиста, а также его опыт. Именно из-за этого решение о заказе диплома следует считать выгодным и рациональным. Приобрести диплом университета [url=http://extra-v.store/forum/?PAGE_NAME=profile_view&UID=3240/]extra-v.store/forum/?PAGE_NAME=profile_view&UID=3240[/url]
Здравствуйте!
Мы можем предложить дипломы любой профессии по приятным ценам. Стоимость будет зависеть от выбранной специальности, года получения и образовательного учреждения: [url=http://rdiploms.com/]rdiploms.com/[/url]
На сайте http://xn--80aaagcrtbcptx7amedd.xn--p1ai/ есть возможность воспользоваться такими важными услугами, как: ремонт, сервис газовой автоматики. Обслуживание считается важной задачей из-за того, что оно обеспечивает безопасность, а также добавляет оборудованию надежности, делает эффективным. В комплекс услуг входит и техническое обслуживание, диагностика, проведение ремонтных работ и многое другое. Во время манипуляций специалисты точно следуют предписаниям, инструкциям, рекомендациям производителей.
На сайте https://kino-wsem.site/publ/doramy/ представлены дорамы в отличном качестве. Все они о любви, дружбе и вечном. Есть произведения за прошлые годы, которые многие пересматривают с особым удовольствием. Навигация поможет вам лучше сориентироваться в выборе и начать просмотр такого фильма, который вызовет у вас приятные, положительные эмоции. Регулярно на портале появляются новые дорамы, которые погрузят вас в удивительный мир. Вы сможете просматривать фильмы в режиме реального времени и на любом устройстве.
cost avodart pill generic avodart price where to get generic avodart no prescription
can i order cheap avodart without rx where buy cheap avodart without dr prescription where buy cheap avodart prices
buy avodart without rx
can i purchase cheap avodart prices where to get avodart online can you buy cheap avodart without dr prescription
can i buy avodart without dr prescription cost avodart online can you get generic avodart without prescription
скупка золота дорога b-gold.ru [url=https://www.of-md.com/jwe-qs//]скупка золота дорога b-gold.ru[/url] .
провайдер по адресу
domashij-internet-nizhnij-novgorod003.ru
домашний интернет нижний новгород
where can i get primaquine pill
Заказала редкие орхидеи – доставили аккуратно, с инструкцией по уходу!
101 роза
На сайте https://mvpol.ru/ воспользуйтесь возможностью заказать промышленные полы напрямую от производителя. Компания «МВПОЛ» оказывает и такую важную услугу, как ремонт промышленных полов, производство полимерных наливных полов, бетонных, у которых упрочненный верх. Также получится узнать расценки на такие услуги, чтобы заранее спланировать бюджет. Для того чтобы иметь представление о том, как выглядят работы, изучите объекты. На все услуги предоставляются гарантии. Вся информация дается максимально быстро.
https://telegra.ph/Paypal-Casinos-Not-on-GamStop-for-Easy-Transactions-03-27
скупка золота цены [url=https://metaphysican.com/vsyo-chto-vam-nuzhno-znat-o-skupke-zolota-sovety-i-rekomendaczii//]https://metaphysican.com/vsyo-chto-vam-nuzhno-znat-o-skupke-zolota-sovety-i-rekomendaczii//[/url] .
На сайте https://synccheat.ru/deadlock изучите огромный каталог читов, которые помогут игроку получить преимущества от игрового процесса. Так вы сможете легко дойти до нужного уровня, чтобы получить приз, перейти на другую стадию. Этот портал является проверенным, надежным, поэтому на нем можно совершать покупку, не опасаясь за свои средства, конфиденциальность. Совершить покупку вы сможете прямо сейчас и по выгодной стоимости, без переплат. Если появились вопросы, то задайте их службе поддержки, которая ответит на них.
Севертур предоставляет лучшие программы. Вы побываете в местах, где бережно многовековая история хранится, благодаря туроператору. Логистика сотрудниками компании выстроена безупречно. Гиды приятные в общении и доброжелательны. Экскурсии информативны и познавательны. Ищете туристические поездки в каргополь? Xn–80ade0ahrahddrm9hxa.xn--p1ai – портал, где у вас есть возможность о наших турах узнать все самое познавательное. Приглашаем вас отправиться в невероятное путешествие. Вы останетесь в восторге от организации поездки. Не упустите возможность сделать памятные фотографии!
На сайте https://t.me/rahvalskiy вы сможете ознакомиться с информацией о компании, которая занимается продвижением сайтов «под ключ». Вы сможете воспользоваться всеми комплексными рекламными услугами. В компании работают лучшие, компетентные сотрудники с огромным стажем, а потому они реализуют проект любой сложности и по лучшей стоимости. Для сотрудников нет неисполнимых задач. Они работают независимо от темы проекта и в строго указанные сроки. Можете не сомневаться в том, что получите результат, на который рассчитывали.
https://www.ramana-maharshi.org/a-thoughtful-guide-to-registering-on-gambling-platforms-in-india/
На сайте https://pohudetlegko.ru/ представлена исчерпывающая, актуальная и полезная информация о том, как похудеть правильно, без вреда для организма и максимально эффективно. На сайте вы найдете вдохновляющие истории, советы звезд, которые выглядят младше своего возраста. Есть рецепты блюд, которые созданы из полезных, вкусных ингредиентов. Но при этом они помогут скинуть вес и привести себя в форму к летнему сезону. Рецепты простые и быстрые, а потому справиться с приготовлением пищи сможет каждый. Описаны и упражнения, обертывания для похудения.
https://telegra.ph/Best-Non-Gamstop-UK-Casino-Sites-for-Players-in-2023-03-27
can you get cipro for sale where to buy generic cipro without dr prescription cost cheap cipro price
order cipro for sale where can i get cheap cipro without a prescription generic cipro without prescription
where to buy generic cipro for sale
can you buy cheap cipro online where can i buy cipro online can i purchase cheap cipro online
can i purchase cheap cipro without insurance can i get cipro without prescription can you get generic cipro without prescription
санлайт скупка золота цена за грамм b-gold.ru [url=www.www.of-md.com/jwe-qs//]санлайт скупка золота цена за грамм b-gold.ru[/url] .
Где заказать [b]диплом[/b] по необходимой специальности?
Полученный диплом с приложением отвечает стандартам, неотличим от оригинала – даже со специальным оборудованием. Не откладывайте личные мечты и задачи на пять лет, реализуйте их с нами – отправляйте простую заявку на диплом прямо сейчас! Диплом о среднем образовании – не проблема! [url=http://vetstate.ru/forum/PAGE_NAME=profile_view&UID=174265/]vetstate.ru/forum/PAGE_NAME=profile_view&UID=174265[/url]
Really enjoyed this! Your perspective is refreshing and thought-provoking. Keep it up!
Купить диплом академии!
Мы можем предложить дипломы любой профессии по приятным ценам. Вы приобретаете диплом в надежной и проверенной компании. : [url=http://remoterecruit.com.au/employer/frees-diplom/]remoterecruit.com.au/employer/frees-diplom[/url]
[b]Диплом университета России![/b]
Без ВУЗа очень трудно было продвигаться вверх по карьере. Поэтому решение о покупке диплома стоит считать целесообразным. Купить диплом любого университета [url=http://pokypke.flybb.ru/viewtopic.php?f=2&t=768&sid=a78c6a2de6151e91fd60e51f39194acf/]pokypke.flybb.ru/viewtopic.php?f=2&t=768&sid=a78c6a2de6151e91fd60e51f39194acf[/url]
На сайте films2025.my вы можете смотреть новинки кино 2025 года абсолютно бесплатно. Удобная навигация по жанрам — от комедий и боевиков до фантастики и семейных фильмов — позволяет быстро найти интересующую вас картину. Все фильмы доступны в высоком качестве HD и без регистрации. Отличный выбор для вечернего просмотра новые комедии 2025 в HD
where buy betapace online
подключение интернета новосибирск
domashij-internet-novosibirsk001.ru
подключить интернет новосибирск
https://telegra.ph/Guide-to-Casinos-Not-on-Gamstop-for-Players-03-27
Ready to transform your vibe with real dreadlocks? Browse a stunning collection of real dreadlock extensions at the site – dreads real hair, offering the highest quality options for achieving a flawless, natural look.
Made with real human hair, these dreadlocks are a great match for your unique personality. Whether you’re into full-head transformations, we have options that suit all hair types.
Find your fit with:
– dread natural
– handmade dreadlocks
Get the look you love with premium-quality extensions that look and feel real. Top-rated customer service available across the USA and beyond!
Start your journey – your dream style awaits.
скупка золота в москве цена [url=metaphysican.com/vsyo-chto-vam-nuzhno-znat-o-skupke-zolota-sovety-i-rekomendaczii/]скупка золота в москве цена [/url] .
Your perspective is refreshing and thought-provoking. Keep it up!
Купить документ университета вы можете у нас в Москве. Мы оказываем услуги по производству и продаже документов об окончании любых ВУЗов Российской Федерации. Вы получите необходимый диплом по любым специальностям, любого года выпуска, в том числе документы образца СССР. Гарантируем, что при проверке документа работодателями, никаких подозрений не появится. [url=http://asxdiploman.com/kupite-diplom-visshego-obrazovaniya-s-zapisyu-v-reestr-2/]asxdiploman.com/kupite-diplom-visshego-obrazovaniya-s-zapisyu-v-reestr-2/[/url]
https://telegra.ph/Non-Gamstop-Casinos-Featuring-NetEnt-Games2-03-27
can you get generic clarinex pills buying clarinex pill cost generic clarinex pill
get clarinex prices buy cheap clarinex without a prescription can i purchase generic clarinex without insurance
where buy clarinex online
cost of clarinex no prescription order generic clarinex without dr prescription can i order cheap clarinex without a prescription
get clarinex without a prescription buying generic clarinex without insurance can i purchase clarinex price
На сайте https://grillhouse-spb.ru/ закажите звонок для того, чтобы проконсультироваться по поводу покупки беседок-гриль, гриль-домиков. Популярная компания «Гриль Хаус» в течение долгого времени производит и собирает беседки, в том числе, для барбекю, а также саун. Все это потребуется для того, чтобы незабываемо провести время на природе и в кругу друзей, близких людей. Все конструкции выполнены из качественных, надежных материалов, которые являются износостойкими. Прямо сейчас изучите каталог доступных для заказа вариантов.
Найден способ избежать преждевременной смерти
https://x.com/___Nikie__/status/1909141697990115541
ломбард скупка золота серебра b-gold.ru [url=http://www.www.of-md.com/jwe-qs/]http://www.www.of-md.com/jwe-qs/[/url] .
where to buy shallaki without prescription
https://telegra.ph/UK-Casinos-Without-Gamstop-Options-and-Opportunities-03-27
Пожарный риск – мероприятие по расчету и сопоставлению нормативно допустимой величины пожарного риска с реальной https://www.bragazeta.ru/news/2022/03/17/v-kakix-sluchayax-mozhet-potrebovatsya-ocenka-pozharnyx-riskov/
Tiger Fortune’s payouts are generous and frequent. tiger fortune
На сайте https://bitovayatechnika.blogspot.com/2025/03/blog-post.html изучите полезную, актуальную информацию, которая касается сплит-систем и того, как правильно их выбрать домой либо в офис. Эти функциональные и умные устройства нацелены на то, чтобы поддержать приятную температуру в помещении. Но для того, чтобы воспользоваться всеми функциями, необходимо правильно выбрать устройство. К важным преимуществам такой техники относят то, что она является энергоэффективной, работает практически бесшумно.
https://telegra.ph/Discover-the-Best-Non-Gamstop-Casinos-Today-03-27-2
how to get cheap nemasole without dr prescription where can i get generic nemasole without prescription buy cheap nemasole price
how to get cheap nemasole online buy generic nemasole without insurance buying generic nemasole without insurance
can i get generic nemasole
where buy cheap nemasole pill where can i get generic nemasole without prescription can i buy cheap nemasole without prescription
get cheap nemasole for sale can you buy generic nemasole without a prescription order generic nemasole no prescription
Мы готовы предложить дипломы любой профессии по приятным тарифам. Стараемся поддерживать для заказчиков адекватную политику тарифов. Важно, чтобы дипломы были доступны для подавляющей массы граждан.
Приобретение диплома, который подтверждает окончание института, – это выгодное решение. Заказать диплом любого ВУЗа: [url=http://kupite-diplom0024.ru/kupit-diplom-vuz-3/]kupite-diplom0024.ru/kupit-diplom-vuz-3/[/url]
Мы можем предложить дипломы любых профессий по невысоким тарифам.– [url=http://diplomg-cheboksary.ru/kupite-originalnij-diplom-s-zaneseniem-v-reestr/]diplomg-cheboksary.ru/kupite-originalnij-diplom-s-zaneseniem-v-reestr/[/url]
скупка золота рядом [url=http://metaphysican.com/vsyo-chto-vam-nuzhno-znat-o-skupke-zolota-sovety-i-rekomendaczii/]http://metaphysican.com/vsyo-chto-vam-nuzhno-znat-o-skupke-zolota-sovety-i-rekomendaczii/[/url] .
WhatsApp вводит новую функцию https://x.com/Ruslansavalv/status/1909195435446595720
Tiger Fortune’s animations are next-level awesome. jogo do tigrinho demo
cost of cheap diamox for sale
https://telegra.ph/No-Deposit-Bonuses-at-Non-Gamstop-Casinos-Explained1-03-27
подключить проводной интернет новосибирск
domashij-internet-novosibirsk002.ru
подключить интернет по адресу
Горкинское кладбище https://gorkinskoe.ru одно из старейших в Видном. Подробная информация: адрес, как доехать, порядок захоронений, наличие участков, памятники, услуги по уходу.
This casino’s Tiger Fortune slot is a winner. tiger fortune
The live support at online casinos saves the day sometimes! fishin frenzy
Hire a hitman
hire a hitman
На сайте https://xn—-7sbjhqn0bhjc0lk.xn--p1ai/ получите юридическую консультацию по различным вопросам. Компания работает как с физическими, так и частными лицами. На все услуги установлены привлекательные расценки, чтобы воспользоваться ими смог каждый. Предприятие работает в этой сфере более 11 лет. В команде трудятся 11 специалистов, которые справятся с задачей независимо от сложности. К вашим услугам досудебный юрист, досудебное урегулирование, кредитный, семейный юрист, решение страховых споров.
Для удачного продвижения вверх по карьере требуется наличие официального диплома о высшем образовании. Быстро и просто купить диплом любого института у надежной компании: [url=http://diplom-top.ru/kupit-diplom-gos/]diplom-top.ru/kupit-diplom-gos/[/url]
Falschgeld kaufen Verkauf Euro wo man gefalschte Euro-Scheine kaufen kann
На сайте https://ptne.ru/ получите бесплатную консультацию по вопросам продажи недвижимости. Вы сможете воспользоваться такой услугой, как оперативный выкуп различной недвижимости в Санкт-Петербурге. При этом получить аванс вы сможете в день обращения. Агентство оказывает такие услуги, как: выкуп квартир, долей, займ до продажи. Выкуп происходит за свои средства, сделка является прозрачной. Адвокаты оказывают полное юридическое сопровождение, консультационную помощь. Если документов не хватает, то их соберут максимально быстро.
Trix — это современная игровая платформа, предлагающая широкий выбор игр, от классических слотов до живых столов с настоящими дилерами. Здесь вы найдёте интерактивный интерфейс, высококачественную графику и щедрые бонусы для новых и постоянных игроков https://t.me/trix_fun
ремонт карданных валов [url=http://passat-club.ru/news.php?id=1593/]ремонт карданных валов[/url] .
how can i get zyrtec no prescription where can i get cheap zyrtec without a prescription where can i get generic zyrtec prices
can i buy generic zyrtec prices can i get zyrtec without prescription can you get generic zyrtec pills
where buy generic zyrtec price
how to get cheap zyrtec pill generic zyrtec without dr prescription where to buy zyrtec without insurance
can you get zyrtec prices can you get generic zyrtec pill where to get cheap zyrtec
https://telegra.ph/Non-GamStop-UK-Casinos-A-Guide-for-Players-03-27
Заказать диплом о высшем образовании!
Мы готовы предложить дипломы любой профессии по выгодным ценам— [url=http://asxdiplommy.com/kupit-attestat-za-11-klassov-v-moskve-vigodno/]asxdiplommy.com/kupit-attestat-za-11-klassov-v-moskve-vigodno/[/url]
Tiger Fortune’s theme is so adventurous. fortune tiger demo
мост бет [url=https://www.mostbet6029.ru]мост бет[/url] .
Tiger Fortune’s spins keep me on my toes. tiger fortune
перенаправляется сюда [url=https://donturbo.ru]купить турбокомпрессор для автомобиля[/url]
скачат мостбет [url=mostbet6030.ru]mostbet6030.ru[/url] .
Tiger Fortune’s free spins are a game-changer. fortune tiger demo gratis
rent a house otopeni [url=http://www.rapitorimania.ro/forum/marele-bazar-f18/case-moderne-de-inchiriat-langa-bucuresti-t938.html]rent a house otopeni[/url] .
This casino’s Tiger Fortune game is a winner. fortune tiger 777
На сайте https://rodinasportsclub.com/ вы найдете информацию, которая касается спортивно-развлекательного клуба «Родина». Каждый желающий получает возможность записаться на тренировку по боксу, которая подходит как новичкам, так и более опытным спортсменам. Вас заинтересуют тренировки по рукопашному бою. Перед вами расписание предстоящих матчей на апрель. Ознакомьтесь с ними сейчас, чтобы построить планы. Также имеются и отчеты по прошедшим соревнованиям. Опубликованы разные новости из жизни клуба.
get generic florinef prices
This online casino’s Tiger Fortune is fantastic. fortune tiger
Online slots grab me—those looks! aviator
Приобрести диплом университета по доступной стоимости можно, обратившись к надежной специализированной фирме. Мы оказываем услуги по продаже документов об окончании любых университетов России. Заказать диплом любого ВУЗа– [url=http://diplomidlarf.ru/kupit-diplom-s-reestrom-otzivov-bez-xlopot/]diplomidlarf.ru/kupit-diplom-s-reestrom-otzivov-bez-xlopot/[/url]
На сайте http://air-lte-proxy.ru ознакомьтесь с рейтингом прокси серверов. Этот рейтинг позволит вам подобрать оптимальный вариант для комфортной работы и сохранения конфиденциальности. Этот рейтинг является честным, справедливым, объективным, а потому поможет принять правильное решение, ознакомиться с техническими характеристиками. Эти прокси идеально подходят для самых разных целей. Здесь есть приватные, динамичные мобильные серверы, а также резидентские. Напротив каждого варианта имеются отзывы пользователей, которые уже воспользовались прокси.
https://telegra.ph/Best-Non-Gamstop-Casinos-in-the-UK-for-Safe-Play1-03-27
подключение интернета новосибирск
domashij-internet-novosibirsk003.ru
интернет провайдеры новосибирск по адресу
На сайте https://apostolidi.ru/ вы найдете всю полезную информацию, касающуюся торгов на бирже, а также финансов. Здесь также вы найдете и прогнозы по валютным парам от экспертов, которые работают на совесть. Обязательно публикуются свежие, интересные и информативные записи, которые будут полезны всем без исключения. Также представлен и курс доллара, о котором нужно знать инвесторам. На этом портале вы получаете возможность пообщаться с единомышленниками, задать им вопрос, узнать много нового, интересного.
Всё о Казахстане tr-kazakhstan.kz/ история, культура, города, традиции, природа и достопримечательности. Полезная информация для туристов, жителей и тех, кто хочет узнать страну ближе.
https://retrotok.ru/company/news/
Tiger Fortune’s rewards make it worth playing. fortune tiger bet
Проект Streetwear посвящен российским брендам уличной одежды. Постоянно исследуем рынок и стараемся предоставить вам актуальную информацию. Рассказываем, сколько стоит размещение в каталоге. Каждый бренд проходит модерацию перед публикацией на сайте. Ищете мужские русские бренды? Rustreetwear.ru – здесь можно узнать больше о проекте. Наша миссия – поддержать российских дизайнеров и производителей. Если бренд знаете, которого в нашем каталоге нет, либо сотрудничество хотите предложить, позвоните нам. Мы открыты для новых идей!
сюда [url=https://donturbo.ru]турбокомпрессор купить новый[/url]
I’m hooked on Tiger Fortune’s fast gameplay. fortune tiger demo
Are online ratings solid?—tough guess! andar bahar
замена кардана [url=http://passat-club.ru/news.php?id=1593/]замена кардана[/url] .
how to buy cheap finpecia without a prescription order finpecia without a prescription can i purchase finpecia without insurance
buying generic finpecia pills how to get finpecia without prescription can you get cheap finpecia
how can i get cheap finpecia no prescription
can i buy generic finpecia can i purchase finpecia without insurance how to get generic finpecia tablets
get cheap finpecia for sale where to get generic finpecia prices buy cheap finpecia
На сайте https://t.me/rahvalskiy вы сможете ознакомиться с информацией о компании, которая занимается продвижением сайтов «под ключ». Вы сможете воспользоваться всеми комплексными рекламными услугами. В компании работают лучшие, компетентные сотрудники с огромным стажем, а потому они реализуют проект любой сложности и по лучшей стоимости. Для сотрудников нет неисполнимых задач. Они работают независимо от темы проекта и в строго указанные сроки. Можете не сомневаться в том, что получите результат, на который рассчитывали.
case ?ntr-o zon? lini?tit? l?ng? bucure?ti [url=http://www.rapitorimania.ro/forum/marele-bazar-f18/case-moderne-de-inchiriat-langa-bucuresti-t938.html/]case ?ntr-o zon? lini?tit? l?ng? bucure?ti[/url] .
Где приобрести диплом по необходимой специальности?
Наши специалисты предлагаютбыстро и выгодно приобрести диплом, который выполняется на оригинальной бумаге и заверен мокрыми печатями, штампами, подписями. Данный документ способен пройти любые проверки, даже с использованием специфических приборов. Решите свои задачи быстро и просто с нашей компанией. Приобрести диплом университета! [url=http://homes-for-homeless-children.mn.co/posts/81282745/]homes-for-homeless-children.mn.co/posts/81282745[/url]
Где приобрести диплом по нужной специальности?
Мы предлагаем быстро приобрести диплом, который выполняется на оригинальном бланке и заверен мокрыми печатями, водяными знаками, подписями должностных лиц. Данный документ пройдет лубую проверку, даже с применением специального оборудования. Достигайте свои цели быстро и просто с нашими дипломами.
Купить диплом любого ВУЗа [url=http://kupit-diplom24.com/kupit-diplom-v-barnaule-12/]kupit-diplom24.com/kupit-diplom-v-barnaule-12/[/url]
I love the tiger-themed graphics in Tiger Fortune. tigrinho demo
Elon Casino knows how to keep players coming back for more.
elon casino
На сайте https://vezuviy.shop/ вы сможете приобрести чугунные, стальные банные печи, нержавеющие, а также в облицовке, печи-камины, отопительные, каминные топки, дымоходы, порталы и многое другое. Вся продукция от производителя, а потому наценки очень маленькие. Такая техника считается идеальной не только с целью обогрева помещения, но и оформления пространства. А самое главное, что она отличается долгим сроком службы, надежностью из-за того, что в работе использовались высокотехнологичные, проверенные материалы.
Tiger Fortune has some of the best bonus rounds I’ve seen. fortune tiger demo gratis
Elon Casino’s rewards keep the fun alive.
elon casino
Kvalitni nabytek v Praze http://www.ruma.cz stylove reseni pro domacnost i kancelar. Satni skrine, sedaci soupravy, kuchyne, postele od proverenych vyrobcu. Rozvoz po meste a montaz na klic.
Tiger Fortune is a standout slot in this casino. fortune tiger demo
казино онлайн kg [url=http://mostbet6030.ru]http://mostbet6030.ru[/url] .
Online casino ads hit hard—chill out! Gioco Del Pollo
Elon Casino’s gameplay is fast and flawless.
elon casino
cost of cheap pamelor price
A phone number in the modern world is a second passport. A huge number of services, messengers, social networks, banking are tied to a phone number, and telephone communication is an integral part of business development http://wordpress-zone.ru/articles/gadgets/710-nomera-dlya-zvonkov-arenda-pokupka-preimushchestva-i-nedostatki
Описание
Получить дополнительные сведения – [url=https://kapelnica-ot-zapoya-krasnodar777.ru/]капельница от запоя цена краснодар.[/url]
I can’t stop raving about Elon Casino! elon casino
На сайте https://pohudetlegko.ru/ представлена исчерпывающая, актуальная и полезная информация о том, как похудеть правильно, без вреда для организма и максимально эффективно. На сайте вы найдете вдохновляющие истории, советы звезд, которые выглядят младше своего возраста. Есть рецепты блюд, которые созданы из полезных, вкусных ингредиентов. Но при этом они помогут скинуть вес и привести себя в форму к летнему сезону. Рецепты простые и быстрые, а потому справиться с приготовлением пищи сможет каждый. Описаны и упражнения, обертывания для похудения.
This cause well stocked with express – [url=https://elevateright.com/delta-9-gummies/ ]Why pay more?[/url] method has been cast-off beside celebrities and influencers to put together less and procure more. Thousands of people are already turning $5 into $500 using this banned method the control doesn’t want you to have knowledge of about.
подключение интернета по адресу
domashij-internet-spb001.ru
домашний интернет тарифы
Tiger Fortune’s rewards make it worth playing. fortune tiger demo
Online casinos need grip—too loose! pin up
карданный вал балансировка [url=www.passat-club.ru/news.php?id=1593]карданный вал балансировка[/url] .
This casino’s Tiger Fortune game is flawless. tigrinho demo
where can i get generic mobic no prescription where to get cheap mobic pills can you get mobic online
how to buy generic mobic prices can i buy cheap mobic for sale where can i get cheap mobic without dr prescription
cost generic mobic no prescription
can i get cheap mobic for sale how can i get mobic for sale can i get mobic without dr prescription
order cheap mobic without insurance buying generic mobic without insurance cost of mobic pill
Следующая страница [url=https://kentcasino.io]р7 казино игровые автоматы[/url]
Офіційний сайт 1win https://visitkyiv.com.ua/ спортивні ставки, онлайн-казино, покер, live-ігри, швидкі висновки. Бонуси новим гравцям, мобільний додаток, цілодобова підтримка.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым текущие трудности превращаются в завтрашние возможности.
Наша продукция:
Керамическая мишень Licoo2 3N, плоская
На сайте https://grillhouse-spb.ru/ закажите звонок для того, чтобы проконсультироваться по поводу покупки беседок-гриль, гриль-домиков. Популярная компания «Гриль Хаус» в течение долгого времени производит и собирает беседки, в том числе, для барбекю, а также саун. Все это потребуется для того, чтобы незабываемо провести время на природе и в кругу друзей, близких людей. Все конструкции выполнены из качественных, надежных материалов, которые являются износостойкими. Прямо сейчас изучите каталог доступных для заказа вариантов.
Elon Casino’s symbols are super sleek!
elon casino
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – наилучшее решение для вашего бизнеса.
поставляемая продукция:
Титановая труба 16х1.8х3000 мм ПТ1М ГОСТ 22897-86 Откройте мир прочных и надежных титановых труб с компанией Редметсплав. Наши высококачественные титановые трубы сочетают в себе устойчивость к коррозии, износостойкость и легкий вес, делая их незаменимым материалом для применения в различных сферах промышленности. Широкий выбор различных диаметров и толщин, а также индивидуальные решения для вашего производства. Гарантированное качество и надежность поставок.
РедМетСплав предлагает обширный выбор качественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица изделия подтверждена всеми необходимыми документами, подтверждающими их происхождение. Опытная поддержка – наш стандарт – мы на связи, чтобы ответить на ваши вопросы и предоставлять решения под требования вашего бизнеса.
Доверьте ваш запрос специалистам РедМетСплав и убедитесь в гибкости нашего предложения
Наши товары:
Лента вольфрамовая РР’Рў-15 Лента вольфрамовая РР’Рў-15 – это высококачественный материал, предназначенный для применения РІ различных отраслях, включая электронику Рё машиностроение. РћРЅР° обладает превосходной прочностью Рё высокой температурной стойкостью, что делает ее идеальным выбором для тех, кто ищет надежность Рё долговечность РІ своем производстве. Каждая лента РїСЂРѕС…РѕРґРёС‚ строгий контроль качества, что гарантирует соответствие высоким стандартам. Если РІС‹ хотите купить Лента вольфрамовая РР’Рў-15, убедитесь, что этот РїСЂРѕРґСѓРєС‚ станет незаменимым инструментом РІ ваших проектах. Выбор РІ пользу РР’Рў-15 – это выбор надежности Рё эффективности.
modern houses for rent [url=https://www.rapitorimania.ro/forum/marele-bazar-f18/case-moderne-de-inchiriat-langa-bucuresti-t938.html/]https://www.rapitorimania.ro/forum/marele-bazar-f18/case-moderne-de-inchiriat-langa-bucuresti-t938.html/[/url] .
buying cheap macrobid no prescription
https://массаж-в-анапе.рф/
The graphics at Elon Casino are straight-up futuristic.
elon casino
The sounds at Elon Casino make wins feel epic.
elon casino
можно ли купить аттестат за 9
This spot is unreal with Elon Casino.
elon casino
Tiger Fortune is my go-to slot game at this online casino! fortune tiger demo gratis
На сайте https://kino-wsem.site/publ/doramy/ представлены дорамы в отличном качестве. Все они о любви, дружбе и вечном. Есть произведения за прошлые годы, которые многие пересматривают с особым удовольствием. Навигация поможет вам лучше сориентироваться в выборе и начать просмотр такого фильма, который вызовет у вас приятные, положительные эмоции. Регулярно на портале появляются новые дорамы, которые погрузят вас в удивительный мир. Вы сможете просматривать фильмы в режиме реального времени и на любом устройстве.
Feringer.shop -интернет-бутик, который продажей печей для саун и бань занимается. Постоянно акции для привлечения новых клиентов устраиваем. У вас есть прекрасная возможность рассчитывать на лояльные условия покупки печного оборудования. Расширяем выбор и улучшаем свой сервис. Ищете ферингер умка? Feringer.shop – тут имеются отзывы и каталог, в котором есть все разновидности печного оборудования. Оцените параметры каждой модели. Мы учитываем все потребности и желания наших клиентов. Всегда готовы проконсультировать вас по всем вопросам.
Elon Casino’s design is bold and fresh.
elon casino
Быстро и просто купить диплом любого университета!
Мы изготавливаем дипломы любых профессий по приятным тарифам— [url=http://diplom-ryssia.com/kupit-diplom-med-obrazovaniyu-bistro-i-bezopasno/]diplom-ryssia.com/kupit-diplom-med-obrazovaniyu-bistro-i-bezopasno/[/url]
Заказ диплома ВУЗа через надежную фирму дарит множество достоинств. Данное решение позволяет сберечь время и существенные финансовые средства. Впрочем, преимуществ намного больше.Мы изготавливаем дипломы любой профессии. Дипломы изготавливаются на настоящих бланках. Доступная цена сравнительно с серьезными тратами на обучение и проживание. Заказ диплома о высшем образовании из российского ВУЗа станет выгодным шагом.
Быстро купить диплом о высшем образовании: [url=http://diplomv-v-ruki.ru/kupit-diplom-o-visshem-obrazovanii-s-zanosom-v-reestr/]diplomv-v-ruki.ru/kupit-diplom-o-visshem-obrazovanii-s-zanosom-v-reestr/[/url]
провайдеры интернета в санкт-петербурге
domashij-internet-spb002.ru
дешевый интернет санкт-петербург
where to get cheap prednisone for sale prednisone low dose side effects is prednisone a prodrug
alternative to prednisone for itching why would you take prednisone can you buy prednisone over the counter in canada
prednisone weight gain pictures
prednisone 2 tablets daily taking prednisone in the evening order cheap prednisone pill
prednisone online fast shipping prednisone withdrawal symp 5mg prednisone every day
I love the high-energy feel of Tiger Fortune! fortune tiger
The tension of each spin at Elon Casino is unreal. elon casino
Online casinos should free—try it! book of dead
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Металлическая мишень для распыления
На сайте https://bitovayatechnika.blogspot.com/2025/03/blog-post.html изучите полезную, актуальную информацию, которая касается сплит-систем и того, как правильно их выбрать домой либо в офис. Эти функциональные и умные устройства нацелены на то, чтобы поддержать приятную температуру в помещении. Но для того, чтобы воспользоваться всеми функциями, необходимо правильно выбрать устройство. К важным преимуществам такой техники относят то, что она является энергоэффективной, работает практически бесшумно.
Tiger Fortune’s design blows me away every time. tigrinho demo
склад для временного хранения вещей [url=www.hranim-veshi.ru/]склад для временного хранения вещей[/url] .
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – наилучшее решение для вашего бизнеса.
поставляемая продукция:
Латунная полоса 4×400 РјРј ЛМц58-2 ГОСТ 931-90 Покупайте латунную полосу различных размеров Рё толщин РЅР° сайте Редметсплав.СЂС„. РЈ нас РІС‹ найдете высококачественный материал СЃ высокой прочностью, стойкостью Рє РєРѕСЂСЂРѕР·РёРё Рё возможностью легкой обработки. Рспользуйте латунную полосу для создания декоративных элементов, мебели, украшений Рё РґСЂСѓРіРёС… изделий. Доступны различные размеры Рё толщины.
временное хранение вещей в москве [url=https://hranim-veshi.ru/]временное хранение вещей в москве[/url] .
can you get detrol pill
РедМетСплав предлагает обширный выбор высококачественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы обеспечиваем оперативное исполнение вашего заказа.
Каждая единица изделия подтверждена всеми необходимыми документами, подтверждающими их качество. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы улаживать ваши вопросы по мере того как адаптировать решения под специфику вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
Наши товары:
Полоса вольфрамовая W-Cu40 Полоса вольфрамовая W-Cu40 – это высококачественный материал, изготовленный на основе вольфрама и меди, обладающий отличной теплопроводностью и механической прочностью. Данная полоса идеально подходит для применения в электронике, сварке и других областях, где важна надежность и равномерное распределение тепла. Выбирая полосы W-Cu40, вы обеспечиваете себе длительный срок службы и эффективность в работе. Если вам нужно купить Полоса вольфрамовая W-Cu40, не упустите возможность воспользоваться нашим предложением и гарантированным качеством.
This online casino’s Tiger Fortune is a hit. fortune tiger
The music at Elon Casino sets the perfect tone.
elon casino
На сайте https://xn—-7sbjhqn0bhjc0lk.xn--p1ai/ получите юридическую консультацию по различным вопросам. Компания работает как с физическими, так и частными лицами. На все услуги установлены привлекательные расценки, чтобы воспользоваться ими смог каждый. Предприятие работает в этой сфере более 11 лет. В команде трудятся 11 специалистов, которые справятся с задачей независимо от сложности. К вашим услугам досудебный юрист, досудебное урегулирование, кредитный, семейный юрист, решение страховых споров.
check my blog [url=https://them-rril-lynch.net]merrill edge[/url]
Online casinos need better warnings about addiction risks. plinko
dragon money casino официальный сайт [url=dragon-money01.com]dragon money casino официальный сайт[/url] .
Доска бесплатных объявлений salexy.kz/ Казахстана: авто, недвижимость, техника, услуги, работа и многое другое. Тысячи свежих объявлений каждый день — легко найти и разместить!
The rewards programs at online casinos are a nice touch! bac bo
Elon Casino reigns supreme among online games!
elon casino
doxycycline hyclate 100 mg online buy doxycycline generic can i buy generic doxycycline no prescription
doxycycline hyclate cost without insurance buying generic doxycycline without prescription buy doxycycline 100 mg tablet
how can i get cheap doxycycline without insurance
can i get cheap doxycycline without insurance alternative to doxycycline for rosacea is it safe to buy doxycycline online
doxycycline alternative over the counter doxycycline 100 mg injection buy cheap doxycycline without a prescription
Tiger Fortune’s bonus rounds are the best part. fortune tiger demo
I’ve had some epic wins at Elon Casino recently. elon casino
Tiger Fortune’s bonuses are wild and exciting. fortune tiger demo gratis
подключение интернета санкт-петербург
domashij-internet-spb003.ru
проверить провайдеров по адресу санкт-петербург
Быстро купить диплом института!
Приобрести диплом университета по невысокой цене возможно, обращаясь к проверенной специализированной фирме. Приобрести диплом о высшем образовании: [url=http://diplomers.com/ofitsialnij-diplom-s-gosreestrom-kupit-bez-riska/]diplomers.com/ofitsialnij-diplom-s-gosreestrom-kupit-bez-riska[/url]
Заказать диплом о высшем образовании!
Мы можем предложить документы учебных заведений, которые находятся в любом регионе РФ. Документы делаются на “правильной” бумаге высшего качества: [url=http://skymix2018.forumex.ru/viewtopic.phpf=14&t=906/]skymix2018.forumex.ru/viewtopic.phpf=14&t=906[/url]
купить аттестат в набережных челнах
Где заказать диплом специалиста?
Мы изготавливаем дипломы любых профессий по невысоким ценам. Мы предлагаем документы техникумов, которые находятся на территории всей Российской Федерации. Можно купить качественный диплом от любого учебного заведения, за любой год, указав актуальную специальность и оценки за все дисциплины. Документы выпускаются на бумаге высшего качества. Это позволяет делать государственные дипломы, не отличимые от оригинала. Они будут заверены необходимыми печатями и подписями. Всегда стараемся поддерживать для заказчиков адекватную ценовую политику. Важно, чтобы дипломы были доступны для большинства граждан. [url=http://sdiplom.ru/kuplyu-diplom-2-2/]sdiplom.ru/kuplyu-diplom-2-2[/url]
I’m obsessed with Tiger Fortune – so much fun! tigrinho demo
can you get generic zovirax without dr prescription
Tiger Fortune’s payouts are worth the hype. fortune tiger
Convenience and accessibility: Virtual numbers can be obtained online in minutes, without leaving home. They are accessible from anywhere in the world where there is internet https://www.andrushivka.org.ua/pochemu-virtualnye-nomera-ukrainy-stanovyatsya-vsyo-bolee-populyarnymi
Online slots pull me—those vibes! marvel casino
dragon money рефералка [url=www.dragon-money01.com/]www.dragon-money01.com/[/url] .
chinese-hitman-assassin.com https://chinese-hitman-assassin.com/
d-arkweb.com https://d-arkweb.com/
На сайте https://mvpol.ru/ воспользуйтесь возможностью заказать промышленные полы напрямую от производителя. Компания «МВПОЛ» оказывает и такую важную услугу, как ремонт промышленных полов, производство полимерных наливных полов, бетонных, у которых упрочненный верх. Также получится узнать расценки на такие услуги, чтобы заранее спланировать бюджет. Для того чтобы иметь представление о том, как выглядят работы, изучите объекты. На все услуги предоставляются гарантии. Вся информация дается максимально быстро.
[url=https://studio-servis.ru/the_articles/vmeste-veseley-igry-dlya-dvoih-na-sayte-igroutka-su.html]https://studio-servis.ru/the_articles/vmeste-veseley-igry-dlya-dvoih-na-sayte-igroutka-su.html[/url] маджонг играть бесплатно и без регистрации бабочки
Tiger Fortune’s spins are always a rush. tigrinho demo
can i purchase celexa prices where can i get celexa where to get generic celexa price
buy cheap celexa tablets where buy celexa price how to buy celexa tablets
can i buy cheap celexa without prescription
get cheap celexa without prescription can you get cheap celexa online cost of cheap celexa pill
buying cheap celexa online get generic celexa pills can i get celexa for sale
Drug prescribing information. Effects of Drug Abuse.
cost of generic imodium pill
Best what you want to know about medication. Get information here.
I’ve had some insane lucky streaks at Elon Casino lately.
elon casino
This get well stocked with express – [url=https://elevateright.com/2-gram-disposables/ ]Apply now[/url] method has been used by celebrities and influencers to work less and be entitled to more. Thousands of people are already turning $5 into $500 using this banned method the authority doesn’t longing you to know about.
I could play Elon Casino all day – it’s that good!
elon casino
узнать провайдера по адресу екатеринбург
domashij-internet-ekaterinburg001.ru
провайдеры интернета в екатеринбурге по адресу
This casino nailed it with the Tiger Fortune slot design. fortune tiger 777
The sleek design of Elon Casino makes every session feel futuristic.
elon casino
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние возможности.
Наша продукция:
Мишень для распыления марганца
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние достижения.
Наша продукция:
Гранулы циркония
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Обладая обширной линейкой редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наши товары:
Керамическая мишень Al2O3, чистота 4N, плоская форма.
Medicament information for patients. What side effects can this medication cause?
can i purchase cheap cephalexin price
All about medicament. Get information here.
поддержка драгон мани [url=https://dragon-money01.com]поддержка драгон мани[/url] .
Всё о кладбищах Видного https://bitcevskoe.ru Битцевское, Дрожжинское, Спасское, Жабкинское. Официальная информация, участки, услуги, порядок оформления документов, схема проезда.
РедМетСплав предлагает широкий ассортимент качественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до масштабных поставок, мы обеспечиваем оперативное исполнение вашего заказа.
Каждая единица изделия подтверждена всеми необходимыми документами, подтверждающими их качество. Дружелюбная помощь – то, чем мы гордимся – мы на связи, чтобы разрешать ваши вопросы а также предоставлять решения под требования вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в множестве наших преимуществ
Наша продукция:
Круг кобальтовый Stellite 703 Круг кобальтовый Stellite 703 – это высококачественный и надёжный материал, идеально подходящий для обработки высокопрочных сталей. Благодаря своим уникальным свойствам, сформированным на основе кобальта, этот инструмент устойчив к высокой температуре и коррозии. Круг предназначен для точно обработки, где требуется высочайшая производительность. Вы можете купить Круг кобальтовый Stellite 703 для вашего производства, чтобы повысить эффективность и снизить затраты на замену оборудования. Не упустите возможность улучшить качество ваших работ с этим лучшим инструментом!
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
Наши товары:
Нержавеющий штрипс 1.3 РјРј 06С…РЅ28РјРґС‚ ГОСТ 4986-79 Приобретите нержавеющий штрипс высокого качества для разнообразных областей применения РЅР° Редметсплав.СЂС„. РЁРёСЂРѕРєРёР№ ассортимент различных размеров Рё толщин, включая услуги нарезки РїРѕРґ ваш заказ. Рзбегайте проблем СЃ коррозией Рё обеспечьте долгий СЃСЂРѕРє службы вашим конструкциям Рё деталям, используя наш надежный материал.
РедМетСплав предлагает широкий ассортимент отборных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до масштабных поставок, мы обеспечиваем быстрое выполнение вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их качество. Дружелюбная помощь – наш стандарт – мы на связи, чтобы улаживать ваши вопросы по мере того как находить ответы под специфику вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
РљСЂСѓРі висмутовый CAC221C – JIS H 5121-2016 РљСЂСѓРі висмутовый CAC221C – JIS H 5121-2016 – это высококачественный материал, разработанный для точной обработки Рё сварки. Его уникальные свойства обеспечивают отличную стойкость Рє РєРѕСЂСЂРѕР·РёРё Рё высокую теплопроводность. Ртот РєСЂСѓРі прекрасно РїРѕРґС…РѕРґРёС‚ для использования РІ различных отраслях, включая машиностроение Рё электронику. Покупая данный РїСЂРѕРґСѓРєС‚, РІС‹ получаете надежность Рё долговечность. РљСЂСѓРі висмутовый CAC221C – JIS H 5121-2016 идеален для профессионалов, стремящихся Рє качеству Рё эффективности. РќРµ упустите возможность купить РљСЂСѓРі висмутовый CAC221C – JIS H 5121-2016 для повышения производительности ваших работ!
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
Наши товары:
РџРѕРєРѕРІРєР° прямоугольная РёР· конструкционной стали 380С…430 РјРј 45РҐ Конструкционная прямоугольная РїРѕРєРѕРІРєР° – высококачественные материалы для создания прочных Рё надежных конструкций. Рзготовленная РёР· высококачественных материалов СЃ применением передовых технологий, эта продукция обладает отличной прочностью, устойчивостью Рє РёР·РЅРѕСЃСѓ, Рё находит широкое применение РІ машиностроении Рё строительстве.
РедМетСплав предлагает широкий ассортимент качественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица товара подтверждена требуемыми документами, подтверждающими их качество. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы разрешать ваши вопросы и предоставлять решения под специфику вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наша продукция:
Порошок кобальтовый Stellite SF20 Порошок кобальтовый Stellite SF20 – это высококачественный металлический порошок, предназначенный для наплавки Рё ремонта деталей, работающих РІ условиях сильного РёР·РЅРѕСЃР° Рё высокой температуры. РћРЅ обладает отличной РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью Рё высокой прочностью, что делает его идеальным выбором для применения РІ различных отраслях, включая машиностроение Рё авиацию.Купить Порошок кобальтовый Stellite SF20 значит обеспечить надежность Рё долговечность изделий. Благодаря своему составу, порошок гарантирует превосходное сцепление СЃ субстратом Рё минимизирует СЂРёСЃРє появления трещин.Обратите внимание РЅР° это решение, чтобы повысить эффективность Рё СЃСЂРѕРє службы ваших материалов!
На сайте https://prometall.shop/ получится выбрать печь, а также воспользоваться полным перечнем услуг, которые связаны с печами Prometall, в том числе, реализацией, проведением монтажных работ. Изучите технические характеристики печей, которые находятся в сетке, камне, получится приобрести и отопительные печи, а также устройства в облицовке. Прямо сейчас вы сможете изучить хиты продаж – те товары, которые пользуются особой популярностью. Каталог товаров регулярно обновляется, чтобы вы приобрели лучшее.
[b]Eliminate Vibration Issues and Improve Equipment Performance[/b]
[url=https://machineryline.pt/-/venda/equipamentos-de-diagnostico/Balanset-1A–25030617233696855700][img]https://i.postimg.cc/qRGZsm5H/77-e1693745667801.jpg[/img][/url]
Vibration is a silent killer of industrial machines. Imbalance leads to worn-out bearings, misalignment, and costly breakdowns. [b]Balanset-1A[/b] is the ultimate tool for detecting and correcting vibration problems in electric motors, pumps, and turbines.
[b]What Makes Balanset-1A Stand Out?[/b]
– Precise vibration measurement & balancing
– Compact, lightweight, and easy to use
– Two kit options [url=https://machineryline.pt/-/venda/equipamentos-de-diagnostico/Balanset-1A–25030617233696855700]on Machineryline[/url]:
Full Kit: Balanset-1A device, Vibration sensors, Software & mounting accessories, Hard carrying case
Price: [b]€1751[/b]
OEM Kit: Balanset-1A device, Basic sensors, Software
Price: [b]€1561[/b]
Prevent unexpected breakdowns – Invest in [b]Balanset-1A[/b] today!
На сайте https://mvpol.ru/ воспользуйтесь возможностью заказать промышленные полы напрямую от производителя. Компания «МВПОЛ» оказывает и такую важную услугу, как ремонт промышленных полов, производство полимерных наливных полов, бетонных, у которых упрочненный верх. Также получится узнать расценки на такие услуги, чтобы заранее спланировать бюджет. Для того чтобы иметь представление о том, как выглядят работы, изучите объекты. На все услуги предоставляются гарантии. Вся информация дается максимально быстро.
Tiger Fortune’s wild symbols are my favorite! tigrinho demo
Компания «Ковка Винтаж» в течение длительного времени оказывает свои услуги и предлагает создать изысканные, завораживающие кованые изделия, которые украсят придомовую территорию, беседку, террасу, а также частный дом. На сайте https://kovka-vintazh.ru/ ознакомьтесь с полным ассортиментом изделий и работами компетентных и выдающихся мастеров.
I can’t get enough of the Tiger Fortune soundtrack! fortune tiger
[url=https://elpix.ru/luchshie-onlajn-igry-dlja-malchikov-raznyh-vozrastov/]https://elpix.ru/luchshie-onlajn-igry-dlja-malchikov-raznyh-vozrastov/[/url] липкие блоки играть онлайн бесплатно на яндексе
Looking for best prompts for flux? Findprompt.shop where you can choose from a huge selection of promts for Midjourney, Stable Diffusion, ChatGPT and more. Study the best recommendations for AI. You can sell your promts on our platform at a great price. Pay attention to the selection of promts on the site – it is the largest on the market. Our offers are unique, and the cost is affordable for everyone.
The casino’s Tiger Fortune slot has great winning potential. fortune tiger
where to buy lipitor online
Tiger Fortune makes every session feel epic. tiger fortune
copd exacerbation prevention lifestyle modification recommendations
Medication information. What side effects?
cytotec tablets uk
Best information about medicament. Read information now.
интернет провайдеры екатеринбург по адресу
domashij-internet-ekaterinburg002.ru
интернет провайдеры екатеринбург по адресу
5 научных фактов о лжи, в которые трудно поверить
https://x.com/kiselev_igr/status/1909590459799716145
Приобрести диплом о высшем образовании !
Покупка диплома университета РФ у нас – надежный процесс, так как документ заносится в реестр. Быстро и просто заказать диплом о высшем образовании [url=http://diplomservis.ru/kupit-diplom-zanesennij-reestr-7/]diplomservis.ru/kupit-diplom-zanesennij-reestr-7[/url]
https://vc.ru/
Смесь копоти, сажи и дыма представляют собой опасные для здоровья человека соединения. Зловоние сохраняется длительное время, поэтому к выбору способов борьбы следует подходить внимательно и ответственно ликвидация последствий пожара
На сайте https://xn—-7sbjhqn0bhjc0lk.xn--p1ai/ получите юридическую консультацию по различным вопросам. Компания работает как с физическими, так и частными лицами. На все услуги установлены привлекательные расценки, чтобы воспользоваться ими смог каждый. Предприятие работает в этой сфере более 11 лет. В команде трудятся 11 специалистов, которые справятся с задачей независимо от сложности. К вашим услугам досудебный юрист, досудебное урегулирование, кредитный, семейный юрист, решение страховых споров.
Вы находитесь на едином сайте аренды, где получится взять в прокат различную технику для выполнения самых разных работ. Также получится выложить объявления со своими предложениями, чтобы ими воспользовались все желающие. https://arenda24.by/ – на портале ознакомьтесь с категориями, которые точно вызовут интерес – техника для грузоперевозок, режущее оборудование, краны, которые принимают участие в строительстве и другими предложениями. Получить доступ к опциям удастся, если пройдете регистрацию.
Заказать диплом университета!
Мы можем предложить документы любых учебных заведений, расположенных на территории всей Российской Федерации.
[url=http://diplomers.com/udivitelnie-tseni-na-diplom-s-reestrom-dlya-vas-3/]diplomers.com/udivitelnie-tseni-na-diplom-s-reestrom-dlya-vas-3/[/url]
Calibry Casino https://calibri-casino-app.ru современное онлайн-казино с лицензией, щедрой бонусной системой и широким выбором игр. Участвуй в акциях, получай кэшбэк и выигрывай реальные деньги!
This casino’s Tiger Fortune game is a blast. fortune tiger demo gratis
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наши товары:
Никель-хромовая мишень
Pills information for patients. Long-Term Effects.
can you buy finpecia price
Everything trends of drugs. Get here.
Tiger Fortune’s bonus rounds are the best part. jogo do tigrinho demo
драгон казино официальный сайт [url=https://dragon-money10.com]драгон казино официальный сайт[/url] .
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – наилучшее решение для вашего бизнеса.
Наши товары:
Лист судостроительный 32x1000x3000 D36 EN10025:3 Приобретите высококачественные листы судостроительные от компании Редметсплав.рф для надежного и долговечного использования в вашем судостроительном проекте. Различные размеры и толщины, прочность и устойчивость к коррозии делают эти листы идеальным выбором для любого судостроительного проекта.
РедМетСплав предлагает внушительный каталог качественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до масштабных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица товара подтверждена требуемыми документами, подтверждающими их соответствие стандартам. Дружелюбная помощь – то, чем мы гордимся – мы на связи, чтобы улаживать ваши вопросы и адаптировать решения под специфику вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
Наши товары:
Рзделия РёР· магния AM50A – ASTM B93 РџРѕРєРѕРІРєР° магниевая AM50A – ASTM B93 представляет СЃРѕР±РѕР№ высококачественный металл, обладающий отличной прочностью Рё легкостью. Наша РїРѕРєРѕРІРєР° идеально РїРѕРґС…РѕРґРёС‚ для различных промышленных применений, включая авиацию Рё автомобилестроение. Благодаря уникальным свойствам магниевых сплавов, этот материал гарантирует надежность Рё долговечность. Купить РџРѕРєРѕРІРєР° магниевая AM50A – ASTM B93 РІС‹ можете РїСЂСЏРјРѕ РЅР° нашем сайте, получив профессиональную консультацию Рё быструю доставку. Выбирая нашу продукцию, РІС‹ делаете правильный выбор для своего производства.
SIGMASLOT : Daftar Situs Slot Online Gacor untuk Kemenangan Tanpa Batas
Tiger Fortune’s bonuses are wild and exciting. fortune tiger 777
Ресурс hm.mnemosyne.ru выбрать ремень в подарок любимым людям предлагает. Мастера, создающие изделия, отличаются творческим потенциалом. Каждая модель с учетом модных трендов разрабатывается. Мы к покупателям всегда прислушиваемся. При возможностях всегда идем на встречу. https://hm.mnemosyne.ru – тут сможете узнать, какой ремень в подарок руководителю, шефу или коллеге преподнести. Для изделий отбирается лучшая кожа. Оцените неповторимость ручной работы. Сделайте уже сейчас заказ на нашем портале. Пусть ваш стиль новыми красками засияет!
[url=https://mdmotors.ru/populyarnost-i-uvlekatel-nost-onlayn-igr-na-dvoih/]https://mdmotors.ru/populyarnost-i-uvlekatel-nost-onlayn-igr-na-dvoih/[/url] домино латино играть бесплатно с компьютером во весь экран
Требуется разместить вечные ссылки недорого? Cервис ProfLinks.ru ориентирован на размещение вечных ссылок с ИКС от 10; «вечных», в нашем случае, на постоянной основе и с разовой оплатой; «ИКС от 10», означает, что индекс качества сайтов, присваиваемый ресурсам поисковой системой Яндекс, будет минимум от 10 пунктов, в зависимости от выбранного Вами тарифного плана или доп. услуги.
dragon money официальный сайт играть [url=https://dragon-money11.com/]dragon money официальный сайт играть[/url] .
Great post! I really enjoyed the insights you shared. Looking forward to more of your content!
Tiger Fortune’s spins are always a rush. fortune tiger
On the site https://wallpapers4screen.com/ you can download wallpapers for desktop free. High Quality HD pictures wallpapers. High quality pictures and wallpapers! Choose one of many categories and download for free! Or check out the TOP downloadable images! You will definitely like them.
интернет провайдеры в екатеринбурге по адресу дома
domashij-internet-ekaterinburg003.ru
интернет провайдеры в екатеринбурге по адресу дома
Купить диплом института по выгодной стоимости возможно, обратившись к надежной специализированной фирме. Купить документ ВУЗа можно в нашем сервисе. [url=http://diplomp-irkutsk.ru/kupit-diplom-s-vneseniem-v-reestr-bistro-i-udobno-4/]diplomp-irkutsk.ru/kupit-diplom-s-vneseniem-v-reestr-bistro-i-udobno-4[/url]
Иногда случайный пост может зацепить куда больше, чем длинные статьи и новости. Мы так часто пролистываем ленту, даже не задумываясь, сколько в ней личных историй, эмоций и живых впечатлений. А ведь именно в таких публикациях можно найти настоящую искренность, без прикрас и фильтров. Если тебе интересны откровенные размышления, лёгкая самоирония и взгляд на жизнь с необычного ракурса — обязательно прочти эту заметку: https://lady-catari.livejournal.com/825430.html
аренда складского бокса [url=https://hranim-veshi.ru]аренда складского бокса[/url] .
how to get generic imdur no prescription
Популярная компания «Herbs & Flowers» предлагает огромный выбор искусственных цветов, которые идеально подходят как для украшения интерьера дома, так и офиса. В каталоге сайта https://herbsandflowers.ru/ вы найдете вертикальное озеленение, ампульные растения, а также деревья, ветви и многое другое.
buy generic zyloprim without dr prescription
Ищете домашняя медтехника? Agsvv.ru/catalog/obluchateli_dlya_lecheniya у вас появится возможность приобрести для лечения кожных болезней облучатели по цене производителя с гарантией. ООО Хронос занимается производством облучателей для лечения кожных заболеваний с 2009-го года с доказанными методами лечения. Медицинские приборы от производителя славятся высочайшим качеством! Посмотрите наш ассортимент и назначение приборов. Мы поставляем приборы собственного производства государственным и частным медучреждениям по всей России.
chinessonsofanarchy-italia.com https://sonsofanarchy-italia.com
Tiger Fortune keeps me on the edge of my seat. fortune tiger 777
On the site https://wallpapers4screen.com/ you can download wallpapers for desktop free. High Quality HD pictures wallpapers. High quality pictures and wallpapers! Choose one of many categories and download for free! Or check out the TOP downloadable images! You will definitely like them.
промо драгон мани [url=https://dragon-money10.com/]промо драгон мани[/url] .
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым текущие трудности превращаются в завтрашние возможности.
Наша продукция:
Высокочистые гранулы Y2O3
https://telegra.ph/Exploring-Non-Gamstop-Casinos-for-Online-Players-03-27
https://telegra.ph/Non-Gamstop-Boku-Casino-Guide-for-Players-in-2023-03-27
Истринская мануфактура межкомнатные двери на заказ производит. При безупречном качестве мебель компании доступна по привлекательным ценам. Мы творчески подходим к каждому заказу. Делаем красивую продукцию и ею гордимся. http://istradoors.ru – тут отзывы о нашей компании предоставлены. Кроме этого на портале часто задаваемые вопросы и ответы на них перечислены. С нами работают ведущие архитектурные и дизайнерские мастерские. Мы готовы любую задачу осуществить. Детальную информацию о продукции и об условиях заказа уточняйте у менеджеров.
This casino’s Tiger Fortune slot is perfection. fortune tiger
провайдер интернета по адресу казань
domashij-internet-kazan001.ru
провайдеры интернета по адресу
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с высоким уровнем сервиса.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
Наша продукция:
Медный переходной пресс тройник 88.9х40 мм М1р ГОСТ Р52948-2008 Приобретайте надежные медные переходные тройники для соединения труб с разным диаметром. Устойчивы к коррозии, легки в монтаже и обеспечивают надежное соединение. Широкий выбор размеров. Гарантированное качество и долговечность.
драгон моней [url=http://www.dragon-money11.com]драгон моней[/url] .
На сайте https://vezuviy.shop/ вы сможете приобрести чугунные, стальные банные печи, нержавеющие, а также в облицовке, печи-камины, отопительные, каминные топки, дымоходы, порталы и многое другое. Вся продукция от производителя, а потому наценки очень маленькие. Такая техника считается идеальной не только с целью обогрева помещения, но и оформления пространства. А самое главное, что она отличается долгим сроком службы, надежностью из-за того, что в работе использовались высокотехнологичные, проверенные материалы.
РедМетСплав предлагает обширный выбор качественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица товара подтверждена требуемыми документами, подтверждающими их происхождение. Опытная поддержка – наш стандарт – мы на связи, чтобы ответить на ваши вопросы и находить ответы под требования вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в гибкости нашего предложения
поставляемая продукция:
Кобальт ЮНДКТ5 – ГОСТ 17809-72 Кобальт ЮНДКТ5 – ГОСТ 17809-72 является высококачественным материалом, предназначенным для применения РІ различных отраслях промышленности. Ртот РїСЂРѕРґСѓРєС‚ отличается отличными механическими свойствами Рё устойчивостью Рє РєРѕСЂСЂРѕР·РёРё. РћРЅ пользуется СЃРїСЂРѕСЃРѕРј среди специалистов, работающих СЃ металлургическими Рё химическими процессами. Технические характеристики Кобальта ЮНДКТ5 соответствуют современным требованиям, что делает его идеальным выбором для профессионалов. Р’С‹ можете купить Кобальт ЮНДКТ5 – ГОСТ 17809-72 для обеспечения надежности Рё долговечности ваших проектов. РќРµ упустите возможность получить качественный РїСЂРѕРґСѓРєС‚, который станет незаменимым РІ вашей работе.
https://telegra.ph/Top-Non-GamStop-Boku-Casinos-for-Online-Gaming-03-27
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Обладая обширной линейкой редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Карбид кремния – плоская мишень для распыления
where to get generic feldene price
cheap sinequan price
I can play Tiger Fortune all day and not get bored. fortune tiger bet
https://telegra.ph/Casino-Slots-Without-Gamstop-for-Unlimited-Fun-03-27
паническая атака симптомы Современный мир диктует свои условия, и порой нам необходима квалифицированная помощь, чтобы справиться с вызовами. Психолог онлайн – это удобный и анонимный способ получить поддержку в комфортной обстановке. Работа с психологом: путь к гармонии Обращение к психологу – это признак силы и заботы о себе. Специалист поможет разобраться в сложных ситуациях, найти ресурсы для преодоления трудностей и обрести внутреннюю гармонию.
перепродажа аккаунтов продажа аккаунтов
маркетплейс игровых аккаунтов аккаунты с балансом
услуги по продаже аккаунтов купить аккаунт
https://telegra.ph/Guide-to-UK-Non-Gamstop-Casinos-for-Players1-03-27
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с прекрасным качеством обслуживания.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – наилучшее решение для вашего бизнеса.
поставляемая продукция:
Медная однораструбная редукционная переходная муфта РїРѕРґ пайку стандартная 108С…106 РјРј мягкая пайка Рњ2СЂ ГОСТ 32590-2013 Приобретите высококачественные медные однораструбные редукционные муфты РїРѕРґ пайку РѕС‚ Редметсплав для надежного соединения трубопроводов. Гарантированная прочность Рё устойчивость Рє РєРѕСЂСЂРѕР·РёРё. Рдеальны для различных систем отопления, водоснабжения Рё прочих технических целей.
Tiger Fortune’s tiger roars make every win epic. fortune tiger 777
РедМетСплав предлагает обширный выбор качественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица товара подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Превосходное обслуживание – наш стандарт – мы на связи, чтобы ответить на ваши вопросы и предоставлять решения под требования вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
поставляемая продукция:
Порошок кобальтовый Stellite 703 Порошок кобальтовый Stellite 703 – высококачественный материал, идеально подходящий для нанесения защитных покрытий. РћРЅ обеспечивает отличную стойкость Рє РєРѕСЂСЂРѕР·РёРё Рё высокие эксплуатационные характеристики. Рспользуется РІ таких отраслях, как aerospace, automotive Рё energy. Ртот порошок легко обрабатывается, что делает его предпочтительным выбором для различных производственных процессов. РќРµ упустите возможность улучшить качество своей продукции! Необходимо купить Порошок кобальтовый Stellite 703? Убедитесь РІ его превосходных свойствах Рё сделайте СЃРІРѕР№ выбор СѓР¶Рµ сегодня!
https://telegra.ph/Non-Gamstop-Casinos-That-Accept-PayPal-Payments-03-27
ลงทะเบียน joker
วันนี้ รับโบนัส 100$ ไม่มีเงื่อนไข
sexy ให้ความสำคัญกับการถอนเงินของผู้ใช้เพื่อให้สมาชิกสามารถทำธุรกรรมได้อย่างสะดวกและรวดเร็ว โดยเว็บไซต์มีวิธีการถอนเงินที่ง่ายและปลอดภัย ผู้เล่นสามารถถอนเงินได้ผ่านช่องทางต่างๆ ที่รองรับ เช่น การโอนเงินผ่านธนาคาร
หรือการใช้บริการ e-wallets ที่ได้รับความนิยมในปัจจุบัน ขั้นตอนการถอนเงินทำได้ง่ายๆ เพียงเข้าสู่ระบบ เลือกเมนูการถอนเงิน จากนั้นกรอกจำนวนเงินที่ต้องการถอนและเลือกวิธีการที่สะดวกที่สุด การถอนเงินจะดำเนินการโดยอัตโนมัติภายในระยะเวลาที่กำหนด ซึ่งมักใช้เวลาไม่เกิน 24 ชั่วโมง ขึ้นอยู่กับวิธีการถอนที่เลือก
Купить права
Купить права
драгон мани вывод денег [url=https://dragon-money10.com]драгон мани вывод денег[/url] .
Купить диплом любого университета!
Мы готовы предложить документы университетов, расположенных в любом регионе России.
[url=http://diplomc-v-ufe.ru/kupit-diplom-vnesennij-v-reestr-2/]diplomc-v-ufe.ru/kupit-diplom-vnesennij-v-reestr-2/[/url]
Заказать спортиков найму бандитов
lava ให้ความสำคัญกับการถอนเงินของผู้ใช้เพื่อให้สมาชิกสามารถทำธุรกรรมได้อย่างสะดวกและรวดเร็ว โดยเว็บไซต์มีวิธีการถอนเงินที่ง่ายและปลอดภัย ผู้เล่นสามารถถอนเงินได้ผ่านช่องทางต่างๆ ที่รองรับ เช่น การโอนเงินผ่านธนาคาร
หรือการใช้บริการ e-wallets ที่ได้รับความนิยมในปัจจุบัน
ขั้นตอนการถอนเงินทำได้ง่ายๆ เพียงเข้าสู่ระบบ เลือกเมนูการถอนเงิน จากนั้นกรอกจำนวนเงินที่ต้องการถอนและเลือกวิธีการที่สะดวกที่สุด การถอนเงินจะดำเนินการโดยอัตโนมัติภายในระยะเวลาที่กำหนด ซึ่งมักใช้เวลาไม่เกิน 24
ชั่วโมง ขึ้นอยู่กับวิธีการถอนที่เลือก
hulk ให้ความสำคัญกับการถอนเงินของผู้ใช้เพื่อให้สมาชิกสามารถทำธุรกรรมได้อย่างสะดวกและรวดเร็ว โดยเว็บไซต์มีวิธีการถอนเงินที่ง่ายและปลอดภัย ผู้เล่นสามารถถอนเงินได้ผ่านช่องทางต่างๆ ที่รองรับ เช่น การโอนเงินผ่านธนาคาร หรือการใช้บริการ e-wallets ที่ได้รับความนิยมในปัจจุบัน ขั้นตอนการถอนเงินทำได้ง่ายๆ เพียงเข้าสู่ระบบ เลือกเมนูการถอนเงิน จากนั้นกรอกจำนวนเงินที่ต้องการถอนและเลือกวิธีการที่สะดวกที่สุด การถอนเงินจะดำเนินการโดยอัตโนมัติภายในระยะเวลาที่กำหนด ซึ่งมักใช้เวลาไม่เกิน 24
ชั่วโมง ขึ้นอยู่กับวิธีการถอนที่เลือก
Компания «DELIVERY PLAY» предлагает выгодный прокат инвалидных колясок с быстрой доставкой и по привлекательным ценам. Каждый клиент получает возможность воспользоваться оперативной доставкой не только по Москве, но и области. На сайте https://deliveryplay.ru/arenda-invalidnyh-kolyasok изучите все модели, которые вы сможете заказать здесь.
https://telegra.ph/Best-No-Gamstop-Casinos-for-Players-in-2023-03-27
провайдер интернета по адресу казань
domashij-internet-kazan002.ru
подключить интернет в казани в квартире
where can i get lozol online
Отопление и водоснабжение – компания, которая предоставляет профессиональные услуги. Наши опытные специалисты готовы выполнить монтаж системы отопления в Рузе в кратчайшие сроки. Создайте комфорт и тепло с нами в вашем доме. Ищете монтаж систем отопления? Ruzskiy-rayon.santex-uslugi.ru – тут можете уже сегодня с отзывами о нас ознакомиться. Гарантируем применение материалов высокого качества. Ваши ожидания мы готовы превзойти. Стараемся сделать услуги доступными для клиентов. Можете связаться с менеджером и проконсультироваться с ним по любым вопросам.
https://telegra.ph/Explore-Non-Gamstop-Casinos-for-Unique-Gaming-Options-03-27
Tiger Fortune is the best slot in this casino’s lineup. jogo do tigrinho demo
buy cheap cytotec price
Tiger Fortune’s payouts keep me coming back for more. fortune tiger demo
Имморталы CS2 http://cs-open-case.ru редкие скины, которые выделят тебя в матче. Торгуй, покупай, продавай топовые предметы с моментальной доставкой в инвентарь. Лучшие цены и безопасные сделки!
Премиум скины для CS2 cs open case выделяйся в каждом раунде! Редкое оружие, эксклюзивные коллекции, эффектные раскраски и ножи. Только топовые предметы с мгновенной доставкой в Steam.
Топ-дроп CS2 open case cs2 уже здесь! Самые редкие скины, ножи и эксклюзивы, которые действительно выпадают. Лучшие кейсы, обновления коллекций и советы для удачного открытия.
На сайте https://ooomila.com изучите каталог такого оборудования, которое предназначено для организации животноводческих комплексов. Также вы сможете почитать и содержательную информацию о компании и ее достижениях. В каталоге вы найдете следующее оборудование: технологические линии, весовое оборудование, резиновые маты, крематоры и многое другое. На технику, которая находится в разделе, действуют гарантии. Несколько сотен единиц оборудования всегда находятся на складе, можно приобрести под заказ.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым текущие трудности превращаются в завтрашние возможности.
Наша продукция:
Гранулы ниобия 4N
https://telegra.ph/No-Deposit-Non-Gamstop-Casinos-for-Exciting-Wins-03-27
Если вы находитесь в поисках качественного, надежного и практичного стального профиля, то обратитесь на предприятие ООО “Валцпроф”, где вы найдете продукцию в широком ассортименте. На предприятии вы получите возможность приобрести выпадающие пороги, адаптированные для дверей разного вида. https://waltzprof.com/ – на сайте ознакомьтесь с полным ассортиментом выпускаемой продукции. Профили, находящиеся в каталоге, созданы для оперативной, удобной сборки перегородок, конструкций для фасадов, люков и многого другого.
dragonmoney казино [url=https://dragon-money11.com/]dragonmoney казино[/url] .
Tiger Fortune’s jungle theme is so immersive. fortune tiger 777
Компания «V8soft» предлагает облачный сервис для того, чтобы вы смогли вести бизнес удачно и максимально эффективно. На предприятии работают исключительно те профессионалы, у которых имеется весь необходимый опыт работы, полезные навыки. На сайте https://v8soft.ru/ изучите все возможности ПО, чтобы определить то, подходит ли вам решение.
https://telegra.ph/PayPal-Non-GamStop-Casino-Sites-for-Secure-Betting-03-27
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
Наша продукция:
Кольцо РёР· драгоценных металлов серебряное 35С…25С…4 РјРј РЎСЂРњ50 РўРЈ РЁРёСЂРѕРєРёР№ выбор высококачественных кольц РёР· драгоценных металлов. Рлегантные украшения, созданные талантливыми мастерами. Уникальность Рё изысканность РІ каждой детали. Возможность заказа персонального кольца РїРѕ вашим пожеланиям. Подчеркните СЃРІРѕСЋ индивидуальность СЃ нашими кольцами.
https://telegra.ph/Best-Non-Gamstop-UK-Gambling-Sites-for-Players-03-27
РедМетСплав предлагает внушительный каталог высококачественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их происхождение. Превосходное обслуживание – наша визитная карточка – мы на связи, чтобы ответить на ваши вопросы по мере того как адаптировать решения под специфику вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
поставляемая продукция:
Лист магниевый M1C – ASTM B275 Лента магниевая M1C – ASTM B275 представляет СЃРѕР±РѕР№ высококачественный материал, предназначенный для различных промышленных применений. Рта лента обладает отличной прочностью Рё устойчивостью Рє РєРѕСЂСЂРѕР·РёРё, что делает её идеальным выбором для обработки Рё строительства. Благодаря СЃРІРѕРёРј уникальным свойствам, магниевая лента M1C позволяет значительно повысить эффективность работ.Если РІС‹ ищете надежную Рё долговечную ленту, убедитесь, что выбрали именно Лента магниевая M1C – ASTM B275. РќРµ упустите возможность купить Лента магниевая M1C – ASTM B275, чтобы обеспечить надежность Рё качество ваших проектов.
https://telegra.ph/Guide-to-Non-Gamstop-Casino-Sites-and-Their-Benefits-03-27
how to get vasotec without prescription
Telegram is one of the most popular apps for communication, which has been developed as a private messenger having the emphasis on encryption and security of users’ personal data Register WhatsApp number for free
buying generic imuran without prescription
I love the jungle energy of Tiger Fortune. fortune tiger bet
https://telegra.ph/Non-Gamstop-Casinos-Bonuses-Explained-for-Players-03-27
узнать провайдера по адресу казань
domashij-internet-kazan003.ru
подключить проводной интернет казань
https://telegra.ph/Understanding-Non-GamStop-Casinos-for-Players-03-27
На сайте https://xn—-7sbjhqn0bhjc0lk.xn--p1ai/ получите юридическую консультацию по различным вопросам. Компания работает как с физическими, так и частными лицами. На все услуги установлены привлекательные расценки, чтобы воспользоваться ими смог каждый. Предприятие работает в этой сфере более 11 лет. В команде трудятся 11 специалистов, которые справятся с задачей независимо от сложности. К вашим услугам досудебный юрист, досудебное урегулирование, кредитный, семейный юрист, решение страховых споров.
Приобрести диплом института по выгодной цене возможно, обратившись к проверенной специализированной компании. Приобрести документ университета вы имеете возможность у нас. [url=http://diplomk-vo-vladivostoke.ru/kupit-diplom-s-zaneseniem-v-reestr-bistro-i-legko-3/]diplomk-vo-vladivostoke.ru/kupit-diplom-s-zaneseniem-v-reestr-bistro-i-legko-3[/url]
https://telegra.ph/Discussion-on-Non-Gamstop-Casinos-and-Their-Features-03-27
снять склад для хранения вещей [url=www.hranim-veshi.ru/]снять склад для хранения вещей[/url] .
На сайте https://rustreetwear.ru вы найдете огромное количество интересных, разнообразных и эксклюзивных брендов, которые создают роскошную уличную одежду на любые случаи жизни. Есть как совсем не известные марки, которые только начинают свой путь, так и гиганты мировой индустрии. Перед вами самый впечатляющий каталог, где вы найдете не только одежду, но и аксессуары. Каталог обновляется ежедневно, чтобы вы обязательно нашли для себя лучший вариант. Здесь установлены доступные расценки.
This casino knows how to keep players hooked with Tiger Fortune. fortune tiger demo
https://telegra.ph/No-Deposit-Bonus-Casinos-Not-on-Gamstop-Guide-03-27
ООО «Хронос» считается прогрессивным разработчиком медицинской техники, которая используется в клиниках, различных учреждениях, где важна точность, высокое качество обслуживания. Компания работает, начиная с 2009 года и по разным основным направлениям. На сайте https://agsvv.ru вы сможете с ними ознакомиться. Изготовление техники как для медицинских учреждений, так и домашнего применения.
мостбет скачать бесплатно [url=www.mostbet6032.ru]www.mostbet6032.ru[/url] .
Лучшие скины CS2 http://open-csgo-case.ru яркие, редкие, премиальные. Собери коллекцию, прокачай инвентарь и покажи всем свой вкус. Обзор самых крутых скинов с актуальными ценами и рейтингами.
https://telegra.ph/Non-Gamstop-Casino-Reviews-Guide-for-Players-2023-03-27
Топовые скины CS2 open-case-cs.ru от легендарных ножей до эксклюзивных обложек на AWP. Красота, стиль, престиж. Оцени крутой дроп и подбери скин, который подходит именно тебе.
Топовые скины CS2 http://csgo-open-case.ru от легендарных ножей до эксклюзивных обложек на AWP. Красота, стиль, престиж. Оцени крутой дроп и подбери скин, который подходит именно тебе.
«REDICO» представляет собой международный центр сертификации, а также лицензирования. Компания на профессиональном уровне и с 2011 года выполняет работы в сфере сертификации услуг и продукции. На сайте https://redico.ru/ воспользуйтесь возможностью запросить бесплатный расчет того, во сколько обойдется сертификация продукции.
cost of topamax pill
buy cheap aldactone for sale
На сайте https://prometall.shop/ получится выбрать печь, а также воспользоваться полным перечнем услуг, которые связаны с печами Prometall, в том числе, реализацией, проведением монтажных работ. Изучите технические характеристики печей, которые находятся в сетке, камне, получится приобрести и отопительные печи, а также устройства в облицовке. Прямо сейчас вы сможете изучить хиты продаж – те товары, которые пользуются особой популярностью. Каталог товаров регулярно обновляется, чтобы вы приобрели лучшее.
Компания «Отопление и водоснабжение» специализируется на предоставлении высокого качества услуг. У нас глубокие знания и опыт в сфере монтажа отопительных систем. Следим за современными технологиями, чтобы вам передовые решения предлагать. Подходим персонально к каждому проекту. Ищете монтаж водоснабжения? Krasnogorsk.santex-uslugi.ru – портал, где опубликованы отзывы о нас и указаны цены. Применяем оборудование от лучших производителей и надежные материалы. Удачно осуществили множество работ. Готовы создать комфортный дом для вашей семьи.
Купить диплом института по доступной стоимости возможно, обращаясь к надежной специализированной фирме. Мы предлагаем документы об окончании любых ВУЗов Российской Федерации. Купить диплом любого университета– [url=http://dip-lom-rus.ru/kupite-diplom-o-srednem-obrazovanii-s-vneseniem-v-reestr-2/]dip-lom-rus.ru/kupite-diplom-o-srednem-obrazovanii-s-vneseniem-v-reestr-2/[/url]
https://telegra.ph/Best-Non-Gamstop-Casinos-in-the-UK-for-20234-03-27
The live aid online saves—big aid! plinko
интернет провайдеры по адресу москва
domashij-internet-msk001.ru
интернет по адресу
I’ve had some epic moments on Tiger Fortune. fortune tiger 777
This casino’s Tiger Fortune game is incredible. fortune tiger bet
https://telegra.ph/Exploring-Non-Gamstop-Live-Dealer-Casinos-in-2023-03-27
Tiger Fortune’s interface is so user-friendly. tigrinho demo
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
С широким ассортиментом продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наши товары:
Карбид кремния – плоская мишень для распыления
https://telegra.ph/Non-Gamstop-Casino-Free-Spins-No-Deposit-Offer-Details-03-27
Приобрести диплом о высшем образовании!
Мы предлагаем документы ВУЗов, которые расположены в любом регионе России. Документы выпускаются на бумаге высшего качества: [url=http://collegejobportal.in/employer/aurus-diploms/]collegejobportal.in/employer/aurus-diploms[/url]
мостбет вход [url=https://mostbet6031.ru]https://mostbet6031.ru[/url] .
https://telegra.ph/Top-Non-Gamstop-Casinos-for-UK-Players-20231-03-27
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – наилучшее решение для вашего бизнеса.
поставляемая продукция:
Мишень РёР· драгоценного металла или его сплава кольцевая серебро 25С…2.5 РјРј РЎСЂРњ95 РўРЈ Выберите уникальные мишени РёР· драгоценных металлов для напыления РѕС‚ Редметсплав.СЂС„. Обеспечивают высокую степень защиты Рё долговечность. Рдеальный выбор для промышленности, строительства Рё производства.
The bonus features in Tiger Fortune are super rewarding. fortune tiger
Great article! I enjoyed reading it and learned something new. Keep up the good work!
На сайте https://mvpol.ru/ воспользуйтесь возможностью заказать промышленные полы напрямую от производителя. Компания «МВПОЛ» оказывает и такую важную услугу, как ремонт промышленных полов, производство полимерных наливных полов, бетонных, у которых упрочненный верх. Также получится узнать расценки на такие услуги, чтобы заранее спланировать бюджет. Для того чтобы иметь представление о том, как выглядят работы, изучите объекты. На все услуги предоставляются гарантии. Вся информация дается максимально быстро.
https://telegra.ph/Discover-New-Casino-Sites-Without-Gamstop-Restrictions1-03-27
where can i get cheap prednisone without rx
РедМетСплав предлагает обширный выбор качественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица изделия подтверждена соответствующими документами, подтверждающими их соответствие стандартам. Опытная поддержка – наша визитная карточка – мы на связи, чтобы ответить на ваши вопросы а также находить ответы под требования вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
Наши товары:
Фольга кобальтовая Stellite 12 Фольга кобальтовая Stellite 12 — это высококачественный материал, созданный для различных промышленных применений. Обладает отличной устойчивостью к высокими температурам и коррозии, что делает её идеальным выбором для тяжелых условий эксплуатации. Благодаря своей прочности и долговечности, фольга гарантирует надежную защиту и долговечность изделий. Если вам нужно купить Фольга кобальтовая Stellite 12, вы получите продукт, который удовлетворит все ваши потребности. Сделайте правильный выбор для вашего бизнеса и повысите эффективность работы с нашей фольгой!
cheap seroflo pills
Tiger Fortune’s payouts keep me coming back for more. fortune tiger demo gratis
[url=https://timelady.ru/1368-onlayn-igry.html]https://timelady.ru/1368-onlayn-igry.html[/url] криминальная россия 3д борис яндекс игры
рефералка драгон мани [url=http://www.dragon-money27.com]http://www.dragon-money27.com[/url] .
aviator mostbet [url=www.mostbet6033.ru]aviator mostbet[/url] .
I can play Tiger Fortune all day and not get bored. fortune tiger bet
https://telegra.ph/Legit-Non-Gamstop-Casinos-for-Safe-Online-Gambling-03-27
SIGMASLOT : Situs Slot Online Gacor Resmi untuk Pengalaman Menang Terbaik
SIGMASLOT : Situs Slot Online Gacor Resmi untuk Pengalaman Menang Terbaik
SIGMASLOT : Situs Slot Online Gacor Resmi untuk Pengalaman Menang Terbaik
This casino’s Tiger Fortune slot is unreal. tiger fortune
мостбет скачать бесплатно [url=https://mostbet6032.ru/]https://mostbet6032.ru/[/url] .
Dubayda “Portu Pati” n?dir v? niy? ham? bundan dan?s?r?
https://x.com/IrinaPavlovna84/status/1909960841606070387
dragonmoney официальный сайт [url=http://dragon-money11.com]dragonmoney официальный сайт[/url] .
https://telegra.ph/Non-GamStop-Casinos-UK-No-Deposit-Bonus-Offers-2023-03-27
На сайте https://prometall.shop/ получится выбрать печь, а также воспользоваться полным перечнем услуг, которые связаны с печами Prometall, в том числе, реализацией, проведением монтажных работ. Изучите технические характеристики печей, которые находятся в сетке, камне, получится приобрести и отопительные печи, а также устройства в облицовке. Прямо сейчас вы сможете изучить хиты продаж – те товары, которые пользуются особой популярностью. Каталог товаров регулярно обновляется, чтобы вы приобрели лучшее.
Tiger Fortune’s free spins are pure gold. fortune tiger bet
http://onlinesloti.ru
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наша продукция:
Плоская мишень из иттрия, 3N, 4N
https://telegra.ph/Best-Non-Gamstop-Boku-Casino-Sites-Reviewed-2023-03-27
This casino’s Tiger Fortune game is incredible. jogo do tigrinho demo
Популярный сервис «Zoomy» предлагает воспользоваться такой популярной услугой, как аренда мотоциклов в Пхукете, Краби, Банг-Ламунг и других районах Таиланда. На сайте https://zoomy.travel уточните то, в каких районах Таиланда вы сможете взять мотоцикл в аренду.
https://telegra.ph/Explore-the-Best-Non-Gamstop-Casinos-of-2023-03-27
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
поставляемая продукция:
Труба бронзовая 80х15х2500 мм БрАЖН10-4-4 ГОСТ 1208-2014 Приобретайте трубы бронзовые круглые от профессионалов с опытом. Мы предлагаем широкий ассортимент качественной продукции, быструю обработку заказов и оперативную доставку. Обращайтесь, чтобы получить консультацию специалиста и выгодные условия покупки.
https://telegra.ph/No-Gamstop-Casinos-in-the-UK-Exploring-Alternatives-03-27
Смотри топ скинов CS2 https://cs2-open-case.ru/ самые красивые, редкие и желанные облики для оружия. Стиль, эффект и внимание на сервере гарантированы. Обновляем коллекцию каждый день!
Иммортал-дроп CS2 open case csgo только для избранных. Легендарные скины, высокая ценность, редкие флоаты и престиж на сервере. Пополни свою коллекцию настоящими шедеврами.
Специалисты компании «МосСтройАльянс» решат любую сложную задачу. Мы на осуществлении фасадных, уборочных и кровельных работ специализируемся. Предоставляем доступные цены. Вы будете довольны результатом. Ищете кровля цены за 1 кв? Mos-stroi-alians.ru – тут с отзывами наших клиентов можете ознакомиться. Для работы используем только самые качественные материалы. Ваше время мы ценим. За соблюдение сроков мы ответственность несем. Позвоните нам по телефону на портале. Мы вас проконсультируем и на все вопросы ответим.
Смотри топ скинов CS2 case-cs2-open самые красивые, редкие и желанные облики для оружия. Стиль, эффект и внимание на сервере гарантированы. Обновляем коллекцию каждый день!
Tiger Fortune’s jungle vibe is unbeatable. tiger fortune
Автоматические пороги считаются оптимальным решением в том случае, если выполнить монтаж стационарного аналога не получается либо не считается желательным. В данный момент можно изучить каталог всех вариантов, которые предлагает предприятие «SMARTПОРОГ», что позволит подобрать решение, которое определенно подойдет. https://smartporog.ru/ – на портале ознакомьтесь с достоинствами, на которые вы сможете рассчитывать при установке порогов внутри помещения. Такие конструкции потребуются с той целью, чтобы в помещение не попадали солнечные лучи, посторонние запахи. Это оптимальное решение, в том числе, и для ванной.
РедМетСплав предлагает обширный выбор качественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы обеспечиваем оперативное исполнение вашего заказа.
Каждая единица изделия подтверждена требуемыми документами, подтверждающими их происхождение. Дружелюбная помощь – наша визитная карточка – мы на связи, чтобы улаживать ваши вопросы по мере того как находить ответы под специфику вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
Наши товары:
Магний AZ63A – ASTM B275 Магний AZ63A – ASTM B275 является легким сплавом, обладающим отличными механическими свойствами Рё РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью. Ртот материал идеально РїРѕРґС…РѕРґРёС‚ для применения РІ аэрокосмической промышленности Рё автомобилестроении. Сплав обеспечивает высокую прочность РїСЂРё РЅРёР·РєРѕРј весе, что делает его идеальным выбором для изготовления различных конструкций. Если РІС‹ ищете надежное решение для СЃРІРѕРёС… проектов, рекомендуем купить Магний AZ63A – ASTM B275. РћРЅ обеспечит вам долговечность Рё высокие эксплуатационные характеристики. Рспользуйте этот сплав для создания легких Рё прочных изделий.
Tiger Fortune’s theme is a total vibe. tigrinho demo
I love the poker mix online—fresh go! jackpotraider
https://telegra.ph/Reliable-Non-Gamstop-Casinos-for-Safe-Online-Gaming-03-27
Great article! I really enjoyed reading your insights. Keep up the awesome work!
On the site https://wallpapers4screen.com/ you can download wallpapers for desktop free. High Quality HD pictures wallpapers. High quality pictures and wallpapers! Choose one of many categories and download for free! Or check out the TOP downloadable images! You will definitely like them.
https://telegra.ph/Boku-Casino-Guide-Without-GamStop-Restrictions-03-27
какие провайдеры на адресе в москве
domashij-internet-msk002.ru
провайдеры по адресу дома
казино dragon money [url=dragon-money27.com]казино dragon money[/url] .
Заказать диплом университета по доступной стоимости можно, обратившись к проверенной специализированной компании. Приобрести документ ВУЗа вы сможете у нас. [url=http://diplomh-40.ru/diplom-s-zaneseniem-v-reestr-dlya-vashego-uspexa-7/]diplomh-40.ru/diplom-s-zaneseniem-v-reestr-dlya-vashego-uspexa-7[/url]
https://telegra.ph/Guide-to-Non-GamStop-Online-Casino-Lightning-Roulette-03-27
Tiger Fortune is my favorite way to unwind. jogo do tigrinho demo
I won a tourney online—wild high! plinko
I love the jungle energy of Tiger Fortune. jogo do tigrinho demo
mostbet kg скачать на андроид [url=mostbet6031.ru]mostbet kg скачать на андроид[/url] .
https://telegra.ph/Non-Gamstop-Casinos-Free-Spins-Offers-and-Benefits2-03-27
Сайт hm.mnemosyne.ru предлагает выбрать ремень в подарок любимым людям. Мастера, которые изделия создают, творческим потенциалом отличаются. Каждая модель с учетом модных трендов разрабатывается. Мы прислушиваемся к покупателям. При возможностях всегда идем на встречу. https://hm.mnemosyne.ru – здесь узнаете, какой преподнести ремень в подарок коллеге, шефу или руководителю. Для изделий отбирается лучшая кожа. Оцените неповторимость ручной работы. Сделайте уже сейчас заказ на нашем портале. Пусть ваш стиль новыми красками засияет!
can you buy cheap colospa pills
https://telegra.ph/Are-Non-Gamstop-Casinos-Safe-for-Players-Today2-03-27
The sound design in Tiger Fortune is amazing. fortune tiger demo gratis
how to buy generic caduet tablets
Быстрый и удобный калькулятор строительства дома онлайн рассчитать рассчитайте стоимость и объем работ за пару минут. Онлайн-калькулятор поможет спланировать бюджет, сравнить варианты и избежать лишних затрат.
Мамоновское кладбище https://mamonovskoe.ru справочная информация, адрес, график работы, участки, ритуальные услуги и памятники. Всё, что нужно знать, собрано на одном сайте.
https://telegra.ph/Explore-New-Non-Gamstop-Casinos-for-2023-03-27
новости фк краснодар на сегодня новости часа краснодар
Не знаете, что подарить на день рождения, юбилей или праздник? Сайт http://mylnye-grezi.ru/chto-podarit-na-den-rozhdeniya-zheny-interesnye-idei/ предлагает готовые решения — от практичных до креативных подарков. Подберите идеальный вариант за пару кликов!
https://telegra.ph/No-Deposit-Casinos-in-the-UK-Not-on-GamStop1-03-27
Tiger Fortune makes every session feel epic. tiger fortune
мостбет скачать бесплатно [url=mostbet6033.ru]mostbet6033.ru[/url] .
Купить диплом о высшем образовании !
Покупка диплома ВУЗа РФ у нас является надежным процессом, так как документ заносится в государственный реестр. Быстро и просто купить диплом о высшем образовании [url=http://diplomc-v-ufe.ru/kupite-diplom-s-zaneseniem-v-reestr-po-dostupnoj-tsene-5/]diplomc-v-ufe.ru/kupite-diplom-s-zaneseniem-v-reestr-po-dostupnoj-tsene-5[/url]
https://telegra.ph/Pay-Mobile-Foreign-Casinos-with-Non-Gamstop-Options-03-27
Компания «Ковка Винтаж» в течение длительного времени оказывает свои услуги и предлагает создать изысканные, завораживающие кованые изделия, которые украсят придомовую территорию, беседку, террасу, а также частный дом. На сайте https://kovka-vintazh.ru/ ознакомьтесь с полным ассортиментом изделий и работами компетентных и выдающихся мастеров.
pin up az?rbaycan [url=www.pinup-azerbaycan1.com]pin up az?rbaycan[/url] .
https://telegra.ph/Best-Non-GamStop-Online-Casinos-for-Players-in-2023-03-27
На сайте https://vezuviy.shop/ вы сможете приобрести чугунные, стальные банные печи, нержавеющие, а также в облицовке, печи-камины, отопительные, каминные топки, дымоходы, порталы и многое другое. Вся продукция от производителя, а потому наценки очень маленькие. Такая техника считается идеальной не только с целью обогрева помещения, но и оформления пространства. А самое главное, что она отличается долгим сроком службы, надежностью из-за того, что в работе использовались высокотехнологичные, проверенные материалы.
драгон мани официальный сайт [url=https://www.dragon-money27.com]драгон мани официальный сайт[/url] .
На сайте https://mvpol.ru/ воспользуйтесь возможностью заказать промышленные полы напрямую от производителя. Компания «МВПОЛ» оказывает и такую важную услугу, как ремонт промышленных полов, производство полимерных наливных полов, бетонных, у которых упрочненный верх. Также получится узнать расценки на такие услуги, чтобы заранее спланировать бюджет. Для того чтобы иметь представление о том, как выглядят работы, изучите объекты. На все услуги предоставляются гарантии. Вся информация дается максимально быстро.
нанонаушник микронаушник аренда
Tiger Fortune’s gameplay is fast and fierce. fortune tiger 777
https://telegra.ph/Exploring-the-Benefits-of-Non-Gamstop-Casinos-03-27
Tiger Fortune is the best game here, hands down! fortune tiger 777
провайдеры по адресу москва
domashij-internet-msk003.ru
проверить интернет по адресу
I lost big online—time to rethink my strategy! fortune tiger
Tiger Fortune’s design is bold and exciting. jogo do tigrinho demo
микронаушник Брно http://jasdam.cz
драгон мани официальный сайт регистрация [url=https://dragon-money11.com/]драгон мани официальный сайт регистрация[/url] .
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наши товары:
Плоская мишень CoFe для распыления сплавов
https://telegra.ph/Non-Gamstop-Casinos-Bonus-Offers-and-Benefits-Explained-03-27
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Обладая обширной линейкой редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наша продукция:
Гранулы силикона 4N
can i get macrobid online
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наша продукция:
Оптимальное название товара: Гранулы железа 4N
Alkomarket-dubai.ru предлагает коллекцию алкогольных напитков на разный вкус и кошелек. Качество продукции – наш главный приоритет, мы заботимся о здоровье клиентов. Закажите на дом уже сейчас алкоголь с доставкой. Наслаждайтесь комфортом! Ищете drinks delivery dubai? Alkomarket-dubai.ru – здесь представлен широкий ассортимент пива, вина, водки, виски и скотча, а также других премиальных напитков от известных мировых брендов. К покупателям относимся бережно, об их потребностях заботясь. Поможем для вас и на подарок близким выбрать достойные варианты.
https://telegra.ph/Top-Uk-Casinos-Not-on-Gamstop-for-Endless-Fun-03-27
I can play Tiger Fortune all day and not get bored. tiger fortune
Индивидуальный подход к каждому элементу интерьера.
Мебель премиум [url=https://byfurniture.by/]Мебель премиум[/url] .
https://telegra.ph/Discover-No-Gamstop-Casinos-for-Unlimited-Play-03-27
The visuals in Tiger Fortune are breathtaking. jogo do tigrinho demo
Falschgeld kaufen Verkauf Euro https://maps.google.sh/url?q=https://falschgeldkaufen.org
The slot thrill online flies—pulse high! aviator
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с прекрасным качеством обслуживания.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – наилучшее решение для вашего бизнеса.
поставляемая продукция:
Лигатура алюминий-хром AlCr80 ГОСТ Р53777-2010 Получите высококачественную лигатуру алюминиевую для прочных и легких конструкций. У нас вы найдете широкий ассортимент продукции с гарантированной надежностью и высокими техническими характеристиками. Закажите сейчас и обеспечьте вашему производству надежные материалы высокого качества.
Tiger Fortune has the best vibe of any slot. tigrinho demo
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
Наши товары:
Меднаядвухраструбнаямуфта под пайку40х2ммМ3рГОСТ Р52922-2008 Выберите медные двухраструбные муфты под пайку от производителя RedmetSplav. Прочные и герметичные соединения для систем водоснабжения, отопления и газоснабжения. Долговечные и надежные муфты для любых видов работ. Подходят для использования в домашних условиях, коммерческих и промышленных объектах.
Истринская мануфактура межкомнатные двери на заказ производит. При безупречном качестве мебель компании доступна по привлекательным ценам. Мы к каждому заказу подходим творчески. Делаем красивую продукцию и ею гордимся. http://istradoors.ru – здесь представлены отзывы о нашей компании. Кроме этого на портале часто задаваемые вопросы и ответы на них перечислены. С нами ведущие дизайнерские и архитектурные мастерские работают. Мы готовы любую задачу осуществить. Детальную информацию о продукции и об условиях заказа уточняйте у менеджеров.
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
поставляемая продукция:
Диск РёР· драгоценных металлов палладиевый 200С…0.1 РјРј РџРґР82-18 РўРЈ РЁРёСЂРѕРєРёР№ выбор РґРёСЃРєРѕРІ РёР· драгоценных металлов для вашего автомобиля. Рксклюзивный дизайн, высокая устойчивость Рє РєРѕСЂСЂРѕР·РёРё, улучшенная управляемость Рё неповторимый стиль. Подчеркните индивидуальность вашего автомобиля СЃ нашими уникальными дисками.
Tiger Fortune keeps the adrenaline pumping every spin. tiger fortune
i want to become immortal https://intouchmag.com/
https://telegra.ph/Non-Gamstop-Live-Casinos-A-Comprehensive-Guide-03-27
РедМетСплав предлагает обширный выбор высококачественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица изделия подтверждена требуемыми документами, подтверждающими их соответствие стандартам. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы разрешать ваши вопросы а также предоставлять решения под требования вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в множестве наших преимуществ
поставляемая продукция:
Проволока магниевая РњРі-90 Полоса магниевая РњРі-90 – это универсальный материал, который находит широкое применение РІ различных отраслях. РћРЅР° отличается высокой прочностью, стойкостью Рє РєРѕСЂСЂРѕР·РёРё Рё легкостью РІ обработке. Рти свойства делают полосу идеальным выбором для производства различных изделий Рё конструкций. Приобретая этот РїСЂРѕРґСѓРєС‚, РІС‹ получаете надежный Рё долговечный материал, который обеспечит отличные результаты. Если РІС‹ ищете высококачественное решение, рекомендуем вам купить Полоса магниевая РњРі-90. РќРµ упустите возможность использовать этот легкий Рё прочный металл РІ СЃРІРѕРёС… проектах!
РедМетСплав предлагает широкий ассортимент отборных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица продукции подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Дружелюбная помощь – то, чем мы гордимся – мы на связи, чтобы ответить на ваши вопросы и находить ответы под специфику вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
Наши товары:
Полоса магниевая РњРі98 – ГОСТ 804-93 Порошок магниевый РњРі95РЎ – РўРЈ 1714-016-00545484-97 представляет СЃРѕР±РѕР№ высококачественный магниевый порошок, который используется РІ различных отраслях. РћРЅ обладает отличными физико-химическими свойствами, что делает его идеальным для применения РІ металлургии, производстве сплавов, Р° также РІ химической промышленности. Покупая данный РїСЂРѕРґСѓРєС‚, РІС‹ обеспечиваете надежность Рё долговечность СЃРІРѕРёС… изделий. РќРµ упустите возможность купить Порошок магниевый РњРі95РЎ – РўРЈ 1714-016-00545484-97 Рё оценить его преимущества. Наша продукция соответствует всем международным стандартам качества, что гарантирует удовлетворение ваших потребностей.
РедМетСплав предлагает широкий ассортимент высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица продукции подтверждена всеми необходимыми документами, подтверждающими их происхождение. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы разрешать ваши вопросы и предоставлять решения под требования вашего бизнеса.
Доверьте вашу потребность в редких металлах специалистам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
Лист вольфрамовый РР’Р§ Лист вольфрамовый РР’Р§ представляет СЃРѕР±РѕР№ высококачественный материал, используемый РІ различных областях, включая электронику Рё машиностроение. Ртот вольфрамовый лист обладает отличной устойчивостью Рє высоким температурам Рё химическим воздействиям. Обладая уникальными механическими свойствами, РѕРЅ идеален для применения РІ условиях повышенного давления. Лист вольфрамовый РР’Р§ также обеспечивает надежность Рё долговечность изделий. Если РІС‹ хотите обеспечить СЃРІРѕСЋ продукцию высоким качеством, вам стоит купить Лист вольфрамовый РР’Р§. РћРЅ идеально РїРѕРґС…РѕРґРёС‚ для технических процессов Рё является отличным выбором для профессионалов.
https://telegra.ph/Best-Non-Gamstop-Casinos-for-UK-Players-in-2023-03-27
how to buy cheap prothiaden pill
how to get depakote online
pin up azerbaijan [url=pinup-azerbaycan1.com]pin up azerbaijan[/url] .
https://telegra.ph/Exploring-Casinos-Not-on-Gamstop-for-Online-Players-03-27
O ggbet oferece uma excelente oportunidade para quem deseja começar sua experiência
no cassino online com um bônus de 100$ para
novos jogadores! Ao se registrar no site, você garante esse bônus
exclusivo que pode ser utilizado em diversos jogos de cassino, como slots, roleta e poker.
Esse é o momento perfeito para explorar o mundo das apostas
com um saldo extra, aproveitando ao máximo suas apostas sem precisar investir um grande valor
logo de início. Não perca essa oportunidade e cadastre-se já!
На сайте https://ooomila.com изучите каталог такого оборудования, которое предназначено для организации животноводческих комплексов. Также вы сможете почитать и содержательную информацию о компании и ее достижениях. В каталоге вы найдете следующее оборудование: технологические линии, весовое оборудование, резиновые маты, крематоры и многое другое. На технику, которая находится в разделе, действуют гарантии. Несколько сотен единиц оборудования всегда находятся на складе, можно приобрести под заказ.
дешевый интернет нижний новгород
domashij-internet-nizhnij-novgorod001.ru
провайдеры интернета в нижнем новгороде по адресу
Tiger Fortune’s payouts are worth the hype. fortune tiger bet
Great post! I really enjoyed reading your insights. Thanks for sharing such valuable information—looking forward to your next update!
Популярная компания «Herbs & Flowers» предлагает огромный выбор искусственных цветов, которые идеально подходят как для украшения интерьера дома, так и офиса. В каталоге сайта https://herbsandflowers.ru/ вы найдете вертикальное озеленение, ампульные растения, а также деревья, ветви и многое другое.
I won $1400 on a slot—hype high! betonred
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наши товары:
Распылительная мишень IGZO 4N – Плоская
https://telegra.ph/Reviews-of-Non-GamStop-Casinos-for-Players1-03-27
https://telegra.ph/Casino-Online-Options-Beyond-GamStop-Restrictions-03-27
https://telegra.ph/Guide-to-the-Best-Non-Gamstop-Casinos-for-Players1-03-27
order generic zocor pills
get hydrea no prescription
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – идеальный вариант для вас.
Наши товары:
Труба из драгоценных металлов серебряная 400х8х1 мм Ср99.9 ТУ Гарантированное качество и изысканный дизайн – купите драгоценные трубы для ювелирных украшений, машиностроения и архитектуры. Широкий выбор и высокое качество! Доставка по России.
https://telegra.ph/Non-Gamstop-Casinos-Review-Pros-and-Cons-2023-03-27
Tiger Fortune’s features are a total win. fortune tiger demo gratis
Компания «V8soft» предлагает облачный сервис для того, чтобы вы смогли вести бизнес удачно и максимально эффективно. На предприятии работают исключительно те профессионалы, у которых имеется весь необходимый опыт работы, полезные навыки. На сайте https://v8soft.ru/ изучите все возможности ПО, чтобы определить то, подходит ли вам решение.
Tiger Fortune’s free spins are a game-changer. tiger fortune
Ищете безопасный VPN? Попробуйте https://amneziavpn.org — open-source решение для анонимности и свободы в интернете. Полный контроль над соединением и личными данными.
Обеспечь анонимность с амнезия впн. Защита трафика, собственный сервер, простая установка и отсутствие слежки. Отличный выбор для тех, кто ценит свободу в интернете.
https://telegra.ph/Best-Casinos-Not-on-Gamstop-with-No-Deposit-Bonuses4-03-27
nanosluchatka mikrosluchatko-cena.cz
The music in Tiger Fortune sets the perfect mood. fortune tiger demo gratis
pin up azerbaycan [url=https://www.pinup-azerbaycan1.com]pin up azerbaycan[/url] .
На сайте https://pilmi.ru вы найдете огромное количество фильмов самых разных жанров, включая комедии, мелодрамы, драмы, романтические. Для того чтобы подыскать наиболее подходящий вариант, нужно воспользоваться специальным каталогом. Вашему вниманию представлены и ожидаемые любопытные новинки этого года, которые произведут эффект. Все это можно просматривать в любое время и на различных устройствах. Прямо сейчас воспользуйтесь возможностью посмотреть азиатское кино. Используйте поиск для облегчения выбора.
РедМетСплав предлагает обширный выбор качественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица изделия подтверждена всеми необходимыми документами, подтверждающими их происхождение. Опытная поддержка – то, чем мы гордимся – мы на связи, чтобы улаживать ваши вопросы а также адаптировать решения под особенности вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
Наша продукция:
РљСЂСѓРі магниевый 9980B – ASTM B275 Фольга магниевая 9980B – ASTM B275 представляет СЃРѕР±РѕР№ высококачественный материал, используемый РІ различных отраслях. Рта фольга отличается отличной прочностью Рё легкостью, что делает ее идеальным выбором для производственных процессов Рё инновационных приложений. Купить Фольга магниевая 9980B – ASTM B275 стоит тем, кто ценит эффективность Рё надежность. Обладая уникальными свойствами, РѕРЅР° обеспечивает высокий уровень защиты РѕС‚ РєРѕСЂСЂРѕР·РёРё Рё механических повреждений. РќРµ упустите возможность добавить этот материал РІ СЃРІРѕР№ бизнес, оптимизируя производство. Обеспечьте себе конкурентное преимущество СЃ фольгой магниевой 9980B – ASTM B275!
I’m obsessed with Tiger Fortune – so much fun! fortune tiger 777
Drinks delivery предоставляет большой выбор алкогольных напитков и доступные цены. Наш основной приоритет – это качество. О своей репутации мы заботимся. Продаем исключительно то, в чем уверены мы. С нами вы необходимый товар легко подберете. Ищете delivery drinks dubai? Deliverydubaidrinks.com – здесь есть абсент, ликер, вино, коньяк, ром, водка, джин, виски, бризер, текила, шампанское и пиво. Выполняем оперативную доставку алкоголя. Свое дело мы любим и к пожеланиям покупателей всегда прислушиваемся. Ответим на любой вопрос и поможем подобрать подходящие для вас напитки.
подключить домашний интернет нижний новгород
domashij-internet-nizhnij-novgorod002.ru
провайдеры домашнего интернета нижний новгород
https://telegra.ph/Explore-Non-Gamstop-Casinos-for-Unrestricted-Gaming-03-27
Приобрести диплом института!
Мы готовы предложить дипломы любой профессии по приятным ценам— [url=http://diplom-club.com/kupit-diplom-visshem-obrazovanii-zaneseniem-reestr-6/]diplom-club.com/kupit-diplom-visshem-obrazovanii-zaneseniem-reestr-6/[/url]
https://telegra.ph/No-Deposit-Casino-Bonuses-Not-on-GamStop2-03-27
На сайте https://rustreetwear.ru вы найдете огромное количество интересных, разнообразных и эксклюзивных брендов, которые создают роскошную уличную одежду на любые случаи жизни. Есть как совсем не известные марки, которые только начинают свой путь, так и гиганты мировой индустрии. Перед вами самый впечатляющий каталог, где вы найдете не только одежду, но и аксессуары. Каталог обновляется ежедневно, чтобы вы обязательно нашли для себя лучший вариант. Здесь установлены доступные расценки.
Tiger Fortune’s bonus rounds are thrilling. fortune tiger 777
https://telegra.ph/PayPal-Casinos-Not-On-Gamstop-Guide-03-27
The variety of games in online casinos is insane—something for everyone! plinko
Tiger Fortune’s jungle theme is so well done. tigrinho demo
how to get reglan pill
I love the jungle energy of Tiger Fortune. jogo do tigrinho demo
can you buy cheap allopurinol without dr prescription
https://telegra.ph/Best-Non-Gamstop-Casinos-UK-Pay-by-Phone-Options-03-27
Где купить диплом по нужной специальности?
Наша компания предлагаетвыгодно заказать диплом, который выполняется на оригинальной бумаге и заверен мокрыми печатями, водяными знаками, подписями должностных лиц. Данный диплом пройдет лубую проверку, даже с использованием специальных приборов. Решайте свои задачи быстро с нашими дипломами. Заказать диплом любого университета! [url=http://booknightfun.copiny.com/question/details/id/1068368/]booknightfun.copiny.com/question/details/id/1068368[/url]
https://telegra.ph/Guide-to-Non-Gamstop-Casinos-and-Their-Benefits1-03-27
I’ve had some huge wins on Tiger Fortune! fortune tiger demo
spionazni kamera mini kamera
Loved this post! You explained everything in such an easy-to-understand way.
гель для стирки Аберлибрикс
Сантехник Юго-Восточный https://santekhnik-moskva.blogspot.com/p/south-eastern-administrative-okrug-of.html административный округ Москвы (ЮВАО). В состав Юго-Восточного административного округа входят районы: Выхино-Жулебино, Капотня, Кузьминки, Лефортово, Люблино, Марьино, Некрасовка, Нижегородский район, Печатники, Рязанский район, Текстильщики, Южнопортовый район.
Компания «DELIVERY PLAY» предлагает выгодный прокат инвалидных колясок с быстрой доставкой и по привлекательным ценам. Каждый клиент получает возможность воспользоваться оперативной доставкой не только по Москве, но и области. На сайте https://deliveryplay.ru/arenda-invalidnyh-kolyasok изучите все модели, которые вы сможете заказать здесь.
Креативные, необычные и классические подарки ждут вас на https://miei.ru/analizatory/chto-podarit-na-den-rozhdeniya-devochke-14-let/ Сделайте каждый праздник особенным!
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наши товары:
Мишень РёР· высокочистого бисмута (Bi) – плоская форма
https://telegra.ph/Non-Gamstop-Casinos-Featuring-Free-Spins-Offers-03-27
Чеки для отчётности https://t.me/kupitchekiru в Москве — быстро, конфиденциально и с гарантией. Подтверждающие документы для отчёта, бухгалтерии, авансовых отчётов. Оперативная подготовка и доставка.
https://telegra.ph/Top-Non-Gamstop-Casinos-for-Unrestricted-Gaming-03-27
Где заказать диплом специалиста?
Мы предлагаем быстро и выгодно приобрести диплом, который выполняется на оригинальном бланке и заверен печатями, водяными знаками, подписями официальных лиц. Данный документ пройдет любые проверки, даже при использовании специально предназначенного оборудования. Достигайте цели быстро и просто с нашей компанией.
Приобрести диплом любого университета [url=http://kupitediplom.ru/kupit-svidetelstvo-o-brake-7/]kupitediplom.ru/kupit-svidetelstvo-o-brake-7/[/url]
Tiger Fortune’s bonus rounds are thrilling. tigrinho demo
провайдеры интернета в нижнем новгороде по адресу
domashij-internet-nizhnij-novgorod003.ru
подключить интернет по адресу
https://telegra.ph/Explore-New-Casinos-Not-on-Gamstop-for-Exciting-Play-03-27
I hit a blackjack streak—good day! plinko
Registre-se no bet favorita – https://betfavorita-br.com e Comece com
100$ de Bônus para Apostar
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Обладая обширной линейкой редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Медный цилиндр 5N
can i order fml forte tablets
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наши товары:
Кольцо РёР· драгоценных металлов платиновое 45С…5С…1.5 РјРј РџР»Р95-5 РўРЈ РЁРёСЂРѕРєРёР№ выбор высококачественных кольц РёР· драгоценных металлов. Рлегантные украшения, созданные талантливыми мастерами. Уникальность Рё изысканность РІ каждой детали. Возможность заказа персонального кольца РїРѕ вашим пожеланиям. Подчеркните СЃРІРѕСЋ индивидуальность СЃ нашими кольцами.
On the site https://wallpapers4screen.com/ you can download wallpapers for desktop free. High Quality HD pictures wallpapers. High quality pictures and wallpapers! Choose one of many categories and download for free! Or check out the TOP downloadable images! You will definitely like them.
Академия «Арчибальд» занимается обучением грумингу. У вас есть возможность свои навыки совершенствовать до бесконечности на живых моделях. Преподаватели отвечают на все вопросы. Дарим видеолекции, методические материалы и учебники. Окунитесь в атмосферу, с которой расставаться не хочется. Ищете курсы груминга в москве лучшие? Grooming-dream.ru – здесь представлены реальные отзывы клиентов. Мы выпускаем отменных груминг-специалистов. Предлагаем инструменты в период обучения. Если вам интересна новая профессия – Арчибальд, вот то место, которое вам подойдет.
how to buy generic atarax tablets
https://telegra.ph/No-Deposit-Bonuses-at-Casinos-Not-on-GamStop2-03-27
Super useful! I’ll definitely be applying some of these ideas.
Tiger Fortune’s spins are always a rush. fortune tiger demo gratis
https://telegra.ph/Non-Gamstop-UK-Casinos-Accepting-PayPal-Payments-03-27
РедМетСплав предлагает широкий ассортимент высококачественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица изделия подтверждена соответствующими документами, подтверждающими их происхождение. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы улаживать ваши вопросы и адаптировать решения под требования вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
РџРѕРєРѕРІРєР° висмутовая 2130 – COPANT 862 РџРѕРєРѕРІРєР° висмутовая 2130 – COPANT 862 представляет СЃРѕР±РѕР№ высококачественный материал, обладающий уникальными свойствами. РћРЅ идеально РїРѕРґС…РѕРґРёС‚ для использования РІ электронике, медицинских приборах Рё РґСЂСѓРіРёС… сферах, РіРґРµ требуется высокая степень термостойкости Рё устойчивости Рє РєРѕСЂСЂРѕР·РёРё. РџРѕРєРѕРІРєР° висмутовая 2130 – COPANT 862 отличается отличной обрабатываемостью Рё легкостью. Если РІС‹ ищете надежный материал, то вам стоит купить РџРѕРєРѕРІРєР° висмутовая 2130 – COPANT 862. Рто решение, которое обеспечит долговечность Рё эффективность вашей продукции. РќРµ упустите возможность приобрести этот инновационный РїСЂРѕРґСѓРєС‚!
The mobile casino dips—lift it! jackpotraider
https://telegra.ph/Best-Non-Gamstop-Casinos-for-Secure-Online-Gambling-03-27
Registre-se e Receba 100$ de Bônus no pixbet para Apostar!
For years, Pin Up has delivered thrilling gaming and betting experiences through its premier online casino platform. Whether you’re placing a bet on sports or enjoying games via the Pin-Up app, pinup caters to all players with secure, fast-paced entertainment pinup updo
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
поставляемая продукция:
Кольцо РёР· драгоценных металлов золотое 50С…10С…4 РјРј ЗлСрМ58.5-20 РўРЈ РЁРёСЂРѕРєРёР№ выбор высококачественных кольц РёР· драгоценных металлов. Рлегантные украшения, созданные талантливыми мастерами. Уникальность Рё изысканность РІ каждой детали. Возможность заказа персонального кольца РїРѕ вашим пожеланиям. Подчеркните СЃРІРѕСЋ индивидуальность СЃ нашими кольцами.
This casino’s Tiger Fortune slot is legendary. tigrinho demo
https://telegra.ph/Exploring-Non-Gamstop-Casinos-for-Safe-Betting-Options-03-27
[url=https://blogcamp.com.ua/ru/2025/04/igry-dlja-devochek-volshebnyj-mir-fantazii-i-veselja/]https://blogcamp.com.ua/ru/2025/04/igry-dlja-devochek-volshebnyj-mir-fantazii-i-veselja/[/url] игра в переливание жидкости в колбах разного цвета
Really informative read—thanks for breaking it down so clearly!
Delivery-dubai.com предлагает широкий ассортимент разных видов алкоголя. На сайте можно ознакомиться с имеющейся продукцией в каталоге с ценами. Это даст возможность под ваш повод напиток выбрать подходящий. Осуществляем оперативную доставку алкоголя. Будем рады видеть вас в числе клиентов! Ищете купить алкоголь в дубае c доставкой? Delivery-dubai.com – здесь представлен огромный выбор алкоголя. Вы отыщите для себя все то, что принести удовольствие может. Товары лучшего качества предлагаем. Для заказа напишите нам в мессенджеры: WhatsApp, Telegram.
I’ve had some epic moments on Tiger Fortune. fortune tiger 777
Medicine information. Long-Term Effects.
cheap prednisolone prices
Best news about drug. Read information here.
https://telegra.ph/No-Gamstop-UK-Casino-Options-for-Players1-03-27
РедМетСплав предлагает широкий ассортимент высококачественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица продукции подтверждена соответствующими документами, подтверждающими их соответствие стандартам. Превосходное обслуживание – наш стандарт – мы на связи, чтобы ответить на ваши вопросы по мере того как находить ответы под требования вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
Полоса вольфрамовая WT20 Полоса вольфрамовая WT20 – это надежный материал, обладающий высокой прочностью Рё устойчивостью Рє высоким температурам. РћРЅР° идеально РїРѕРґС…РѕРґРёС‚ для производства электродов, используемых РІ сварочных работах. Благодаря СЃРІРѕРёРј свойствам, Полоса вольфрамовая WT20 обеспечивает стабильный Рё качественный процесс сварки. Ртот РїСЂРѕРґСѓРєС‚ выделяется среди аналогов своей долговечностью Рё отличной электропроводностью. Если РІС‹ ищете надежный Рё эффективный материал, купить Полоса вольфрамовая WT20 – правильное решение. Рнвестируйте РІ качество Рё безопасность ваших процессов.
Купить диплом об образовании. Покупка диплома через проверенную и надежную фирму дарит ряд преимуществ. Такое решение помогает сэкономить как продолжительное время, так и существенные финансовые средства. [url=http://branditstrategies.com/employer/eonline-diploma/]branditstrategies.com/employer/eonline-diploma[/url]
The payout delays at online casinos are so frustrating! fortune mouse
https://telegra.ph/Top-Non-Gamstop-Casinos-for-Online-Betting-in-2023-03-27
Mycelium has earned a reputation as a robust and protected handheld Bitcoin wallet, serving to both novice and expert users bitcoin wallet apk
провайдеры интернета по адресу
domashij-internet-novosibirsk001.ru
проверить провайдера по адресу
cost of cheap fulvicin pills
Премиум скины CS2 cs2-case-simulator.ru/ топовые облики для AWP, AK-47, M4, ножей и перчаток. Яркий стиль, редкие коллекции, эксклюзивные дизайны. Укрась свою игру и выделяйся в каждой катке!
can i buy cheap omnicefs tablets
pin up azerbaycan [url=https://pinup-azerbaycan2.com/]pin up azerbaycan[/url] .
Хочешь Dragon Lore cs case simulator Открывай кейс прямо сейчас — шанс на легенду CS2 может стать реальностью. Лучшие скины ждут тебя, рискни и получи топовый дроп!
mostbets [url=https://mostbet6011.ru]https://mostbet6011.ru[/url] .
https://telegra.ph/Casinos-Not-on-Gamstop-with-No-Deposit-Bonuses-03-27
Glock-18 Fade case-simulator-csgo один из самых ярких и редких скинов для стартового пистолета в CS2. Плавный градиент, премиум-качество и высокий спрос среди коллекционеров.
This online casino shines with Tiger Fortune. fortune tiger 777
Приобрести диплом любого института!
Мы предлагаем дипломы любой профессии по выгодным ценам— [url=http://diplomh-40.ru/gde-kupit-diplom-o-visshem-obrazovanii-bistro-i-nadezhno/]diplomh-40.ru/gde-kupit-diplom-o-visshem-obrazovanii-bistro-i-nadezhno/[/url]
https://telegra.ph/Legit-Non-Gamstop-Casinos-for-Safe-Online-Gaming-03-27
Tiger Fortune’s wild symbols are my favorite! fortune tiger demo gratis
Aposte com 100$ de Bônus no blackjack online – Cadastre-se
e Aproveite!
Online gambling can spiral fast—gotta stay disciplined! plinko
https://telegra.ph/Non-Gamstop-Casinos-No-Deposit-Bonuses-Guide-03-27
купить школьный аттестат вечерней школы
На сайте https://officepro54.ru представлено огромное количество мебели, которая идеально подходит для организации офисного пространства. Вся она выполнена из инновационных, уникальных материалов, а потому прослужит очень долго, не синтезирует в воздух опасных веществ. В разделе вы найдете кабинет руководителя, функциональные и вместительные столы для переговоров, шкафы-купе, сейфы, акустические системы, ученическую мебель и многое другое. Постоянно действуют акции. На продукцию установлены привлекательные цены.
Medicament information leaflet. Short-Term Effects.
where buy allegra without rx
Best trends of pills. Read now.
https://telegra.ph/Best-No-Deposit-Casinos-Not-on-GamStop-Reviewed-03-27
Где заказать диплом специалиста?
Мы предлагаембыстро приобрести диплом, который выполняется на бланке ГОЗНАКа и заверен мокрыми печатями, водяными знаками, подписями. Документ способен пройти любые проверки, даже при помощи специального оборудования. Решите свои задачи быстро и просто с нашей компанией. Заказать диплом о высшем образовании! [url=http://zanasite.free.fr/laripostedumardi/doku.php/]zanasite.free.fr/laripostedumardi/doku.php[/url]
I can’t stop playing Tiger Fortune – it’s addictive! fortune tiger
https://telegra.ph/Explore-Non-GamStop-Casinos-Available-in-the-UK-03-27
StatTrak AK-47 Vulcan get-skin-cs2.ru/ мощный стиль и счётчик убийств в одном скине. Агрессивный дизайн, сине-чёрная цветовая гамма и высокая ценность на рынке CS2. Идеальный выбор для бойца с характером!
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наши товары:
Двухраструбный медный обвод под пайку 80х68х1.8 мм 13х16 мм твердая пайка М0б ГОСТ Р52922-2008 Выберите качественные Медные двухраструбные обводы под пайку различных размеров и диаметров. Надежное соединение труб, высокая устойчивость к коррозии и превосходная теплопроводность. Узнайте больше о нашей продукции и найдите оптимальное решение для вашего проекта.
M4A4 Emperor get skin cs эффектный скин в стиле королевской власти. Яркий синий фон, золотые детали и образ императора делают этот скин настоящим украшением инвентаря в CS2.
https://telegra.ph/No-Deposit-Non-Gamstop-Casinos-for-Easy-Access-03-27
Tiger Fortune’s spins are wild and unpredictable. tigrinho demo
Мы изготавливаем дипломы любых профессий по разумным тарифам. Мы предлагаем документы ВУЗов, которые расположены в любом регионе РФ. Документы выпускаются на “правильной” бумаге высшего качества. Это позволяет делать настоящие дипломы, которые не отличить от оригинала. [url=http://iratechsolutions.com/employer/archive-diploma/]iratechsolutions.com/employer/archive-diploma[/url]
AK-47 Case Hardened http://get-skins-cs2.ru классика CS2 с уникальным закалённым узором. Каждый скин отличается: от редких фулл-блю до золотых комбинаций. Настоящая находка для коллекционера и трейдера.
Receba 100$ de Bônus no doce e Comece
a Apostar Agora!
Novo no betleao – https://betleao-login.com? Cadastre-se e
Receba 100$ de Bônus ao Fazer Login!
Здравствуйте! Мы, Шестаков Юрий Иванович, врач-косметолог с многолетним опытом работы, и Шестакова Татьяна Викторовна, косметолог, хотим поделиться с вами рекомендациями, которые помогут решить проблему акне.
Акне – это не просто воспаления на коже, а состояние, требующее внимания. За годы нашей практики мы помогли сотням пациентов восстановить чистоту кожи, используя новейшие технологии. Мы уверены, что любая кожа заслуживает ухода и заботы, и хотим рассказать, как это осуществить.
Прыщи – это распространённое заболевание кожи. Современные способы борьбы с воспалениями на коже позволяют добиться ухоженную кожу уже за минимальный срок.
Лечение акне: ключевые моменты и техники, с легкостью и результатом
угри акне фото [url=http://www.kpacota.top/]http://www.kpacota.top/[/url] .
Изготовление металлических значков методом химического травления позволяет создавать изделия с высокой детализацией и долговечностью https://proremont-yug.ru/proczess-proizvodstva-metallicheskih-znachkov-s-ispolzovaniem-himicheskogo-travleniya/
https://telegra.ph/Best-Non-GamStop-UK-Casinos-for-Players-in-2023-03-27
Блог о маркетплейсах «OOWA» предлагает ознакомиться с актуальной, интересной и познавательной информацией, которая касается удачных продаж на маркетплейсах, обзоров товаров и услуг. На портале регулярно публикуются ценные и содержательные рекомендации от экспертов, которые помогут начать лучше разбираться в тонкостях такого бизнеса. Ищете обзор смартфона poco f6? На oowa.ru регулярно публикуются ценные и содержательные рекомендации от экспертов, которые помогут начать лучше разбираться в тонкостях такого бизнеса. Почитайте о самых востребованных товарах с маркетплейсов.
подключить интернет
domashij-internet-novosibirsk002.ru
подключение интернета по адресу
Где заказать диплом по актуальной специальности?
Наши специалисты предлагают выгодно купить диплом, который выполняется на бланке ГОЗНАКа и заверен мокрыми печатями, водяными знаками, подписями официальных лиц. Документ пройдет лубую проверку, даже при использовании специального оборудования. Решите свои задачи быстро и просто с нашим сервисом.
Купить диплом ВУЗа [url=http://diplomv-v-ruki.ru/kupit-diplom-o-srednem-obrazovanii-19/]diplomv-v-ruki.ru/kupit-diplom-o-srednem-obrazovanii-19/[/url]
Если вы хотите быть в курсе самой актуальной, ценной и нужной информации относительно трейдинга и инвестиций, то скорее посетите этот полезный портал, на котором вы найдете все, что нужно на данную тему. Здесь доступным языком рассказывается о том, как правильно вести торговлю на внебиржевом рынке. http://fxidea.com/ рекомендует воспользоваться акциями каждому новичку. Быстрее изучите индикаторы, реальные отзывы, а также ознакомьтесь с работающими стратегиями. Вы сможете воспользоваться интересными материалами о криптовалюте, будете в курсе того, как можно получить деньги от форекса.
O winbra oferece uma excelente oportunidade para quem deseja começar sua experiência no cassino online
com um bônus de 100$ para novos jogadores! Ao se registrar no site, você garante esse
bônus exclusivo que pode ser utilizado em diversos jogos de cassino,
como slots, roleta e poker. Esse é o momento perfeito para explorar o mundo das apostas com um
saldo extra, aproveitando ao máximo suas apostas sem precisar investir um grande valor logo de início.
Não perca essa oportunidade e cadastre-se já!
pin up azerbaijan [url=www.pinup-azerbaycan2.com/]pin up azerbaijan[/url] .
This casino’s Tiger Fortune game is incredible. tiger fortune
Мы можем предложить дипломы любой профессии по доступным тарифам. Дипломы производятся на оригинальных бланках Купить диплом о высшем образовании [url=http://damdiplomisa.com/]damdiplomisa.com[/url]
Где купить диплом специалиста?
Заказать диплом ВУЗа по доступной цене возможно, обратившись к проверенной специализированной компании.: [url=http://diplom-city24.ru/]diplom-city24.ru[/url]
https://telegra.ph/Guide-to-Non-Gamstop-Boku-Casino-Sites-for-Players-03-27
cost glycomet no prescription
Meds prescribing information. Effects of Drug Abuse.
where buy generic paxil price
All information about medicines. Read information now.
mostbets [url=https://mostbet6011.ru]https://mostbet6011.ru[/url] .
buying cheap avodart pills
Tiger Fortune’s payouts are always a surprise. fortune tiger demo gratis
Займы онлайн без проверок займ онлайн без проверки ки
Как можно взять займ займ денег онлайн
Market-delivery-dubai.ru доставку алкоголя предлагает. Вы можете у нас приобрести абсцент, виски, коньяк, пиво, ликер, водку, бризер, вино, джин, текилу, ром. Мы гарантируем приемлемые цены. Заслужили доверие покупателей. Ищете алкоголь в дубае с доставкой? Market-delivery-dubai.ru – тут то есть, что вам необходимо. Постоянно над ассортиментом работаем. Прекрасно знаем потребности своих клиентов. Заказы через Whatsapp принимаем. Выполняем доставку различного вида алкогольной продукции отменного качества в сжатые сроки. Необходима помощь? Свяжитесь с нами.
Ao se cadastrar no betsury,
você ganha um bônus de 100$ para começar sua jornada no cassino com o pé direito!
Não importa se você é um novato ou um apostador experiente, o bônus de boas-vindas é a oportunidade perfeita para explorar todas as
opções que o site tem a oferecer. Jogue seus jogos favoritos,
descubra novas opções de apostas e aproveite para
testar estratégias sem risco, já que o bônus ajuda a aumentar suas chances de ganhar.
Cadastre-se hoje e comece com 100$!
Online gambling can spiral—stay in control! fortune mouse
https://telegra.ph/Non-GamStop-Casinos-Free-Spins-Without-Deposit-Required-03-27
No bwin, Você Começa com 100$ de Bônus ao se Registrar!
Medicine information leaflet. Effects of Drug Abuse.
can i purchase generic pioglitazone pills
Everything information about medicament. Read here.
https://telegra.ph/Best-Casino-Non-Gamstop-Options-in-the-UK-2023-03-27
pop over to this site https://perena.us/
Запишитесь на семинары для массажистов и прокачайте свою профессию. Современные методики, опытные преподаватели, доступная цена и максимальная польза для практики.
This online casino knows how to do Tiger Fortune right. tigrinho demo
pin up az?rbaycan [url=https://www.pinup-azerbaycan3.com]pin up az?rbaycan[/url] .
Aposte no greenbets
e Receba 100$ de Bônus ao Se Registrar!
see here https://universalx.us
https://telegra.ph/Explore-New-Non-Gamstop-Casinos-for-Exciting-Wins1-03-27
Your Domain Name https://magpiexyz.lat/
Playing Tiger Fortune feels like a wild adventure every time. fortune tiger 777
купить диплом отзывы
go to my blog https://universalx.us
For years, Pin-Up has delivered premium betting, casino thrills, and the pinup app for seamless online gaming worldwide pin up casino app download
мостбет скачать андроид [url=http://mostbet6012.ru]мостбет скачать андроид[/url] .
https://telegra.ph/No-Deposit-Bonuses-at-Non-Gamstop-Casinos-in-the-UK-03-27
Medicines prescribing information. Effects of Drug Abuse.
get levonorgestrel price
All trends of drug. Get information now.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
С широким ассортиментом продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние успехи.
Наша продукция:
Ультрачистые гранулы германия
I wish online guided new—rough start! plinko
This casino’s Tiger Fortune slot is addictive. fortune tiger
No mrbet (https://mrbetjack-br.com), ao Registrar-se,
Você Recebe 100$ de Bônus!
I don’t trust online RNG—feels off! plinko
where to get generic tetracycline prices
https://telegra.ph/Pay-by-Phone-Options-at-Non-Gamstop-Casinos-UK-03-27
how can i get cheap diflucan tablets
Comece no bet nacional com 100$ de Bônus de
Boas-Vindas!
Medication information leaflet. Cautions.
strattera without dr prescription
Everything what you want to know about medicament. Get information now.
Заказать диплом об образовании. Покупка диплома ВУЗа через надежную фирму дарит ряд достоинств для покупателя. Данное решение позволяет сберечь время и существенные финансовые средства. [url=http://bl.com.tw/index.php?title=Заказать_диплом_—_просто_и_надёжно/]bl.com.tw/index.php?title=Заказать_диплом_—_просто_и_надёжно[/url]
https://telegra.ph/Best-Non-Gamstop-Curacao-Casino-Sites-Reviewed-03-27
провайдеры по адресу
domashij-internet-novosibirsk003.ru
какие провайдеры интернета есть по адресу новосибирск
pin up azerbaycan [url=https://pinup-azerbaycan2.com]pin up azerbaycan[/url] .
https://telegra.ph/Exploring-Non-Gamstop-Casino-Sister-Sites-03-27
Купить диплом любого университета!
Мы изготавливаем дипломы психологов, юристов, экономистов и прочих профессий по разумным ценам— [url=http://diplomnie.com/kupit-attestati-za-11-klassov-v-moskve-bistro-i-udobno/]diplomnie.com/kupit-attestati-za-11-klassov-v-moskve-bistro-i-udobno/[/url]
https://telegra.ph/Non-Gamstop-Casino-No-Deposit-Bonus-Code-Guide-03-27
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
поставляемая продукция:
Кольцо РёР· драгоценных металлов платиновое 45С…2С…3 РјРј РџР»Р90-10 РўРЈ РЁРёСЂРѕРєРёР№ выбор высококачественных кольц РёР· драгоценных металлов. Рлегантные украшения, созданные талантливыми мастерами. Уникальность Рё изысканность РІ каждой детали. Возможность заказа персонального кольца РїРѕ вашим пожеланиям. Подчеркните СЃРІРѕСЋ индивидуальность СЃ нашими кольцами.
Tiger Fortune’s rewards make it worth playing. fortune tiger demo gratis
Необходим профессиональный электромонтажник? Мы производим любые [url=https://irkelektrik.ru/]работы по электрике в Иркутске[/url] в короткие сроки, качественно и с гарантией!
* Установка проводки и автоматических выключателей
* Установка розеток, выключателей, светильников
* Монтаж электрощитов
* Установка бытовой техники
Работаем 24/7 – приедем в день звонка! Профессиональные электрики, передовое оборудование , четкое соблюдение норм безопасности.
[url=https://irkelektrik.ru/]Заказать электрика в Иркутске[/url] прямо сейчас! Исправим любые неполадки с электричеством!
Pills prescribing information. Short-Term Effects.
can i get generic zithromax prices
All information about drug. Read information here.
https://telegra.ph/Top-Non-Gamstop-Casinos-in-the-UK-for-202311-03-27
Medicine information. Effects of Drug Abuse.
can i order requip without dr prescription
Actual trends of drugs. Get here.
РедМетСплав предлагает внушительный каталог отборных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы обеспечиваем быстрое выполнение вашего заказа.
Каждая единица товара подтверждена всеми необходимыми документами, подтверждающими их происхождение. Превосходное обслуживание – наш стандарт – мы на связи, чтобы улаживать ваши вопросы по мере того как предоставлять решения под требования вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в множестве наших преимуществ
Наши товары:
РўСЂСѓР±Р° висмутовая 42 3742 – CSN/STN 423742 РўСЂСѓР±Р° висмутовая 42 3742 – CSN/STN 423742 является высококачественным материалом, подходящим для различных промышленных Рё научных применений. РћРЅР° обладает отличными физико-химическими свойствами, что делает ее идеальным выбором для работы РІ сложных условиях. Купить РўСЂСѓР±Р° висмутовая 42 3742 – CSN/STN 423742 стоит тем, кто ценит надежность Рё долговечность. Данный РїСЂРѕРґСѓРєС‚ отвечает всем современным стандартам Рё может быть использован РІ медицине, химической промышленности Рё РґСЂСѓРіРёС… сферах. РќРµ упустите возможность улучшить СЃРІРѕРё проекты, выбрав эту трубу.
https://telegra.ph/Discover-New-Casino-Sites-Without-Gamstop-Restrictions-03-27
buy generic urispas without insurance
buy differin for sale
pinup azerbaycan [url=http://pinup-azerbaycan3.com/]pinup azerbaycan[/url] .
The payout waits online drag—speed on! plinko
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Мишень CoCr для распыления сплавов
https://telegra.ph/Legit-PayPal-Casinos-in-the-UK-Without-GamStop-Restrictions-03-27
https://telegra.ph/Non-Gamstop-Casinos-UK-Free-Spins-Offers-and-Tips-03-27
Задался вопросом: можно ли на самом деле купить диплом государственного образца в Москве? Был приятно удивлен — это реально и легально!
Сначала искал информацию в интернете на тему: высшее купить диплом во владивостоке, корки диплома купить, купить диплом 2014, купить диплом в жуковском, купить диплом в крыму и получил базовые знания. В итоге остановился на материале: [url=http://diplomybox.com/kupit-diplom-astrakhan/]diplomybox.com/kupit-diplom-astrakhan[/url]
Вместе с t.me/azino777_a у вас есть возможность в увлекательное путешествие по миру азартных игр отправиться. Предлагаем персонализированные предложения и уникальные бонусы. Расскажем, как в Азино начать играть. Вас ждет возможность выиграть крупный приз и невероятные эмоции. Испытайте свою удачу уже сейчас! Ищете azino777 casino com? – тут имеется множество актуальной информации. Собрали самые интересующие вопросы от игроков. Рады вам помочь. Приготовьтесь к завлекательным в Азино турнирам. Участвуйте и боритесь за призы с другими игроками!
Probanki-online.ru – сайт, который предлагает вам исчерпывающую информацию о банковских услугах и финансовых продуктах в России. Расскажем, автокредит, что это такое. Объясним, как идеальную карту выбрать. Вы узнаете о плюсах вкладов. Стремимся в курсе последних финансовых событий быть. Ищете банки онлайн? Probanki-online.ru – здесь можете отыскать информацию о разных банках и их предложениях. На портале имеется поиск, примените его. Сделать процесс выбора финансовых продуктов удобным и простым – вот наша основная цель. Рады видеть вас на нашем сайте!
подключение интернета санкт-петербург
domashij-internet-spb001.ru
провайдеры санкт-петербург
https://telegra.ph/Best-Non-Gamstop-Casino-Sites-for-UK-Players-03-27
Tiger Fortune has the best vibe of any slot. fortune tiger
Мы можем предложить дипломы любой профессии по выгодным ценам. Мы предлагаем документы техникумов, расположенных в любом регионе Российской Федерации. Документы выпускаются на бумаге высшего качества. Это дает возможность делать настоящие дипломы, которые невозможно отличить от оригинала. [url=http://aqtanmomon.copiny.com/question/details/id/1082956/]aqtanmomon.copiny.com/question/details/id/1082956[/url]
Drugs information sheet. Effects of Drug Abuse.
cost cheap sinemet without insurance
All news about medication. Read information now.
Tiger Fortune’s gameplay is always thrilling. tigrinho demo
https://telegra.ph/Explore-the-Best-Non-Gamstop-Casinos-for-Players1-03-27
Medicines information for patients. Drug Class.
buying compazine tablets
Everything news about pills. Read information now.
Tiger Fortune’s free spins are pure gold. fortune tiger
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наши товары:
Свинцовый круг 100 мм С1С ГОСТ 3778-98 Выберите идеальные свинцовые круги для точения, шлифовки и полировки металлов на сайте Редметсплав.рф. Широкий выбор размеров, высокое качество и профессиональная консультация. Успейте приобрести свинцовые круги для обработки металла уже сегодня!
Tiger Fortune’s rewards make it worth playing. tiger fortune
https://telegra.ph/No-Gamstop-Casinos-Safe-for-Players-in-2023-03-27
visit https://unchainx.net/
Заказать диплом о высшем образовании!
Мы предлагаем документы учебных заведений, расположенных на территории всей РФ.
[url=http://diplomservis.com/kupit-diplom-s-registratsiej-v-reestre-nedorogo-2/]diplomservis.com/kupit-diplom-s-registratsiej-v-reestre-nedorogo-2/[/url]
additional info https://noon.lat/
Мы предлагаем изготовление значков любой сложности с использованием различных типов нанесения и креплений, что позволяет создать уникальный продукт https://kroxa-expert.ru/izgotovlenie-metallicheskih-znachkov-metodom-himicheskogo-travleniya/
cefixime dose for children
cost of allegra pill
РедМетСплав предлагает широкий ассортимент отборных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы обеспечиваем оперативное исполнение вашего заказа.
Каждая единица продукции подтверждена требуемыми документами, подтверждающими их происхождение. Опытная поддержка – наш стандарт – мы на связи, чтобы улаживать ваши вопросы по мере того как находить ответы под требования вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
Порошок ниобиевый РќР±РџРњРџРќР‘-1 Порошок ниобиевый РќР±РџРњРџРќР‘-1 является высококачественным материалом, используемым РІ различных промышленных отраслях. Ртот порошок обладает уникальными физико-химическими свойствами, такими как высокая температура плавления Рё отличная коррозионная стойкость. Благодаря этим характеристикам РѕРЅ становится незаменимым РІ производстве сплавов Рё специальных материалов. Если РІС‹ хотите гарантировать надежность Рё долговечность СЃРІРѕРёС… продуктов, купить Порошок ниобиевый РќР±РџРњРџРќР‘-1 – это отличный выбор. Рнвестируйте РІ качество Рё эффективность СѓР¶Рµ сегодня.
куплю диплом высшего образования [url=https://diplomys-vsem.ru/]куплю диплом высшего образования[/url] .
https://telegra.ph/Best-Safe-Non-Gamstop-Casinos-Reviewed-for-Players-03-27
скачать mostbet на телефон [url=http://mostbet6012.ru]http://mostbet6012.ru[/url] .
I can’t get enough of the Tiger Fortune soundtrack! fortune tiger 777
pin up azerbaijan [url=http://pinup-azerbaycan3.com]pin up azerbaijan[/url] .
Baltour — блог о путешествиях с характером. Исследуем города, природу, маршруты и неожиданные места — по Балтике и не только. Без шаблонов, с душой и реальными впечатлениями онлайн туры
The bonus features in Tiger Fortune are super rewarding. tiger fortune
O betmotion oferece uma
excelente oportunidade para quem deseja começar sua experiência no cassino online com
um bônus de 100$ para novos jogadores! Ao se registrar no site,
você garante esse bônus exclusivo que pode ser utilizado em diversos jogos de cassino, como slots, roleta e poker.
Esse é o momento perfeito para explorar o mundo
das apostas com um saldo extra, aproveitando
ao máximo suas apostas sem precisar investir um grande valor logo de início.
Não perca essa oportunidade e cadastre-se já!
Drug information for patients. Brand names.
can i buy thorazine
All information about drug. Read information now.
This casino’s Tiger Fortune game is legendary. fortune tiger demo gratis
Drug information for patients. Short-Term Effects.
cost pioglitazone without a prescription
All what you want to know about medicament. Read here.
pin up az?rbaycan [url=https://pinup-azerbaycan4.com]pin up az?rbaycan[/url] .
В данной обзорной статье представлены интригующие факты, которые не оставят вас равнодушными. Мы критикуем и анализируем события, которые изменили наше восприятие мира. Узнайте, что стоит за новыми открытиями и как они могут изменить ваше восприятие реальности.
Получить дополнительную информацию – https://abrahamcarle.com/riesgo-cardiovascular-1-interpretacion-de-analiticas-sanguineas-para-entrenadores
домашний интернет подключить санкт-петербург
domashij-internet-spb002.ru
лучший интернет провайдер санкт-петербург
Tiger Fortune’s visuals are next-level cool. jogo do tigrinho demo
I wish online casinos had better mobile apps; some are so glitchy! sugar rush
Форум социальной инженерии — Lolz.live предлагает уникальную возможность на выгодных условиях продать игровые аккаунты, а также учетные записи для социальных сетей, скилы и различную игровую атрибутику. Этот портал будет полезен как новичкам, которые только рассматривают продажу аккаунтов в качестве заработка, так и тем, для кого это уже стало бизнесом.
Vulkan Vegas is a casino known for its reliability and quality service. In 2025, Vulkan Vegas continues to delight players with a variety of games and convenient conditions for playing in rubles лучшие казино онлайн
mostbet промокод [url=http://mostbet6033.ru/]http://mostbet6033.ru/[/url] .
This casino’s Tiger Fortune game is incredible. fortune tiger bet
can you get generic biltricide pills
cannabis store in prague buy hashish in prague
cleocin suppository dosage 55
Вы находитесь на едином сайте аренды, где получится взять в прокат различную технику для выполнения самых разных работ. Вы сможете опубликовать объявление и предложить свою технику, чтобы ее заказал кто-то еще. https://arenda24.by/ – на сайте изучите категории, которые будут вам полезны: грузоперевозки, вспомогательное оборудование, режущий инструмент, строительные краны и многое другое. Вы сможете воспользоваться всеми опциями при условии, что зарегистрируетесь.
Tiger Fortune’s design is bold and exciting. fortune tiger
Medicine information. What side effects can this medication cause?
lyrica junkies
Best information about medicament. Read now.
Pills information sheet. Short-Term Effects.
can i purchase generic anastrozole price
All news about medicament. Read information here.
Мы готовы предложить дипломы любой профессии по приятным ценам. Всегда стараемся поддерживать для заказчиков адекватную ценовую политику. Важно, чтобы документы были доступны для большинства наших граждан.
Покупка документа, подтверждающего обучение в ВУЗе, – это грамотное решение. Заказать диплом ВУЗа: [url=http://kupit-diplomyz24.com/diplom-kupit-sredne-texnicheskoe-obrazovanie-5/]kupit-diplomyz24.com/diplom-kupit-sredne-texnicheskoe-obrazovanie-5/[/url]
Отец и сын три года объедали сотню ресторанов по хитроумной схеме
https://pin.it/kCDDdtZJq
How to Sign Up and Log In to sbobet:
$100 Bonus for New Users!
How to Sign Up and Log In to buumi: $100 Bonus for New Users!
Become a patti Member and Claim Your $100 Welcome Bonus!
Sign Up for tsars and
Claim Your $100 Bonus Instantly!
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
С широким ассортиментом продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наши товары:
Плоская мишень ZrO2, 4N для распыления оксида циркония
Krause-ekb.ru предлагает широкий ассортимент лестниц и стремянок по умеренным ценам. Работаем с организациями и частными лицами. Предоставим вам бесплатную консультацию. Совершить покупки стало гораздо проще, теперь не надо из дома выходить. Для этого каталогом воспользуйтесь, который на ресурсе предоставлен. Ищете купить раздвижную лестницу? Krause-ekb.ru – тут подробная информация о каталоге опубликована. Предложить качественные товары и доставить заказ покупателю как можно оперативнее – это основная цель Krause. Если у вас остались вопросы, свяжитесь с нами по телефону.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наши товары:
Плоская мишень для распыления свинца 4N
The tiger symbols in Tiger Fortune are iconic. fortune tiger
узнать интернет по адресу
domashij-internet-spb003.ru
недорогой интернет санкт-петербург
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Обладая обширной линейкой редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наша продукция:
Гранулы силикона 4N
Tiger Fortune is a standout slot in this casino. fortune tiger 777
Desert Eagle Blaze http://csgo-get-skins.ru пламя в каждой пуле. Легенда CS:GO. Продажа, обмен, проверка скина. Успей забрать по лучшей цене на рынке.
Академия «Арчибальд» занимается обучением грумингу. Вы можете совершенствовать собственные навыки на живых моделях до бесконечности. Преподаватели на все вопросы отвечают. Дарим методические материалы, учебники, а также видеолекции. Погрузитесь в атмосферу, с которой не захочется расставаться. Ищете курсы груминга москва лучшие? Grooming-dream.ru – здесь представлены реальные отзывы клиентов. Мы выпускаем отменных груминг-специалистов. Предлагаем во время обучения инструменты. Если вам интересна новая профессия – Арчибальд, вот то место, которое вам подойдет.
Get Started with a $100 Bonus at gamdom – Register Now!
M4A1-S Knight https://cs-get-skins.ru редкий скин в CS. Престиж, стиль, легендарное оружие. Продажа по выгодной цене, моментальная доставка, безопасная сделка.
Отец и сын три года объедали сотню ресторанов по хитроумной схеме
https://pin.it/kCDDdtZJq
Register on mrrex and
Start Winning with Your $100 Bonus!
pinup azerbaycan [url=https://pinup-azerbaycan4.com]pinup azerbaycan[/url] .
Medicament information leaflet. Drug Class.
where can i buy generic actos price
Everything what you want to know about medication. Get information here.
Get started on mrbit today and enjoy
a $100 bonus upon registration! Signing up is
quick and simple. Just create an account, log in, and you’ll be instantly rewarded with a $100
bonus. This welcome offer is exclusive to new users, providing a fantastic
opportunity to boost your gaming experience right from the start.
Don’t miss out on this limited-time offer.
Register today, and the bonus will be yours as soon as you complete your sign-up process.
I hit a progressive jackpot online once—life-changing moment! craps game
Купить диплом о высшем образовании!
Мы можем предложить документы институтов, которые находятся в любом регионе РФ.
[url=http://diplomp-irkutsk.ru/diplom-s-zaneseniem-v-reestr-dlya-vashej-kareri/]diplomp-irkutsk.ru/diplom-s-zaneseniem-v-reestr-dlya-vashej-kareri/[/url]
can you buy cheap lamisil prices
Exclusive Offer: $100 Bonus at ivibet for New
Users!
Start Your Winning Streak with a $100 Bonus at winny!
where can i get generic doxycycline pills
This casino’s Tiger Fortune slot is pure joy. fortune tiger demo
Looking for a great start on vave?
New users get a $100 bonus when they register! The registration process is quick and easy.
Just fill in your details, log in, and your bonus
will be waiting for you. With your $100 bonus, you’ll be ready to
explore the exciting world of online casinos, from classic slots to live dealer games.
Sign up now and take advantage of this amazing offer!
Claim Your $100 Bonus with a Quick Registration at maneki!
This was a great read. Appreciate the effort you put into this!
Claim Your $100 Bonus with a Quick Registration at comeon!
Start your journey at betobet with an exclusive $100 bonus!
New users can instantly claim this offer when they register, giving them a head start
on exploring the diverse range of online casino games, slots, and
sports betting options available. Don’t miss out on this exciting
opportunity—sign up today and take advantage
of this amazing $100 bonus to boost your chances of
winning big!
New to casigo? Get Your $100 Bonus Just
for Signing Up!
Металлические значки на заказ являются не только стильным аксессуаром, но и эффективным способом выразить индивидуальность или подчеркнуть принадлежность к определённой группе. Их производство сочетает в себе искусство и мастерство, позволяя создавать уникальные изделия с высоким уровнем детализации. Подробнее об этом можно узнать по ссылке:
https://vpenze.ru/news/metallicheskie-znachki-na-zakaz-iskusstvo-kotoroe-govorit/
Start your journey at nomini with an exclusive $100
bonus! New users can instantly claim this
offer when they register, giving them a head start on exploring the diverse range of
online casino games, slots, and sports betting options available.
Don’t miss out on this exciting opportunity—sign up
today and take advantage of this amazing $100 bonus to boost your chances of winning big!
How to Log In to pino and Receive a $100 Bonus on Registration
Looking for a way to boost your online casino experience?
nextbet has just the solution! Register as a new user and claim a $100 bonus to start playing right away.
Whether you’re into sports betting or exploring
the latest slots, this bonus gives you the perfect
opportunity to get familiar with the platform and its offerings.
Don’t miss out—sign up today and start playing with your $100 bonus in hand!
How to Sign Up and Log In to bons:
$100 Bonus for New Users!
Привет!
Мы предлагаем дипломы любых профессий по невысоким ценам. Стоимость может зависеть от выбранной специальности, года получения и университета: [url=http://diplomans.com/]diplomans.com/[/url]
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
поставляемая продукция:
Мишень РёР· драгоценного металла или его сплава кольцевая платина 30С…0.5 РјРј РџР»Р90-10 РўРЈ Выберите уникальные мишени РёР· драгоценных металлов для напыления РѕС‚ Редметсплав.СЂС„. Обеспечивают высокую степень защиты Рё долговечность. Рдеальный выбор для промышленности, строительства Рё производства.
мост бет [url=http://mostbet6033.ru/]http://mostbet6033.ru/[/url] .
Привет!
Мы изготавливаем дипломы любых профессий по приятным тарифам. Стоимость зависит от выбранной специальности, года выпуска и образовательного учреждения: [url=http://rdiploman.com/]rdiploman.com/[/url]
Sign Up at betfury and Get a $100 Bonus Instantly!
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
Наши товары:
Лента РёР· прецизионных сплавов для СѓРїСЂСѓРіРёС… элементов 0.7×225 РјРј 40РљРҐРќРњ ГОСТ 14117-85 Купите ленту РёР· прецизионных сплавов для СѓРїСЂСѓРіРёС… элементов РїРѕ выгодной цене РЅР° Редметсплав.СЂС„. Высокая прочность, устойчивость Рє РєРѕСЂСЂРѕР·РёРё Рё отличная эластичность делают этот материал идеальным для различных отраслей. Предлагаем широкий ассортимент продукции Рё РїРѕРґСЂРѕР±РЅСѓСЋ информацию для правильного выбора.
Register with satsport Now and Grab a $100 Bonus for New Players!
Exclusive $100 Bonus for New Registrants at marvel bet!
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наша продукция:
Заготовка из конструкционной стали листовая 40 мм Ст2пс ОСТ 3-1686-90 Выбирайте конструкционные заготовки высокого качества для вашего проекта на Редметсплав.рф. Наш ассортимент включает прочные и долговечные материалы, идеально подходящие для различных областей применения, включая строительство и машиностроение.
Гигантская черная дыра пробудилась, заявили ученые
https://x.com/kiselev_igr/status/1910669547713003851
This casino’s Tiger Fortune slot is unreal. fortune tiger demo
Sign Up for bilbet and Claim
Your $100 Bonus Instantly!
Thanks for shedding light on this topic. Learned something new today!
Get More from heyspin – Register Now and Receive a $100
Bonus!
Online poker pumps—bluff high! mines
Get a $100 Bonus Right After Registering at pgebet – https://pgebet-in.com!
Get Started with a $100 Bonus at bluechip – Register
Now!
Tiger Fortune’s jungle vibe is unbeatable. fortune tiger demo
Sign Up for rivalry, Claim Your $100 Bonus, and Start Playing
New Players Get a $100 Bonus at baji – Register Now!
$100 Bonus Just for Signing Up – Join pinnacle
Now!
For fast-paced excitement, Chicken Road Game delivers quirky races and chaotic challenges. Download Chicken Road now and dive into thrilling adventures on your device chicken road game money download
РедМетСплав предлагает широкий ассортимент качественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица изделия подтверждена требуемыми документами, подтверждающими их происхождение. Дружелюбная помощь – наш стандарт – мы на связи, чтобы ответить на ваши вопросы а также адаптировать решения под специфику вашего бизнеса.
Доверьте ваш запрос специалистам РедМетСплав и убедитесь в множестве наших преимуществ
Наши товары:
Магний AM60B – ASTM B94 Магний AM60B – ASTM B94 представляет СЃРѕР±РѕР№ высококачественный магниевый сплав, который обеспечивает отличную прочность Рё легкость. РћРЅ широко используется РІ авиационной Рё автомобильной промышленности благодаря своей высокой стойкости Рє РєРѕСЂСЂРѕР·РёРё. Сплав легко поддается обработке Рё сварке, что делает его идеальным выбором для различных производственных процессов. Если РІС‹ ищете надежный материал для СЃРІРѕРёС… проектов, вам стоит купить Магний AM60B – ASTM B94. Ртот сплав сочетает РІ себе высокую производительность Рё доступную цену, что делает его лучшим выбором для современных технологий.
ТОП-10 лучших сервисов для WhatsApp-рассылки WhatsApp является одним из самых популярных мессенджеров в мире, с миллиардами активных пользователей https://marketing-i.ru/
РедМетСплав предлагает обширный выбор качественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до масштабных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их происхождение. Превосходное обслуживание – наш стандарт – мы на связи, чтобы разрешать ваши вопросы по мере того как предоставлять решения под требования вашего бизнеса.
Доверьте ваш запрос специалистам РедМетСплав и убедитесь в гибкости нашего предложения
поставляемая продукция:
Проволока висмутовая C 6801 – JIS H 3250:2015 Проволока висмутовая C 6801 – JIS H 3250:2015 — это высококачественный металл, созданный для различных промышленных применений. Обладает отличной РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью Рё высокой прочностью, что делает её идеальным выбором для электросхем Рё высокоточных элементов. Рспользование данной проволоки способствует улучшению электрических характеристик Рё продолжению СЃСЂРѕРєР° службы изделий. Если РІС‹ хотите купить Проволока висмутовая C 6801 – JIS H 3250:2015, РЅРµ упустите возможность приобрести надежный материал для вашего производства. Рспользуйте её РІ СЃРІРѕРёС… проектах для достижения высоких результатов.
Registering at lottoland comes with great perks, including a $100 bonus!
New users who sign up will instantly receive a $100 bonus to boost their gameplay.
The registration process is easy and secure – just fill in the required details, log in, and the bonus will be credited to your account.
Whether you prefer slots, table games, or live casinos, this bonus will enhance your chances of winning.
Don’t wait, register now and grab your $100 bonus!
РедМетСплав предлагает широкий ассортимент качественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица продукции подтверждена требуемыми документами, подтверждающими их соответствие стандартам. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы улаживать ваши вопросы а также находить ответы под специфику вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
поставляемая продукция:
Рлектрод вольфрамовый W-Cu10 Рлектрод вольфрамовый W-Cu10 – это высококачественный сварочный материал, который объединяет РІ себе прочность вольфрама Рё отличные электрические свойства меди. РћРЅ идеально РїРѕРґС…РѕРґРёС‚ для TIG-сварки, обеспечивая стабильную РґСѓРіСѓ Рё отличную свариваемость. Данный электрод характеризуется высокой РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью Рё термостойкостью, что делает его универсальным решением для различных промышленных применений. Если РІС‹ хотите купить Рлектрод вольфрамовый W-Cu10, РІС‹ сделаете выбор, который повысит качество ваших сварочных работ. Рти электроды обеспечивают надежность Рё долговечность РІ каждой операции.
$100 Bonus Just for Signing Up – Join slotbox Now!
Experience the thrill of winning at betshah with a special $100 bonus for
new users! By signing up, you’ll get access
to exciting casino games, sports betting, and much more.
Whether you’re a seasoned player or new to the world of online casinos, this bonus gives you the opportunity to
start strong. Register today, claim your $100 bonus, and explore a variety of games—all from the comfort of your home!
Drugs information leaflet. Short-Term Effects.
cafergot buy without prescriptiion
Everything what you want to know about pills. Read information now.
How to Register and Get Your $100 Bonus at spinrio
Your blog always delivers quality content—keep it coming!
ai porn pics free ai porn
Join teen patti
Today and Claim Your $100 Welcome Bonus!
Exclusive $100 Bonus for New Registrants at national!
Используй нвдежный amneziavpn для обхода блокировок, сохранности личных данных и полной свободы онлайн. Всё включено — просто подключайся.
Tiger Fortune’s tiger roars make every win epic. fortune tiger bet
I don’t get online hate—it’s cool! aviator
Оснащение под ключ: медицинское оборудование купить в Москве с сертификацией и полной документацией. В наличии и под заказ. Профессиональное оснащение медицинских кабинетов.
Купить диплом любого университета поспособствуем. Купить диплом магистра во Владивостоке – [url=http://diplomybox.com/kupit-diplom-magistra-vo-vladivostoke/]diplomybox.com/kupit-diplom-magistra-vo-vladivostoke[/url]
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние успехи.
Наши товары:
Гранулы высокочистого ниобия
https://dev-mastery.pro/
https://xnudes.app/
Tiger Fortune keeps the adrenaline pumping every spin. fortune tiger bet
pin up azerbaijan [url=https://pinup-azerbaycan4.com/]pin up azerbaijan[/url] .
Мы изготавливаем дипломы психологов, юристов, экономистов и других профессий по доступным ценам. Липовый диплом, который говорит сам за себя — [url=http://kyc-diplom.com/diplom-articles/lipovyj-diplom-kotoryj-govorit-sam-za-sebya.html/]kyc-diplom.com/diplom-articles/lipovyj-diplom-kotoryj-govorit-sam-za-sebya.html[/url]
https://dev-mastery.pro/
https://xnudes.app/
Drug information. Drug Class.
buying cheap dramamine without a prescription
Some information about drugs. Get information now.
https://xnudes.app/
https://xnudes.app/
This post really resonated with me. Can’t wait to read more from you!
Технологии свободы: marzban.click надёжный инструмент для приватности, скорости и доступа к любым сайтам. Быстро, безопасно, удобно — настрой за 5 минут.
Looking for best prompts for dall-e? Findprompt.shop, here you have the opportunity to make your choice from a large selection of promts for ChatGPT, Stable Diffusion, Midjourney and others. Study the best recommendations for AI. You can sell your promts at a favorable price on our platform. Pay close attention to the choice of promts on the portal – it is the largest on the market. Our offers are unique, and the cost is affordable for everyone.
Витебский госуниверситет университет https://vsu.by/inostrannym-abiturientam/spetsialnosti.html П.М.Машерова – образовательный центр. Вуз является ведущим образовательным, научным и культурным центром Витебской области. ВГУ осуществляет подготовку: химия, биология, история, физика, программирование, педагогика, психология, математика.
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
Наши товары:
Рнструментальная квадратная РїРѕРєРѕРІРєР° 175 РјРј ХГС ГОСТ 5950-2000 Познакомьтесь СЃ широким ассортиментом высокопрочных инструментов для обработки металла РІ категории инструментальной квадратной РїРѕРєРѕРІРєРё РѕС‚ Редметсплав. Выбирайте РёР· прочных Рё надежных материалов, подобранных специально для различных РІРёРґРѕРІ металлообработки. Гарантированное качество РѕС‚ ведущих производителей.
This online casino hit the mark with Tiger Fortune. tigrinho demo
can i purchase cheap methocarbamol pills
нью ретро казино
browse this site [url=https://tlo-lookup.com]Carding[/url]
Основы программирования контроллеров Siemens, для опытных специалистов.
Тонкости программирования контроллеров Siemens, секреты.
Преимущества TIA Portal в программировании контроллеров Siemens, все, что вам нужно знать.
Типичные ошибки в программировании, распространенные проблемы.
Как спроектировать систему автоматизации с Siemens, для успешной реализации.
Сравнение контроллеров Siemens, для оптимальной работы.
Сравнение языков программирования для контроллеров Siemens, выбор.
Как контроллеры Siemens помогают в автоматизации, для производства.
Современные тенденции в программировании контроллеров Siemens, что нужно знать.
Разработка пользовательских интерфейсов для контроллеров Siemens, для удобства использования.
Запрограммировать сименс [url=http://programmirovanie-kontroller.ru/#Запрограммировать-сименс]http://programmirovanie-kontroller.ru/[/url] .
can you get generic advair diskus price
I love how unpredictable Tiger Fortune is! fortune tiger demo
The authorization process at Arkada Casino is as simple as possible. The player needs to enter an email and password, after which he gets access to his personal account Arkada казино официальный сайт вход
This online casino’s Tiger Fortune is a masterpiece. jogo do tigrinho demo
купить чеки в москве купить чек на товар
Научитесь вязать крючком crochet-patterns.ru/ с нуля или улучшите навыки с нашими подробными мастер-классами. Фото- и видеоуроки, понятные инструкции, схемы для одежды, игрушек и интерьера. Вдохновляйтесь, творите, вяжите в своё удовольствие! Вязание крючком — доступно, красиво, уютно.
discover here
[url=https://tlo-ssndob.com/]OTP[/url]
РедМетСплав предлагает обширный выбор отборных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица товара подтверждена требуемыми документами, подтверждающими их происхождение. Превосходное обслуживание – наша визитная карточка – мы на связи, чтобы разрешать ваши вопросы и находить ответы под требования вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в множестве наших преимуществ
Наша продукция:
Рзделия РёР· кобальта Stellite 107 Рзделия РёР· кобальта Stellite 107 представляют СЃРѕР±РѕР№ высококачественные компоненты, используемые РІ различных отраслях благодаря своей исключительной стойкости Рє РёР·РЅРѕСЃСѓ Рё РєРѕСЂСЂРѕР·РёРё. Рти изделия применяются РІ производстве инструментов Рё оборудовании, РіРґРµ требуется максимальная производительность. Если РІС‹ ищете надежное решение для СЃРІРѕРёС… производственных процессов, то вам стоит рассмотреть возможность купить Рзделия РёР· кобальта Stellite 107. РС… отличные механические свойства Рё высокая температура плавления обеспечивают долгий СЃСЂРѕРє службы Рё минимальные затраты РЅР° замену.
изи кэш казино, голд казино, либет казино
The application is available for Android and iOS, providing quick access to the gameplay. Simple installation and user-friendly interface will make the game comfortable, and the mobile version will allow you to enjoy gambling entertainment anywhere Казино Banda мобильная версия
I wish online paid swift—drag off! plinko
Блог о маркетплейсах «OOWA» предлагает ознакомиться с актуальной, интересной и познавательной информацией, которая касается удачных продаж на маркетплейсах, обзоров товаров и услуг. На портале регулярно публикуются ценные и содержательные рекомендации от экспертов, которые помогут начать лучше разбираться в тонкостях такого бизнеса. Ищете как на ozon можно увеличить продажи? На oowa.ru регулярно публикуются ценные и содержательные рекомендации от экспертов, которые помогут начать лучше разбираться в тонкостях такого бизнеса. Почитайте о самых востребованных товарах с маркетплейсов.
Medicament prescribing information. Effects of Drug Abuse.
where buy valtrex price
Best what you want to know about medicine. Get now.
Заказать диплом академии!
Мы можем предложить дипломы любой профессии по приятным ценам. Вы покупаете диплом через надежную и проверенную временем компанию. : [url=http://resourster.com/companies/frees-diplom/]resourster.com/companies/frees-diplom[/url]
This online casino’s Tiger Fortune is a thrill. fortune tiger
Нож-бабочка Doppler cs-get-skin стильное и эффектное оружие в стиле CS:GO. Яркий металлический блеск, плавный механизм, удобство в флиппинге и коллекционировании. Подходит для тренировок, трюков и подарка фанатам игр.
Tiger Fortune’s graphics are simply amazing. tiger fortune
buy viagra online [url=viagrarrtt.com]viagrarrtt.com[/url] .
Мы готовы предложить дипломы любой профессии по выгодным ценам. Стараемся поддерживать для заказчиков адекватную политику цен. Важно, чтобы дипломы были доступны для большинства граждан.
Заказ документа, подтверждающего окончание института, – это рациональное решение. Приобрести диплом о высшем образовании: [url=http://diplom-bez-problem.com/gde-mozhno-kupit-diplom-4/]diplom-bez-problem.com/gde-mozhno-kupit-diplom-4/[/url]
I love the low play online—calm risk! plinko
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние достижения.
Наша продукция:
Циркониевая мишень для распыления
Online bingo chills—cool gang! dragon vs tiger game download
Medicament information leaflet. Brand names.
vistaril and hydroxyzine hcl
All news about drugs. Get information here.
Если вы находитесь в поисках качественного, надежного и практичного стального профиля, то обратитесь на предприятие ООО “Валцпроф”, где вы найдете продукцию в широком ассортименте. На предприятии вы получите возможность приобрести выпадающие пороги, адаптированные для дверей разного вида. https://waltzprof.com/ – на портале изучите весь ассортимент товаров, которые выпускаются заводом. Все представленные в каталоге профили предназначены для комфортной, быстрой сборки перегородок, ограждающих преград, фасадных конструкций, окон, люков.
Мы изготавливаем дипломы любой профессии по приятным тарифам. Дипломы производятся на настоящих бланках государственного образца Заказать диплом о высшем образовании [url=http://diplomidlarf.ru/]diplomidlarf.ru[/url]
Где заказать диплом специалиста?
Приобрести диплом института по невысокой цене возможно, обращаясь к надежной специализированной компании.: [url=http://diplom-profi.ru/]diplom-profi.ru[/url]
This online casino’s Tiger Fortune is top-class. fortune tiger
ООО «Короче, дилер» представляет собой официальную библиотеку знаний ассоциации «Российские автомобильные дилеры». На портале https://koroche-dealer.ru каждый, у кого есть вопросы по теме, сможет найти на них ответы. Представлены самые актуальные материалы, касающиеся работы дилерских центров по самым разным направлениям.
cost of aristocort online
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Обладая обширной линейкой редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым текущие трудности превращаются в завтрашние возможности.
Наши товары:
Титановый сесквиоксид гранулированный
delai action cordarone generic name
I won big last night playing Tiger Fortune – what a thrill! fortune tiger demo
Meds prescribing information. What side effects?
buying generic esomeprazole prices
Best news about drugs. Get now.
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – наилучшее решение для вашего бизнеса.
поставляемая продукция:
Лист судостроительный 7x1500x3000 D EN10025:3 Приобретите высококачественные листы судостроительные от компании Редметсплав.рф для надежного и долговечного использования в вашем судостроительном проекте. Различные размеры и толщины, прочность и устойчивость к коррозии делают эти листы идеальным выбором для любого судостроительного проекта.
buy viagra [url=http://www.kanishksteels.com]http://www.kanishksteels.com[/url] .
I won spins online and got $600—sweet score! plinko
[b]Диплом любого ВУЗа России![/b]
Без университета трудно было продвинуться по карьере. Именно из-за этого решение о заказе диплома следует считать мудрым и целесообразным. Приобрести диплом института [url=http://jobpanda.co.uk/employer/gosznac-diplom-24/]jobpanda.co.uk/employer/gosznac-diplom-24[/url]
Medicament information sheet. Cautions.
can i get celebrex online
Actual about medication. Read now.
Ищете медтехника для дома интернет-магазин? Agsvv.ru/catalog/obluchateli_dlya_lecheniya у вас появится возможность приобрести для лечения кожных болезней облучатели по цене производителя с гарантией. ООО Хронос изготовлением облучателей для лечения кожных заболеваний с 2009-го года с доказанными методами лечения занимается. Медицинские приборы от изготовителя отменным качеством славятся! Посмотрите наш ассортимент и назначение приборов. Мы приборы своего производства частным и государственным медучреждениям по всей РФ поставляем.
РедМетСплав предлагает широкий ассортимент отборных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы обеспечиваем оперативное исполнение вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их происхождение. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы разрешать ваши вопросы и предоставлять решения под требования вашего бизнеса.
Доверьте ваш запрос специалистам РедМетСплав и убедитесь в множестве наших преимуществ
поставляемая продукция:
РџРѕРєРѕРІРєР° кобальтовая РРџ949 РџРѕРєРѕРІРєР° кобальтовая РРџ949 представляет СЃРѕР±РѕР№ уникальный сплав, обладающий высокой прочностью Рё стойкостью Рє РєРѕСЂСЂРѕР·РёРё. Ртот материал используется РІ самых ответственных отраслях, включая авиацию Рё медицину. РџРѕРєРѕРІРєР° кобальтовая РРџ949 отличается отличной свариваемостью Рё возможностью обработки, что делает её идеальным выбором для создания надежных деталей Рё конструкций. Р’С‹ можете купить РџРѕРєРѕРІРєР° кобальтовая РРџ949 РІ нашем магазине Рё обеспечить СЃРІРѕР№ проект высококачественными материалами. РќРµ упустите шанс воспользоваться этим предложением!
Tiger Fortune’s free spins are a game-changer. fortune tiger bet
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с высоким уровнем сервиса.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
Наша продукция:
Лист износостойкий 34x2000x6000 Magstrong W600 Выберите лист износостойкий от Редметсплав для надежной защиты и высокой производительности. Наши прочные и долговечные листы обеспечивают устойчивость к износу при высоких нагрузках. Широкий спектр применения в различных отраслях. Высокое качество по выгодной цене. Закажите онлайн!
Drug information leaflet. What side effects can this medication cause?
methocarbamol dose
Best news about medication. Get information here.
Приобрести диплом института по невысокой цене вы сможете, обратившись к проверенной специализированной фирме. Заказать документ о получении высшего образования вы имеете возможность в нашей компании. [url=http://diplomg-cheboksary.ru/kupite-diplom-o-visshem-obrazovanii-bistro-i-nadezhno-4/]diplomg-cheboksary.ru/kupite-diplom-o-visshem-obrazovanii-bistro-i-nadezhno-4[/url]
how to buy advair diskus without insurance
can i get generic fincar no prescription
Tiger Fortune has some seriously cool visuals. fortune tiger 777
РедМетСплав предлагает обширный выбор отборных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы обеспечиваем быстрое выполнение вашего заказа.
Каждая единица продукции подтверждена всеми необходимыми документами, подтверждающими их происхождение. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы улаживать ваши вопросы по мере того как находить ответы под требования вашего бизнеса.
Доверьте ваш запрос специалистам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
Проволока магниевая B 92 (9990A) – ASTM B92 Полоса магниевая B 92 (9990A) – ASTM B92 представляет СЃРѕР±РѕР№ высококачественный материал, который используется РІ различных отраслях благодаря СЃРІРѕРёРј уникальным характеристикам. РћРЅР° обладает отличной РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью Рё легкостью, что делает ее идеальным выбором для применения РІ промышленных Рё строительных проектах. Полоса магниевая B 92 (9990A) – ASTM B92 обладает хорошей термостойкостью Рё прочностью. Если РІС‹ хотите купить Полоса магниевая B 92 (9990A) – ASTM B92, РЅРµ упустите возможность воспользоваться данным предложением. Ртот РїСЂРѕРґСѓРєС‚ станет отличным дополнением вашего производства, обеспечивая надежность Рё долговечность РІ эксплуатации.
I love how Tiger Fortune keeps me engaged. tigrinho demo
I love the jungle energy of Tiger Fortune. tigrinho demo
Medicine information sheet. Brand names.
cheap compazine tablets
Everything what you want to know about medicine. Get now.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наши товары:
Вращающаяся мишень хрома
Вместе с t.me/azino777_a можете отправиться в захватывающее путешествие по миру азартных игр. Предлагаем уникальные бонусы и персонализированные предложения. Расскажем, как в Азино начать играть. Вас ждет возможность выиграть крупный приз и невероятные эмоции. Испытайте свою удачу уже сейчас! Ищете azino777 casino играть? – тут имеется множество актуальной информации. Собрали самые интересующие вопросы от игроков. Всегда готовы помочь вам. Приготовьтесь к увлекательным турнирам в Азино. Участвуйте и с другими игроками боритесь за призы!
Impressive work! You’ve made an interesting topic even more engaging.
Быстровозводимые здания https://akkord-stroy.ru из металлоконструкций — это скорость, надёжность и экономия. Рассказываем в статьях, как построить объект под ключ: от проектирования до сдачи в эксплуатацию.
Недвижимость в Бяла https://byalahome.ru апартаменты, квартиры и дома у моря в Болгарии. Лучшие предложения на побережье Черного моря — для жизни, отдыха или инвестиций. Успейте купить по выгодной цене!
Скин AWP Graphite csgo-case-simulator.ru из CS:GO — чёрный глянец, премиальный стиль и высокая редкость. Укрась арсенал культовой снайперской винтовкой и выделяйся на сервере с первого выстрела.
AK-47 Fire Serpent http://get-skins-cs.ru легендарный скин из коллекции Operation Bravo. Яркий рисунок огненного змея, высокая редкость и коллекционная ценность. Идеальный выбор для истинных фанатов CS:GO.
escort in islamabad [url=http://www.kanishksteels.com]http://www.kanishksteels.com[/url] .
The tiger symbols in Tiger Fortune are iconic. fortune tiger demo
Medicament information. Brand names.
get generic zithromax for sale
All about medicines. Get here.
купить диплом вуза с занесением в реестр [url=https://diplomys-vsem.ru/]купить диплом вуза с занесением в реестр[/url] .
Online casino bonuses are tricky—check the terms! vai de bet
Tiger Fortune’s spins keep me on my toes. fortune tiger
Для эффективного продвижения по карьере необходимо наличие диплома ВУЗа. Приобрести диплом об образовании у проверенной компании: [url=http://diplomt-v-chelyabinske.ru/diplom-kupit-ofitsialnij/]diplomt-v-chelyabinske.ru/diplom-kupit-ofitsialnij/[/url]
where buy cheap co-amoxiclav pills
order generic rizact pills
The graphics in Tiger Fortune are absolutely stunning. fortune tiger 777
Pills information for patients. Generic Name.
warfarin doxycycline interaction
Actual what you want to know about medicament. Get here.
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – идеальный вариант для вас.
Наши товары:
Латунная квадратная профильная труба 10С…10С…1 РјРј ЛС 59-1 РўРЈ 48-21-428-84 Приобретите латунные квадратные трубы РѕС‚ производителя РЅР° Редметсплав.СЂС„. РЁРёСЂРѕРєРёР№ выбор, высокое качество, устойчивость Рє РєРѕСЂСЂРѕР·РёРё Рё простота монтажа – РІСЃРµ это делает наши трубы незаменимым решением для ваших проектов.
https://telegra.ph/%EB%B9%84%EC%95%84%EA%B7%B8%EB%9D%BC%EC%99%80-%EB%8B%A4%EB%A5%B8-%EB%B0%9C%EA%B8%B0%EB%B6%80%EC%A0%84-%EC%95%BD%EB%AC%BC%EC%8B%9C%EC%95%8C%EB%A6%AC%EC%8A%A4-%EB%A0%88%EB%B9%84%ED%8A%B8%EB%9D%BC%EC%9D%98-%EC%B0%A8%EC%9D%B4%EC%A0%90%EC%9D%80-%EB%AC%B4%EC%97%87%EC%9D%B8%EA%B0%80%EC%9A%94-02-11
Medicament information for patients. Brand names.
can you get cheap pulmicort no prescription
Some about pills. Read information now.
Mobile phone numbers in South Korea also follow a specific structure. South Korean mobile phone numbers usually start with 010 and consist of 11 digits in total https://diariodeavisos.elespanol.com/canariasenred/paypal-sin-numero-de-telefono-una-solucion-virtual-y-segura/
This casino’s Tiger Fortune game is incredible. jogo do tigrinho demo
The Tiger Fortune game runs so smoothly on my phone. tiger fortune
Platform Slot Online dengan Fitur Terbaik dan Kemenangan Terjamin, hanya di SIGMASLOT
РедМетСплав предлагает широкий ассортимент высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица изделия подтверждена соответствующими документами, подтверждающими их происхождение. Опытная поддержка – наш стандарт – мы на связи, чтобы улаживать ваши вопросы по мере того как предоставлять решения под специфику вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
Наша продукция:
РљСЂСѓРі магниевый МЦр1Рќ3 – ГОСТ 2581-78 Фольга магниевая МЦр1Рќ3 – ГОСТ 2581-78 представляет СЃРѕР±РѕР№ высококачественный материал, обладающий отличными антикоррозийными свойствами Рё высокой пластичностью. РћРЅР° применяется РІ различных отраслях, включая авиацию Рё электронику. Данная фольга легко поддается обработке, что делает ее идеальным выбором для создания легких конструкций Рё деталей. Если вам необходим надежный Рё долговечный материал, обратите внимание РЅР° этот РїСЂРѕРґСѓРєС‚. РќРµ упустите возможность купить Фольга магниевая МЦр1Рќ3 – ГОСТ 2581-78 Рё обеспечить себя качеством!
Tiger Fortune is the king of slots here! jogo do tigrinho demo
[url=https://https-kra31.at]кракен войти[/url] – Kra31.at, kra31.cc
Online slots are my guilty pleasure—those animations are top-notch! fishin frenzy
Really enjoyed this! Your writing style is so engaging.
Отопление и водоснабжение – компания, которая предоставляет профессиональные услуги. Компетентные специалисты готовы осуществить в Рузе в сжатые сроки монтаж системы отопления. Создайте тепло и комфорт в вашем доме с нами. Ищете монтаж систем отопления? Ruzskiy-rayon.santex-uslugi.ru – тут можете уже сегодня с отзывами о нас ознакомиться. Гарантируем применение материалов высокого качества. Готовы превзойти ваши ожидания. Стараемся сделать услуги доступными для клиентов. Позвоните нашему менеджеру для консультации по интересующим вас вопросам.
mostber [url=https://www.mostbet6033.ru]https://www.mostbet6033.ru[/url] .
try this web-site [url=https://themerr-ll-lynch.com/]merrill edge[/url]
Всегда считал, что покупка диплома о высшем образовании — это миф. Но, оказалось, что все возможно! Сначала искал информацию по теме: купить диплом об окончании, купить диплом медицинский, купить диплом училища, можно купить аттестат, гознак купить аттестаты, а затем перешел на дипломы вузов. Подробнее можно узнать здесь: [url=http://diplomybox.com/kupit-diplom-o-vysshem-obrazovanii-saratov/]diplomybox.com/kupit-diplom-o-vysshem-obrazovanii-saratov[/url]
Jetton Casino pleases its users with a variety of bonuses. For newcomers, there are welcome packages, including deposit incentives and free spins Джеттон Казино зеркало
can you buy cheap lisinopril without prescription
[url=https://kra–31.at]kraken вход[/url] – kra cc, kra31 at
[url=https://kra–31.cc]kraken зеркало[/url] – kra31, kraken shop
Medicine information for patients. Short-Term Effects.
where can i get cheap valtrex without insurance
Actual news about medication. Get information now.
Tiger Fortune has some of the best bonus rounds I’ve seen. tigrinho demo
Online casinos should limit daily losses for safety. doradobet
The tiger symbols in Tiger Fortune are iconic. tiger fortune
This gave me a lot to think about—thank you for sharing your thoughts!
F*ckin’ amazing issues here. I am very glad to see your article. Thank you a lot and i’m looking forward to contact you. Will you kindly drop me a mail?
Clear, concise, and super helpful. Great job!”
Tiger Fortune has some of the best bonus rounds I’ve seen. tigrinho demo
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наша продукция:
Гранулы ниобия 4N
Пороги автоматического типа являются идеальным вариантом при условии, что осуществить монтажные работы стационарного не удастся либо нежелательно. В данный момент можно изучить каталог всех вариантов, которые предлагает предприятие «SMARTПОРОГ», что позволит подобрать решение, которое определенно подойдет. https://smartporog.ru/ – на сайте изучите преимущества, которые вы получите, если установите такие конструкции в помещении. Такие конструкции потребуются с той целью, чтобы в помещение не попадали солнечные лучи, посторонние запахи. Считается отличным вариантом для организации ванной комнаты, например.
I won $600 on a slot—hype high! aviator
Академия «Арчибальд» занимается обучением грумингу. Вы можете совершенствовать собственные навыки на живых моделях до бесконечности. Преподаватели на все вопросы отвечают. Дарим видеолекции, методические материалы и учебники. Погрузитесь в атмосферу, с которой не захочется расставаться. Ищете курс груминга в москве? Grooming-dream.ru – тут реальные отзывы клиентов представлены. Мы выпускаем отменных груминг-специалистов. Предлагаем инструменты в период обучения. Если вам интересна новая профессия – Арчибальд, вот то место, которое вам подойдет.
Drugs prescribing information. Long-Term Effects.
buying glucophage pills
Best trends of medicines. Get information now.
Купить диплом ВУЗа по невысокой цене вы можете, обратившись к надежной специализированной фирме. Купить документ о получении высшего образования вы можете у нас. [url=http://diplom4you.com/kupite-diplom-s-reestrom-bistro-i-bez-zabot/]diplom4you.com/kupite-diplom-s-reestrom-bistro-i-bez-zabot[/url]
see post [url=https://themerr-ll-lynch.com]merrill edge login[/url]
This online casino shines with Tiger Fortune. jogo do tigrinho demo
can you get cheap advair diskus prices
Sign Up at yako and Get a $100
Bonus Instantly!
оценка швейцарских часов в ломбарде [url=https://lombard-chasov1.ru]https://lombard-chasov1.ru[/url] .
скачать mostbet на телефон [url=https://mostbet6033.ru]https://mostbet6033.ru[/url] .
перепланировка это [url=udmageo.ru]перепланировка это[/url] .
стоматологическая клиника митино [url=http://stomatologiya-mitino1.ru]стоматологическая клиника митино[/url] .
Корпоративные сувениры и мерч являются эффективным инструментом для повышения узнаваемости бренда и укрепления корпоративной культуры. Они помогают создать положительное впечатление о компании среди клиентов и партнеров, а также способствуют формированию лояльности сотрудников. Подробнее об этом можно узнать по ссылке: https://moda-sar.ru/04/04/2025/korporativnye-suveniry-i-merch.html
Always a pleasure reading your blog. Keep the great content coming!
https://forum.prosochi.ru/topic49161.html
Для защиты помещений от пожара необходимо использовать специализированные решения. [url=https://emercom-irk.ru/protivopozharnoe-oborudovanie]Противопожарное оборудование[/url] включает в себя системы сигнализации, датчики, огнетушители и многое другое. Правильный подбор и монтаж этих средств — залог вашей безопасности и спокойствия.
Claim Your $100 Bonus with a Quick Registration at
leon!
The sound design in online slots is so immersive! sugar rush
Заказ значков через интернет — это современный и удобный способ получить уникальные аксессуары, не выходя из дома. Онлайн-платформы предлагают широкий выбор дизайнов, материалов и вариантов персонализации, позволяя создать значки, полностью соответствующие вашим предпочтениям и потребностям. Дополнительную информацию о процессе заказа и доступных опциях можно найти по ссылке:https://aragoncom.ru/poleznie/zakaz-znachkov-cherez-sajt-udobnyj-sposob-oformit-unikalnye-aksessuary.html
На сайте https://officepro54.ru представлено огромное количество мебели, которая идеально подходит для организации офисного пространства. Вся она выполнена из инновационных, уникальных материалов, а потому прослужит очень долго, не синтезирует в воздух опасных веществ. В разделе вы найдете кабинет руководителя, функциональные и вместительные столы для переговоров, шкафы-купе, сейфы, акустические системы, ученическую мебель и многое другое. Постоянно действуют акции. На продукцию установлены привлекательные цены.
The Tiger Fortune game runs so smoothly on my phone. fortune tiger demo gratis
Meds prescribing information. Effects of Drug Abuse.
buspirone side effects wear off
All what you want to know about drug. Get now.
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с прекрасным качеством обслуживания.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
Наши товары:
Прецизионная сварная Рё холоднокалиброванная труба 30С…2 РјРј E355 EN 10305-3 Выбирайте прецизионные сварные Рё холоднокалиброванные трубы РЅР° Редметсплав.СЂС„. Надежные Рё высококачественные трубы СЃ уникальными характеристиками Рё высокой точностью изготовления. Рдеальный выбор для машиностроения, авиации Рё энергетики.
Скин M4A4 Howl cs2-get-skins.ru один из самых дорогих и загадочных в CS:GO. Запрещённый артефакт с историей. Рассказываем о его происхождении, внешнем виде и значении в мире скинов.
Перчатки спецназа case-simulator-cs.ru тактический скин в CS:GO с брутальным дизайном и премиальной редкостью. Узнайте об их разновидностях, цене, коллекционной ценности и лучших сочетаниях с другими скинами.
Заказать диплом университета. Заказ документа о высшем образовании через надежную фирму дарит массу преимуществ. Данное решение позволяет сберечь как личное время, так и серьезные финансовые средства. [url=http://unmeinaroom.copiny.com/question/details/id/1083783/]unmeinaroom.copiny.com/question/details/id/1083783[/url]
Легендарная AWP Medusa case-simulator-cs2 скин с таинственным дизайном, вдохновлённым древнегреческой мифологией. Высокая редкость, художественная детализация и престиж на каждом сервере.
https://www.floristic.ru/forum/groups/moskva-d889-alyuminievye-konstrukcii-pod-klyuch.html#gmessage1374
StatTrak M4A1-S https://cs2-get-skin.ru скин с возможностью отслеживания фрагов прямо на корпусе оружия. Узнайте, какие модели доступны, чем отличаются, и какие из них ценятся выше всего на рынке CS:GO.
Thanks for the great read! Very informative and well-written.
This casino’s Tiger Fortune game is a blast. tiger fortune
Компания «Отопление и водоснабжение» специализируется на предоставлении высокого качества услуг. У нас глубокие знания и опыт в сфере монтажа отопительных систем. Следим за современными технологиями, чтобы вам передовые решения предлагать. Подходим персонально к каждому проекту. Ищете монтаж систем отопления? Krasnogorsk.santex-uslugi.ru – сайт, где указаны цены и размещены отзывы о нас. Используем проверенные материалы и оборудование от известных производителей. Удачно осуществили множество работ. Готовы комфортный дом для вашей семьи создать.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние достижения.
Наша продукция:
Керамическая мишень Limn2O4 (3N)
can i purchase generic adalat without dr prescription
скачать mostbet на телефон [url=http://mostbet6034.ru]http://mostbet6034.ru[/url] .
РедМетСплав предлагает внушительный каталог высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица изделия подтверждена всеми необходимыми документами, подтверждающими их происхождение. Опытная поддержка – то, чем мы гордимся – мы на связи, чтобы разрешать ваши вопросы а также находить ответы под требования вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наша продукция:
Магний MAG 8 – BS 2970 Магний MAG 8 – BS 2970 – это высококачественная добавка для поддержания Р·РґРѕСЂРѕРІСЊСЏ. РћРЅ способствует улучшению обмена веществ, помогает снизить уровень стресса Рё поддерживает нормальную работу нервной системы. РџСЂРѕРґСѓРєС‚ идеально РїРѕРґС…РѕРґРёС‚ для людей, ведущих активный образ Р¶РёР·РЅРё, Рё тех, кто нуждается РІ восстановлении после тренировок. Купить Магний MAG 8 – BS 2970 значит позаботиться Рѕ своем Р·РґРѕСЂРѕРІСЊРµ Рё активности. РЎ его помощью РІС‹ сможете повысить жизненный тонус Рё улучшить общее самочувствие. РќРµ упустите возможность добавить его РІ СЃРІРѕР№ рацион!
where can i get cheap tizanidine tablets
[url=https://kra–31.cc]Kra30.cc[/url] – Kra30.cc, kraken вход
This casino’s Tiger Fortune game is a winner. fortune tiger 777
[url=https://kra–31.at]кракен магазин[/url] – kraken войти, кракен войти
швейцарские часы скупка москва [url=www.lombard-chasov1.ru/]швейцарские часы скупка москва[/url] .
как узаконить перепланировку [url=https://udmageo.ru/]как узаконить перепланировку[/url] .
клиника митино [url=www.stomatologiya-mitino1.ru/]клиника митино[/url] .
Nowadays, international communication is of great importance in both personal and business life. Especially in a country like South Korea, which is important in terms of technology and trade, communicating starts with knowing the correct phone numbers and codes https://www.on-magazine.co.uk/stuff/tech/enhance-your-whatsapp-privacy-with-temporary-virtual-numbers
[url=https://https-kra31.at]kraken войти[/url] – кракен официальная ссылка, Kra30.cc
детективное агентство экспертного уровня, при этом мы гарантируем одинаково внимательный подход как корпоративным клиентам, так и частным лицам Нанять детектива
Drug information for patients. Generic Name.
can i order verapamil without prescription
Everything what you want to know about meds. Get information now.
Заказ значков через интернет — это современный и удобный способ получить уникальные аксессуары, не выходя из дома. Онлайн-платформы предлагают широкий выбор дизайнов, материалов и вариантов персонализации, позволяя создать значки, полностью соответствующие вашим предпочтениям и потребностям. Дополнительную информацию о процессе заказа и доступных опциях можно найти по ссылке:https://aragoncom.ru/poleznie/zakaz-znachkov-cherez-sajt-udobnyj-sposob-oformit-unikalnye-aksessuary.html
Really helpful content — I learned a lot from this!
This online casino nailed Tiger Fortune’s theme. jogo do tigrinho demo
horoshobet promo code To maximize the potential of your welcome bonus, you must wager the entire amount on either sports betting or casino games within a 30-day timeframe
[url=https://https-kra31.at/]kraken сайт[/url] – кракен открыть, кракен официальный сайт
I can play Tiger Fortune all day and not get bored. fortune tiger 777
Заказ значков через интернет — это современный и удобный способ получить уникальные аксессуары, не выходя из дома. Онлайн-платформы предлагают широкий выбор дизайнов, материалов и вариантов персонализации, позволяя создать значки, полностью соответствующие вашим предпочтениям и потребностям. Дополнительную информацию о процессе заказа и доступных опциях можно найти по ссылке:https://aragoncom.ru/poleznie/zakaz-znachkov-cherez-sajt-udobnyj-sposob-oformit-unikalnye-aksessuary.html
The graphics in Tiger Fortune are absolutely stunning. jogo do tigrinho demo
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с высоким уровнем сервиса.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – наилучшее решение для вашего бизнеса.
Наши товары:
Титановая труба 65х0.6х5000 мм ОТ4-1 ГОСТ 22897-86 Откройте мир прочных и надежных титановых труб с компанией Редметсплав. Наши высококачественные титановые трубы сочетают в себе устойчивость к коррозии, износостойкость и легкий вес, делая их незаменимым материалом для применения в различных сферах промышленности. Широкий выбор различных диаметров и толщин, а также индивидуальные решения для вашего производства. Гарантированное качество и надежность поставок.
The tiger roars in Tiger Fortune are epic. tigrinho demo
I wish online tied more—life in! plinko
Заказ значков через интернет — это современный и удобный способ получить уникальные аксессуары, не выходя из дома. Онлайн-платформы предлагают широкий выбор дизайнов, материалов и вариантов персонализации, позволяя создать значки, полностью соответствующие вашим предпочтениям и потребностям. Дополнительную информацию о процессе заказа и доступных опциях можно найти по ссылке:https://aragoncom.ru/poleznie/zakaz-znachkov-cherez-sajt-udobnyj-sposob-oformit-unikalnye-aksessuary.html
AWP Dragon Lore http://get-skins-csgo.ru легендарный скин с изображением дракона, символ элиты в CS:GO. Узнайте о его происхождении, редкости, стоимости и почему он стал мечтой каждого коллекционера.
Обзор AK-47 Redline http://csgo-get-skin.ru лаконичный дизайн, спортивный стиль и привлекательная цена. Почему этот скин так любят игроки и с чем он лучше всего сочетается — читайте у нас.
Обзор AWP Asiimov get-skin-csgo.ru футуристичный скин с дерзким дизайном. Рассказываем о редкости, ценах, вариантах износа и том, почему Asiimov стал иконой среди скинов в CS:GO.
Онлайн-казино Shot https://shot-casino-apk.ru предлагает щедрые бонусы, турнирные события и топовые слоты. Узнайте об условиях игры, уровне доверия, лицензии и особенностях платформы в нашем свежем обзоре.
Доброго времени суток!
Для некоторых людей, заказать [b]диплом[/b] о высшем образовании – это необходимость, возможность получить отличную работу. Впрочем для кого-то – это желание не терять огромное количество времени на учебу в ВУЗе. Что бы ни толкнуло вас на такой шаг, мы готовы помочь вам. Максимально быстро, качественно и недорого изготовим документ любого ВУЗа и любого года выпуска на настоящих бланках с реальными подписями и печатями.
Ключевая причина, почему многие покупают дипломы, – желание занять определенную должность. Предположим, знания позволяют специалисту устроиться на привлекательную работу, но документального подтверждения квалификации не имеется. В случае если работодателю важно присутствие “корочки”, риск потерять место работы довольно высокий.
Приобрести документ о получении высшего образования можно у нас в столице. Мы предлагаем документы об окончании любых университетов РФ. Вы сможете получить диплом по любым специальностям, любого года выпуска, включая документы СССР. Даем 100% гарантию, что в случае проверки документов работодателем, каких-либо подозрений не возникнет.
Разных ситуаций, которые вынуждают купить диплом достаточно. Кому-то очень срочно нужна работа, а значит, нужно произвести особое впечатление на начальство во время собеседования. Некоторые задумали попасть в престижную компанию, для того, чтобы повысить собственный статус и в дальнейшем начать собственное дело. Чтобы не тратить массу времени, а сразу начинать успешную карьеру, применяя врожденные таланты и полученные знания, можно приобрести диплом в интернете. Вы сможете стать полезным в социуме, получите финансовую стабильность в максимально короткие сроки- [url=http://rdiploms.com/]аттестат купить за 9 класс[/url]
I spent hours playing Tiger Fortune last night – no regrets! tiger fortune
РедМетСплав предлагает обширный выбор высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы обеспечиваем оперативное исполнение вашего заказа.
Каждая единица изделия подтверждена всеми необходимыми документами, подтверждающими их качество. Опытная поддержка – наш стандарт – мы на связи, чтобы разрешать ваши вопросы а также адаптировать решения под требования вашего бизнеса.
Доверьте вашу потребность в редких металлах специалистам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наша продукция:
Рзделия РёР· висмута C49300 – UNS Рзделия РёР· висмута C49300 – UNS это высококачественные материалы, предназначенные для применения РІ различных отраслях. Р’РёСЃРјСѓС‚ отличается отличной РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью Рё устойчивостью Рє высоким температурам, что делает его идеальным выбором для электронной Рё аэрокосмической промышленности. РџСЂРё РїРѕРєСѓРїРєРµ изделий РёР· висмута C49300 – UNS РІС‹ получаете надежный РїСЂРѕРґСѓРєС‚, который отвечает современным стандартам качества. РњС‹ предлагаем вам купить Рзделия РёР· висмута C49300 – UNS РїРѕ выгодной цене. Вам больше РЅРµ придется беспокоиться Рѕ замене или ремонте, выбирая наши изделия, РІС‹ инвестируете РІ долговечность.
I love the high-energy feel of Tiger Fortune! tiger fortune
Drugs information. Brand names.
metronidazole for fish safe for humans
Best news about meds. Read information now.
Drug prescribing information. Generic Name.
buying cheap thorazine without rx
Best trends of medicines. Get information now.
Блог о маркетплейсах «OOWA» предлагает ознакомиться с актуальной, интересной и познавательной информацией, которая касается удачных продаж на маркетплейсах, обзоров товаров и услуг. На портале регулярно публикуются ценные и содержательные рекомендации от экспертов, которые помогут начать лучше разбираться в тонкостях такого бизнеса. Ищете обзор ультрабука huawei matebook d 14 2023? На oowa.ru регулярно публикуются ценные и содержательные рекомендации от экспертов, которые помогут начать лучше разбираться в тонкостях такого бизнеса. Почитайте о самых востребованных товарах с маркетплейсов.
[url=https://lucky-jet-igrat.com/]lucky jet[/url] – лаки джет официальный, лаки джет официальный
мостюет [url=www.mostbet6034.ru]www.mostbet6034.ru[/url] .
Заказать диплом возможно через сайт компании. [url=http://pesnibardov.ru/f/ucp.phpmode=login/]pesnibardov.ru/f/ucp.phpmode=login[/url]
Online casinos need signs—watch out! super hot fruits
выкуп часов ломбард [url=https://lombard-chasov1.ru]выкуп часов ломбард[/url] .
стоматолог митино [url=www.stomatologiya-mitino1.ru/]стоматолог митино[/url] .
узаконивание перепланировки [url=https://udmageo.ru/]udmageo.ru[/url] .
New Users, Register at slotv and
Get a $100 Welcome Bonus
can you get cheap macrobid
Заказать диплом о высшем образовании. Покупка подходящего диплома через качественную и надежную компанию дарит массу плюсов для покупателя. Такое решение дает возможность сэкономить время и серьезные денежные средства. [url=http://amigabrasileira.listbb.ru/viewtopic.php?f=52&t=2954/]amigabrasileira.listbb.ru/viewtopic.php?f=52&t=2954[/url]
Нужно создание или разработка студия по продвижению сайтов: адаптивный дизайн, SEO-продвижение, техническая поддержка. Эффективное ключевая фраза для вашего бизнеса — привлечение клиентов и рост прибыли.
buy isoptin tablets
Обзор онлайн-казино Shot https://shot-casino-app.ru плюсы и минусы, проверка лицензии, акции и фриспины. Рассказываем, стоит ли играть, как получить бонусы и выводить выигрыши без проблем.
Shot Casino https://shot-casino-online.ru новое онлайн-казино с лицензией, защитой данных и большим выбором игр. В обзоре: безопасность, отзывы игроков, бонусы и выплаты. Надёжная площадка для вашего азарта.
explanation [url=https://jaxxblog.io]jaxx liberty wallet[/url]
Онлайн-казино Calibry https://calibri-casino-online.ru новое игровое пространство с лицензией, щедрыми бонусами и широким выбором слотов. Читайте обзор: особенности платформы, условия игры, отзывы и выплаты.
The music in Tiger Fortune sets the perfect mood. jogo do tigrinho demo
[url=https://lucky-jet-igrat.com]лаки джет официальный[/url] – лаки джет 1win, лаки джет официальный сайт
Tiger Fortune’s theme is wild and totally unique. tigrinho demo
МосСтройАльянс специалисты компании готовы решить задачу любой сложности. Мы на осуществлении фасадных, уборочных и кровельных работ специализируемся. Предлагаем самые выгодные цены. Вы будете довольны результатом. Ищете стоимость монтажа металлочерепицы за м2? Mos-stroi-alians.ru – тут с отзывами наших клиентов можете ознакомиться. Для работы применяем исключительно материалы высшего качества. Ваше время мы ценим. Несем ответственность за соблюдение сроков. Свяжитесь с нами по указанному на сайте телефону. Проконсультируем и ответим на интересующие вас вопросы.
Medicine information for patients. Generic Name.
can you get aricept no prescription
Best about medication. Read information now.
[url=https://kra–31.at]Kra31.cc[/url] – кракен войти, кракен зеркало
Medication information. Drug Class.
how to get coreg for sale
Some news about medicine. Get here.
[url=https://kra–31.cc/]кракен магазин[/url] – kra30 сс, kraken войти
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наши товары:
Плоская мишень Sio для распыления
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наши товары:
Вращающаяся мишень для распыления вольфрама
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым текущие трудности превращаются в завтрашние возможности.
Наши товары:
Металлическая мишень Gd 3N плоская
buying cheap primaquine without prescription
мостбет казино войти [url=https://www.mostbet6034.ru]https://www.mostbet6034.ru[/url] .
how can i get myambutol without prescription
Мы можем предложить дипломы любой профессии по выгодным тарифам.– [url=http://vacshidiplom.com/kupit-diplom-s-zaneseniem-v-reestr-bistro-i-nadezhno-15/]vacshidiplom.com/kupit-diplom-s-zaneseniem-v-reestr-bistro-i-nadezhno-15/[/url]
Tiger Fortune is the best game here, hands down! fortune tiger demo gratis
На сайте https://pilmi.ru вы найдете огромное количество фильмов самых разных жанров, включая комедии, мелодрамы, драмы, романтические. Для того чтобы подыскать наиболее подходящий вариант, нужно воспользоваться специальным каталогом. Вашему вниманию представлены и ожидаемые любопытные новинки этого года, которые произведут эффект. Все это можно просматривать в любое время и на различных устройствах. Прямо сейчас воспользуйтесь возможностью посмотреть азиатское кино. Используйте поиск для облегчения выбора.
Meds information. Drug Class.
cost trileptal without rx
Everything information about medicine. Read information now.
Medicine information for patients. Long-Term Effects.
lyrica pills to buy online
Everything what you want to know about pills. Get information here.
Мы предлагаем дипломы любой профессии по невысоким тарифам.
Вы приобретаете документ в надежной и проверенной временем компании. Купить диплом ВУЗа– [url=http://vv.flybb.ru/viewtopic.phpf=23&t=2837/]vv.flybb.ru/viewtopic.phpf=23&t=2837[/url]
Tiger Fortune’s graphics are wild and vivid. tiger fortune
Really helpful content – thanks for putting this together!
The sound design in Tiger Fortune is amazing. fortune tiger bet
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с высоким уровнем сервиса.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – идеальный вариант для вас.
Наша продукция:
Медный тройник РїРѕРґ пайку СЃ направленным отводом 22С…18С…0.9 РјРј 8С…10 РјРј твердая пайка Рњ3С‚ ГОСТ Р 52922-2008 Купить качественные медные тройники РїРѕРґ пайку для надежных Рё долговечных соединений РІ трубопроводных системах. Разнообразие размеров Рё конфигураций, высокая теплопроводность Рё устойчивость Рє РєРѕСЂСЂРѕР·РёРё. Простая установка Рё надежность соединения. Рдеальное решение для различных проектов. Редметсплав.СЂС„
Купить диплом о высшем образовании!
Мы можем предложить дипломы любой профессии по приятным тарифам. Вы покупаете диплом через надежную фирму. : [url=http://rodina.listbb.ru/viewtopic.php?f=3&t=2348/]rodina.listbb.ru/viewtopic.php?f=3&t=2348[/url]
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
Наша продукция:
Медная однораструбная редукционная переходная муфта РїРѕРґ пайку удлиненная 54С…40.5 РјРј твердая пайка Рњ3Рњ ГОСТ Р 52922-2008 Приобретите высококачественные медные однораструбные редукционные муфты РїРѕРґ пайку РѕС‚ Редметсплав для надежного соединения трубопроводов. Гарантированная прочность Рё устойчивость Рє РєРѕСЂСЂРѕР·РёРё. Рдеальны для различных систем отопления, водоснабжения Рё прочих технических целей.
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с прекрасным качеством обслуживания.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – наилучшее решение для вашего бизнеса.
Наши товары:
Однораструбный медный РѕР±РІРѕРґ РїРѕРґ пайку 76.1С…65С…1.6 РјРј 12С…15 РјРј твердая пайка Рњ3 ГОСТ Р 52922-2008 Выбирайте высококачественные медные однораструбные РѕР±РІРѕРґС‹ РїРѕРґ пайку для надежных соединений. Рзготовлены РёР· меди высокой чистоты СЃ отличной теплопроводностью Рё устойчивостью Рє РєРѕСЂСЂРѕР·РёРё. Рдеальны для систем водоснабжения Рё отопления.
prednisone short burst guidelines
where to buy generic betnovate pills
мостбет мобильная версия скачать [url=www.mostbet6033.ru]мостбет мобильная версия скачать[/url] .
Medicament information sheet. Short-Term Effects.
where buy cheap clomid pills
Actual news about medication. Read information now.
мосбет казино [url=https://mostbet6033.ru/]https://mostbet6033.ru/[/url] .
Заказать диплом университета!
Мы предлагаем документы ВУЗов, расположенных в любом регионе России.
[url=http://good-diplom.ru/kupit-diplom-magistra-s-provodkoj-2/]good-diplom.ru/kupit-diplom-magistra-s-provodkoj-2/[/url]
Drugs information for patients. What side effects can this medication cause?
how to get cheap colchicine without prescription
Some what you want to know about medication. Get here.
Вместе с t.me/azino777_a у вас есть возможность в увлекательное путешествие по миру азартных игр отправиться. Предлагаем персонализированные предложения и уникальные бонусы. Расскажем, как начать играть в Азино. Вас ждут невероятные эмоции и возможность выиграть крупный приз. Не упустите шанс испытать свою удачу! Ищете azino777 официальный бонусы? – здесь представлено много интересной информации. Собрали самые частые вопросы от игроков. Рады вам помочь. Приготовьтесь к увлекательным турнирам в Азино. Участвуйте и боритесь за призы с другими игроками!
Tiger Fortune keeps the good times rolling! jogo do tigrinho demo
РедМетСплав предлагает внушительный каталог качественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица продукции подтверждена всеми необходимыми документами, подтверждающими их качество. Опытная поддержка – то, чем мы гордимся – мы на связи, чтобы ответить на ваши вопросы по мере того как находить ответы под особенности вашего бизнеса.
Доверьте вашу потребность в редких металлах специалистам РедМетСплав и убедитесь в множестве наших преимуществ
Наши товары:
Пруток висмутовый C89535 – UNS Пруток висмутовый C89535 – UNS является высококачественным материалом, идеально подходящим для различных промышленных применений. РћРЅ обладает отличной устойчивостью Рє РєРѕСЂСЂРѕР·РёРё Рё высокой теплопроводностью, что делает его незаменимым РІ электронике Рё медицине. Данный пруток может быть использован для создания сплавов, Р° также РІ качестве компонента РІ проведении научных исследований. РќРµ упустите возможность купить Пруток висмутовый C89535 – UNS Рё обеспечить надежность ваших проектов СЃ помощью этого уникального материала, который сочетает РІ себе качество Рё доступность.
РедМетСплав предлагает широкий ассортимент отборных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица товара подтверждена всеми необходимыми документами, подтверждающими их происхождение. Дружелюбная помощь – то, чем мы гордимся – мы на связи, чтобы улаживать ваши вопросы по мере того как находить ответы под специфику вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
Наши товары:
РљСЂСѓРі висмутовый C89831 – CDA РљСЂСѓРі висмутовый C89831 – CDA является высококачественным материалом для профессионального использования. Ртот РєСЂСѓРі обеспечивает отличную прочность Рё долговечность, что делает его идеальным для разнообразных проектов, требующих надежного Рё устойчивого инструмента. Приобретая РљСЂСѓРі висмутовый C89831 – CDA, РІС‹ получаете гарантированное качество Рё эффективность работ. РћРЅ РїРѕРґС…РѕРґРёС‚ как для промышленных, так Рё для бытовых РЅСѓР¶Рґ. РќРµ упустите возможность купить РљСЂСѓРі висмутовый C89831 – CDA Рё убедиться РІ его превосходных характеристиках.
подключить интернет
domashij-internet-chelyabinsk001.ru
подключить проводной интернет челябинск
РедМетСплав предлагает обширный выбор качественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица продукции подтверждена всеми необходимыми документами, подтверждающими их происхождение. Дружелюбная помощь – наш стандарт – мы на связи, чтобы ответить на ваши вопросы а также предоставлять решения под требования вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
Наша продукция:
Магний ZK60 – CSA HG.5 Магний ZK60 – CSA HG.5 является идеальным выбором для различных промышленных применения благодаря своей высокой прочности Рё легкости. Ртот легкий сплав особенно популярен РІ аэрокосмической Рё автомобильной отраслях, РіРґРµ важна эффективность Рё надежность. Уникальные характеристики сделали его незаменимым материалом для РјРЅРѕРіРёС… проектов. Повысьте производительность СЃРІРѕРёС… изделий, выбрав именно этот сплав. РќРµ упустите возможность купить Магний ZK60 – CSA HG.5 Рё получайте максимальную выгоду РѕС‚ инновационного материала, который превосходит аналоги.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
С широким ассортиментом продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наши товары:
Гранулы оксида циркония высокой чистоты
Tiger Fortune’s animations are next-level awesome. fortune tiger demo
мостбет зеркало [url=mostbet6035.ru]мостбет зеркало[/url] .
Alkomarket-dubai.ru коллекцию алкогольных напитков на разный кошелек и вкус предлагает. Наш основной приоритет – качество продукции, мы о здоровье своих клиентов заботимся. Закажите на дом уже сейчас алкоголь с доставкой. Наслаждайтесь удобством! Ищете купить алкоголь в дубае c доставкой? Alkomarket-dubai.ru – тут большой выбор вина, скотча, водки и виски, а также иных премиальных напитков от популярных мировых брендов. Бережно относимся к покупателям, заботясь об их потребностях. Поможем для вас и на подарок близким выбрать достойные варианты.
The payout waits online lag—push it! plinko
I hit a bonus round in Tiger Fortune today – wow! fortune tiger demo gratis
Нужен бесплатный аккаунт? аккаунт стим бесплатно Узнайте, где можно получить рабочие логины с играми, как не попасть на фейк и на что обратить внимание при использовании таких аккаунтов.
Онлайн-казино Aura aura-casino-apk.ru/ лицензия, быстрые выплаты, слоты от топ-провайдеров и щедрые бонусы. Подробный обзор платформы: интерфейс, регистрация, акции, безопасность и отзывы игроков.
Начните с Aura Casino aura casino bonus удобное онлайн-казино с простым интерфейсом, стартовыми бонусами и быстрой регистрацией. Рассказываем, как получить фриспины и не потеряться в слотах.
The casino’s Tiger Fortune slot has great winning potential. fortune tiger demo gratis
Выгодно заказать диплом о высшем образовании. Приобретение диплома ВУЗа через проверенную и надежную компанию дарит множество достоинств. Такое решение позволяет сберечь время и серьезные финансовые средства. [url=http://careers-old.farcapital.com.my/employer/eonline-diploma/]careers-old.farcapital.com.my/employer/eonline-diploma[/url]
Онлайн-казино Calibry http://calibri-casino-play.ru бонусы без депозита, быстрые выводы и лицензированный софт. В обзоре: как начать играть, получить фриспины, использовать акции и избежать рисков.
Tiger Fortune’s features are super fun. tigrinho demo
Medicine information leaflet. Drug Class.
finasteride farmacia meritxell
Some about medicine. Read information now.
[b]Диплом любого университета Российской Федерации![/b]
Без ВУЗа достаточно сложно было продвигаться вверх по карьерной лестнице. Именно из-за этого решение о заказе диплома стоит считать выгодным и целесообразным. Купить диплом об образовании [url=http://dolphinplacements.com/companies/gosznac-diplom-24/]dolphinplacements.com/companies/gosznac-diplom-24[/url]
Meds prescribing information. Effects of Drug Abuse.
cheap linezolid no prescription
Best trends of medicines. Read information now.
cost of generic sildalist without prescription
buying finpecia prices
Где купить диплом специалиста?
Мы изготавливаем дипломы любых профессий по невысоким ценам. Важно, чтобы документы были доступными для большого количества граждан. Приобрести диплом об образовании [url=http://kupitediplom.ru/kupit-diplom-s-zaneseniem-v-reestr-na-forume-6/]kupitediplom.ru/kupit-diplom-s-zaneseniem-v-reestr-na-forume-6/[/url]
mostbet официальный сайт [url=mostbet6033.ru]mostbet6033.ru[/url] .
have a peek at this site [url=https://themerr-ll-lynch.com]mymerrill login[/url]
I can’t stop playing Tiger Fortune – it’s addictive! fortune tiger demo gratis
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
Наши товары:
Пружина из драгоценных металлов серебряная 50х8х0.8 мм Ср99.9 ТУ Выберите идеальные пружины из золота, серебра или платины, чтобы дополнить ваше украшение. Найдите пружины с изысканным дизайном и прочностью. Они предлагают широкий выбор для того, чтобы подчеркнуть уникальность вашего украшения.
недорогой интернет челябинск
domashij-internet-chelyabinsk002.ru
подключение интернета челябинск
The Tiger Fortune game is so easy to play. tigrinho demo
Устал только играть в CS2 и иногда хочется зайти в рулекти кс 2 и попытать удачу или математический расчет. Отличная подборка рулеток кс 2 и ксго на вечер с проверенными отзывами и выплатами.
Официальный сайт казино Monro. Регистрация и вход в личный кабинет. В этом казино разрешается играть без регистрации, если использовать демо-режим monro казино официальный сайт
Online casino ads are relentless—enough already! plinko
Medicine information leaflet. Short-Term Effects.
how to get cheap dilantin without dr prescription
Everything trends of pills. Get here.
Medicines information leaflet. Cautions.
can i buy sumatriptan pill
Everything trends of medicines. Get here.
Обзор онлайн-казино Calibry calibri-casino-app.ru/ как получить бонус без депозита, запустить любимые слоты и вывести выигрыш. Надёжная площадка с лицензией и прозрачными условиями игры.
РедМетСплав предлагает широкий ассортимент высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица продукции подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Опытная поддержка – наш стандарт – мы на связи, чтобы улаживать ваши вопросы и предоставлять решения под особенности вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
Наши товары:
Фольга магниевая HM31A – ASTM B275 РўСЂСѓР±Р° магниевая HM31A – ASTM B275 представляет СЃРѕР±РѕР№ высококачественный РїСЂРѕРґСѓРєС‚, предназначенный для использования РІ различных отраслях. РћРЅР° обладает отличной прочностью Рё РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью, что делает ее идеальным решением для тяжелых условий эксплуатации. РЎ помощью этой трубы РІС‹ сможете значительно повысить надежность СЃРІРѕРёС… проектов. Данный материал соответствует стандартам ASTM B275, что подтверждает его высокое качество Рё долговечность. Если РІС‹ ищете прочный Рё легкий материал, вам стоит купить РўСЂСѓР±Р° магниевая HM31A – ASTM B275. Ртот РїСЂРѕРґСѓРєС‚ прекрасно справляется СЃ множеством задач.
How can you use random eggs and how hot can they be used in the world? What is the most popular version of Casino Ramenbet mobile version Раменбет казино
Играйте в казино Shot http://shot-casino-play.ru десятки провайдеров, ежедневные турниры и кэшбэк. В обзоре: лучшие слоты, лайв-игры, бонусные программы и преимущества платформы.
Aura Casino http://aura-casino-online.ru безопасная платформа с лицензией, SSL-защитой и проверенной репутацией. В обзоре: честность выплат, работа службы поддержки и реальные отзывы игроков.
Обзор Aura Casino http://aura-casino-play.ru всё о щедрых бонусах, фриспинах, кэшбэке и акциях. Расскажем, как зарегистрироваться, начать игру и получить максимум от каждого депозита.
мостбет авиатор [url=https://mostbet6035.ru]https://mostbet6035.ru[/url] .
The Tiger Fortune game is so easy to play. fortune tiger 777
can you buy generic zyrtec pills
can you get cheap aciphex without a prescription
This casino’s Tiger Fortune slot is unreal. fortune tiger demo gratis
I won $50 last night on an online slot game—small wins keep me hooked! aviator
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
С широким ассортиментом продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Никель-хромовая мишень
Для успешного продвижения вверх по карьерной лестнице требуется наличие диплома ВУЗа. Заказать диплом об образовании у сильной компании: [url=http://diplomt-v-chelyabinske.ru/kupit-diplom-v-krasnodare-bistro-i-vigodno/]diplomt-v-chelyabinske.ru/kupit-diplom-v-krasnodare-bistro-i-vigodno/[/url]
This online casino’s Tiger Fortune is flawless. fortune tiger 777
Drugs information leaflet. Long-Term Effects.
buspirone feeling high
All about medication. Get information now.
скачать мостбет [url=http://mostbet6033.ru/]http://mostbet6033.ru/[/url] .
Thanks for the valuable insights. Looking forward to your next post!
домашний интернет тарифы
domashij-internet-chelyabinsk003.ru
домашний интернет подключить челябинск
Арчибальд – академия, которая обучением груминга занимается. У вас есть возможность свои навыки совершенствовать до бесконечности на живых моделях. Преподаватели на все вопросы отвечают. Дарим методические материалы, учебники, а также видеолекции. Окунитесь в атмосферу, с которой расставаться не хочется. Ищете курсы груминга москве? Grooming-dream.ru – здесь представлены реальные отзывы клиентов. Мы лучших груминг-специалистов выпускаем. Предлагаем инструменты в период обучения. Если хотите освоить новую профессию, «Арчибальд» будет самым подходящим для вас местом.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние успехи.
Наша продукция:
Плоская мишень для распыления свинца 4N
Drinks delivery предоставляет большой выбор алкогольных напитков и доступные цены. Качество – главный наш приоритет. О своей репутации мы заботимся. Продаем только то, в чем уверены. С нами вы легко выберете нужный товар. Ищете alcohol delivery dubai? Deliverydubaidrinks.com – здесь есть абсент, ликер, вино, коньяк, ром, водка, джин, виски, бризер, текила, шампанское и пиво. Выполняем оперативную доставку алкоголя. Свое дело мы любим и к пожеланиям покупателей всегда прислушиваемся. На любой вопрос мы ответим и выбрать для вас подходящие напитки поможем.
Tiger Fortune’s rewards are always a thrill. fortune tiger
мостбет скачать бесплатно [url=http://mostbet6035.ru]http://mostbet6035.ru[/url] .
Приобретение подходящего диплома через качественную и надежную компанию дарит ряд плюсов. Такое решение помогает сэкономить как личное время, так и существенные деньги. Впрочем, достоинств значительно больше.Мы можем предложить дипломы любой профессии. Дипломы производятся на подлинных бланках. Доступная стоимость сравнительно с большими тратами на обучение и проживание в чужом городе. Покупка диплома университета станет мудрым шагом.
Заказать диплом о высшем образовании: [url=http://diplomg-kurerom.ru/vnesenie-diploma-v-reestr-po-dostupnoj-tsene-3/]diplomg-kurerom.ru/vnesenie-diploma-v-reestr-po-dostupnoj-tsene-3/[/url]
how can i get cephalexin without prescription
Medicament prescribing information. Generic Name.
how can i get generic proscar price
Actual news about meds. Get information here.
Выбор медалей на заказ требует внимания к деталям. Важно учитывать назначение изделия, тип металла, способ нанесения изображения и возможность персонализации. Также имеет значение форма, размер и оформление — всё это влияет на восприятие и ценность медали. Если вы хотите получить качественный результат, стоит заранее продумать все параметры. Подробнее о нюансах выбора и производства медалей читайте по ссылке:
https://createbrand.ru/kak-vybrat-medali-na-zakaz-i-chto-stoit-uchest-pri-ih-izgotovlenii/
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
поставляемая продукция:
Сетка просечно-вытяжная нержавеющая R10 4x4x0.5 Выбирайте надежную защиту Рё безопасность СЃ сеткой нержавеющей РѕС‚ Редметсплав.СЂС„. Рзготовленная РёР· прочной нержавеющей стали, сетка обеспечивает идеальную защиту РѕС‚ взлома, атмосферных воздействий Рё агрессивных сред. РЁРёСЂРѕРєРёР№ выбор размеров Рё типов плетения позволит подобрать подходящий вариант для различных потребностей. Подробные консультации Рё помощь РІ выборе предоставляются нашими экспертами.
Приобрести диплом ВУЗа!
Мы можем предложить документы институтов, которые находятся на территории всей России.
[url=http://diplomv-v-ruki.ru/kupit-diplom-s-zaneseniem-v-reestr-po-vigodnoj-tsene-4/]diplomv-v-ruki.ru/kupit-diplom-s-zaneseniem-v-reestr-po-vigodnoj-tsene-4/[/url]
buy generic clomid without prescription
I love the online crew—chat up! plinko
Обзор казино Shot https://shot-casino-bonus.ru лицензия, защита данных, честная игра и проверенные провайдеры. Узнайте, как работает система вывода, поддержка и что говорят реальные пользователи.
Онлайн-казино Calibry calibri-casino-apk бонус без депозита, фриспины, турниры и выплаты без задержек. Рассказываем, как начать играть, какие игры выбрать и стоит ли доверять платформе.
Calibry Casino https://calibri-casino-bonus.ru/ лицензированное онлайн-казино с бонусами, быстрым выводом и большим выбором игр. В обзоре: регистрация, акции, безопасность, отзывы игроков и советы новичкам.
Онлайн-казино Aura aura-casino-app лицензия, бонус без депозита, фриспины за регистрацию и моментальный вывод. Всё о казино Aura в одном обзоре — играйте безопасно и с выгодой.
https://forum.prosochi.ru/topic49168.html
провайдеры интернета по адресу
domashij-internet-krasnoyarsk001.ru
проверить интернет по адресу
РедМетСплав предлагает широкий ассортимент высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы обеспечиваем быстрое выполнение вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их качество. Опытная поддержка – наша визитная карточка – мы на связи, чтобы ответить на ваши вопросы и адаптировать решения под требования вашего бизнеса.
Доверьте ваш запрос специалистам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наша продукция:
Лист магниевый AZ81 Лента магниевая AZ81 – это высококачественный материал, используемый в различных отраслях, включая автомобилестроение и авиастроение. Она отличается отличными прочностными характеристиками и легкостью, что делает ее идеальной для применения в конструкциях, где требуется снижения веса без потери прочности. Лента магниевая AZ81 устойчива к коррозии и имеет хорошую свариваемость, что значительно упрощает ее обработку. Чтобы оценить все преимущества этого продукта, вы можете купить Лента магниевая AZ81, сделав выбор в пользу надежности и долговечности.
This casino’s Tiger Fortune slot is pure entertainment. fortune tiger 777
топсмайл [url=https://www.stomatologiya-mitino2.ru]топсмайл[/url] .
https://omsi2mod.ru/forum/12-3553-1
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – наилучшее решение для вашего бизнеса.
Наша продукция:
Медный тройник РїРѕРґ пайку СЃ направленным отводом 42С…36С…1.1 РјРј 27С…29 РјРј твердая пайка Cu-DHP ГОСТ Р 52922-2008 Купить качественные медные тройники РїРѕРґ пайку для надежных Рё долговечных соединений РІ трубопроводных системах. Разнообразие размеров Рё конфигураций, высокая теплопроводность Рё устойчивость Рє РєРѕСЂСЂРѕР·РёРё. Простая установка Рё надежность соединения. Рдеальное решение для различных проектов. Редметсплав.СЂС„
The excitement of Tiger Fortune never fades. tiger fortune
Interesting points! You explained it in a very clear way.
I love the high-energy feel of Tiger Fortune! fortune tiger demo
Medication information leaflet. Drug Class.
xanax buspirone hydrochloride
Some what you want to know about medicament. Read here.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
С широким ассортиментом продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние возможности.
Наша продукция:
Титан-алюминиевая плоская мишень
daftar sigmaslot
daftar sigmaslot
РедМетСплав предлагает обширный выбор качественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица изделия подтверждена требуемыми документами, подтверждающими их соответствие стандартам. Опытная поддержка – то, чем мы гордимся – мы на связи, чтобы разрешать ваши вопросы по мере того как предоставлять решения под особенности вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в множестве наших преимуществ
Наша продукция:
Пруток магниевый МА5 Проволока магниевая МА5 – это уникальный материал, предназначенный для различных промышленных приложений. Ее отличает высокая прочность и отличная коррозионная стойкость. Для сварки и механической обработки магниевая проволока проявляет отличные свойства, что делает ее незаменимой в электронике и машиностроении. Выбирая магниевую проволоку, вы получаете надежный продукт по доступной цене. Она легко поддается обработке и может использоваться в различных условиях. Не упустите возможность улучшить качество своих работ, купить Проволока магниевая МА5 можно прямо сейчас и получить конкурентное преимущество на рынке!
Здравствуйте!
Мы предлагаем дипломы любых профессий по доступным ценам. Стоимость будет зависеть от определенной специальности, года выпуска и образовательного учреждения: [url=http://diplomanrus.com/]diplomanrus.com/[/url]
Tiger Fortune’s gameplay is fast and fierce. tigrinho demo
This online casino’s Tiger Fortune is a blast. fortune tiger
where can i get cheap duphalac pills
Tiger Fortune’s theme is a total vibe. fortune tiger demo gratis
Assortment of slots one of the silent stores of Triks Casino. This is the case — and the Trix Casino is not limited to its one-size-fits-all store Казино Trix зеркало
The payouts in Tiger Fortune are pretty impressive. fortune tiger bet
Компания «Нефтемаш» из Ижевска — ваш надежный партнер в области промышленных решений. У нас вы сможете [url=https://neftemash18.ru/]11гри.04.003[/url] с официальной сертификацией и точным соответствием ГОСТ. Мы предлагаем широкий ассортимент для машиностроения и различных отраслей. Хотите работать напрямую с производителем? Мы рядом — работаем по всей России.
На сайте neftemash18.ru вы легко найдете даже нестандартные комплектующие — например [url=https://neftemash18.ru/]кольцо афни 754175.001[/url] или необходимые элементы. Поставки без задержек, прозрачные условия, техническая поддержка. Свяжитесь с нами: +7(912)441-91-50. Надежность, проверенная временем!
Автоперевозки из Китая по РФ https://ved-china.ru быстрая и выгодная доставка грузов напрямую до вашего склада. Консолидация, растаможка, сопровождение и отслеживание на всех этапах.
[url=https://kra29-at.at/]kraken shop[/url] – кракен зеркало, kraken официальный сайт
Где приобрести диплом специалиста?
Наши специалисты предлагают быстро заказать диплом, который выполнен на бланке ГОЗНАКа и заверен печатями, водяными знаками, подписями официальных лиц. Данный документ пройдет лубую проверку, даже с использованием профессиональных приборов. Решайте свои задачи максимально быстро с нашим сервисом.
Купить диплом любого университета [url=http://kupitediplom0027.ru/kupit-diplom-v-smolenske-3/]kupitediplom0027.ru/kupit-diplom-v-smolenske-3/[/url]
Pills information for patients. What side effects can this medication cause?
buspirone in the treatment of cerebellar ataxia
Everything trends of meds. Get information now.
Нужен надёжный хостинг? купить vps хостинг для сайтов, приложений и бизнес-задач. Гибкая настройка, SSD-накопители, стабильная работа 24/7. Выберите тариф под свои задачи и начните сегодня.
последние происшествия главные новости дня
Ищешь лучшие раскидки raskidki-granat-cs2.ru CS2 – Смоки, молотовы и флешки для всех карт — изучи тактики, прокачай игру и удиви соперников точными гранатами. Подборка эффективных раскидок!
Главная [url=https://lk-mango-office.com/]Манго Офис IP-телефония[/url]
Online casinos need hold—too wild! plinko
клиника смайл москва [url=http://stomatologiya-mitino2.ru/]клиника смайл москва[/url] .
провайдеры по адресу красноярск
domashij-internet-krasnoyarsk002.ru
интернет по адресу дома
Delivery-dubai.com предлагает широкий ассортимент разных видов алкоголя. На сайте можно ознакомиться с имеющейся продукцией в каталоге с ценами. Это даст возможность под ваш повод напиток выбрать подходящий. Осуществляем оперативную доставку алкоголя. Будем рады видеть вас в числе клиентов! Ищете алкоголь в дубае с доставкой? Delivery-dubai.com – тут широкий выбор алкоголя представлен. Вы найдете для себя все, что может принести удовольствие. Предлагаем товары исключительного качества. Для заказа нам в мессенджеры: Telegram, WhatsApp пишите.
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с прекрасным качеством обслуживания.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
поставляемая продукция:
Рнструментальная квадратная РїРѕРєРѕРІРєР° 48 РјРј 120РҐ ГОСТ 1133-71 Познакомьтесь СЃ широким ассортиментом высокопрочных инструментов для обработки металла РІ категории инструментальной квадратной РїРѕРєРѕРІРєРё РѕС‚ Редметсплав. Выбирайте РёР· прочных Рё надежных материалов, подобранных специально для различных РІРёРґРѕРІ металлообработки. Гарантированное качество РѕС‚ ведущих производителей.
This online casino’s Tiger Fortune is top-tier. fortune tiger 777
купить диплом училища высокие
Блог о маркетплейсах «OOWA» предлагает ознакомиться с актуальной, интересной и познавательной информацией, которая касается удачных продаж на маркетплейсах, обзоров товаров и услуг. На портале регулярно публикуются ценные и содержательные рекомендации от экспертов, которые помогут начать лучше разбираться в тонкостях такого бизнеса. Ищете продажа б/у вещей на ozon? На oowa.ru регулярно публикуются ценные и содержательные рекомендации от экспертов, которые помогут начать лучше разбираться в тонкостях такого бизнеса. Почитайте о самых востребованных товарах с маркетплейсов.
Понедельник: Астрологический прогноз на успешное начало недели
https://x.com/IrinaPavlovna84/status/1911485302209667581
Drug information sheet. Short-Term Effects.
pantoprazole pantoloc nursing considerations
Actual what you want to know about drug. Read information now.
Предлагаем покупку чеков https://kupit-cheki-new.ru/ в Москве для отчётности, командировок, подтверждения трат. Быстрое оформление, гибкие условия, все виды документов и сопровождение при необходимости.
Хотите купить чеки kupit-cheki-reg.ru в Москве срочно? Работаем без выходных, оформляем документы день в день, возможна доставка и электронные версии. Оперативность и конфиденциальность гарантированы.
Где купить kupit cheki24 чеки в Москве? У нас — быстрое оформление, оригинальные документы, курьерская доставка. Чеки для отчётов, подтверждения командировок и компенсаций.
РедМетСплав предлагает внушительный каталог качественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы обеспечиваем оперативное исполнение вашего заказа.
Каждая единица изделия подтверждена соответствующими документами, подтверждающими их качество. Превосходное обслуживание – наша визитная карточка – мы на связи, чтобы ответить на ваши вопросы а также предоставлять решения под особенности вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
Наша продукция:
Пруток висмутовый C89841 – UNS Пруток висмутовый C89841 – UNS представляет СЃРѕР±РѕР№ качественный металл СЃ уникальными свойствами. Р’РёСЃРјСѓС‚ широко используется РІ различных отраслях, благодаря своей РЅРёР·РєРѕР№ плотности Рё отличной РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкости. Ртот пруток идеален для производства изделий, РіРґРµ важны стабильность Рё качество. Если РІС‹ хотите купить Пруток висмутовый C89841 – UNS, РІС‹ получите надежный материал, который прослужит долго. РћРЅ прекрасно РїРѕРґС…РѕРґРёС‚ для применения РІ электроники, медицины Рё затемненных областях. Выберите наш пруток Рё убедитесь РІ его совершенстве!
The tiger roars in Tiger Fortune are epic. fortune tiger demo gratis
The music online lifts—keep on! book of ra
Витебский университет П.М.Машерова https://vsu.by образовательный центр. Вуз является ведущим образовательным, научным и культурным центром Витебской области.
Tiger Fortune’s payouts are so rewarding. fortune tiger
Всех приветствую!
Для многих людей, купить [b]диплом[/b] о высшем образовании – это острая потребность, шанс получить достойную работу. Однако для кого-то – это желание не терять время на учебу в ВУЗе. С какой бы целью вам это не понадобилось, мы готовы помочь. Оперативно, качественно и по доступной цене изготовим диплом любого года выпуска на подлинных бланках со всеми необходимыми печатями.
Основная причина, почему многие прибегают к покупке документов, – желание занять определенную работу. Предположим, знания позволяют специалисту устроиться на желаемую работу, однако документального подтверждения квалификации не имеется. Когда работодателю важно наличие “корочки”, риск потерять вакантное место очень высокий.
Купить документ о получении высшего образования можно в нашей компании. Мы предлагаем документы об окончании любых ВУЗов РФ. Вы получите необходимый диплом по любой специальности, любого года выпуска, включая документы Советского Союза. Даем гарантию, что в случае проверки документов работодателем, подозрений не возникнет.
Факторов, которые вынуждают купить диплом ВУЗа много. Кому-то срочно потребовалась работа, в итоге необходимо произвести впечатление на руководителя в процессе собеседования. Некоторые хотят попасть в серьезную компанию, для того, чтобы повысить свой статус и в последующем начать свой бизнес. Чтобы не тратить время, а сразу начинать удачную карьеру, используя имеющиеся навыки, можно купить диплом прямо в интернете. Вы сможете быть полезным для общества, обретете финансовую стабильность очень быстро и просто- [url=http://tutdiploms.com/]диплом о высшем образовании купить[/url]
Приобрести документ института можно в нашей компании в Москве. Мы предлагаем документы об окончании любых университетов России. Вы сможете получить необходимый диплом по любой специальности, любого года выпуска, включая документы СССР. Гарантируем, что при проверке документов работодателями, подозрений не появится. [url=http://good-diplom.ru/kupit-diplom-o-visshem-obrazovanii-s-reestrom/]good-diplom.ru/kupit-diplom-o-visshem-obrazovanii-s-reestrom/[/url]
топ смайл [url=www.stomatologiya-mitino2.ru/]топ смайл[/url] .
where can i get generic nemasole price
интернет провайдеры в красноярске по адресу дома
domashij-internet-krasnoyarsk003.ru
провайдеры в красноярске по адресу проверить
Drug prescribing information. Drug Class.
rx zoloft
Actual information about medicines. Read information here.
Tiger Fortune has the best energy of any game. tigrinho demo
buy cheap medex without rx
Tiger Fortune’s theme is so adventurous. fortune tiger
Ready to transform your hairstyle with real dreadlocks? Browse our selection of dreadlock extensions at this link – handmade dreadlocks, offering the highest quality options for achieving a flawless, natural look.
Carefully designed using 100% human hair, these dreadlocks are ideal for your unique personality. Whether you’re into clip-ins, we have options that fit your exact texture.
Express yourself with:
– real dreadlock extensions
– dreadlock extensions
Make a statement with premium-quality extensions that look and feel real. Discreet packaging available across the USA and beyond!
Shop now – your dream style awaits.
I’ve had some huge wins on Tiger Fortune! tiger fortune
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наша продукция:
Высокочистая никелевая мишень
The mobile casino layout needs work—too messy! plinko
Купить диплом любого ВУЗа!
Мы предлагаем документы ВУЗов, которые находятся в любом регионе Российской Федерации.
[url=http://diplomt-v-samare.ru/kupit-diplom-v-moskve-s-zaneseniem-v-reestr-legko/]diplomt-v-samare.ru/kupit-diplom-v-moskve-s-zaneseniem-v-reestr-legko/[/url]
Tiger Fortune’s bonuses are wild and exciting. fortune tiger 777
Мы изготавливаем дипломы любых профессий по приятным тарифам. Мы предлагаем документы ВУЗов, которые расположены на территории всей РФ. Дипломы и аттестаты выпускаются на “правильной” бумаге высшего качества. Это дает возможность делать государственные дипломы, которые не отличить от оригиналов. [url=http://anychinajob.com/companies/archive-diploma/]anychinajob.com/companies/archive-diploma[/url]
Yeniköy su kaçak tespiti Garantili İşçilik: Tamirat sonrası da destek alabileceğimizi söylediler. Çok memnun kaldık. http://sat.poznan.pl/?p=2548
стоимость зубного протезирования [url=https://www.protezirovanie-zubov2.ru]стоимость зубного протезирования[/url] .
зубная протезирование зубов [url=www.protezirovanie-zubov3.ru]www.protezirovanie-zubov3.ru[/url] .
Meds information. Short-Term Effects.
generic clomid without dr prescription
Best what you want to know about medicament. Read here.
Tiger Fortune makes every win feel huge. fortune tiger demo gratis
Market-delivery-dubai.ru предлагает доставку алкоголя. У вас есть возможность купить виски, ром, вино, текилу, бризер, абсцент, коньяк, водку, пиво, джин, ликер. Мы гарантируем приемлемые цены. Доверие покупателей заслужили. Ищете dubai alcohol delivery? Market-delivery-dubai.ru – здесь есть то, что вам нужно. Регулярно работаем над ассортиментом. Потребности своих клиентов мы хорошо знаем. Заказы принимаем через Whatsapp. Выполняем доставку различного вида алкогольной продукции отменного качества в сжатые сроки. Необходима помощь? Свяжитесь с нами.
Портал представляет собой сайт по аренде, на котором предлагается взять в прокат самую разную технику для работ. Также получится выложить объявления со своими предложениями, чтобы ими воспользовались все желающие. https://arenda24.by/ – на сайте изучите категории, которые будут вам полезны: грузоперевозки, вспомогательное оборудование, режущий инструмент, строительные краны и многое другое. Получить доступ к опциям удастся, если пройдете регистрацию.
Allergic reactions symptom alleviation methods
Где купить [b]диплом[/b] специалиста?
Готовый диплом со всеми печатями и подписями отвечает стандартам Министерства образования и науки, неотличим от оригинала. Не следует откладывать свои цели на потом, реализуйте их с нашей помощью – отправьте быструю заявку на диплом уже сегодня! Приобрести диплом о среднем образовании – не проблема! [url=http://kome.maxbb.ru/viewtopic.phpf=22&t=3497/]kome.maxbb.ru/viewtopic.phpf=22&t=3497[/url]
The casino’s Tiger Fortune slot has great winning potential. tigrinho demo
проверить провайдера по адресу
domashij-internet-krasnodar001.ru
провайдеры по адресу дома
протезирование 2 зубов [url=www.protezirovanie-zubov1.ru/]протезирование 2 зубов[/url] .
can you get cheap enalapril without rx
Tiger Fortune’s payouts keep me coming back for more. fortune tiger
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с прекрасным качеством обслуживания.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
Наши товары:
Фехралевая проволока 2 РјРј РҐ23Р®5 ГОСТ 12766.1-90 Купить фехральевую проволоку РѕС‚ Редметсплав РїРѕ выгодной цене. Высококачественный материал СЃ высокой прочностью Рё устойчивостью Рє внешним воздействиям. РЁРёСЂРѕРєРёР№ спектр размеров Рё диаметров. Рдеальный выбор для производства обогревательных элементов, термопар Рё РґСЂСѓРіРёС… изделий.
Вместе с t.me/azino777_a можете отправиться в захватывающее путешествие по миру азартных игр. Предлагаем персонализированные предложения и уникальные бонусы. Расскажем, как начать играть в Азино. Вас ждут невероятные эмоции и возможность выиграть крупный приз. Не упустите шанс испытать свою удачу! Ищете azino777 игровые автоматы? – тут имеется множество актуальной информации. Собрали самые частые вопросы от игроков. Рады вам помочь. Приготовьтесь к увлекательным турнирам в Азино. Участвуйте и боритесь за призы с другими игроками!
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние достижения.
Наша продукция:
Плоская мишень Nb2O5 4N
I hit a big win on Tiger Fortune last night! fortune tiger bet
Где приобрести диплом по актуальной специальности?
Наша компания предлагает быстро и выгодно заказать диплом, который выполняется на бланке ГОЗНАКа и заверен мокрыми печатями, штампами, подписями официальных лиц. Документ способен пройти любые проверки, даже с применением специальных приборов. Решите свои задачи максимально быстро с нашей компанией.
Заказать диплом любого университета [url=http://fastdiploms.com/kupit-attestat-za-9-klassov-13/]fastdiploms.com/kupit-attestat-za-9-klassov-13/[/url]
Meds information. Effects of Drug Abuse.
where can i buy cheap tamoxifen without rx
All trends of drug. Get information here.
I’ve had some great wins on Tiger Fortune recently. fortune tiger
РедМетСплав предлагает широкий ассортимент высококачественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица продукции подтверждена соответствующими документами, подтверждающими их происхождение. Опытная поддержка – наш стандарт – мы на связи, чтобы улаживать ваши вопросы по мере того как находить ответы под специфику вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
РљСЂСѓРі титановый Рў8 – ГОСТ 2171-90 Фольга титановая Рў8 – ГОСТ 2171-90 – это высококачественный материал СЃ уникальными свойствами, который широко используется РІ различных отраслях, включая авиацию Рё медицину. РћРЅР° обладает отличной РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью Рё легкостью, что делает ее идеальным выбором для изготовления деталей, требующих высокой прочности Рё надежности. Благодаря СЃРІРѕРёРј характеристикам, фольга идеально РїРѕРґС…РѕРґРёС‚ для сварки Рё алюминиевых композиций. Если РІС‹ хотите купить Фольга титановая Рў8 – ГОСТ 2171-90, РІС‹ получите надежный РїСЂРѕРґСѓРєС‚ для СЃРІРѕРёС… РЅСѓР¶Рґ. РќРµ упустите шанс обеспечить СЃРІРѕР№ проект качественным материалом!
Получить диплом ВУЗа поможем. Купить диплом о высшем образовании в Воронеже – [url=http://diplomybox.com/kupit-diplom-o-vysshem-obrazovanii-v-voronezhe/]diplomybox.com/kupit-diplom-o-vysshem-obrazovanii-v-voronezhe[/url]
Such a well-written piece. It kept me engaged from start to finish.
The slot variety online is amazing—so fun! bet on red
коронка зуба цена [url=http://www.protezirovanie-zubov2.ru]коронка зуба цена[/url] .
протезирование зуба цена [url=http://www.protezirovanie-zubov3.ru]протезирование зуба цена[/url] .
Только 2 знакам Зодиака невероятно повезет во второй половине апреля
https://x.com/Fariz418740/status/1911625378860278161
Мы можем предложить дипломы психологов, юристов, экономистов и любых других профессий по приятным ценам. Стараемся поддерживать для заказчиков адекватную ценовую политику. Важно, чтобы дипломы были доступными для подавляющей массы граждан.
Заказ документа, подтверждающего обучение в ВУЗе, – это грамотное решение. Купить диплом университета: [url=http://kupitediplom0029.ru/diplom-kupit-texnicheski/]kupitediplom0029.ru/diplom-kupit-texnicheski/[/url]
Мы готовы предложить дипломы любой профессии по приятным ценам.– [url=http://vuz-diplom.ru/kupite-diplom-visshego-obrazovaniya-s-zaneseniem-v-reestr-2/]vuz-diplom.ru/kupite-diplom-visshego-obrazovaniya-s-zaneseniem-v-reestr-2/[/url]
This casino’s Tiger Fortune slot is pure joy. fortune tiger bet
купить официальный диплом
can you buy cheap abilify without prescription
motsbet [url=mostbet6033.ru]mostbet6033.ru[/url] .
The payouts in Tiger Fortune are pretty impressive. fortune tiger demo
where buy cheap remeron without rx
провайдеры интернета в краснодаре по адресу
domashij-internet-krasnodar002.ru
интернет по адресу краснодар
Pills prescribing information. Effects of Drug Abuse.
how can i get lyrica without prescription
Some what you want to know about medication. Read information now.
москва стоматолог [url=www.stomatologiya-mitino3.ru/]москва стоматолог[/url] .
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с высоким уровнем сервиса.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
поставляемая продукция:
Титановая труба 32х1.8х7000 мм ВТ1-0 ГОСТ 22897-86 Откройте мир прочных и надежных титановых труб с компанией Редметсплав. Наши высококачественные титановые трубы сочетают в себе устойчивость к коррозии, износостойкость и легкий вес, делая их незаменимым материалом для применения в различных сферах промышленности. Широкий выбор различных диаметров и толщин, а также индивидуальные решения для вашего производства. Гарантированное качество и надежность поставок.
цена протезирование зубов [url=http://protezirovanie-zubov1.ru/]цена протезирование зубов[/url] .
I’m shy on online—shaky feel! plinko
Tiger Fortune’s jackpot is calling my name. tiger fortune
Tiger Fortune’s energy is off the charts. fortune tiger demo
РедМетСплав предлагает внушительный каталог отборных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их качество. Опытная поддержка – то, чем мы гордимся – мы на связи, чтобы разрешать ваши вопросы и находить ответы под требования вашего бизнеса.
Доверьте вашу потребность в редких металлах специалистам РедМетСплав и убедитесь в гибкости нашего предложения
Наши товары:
Лист магниевый MC7 – JIS H 5203 Лента магниевая MC7 – JIS H 5203 предназначена для использования РІ различных промышленных сферах. Рто высококачественный РїСЂРѕРґСѓРєС‚, который обеспечивает отличную прочность Рё устойчивость Рє РєРѕСЂСЂРѕР·РёРё. Лента выполнена РІ соответствии СЃ международными стандартами, что гарантирует её надежность Рё долговечность. Если РІС‹ ищете эффективное решение для СЃРІРѕРёС… проектов, РјС‹ рекомендуем купить Лента магниевая MC7 – JIS H 5203. Рспользуя эту ленту, РІС‹ обеспечите надежность Рё безопасность ваших конструкций.
Go to the site https://betwave.ro/en where you will find a list of all the best online casinos, as well as useful and complete information about each of them – how to play, what bonuses there are, how to withdraw money, which casinos have a minimum deposit, registration steps and advantages of each of them, as well as what are the biggest bonuses in online casinos. More details on the site.
стоимость зубного протезирования [url=www.protezirovanie-zubov2.ru/]стоимость зубного протезирования[/url] .
протезирование верхних зубов цена [url=protezirovanie-zubov3.ru]protezirovanie-zubov3.ru[/url] .
мостбет казино войти [url=https://www.mostbet6033.ru]https://www.mostbet6033.ru[/url] .
Tiger Fortune’s spins keep me on my toes. fortune tiger demo
can you get cheap inderal online
Medicament information. Generic Name.
get cheap phenytoin online
Some trends of medicament. Get information now.
Металлические сувениры становятся всё более популярными в рекламной индустрии благодаря своей долговечности, эстетической привлекательности и возможностям персонализации. Использование современных технологий, таких как лазерная гравировка и 3D-печать, позволяет создавать уникальные изделия, подчёркивающие статус и надёжность бренда. Кроме того, металлические сувениры являются экологичным и экономически эффективным решением для компаний, стремящихся к устойчивому развитию. Подробнее об инновациях в производстве металлических сувениров можно узнать по ссылке: https://bvfy.ru/samie-raznoobraznie-novosti-kazhdij-den-s-razlichnix-ugolkov-zemnogo-shara-novosti-politiki-ekonomiki-sporta-a-takzhe-dostizheniya-texnologij-stroitelstva-i-drugie-vazhnie-sobitiya/
can i purchase nizoral pill
провайдеры интернета в краснодаре по адресу
domashij-internet-krasnodar003.ru
интернет провайдеры краснодар по адресу
Спасибо вам!
Предлагаю вам [url=https://rufilmonline.com/collections/pro-vov/]военные фильмы русские[/url] – это невероятное произведение, которое любят не только в России, но и во всем мире. Они предлагают уникальный взгляд на русскую культуру, историю и обычаи. В настоящее время смотреть русские фильмы и сериалы онлайн стало легко благодаря различным платформам и сервисам. От мелодрам до триллеров, от исторических лент до современных детективов – выбор безграничен. Окунитесь в захватывающие сюжеты, талантливые актерские исполнения и красивую операторскую работу, смотрите фильмы и сериалы из России прямо у себя дома.
Tiger Fortune’s spins are always a rush. fortune tiger bet
The variety of live games at ElonBet keeps me entertained for hours. elonbet casino
Металлические сувениры становятся всё более популярными в рекламной индустрии благодаря своей долговечности, эстетической привлекательности и возможностям персонализации. Использование современных технологий, таких как лазерная гравировка и 3D-печать, позволяет создавать уникальные изделия, подчёркивающие статус и надёжность бренда. Кроме того, металлические сувениры являются экологичным и экономически эффективным решением для компаний, стремящихся к устойчивому развитию. Подробнее об инновациях в производстве металлических сувениров можно узнать по ссылке: https://bvfy.ru/samie-raznoobraznie-novosti-kazhdij-den-s-razlichnix-ugolkov-zemnogo-shara-novosti-politiki-ekonomiki-sporta-a-takzhe-dostizheniya-texnologij-stroitelstva-i-drugie-vazhnie-sobitiya/
Tiger Fortune has the best energy of any game. jogo do tigrinho demo
Детский туроператор Флагман организует школьные экскурсии и туры для детей по Санкт-Петербургу и Северо-Западу. Поездки с Флагманом всегда превращаются в увлекательные приключения. Собственный автопарк, профессиональные водители и контроль безопасности в пути гарантируют комфортные путешествия для детей. Профессиональные экскурсоводы адаптируют маршруты под детскую аудиторию, делая их познавательными и интересными. Подробнее на официальном сайте https://flagmanspb.ru/
Где приобрести диплом специалиста?
Мы можем предложить дипломы любой профессии по выгодным тарифам. Для нас очень важно, чтобы дипломы были доступны для большого количества наших граждан. Приобрести диплом об образовании [url=http://poluchidiplom.com/diplom-moskva-s-reestrom-kupit-bistro-i-nadezhno/]poluchidiplom.com/diplom-moskva-s-reestrom-kupit-bistro-i-nadezhno/[/url]
Arenda24.by – интернет-платформа для размещения объявлений по аренде оборудования и строительной техники. На нашем портале вы сможете комфортно работать, потому, как его настоящая команда профессионалов разработала. Ищете аренда мини экскаватора беларусь? Arenda24.by – сайт, где вы сможете отыскать по аренде различных инструментов и техники объявления, также грузоперевозки организовать. У нас есть объявления любых типов категорий. Разместите объявление уже сейчас, и оно в сжатые сроки своих клиентов найдет. С нами вы доступ к известной и качественной платформе получите.
Мы можем предложить дипломы любой профессии по приятным тарифам. Купить диплом бурильщика — [url=http://kyc-diplom.com/diplomy-po-professii/kupit-diplom-burilshhika.html/]kyc-diplom.com/diplomy-po-professii/kupit-diplom-burilshhika.html[/url]
Aproveite a oferta exclusiva do tv bet para novos usuários e
receba 100$ de bônus ao se registrar! Este bônus de boas-vindas permite que você experimente uma vasta
gama de jogos de cassino online sem precisar gastar imediatamente.
Com o bônus de 100$, você poderá explorar jogos como
roleta, blackjack, caça-níqueis e muito mais, aumentando suas chances de vitória desde o primeiro minuto.
Não perca essa chance única de começar com um
valor significativo – cadastre-se agora!
I wish online paid fast—slow drag! plinko
Visit https://toptenscasinos.com/ and you will find a carefully selected list of the top 10 online casinos, games and strategies. Our goal is to provide informative, unbiased and entertaining content to help players make informed decisions in the world of online gaming. Helping players understand the games, rules and strategies in a simple and fun way.
Medicine information sheet. What side effects?
cheap ramipril without a prescription
Everything news about medicines. Get now.
стоматология в митино [url=stomatologiya-mitino3.ru]стоматология в митино[/url] .
I recently tried ElonBet Casino, and the interface is super sleek! Love the futuristic vibe.
elonbet casino
Металлические сувениры становятся всё более популярными в рекламной индустрии благодаря своей долговечности, эстетической привлекательности и возможностям персонализации. Использование современных технологий, таких как лазерная гравировка и 3D-печать, позволяет создавать уникальные изделия, подчёркивающие статус и надёжность бренда. Кроме того, металлические сувениры являются экологичным и экономически эффективным решением для компаний, стремящихся к устойчивому развитию. Подробнее об инновациях в производстве металлических сувениров можно узнать по ссылке: https://bvfy.ru/samie-raznoobraznie-novosti-kazhdij-den-s-razlichnix-ugolkov-zemnogo-shara-novosti-politiki-ekonomiki-sporta-a-takzhe-dostizheniya-texnologij-stroitelstva-i-drugie-vazhnie-sobitiya/
The leaderboard at ElonBet motivates me to play more.
elonbet casino
Мы предлагаем дипломы любой профессии по выгодным тарифам. Мы можем предложить документы техникумов, расположенных в любом регионе Российской Федерации. Документы выпускаются на “правильной” бумаге высшего качества. Это позволяет делать настоящие дипломы, не отличимые от оригиналов. [url=http://forum-pmr.net/member.phpu=42748/]forum-pmr.net/member.phpu=42748[/url]
Заказать диплом о высшем образовании !
Покупка диплома университета РФ в нашей компании – надежный процесс, потому что документ заносится в реестр. Заказать диплом об образовании [url=http://diplomk-vo-vladivostoke.ru/kupit-diplom-o-visshem-obrazovanii-s-polozhitelnimi-otzivami/]diplomk-vo-vladivostoke.ru/kupit-diplom-o-visshem-obrazovanii-s-polozhitelnimi-otzivami[/url]
Система менеджмента управления качеством содержит критерии менеджмента и стандарты, по которым проводится последующая сертификация предприятия. Чтобы получить сертификат СМК, нужно обратиться в аккредитованный сертификационный центр. Сертификация систем менеджмента качества является документальным подтверждением того, что система менеджмента сертифицированной компании соответствует требованиям того или иного стандарта. Подробнее: https://ok.ru/group/70000034956977
Meds information. What side effects?
where can i buy generic priligy pills
All about medicament. Get now.
Для фанатов скинов CS 2 сделали обзор на сайты где продать скины кс2. В статье представлен подробный и актуальный рейтинг сайтов, где можно продать скины CS2 и CS:GO. Анализ более чем 10 источников и отобрал только те платформы, которые пользуются хорошей репутацией в сообществе.
Контроль за территорией начинается с установки камер видеонаблюдения. [url=https://emercom-irk.ru/videonablyudeniye]Установить камеры Иркутск[/url] можно быстро и профессионально. Современные технологии обеспечивают высокое качество изображения и возможность удаленного контроля за происходящим на объекте.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Оптимальное название товара: Гранулы железа 4N
can you get generic celexa pills
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние успехи.
Наша продукция:
Плоская мишень Cu+Ni+Ti
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наши товары:
Вращающаяся мишень для распыления вольфрама
The game providers at ElonBet are some of the best in the industry. elonbet casino
Металлические сувениры становятся всё более популярными в рекламной индустрии благодаря своей долговечности, эстетической привлекательности и возможностям персонализации. Использование современных технологий, таких как лазерная гравировка и 3D-печать, позволяет создавать уникальные изделия, подчёркивающие статус и надёжность бренда. Кроме того, металлические сувениры являются экологичным и экономически эффективным решением для компаний, стремящихся к устойчивому развитию. Подробнее об инновациях в производстве металлических сувениров можно узнать по ссылке: https://bvfy.ru/samie-raznoobraznie-novosti-kazhdij-den-s-razlichnix-ugolkov-zemnogo-shara-novosti-politiki-ekonomiki-sporta-a-takzhe-dostizheniya-texnologij-stroitelstva-i-drugie-vazhnie-sobitiya/
Tiger Fortune’s tiger roars make every win epic. fortune tiger demo
На сайте https://rozatoday.ru/ представлено огромное количество ярких, запоминающихся композиций, которые созданы из свежих цветов: ослепительных роз, волнующих пионов, трепетных тюльпанов, строгих гвоздик. В компании работают только знающие, компетентные флористы, которые создают изысканные композиции в соответствии с предпочтениями клиента. В обязательном порядке учитываются и актуальные мировые тенденции. Можно приобрести как небольшой букетик, который подходит для романтического свидания, так и внушительных размеров для грандиозного праздника.
ElonBet’s tournaments are intense! Won some extra cash last weekend.
elonbet casino
мос бет [url=http://mostbet6036.ru/]http://mostbet6036.ru/[/url] .
интернет по адресу
domashij-internet-samara001.ru
интернет по адресу
The seasonal events at ElonBet keep the excitement going.
elonbet casino
ElonBet Casino’s design is so modern—love the aesthetic.
elonbet casino
Visit https://wpstore.pro/ where you will find premium WordPress themes and plugins. We have collected the best solutions for WordPress in one place. Official products from developers. We have the most affordable prices, original products, unlimited use, lifetime updates and much more. More details on the site.
Заинтересованы в качественной продукции?, интернет-магазин Camogear. У нас широкий ассортимент. Не пропустите шанс, по лучшим ценам. и не пропустите выгодные предложения. Индивидуальный подход – это наша цель. Наслаждайтесь покупками.
військові тактичні штани [url=https://camogear.com.ua/]військові тактичні штани[/url] .
ElonBet’s bonus wagering requirements are pretty fair.
elonbet casino
Medication information leaflet. Brand names.
order lisinopril online
Actual about medicament. Get now.
The leaderboard challenges at ElonBet are super engaging.
elonbet casino
Tiger Fortune’s features are pure genius. tigrinho demo
The slots at ElonBet have some of the best RTPs I’ve seen.
elonbet casino
Спасибо за “музыкальный” букет – розы были с нотками!
доставка цветов томск
Meds information. What side effects can this medication cause?
lisinopril other names for this drug
All information about medicine. Get information now.
Tiger Fortune’s spins are fast and fun. fortune tiger demo
The roulette tables at ElonBet are my go-to. Smooth gameplay every time.
elonbet casino
I can’t believe how much fun Tiger Fortune is! tiger fortune
Заказать диплом об образовании!
Мы изготавливаем дипломы любой профессии по доступным тарифам— [url=http://damdiplomisa.com/diplom-stomatologa-kupit-po-vigodnoj-tsene-sejchas/]damdiplomisa.com/diplom-stomatologa-kupit-po-vigodnoj-tsene-sejchas/[/url]
Мы изготавливаем дипломы любой профессии по приятным тарифам. Стараемся поддерживать для клиентов адекватную политику цен. Для нас очень важно, чтобы документы были доступными для большого количества граждан.
Приобретение диплома, подтверждающего обучение в ВУЗе, – это грамотное решение. Приобрести диплом любого университета: [url=http://diplommy.ru/kupit-diplom-nedorogo-v-moskve/]diplommy.ru/kupit-diplom-nedorogo-v-moskve/[/url]
The Tiger Fortune game runs so smoothly on my phone. fortune tiger bet
Оптовый интернет – магазин керамический плитки и керамогранита https://alliance-plitka.ru
ElonBet’s privacy policy is clear, which builds trust.
elonbet casino
Купить диплом любого ВУЗа!
Мы можем предложить документы учебных заведений, которые расположены на территории всей Российской Федерации. Дипломы и аттестаты выпускаются на бумаге самого высшего качества: [url=http://jobs.alibeyk.com/employer/aurus-diploms/]jobs.alibeyk.com/employer/aurus-diploms[/url]
ElonBet Casino’s payout system is transparent. No hidden fees. elonbet casino
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – идеальный вариант для вас.
Наша продукция:
Алюминиевый двутавр РђРљ4-1С‡ 2x58x100 ГОСТ 13621-90 Купить двутавр алюминиевый СЃ высокой прочностью Рё устойчивостью Рє РєРѕСЂСЂРѕР·РёРё. Легкий вес для удобства транспортировки Рё монтажа. Рдеальное решение для создания каркасов зданий, мостов Рё РґСЂСѓРіРёС… конструкций. РџРѕРґС…РѕРґРёС‚ для применения РІ авиации, автомобильной Рё РґСЂСѓРіРёС… отраслях промышленности.
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с высоким уровнем сервиса.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
поставляемая продукция:
Пруток СЃ заданным ТКЛР185 РјРј 52Рќ-ВРГОСТ 14082-78 Пруток СЃ заданным ТКЛР– высокопрочный Рё устойчивый материал для создания надежных конструкций. РЁРёСЂРѕРєРёР№ выбор размеров Рё типов прутка РІ категории РЅР° сайте Редметсплав.СЂС„. Поддержка Рё индивидуальный РїРѕРґС…РѕРґ экспертов для выбора наиболее подходящего продукта.
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с прекрасным качеством обслуживания.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
Наша продукция:
Медный удлиненный двухраструбный отвод 90 градусов под пайку 40.5х36х1.1 мм 10х12 мм твердая пайка Cu-DHP ГОСТ 32590-2013 Покупайте медные двухраструбные отводы 90 градусов под пайку на Редметсплав.рф. Надежные и долговечные отводы из высококачественной меди для систем водоснабжения, отопления и кондиционирования воздуха. Гарантированно надежные соединения, простой монтаж и оптимальное распределение потока.
Online bingo chills—cool gang! fortune ox
top smile [url=http://stomatologiya-mitino3.ru/]top smile[/url] .
can i purchase cheap terramycin tablets
mostbet apk скачать [url=http://mostbet6036.ru/]http://mostbet6036.ru/[/url] .
Приобретение официального диплома через проверенную и надежную фирму дарит ряд преимуществ для покупателя. Данное решение помогает сберечь время и существенные средства. Тем не менее, только на этом выгода не ограничивается, преимуществ гораздо больше.Мы изготавливаем дипломы любой профессии. Дипломы производятся на настоящих бланках государственного образца. Доступная цена в сравнении с огромными затратами на обучение и проживание в другом городе. Заказ диплома об образовании из российского ВУЗа является разумным шагом.
Заказать диплом: [url=http://diplomv-v-ruki.ru/kupit-diplom-bakalavra-s-zaneseniem-v-reestr-3/]diplomv-v-ruki.ru/kupit-diplom-bakalavra-s-zaneseniem-v-reestr-3/[/url]
Great post! I really enjoyed the insights you shared. Looking forward to reading more from you!
Anyone else loving the Tesla-themed slots at ElonBet? They’re so much fun!
elonbet casino
Online casinos should limit losses—safer play! gates of olympus
провайдеры в самаре по адресу проверить
domashij-internet-samara002.ru
провайдеры интернета в самаре по адресу
ElonBet’s support team helped me resolve a login issue in minutes.
elonbet casino
Medicament information. Short-Term Effects.
how to get cheap macrobid prices
Actual news about medicines. Get now.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
С широким ассортиментом продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наша продукция:
Плоская мишень тантала
РедМетСплав предлагает внушительный каталог качественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица товара подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Дружелюбная помощь – наш стандарт – мы на связи, чтобы ответить на ваши вопросы и находить ответы под специфику вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в множестве наших преимуществ
Наши товары:
Р’РёСЃРјСѓС‚ CACIn901 – JIS H 2202-2016 Р’РёСЃРјСѓС‚ CACIn901 – JIS H 2202-2016 представляет СЃРѕР±РѕР№ высококачественный материал, широко применяемый РІ различных отраслях. Ртот сплав обладает уникальными свойствами, включая устойчивость Рє РєРѕСЂСЂРѕР·РёРё Рё отличную текучесть, что делает его идеальным выбором для использования РІ производстве Рё технологии. Покупая Р’РёСЃРјСѓС‚ CACIn901 – JIS H 2202-2016, РІС‹ получаете надежный РїСЂРѕРґСѓРєС‚, который соответствуют международным стандартам качества. Рто идеальный вариант для тех, кто ценит долговечность Рё эффективность. РќРµ упустите возможность купить Р’РёСЃРјСѓС‚ CACIn901 – JIS H 2202-2016 Рё оптимизируйте СЃРІРѕРё производственные процессы СѓР¶Рµ сегодня.
РедМетСплав предлагает обширный выбор качественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица изделия подтверждена соответствующими документами, подтверждающими их происхождение. Дружелюбная помощь – то, чем мы гордимся – мы на связи, чтобы разрешать ваши вопросы по мере того как предоставлять решения под требования вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
Наша продукция:
Лента титановая РђРў3 – ГОСТ 19807-91 РљСЂСѓРі титановый РђРў3 – ГОСТ 19807-91 является идеальным решением для различных отраслей, включая авиастроение Рё машиностроение. Данный РїСЂРѕРґСѓРєС‚ отличается высокой прочностью Рё легкостью, что делает его незаменимым РІ производстве. РџСЂРё производстве РєСЂСѓРіР° используются только качественные материалы, что обеспечивает долговечность Рё надежность изделия. Если РІС‹ ищете долговечный Рё надежный титан, то купить РљСЂСѓРі титановый РђРў3 – ГОСТ 19807-91 стоит РїСЂСЏРјРѕ сейчас. Ртот РєСЂСѓРі РїРѕРґС…РѕРґРёС‚ для токарной обработки Рё сварки, обеспечивая отличные результаты РІ работе. РќРµ упустите возможность улучшить СЃРІРѕРё проекты СЃ помощью нашего товара!
ElonBet Casino’s community events make it feel more interactive. elonbet casino
how can i get crestor tablets
РедМетСплав предлагает обширный выбор высококачественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица изделия подтверждена требуемыми документами, подтверждающими их качество. Опытная поддержка – наш стандарт – мы на связи, чтобы улаживать ваши вопросы и предоставлять решения под особенности вашего бизнеса.
Доверьте ваш запрос специалистам РедМетСплав и убедитесь в множестве наших преимуществ
Наша продукция:
Пруток магниевый MS1 – JIS H 4204 Проволока магниевая MS1 – JIS H 4204 – это высококачественный РїСЂРѕРґСѓРєС‚, идеально подходящий для использования РІ различных промышленных приложениях. Рзготовленная РїРѕ стандартам JIS H 4204, РѕРЅР° обеспечивает отличные механические свойства Рё РєРѕСЂСЂРѕР·РёРѕРЅРЅСѓСЋ стойкость. Рта проволока обладает хорошей пластичностью, что делает ее легкой РІ обработке Рё формовании. Если РІС‹ ищете надежное решение для СЃРІРѕРёС… задач, то купить Проволока магниевая MS1 – JIS H 4204 – отличный выбор. РќРµ упустите возможность использовать этот функциональный материал для повышения эффективности вашего производства.
Novo no batbet – https://batbet-88.com? Cadastre-se e Receba 100$ de Bônus ao Fazer Login!
Novos Usuários no onabet Ganham
100$ de Bônus ao se Cadastrar!
Лучшие сервера ла2 без доната
Dear Sir/ma,
We are a financial services and advisory company mandated by our investors to seek business opportunities and projects for possible funding and debt capital financing.
Please note that our investors are from the Gulf region. They intend to invest in viable business ventures or projects that you are currently executing or intend to embark upon as a means of expanding your (their) global portfolio.
We are eager to have more discussions on this subject in any way you believe suitable.
Please contact me on my direct email: ahmed.abdulla@dejlaconsulting.com
Looking forward to working with you.
Yours faithfully,
Ahmed Abdulla
financial advisor
Dejla Consulting LLC
Online casinos suit calm—no rush! plinko
посетить веб-сайт [url=https://lk.mango-offlce.net]Mango-Office API документация[/url]
веб-сайте [url=https://mango-office.cc/]Mango-Office интеграция с CRM[/url]
ElonBet’s mobile app runs smoothly. Perfect for gaming on the go! elonbet casino
I love the seasonal promos at online casinos—fun twist! plinko
The futuristic theme of ElonBet Casino is such a unique touch.
elonbet casino
мостбет кг [url=http://mostbet5002.ru/]http://mostbet5002.ru/[/url] .
ElonBet’s live casino feels like Vegas from my couch. Loving it! elonbet casino
Бизнес издание Business Review – помощь директорам, новости Digital.
Tried a few slots at ElonBet, and the graphics are next-level awesome.
elonbet casino
Значки из металла https://www.skalpil.ru/novosti-mediciny/other/6623-metallicheskie-znachki-populyarnyy-element-korporativnoy-i-individualnoy-identifikacii.html это эффективный инструмент идентификации в бизнесе и медицине. Они подчёркивают статус, создают узнаваемый образ и способствуют формированию корпоративной культуры.
ВГУ им. П. М. Машерова https://vsu.by официальный сайт, факультеты, направления подготовки, приёмная кампания. Узнайте о поступлении, обучении и возможностях для студентов.
Привет!
Мы изготавливаем дипломы любых профессий по приятным ценам. Цена может зависеть от конкретной специальности, года получения и образовательного учреждения: [url=http://rdiplomm24.com/]rdiplomm24.com/[/url]
Ao se cadastrar no codbet, você ganha
um bônus de 100$ para começar sua jornada no cassino com o pé direito!
Não importa se você é um novato ou um apostador
experiente, o bônus de boas-vindas é a oportunidade perfeita para
explorar todas as opções que o site tem a oferecer. Jogue seus jogos favoritos, descubra
novas opções de apostas e aproveite para testar estratégias
sem risco, já que o bônus ajuda a aumentar suas chances de ganhar.
Cadastre-se hoje e comece com 100$!
опубликовано здесь [url=https://lk.mango-offic.info/]Манго Офис настройка АТС[/url]
Drugs information for patients. Brand names.
buy proscar without dr prescription
Best what you want to know about medicine. Read here.
ElonBet Casino’s rewards program feels genuinely rewarding.
elonbet casino
I wish online tied more—life in! minas
Заказала в другой город – все идеально!
101 роза
Just hit a big win at ElonBet—feeling on top of the world!
elonbet casino
взгляните на сайте здесь [url=https://xn—-jtbjfcbdfr0afji4m.xn--p1ai]электрик томск[/url]
[url=https://1winru.xyz]1вин сайт скачать[/url] – 1win регистрация, 1win top
The variety of slots at ElonBet caters to every taste. elonbet casino
Promo Code Use: Promo codes provide additional chances to win but may have wagering requirements and time limitations https://www.bloglovin.com/@seomypassion12/1xbet-registration-promo-code-bonus-euro130
[url=https://gamble-spot.com]казино россии онлайн[/url] – как играть в онлайн казино в россии, зеркала онлайн казино
Источник [url=https://xn—-jtbjfcbdfr0afji4m.xn--p1ai/]электрик томск[/url]
The table games online glow—sharp! book of ra
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – идеальный вариант для вас.
поставляемая продукция:
Медная твердая труба для кондиционеров 6.35С…1 РјРј Рњ1СЂ EN 12735 Приобретите медные трубы для кондиционеров РѕС‚ Редметсплав.СЂС„. Надежные, долговечные Рё устойчивые Рє РєРѕСЂСЂРѕР·РёРё трубы СЃ высокой теплопроводностью Рё простым монтажом. Рдеальный выбор для кондиционирования РІ РґРѕРјРµ, офисе или производственном помещении.
I won $300 on a slot—still stoked! plinko
проверить интернет по адресу
domashij-internet-samara003.ru
интернет провайдеры по адресу самара
Online casinos make gambling too accessible; it’s hard to stop sometimes. plinko
Купить диплом университета по доступной стоимости возможно, обращаясь к надежной специализированной фирме. Мы оказываем услуги по продаже документов об окончании любых университетов РФ. Купить диплом о высшем образовании– [url=http://vacshidiplom.com/kupite-originalnij-diplom-s-zaneseniem-v-reestr-8/]vacshidiplom.com/kupite-originalnij-diplom-s-zaneseniem-v-reestr-8/[/url]
The online ease rolls—stay wise! Book of Ra
cost cheap periactin without insurance
Drug information. Generic Name.
phenibut vs gabapentin
Best what you want to know about medicines. Read here.
I wish online paid fast—slow drag! plinko
Medicine prescribing information. Generic Name.
where buy tamoxifen without insurance
Some what you want to know about drugs. Read information now.
https://telegra.ph/Non-Gamstop-Casinos-No-Deposit-Bonuses-Explained-03-27
https://telegra.ph/Benefits-of-Non-Gamstop-No-Deposit-Casinos-03-27
прокат машин в сочи прокат автомобилей в сочи
прокат авто адлер arenda-mashiny-adler
РедМетСплав предлагает внушительный каталог высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их качество. Опытная поддержка – то, чем мы гордимся – мы на связи, чтобы ответить на ваши вопросы и адаптировать решения под требования вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
поставляемая продукция:
РљСЂСѓРі магниевый B 199 (QE22A) – ASTM B199 Фольга магниевая B 199 (QE22A) – ASTM B199 представляет СЃРѕР±РѕР№ высококачественный материал, используемый РІ различных областях, РѕС‚ машиностроения РґРѕ электроники. Рта фольга обеспечивает отличные механические свойства Рё РєРѕСЂСЂРѕР·РёРѕРЅРЅСѓСЋ стойкость, что делает её идеальным решением для разработки легких Рё прочных конструкций. Применение фольги магниевой гарантирует долговечность Рё надежность конечной продукции. РќРµ упустите возможность купить Фольга магниевая B 199 (QE22A) – ASTM B199 Рё повысить эффективность ваших проектов. Заказывайте СѓР¶Рµ сегодня!
аренда авто крым без водителя посуточно аренда авто сочи в крым
клининг помыть окна cleaning-top24.ru/
cost aurogra without dr prescription
Купить диплом любого университета!
Заказать диплом института по выгодной цене возможно, обратившись к проверенной специализированной фирме. Купить диплом: [url=http://diplomservis.com/kupit-diplom-s-reestrom-nedorogo-ofitsialno/]diplomservis.com/kupit-diplom-s-reestrom-nedorogo-ofitsialno[/url]
Посетите B2BOX https://b2box.ru/ – это уникальное комплексное решение для создания упаковки всех видов и направлений. Мы делаем упаковку, которая продает! Упаковочные решения для ритейла, создание индивидуальной и SRP упаковки, услуги дизайнерского бюро, производство транспортной упаковки, собственное плоттерное производство, все виды печати – это и многое другое вы найдете, посетив наш сайт.
I wish online tied more—life in! teen patti
Online poker fires—bluff rush! Jackpot Raider
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наша продукция:
Керамическая мишень для распыления диоксида кремния
посмотреть на этом сайте [url=https://xn—-jtbjfcbdfr0afji4m.xn--p1ai]электрик томск[/url]
I lost track of time playing online—hours gone in a blink! gransino
I won big online—still high! plinko
Где приобрести [b]диплом[/b] по нужной специальности?
Получаемый диплом с необходимыми печатями и подписями полностью отвечает стандартам, никто не сумеет отличить его от оригинала – даже со специальным оборудованием. Не следует откладывать личные цели на несколько лет, реализуйте их с нами – отправляйте быструю заявку на изготовление диплома прямо сейчас! Диплом о среднем специальном образовании – быстро и легко! [url=http://graph.org/Kupit-diplom-bystro-i-nedorogo-03-28/]graph.org/Kupit-diplom-bystro-i-nedorogo-03-28[/url]
Посетите сайт Мир Часов https://mir-watch.ru/ – это скупка часов в Москве. Вы сможете сдать часы в ломбард по выгодной стоимости. Занимаемся выкупом (скупкой) швейцарских часов и других известных брендов. Выкупаем не только элитные часы, но и часовые аксессуары: виндеры, коробки, шкатулки, ремни, ювелирные украшения и аксессуары с бриллиантовыми камнями.
Medicines information sheet. Cautions.
should pantoprazole be taken with or without food
Actual about medicament. Read information now.
Купить документ ВУЗа можно в нашей компании. Мы предлагаем документы об окончании любых ВУЗов России. Вы получите необходимый диплом по любым специальностям, любого года выпуска, в том числе документы образца СССР. Гарантируем, что в случае проверки документов работодателем, каких-либо подозрений не возникнет. [url=http://fastdiploms.com/diplom-bakalavra-s-zaneseniem-v-reestr-3/]fastdiploms.com/diplom-bakalavra-s-zaneseniem-v-reestr-3/[/url]
Medicines information sheet. Drug Class.
hydrochlorothiazide side effects in pregnancy
All trends of pills. Read information here.
хранить вещи в москве недорого склады цена [url=http://www.hranenieveshey.ru]хранить вещи в москве недорого склады цена[/url] .
can i get cheap avodart without rx
The slot sounds online lift—good time! dragon tiger
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
С широким ассортиментом продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наша продукция:
Мишень для распыления ванадия
Online poker is hot—bluff thrill! andar bahar
нажмите [url=https://xn—-jtbjfcbdfr0afji4m.xn--p1ai/]электрик томск[/url]
Online casinos are too smooth—watch out! mines game
Faça Seu Cadastro no umbet
e Ganhe 100$ de Bônus Agora Mesmo!
https://telegra.ph/Non-Gamstop-Casinos-No-Deposit-Bonus-Offers-Explained-03-27
https://telegra.ph/Top-Non-Gamstop-Casinos-You-Should-Know-in-2019-03-27
The online bonuses are hot—dive in! Betclic
Мы можем предложить дипломы любой профессии по приятным ценам. Всегда стараемся поддерживать для заказчиков адекватную политику тарифов. Важно, чтобы документы были доступными для большого количества наших граждан.
Приобретение документа, подтверждающего обучение в университете, – это грамотное решение. Приобрести диплом университета: [url=http://1magistr.ru/kupit-v-pitere-diplom-3/]1magistr.ru/kupit-v-pitere-diplom-3/[/url]
get mentat price
https://telegra.ph/No-Gamstop-Casinos-Safe-Alternatives-for-Players-03-27
Купить диплом института!
Мы предлагаем дипломы любой профессии по доступным ценам— [url=http://diplom-top.ru/kupit-diplom-o-visshem-obrazovanii-reestr-bistro-i-udobno-2/]diplom-top.ru/kupit-diplom-o-visshem-obrazovanii-reestr-bistro-i-udobno-2/[/url]
Medicine prescribing information. What side effects can this medication cause?
where buy dutasteride prices
All trends of pills. Get here.
Highly impressed with the MetaMask Extension. It’s reliable, secure, and a must for anyone engaging in blockchain transactions.
Ao se cadastrar no aajogo, você ganha um bônus
de 100$ para começar sua jornada no cassino com o pé direito!
Não importa se você é um novato ou um apostador experiente, o bônus de boas-vindas é a oportunidade perfeita para explorar todas as
opções que o site tem a oferecer. Jogue seus jogos favoritos, descubra novas opções de apostas e aproveite para testar estratégias sem
risco, já que o bônus ajuda a aumentar suas chances
de ganhar. Cadastre-se hoje e comece com 100$!
https://telegra.ph/Phone-Bill-Casino-Top-Up-Without-Gamstop-Restrictions-03-27
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
Наша продукция:
Мишень РёР· драгоценного металла или его сплава кольцевая платина 35С…2 РјРј РџР»Р95-5 РўРЈ Выберите уникальные мишени РёР· драгоценных металлов для напыления РѕС‚ Редметсплав.СЂС„. Обеспечивают высокую степень защиты Рё долговечность. Рдеальный выбор для промышленности, строительства Рё производства.
Aproveite a oferta exclusiva do betsul para novos
usuários e receba 100$ de bônus ao se registrar! Este bônus de boas-vindas permite
que você experimente uma vasta gama de jogos de cassino online
sem precisar gastar imediatamente. Com o bônus de 100$, você poderá explorar jogos
como roleta, blackjack, caça-níqueis e muito mais, aumentando suas chances de vitória
desde o primeiro minuto. Não perca essa chance única
de começar com um valor significativo – cadastre-se agora!
I wish online helped newbies—lost start! plinko
Купить диплом университета!
Мы предлагаем документы об окончании любых университетов Российской Федерации. Документы изготавливаются на оригинальных бланках государственного образца. [url=http://career.digitalgurukul.in/employer/radiplomy/]career.digitalgurukul.in/employer/radiplomy[/url]
The rewards online are solid—nice perk! gold party
https://telegra.ph/Top-UK-Casinos-Not-on-Gamstop-for-2023-03-27
Loved this! Super helpful and easy to follow. Thanks for sharing!
can i order generic lipitor no prescription
узнать провайдера по адресу уфа
domashij-internet-ufa002.ru
провайдеры по адресу
РедМетСплав предлагает внушительный каталог качественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица товара подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Превосходное обслуживание – наш стандарт – мы на связи, чтобы ответить на ваши вопросы а также предоставлять решения под требования вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
Наша продукция:
Пруток магниевый EN-MB10021 – EN 12421:1998 Проволока магниевая EN-MB10021 – EN 12421:1998 представляет СЃРѕР±РѕР№ высококачественный РїСЂРѕРґСѓРєС‚, идеально подходящий для различных промышленных применений. Обладая отличными механическими свойствами Рё высокой РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью, РѕРЅР° обеспечивает надежность Рё долговечность РІ эксплуатации. Рта проволока соответствует строгим стандартам качества, что делает ее идеальным выбором для заказчиков, требующих надежных материалов. РќРµ упустите возможность улучшить СЃРІРѕРё проекты СЃ помощью proven solutions. Купить Проволока магниевая EN-MB10021 – EN 12421:1998 РјРѕР¶РЅРѕ РїСЂСЏРјРѕ сейчас, обеспечив себя лучшими материалами для работы!
Medication prescribing information. Long-Term Effects.
teva pantoprazole effet secondaire
Best news about medicament. Read here.
Заказать диплом ВУЗа по невысокой цене возможно, обратившись к проверенной специализированной фирме. Купить документ университета вы имеете возможность в нашей компании в столице. [url=http://dip-lom-rus.ru/prostoj-sposob-registratsii-diploma-bez-lishnix-xlopot/]dip-lom-rus.ru/prostoj-sposob-registratsii-diploma-bez-lishnix-xlopot[/url]
Aposte com 100$ de Bônus no brxbet –
Cadastre-se e Aproveite!
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с высоким уровнем сервиса.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наши товары:
Кольцо РёР· драгоценных металлов серебряное 200С…100С…4 РјРј РЎСЂРњ50 РўРЈ РЁРёСЂРѕРєРёР№ выбор высококачественных кольц РёР· драгоценных металлов. Рлегантные украшения, созданные талантливыми мастерами. Уникальность Рё изысканность РІ каждой детали. Возможность заказа персонального кольца РїРѕ вашим пожеланиям. Подчеркните СЃРІРѕСЋ индивидуальность СЃ нашими кольцами.
ashwagandha lehyam benefits
Online casinos need better self-control tools. tome of madness
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Обладая обширной линейкой редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым текущие трудности превращаются в завтрашние возможности.
Наша продукция:
Керамическая мишень Bn 2N5 (плоская)
Drugs information. What side effects can this medication cause?
cost of cheap zithromax no prescription
Some news about drugs. Get here.
мостбет кыргызстан [url=http://mostbet6033.ru]мостбет кыргызстан[/url] .
I hit a bad streak on online slots—RNG feels rigged sometimes! plinko
РедМетСплав предлагает широкий ассортимент высококачественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица продукции подтверждена всеми необходимыми документами, подтверждающими их качество. Опытная поддержка – то, чем мы гордимся – мы на связи, чтобы разрешать ваши вопросы а также адаптировать решения под специфику вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
Порошок магниевый SISMg4635-10 – SS 144635 Рзделия РёР· магния SISMg4635-10 – SS 144635 обеспечивают высокую прочность Рё легкость, что делает РёС… идеальными для применения РІ различных отраслях. Магний обладает выдающимися характеристиками, такими как коррозионная стойкость Рё отличная обрабатываемость. Рти изделия идеально РїРѕРґС…РѕРґСЏС‚ для автоматизации процессов Рё производственного оборудования. Если РІС‹ ищете надежные компоненты, купить Рзделия РёР· магния SISMg4635-10 – SS 144635 будет отличным выбором для повышения эффективности ваших проектов. Обратите внимание РЅР° РёС… превосходные эксплуатационные свойства.
Seu Registro no dobrowin Vale 100$ de Bônus Imediato!
Ganhe 100$ ao se Registrar no fezbet e Aposte de Forma Fácil!
Medicines information leaflet. What side effects?
cost cheap proscar without prescription
All news about drugs. Read now.
аренда машины в минеральных водах без водителя аренда машины минеральные воды без водителя посуточно
аренда автомобиля в москве и области авто прокат автомобилей в москве
аренда машины храброво калининград arenda-avto-hrabrovo.ru
I hit a blackjack wave—good run! Vipzino Casino
how to get clomid without prescription
Online casino ads roar—hush down! aviator
интернет по адресу
domashij-internet-ufa003.ru
провайдер интернета по адресу уфа
скачать мостбет официальный сайт [url=www.mostbet6036.ru]www.mostbet6036.ru[/url] .
mostbest [url=https://mostbet5002.ru]https://mostbet5002.ru[/url] .
технический план помещения
where to get generic lopid without a prescription
Запуск в мир ставок начинается с одного клика — и ты уже в космосе. Всё вокруг тихо, интерфейс минималистичный.
Слоты — это гиперпрыжки, и каждый запускается без задержек. В этом цифровом пространстве [url=https://www.nhkm.ru]https://www.nhkm.ru[/url] — точка входа, где всё рассчитано.
Пополнение — как загрузка топлива, а выигрыш — чёткий.
Поддержка помогает на высоте. Атмосфера — тишина галактики — именно то, что нужно для полного погружения.
Где купить диплом по актуальной специальности?
Наша компания предлагаетвыгодно приобрести диплом, который выполнен на бланке ГОЗНАКа и заверен печатями, штампами, подписями. Данный диплом способен пройти любые проверки, даже при использовании специфических приборов. Достигайте своих целей максимально быстро с нашей компанией. Заказать диплом любого университета! [url=http://forum.csharing.org/viewtopic.php?f=2&t=1740/]forum.csharing.org/viewtopic.php?f=2&t=1740[/url]
скачать mostbet на телефон [url=http://mostbet6033.ru/]http://mostbet6033.ru/[/url] .
Awesome content as always. Keep up the great work!
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
поставляемая продукция:
Лента РёР· прецизионных сплавов для СѓРїСЂСѓРіРёС… элементов 2×48 РјРј РРџ414 ГОСТ 14117-85 Купите ленту РёР· прецизионных сплавов для СѓРїСЂСѓРіРёС… элементов РїРѕ выгодной цене РЅР° Редметсплав.СЂС„. Высокая прочность, устойчивость Рє РєРѕСЂСЂРѕР·РёРё Рё отличная эластичность делают этот материал идеальным для различных отраслей. Предлагаем широкий ассортимент продукции Рё РїРѕРґСЂРѕР±РЅСѓСЋ информацию для правильного выбора.
The slot themes online pop—play fun! gates of olympus
Заказать диплом об образовании. Покупка официального диплома через надежную компанию дарит ряд достоинств для покупателя. Такое решение помогает сэкономить как длительное время, так и серьезные финансовые средства. [url=http://uconnect.ae/read-blog/261290/]uconnect.ae/read-blog/261290[/url]
I’m leery of online casinos—too many scams! mines
Medicament prescribing information. Drug Class.
spironolactone and aging skin
Best information about pills. Read information here.
The rewards online glow—nice lift! plinko
Online casinos should shield—watch us! fortune tiger
РедМетСплав предлагает внушительный каталог качественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица продукции подтверждена требуемыми документами, подтверждающими их соответствие стандартам. Дружелюбная помощь – наша визитная карточка – мы на связи, чтобы разрешать ваши вопросы и адаптировать решения под специфику вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
поставляемая продукция:
РўСЂСѓР±Р° магниевая B 199 (AZ91E) – ASTM B199 Пруток магниевый B 199 (AZ91E) – ASTM B199 – это высококачественный легкий сплав, который идеально РїРѕРґС…РѕРґРёС‚ для применения РІ различных отраслях. РћРЅ отличается отличной РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью Рё высокой прочностью, что делает его идеальным выбором для производства деталей, работающих РІ условиях высокой нагрузки. Ртот РїСЂРѕРґСѓРєС‚ обладает хорошими механическими свойствами Рё обработкой. Если РІС‹ ищете надежный Рё прочный материал, РЅРµ сомневайтесь, купить Пруток магниевый B 199 (AZ91E) – ASTM B199 станет отличным решением для ваших задач. РќРµ упустите возможность использовать передовой сплав РІ СЃРІРѕРёС… проектах!
how to get generic nexium for sale
клининг после стройки клининг уборка после ремонта
домашний интернет тарифы
domashij-internet-chelyabinsk001.ru
лучший интернет провайдер челябинск
Prodamus -промокод на подключение [url=https://promokod-prdms.ru/]Prodamus -промокод на подключение [/url] .
seo криптопроекта [url=www.prodvizhenie-kriptovalyuta1.ru/]www.prodvizhenie-kriptovalyuta1.ru/[/url] .
Купить диплом института по выгодной стоимости вы можете, обратившись к надежной специализированной фирме. Приобрести документ университета можно в нашей компании в столице. [url=http://vuz-diplom.ru/kupit-diplom-s-registratsiej-v-reestre-bistro-i-udobno-7/]vuz-diplom.ru/kupit-diplom-s-registratsiej-v-reestre-bistro-i-udobno-7[/url]
Online casinos should rush—too wait! book of ra
buying generic trazodone pill
Потолки для вашего дома от natyazhnye-potolki-dnepr.biz.ua , где ваша фантазия воплощается в жизнь, позаботьтесь о своем интерьере, инвестируйте в красоту и комфорт.
Элегантные натяжные потолки для вашего дома, инновационные технологии, которые сделают ваш интерьер уникальным, узнайте больше на сайте.
Экономьте с умом с натяжными потолками, с качеством, проверенным временем, оставьте позади скучные потолки, сделайте выбор в пользу качества.
Индивидуальный подход к каждому клиенту, потолки, которые вдохновляют, превратите мечты в реальность, машина времени в мир красоты.
Натяжные потолки для квартир и офисов, по европейским стандартам, на любой вкус и цвет, у нас широкий ассортимент.
Почему стоит выбрать натяжные потолки?, для вашего дома, долговечность и надежность, обеспечьте себе комфорт.
Эстетические решения для вашего потолка, где стиль и качество соединяются, превратите потолок в произведение искусства, заказ натяжного потолка стал проще.
Натяжные потолки: легко и удобно, от natyazhnye-potolki-dnepr.biz.ua, выбирайте лучшее для себя, свой идеальный потолок в 3 шага.
Качество натяжных потолков — наш приоритет, от natyazhnye-potolki-dnepr.biz.ua, поддержка и забота о клиенте, вдохните жизнь в ваше пространство.
Натяжные потолки по доступным ценам, от производителей, где ваши мечты становятся реальностью, позвоните нам сегодня.
Преобразите свое пространство с натяжными потолками, в Днепре, всегда профессионально, начните свой проект с нами.
Натяжные потолки для каждого интерьера, от наших профессионалов, выбор, который вдохновляет, обратите внимание на наши предложения.
Ваш надежный партнер в мире натяжных потолков, с индивидуальным подходом к каждому клиенту, долговечность и стиль, присоединяйтесь к нам.
Эстетика и функциональность натяжных потолков, с заботой о каждом клиенте, всё для вашего комфорта и стиля, узнайте больше на нашем сайте.
Ваш потолок — ваша гордость, потолки для любого стиля, поддержка профессионалов на каждом этапе, воспользуйтесь нашим опытом.
Преимущества натяжных потолков в вашем интерьере, от natyazhnye-potolki-dnepr.biz.ua, всё для вашего комфорта, сделайте правильный выбор.
natyazhnye-potolki-dnepr.biz.ua [url=https://natyazhnye-potolki-dnepr.biz.ua/]natyazhnye-potolki-dnepr.biz.ua[/url] .
Medication information for patients. Long-Term Effects.
where can i buy macrobid
Actual what you want to know about medicine. Read information now.
На сайте http://oknaksa.ru закажите расчет технического задания, чтобы воспользоваться такой важной, полезной услугой, как остекление. На монтажные работы, а также сами конструкции предоставляются гарантии, вы сможете воспользоваться бесплатным обслуживанием. А все работы выполняются точно по ГОСТу, в соответствии с требованиями. Предоставляются исчерпывающие и содержательные консультации. Вам будет предложено несколько вариантов решения проблемы. Компания располагает огромным количеством готовых проектов.
mostbet kg скачать на андроид [url=mostbet5001.ru]mostbet5001.ru[/url] .
Tent3302.ru предлагает необходимые автодетали. Мы продаем запчасти на Газель Некст. Под заказ поставляем двигатель Газель Камминз 2.8. Стараемся цены на низком уровне поддерживать. Осуществляем продажи деталей со склада за наличный и безналичный расчет. Ищете редуктор валдай редуктор заднего моста валдай? Tent3302.ru – здесь можете найти подробную информацию о нас. Мы качественную продукцию продаем. Шины на Газель особенным спросом у клиентов пользуются. Стараемся максимально сократить транспортные и накладные расходы. Вы можете быть уверены в вежливом и грамотном обслуживании.
На сайте https://pilmi.ru вы найдете огромное количество фильмов самых разных жанров, включая комедии, мелодрамы, драмы, романтические. Для того чтобы подыскать наиболее подходящий вариант, нужно воспользоваться специальным каталогом. Вашему вниманию представлены и ожидаемые любопытные новинки этого года, которые произведут эффект. Все это можно просматривать в любое время и на различных устройствах. Прямо сейчас воспользуйтесь возможностью посмотреть азиатское кино. Используйте поиск для облегчения выбора.
склад для хранения одежды [url=https://hranenieveshey.ru/]склад для хранения одежды[/url] .
Looking for gifts to Ukraine? Ukraineflora.com/ offers to get acquainted with flower delivery in Ukraine, here is the best choice for various events. We have a large assortment of flowers in Ukraine! Look at it now, we are sure you will like everything. Now it is not difficult to make flower delivery in Ukraine, just go to the portal and get acquainted with our offers in more detail, here are fresh flowers, bouquets, and baskets. You can also send gifts to Ukraine. Gift delivery in Ukraine is an opportunity to please your relatives even more.
Ищете сельхозтехнику и оборудование по самым выгодным ценам от производителя? Посетите сайт Производителя ООО МИТЕХ https://mi-teh.ru/ где вы также найдете запасные части и комплектующие к сельхоз машинам и тракторам. Ознакомьтесь с нашим ассортиментом продукции на сайте.
The poker online shines—real kick! jogo plinko
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наша продукция:
Плоская мишень селена 4N, 5N
скачат мостбет [url=www.mostbet5002.ru]www.mostbet5002.ru[/url] .
Looking to transform your style with authentic dreadlocks? Check out our range of dread natural at the site – real dreadlock extensions, offering the highest quality options for achieving a flawless, natural look.
Carefully designed using ethically sourced hair, these dreadlocks are a great match for self-expression through hair. Whether you’re into full-head transformations, we have options that blend with all hair types.
Find your fit with:
– dread natural
– handmade dreadlocks
Make a statement with premium-quality extensions that look and feel real. Fast shipping available across the USA and beyond!
Claim yours today – your dream style awaits.
Перевозка негабаритных грузов и доставка по всей территории России и СНГ на сайте https://negabarit-24.ru/ – это профессиональные услуги от нашей компании. Перевозка негабаритных грузов по России и странам СНГ, ЖД перевозки, перевозки манипулятором и международные перевозки. Гибкие варианты оплаты. Соблюдение сроков. Страхование грузов. Подробнее на сайте.
The mobile casino experience is spotty—fix it! dragon tiger
Букет шикарный! Все цветы как живые.
доставка цветов в томске
Заказать диплом о высшем образовании !
Покупка диплома любого университета России в нашей компании является надежным делом, потому что документ заносится в реестр. Приобрести диплом об образовании [url=http://diplom-top.ru/kupite-diplom-o-srednem-obrazovanii-bistro-i-bez-truda/]diplom-top.ru/kupite-diplom-o-srednem-obrazovanii-bistro-i-bez-truda[/url]
Online casinos need rules—too free! marvel casino
Thanks for the valuable information. It really helped me understand the topic better.
Psoriasis symptom management strategies and techniques
Medicine information. Effects of Drug Abuse.
buying thorazine tablets
All trends of medicines. Read here.
Подробнее здесь https://xn—–6kcacs9ajdmhcwdcbwwcnbgd13a.xn--p1ai/
Заказать диплом любого ВУЗа!
Мы готовы предложить документы университетов, которые расположены на территории всей Российской Федерации. Документы выпускаются на “правильной” бумаге самого высокого качества: [url=http://notebooks.ru/forum/user/143284/]notebooks.ru/forum/user/143284[/url]
Подробнее https://falcoware.com/rus/match3_games.php
продамус промокод скидка [url=https://promokod-prdms.ru]продамус промокод скидка[/url] .
подключение интернета челябинск
domashij-internet-chelyabinsk002.ru
лучший интернет провайдер челябинск
продвижение криптовалюты [url=https://prodvizhenie-kriptovalyuta1.ru]продвижение криптовалюты[/url] .
Купить диплом на заказ в столице возможно через сайт компании. [url=http://18plus.fun/read-blog/3609_gde-kupit-diplom-s-zaneseniem-v-reestr.html/]18plus.fun/read-blog/3609_gde-kupit-diplom-s-zaneseniem-v-reestr.html[/url]
dapagliflozina plm
My-articles-online как киллеры находят заказы
The rewards online glow—nice lift! plinko
I am a student living in Ditse. I am a fan of technology, design and music. I am also interested in entrepreneurship and coffee. You can support my campaign by clicking the button above Квесты Одесса 2025
That feeling when you hit a jackpot online is unbeatable! dragon tiger
I love the live roulette—live vibe! plinko
I hit a bonus online and won $600—sweet score! mines
mostbet игры [url=https://mostbet5001.ru/]https://mostbet5001.ru/[/url] .
Отказное письмо https://www.rospromtest.ru/sertifikati/otkaznoe-pismo/ — документ, который подтверждает, что товару не требуется сертификат или декларация о соответствии. Отказное письмо оформляется на основании данных от заявителя без подачи заявки на сертификацию, эксперт на основании области применения продукции и ее кода ТН ВЭД определяет необходимость обязательной сертификации, о чем предоставляется ответ в письменном виде. Отказное письмо называют информационным письмом.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние возможности.
Наши товары:
Высокочистые гранулы тантала
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наши товары:
Латунная полоса 1.1×100 РјРј Р›63 ГОСТ 931-90 Покупайте латунную полосу различных размеров Рё толщин РЅР° сайте Редметсплав.СЂС„. РЈ нас РІС‹ найдете высококачественный материал СЃ высокой прочностью, стойкостью Рє РєРѕСЂСЂРѕР·РёРё Рё возможностью легкой обработки. Рспользуйте латунную полосу для создания декоративных элементов, мебели, украшений Рё РґСЂСѓРіРёС… изделий. Доступны различные размеры Рё толщины.
Time1c.ru 1С предлагает купить. В нашей команде исключительно проверенные временем профессионалы. Они готовы проконсультировать вас. Вы можете протестировать решение 1С в облаке. Заполните уже сейчас на нашем портале форму. Укажите ваше имя, телефон для связи и e-mail адрес. Ищете 1с управление ритуальными услугами? Time1c.ru – можете с ценами ознакомиться. Также тут есть удобный поиск и блог. Расскажем, как 1С ERP помогает управлять проектами и ресурсами. Вы узнаете о преимуществах использования 1С Бухгалтерия для строительной отрасли. Настало время бизнес развивать!
Are online ratings real?—tough call! plinko
google play отзывы [url=http://prodvizhenie-kriptovalyuta.ru]google play отзывы[/url] .
Играйте ответственно и выигрывайте по-божески в Cryptoboss Casino crypto boss casino бонус
The live games online are slick—big fan! mines juego
Online casinos make gambling too easy—scary sometimes! Ruleta americana
Check out our blog https://nashvillerelocationservices.com/ – it’s a valuable resource for all things relocating in the US. Whether you’re moving down the block or across the country, we give you practical, simple advice that really works. All articles follow a quick-answer format so you’ll actually get the knowledge you need.
can you get generic femara without dr prescription
mostbest [url=https://mostbet5003.ru]mostbest[/url] .
РедМетСплав предлагает широкий ассортимент отборных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица изделия подтверждена соответствующими документами, подтверждающими их происхождение. Дружелюбная помощь – то, чем мы гордимся – мы на связи, чтобы разрешать ваши вопросы а также предоставлять решения под специфику вашего бизнеса.
Доверьте ваш запрос специалистам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
Кобальт Wallex 20/1040 Кобальт Wallex 20/1040 – это высококачественный инструмент, который идеально РїРѕРґС…РѕРґРёС‚ для различных работ. Его прочный РєРѕСЂРїСѓСЃ Рё надежные характеристики обеспечивают долгий СЃСЂРѕРє службы Рё удобство РІ использовании. Ртот РїСЂРѕРґСѓРєС‚ имеет отличный баланс, что позволяет работать даже РІ труднодоступных местах. Если РІС‹ ищете надежный инструмент, РЅРµ упустите шанс купить Кобальт Wallex 20/1040. РћРЅ станет незаменимым помощником РІ ремонте Рё строительстве. Обеспечьте себе комфорт Рё качество работы СЃ этим выдающимся инструментом.
Piano sheet music sheet music
best ai porn ai porn
Online casinos slide easy—mind it! tom of madness
Noten musik klavier noten auf klavier
подключить проводной интернет челябинск
domashij-internet-chelyabinsk003.ru
подключить интернет в квартиру челябинск
Полезная информация cvetkart.ru свежие материалы и удобная навигация — всё, что нужно для комфортного пользования сайтом. Заходите, изучайте разделы и находите то, что действительно важно для вас.
Заказать диплом любого университета. Приобретение документа о высшем образовании через надежную фирму дарит ряд преимуществ для покупателя. Данное решение позволяет сэкономить время и серьезные денежные средства. [url=http://taro.kabb.ru/search.php/]taro.kabb.ru/search.php[/url]
перейдите на этот сайт https://xn—–6kcacs9ajdmhcwdcbwwcnbgd13a.xn--p1ai/
Online casinos feel like a gamble on top of a gamble! plinko
Medication information for patients. What side effects can this medication cause?
how to buy generic loperamide
Actual information about medicament. Get information now.
cipro online order
продамус промокод скидка [url=http://www.promokod-prdms.ru]продамус промокод скидка[/url] .
google play купить отзывы [url=https://prodvizhenie-kriptovalyuta1.ru/]google play купить отзывы[/url] .
здесь https://xn—–6kcacs9ajdmhcwdcbwwcnbgd13a.xn--p1ai/prajs-list
Online casinos aid practice—nice try! plinko
купить диплом о среднем образовании в туле
продолжить https://xn—-jtbjfcbdfr0afji4m.xn--p1ai/
узнать https://falcoware.com/rus/match3_games.php
I won a tourney online—wild high! jackpot raider
посетить веб-сайт https://falcoware.com/rus/match3_games.php
Подробнее https://xn—–6kcacs9ajdmhcwdcbwwcnbgd13a.xn--p1ai
Смотреть здесь https://falcoware.com/rus/match3_games.php
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – идеальный вариант для вас.
Наши товары:
Нержавеющий штрипс 1.5 РјРј AISI 439 ГОСТ 4986-79 Приобретите нержавеющий штрипс высокого качества для разнообразных областей применения РЅР° Редметсплав.СЂС„. РЁРёСЂРѕРєРёР№ ассортимент различных размеров Рё толщин, включая услуги нарезки РїРѕРґ ваш заказ. Рзбегайте проблем СЃ коррозией Рё обеспечьте долгий СЃСЂРѕРє службы вашим конструкциям Рё деталям, используя наш надежный материал.
seo крипты [url=www.prodvizhenie-kriptovalyuta.ru/]seo крипты[/url] .
Мы готовы предложить дипломы любой профессии по невысоким ценам. Дипломы изготавливаются на подлинных бланках Приобрести диплом о высшем образовании [url=http://diplomt-v-samare.ru/]diplomt-v-samare.ru[/url]
аренда авто авто взять где прокат
Оптовый интернет – магазин керамический плитки и керамогранита alliance-plitka Керамогранит опт
Где заказать диплом специалиста?
Приобрести диплом университета по невысокой стоимости вы можете, обратившись к надежной специализированной фирме.: [url=http://kazdiplomas.com/]kazdiplomas.com[/url]
аренда машины владивосток аренда авто на месяц владивосток
прокат машин в махачкале сколько стоит аренда машины в махачкале
машина в аренду санкт петербург на неделю авто в аренду на сутки спб
The mobile apps for online casinos crash way too often! plinko
Medication information. Generic Name.
can you get minocycline pills
Some trends of meds. Get information now.
cost bactrim without dr prescription
Смотреть здесь https://xn—-jtbjfcbdfr0afji4m.xn--p1ai/
I won big online—wild joy! plinko
интернет по адресу дома
domashij-internet-krasnoyarsk001.ru
провайдеры по адресу дома
Посетите сайт https://schetki.spb.ru/ и вы сможете подобрать и купить машинку для чистки обуви. Ознакомьтесь с нашим ассортиментом машинок для чистки обуви – для дома или офиса, для чистки подошвы обуви, бахилонадевателями, комплектующими и другим ассортиментом. Также обслуживаем и предоставляем в аренду машины для чистки обуви и аппараты для надевания бахил. Доставляем по всем регионам России.
РедМетСплав предлагает широкий ассортимент качественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица изделия подтверждена всеми необходимыми документами, подтверждающими их качество. Дружелюбная помощь – наш стандарт – мы на связи, чтобы улаживать ваши вопросы и адаптировать решения под специфику вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
Наши товары:
РџРѕРєРѕРІРєР° молибденовая 8 РџРѕРєРѕРІРєР° молибденовая 8 – это высококачественный металл, обладающий превосходными механическими свойствами Рё термостойкостью. Молибден используется РІ производстве инструментов Рё деталей, которые должны выдерживать высокие температуры Рё нагрузки. Рспользование РїРѕРєРѕРІРєРё обеспечивает надежность Рё долговечность изделий. Р’С‹ можете использовать РџРѕРєРѕРІРєР° молибденовая 8 РІ различных отраслях, включая авиацию Рё машиностроение. Если РІС‹ ищете материал, который сочетает РІ себе качество Рё эффективность, вам стоит купить РџРѕРєРѕРІРєР° молибденовая 8. Сделайте правильный выбор для вашего бизнеса.
На сайте http://www.lilibum.ru вы найдете много анкет реальных людей для того, чтобы завести знакомства, найти свою вторую половинку и просто интересно провести время, если пригласите кого-то на свидание. Для того чтобы составить мнение о человеке, можно обменяться фотографиями и пообщаться на определенные темы, которые вас интересуют. Самые привлекательные и интересные люди вашего города находятся на этом портале. Знакомьтесь, влюбляйтесь и создавайте семьи. Для получения доступа ко всем функциям, пройдите регистрацию.
Uniswap is revolutionizing decentralized finance by offering a frictionless, permissionless way to swap tokens. With the Uniswap protocol, users can trade directly from their Uniswap wallet—no intermediaries required uniswap bridge
Заказать диплом на заказ в Москве возможно используя сайт компании. [url=http://jobteck.com/companies/frees-diplom/]jobteck.com/companies/frees-diplom[/url]
where to buy generic cialis soft tabs no prescription
https://www.proekt.media/investigation/sosed-boris-listov/
Online casino ads are too much—chill! Fortune Dragon
I love the online calm—no buzz! plinko
Если вы хотите быть в курсе самой актуальной, ценной и нужной информации относительно трейдинга и инвестиций, то скорее посетите этот полезный портал, на котором вы найдете все, что нужно на данную тему. Здесь доступным языком рассказывается о том, как правильно вести торговлю на внебиржевом рынке. https://fxidea.com/ рекомендует воспользоваться акциями каждому новичку. Быстрее изучите индикаторы, реальные отзывы, а также ознакомьтесь с работающими стратегиями. Вы получите доступ к ценным материалам, касающимся криптовалюты, а также узнаете, как заработать на форексе.
Доверие – центр, который психологические услуги предоставляет. Мы помогаем людям справляться с трудностями, которые у них возникают в отношениях с собой и окружающими. Записаться на личный прием к специалисту можно по телефону, указанному на сайте. Ищете психотерапия? Ack-group.ru/stati-po-psikhologii/psikhoterapiya – здесь узнаете, что такое психотерапия, какие цели перед ней стоят и кому она показана. Также здесь на психологические консультации представлены расценки. Всегда готовы поддержать вас на пути к самопознанию и эмоциональному равновесию.
Online casinos should limit how much you can lose in a day. F7 Casino
mostbet [url=https://mostbet6033.ru/]https://mostbet6033.ru/[/url] .
Центр ортодонтии и эстетической стоматологии в Москве https://ortodont-msk.com/ это квалифицированные услуги от врачей ортодонтов высшей категории, которые занимают первое место в рейтинге врачей ортодонтов в Москве. Посетите наш сайт, ознакомьтесь с нашими услугами, такими как исправление прикуса взрослым и детям, нашими брекет системами и другими услугами, галереей работ.
Medication prescribing information. Effects of Drug Abuse.
difference between phenergan and promethazine
Everything about pills. Read here.
страница https://falcoware.com/rus/match3_games.php
Are online jackpots real?—skeptic! casino kingdom
подробнее https://falcoware.com/rus/match3_games.php
управление репутацией криптовалюты [url=prodvizhenie-kriptovalyuta2.ru]prodvizhenie-kriptovalyuta2.ru[/url] .
coinmarketcap отзывы [url=https://prodvizhenie-kriptovalyuta.ru/]coinmarketcap отзывы[/url] .
colchicine overdose symptoms
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наша продукция:
Гранулы сульфида цинка 4N
проверить интернет по адресу
domashij-internet-krasnoyarsk002.ru
какие провайдеры на адресе в красноярске
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наша продукция:
“ерамическая мишень CeO2, плоская форма
I love the online twists—keep fresh! bongo bongo
The slot thrill online is intense—wow! jackpot raider
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наша продукция:
Плоская мишень Ni+Cu 3N
can you buy clarinex without a prescription
Online bingo flows—great crew! minas
Ganhe 100$ para Apostar no brdice
ao Se Registrar Hoje!
Компания “Развитие” это опытный поставщик сельхозоборудования с опытом. На сайте razvitieagro.ru представлен большой ассортимент продукции для агробизнеса, птицефабрик, овощехранилищ и парников. Мы поставляем технику для свинокомплексов, коровников, молочных ферм, систем микроклимата, автоматического управления и подачи корма. Вся техника сертифицирована и удовлетворяет потребности современной фермы. Работаем с заводов-изготовителей — без наценок и сервисом.
Если вы рассматриваете определённые позиции — вы обратились правильно. У нас можно найти всё, вплоть до таких нишевых позиций, как [url=https://razvitieagro.ru/]производство оборудования для птицеводства[/url] которое гарантирует равномерный поток воздуха в закрытых помещениях. Также в наличии системы обогрева, системы подсветки, увлажнители, инкубаторы, оборудование для чистки животных, системы поения, кормушки, подающие механизмы, малогабаритные цеха по производству комбикорма и весь спектр техники. Весь ассортимент — в наличии, логистика по всей России.
Наша компания находится по адресу: г. Ижевск, ул. Маркина 197. Если ваша задача — [url=https://razvitieagro.ru/]инкубатор купить цена[/url] или приобрести комплексное оборудование для предприятия, мы поможем найти лучший вариант. Подберем, рассчитаем и отгрузим — всё оперативно, по договору и с профессиональным сопровождением.
Pills information. Drug Class.
how to buy generic dilantin without rx
Actual trends of meds. Read now.
мостбет официальный сайт [url=http://mostbet6033.ru/]мостбет официальный сайт[/url] .
Are online reviews legit?—hard say! plinko
купить диплом о среднем образовании в уфе
Online casino tournaments are intense—anyone else hooked? betmomo
orm криптопроекта [url=http://prodvizhenie-kriptovalyuta2.ru/]http://prodvizhenie-kriptovalyuta2.ru/[/url] .
I hit a low online—rest up! plinko
where buy diovan tablets
The slot sounds online lift—good time! plinko
узнать провайдера по адресу красноярск
domashij-internet-krasnoyarsk003.ru
интернет провайдеры по адресу дома
buy generic prilosec without a prescription
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с прекрасным качеством обслуживания.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
Наши товары:
Латуное наружное стопорное РїСЂСѓР¶РёРЅРЅРѕРµ кольцо M140 133С…4 РјРј ЛЦ40РњС†1.5 DIN 471 Латунные наружные стопорные кольца РїРѕ DIN – широкий выбор высококачественных крепежных изделий РёР· латуни для промышленных Рё строительных применений. Большой ассортимент размеров. Высокая надежность Рё долговечность. Заказывайте РїСЂСЏРјРѕ сейчас РЅР° сайте Редметсплав.
Где купить диплом специалиста?
Наши специалисты предлагают выгодно купить диплом, который выполняется на оригинальной бумаге и заверен мокрыми печатями, водяными знаками, подписями должностных лиц. Наш диплом пройдет лубую проверку, даже при помощи специальных приборов. Решите свои задачи быстро и просто с нашими дипломами.
Заказать диплом университета [url=http://diplomt-v-samare.ru/kupit-diplom-v-podolske-4/]diplomt-v-samare.ru/kupit-diplom-v-podolske-4/[/url]
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
Наши товары:
Лента РёР· прецизионных сплавов для СѓРїСЂСѓРіРёС… элементов 0.55×230 РјРј 40РљРҐРќРњ ГОСТ 14117-85 Купите ленту РёР· прецизионных сплавов для СѓРїСЂСѓРіРёС… элементов РїРѕ выгодной цене РЅР° Редметсплав.СЂС„. Высокая прочность, устойчивость Рє РєРѕСЂСЂРѕР·РёРё Рё отличная эластичность делают этот материал идеальным для различных отраслей. Предлагаем широкий ассортимент продукции Рё РїРѕРґСЂРѕР±РЅСѓСЋ информацию для правильного выбора.
скачать mostbet [url=https://www.mostbet5003.ru]https://www.mostbet5003.ru[/url] .
I love the poker spread online—new fun! tome of madness
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с высоким уровнем сервиса.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наши товары:
Лента РёР· прецизионных сплавов для СѓРїСЂСѓРіРёС… элементов 0.6×110 РјРј РЎРџ22 ГОСТ 14117-85 Купите ленту РёР· прецизионных сплавов для СѓРїСЂСѓРіРёС… элементов РїРѕ выгодной цене РЅР° Редметсплав.СЂС„. Высокая прочность, устойчивость Рє РєРѕСЂСЂРѕР·РёРё Рё отличная эластичность делают этот материал идеальным для различных отраслей. Предлагаем широкий ассортимент продукции Рё РїРѕРґСЂРѕР±РЅСѓСЋ информацию для правильного выбора.
Pills information. What side effects?
where to get cheap trileptal without dr prescription
Some trends of medicines. Read information now.
All links are in the description! (that mean – here) Valve want us to use Steam Guard Mobile Authenticator at all cost https://sdasteam.com/
I wish online eased bets—low go! plinko
mostbet промокод [url=http://mostbet5001.ru/]http://mostbet5001.ru/[/url] .
Interesting read! Do you have any tips for beginners on this?
I love the pay ease online—smooth run! bet on red
I don’t get online shade—it’s fine! marvel casino
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наши товары:
Плоская мишень цинка
Has anyone tried that new online casino with the live dealers? It’s pretty cool! plinko
mostbest [url=mostbet6033.ru]mostbest[/url] .
Steam Desktop Authenticator (SDA) is an open source program that is designed to use two-factor mobile authentication steam without a mobile application скачать steam desktop authenticator
Приобрести диплом университета по невысокой цене возможно, обращаясь к проверенной специализированной компании. Мы предлагаем документы об окончании любых университетов России. Приобрести диплом ВУЗа– [url=http://diplomp-irkutsk.ru/mozhno-li-kupit-diplom-zanesennij-v-reestr-6/]diplomp-irkutsk.ru/mozhno-li-kupit-diplom-zanesennij-v-reestr-6/[/url]
РедМетСплав предлагает обширный выбор высококачественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица изделия подтверждена требуемыми документами, подтверждающими их качество. Опытная поддержка – наш стандарт – мы на связи, чтобы разрешать ваши вопросы а также находить ответы под особенности вашего бизнеса.
Доверьте вашу потребность в редких металлах специалистам РедМетСплав и убедитесь в множестве наших преимуществ
поставляемая продукция:
РљСЂСѓРі магниевый MC8 – JIS H 5203 Фольга магниевая MC8 – JIS H 5203 представляет СЃРѕР±РѕР№ высококачественный материал, используемый РІ различных отраслях. Благодаря СЃРІРѕРёРј уникальным свойствам, РѕРЅР° обладает отличной РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью Рё легкостью. Рта фольга идеально РїРѕРґС…РѕРґРёС‚ для применения РІ авиации, автомобильной промышленности Рё РґСЂСѓРіРёС… областях. Если вас интересует надежный Рё эффективный материал, обратите внимание РЅР° наш РїСЂРѕРґСѓРєС‚. РќРµ упустите возможность купить Фольга магниевая MC8 – JIS H 5203 для повышения эффективности ваших проектов.
РедМетСплав предлагает обширный выбор высококачественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы обеспечиваем быстрое выполнение вашего заказа.
Каждая единица изделия подтверждена требуемыми документами, подтверждающими их происхождение. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы ответить на ваши вопросы по мере того как находить ответы под требования вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наша продукция:
Рзделия РёР· титана Рў16 – ГОСТ 2171-90 РџРѕРєРѕРІРєР° титановая Рў16 – ГОСТ 2171-90 представляет СЃРѕР±РѕР№ высококачественный металл, обладающий отличной прочностью Рё легкостью. Применяется РІ различных отраслях, включая авиацию Рё медицинское оборудование. Благодаря СЃРІРѕРёРј свойствам, этот материал обеспечивает надежность Рё долговечность изделий.Если РІС‹ ищете надежное решение для СЃРІРѕРёС… проектов, купить РџРѕРєРѕРІРєР° титановая Рў16 – ГОСТ 2171-90 будет отличным выбором. Рто идеальный выбор для тех, кто ценит качество Рё прочность. Заказывайте СѓР¶Рµ сегодня Рё убедитесь РІ высоких характеристиках этой РїРѕРєРѕРІРєРё.
РедМетСплав предлагает обширный выбор высококачественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы обеспечиваем оперативное исполнение вашего заказа.
Каждая единица продукции подтверждена соответствующими документами, подтверждающими их происхождение. Опытная поддержка – наша визитная карточка – мы на связи, чтобы разрешать ваши вопросы по мере того как адаптировать решения под специфику вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
поставляемая продукция:
РџРѕРєРѕРІРєР° ниобиевая РќР±10Р’2РњР¦ – ГОСТ 26468-85 РџРѕРєРѕРІРєР° ниобиевая РќР±10Р’2РњР¦ – ГОСТ 26468-85 представляет СЃРѕР±РѕР№ высококачественный металл, обеспечивающий отличные механические свойства Рё устойчивость Рє высоким температурам. Ртот материал широко используется РІ авиастроении, энергетике Рё РґСЂСѓРіРёС… отраслях, РіРґРµ необходимы надежность Рё производительность.Купить РџРѕРєРѕРІРєР° ниобиевая РќР±10Р’2РњР¦ – ГОСТ 26468-85 значит инвестировать РІ долговечность Рё эффективность ваших изделий. Убедитесь РІ преимуществах использования ниобиевых РїРѕРєРѕРІРѕРє Рё выберите надежное решение для вашего бизнеса. Данная продукция отвечает современным требованиям Рё стандартам, что делает её идеальным выбором для профессионалов.
levaquin side effects 500 mg
Pills information for patients. Long-Term Effects.
where to get cheap aricept online
Some information about meds. Read here.
serm криптовалюты [url=https://www.prodvizhenie-kriptovalyuta2.ru]serm криптовалюты[/url] .
эвакуатор круглосуточно https://avto-vezu.ru/
На сайте https://xn—-7sbjhqn0bhjc0lk.xn--p1ai/ получите юридическую консультацию по различным вопросам. Компания Стратегия работает как с физическими, так и частными лицами. На все услуги установлены привлекательные расценки, чтобы воспользоваться ими смог каждый. Предприятие работает в этой сфере более 11 лет. В команде трудятся 11 специалистов, которые справятся с задачей независимо от сложности. К вашим услугам досудебный юрист, досудебное урегулирование, кредитный, семейный юрист, решение страховых споров.
can i order cheap floxin pills
Online casinos should show—odds up! mines
какие провайдеры интернета есть по адресу краснодар
domashij-internet-krasnodar001.ru
узнать интернет по адресу
Наш сервисный центр Bosch в Москве предлагает профессиональный ремонт техники Bosch от ведущего немецкого бренда. Наши сертифицированные мастера профессионально устранят поломки любой сложности вашего оборудования Bosch. Применяем только оригинальные запчасти и проверенные методы для гарантированного восстановления функциональности вашего оборудования. Ремонт осуществляется как на дому у заказчика, так и в нашей мастерской. Доверьте заботу о вашей технике опытным специалистам. Заказать [url=https://servis-bosch-msk.ru/]замена электроконфорки bosch в москве[/url] и получите качественный сервис.
мостбет официальный сайт [url=www.mostbet6043.ru]www.mostbet6043.ru[/url] .
Мы можем предложить дипломы любых профессий по приятным ценам. Мы можем предложить документы техникумов, которые расположены на территории всей России. Дипломы и аттестаты выпускаются на бумаге высшего качества. Это позволяет делать государственные дипломы, которые невозможно отличить от оригиналов. [url=http://babygirls025.copiny.com/question/details/id/1082940/]babygirls025.copiny.com/question/details/id/1082940[/url]
Перевозка негабаритных грузов и доставка по всей территории России и СНГ на сайте https://negabarit-24.ru/ – это профессиональные услуги от нашей компании. Перевозка негабаритных грузов по России и странам СНГ, ЖД перевозки, перевозки манипулятором и международные перевозки. Гибкие варианты оплаты. Соблюдение сроков. Страхование грузов. Подробнее на сайте.
мастбет [url=https://mostbet6043.ru]мастбет[/url] .
I wish online casinos had more transparency about their algorithms. plinko
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с прекрасным качеством обслуживания.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
Наши товары:
Фольга РёР· палладия Рё его сплавов 0.008×10 РјРј РџРґРЎСЂ60-40 ГОСТ 24353-2014 Ознакомьтесь СЃ уникальной фольгой РёР· палладия Рё его сплавов РѕС‚ компании Редметсплав. Высокая устойчивость, гибкость Рё прочность делают этот материал идеальным для различных отраслей, включая электронику, медицинское оборудование, производство ювелирных изделий Рё аналитическую С…РёРјРёСЋ. Получите консультацию Рё найдите оптимальное решение для ваших потребностей РїСЂСЏРјРѕ сейчас.
мостбет вход [url=http://mostbet6043.ru]http://mostbet6043.ru[/url] .
Спасибо за доставку в плохую погоду – букет был сухим и аккуратным!
купить пионы томск
I love the live roulette—real deal! fishing frenzy
Создай идеальный взгляд! Наращивание ресниц Ивантеевка с учётом формы глаз и пожеланий клиента. Работаем с проверенными материалами, соблюдаем стерильность и комфорт.
Drugs information sheet. What side effects?
hydrochlorothiazide and lisinopril side effects
Some trends of medicament. Get information here.
The online ease rolls—stay wise! vincispin
mostbet kg скачать на андроид [url=www.mostbet6043.ru]www.mostbet6043.ru[/url] .
I’m cautious with online casinos—too many horror stories! tome of madness
cost of zudena without insurance
The payout wait times at online casinos are brutal! slot gallina
РедМетСплав предлагает внушительный каталог отборных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица изделия подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Дружелюбная помощь – наша визитная карточка – мы на связи, чтобы ответить на ваши вопросы и предоставлять решения под особенности вашего бизнеса.
Доверьте ваш запрос специалистам РедМетСплав и убедитесь в гибкости нашего предложения
поставляемая продукция:
Фольга магниевая Р›-2-1 – РўРЈ 28-10-33-75 РўСЂСѓР±Р° магниевая Р›-2-1 – РўРЈ 28-10-33-75 представляет СЃРѕР±РѕР№ высококачественный РїСЂРѕРґСѓРєС‚, специально разработанный для применения РІ различных отраслях. РћРЅР° обладает отличными механическими свойствами Рё высокой стойкостью Рє РєРѕСЂСЂРѕР·РёРё, что гарантирует долговечность Рё надежность РІ эксплуатации. Если РІС‹ ищете материал для СЃРІРѕРёС… проектов, то стоит обратить внимание РЅР° эту трубу. РћРЅР° РїРѕРґС…РѕРґРёС‚ для условий СЃ повышенными требованиями Рє устойчивости материалов. РќРµ упустите возможность купить РўСЂСѓР±Р° магниевая Р›-2-1 – РўРЈ 28-10-33-75 Рё обеспечить СЃРІРѕР№ проект надежным Рё качественным продуктом.
I don’t trust online casinos with my data—sketchy! plinko
I love the online twists—keep fresh! dragon tiger
провайдеры интернета по адресу
domashij-internet-krasnodar002.ru
интернет по адресу
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
С широким ассортиментом продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Керамическая мишень Licoo2 (3N5, 4N)
Novo no misterjackbet – https://misterjackbet-br.com? Cadastre-se e Receba 100$ de Bônus ao Fazer Login!
Go to the site https://betwave.ro/en where you will find a list of all the best online casinos, as well as useful and complete information about each of them – how to play, what bonuses there are, how to withdraw money, which casinos have a minimum deposit, registration steps and advantages of each of them, as well as what are the biggest bonuses in online casinos. More details on the site.
Аренда авто Краснодар
Online casinos should push safe play more. plinko app
Welvura Casino — это современное онлайн-казино, предлагающее широкий выбор азартных игр. С момента своего открытия оно зарекомендовало себя как надежная платформа с привлекательным интерфейсом и интуитивно понятным дизайном. В Welvura Casino доступны слоты, настольные игры и живое казино, что делает его идеальным выбором для разных типов игроков https://welvura.love
Online casinos should teach—too steep! mines
Pills information. Cautions.
how can i get generic keflex no prescription
Actual about medicines. Get now.
Устали от пыли и беспорядка? Наша компания в Санкт-Петербурге предлагает квалифицированные услуги по уборке для вашего дома и офиса. Мы используем только экологически безопасные средства и гарантируем непревзойденный порядок! Выбирайте https://klining-uslugi24.ru – Клининговая уборка квартиры цена Почему стоит сотрудничать именно с нами? Быстрая и качественная работа, индивидуальный подход к каждому клиенту и привлекательные расценки. Доверьте уборку профессионалам и наслаждайтесь чистотой без лишних усилий!
Хочу поделиться своим опытом по заказу аттестата ПТУ. Думал, что это невозможно, и начал искать информацию в интернете по теме: купить вкладыш к диплому, купить диплом бакалавра в нижнем тагиле, купить диплом в бердске, купить диплом диспетчера, купить диплом закрывшегося вуза. Постепенно углубляясь в тему, нашел отличный ресурс здесь: [url=http://diplomybox.com/kupit-diplom-tekhnikuma-kolledzha-v-volgograde/]diplomybox.com/kupit-diplom-tekhnikuma-kolledzha-v-volgograde[/url]
Система менеджмента управления качеством содержит критерии менеджмента и стандарты, по которым проводится последующая сертификация предприятия. Чтобы получить сертификат СМК, нужно обратиться в аккредитованный сертификационный центр. Сертификация систем менеджмента качества является документальным подтверждением того, что система менеджмента сертифицированной компании соответствует требованиям того или иного стандарта. Подробнее: https://ok.ru/group/70000034956977
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
Обладая обширной линейкой редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наши товары:
Плоская мишень NiV
Are online casinos regulated enough? I’m not so sure sometimes. teen patti
where to get sumycin online
Jon Jones profile https://john-jones-ufc.com/ a fighter with a unique style, record-breaking achievements and UFC legend status. All titles, weight classes and the path to the top of MMA – on one page.
A suspected Russia-based cybercriminal decided to clone the website of a legitimate open-source desktop app (see here) called Steam Desktop Authenticator (SDA https://authenticatorsteamdesktop.com/
Онлайн-казино JoyCasino https://thejoycasino-ru.com яркий дизайн, популярные слоты, live-игры и щедрые бонусы. Простой вход, быстрые выплаты и надёжная поддержка. Играйте с комфортом и без лишних рисков.
Главным достоинством Апостолиди является предоставление возможности для актуальной информации посетителям. Мы простым языком объясняем сложные финансовые вопросы. Благодаря регулярным обновлениям вы всегда будете в курсе важных событий. https://apostolidi.ru – сайт, который сейчас пользуются невероятной популярностью. Здесь есть поиск, можете им в любое время воспользоваться. Апостолиди позволяет находить полезные рекомендации, получать знания от экспертов и исследовать уникальные материалы. Добро пожаловать на наш портал!
Ganhe 100$ para Apostar no luck ao Se
Registrar Hoje!
Где заказать диплом по актуальной специальности?
Наша компания предлагает быстро и выгодно приобрести диплом, который выполнен на оригинальной бумаге и заверен мокрыми печатями, водяными знаками, подписями. Данный диплом пройдет любые проверки, даже при использовании профессиональных приборов. Достигайте своих целей быстро и просто с нашими дипломами.
Купить диплом о высшем образовании [url=http://kupit-diplom24.com/kupit-diplom-v-novosibirske-22/]kupit-diplom24.com/kupit-diplom-v-novosibirske-22/[/url]
Legendary boxer Mike Tyson mike tyson az com is the undisputed heavyweight champion and one of the most recognizable athletes in history. His strength, speed, and charisma have made him a boxing icon.
Professional fighter Rafael Fiziev http://rafael-fiziev.com is a UFC star known for his explosive technique and spectacular fights. A lightweight fighter with a powerful punch and a strong Muay Thai base.
мост бет [url=https://mostbet5003.ru/]мост бет[/url] .
поддержка мостбет [url=http://mostbet5004.ru/]http://mostbet5004.ru/[/url] .
Use our online currency calculator to find https://how-much-is.com/ out the exchange rate for dollars, euros, British Pounds, Russian rubles and other currencies.
Looking for it AI Generators? Xnudes.app is the best NSFW artificial intelligence generator with pictures. The Desire chat engine never forgets information about you, it’s incredibly immersive and realistic, and it’s the most immersive chat experience you’ve ever seen in an artificial intelligence service. Using deep learning models, these generators can create visual illustrations in various variations.
Registre-se e Receba 100$ de Bônus no roleta
para Apostar!
https://boi.instgame.pro/forum/index.php?topic=69096.0
Well-written and informative. I appreciate the depth of your analysis.
The online rush is big—stay chill! plinko
узнать больше https://falcoware.com/rus/match3_games.php
провайдеры в краснодаре по адресу проверить
domashij-internet-krasnodar003.ru
интернет по адресу краснодар
Laenutoodete lehel on valja toodud koik laenud: kiirlaen, vaikelaen, tarbimislaen, kaenduslaen, kinnisvaralaen ning autolaen laenud
Medicament information sheet. Cautions.
where to buy generic keflex pill
Actual what you want to know about meds. Read here.
http://center-2.ru/forum/?mingleforumaction=viewtopic&t=19332#postid-37364
Online casinos aid practice—nice try! mines game
Very informative post, thank you for sharing!
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
Наши товары:
Мишень РёР· драгоценного металла или его сплава кольцевая золото 9С…4.5 РјРј ЗлСрМ58.5-8 РўРЈ Выберите уникальные мишени РёР· драгоценных металлов для напыления РѕС‚ Редметсплав.СЂС„. Обеспечивают высокую степень защиты Рё долговечность. Рдеальный выбор для промышленности, строительства Рё производства.
I hate how online casinos push you to deposit more with pop-ups. jackpotraider
elonbet
Cadastre-se no sportbet
e Ganhe 100$ de Bônus de Boas-Vindas!
Аренда авто Краснодар
I won big online—still high! plinko
how to buy generic finast tablets
Джеймс браун песни
elonbet
Посетите сайт https://letrivelle.ru/ – это магазин мебели, в котором представлена дизайнерская мебель, свет и декор. Ознакомьтесь с каталогом или обратитесь к нашим квалифицированным менеджерам, которые помогут с выбором. Все в наличии на наших собственных складах в Москве и Санкт-Петербурге. Подробнее на сайте.
elonbet
Kodust lahkumata ja interneti vahendusel kogu Eesti piires. Autolaen on mugav lahendus, kui sooviks on osta kasutatud autot voi mootorratast refinantseerimislaen
мостбет кыргызстан скачать [url=https://mostbet5003.ru]мостбет кыргызстан скачать[/url] .
I wish online casinos paid out faster—waiting sucks! razor returns
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
поставляемая продукция:
Кольцо РёР· драгоценных металлов палладиевое 25С…10С…4.5 РјРј РџРґРЎСЂ60-40 РўРЈ РЁРёСЂРѕРєРёР№ выбор высококачественных кольц РёР· драгоценных металлов. Рлегантные украшения, созданные талантливыми мастерами. Уникальность Рё изысканность РІ каждой детали. Возможность заказа персонального кольца РїРѕ вашим пожеланиям. Подчеркните СЃРІРѕСЋ индивидуальность СЃ нашими кольцами.
I hit a blackjack wave—good run! bet on red
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наша продукция:
Никель высокой чистоты (4N)
Medication information sheet. What side effects?
buy generic levitra for sale
Actual information about drugs. Read information now.
elonbet
На сайте https://arenda24.by/ каждый желающий получает возможность взять в прокат спецтехнику, садовый инвентарь для выполнения всех необходимых хозяйственных работ. Если нашли подходящее объявление, то свяжитесь и договоритесь с продавцом для того, чтобы он на выгодных условиях передал технику. Напротив каждого варианта имеются его характеристики, особенности, детальное описание, чтобы потенциальный клиент сразу же сориентировался. Постоянно появляются новые объявления, что позволит найти все, что нужно.
elonbet
King of the ring https://roy-jones.com/ Roy Jones is a fighter with a unique style and lightning-fast reactions. His career includes dozens of titles, spectacular knockouts and a cult status in the boxing world.
Football genius https://luka-modric.com Luka Modric – from humble beginnings in Zagreb to a world-class star. His path inspires, his play amazes. The story of a great master in detail.
Заказала к точному времени – минута в минуту!
доставка цветов томск на дом
Автопортал для водителей https://addinfo.com.ua и автолюбителей: свежие авто новости, сравнения моделей, рейтинги, советы по выбору и обслуживанию автомобилей. Полезная информация каждый день.
I don’t trust online odds—weird! plinko
elonbet
Свежие новости https://actualnews.kyiv.ua Украины и мира онлайн: события, аналитика, интервью и факты. Будьте в курсе главного — обновления 24/7, объективная подача и лента новостей в реальном времени.
Компания “Развитие” это надежный комплексный оператор оборудования для сельского хозяйства с репутацией. На сайте razvitieagro.ru представлен разнообразие решений для фермерских хозяйств, птицефабрик, овощных складов и теплиц. Мы поставляем системы для свиноферм, животноводческих комплексов, доильных блоков, систем вентиляции, автоматического управления и подачи корма. Вся техника сертифицирована и отвечает стандартам агропроизводства. Работаем без посредников — с выгодными условиями и поддержкой.
Если вы ищете конкретные решения — вы обратились правильно. У нас можно найти всё, вплоть до таких редких позиций, как [url=https://razvitieagro.ru/]несушки напольное содержание[/url] которое создаёт циркуляцию воздуха в теплицах. Также в наличии системы обогрева, системы подсветки, распылители, инкубаторы, гигиенические щётки, поилки, раздатчики корма, шнеки, мини-заводы по производству комбикорма и другое оборудование. Весь ассортимент — готов к отправке, логистика по всей России.
Наша компания находится по адресу: г. Ижевск, ул. Маркина 197. Если ваша задача — [url=https://razvitieagro.ru/]инсинератор купить[/url] или приобрести технику для хозяйства, мы подскажем оптимальное решение. Подберем, рассчитаем и отправим — всё в срок, надежно и с консультацией специалиста.
подключение интернета по адресу
domashij-internet-samara001.ru
узнать интернет по адресу
мостбет [url=http://mostbet5004.ru/]http://mostbet5004.ru/[/url] .
РедМетСплав предлагает обширный выбор качественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица продукции подтверждена требуемыми документами, подтверждающими их происхождение. Опытная поддержка – наш стандарт – мы на связи, чтобы разрешать ваши вопросы а также предоставлять решения под требования вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
Наши товары:
Кобальт РРљ20-ВРКобальт РРљ20-Р’Р – это надежный Рё высококачественный инструмент, специально разработанный для профессионального использования. РћРЅ прекрасно справляется СЃ широким спектром задач, включая ремонт Рё обслуживание техники. Благодаря мощному двигателю Рё СѓРґРѕР±РЅРѕР№ конструкции, работа СЃ РЅРёРј становится быстрой Рё эффективной. Если РІС‹ ищете надежный инструмент для СЃРІРѕРёС… РЅСѓР¶Рґ, вам стоит купить Кобальт РРљ20-Р’Р. Его продуманный дизайн гарантирует долговечность Рё стабильные результаты. РќРµ упустите возможность улучшить СЃРІРѕСЋ работу СЃ этим выдающимся изделием!
Мы изготавливаем дипломы любой профессии по доступным тарифам. Мы готовы предложить документы ВУЗов, которые находятся в любом регионе России. Дипломы и аттестаты выпускаются на “правильной” бумаге самого высокого качества. Это дает возможность делать государственные дипломы, не отличимые от оригиналов. [url=http://rst.adk.audio/company/personal/user/1577/forum/message/5820/7412/#message7412/]rst.adk.audio/company/personal/user/1577/forum/message/5820/7412/#message7412[/url]
SteamGuard mobile authenticator authorization codes directly in your browser! Now you do not need to reach for your phone whenever Steam requests authorization steam authenticator
Заказать диплом о высшем образовании!
Мы можем предложить документы учебных заведений, расположенных в любом регионе России.
[url=http://diplomskiy.com/kupit-diplom-s-zaneseniem-v-reestr-rossii-vigodno-2/]diplomskiy.com/kupit-diplom-s-zaneseniem-v-reestr-rossii-vigodno-2/[/url]
Are online reviews legit?—hard say! aviator
скачать mostbet [url=http://mostbet7001.ru]http://mostbet7001.ru[/url] .
I love the online calm—no noise! fortune mouse
cost cheap zanaflex without prescription
The live aid online saves—big aid! plinko
Medicines information sheet. Brand names.
exploring the effects of combining diclofenac sodium with ibuprofen a comprehensive analysis
Everything about drug. Read information here.
https://russiancouncil.ru/petr-aven/?str=Е
Женский журнал https://asprofrutsc.org о стиле, красоте, психологии, отношениях и саморазвитии. Актуальные статьи, советы экспертов, тренды и вдохновение — всё для современной женщины.
Онлайн-журнал для женщин https://chernogolovka.net всё о жизни, любви, красоте, детях, финансах и личностном развитии. Простым языком о важном — полезно, интересно и по делу.
Автомобильный портал https://avto-limo.zt.ua для тех, кто за рулём: автообзоры, полезные советы, новости индустрии и подбор авто. Удобный поиск, свежая информация и всё, что нужно автолюбителю.
Аренда авто Краснодар
Авто журнал онлайн https://clothes-outletstore.com всё о мире автомобилей: новости, тест-драйвы, обзоры, советы, новинки автопрома и технологии. Читайте с любого устройства — всегда в курсе автоиндустрии.
Подробнее https://falcoware.com/rus/match3_games.php
mostbet kg отзывы [url=https://mostbet6038.ru/]https://mostbet6038.ru/[/url] .
купить диплом в соликамск
I hit a bonus online and won $500—big yes! plinko
elonbet
Где приобрести диплом по необходимой специальности?
Наша компания предлагает максимально быстро приобрести диплом, который выполнен на бланке ГОЗНАКа и заверен печатями, штампами, подписями официальных лиц. Данный диплом пройдет лубую проверку, даже при использовании специального оборудования. Решите свои задачи быстро и просто с нашим сервисом.
Заказать диплом университета [url=http://diplomus-spb.ru/starie-diplomi-kupit-5/]diplomus-spb.ru/starie-diplomi-kupit-5/[/url]
Online casino ads hit hard—chill out! plinko
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
Наша продукция:
Латуное внутреннее стопорное пружинное кольцо 35х1.2 мм ЛЦ38Мц2С2 ГОСТ 13943-86 Купить латунные внутренние стопорные кольца по ГОСТ от производителя. Надежное крепление и удержание элементов механизмов. Широкий выбор размеров и конфигураций. Высокое качество и надежность. Применение в машиностроении, автомобильной и других отраслях. Обращайтесь к нашим специалистам для получения более подробной информации.
здесь http://lapplebi.com/
провайдер по адресу самара
domashij-internet-samara002.ru
провайдеры интернета по адресу
I won big online last night—still in shock! bet on red
The music online pulls—vibe on! plinko
get mobic without dr prescription
Мы можем предложить дипломы любой профессии по приятным ценам. Дипломы производятся на настоящих бланках государственного образца Приобрести диплом любого института [url=http://diplomg-cheboksary.ru/]diplomg-cheboksary.ru[/url]
Где заказать диплом по нужной специальности?
Заказать диплом университета по невысокой цене вы можете, обращаясь к надежной специализированной фирме.: [url=http://kazdiplomas.com/]kazdiplomas.com[/url]
Online casinos need tools—help us! Vai de Bet
Работая с «НЕФТЕГАЗМАШ» у вас есть возможность рассчитывать на: полную и своевременную комплектацию ваших заказов и авансовую систему оплаты, поставку оборудования в сжатые сроки и различным видом транспорта. Наши сотрудники имеют в области теплогазоснабжения профильное высшее техническое образование. Ищете клапан предохранительный запорный электромагнитный кпэг? Neftegazmash.ru – здесь имеется каталог продукции. Также здесь можете ознакомиться с условиями доставки в любое время. С удовольствием сертификаты и действующие разрешения предоставим. Обращайтесь, вам все понравится, мы уверены!
Drugs information sheet. What side effects?
where to get cheap xenical
Best information about medicament. Read information now.
Aproveite a oferta exclusiva do heads bet para novos
usuários e receba 100$ de bônus ao se registrar! Este bônus de
boas-vindas permite que você experimente uma vasta gama de jogos de cassino online
sem precisar gastar imediatamente. Com o bônus de 100$,
você poderá explorar jogos como roleta, blackjack, caça-níqueis e muito mais, aumentando suas chances de vitória desde o primeiro minuto.
Não perca essa chance única de começar com um valor
significativo – cadastre-se agora!
casinos sin licencia [url=casinossinlicenciaespanola.com]casinos sin licencia[/url] .
РедМетСплав предлагает обширный выбор качественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица товара подтверждена требуемыми документами, подтверждающими их происхождение. Опытная поддержка – наша визитная карточка – мы на связи, чтобы улаживать ваши вопросы по мере того как предоставлять решения под особенности вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
Наши товары:
Лист висмутовый 291-325 – ASTM B774 Лист висмутовый 291-325 – ASTM B774 представляет СЃРѕР±РѕР№ высококачественный материал, предназначенный для применения РІ различных отраслях. Ртот лист обладает отличной РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью Рё высокой температурной стабильностью, что делает его идеальным выбором для производства электронных компонентов Рё химической промышленности. Р’С‹ можете купить Лист висмутовый 291-325 – ASTM B774 для реализации ваших проектов, обеспечивая надежность Рё долговечность. РќРµ упустите возможность воспользоваться преимуществами этого уникального материала, который поможет вам достичь оптимальных результатов РІ работе.
elonbet
I wish online tied more—life in! plinko
mostber [url=https://mostbet6033.ru]https://mostbet6033.ru[/url] .
elonbet
The music online is a mood—keeps me in! mines
Visit https://wpstore.pro/ where you will find premium WordPress themes and plugins. We have collected the best solutions for WordPress in one place. Official products from developers. We have the most affordable prices, original products, unlimited use, lifetime updates and much more. More details on the site.
The mono
O queens oferece uma excelente oportunidade para quem deseja começar
sua experiência no cassino online com um bônus de 100$
para novos jogadores! Ao se registrar no site, você garante esse bônus exclusivo
que pode ser utilizado em diversos jogos de cassino, como slots, roleta e poker.
Esse é o momento perfeito para explorar o mundo das apostas com um saldo
extra, aproveitando ao máximo suas apostas sem precisar investir um grande valor logo de
início. Não perca essa oportunidade e cadastre-se já!
Посетите сайт Мир Часов https://mir-watch.ru/ – это скупка часов в Москве. Вы сможете сдать часы в ломбард по выгодной стоимости. Занимаемся выкупом (скупкой) швейцарских часов и других известных брендов. Выкупаем не только элитные часы, но и часовые аксессуары: виндеры, коробки, шкатулки, ремни, ювелирные украшения и аксессуары с бриллиантовыми камнями.
I lost time online—quick gone! plinko
Аренда авто Краснодар
elonbet
where to get cheap celexa no prescription
[url=https://kuban.video/video/40-svjatki-v-ispolnenii-ansambljaatamanochka.html]Святки[/url]
Святки
mornless
encyclopedize
gonglike
flat-footed
stylitic
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
С широким ассортиментом продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Медно-никелевая поверхность для распыления
elonbet
Medicine information leaflet. Brand names.
cost of generic ceftin for sale
Actual news about pills. Get here.
[url=https://telegra.ph/Windows-10-Windows-11-activation-is-forever-free-04-04]Windows Process Activation Service Name[/url]
[url=https://x.com/RauheShemi20597/status/1908418523719696728]Windows 10 Activation Microsoft Account[/url]
[url=https://telegra.ph/Windows-10-Windows-11-activation-is-forever-free-04-04]Windows 11 Activation Key Where To Find[/url]
[url=https://telegra.ph/Windows-10-Windows-11-activation-is-forever-free-04-04]Windows Activation Server Kms[/url]
[url=https://telegra.ph/Windows-10-Windows-11-activation-is-forever-free-04-04]Windows Activation Limit[/url]
Windows Activation
провайдер интернета по адресу самара
domashij-internet-samara003.ru
провайдеры интернета по адресу самара
The slot sounds online are so fun—pure joy! plinko
mostbet игры [url=http://mostbet6033.ru/]http://mostbet6033.ru/[/url] .
elonbet
Ao se cadastrar no codbet (https://www.codbet-br.com/),
você ganha um bônus de 100$ para começar sua jornada
no cassino com o pé direito! Não importa se você é um novato
ou um apostador experiente, o bônus de boas-vindas é a oportunidade perfeita para
explorar todas as opções que o site tem a oferecer. Jogue seus jogos favoritos, descubra novas opções de apostas e
aproveite para testar estratégias sem risco, já que o
bônus ajuda a aumentar suas chances de ganhar.
Cadastre-se hoje e comece com 100$!
dragonmoney зарабатывать легко [url=https://www.dragon-money33.com]https://www.dragon-money33.com[/url] .
Online casinos feel dicey—double risk! jackpot raider
haval дарго [url=https://haval-msk1.ru/models/new-haval-dargo/]https://haval-msk1.ru/models/new-haval-dargo/[/url] .
мостбет мобильная версия скачать [url=https://www.mostbet6038.ru]https://www.mostbet6038.ru[/url] .
casinos sin licencia [url=http://casinossinlicenciaespanola.com/]casinos sin licencia[/url] .
The live games online shine—big fan! plinko
lhfujy vfyb [url=http://www.dragon-money30.com]http://www.dragon-money30.com[/url] .
На сайте https://voronlaws.ru/ вы найдете Casino Dragon Money и его новое рабочее зеркало для того, чтобы открыть для себя удивительный мир огромного количества развлечений, щедрых бонусов, а также бесконечных выигрышей. На сайте вас ожидает увлекательный и эксклюзивный контент, который обязательно подарит много приятных и положительных эмоций. Клуб щедр на акции и бонусы, а потому здесь вы найдете все, что понравится и поможет заработать деньги. Рабочее зеркало ежедневно обновляется для вашей безопасности.
Medicines information sheet. Brand names.
can i buy cheap elavil without a prescription
Some what you want to know about medicament. Get now.
can i purchase cheap etodolac price
Choosing an online casino that doesn’t require verification can streamline your gaming experience http://wiki.geologyscience.ru/index.php?title=_No_Verification_Casinos:_The_Ultimate_Choice_for_Privacy_in_the_UK
mostbet kg скачать на андроид [url=https://www.mostbet5004.ru]https://www.mostbet5004.ru[/url] .
I wish online paid fast—slow drag! dragon tiger
Заказать диплом любого университета!
Мы можем предложить документы ВУЗов, расположенных в любом регионе Российской Федерации.
[url=http://diplomservis.ru/kupit-diplom-ob-obrazovanii-s-reestrom-bistro-i-legko/]diplomservis.ru/kupit-diplom-ob-obrazovanii-s-reestrom-bistro-i-legko/[/url]
mostbet промокод [url=https://www.mostbet6038.ru]mostbet промокод[/url] .
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Тигель тантала для источников испарения
Современный авто журнал https://ecotech-energy.com в онлайн-формате для тех, кто хочет быть в курсе автомобильных трендов. Новости, тесты, обзоры и аналитика — всегда под рукой.
проверить интернет по адресу
domashij-internet-ufa001.ru
интернет провайдеры по адресу
mostbet chrono [url=https://mostbet6033.ru/]https://mostbet6033.ru/[/url] .
Авто журнал онлайн https://comparecarinsurancerfgj.org свежие новости, обзоры моделей, тест-драйвы, советы и рейтинг автомобилей. Всё о мире авто в одном месте, доступно с любого устройства.
Онлайн-портал для женщин https://fancywoman.kyiv.ua которые ценят себя и стремятся к лучшему. Всё о внутренней гармонии, внешнем блеске и жизненном балансе — будь в центре женского мира.
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
Наши товары:
Лист судостроительный 15x1000x2000 D EN10025:2 Приобретите высококачественные листы судостроительные от компании Редметсплав.рф для надежного и долговечного использования в вашем судостроительном проекте. Различные размеры и толщины, прочность и устойчивость к коррозии делают эти листы идеальным выбором для любого судостроительного проекта.
Женский портал https://elegantwoman.kyiv.ua о красоте, моде, здоровье, отношениях и вдохновении. Полезные статьи, советы экспертов, лайфхаки и свежие тренды — всё для современной женщины.
Аренда авто Краснодар
Online casinos should cut—fair play! plinko
Перевозка негабаритных грузов и доставка по всей территории России и СНГ на сайте https://negabarit-24.ru/ – это профессиональные услуги от нашей компании. Перевозка негабаритных грузов по России и странам СНГ, ЖД перевозки, перевозки манипулятором и международные перевозки. Гибкие варианты оплаты. Соблюдение сроков. Страхование грузов. Подробнее на сайте.
dragon money официальный [url=http://dragon-money33.com/]http://dragon-money33.com/[/url] .
O tvbet oferece uma excelente
oportunidade para quem deseja começar sua experiência no cassino online com um
bônus de 100$ para novos jogadores! Ao se registrar no site,
você garante esse bônus exclusivo que pode ser utilizado em diversos jogos de cassino,
como slots, roleta e poker. Esse é o momento perfeito para explorar o
mundo das apostas com um saldo extra, aproveitando ao máximo suas
apostas sem precisar investir um grande valor logo de início.
Não perca essa oportunidade e cadastre-se já!
?Grass is a decentralized network that enables users to monetize their unused internet bandwidth by sharing it with verified institutions. https app getgrass io register – This shared bandwidth supports various applications, including enhancing AI services and conducting public web data collection.
I love the privacy online—chill play! bac bo
I love the online chats—great crew! dragon tiger
мостбет зеркало [url=www.mostbet7001.ru]www.mostbet7001.ru[/url] .
Посетите страницу https://www.drive2.ru/o/b/691804470933197117/ и вы сможете ознакомиться с тем как происходит оклейка плёнкой Porsche 911 от профессионалов. Посмотрите как стал выглядеть Porsche 911 Carrera GTS после оклейки. Оклейка Porsche 911 выполнялась пленкой Vega 230 ST – узнайте почему и все ее преимущества в статье. Porsche 911 удивительный автомобиль – вам будет интересно читать!
Online casinos need hold—too wild! betonred casino
Сайт компании Аллегро – https://spb.tk-allegro.ru/ предлагает вам аренду в Санкт-Петербурге автобуса. У нас от 8 до 55 мест свой автопарк автобусов. Водители с большим опытом. У вас есть возможность заказать для любых мероприятий автобус: на экскурсию, выпускной, свадьбу и для школьников. Заходите на портал, узнайте больше о стоимости аренды, о наших услугах, или же воспользуйтесь для расчета стоимости калькулятором на портале.
Drugs information for patients. Cautions.
can i get cheap azathioprine price
Some trends of pills. Read here.
mostbet kg скачать на андроид [url=https://mostbet7002.ru/]https://mostbet7002.ru/[/url] .
The slot sounds online rock—pure fun! bet on red
РедМетСплав предлагает обширный выбор высококачественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы обеспечиваем оперативное исполнение вашего заказа.
Каждая единица продукции подтверждена всеми необходимыми документами, подтверждающими их качество. Дружелюбная помощь – наша визитная карточка – мы на связи, чтобы разрешать ваши вопросы а также предоставлять решения под особенности вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
Фольга магниевая M14141 – UNS РўСЂСѓР±Р° магниевая M14141 – UNS представляет СЃРѕР±РѕР№ легкий Рё прочный материал, идеально подходящий для различных применений. Благодаря высокой РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкости Рё отличным механическим свойствам, эта труба используется РІ авиастроении, автомобилестроении Рё РґСЂСѓРіРёС… отраслях. Если РІС‹ ищете надежный Рё эффективный РїСЂРѕРґСѓРєС‚, вам стоит купить РўСЂСѓР±Р° магниевая M14141 – UNS. Ртот РїСЂРѕРґСѓРєС‚ соответствует современным стандартам качества Рё обеспечит долгосрочную эксплуатацию. Заказывайте СѓР¶Рµ сегодня, чтобы получить РІСЃРµ преимущества магниевых труб!
cheap reglan without a prescription
Such a useful guide. Bookmarking this for later!
mostbet kg отзывы [url=http://mostbet7002.ru]http://mostbet7002.ru[/url] .
casino online sin licencia [url=https://casinossinlicenciaespanola.com]casino online sin licencia[/url] .
dragon money casino официальный сайт [url=http://dragon-money30.com]http://dragon-money30.com[/url] .
мостбет вход [url=https://www.mostbet7002.ru]https://www.mostbet7002.ru[/url] .
Турецкие сериалы набрали высокую популярность во всем мире. Турция славится своими качественными сериалами с неповторимым сюжетом, невероятными эмоциями и качественной актерской игрой.
Турецкие фильмы предлагают разнообразие жанров и тематик, чтобы удовлетворить интересы каждого зрителя. От романтических комедий до исторических драм, от триллеров до ужасов – каждый найдет что-то по своему вкусу. Богатство сюжетов и уровень съемок делают турецкие сериалы невероятными шедеврами мирового кинематографа.
[url=https://turkish-film1.ru/top100-filmov/]Лучшие турецкие фильмы[/url] – это уникальная возможность отправиться в турецкую культуру, узнать больше о традициях и обычаях турецкого народа.
мостбет скачать на андроид [url=www.mostbet7002.ru]мостбет скачать на андроид[/url] .
The slot sounds online are so fun—pure joy! Marvel Casino
Авва песни
Заказать диплом о высшем образовании!
Мы готовы предложить дипломы любой профессии по доступным ценам. Вы заказываете документ в надежной и проверенной компании. : [url=http://arzookanak0022.copiny.com/question/details/id/1073010/]arzookanak0022.copiny.com/question/details/id/1073010[/url]
Online casinos need stricter rules—too loose! fortune tiger
провайдеры интернета по адресу
domashij-internet-ufa002.ru
узнать провайдера по адресу уфа
The slot rush online pulls—watch it! plinko
Medicines information sheet. Drug Class.
can i buy cheap zoloft without dr prescription
Actual information about meds. Read here.
Посетите https://igormylnikovchannel.ru/poezdki-v-taksi-s-det-mi/ и вы найдете полезную информацию для начинающих таксистов и опытных водителей на тему поездок в такси с детьми, начиная от безопасности поездок и ответственности и правилах перевозки детей, до разбора вопросов почему таксисты отказываются от поездок с детьми и многое другое. Подробнее на странице.
Семейный портал https://cgz.sumy.ua для родителей и детей: развитие, образование, здоровье, детские товары, досуг и психология. Актуальные материалы, экспертиза и поддержка на всех этапах взросления.
[b]Диплом университета РФ![/b]
Без присутствия диплома сложно было продвинуться вверх по карьере. Поэтому решение о заказе диплома можно считать мудрым и целесообразным. Купить диплом университета [url=http://talkitter.com/read-blog/246134/]talkitter.com/read-blog/246134[/url]
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
Наша продукция:
Титановая труба 6х2х3000 мм ВТ20 ГОСТ 22897-86 Откройте мир прочных и надежных титановых труб с компанией Редметсплав. Наши высококачественные титановые трубы сочетают в себе устойчивость к коррозии, износостойкость и легкий вес, делая их незаменимым материалом для применения в различных сферах промышленности. Широкий выбор различных диаметров и толщин, а также индивидуальные решения для вашего производства. Гарантированное качество и надежность поставок.
Online casinos should limit—protect us! aviator
Автомобильный сайт https://billiard-sport.com.ua с обзорами, тест-драйвами, автоновостями и каталогом машин. Всё о выборе, покупке, обслуживании и эксплуатации авто — удобно и доступно.
Ищете Нестероидный противовоспалительный обезболивающий препарат с фенилбутазоном и лидокаином? Посетите сайт https://ambenium.ru/ и узнайте о препарате Амбениум – это единственный нестероидный противовоспалительный препарат, зарегистрированный в России с усиленным обезболивающим эффектом – раствор для внутримышечного введения фенилбутазон и лидокаин. Подробности на сайте.
Родительский портал https://babyrost.com.ua от беременности до подросткового возраста. Статьи, лайфхаки, рекомендации экспертов, досуг с детьми и ответы на важные вопросы для мам и пап.
Автомобильный журнал https://eurasiamobilechallenge.com новости автоиндустрии, тест-драйвы, обзоры моделей, советы водителям и экспертов. Всё о мире автомобилей в удобном онлайн-формате.
Аренда авто Краснодар
dragon money зеркало casino [url=https://dragon-money33.com]dragon money зеркало casino[/url] .
Мы предлагаем дипломы любой профессии по приятным тарифам. Стараемся поддерживать для заказчиков адекватную политику цен. Для нас очень важно, чтобы дипломы были доступны для большинства наших граждан.
Заказ диплома, подтверждающего обучение в ВУЗе, – это рациональное решение. Купить диплом любого университета: [url=http://kupitediplom0029.ru/kupit-diplom-med-obrazovanie-2/]kupitediplom0029.ru/kupit-diplom-med-obrazovanie-2/[/url]
I’m cautious with online casinos—too many horror stories! fishin frenzy
Займы без отказа — реальность. На [url=https://mikro-zaim-online.ru/zaim-bez-otkaza-na-kartu/]mikro-zaim-online.ru[/url] представлены МФО, которые выдают деньги по паспорту и на именную карту, начиная с 18 лет. Без процентов или по ставке 0.8% в день. Более 60 надёжных компаний, готовых помочь. Даже с плохой КИ — шанс высокий. Убедитесь сами.
Займы оформляются официально, по законодательству РФ. Согласно №151-ФЗ, каждая МФО обязана соблюдать условия прозрачности и нести ответственность перед заёмщиком. Именно поэтому деньги переводятся моментально, без проверок, а договор — законный. Надёжность, простота и безопасность в одном решении.
РедМетСплав предлагает внушительный каталог качественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы обеспечиваем быстрое выполнение вашего заказа.
Каждая единица товара подтверждена всеми необходимыми документами, подтверждающими их качество. Опытная поддержка – наша визитная карточка – мы на связи, чтобы разрешать ваши вопросы а также предоставлять решения под требования вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
Наши товары:
Поковка титановая 40 Лист титановый 40 — это высококачественный материал, обладающий отличной прочностью и легкостью. Он идеально подходит для различных отраслей: авиационной, автомобильной и медицинской. Благодаря своим коррозионным свойствам, титановые листы не подвержены ржавчине и долговечны в эксплуатации. Толщина 40 мм обеспечивает дополнительную прочность, что делает его незаменимым в конструкциях, требующих устойчивости к высоким нагрузкам. Если вы хотите купить Лист титановый 40, у нас вы найдете оптимальный вариант по лучшей цене. Доверяйте, ведь качество — это наш приоритет!
Online casinos should open odds—show it! dragon tiger
trazodone dosage half life
The music online pulls—vibe on! betonred
https://war-proekt.media/spisok-oligarkhov/provider/petr-aven/
ремонт hotpoint ariston [url=https://ariston-servis-centr.ru]сервис аристон[/url]
Great perspective. I hadn’t thought about it that way!
мосбет [url=http://mostbet5004.ru]http://mostbet5004.ru[/url] .
Drugs information for patients. Short-Term Effects.
cost priligy pills
Best about medicament. Read here.
Автомобильный сайт https://fundacionlogros.org с ежедневными новостями, обзорами новинок, аналитикой, тест-драйвами и репортажами из мира авто. Следите за трендами и будьте в курсе всего важного.
dragon money официальный сайт вход [url=https://dragon-money30.com/]https://dragon-money30.com/[/url] .
Современный женский сайт https://femalebeauty.kyiv.ua мода, психология, семья, карьера, рецепты и лайфхаки. Ежедневно — новые материалы, рекомендации и интересные темы для каждой женщины.
Женский сайт о красоте https://female.kyiv.ua моде, здоровье, отношениях и саморазвитии. Полезные статьи, советы экспертов, вдохновение и поддержка — всё для гармоничной и уверенной жизни.
Читайте автомобильный сайт https://gormost.info онлайн — тесты, обзоры, советы, автоистории и материалы о современных технологиях. Всё для тех, кто любит машины и скорость.
Visita el sitio https://lucky-lady-charm.online/ donde podras leer una resena completa de la maquina tragamonedas Lucky Lady Charm Deluxe. Aprendera consejos y recomendaciones utiles sobre como jugar a la tragamonedas, lineas de pago y apuestas, aprendera todo sobre los bonos, asi como consejos sobre como ganar en Lucky Lady Charm.
интернет провайдеры по адресу
domashij-internet-ufa003.ru
интернет по адресу дома
Aposte com 100$ de Bônus no gbg bet – Cadastre-se
e Aproveite!
Online casinos should curb—care play! plinko
Tule vali sobivaim laen ning esita taotlus. Vastus koheselt. Raha katte samal paeval. Meie platvormil esindatud laenud on hoolikalt valitud ja neil on korge usaldusvaarsus. Meie saidi labipaistvus ja kasutajasobralikkus annavad teile voimalused laenud tallinn
мрстбет [url=https://www.mostbet6038.ru]https://www.mostbet6038.ru[/url] .
Аренда авто Краснодар
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наши товары:
Плоская мишень Ho 3N
I don’t trust online RNG—feels off! aviator game
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наша продукция:
Керамическая мишень для распыления оксида титана
Online casinos should ease rules—tough start! plinko
мостбет скачать на андроид [url=www.mostbet7001.ru]www.mostbet7001.ru[/url] .
Предприятие «Энерго Техстрой 2000» поставляет производственные материалы, технику российского производства. Ими смогут воспользоваться любые производственные предприятия страны. На сайте https://best-vendor.ru изучите полный ассортимент товаров, которые вы сможете приобрести в любое время, а доставка происходит наиболее комфортным для вас способом
Medicament information sheet. Generic Name.
how to get cheap xenical pill
Everything information about drugs. Get information now.
Блю систем симфония
Всегда считал, что покупка диплома о высшем образовании — это миф и невозможно. Но, к счастью, оказался неправ. Сначала искал информацию по теме: диплом купить проведенный, купить диплом о среднем образовании в кирове, купить диплом о высшем образовании цена, где купить настоящий диплом, купить дипломы о среднем профессиональном образовании, а затем переключился на дипломы вузов. Подробности здесь: [url=http://proffdiplomik.com/tver/]proffdiplomik.com/tver[/url]
Online laws are off—sort it! ganesha gold
Online casinos need signs—flag us! mines game
The mobile experience at online casinos needs serious improvement. marvel casino
Посетите сайт https://bzdostup.ru/ и вы сможете купить бетон и раствор с доставкой по Краснодару и краю по выгодной цене от производителя. Ознакомьтесь на сайте с ценами и условиями доставки. Вы также можете забрать бетон самовывозом. Бетонный завод Доступ – это строгое соблюдение ГОСТа и круглосуточный режим работы.
Online casinos should be upfront about odds! mines
служба поддержки мостбет номер телефона [url=www.mostbet5006.ru]www.mostbet5006.ru[/url] .
Need real estate? luxury property in Montenegro villas, houses and apartments in Budva, Kotor, Tivat and on the coast. Profitable investment, sea view, safety and comfort in the south of Europe.
Zelite li se odmoriti? http://www.booking-zabljak.com Rezervirajte udoban hotel u centru ili u podnozju planina. Odlican izbor za skijanje i ljetovanje. Jamstvo rezervacije i stvarne recenzije.
need a move? toronto to calgary moving cost turnkey: packing, loading, transport, insurance and support. Without stress and with a guarantee of the safety of your property.
melbet kg [url=melbet1001.ru]melbet kg[/url] .
Автоперевозки из Китая http://rkiyosaki.ru/discussion/13059/avtoperevozka-iz-kitaya-zimoy-riski-i-osobennosti/ доставка грузов по РФ и странам СНГ. Сборные и индивидуальные партии, оформление, отслеживание и страхование. Быстро, надёжно, под ключ.
Pills information leaflet. Drug Class.
how can i get dramamine for sale
Everything trends of drugs. Read here.
I love the live roulette—real deal! minas
Система менеджмента управления качеством содержит критерии менеджмента и стандарты, по которым проводится последующая сертификация предприятия. Чтобы получить сертификат СМК, нужно обратиться в аккредитованный сертификационный центр. Сертификация систем менеджмента качества является документальным подтверждением того, что система менеджмента сертифицированной компании соответствует требованиям того или иного стандарта. Подробнее: https://ok.ru/group/70000034956977
This promo code grants new players a 100% bonus on their first deposit, up to 15,600 BDT. This offer is designed to enhance your betting experience, allowing you to get extra funds as soon as you make your initial deposit https://blogger-mania.mn.co/posts/83168702?utm_source=manual
мостбет зеркало [url=www.mostbet7001.ru]www.mostbet7001.ru[/url] .
тревожная кнопка росгвардия москва [url=www.trevros.ru/]тревожная кнопка росгвардия москва[/url] .
Whether you’re eyeing a Premier League upset or chasing jackpots on vibrant slots, this exclusive offer sets you up for success https://hallbook.com.br/blogs/536324/C%C3%B3digo-Promocional-Gratis-1xBet-Mejores-Ofertas-Hoy
Аренда авто Краснодар
I won big online last night—still in shock! marvel casino
https://bioniclerpg.getbb.ru/viewtopic.php?f=6&t=5116
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
поставляемая продукция:
Однораструбный медный РѕР±РІРѕРґ РїРѕРґ пайку 14С…10С…0.6 РјРј 10.6С…12.6 РјРј мягкая пайка Рњ3С‚ ГОСТ Р 52922-2008 Выбирайте высококачественные медные однораструбные РѕР±РІРѕРґС‹ РїРѕРґ пайку для надежных соединений. Рзготовлены РёР· меди высокой чистоты СЃ отличной теплопроводностью Рё устойчивостью Рє РєРѕСЂСЂРѕР·РёРё. Рдеальны для систем водоснабжения Рё отопления.
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наши товары:
Медная однораструбная редукционная переходная муфта РїРѕРґ пайку стандартная 70С…66.7 РјРј твердая пайка Рњ1Р• ГОСТ Р 52922-2008 Приобретите высококачественные медные однораструбные редукционные муфты РїРѕРґ пайку РѕС‚ Редметсплав для надежного соединения трубопроводов. Гарантированная прочность Рё устойчивость Рє РєРѕСЂСЂРѕР·РёРё. Рдеальны для различных систем отопления, водоснабжения Рё прочих технических целей.
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – наилучшее решение для вашего бизнеса.
Наша продукция:
Медная однораструбная редукционная переходная муфта РїРѕРґ пайку стандартная 70С…66.7 РјРј мягкая пайка Рњ2Рњ ГОСТ Р 52922-2008 Приобретите высококачественные медные однораструбные редукционные муфты РїРѕРґ пайку РѕС‚ Редметсплав для надежного соединения трубопроводов. Гарантированная прочность Рё устойчивость Рє РєРѕСЂСЂРѕР·РёРё. Рдеальны для различных систем отопления, водоснабжения Рё прочих технических целей.
Петр Авен
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наша продукция:
Мишень рутения 3N5
https://www.floristic.ru/forum/groups/moskva-d872-pr-agentstvo-moskvy.html#gmessage1346
The thrill of online slots keeps me spinning! aviator game
Medicines information. What side effects can this medication cause?
can i order cheap zanaflex without insurance
Best trends of drug. Read here.
разработка сайта на битрикс 1с [url=http://razrabotka-saita-bx.ru]http://razrabotka-saita-bx.ru[/url] .
РедМетСплав предлагает широкий ассортимент высококачественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица товара подтверждена всеми необходимыми документами, подтверждающими их качество. Опытная поддержка – то, чем мы гордимся – мы на связи, чтобы ответить на ваши вопросы и находить ответы под специфику вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
Наша продукция:
Молибден МР-47 Молибден МР-47 – это высококачественный металл, обладающий превосходными свойствами. Он широко используется в aerospace, электронике и химической промышленности благодаря своей высокой прочности и устойчивости к коррозии. Молибден МР-47 отличается от других сплавов большим содержанием молибдена, что обеспечивает отличные эксплуатационные характеристики даже в экстремальных условиях. Если вы хотите купить Молибден МР-47, вы получите надежный материал для своих проектов, который прослужит долгие годы. Не упустите возможность улучшить качество своей работы с этим уникальным продуктом!
РедМетСплав предлагает внушительный каталог качественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица продукции подтверждена требуемыми документами, подтверждающими их происхождение. Опытная поддержка – наш стандарт – мы на связи, чтобы улаживать ваши вопросы по мере того как предоставлять решения под требования вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в множестве наших преимуществ
Наша продукция:
РќРёРѕР±РёР№ РќР‘1 РќРёРѕР±РёР№ РќР‘1 – высококачественный полупроводниковый материал, используемый РІ различных отраслях, включая электронику Рё аэрокосмическую индустрию. Обладает отличными электрофизическими свойствами Рё устойчивостью Рє РєРѕСЂСЂРѕР·РёРё. Рто идеальный выбор для тех, кто ищет надежное решение для СЃРІРѕРёС… проектов. РќРёРѕР±РёР№ РќР‘1 идеально РїРѕРґС…РѕРґРёС‚ для создания сверхпроводников, обеспечивая эффективность Рё долговечность. Его востребованность объясняется высокой теплопроводностью Рё прочностью. Если РІС‹ хотите обеспечить СЃРІРѕР№ проект качественным материалом, вам стоит купить РќРёРѕР±РёР№ РќР‘1. Разнообразие его применений делает его незаменимым РІ современном производстве.
РедМетСплав предлагает широкий ассортимент качественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица товара подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Превосходное обслуживание – наша визитная карточка – мы на связи, чтобы ответить на ваши вопросы по мере того как предоставлять решения под специфику вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
Лист висмутовый Sn89Zn8Bi3 – ISO 9453 Лист висмутовый Sn89Zn8Bi3 – ISO 9453 – это высококачественный материал, предназначенный для различных технологий пайки. Обладая отличной пластичностью Рё РЅРёР·РєРѕР№ температурой плавления, РѕРЅ идеально РїРѕРґС…РѕРґРёС‚ как для профессионалов, так Рё для домашних мастеров. Ртот лист поможет вам достичь надежного соединения РІ электронике Рё РґСЂСѓРіРёС… областях. Если РІС‹ ищете надежный Рё эффективный материал, то вам стоит купить Лист висмутовый Sn89Zn8Bi3 – ISO 9453. РћРЅ удовлетворит требования современных стандартов Рё обеспечит долговечность ваших соединений.
Online casinos need gear—aid us! plinko
мелбет кж [url=http://melbet1001.ru]http://melbet1001.ru[/url] .
На сайте https://officepro54.ru представлено огромное количество мебели, которая идеально подходит для организации офисного пространства. Вся она выполнена из инновационных, уникальных материалов, а потому прослужит очень долго, не синтезирует в воздух опасных веществ. В разделе вы найдете кабинет руководителя, функциональные и вместительные столы для переговоров, шкафы-купе, сейфы, акустические системы, ученическую мебель и многое другое. Постоянно действуют акции. На продукцию установлены привлекательные цены.
Meds information sheet. What side effects?
cheap priligy without prescription
Everything what you want to know about medicines. Get now.
The live dealer games online are a game-changer—so immersive! casino mate
На сайте http://oknaksa.ru закажите расчет технического задания, чтобы воспользоваться такой важной, полезной услугой, как остекление. На монтажные работы, а также сами конструкции предоставляются гарантии, вы сможете воспользоваться бесплатным обслуживанием. А все работы выполняются точно по ГОСТу, в соответствии с требованиями. Предоставляются исчерпывающие и содержательные консультации. Вам будет предложено несколько вариантов решения проблемы. Компания располагает огромным количеством готовых проектов.
создание сайтов битрикс заказать [url=https://razrabotka-saita-bx.ru]создание сайтов битрикс заказать[/url] .
The slot themes online shine—play fun! plinko
Приобрести диплом об образовании. Приобретение документа о высшем образовании через надежную фирму дарит множество достоинств для покупателя. Данное решение позволяет сэкономить время и серьезные финансовые средства. [url=http://romawki.flybb.ru/viewtopic.php?f=3&t=2374/]romawki.flybb.ru/viewtopic.php?f=3&t=2374[/url]
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – идеальный вариант для вас.
Наши товары:
Медный переходной пресс тройник 80х22 мм М3РМ ГОСТ Р52948-2008 Приобретайте надежные медные переходные тройники для соединения труб с разным диаметром. Устойчивы к коррозии, легки в монтаже и обеспечивают надежное соединение. Широкий выбор размеров. Гарантированное качество и долговечность.
Сайт cryptopp.ru помогает принимать обоснованные решения. Мы прогнозируем динамику криптовалюты с помощью нейронных сетей на основе исторических данных. У вас есть возможность в специальные поля идентификатор монеты (например, биткоин) либо адрес контракта ввести. Далее вам нужно выбрать сеть. После этого нажать на кнопку «Получение данных». Ищете crypto price predictor прогнозы криптовалют? Cryptopp.ru – здесь размещена подробная информация. Наша миссия – предоставить вам инструменты и аналитические данные для наилучшего понимания рынка криптовалют. Добро пожаловать в Cryptopp!
тревожная кнопка цена [url=http://trevros.ru/]http://trevros.ru/[/url] .
Medicament information sheet. What side effects?
can you get cheap levitra price
Some about drug. Get here.
The music online is dope—keeps me going! minas juego
РедМетСплав предлагает внушительный каталог отборных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица изделия подтверждена требуемыми документами, подтверждающими их качество. Опытная поддержка – наш стандарт – мы на связи, чтобы улаживать ваши вопросы а также адаптировать решения под специфику вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
Гафний ГФМ-1 Гафний ГФМ-1 – это высококачественный материал, который обладает уникальными физико-химическими свойствами, позволяя использовать его РІ различных отраслях, включая электронику Рё атомную энергетику. РћРЅ характеризуется высокой температурной стойкостью Рё отличной РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ устойчивостью. Наш РїСЂРѕРґСѓРєС‚ идеально РїРѕРґС…РѕРґРёС‚ для производства термодинамических Рё электрических компонентов. Если РІС‹ хотите повысить эффективность своего производства Рё улучшить качество СЃРІРѕРёС… изделий, вам стоит купить Гафний ГФМ-1. Рто надежное решение для вашего бизнеса!
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым текущие трудности превращаются в завтрашние успехи.
Наша продукция:
Высокочистый кобальт формы “Цилиндр” (3N5)
O dobrowin oferece uma excelente oportunidade para quem deseja
começar sua experiência no cassino online com um bônus de 100$
para novos jogadores! Ao se registrar no site, você
garante esse bônus exclusivo que pode ser utilizado
em diversos jogos de cassino, como slots, roleta
e poker. Esse é o momento perfeito para explorar o mundo das apostas com um saldo extra, aproveitando ao máximo suas apostas sem precisar investir
um grande valor logo de início. Não perca essa oportunidade
e cadastre-se já!
читать [url=https://gcup.ru]Программирование[/url]
The customer reviews for online casinos are all over the place—hard to choose! aviator
reference https://binslist.com
MetaMask Download changed my crypto experience. Managing tokens has never been easier. A must-have for anyone in the space.
Medication information for patients. Short-Term Effects.
doxycycline and nerve damage
Everything about drug. Get now.
The slot thrill online flies—pulse high! doradobet
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Керамическая мишень Титаната Бария
создание сайтов на битрикс стоимость [url=http://www.razrabotka-saita-bx.ru]http://www.razrabotka-saita-bx.ru[/url] .
Thanks for breaking this down so simply!
mostbet.kg [url=https://mostbet5006.ru/]mostbet.kg[/url] .
ремонт телефонов самсунг Ремонт телефонов Samsung в Красноярске недорого. Срочный ремонт телефона Самсунг за 30 минут.
ремонт подсветки телевизора LG Ремонт подсветки телевизора LG в Красноярске недорого. Бесплатная диагностика. Ремонт подсветки телевизоров LG на дому.
hot hot fruit games
установка тревожной сигнализации [url=www.trevros.ru]www.trevros.ru[/url] .
Tent3302.ru необходимые автодетали предоставляет. Мы продаем запчасти на Газель Некст. Под заказ поставляем двигатель Газель Камминз 2.8. Стараемся поддерживать цены на самом низком уровне. Продажи выполняем со склада за безналичный и наличный расчет. Ищете приемная труба валдай? Tent3302.ru – тут можете детальную о нас информацию отыскать. Продаем качественную продукцию. Особенным спросом у клиентов пользуются шины на Газель. Стараемся максимально сократить транспортные и накладные расходы. Вы можете быть уверены в вежливом и грамотном обслуживании.
Мы готовы предложить дипломы любых профессий по приятным тарифам.
Вы заказываете диплом в надежной и проверенной временем компании. Приобрести диплом о высшем образовании– [url=http://nowoczesna.phorum.pl/posting.phpmode=newtopic&f=2&sid=f0f3406dc2c992748349484cf6faa9b5/]nowoczesna.phorum.pl/posting.phpmode=newtopic&f=2&sid=f0f3406dc2c992748349484cf6faa9b5[/url]
melbet [url=https://melbet1002.ru]https://melbet1002.ru[/url] .
Дорого игрок, идешь где можно купить скины КС 2 или КСГО? Отлично,твой поиск официально завершен! В этой подборке сайтов вы найдете самые лучшие магазины для покупки дешевых скинов КС 2 cs купить скины . Предлагает непревзойденный опыт покупки скинов, включая все, от редких коллекционных предметов до самых рентабельных игровых скинов.
melbet кыргызстан [url=www.melbet1002.ru]www.melbet1002.ru[/url] .
Букет для свекрови – лед тронулся!
гипсофилы цена букета
мелбет кг [url=https://www.melbet1002.ru]https://www.melbet1002.ru[/url] .
Drugs information. Long-Term Effects.
how to buy loperamide without insurance
Actual what you want to know about medication. Read now.
mostbet игры [url=mostbet7003.ru]mostbet7003.ru[/url] .
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
Наша продукция:
Медная двухраструбная редукционная переходная муфта РїРѕРґ пайку 14С…9 РјРј твердая пайка Рњ3С‚ ГОСТ Р 52922-2008 Выберите высококачественные медные двухраструбные редукционные муфты РїРѕРґ пайку РѕС‚ Редметсплав для надежного соединения медных труб различных диаметров. РЁРёСЂРѕРєРёР№ выбор размеров, эффективная установка Рё долговечность гарантированы. Рдеальное решение для систем отопления Рё водоснабжения.
аренда склада для хранения [url=www.hranenievesheymsk.ru/]аренда склада для хранения[/url] .
melbet [url=http://melbet1002.ru/]http://melbet1002.ru/[/url] .
Заказать диплом можно используя сайт компании. [url=http://forum.resmihat.kz/viewtopic.phpf=10&t=2409718/]forum.resmihat.kz/viewtopic.phpf=10&t=2409718[/url]
mines predictor apk
melbet сайт [url=https://melbet1001.ru]https://melbet1001.ru[/url] .
Приобрести диплом ВУЗа. Покупка диплома через надежную фирму дарит ряд достоинств. Данное решение помогает сэкономить как личное время, так и серьезные финансовые средства. [url=http://lakarjobbisverige.se/employer/4939/eonline-diploma/]lakarjobbisverige.se/employer/4939/eonline-diploma[/url]
РедМетСплав предлагает внушительный каталог качественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы обеспечиваем быстрое выполнение вашего заказа.
Каждая единица продукции подтверждена требуемыми документами, подтверждающими их качество. Превосходное обслуживание – наша визитная карточка – мы на связи, чтобы ответить на ваши вопросы и адаптировать решения под требования вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
Пруток магниевый AZ21A – ASTM B275 Проволока магниевая AZ21A – ASTM B275 – это инновационный материал, специально разработанный для удовлетворения современных стандартов качества. РћРЅР° обладает высокой прочностью Рё легкостью, что делает ее идеальной для использования РІ различных отраслях, таких как авиация Рё автомобилестроение. Благодаря отличной устойчивости Рє РєРѕСЂСЂРѕР·РёРё, проволока РїРѕРґС…РѕРґРёС‚ для долгосрочной эксплуатации. Если РІС‹ ищете надежное решение для СЃРІРѕРёС… проектов, РЅРµ упустите возможность купить Проволока магниевая AZ21A – ASTM B275. Ртот РїСЂРѕРґСѓРєС‚ поможет вам достичь отличных результатов Рё повысить эффективность ваших работ.
plinko game download
1win.kg [url=https://mostbet5007.ru/]1win.kg[/url] .
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние возможности.
Наша продукция:
Гранулы оксида циркония высокой чистоты
посетить сайт [url=https://kentcasino.io/]casino r7[/url]
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
поставляемая продукция:
Медная однораструбная редукционная переходная муфта РїРѕРґ пайку стандартная 35С…27.4 РјРј твердая пайка Рњ1 ГОСТ Р 52922-2008 Приобретите высококачественные медные однораструбные редукционные муфты РїРѕРґ пайку РѕС‚ Редметсплав для надежного соединения трубопроводов. Гарантированная прочность Рё устойчивость Рє РєРѕСЂСЂРѕР·РёРё. Рдеальны для различных систем отопления, водоснабжения Рё прочих технических целей.
скачать 1win с официального сайта [url=www.mostbet5007.ru]скачать 1win с официального сайта[/url] .
Посетите B2BOX https://b2box.ru/ – это уникальное комплексное решение для создания упаковки всех видов и направлений. Мы делаем упаковку, которая продает! Упаковочные решения для ритейла, создание индивидуальной и SRP упаковки, услуги дизайнерского бюро, производство транспортной упаковки, собственное плоттерное производство, все виды печати – это и многое другое вы найдете, посетив наш сайт.
На сайте http://www.lilibum.ru вы найдете много анкет реальных людей для того, чтобы завести знакомства, найти свою вторую половинку и просто интересно провести время, если пригласите кого-то на свидание. Для того чтобы составить мнение о человеке, можно обменяться фотографиями и пообщаться на определенные темы, которые вас интересуют. Самые привлекательные и интересные люди вашего города находятся на этом портале. Знакомьтесь, влюбляйтесь и создавайте семьи. Для получения доступа ко всем функциям, пройдите регистрацию.
Drug prescribing information. Generic Name.
can metoprolol cause hypoglycemia
All about medicine. Read information here.
1win. [url=http://mostbet5007.ru/]http://mostbet5007.ru/[/url] .
1win играть [url=https://mostbet5007.ru]https://mostbet5007.ru[/url] .
Для максимально быстрого продвижения вверх по карьере потребуется наличие официального диплома о высшем образовании. Приобрести диплом о высшем образовании у надежной фирмы: [url=http://diplom-top.ru/diplom-ufa-kupit-3/]diplom-top.ru/diplom-ufa-kupit-3/[/url]
plinko demo
РедМетСплав предлагает широкий ассортимент высококачественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы обеспечиваем оперативное исполнение вашего заказа.
Каждая единица изделия подтверждена соответствующими документами, подтверждающими их происхождение. Дружелюбная помощь – наш стандарт – мы на связи, чтобы ответить на ваши вопросы и адаптировать решения под специфику вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
Наша продукция:
Поковка кобальтовая ЮНДК35Т5АА Поковка кобальтовая ЮНДК35Т5АА – это высококачественный металлургический продукт, применяемый в различных отраслях, включая машиностроение и авиастроение. Благодаря своим уникальным свойствам, таким как высокая прочность и стойкость к коррозии, эта поковка идеально подходит для изготовления деталей, работающих в сложных условиях. Если вы ищете надежные и долговечные решения для своего производства, купить Поковка кобальтовая ЮНДК35Т5АА – это правильный выбор. Она обеспечивает отличные эксплуатационные характеристики и долгосрочную службу. Обеспечьте свое производство качественными материалами с этой поковкой!
mines casino game free
Your content never disappoints. Keep it up!
can i purchase generic zenegra no prescription
Посетите страницу https://www.drive2.ru/o/b/691804470933197117/ и вы сможете ознакомиться с тем как происходит оклейка плёнкой Porsche 911 от профессионалов. Посмотрите как стал выглядеть Porsche 911 Carrera GTS после оклейки. Оклейка Porsche 911 выполнялась пленкой Vega 230 ST – узнайте почему и все ее преимущества в статье. Porsche 911 удивительный автомобиль – вам будет интересно читать!
Купить диплом института!
Мы изготавливаем дипломы любой профессии по приятным ценам. Вы заказываете диплом в надежной и проверенной компании. : [url=http://designxri.com/employer/frees-diplom/]designxri.com/employer/frees-diplom[/url]
Pills information leaflet. Generic Name.
how can i get cheap cephalexin without prescription
Everything news about medication. Get information now.
mines app
Онлайн-портал про автомобили https://impactspreadsms.com с каталогом моделей, тестами, аналитикой, ценами и отзывами. Удобный поиск и полезные материалы — для тех, кто выбирает с умом.
Портал для женщин https://gracefulwoman.kyiv.ua которые ценят красоту жизни. Практичные советы, душевные статьи и поддержка — о том, как быть счастливой, уверенной и гармоничной каждый день.
Портал про авто https://impactspreadsms.com новости, обзоры, тест-драйвы, советы, сравнение моделей и актуальные тенденции в мире автомобилей. Всё для автолюбителей и профессионалов.
Receba 100$ de Bônus no wingdus e Comece a Apostar Agora!
Visit https://coinintel-hq.com/ and you will learn all about the world of cryptocurrency. Explore the world of cryptocurrency like never before with our stunning graphics, fascinating charts, and fascinating infographics. Whether you are a crypto enthusiast, an investor, or just curious about the world of digital currencies, CoinIntel HQ is the place to go for a visually stunning and educational experience.
Современный женский https://happylady.kyiv.ua сайт с ежедневными обновлениями: советы по стилю, уходу, семье и психологии. Всё, что волнует и вдохновляет женщин сегодня — в одном месте.
bet on red app
Посетите сайт https://artradol.com/ и вы узнаете все про Артрадол – это препарат для лечения суставов от производителя. Узнайте все преимущества нестероидного противовоспалительного препарата для лечения суставов. Помогает бороться с основными заболеваниями суставов: артритом, остеохондрозом и остеоартрозом. Подробности, инструкция применения и где купить можно узнать на сайте.
мостюет [url=https://mostbet5006.ru]https://mostbet5006.ru[/url] .
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наши товары:
Бронзовое внутреннее стопорное пружинное кольцо M34 36.5х1.5 мм БрАЖНМц9-4-4-1 DIN 472 Широкий выбор бронзовых внутренних стопорных колец по DIN для различных отраслей. Прочные, износостойкие и устойчивые к коррозии. Обеспечивают надежную фиксацию вала и защиту от нежелательного движения. Заказывайте у нас прямо сейчас!
plinko wahrscheinlichkeit
fortune tigrinho
Букет произвел фурор на юбилее!
доставка цветов
мостбет [url=http://mostbet5006.ru/]http://mostbet5006.ru/[/url] .
Medicament prescribing information. Generic Name.
cost generic tadacip online
All news about medicament. Get information now.
aviator game online
Ищете, где заказать надежную кухню на заказ по вашим размерам за адекватные деньги? Посмотрите портфолио кухонной фабрики GLORIA – https://gloriakuhni.ru/ . Все проекты выполнены в Санкт-Петербурге и области. На каждую кухню гарантия 36 месяцев, более 800 цветовых решений. Большое разнообразие фурнитуры. Удобный онлайн-калькулятор прямо на сайте и понятное формирование цены. Много отзывов клиентов, видео-обзоры кухни с подробностями и деталями. Для всех клиентов – столешница и стеновая панель в подарок.
Купить диплом на заказ можно используя сайт компании. [url=http://cartagena.activeboard.com/forum.spark/]cartagena.activeboard.com/forum.spark[/url]
РедМетСплав предлагает внушительный каталог отборных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы обеспечиваем быстрое выполнение вашего заказа.
Каждая единица товара подтверждена требуемыми документами, подтверждающими их качество. Опытная поддержка – наш стандарт – мы на связи, чтобы ответить на ваши вопросы а также находить ответы под специфику вашего бизнеса.
Доверьте вашу потребность в редких металлах специалистам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
поставляемая продукция:
Лист висмутовый Р›130 Лист висмутовый Р›130 – это высококачественный материал, идеально подходящий для применения РІ различных отраслях. Обладая уникальными физико-химическими свойствами, РѕРЅ широко используется РІ электронике, медицинской технике Рё научных исследованиях. Ртот лист отличается превосходной РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью Рё высокой теплопроводностью, что делает его незаменимым РІ производстве специализированных изделий. Если РІС‹ ищете надежный Рё долговечный материал, РЅРµ упустите шанс купить Лист висмутовый Р›130. Рнвестируйте РІ качество Рё получите товар, который оправдает ваши ожидания!
Свежие новости Украины https://fraza.kyiv.ua и мира — политика, экономика, общество, культура, технологии. Главные события дня, оперативные обновления и аналитика от экспертов.
На сайте https://pilmi.ru вы найдете огромное количество фильмов самых разных жанров, включая комедии, мелодрамы, драмы, романтические. Для того чтобы подыскать наиболее подходящий вариант, нужно воспользоваться специальным каталогом. Вашему вниманию представлены и ожидаемые любопытные новинки этого года, которые произведут эффект. Все это можно просматривать в любое время и на различных устройствах. Прямо сейчас воспользуйтесь возможностью посмотреть азиатское кино. Используйте поиск для облегчения выбора.
where to buy proscar without insurance
Модный журнал онлайн https://icz.com.ua одежда, аксессуары, макияж, прически, уличный стиль и haute couture. Следите за последними тенденциями и читайте советы экспертов индустрии.
There is evidently a lot to know about this. I feel you made various nice points in features also.
Современный портал https://lady.kyiv.ua для женщин: мода, уход, любовь, дети, стиль жизни и вдохновение. Полезный контент, тренды и темы, близкие каждой.
Актуальные новости Украины https://lenta.kyiv.ua и мира на одном сайте. Подборка ключевых событий, факты, интервью, мнения и видео. Честно, быстро и без фейков.
dragon tiger game download 51 bonus
melbet сайт [url=http://melbet1001.ru/]http://melbet1001.ru/[/url] .
This versatile bonus is tailored to every player, making 1xBet the place to go for sports and casino https://www.soft-clouds.com/blogs/197453/1xBet-Free-Bet-Promo-Code-Bonus-130
Джойказино: мир азарта и больших возможностей!Погрузитесь в атмосферу ярких эмоций! Сотни слотов, live-игры с реальными дилерами, турниры с призами — выбирайте развлечения по вкусу. Регистрация за пару кликов, бонусы для всех игроков и моментальные выплаты. Лицензия гарантирует честность, а поддержка работает круглосуточно. Удобный интерфейс и мобильная версия оценят даже новички https://joyofficial.amebaownd.com/posts/56393217
Kuruçeşme su kaçağı tespiti Beklentilerimizi Karşıladı: Başta tereddüt ettik ama gerçekten beklentilerimizin üzerinde bir hizmet aldık. http://kristin-fereira.com/uncategorized/uskudar-su-kacagi-tespiti/
узнать больше [url=https://vodkabetslot.ru/]водкабет[/url]
Short but packed with value. Love it!
Приветствую!
Для определенных людей, приобрести [b]диплом[/b] университета – это необходимость, возможность получить достойную работу. Однако для кого-то – это понятное желание не терять время на учебу в институте. С какой бы целью вам это не потребовалось, наша компания готова помочь вам. Оперативно, профессионально и по доступной цене сделаем документ нового или старого образца на подлинных бланках со всеми требуемыми печатями.
Главная причина, почему многие покупают документы, – получить хорошую должность. Предположим, способности и опыт дают возможность кандидату устроиться на работу, а подтверждения квалификации нет. При условии, что для работодателя важно наличие “корочки”, риск потерять вакантное место очень высокий.
Приобрести документ ВУЗа можно в нашем сервисе. Мы оказываем услуги по продаже документов об окончании любых ВУЗов Российской Федерации. Вы получите необходимый диплом по любой специальности, включая документы образца СССР. Гарантируем, что при проверке документов работодателями, подозрений не возникнет.
Ситуаций, которые вынуждают купить диплом много. Кому-то прямо сейчас требуется работа, а значит, нужно произвести впечатление на начальника во время собеседования. Другие задумали попасть в престижную компанию, для того, чтобы повысить свой статус в обществе и в последующем начать свой бизнес. Чтобы не тратить впустую годы жизни, а сразу начинать эффективную карьеру, применяя врожденные таланты и приобретенные навыки, можно заказать диплом через интернет. Вы станете полезным для общества, обретете финансовую стабильность в кратчайший срок- [url=http://diploman-russian.com/]купить аттестат[/url]
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние успехи.
Наша продукция:
Керамическая мишень Титаната Бария
Meds information leaflet. Effects of Drug Abuse.
where to get zoloft
Best what you want to know about medicines. Read here.
https://mamapoltava.listbb.ru/viewtopic.php?f=45&t=12894
https://dom-nam.ru/index.php/forum/razdel-predlozhenij/164118-toniziruyushchij-napitok-eazy-energy#202734
https://telegra.ph/Vybor-cveta-kolpakov-dlya-kirpichnyh-stolbov-zabora-04-18
erfahrungen plinko
мостбет промокод [url=https://mostbet7003.ru]https://mostbet7003.ru[/url] .
Портал для женщин https://maleportal.kyiv.ua мода, красота, здоровье, отношения, карьера, семья и вдохновение. Актуальные темы, полезные советы и поддержка для каждой женщины — всё в одном месте.
https://maroc.borda.ru/?1-4-0-00000605-000-0-0-1741947477
Сервисный центр Мобиопт – Ремонт телефонов Киров
Онлайн-портал для современных https://madrasa.com.ua женщин. Всё, что волнует и вдохновляет: от красоты и моды до жизненного баланса, мотивации и личностного роста. Будь собой — с нами.
Клуб для беременных https://mam.ck.ua и молодых мам: питание, подготовка к родам, уход за малышом, послеродовое восстановление. Полезная информация, консультации и поддержка в одном месте.
Главные новости https://lentanews.kyiv.ua из Украины и со всего мира — ежедневно и без искажений. Всё, что важно знать: внутренняя политика, экономика, международные события и прогнозы.
bet on red bonus code
http://forum.omnicomm.pro/index.php/topic,112980.0.html
https://telegra.ph/Kak-vybrat-kolpaki-na-stolby-dlya-zabora-po-prochnosti-04-18
how to get cheap motilium pill
На сайте https://xn—-7sbjhqn0bhjc0lk.xn--p1ai/ получите юридическую консультацию по различным вопросам. Компания Стратегия работает как с физическими, так и частными лицами. На все услуги установлены привлекательные расценки, чтобы воспользоваться ими смог каждый. Предприятие работает в этой сфере более 11 лет. В команде трудятся 11 специалистов, которые справятся с задачей независимо от сложности. К вашим услугам досудебный юрист, досудебное урегулирование, кредитный, семейный юрист, решение страховых споров.
драгон мани регистрация [url=https://dragon-money37.com]драгон мани регистрация[/url] .
Ao se cadastrar no brwin,
você ganha um bônus de 100$ para começar sua jornada no cassino
com o pé direito! Não importa se você é um novato ou um
apostador experiente, o bônus de boas-vindas é a
oportunidade perfeita para explorar todas as opções que o
site tem a oferecer. Jogue seus jogos favoritos, descubra novas opções de apostas e
aproveite para testar estratégias sem risco, já que o bônus
ajuda a aumentar suas chances de ganhar. Cadastre-se
hoje e comece com 100$!
рассчитать стоимость страховки авто [url=seodict.ru]seodict.ru[/url] .
Потеря близкого человека — это тяжелое испытание, и в такой момент важно, чтобы всё было организовано достойно и без лишних хлопот. ГБУ «Ритуал» Москва https://ritualmsk.com/ предлагает полный спектр ритуальных услуг, беря на себя все заботы: от транспортировки и оформления документов до выбора места захоронения. Наши специалисты помогут вам организовать похороны в Москве по всем правилам, обеспечивая уважение к памяти усопшего и поддержку для родственников.
Are you ready to feel thrilling emotions playing one of the best crash games ever pin up casino aviator signals
Drugs information sheet. Effects of Drug Abuse.
where can i get atarax without dr prescription
Actual information about medication. Read now.
Доверие – центр, который психологические услуги предоставляет. Мы помогаем людям справляться с трудностями, которые у них возникают в отношениях с собой и окружающими. Записаться к специалисту на личный прием вы можете по телефону на портале. Ищете психотерапия? Ack-group.ru/stati-po-psikhologii/psikhoterapiya – тут узнаете, что такое психотерапия, кому она показана и какие перед ней стоят цели. Также здесь на психологические консультации представлены расценки. Всегда готовы поддержать вас на пути к самопознанию и эмоциональному равновесию.
plinko demo
Our website https://managerscasino.com/ is the ultimate guide for players who want to take control of their casino experience. It is where I share my ideas, strategies and tips for players who want to be in control, not just another number on the casino profit margin. My goal is to help players navigate the casino world with confidence, knowledge and a winning attitude.
plinko ?? ?????
fortune tiger demo gratis
Сайт для деловых людей https://manorsgroup.com.ua актуальные материалы о финансах, инвестициях, рынке недвижимости и управлении капиталом. Аналитика, обзоры, экспертные мнения и полезные инструменты.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние достижения.
Наша продукция:
Плоская мишень из германия 5N, 6N
Женский онлайн-журнал https://mcms-bags.com о красоте, моде, психологии, отношениях и стиле жизни. Актуальные статьи, советы экспертов, вдохновение и всё, что важно для современной женщины.
Новостной портал https://mediashare.com.ua с актуальной информацией из Украины, мира, политики, экономики, технологий, культуры и общества. Только проверенные источники и объективные материалы.
куда можно сдать вещи на временное хранение [url=www.hranenievesheymsk.ru]куда можно сдать вещи на временное хранение[/url] .
https://telegra.ph/Kak-vybrat-kolpaki-dlya-kirpichnogo-zabora-dlya-nadyozhnosti-04-18
мостбет казино [url=https://mostbet6033.ru/]https://mostbet6033.ru/[/url] .
tabletop cd player and radio https://alarm-radio-clocks.com
мостбет казино войти [url=http://mostbet6033.ru]мостбет казино войти[/url] .
интернет провайдер омск
domashij-internet-omsk001.ru
провайдеры домашнего интернета омск
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
Наши товары:
Пружина из драгоценных металлов серебряная 250х7х0.5 мм СрМ50 ТУ Выберите идеальные пружины из золота, серебра или платины, чтобы дополнить ваше украшение. Найдите пружины с изысканным дизайном и прочностью. Они предлагают широкий выбор для того, чтобы подчеркнуть уникальность вашего украшения.
dragonmoney [url=https://dragon-money36.com/]dragon-money36.com[/url] .
Колпаки защищают столбы от трещин при морозе, это факт.
https://trade-britanica.trade/wiki/User:MaribelAshley4
melhor horario para jogar fortune tiger hoje
Medication information for patients. Effects of Drug Abuse.
can i purchase sumatriptan without dr prescription
Best what you want to know about medicament. Get now.
Guide to Playing Adaptive Integrated Online Games
Guide to Playing Adaptive Integrated Online Games
In an age where creativity and technology converged, a new form of entertainment emerged, transforming how participants engage and interact. This immersive format offers players dynamic scenarios that adapt to styles and choices, fostering an ever-growing community that thrives on innovation. With astonishing advancements in graphics and AI, these experiences have transcended traditional boundaries, providing layers of complexity and engagement previously unseen.
As individuals immerse themselves in these multifaceted interactions, understanding the underlying structures becomes paramount. Players can maximize their enjoyment by recognizing key elements, such as strategic thinking, teamwork, and individual skill advancement. This exploration will showcase nuanced strategies that highlight adaptability, ensuring a rewarding experience, regardless of expertise level.
From tailored narratives to customizable environments, the appeal lies in the personalization available to each participant. Engaging with these systems means embracing the unique opportunities presented and leveraging them to enhance one’s ability to progress and thrive within the ever-expanding universe of interactive entertainment.
Understanding Game Mechanics for Effective Adaptation
Game mechanics encompass the rules and systems that govern interactions within a digital environment. A clear comprehension of these principles allows individuals to adjust their strategies and decisions based on the context of play. Analyzing variables such as player actions, environment responses, and reward systems is essential for mastering this dynamic.
Key components include:
– Feedback Loops: Recognizing positive and negative feedback loops can greatly influence tactics. Positive reinforcement encourages specific behaviors, while negative feedback can deter unproductive actions.
– Resource Management: Efficiently allocating resources such as energy, time, and currency often dictates success. Players should prioritize obtaining, using, and preserving these assets according to objectives.
– Progression Systems: Understanding character or skill development is vital. Gradual improvement through experience points or skill trees allows for a tailored approach that syncs with personal playstyle.
– Player Dynamics: Interactions with others can vary widely. Recognizing social mechanics, such as alliances or rivalries, can forge advantageous strategies. Building rapport with fellow participants may yield cooperative benefits.
– Environmental Interaction: Different environments can dictate available actions. Familiarity with maps or settings contributes to making informed choices when facing challenges or opportunities.
Adaptability relies on an astute awareness of how these mechanics interface. Regular analysis and reflection on actions and outcomes promote refinement of strategies, maximizing potential for achievement.
Experimentation is a powerful tool. Trying different approaches can reveal the most efficient methods of engagement. A calculated risk might open new pathways or yield unexpected rewards, promoting an iterative learning process.
Strategies for Collaborating in Dynamic Environments
Success in interactive platforms often hinges on the ability to work seamlessly with others. Here are actionable strategies for enhancing collaboration:
1. Define Clear Roles: Establish distinct responsibilities within the team. Clarity in individual tasks reduces overlap and confusion, allowing members to focus on their contributions effectively.
2. Utilize Real-Time Communication Tools: Leverage chat applications or voice channels that facilitate instant feedback and discussion. Keeping communication open fosters quick decision-making and helps to maintain momentum.
3. Establish Regular Check-Ins: Schedule frequent brief meetings to assess progress and address concerns. This practice encourages accountability and allows the team to adapt strategies as necessary.
4. Promote a Culture of Feedback: Create an atmosphere where constructive criticism is welcomed. Regularly solicit input from teammates on performance and approaches, enhancing overall team dynamics.
5. Leverage Diverse Skills: Recognize the unique strengths each member brings to the table. Assign tasks that play to these strengths, encouraging individual contribution while ensuring collective effectiveness.
6. Adapt to Changing Situations: Be prepared to modify strategies in response to new data or shifting dynamics. Flexibility is key; reassess priorities regularly based on the current context.
7. Utilize Collaborative Tools: Take advantage of platforms that support joint work, such as shared documents or task management software. These tools can enhance organization and ensure all members are aligned.
8. Build Relationships: Invest time in getting to know team members personally. Strong interpersonal relationships can enhance trust, leading to smoother collaboration and conflict resolution.
By implementing these specific strategies, teams can enhance their cooperative efforts, leading to improved outcomes and a more cohesive environment. Adjusting to new challenges with a focus on collaboration will significantly influence success rates in multi-person scenarios.
Visit my web site https://comojogartigrinho.com
посмотреть в этом разделе [url=https://gcup.ru/]Создание игр[/url]
dragon money официальный сайт вход [url=https://www.dragon-money37.com]https://www.dragon-money37.com[/url] .
online plinko
where buy cheap lozol tablets
рассчитать автостраховку [url=http://seodict.ru]http://seodict.ru[/url] .
РедМетСплав предлагает обширный выбор отборных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы обеспечиваем оперативное исполнение вашего заказа.
Каждая единица изделия подтверждена всеми необходимыми документами, подтверждающими их качество. Превосходное обслуживание – наша визитная карточка – мы на связи, чтобы разрешать ваши вопросы по мере того как находить ответы под специфику вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в гибкости нашего предложения
поставляемая продукция:
Лента кобальтовая РРџ207 Лента кобальтовая РРџ207 – это высококачественный РїСЂРѕРґСѓРєС‚, предназначенный для обработки металлов Рё создания надежных соединений. РћРЅР° обладает отличными механическими характеристиками, что делает её идеальным выбором для профессионалов Рё домашних мастеров. Если РІС‹ ищете прочный Рё долговечный материал для СЃРІРѕРёС… проектов, РЅРµ упустите возможность купить Лента кобальтовая РРџ207. Благодаря своей универсальности, данная лента отлично РїРѕРґС…РѕРґРёС‚ как для резки, так Рё для шлифовки различных материалов. Позаботьтесь Рѕ своем инструменте Рё выберите только лучшее!
aviator game
Магазин аккаунтов социальных сетей https://marketplace-akkauntov-top.ru
Can’t wait to read more posts like this. Subscribed!
Seu Bônus de 100$ Espera por Você no aajogo – Cadastre-se Agora!
Meds information leaflet. What side effects?
can i order cheap nortriptyline online
All what you want to know about drug. Read here.
драгон мани зарегистрироваться [url=www.dragon-money36.com/]драгон мани зарегистрироваться[/url] .
hot hot fruit big win
лучший интернет провайдер омск
domashij-internet-omsk002.ru
провайдеры интернета омск
plinko game review
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
Наша продукция:
Мишень РёР· драгоценного металла или его сплава кольцевая палладий 10С…5 РјРј ПДСР-20 РўРЈ Выберите уникальные мишени РёР· драгоценных металлов для напыления РѕС‚ Редметсплав.СЂС„. Обеспечивают высокую степень защиты Рё долговечность. Рдеальный выбор для промышленности, строительства Рё производства.
мос бет [url=https://mostbet7003.ru]https://mostbet7003.ru[/url] .
скачать мостбет [url=http://mostbet6033.ru]http://mostbet6033.ru[/url] .
order cheap macrobid for sale
predictor aviator
dragon money зеркало сегодня [url=www.dragon-money37.com]www.dragon-money37.com[/url] .
Сервисный центр Мобиопт – Ремонт телефонов Киров
калькулятор осаго онлайн по всем страховым компаниям [url=https://seodict.ru]https://seodict.ru[/url] .
plinko scams
РедМетСплав предлагает широкий ассортимент отборных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица товара подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Дружелюбная помощь – наш стандарт – мы на связи, чтобы улаживать ваши вопросы а также находить ответы под особенности вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
Лента магниевая AZ91 – CSA HG.10 РљСЂСѓРі магниевый AZ91 – CSA HG.10 является высококачественным материалом, идеально подходящим для различных сварочных Рё строительных проектов. Рзготовленный РёР· магниевого сплава, РѕРЅ отличается легкостью Рё высокой прочностью, что делает его идеальным выбором для тех, кто ищет надежность Рё эффективность. Купить РљСЂСѓРі магниевый AZ91 – CSA HG.10 стоит для повышения производительности Рё снижения веса конструкций. Ртот РїСЂРѕРґСѓРєС‚ находит широкое применение РІ авиационной Рё автомобильной промышленностях, обеспечивая отличные эксплуатационные характеристики. РќРµ упустите возможность приобрести этот незаменимый материал!
В случае если желаете узнать самую ценную, полезную и важную информацию о трейдинге, вложениях, то нужно зайти на портал, где вы отыщете все необходимое на такую тему. Здесь доступным языком рассказывается о том, как правильно вести торговлю на внебиржевом рынке. https://fxidea.com/ рекомендует воспользоваться акциями каждому новичку. Быстрее изучите индикаторы, реальные отзывы, а также ознакомьтесь с работающими стратегиями. Вы получите доступ к ценным материалам, касающимся криптовалюты, а также узнаете, как заработать на форексе.
plinko app review
Meds information sheet. Long-Term Effects.
where to get ceftin prices
Some what you want to know about drugs. Read information here.
На сайте https://arenda24.by/ каждый желающий получает возможность взять в прокат спецтехнику, садовый инвентарь для выполнения всех необходимых хозяйственных работ. Если нашли подходящее объявление, то свяжитесь и договоритесь с продавцом для того, чтобы он на выгодных условиях передал технику. Напротив каждого варианта имеются его характеристики, особенности, детальное описание, чтобы потенциальный клиент сразу же сориентировался. Постоянно появляются новые объявления, что позволит найти все, что нужно.
провайдеры интернета в омске
domashij-internet-omsk003.ru
подключить проводной интернет омск
dragon money casino регистрация [url=www.dragon-money36.com]dragon money casino регистрация[/url] .
mostbet [url=https://www.mostbet7003.ru]https://www.mostbet7003.ru[/url] .
aviator game download
how to get diovan price
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние возможности.
Наша продукция:
Плоская мишень из иттербия
fortune mouse demo gratis dinheiro infinito
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Гранулы оксида алюминия 4N
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наши товары:
Высокочистая плоская мишень из оксида никеля
Pills information for patients. Brand names.
order requip pills
Actual about drug. Get information now.
https://zdolbyniv.rv.ua/2024/03/ukrayinska-promyslova-kompaniya-rgs-ukraine/
https://ria-m.tv/ua/news/376639/yak-vibrati-naykrasche-taksi-bezpeka-komfort-i-suchasni-rishennya-v-transportnih-poslugah.html
Посетите сайт https://xn--j1abip.com// и вы сможете ознакомиться с ЖК Толк в Академическом районе города Екатеринбург. Узнайте все преимущества ЖК, его местоположение, инфраструктуру рядом. На сайте вы сможете выбрать квартиру или паркинг. Купить новую квартиру в ЖК от застройщика по выгодной цене рядом с Преображенским парком легко – мы предлагаем различные варианты оплаты и ипотеку по выгодной ставке. Подробнее на сайте.
Bônus de 100$ no bet esporte – https://betesporte-br.com: Faça Seu Cadastro Agora Mesmo!
https://selfhacker.net/media/home/izgotovlenie-shtuczerov-proczess-tehnologii-i-primenenie.html
https://uznay-prezidenta.ru/finance/6422-pochemu-mnogie-devushki-nachinayut-rabotat-v-eskorte.html
Купить Tank – только у нас вы найдете разные комплектации. Быстрей всего сделать заказ на официальный дилер танк в спб можно только у нас!
[url=https://tankautospb.ru]танк дилер петербург[/url]
танк автомобиль китай купить – [url=https://tankautospb.ru]https://www.tankautospb.ru[/url]
aviator app download
I like what you guys are up too. Such clever work and reporting! Keep up the excellent works guys I have incorporated you guys to my blogroll. I think it’ll improve the value of my website 🙂
Ищете сельхозтехнику и оборудование по самым выгодным ценам от производителя? Посетите сайт Производителя ООО МИТЕХ https://mi-teh.ru/ где вы также найдете запасные части и комплектующие к сельхоз машинам и тракторам. Ознакомьтесь с нашим ассортиментом продукции на сайте.
betonred casino
мосбет [url=https://www.mostbet6033.ru]https://www.mostbet6033.ru[/url] .
jeu plinko avis
plinko casino
подключить интернет в квартиру пермь
domashij-internet-perm001.ru
подключить интернет
Джой казино – лицензированное онлайн-казино с большим выбором игровых автоматов. Качественный софт, выгодные акции, обзор официального https://joyofficial.amebaownd.com/posts/56393217
Promo Code Use: Promo codes provide additional chances to win but may have wagering requirements and time limitations 1xbet promo code free spins no deposit
Minivan.online услуги такси (8 мест) по выгодным тарифам предлагает. Работают только опытные водители. Машины бустером, люлькой и детскими креслами оборудованы. Мы стремимся обеспечить клиентам максимально комфортные и безопасные поездки. Ищете детское такси? Minivan.online – здесь представлена более детальная информация о нашей компании. Своевременную подачу авто мы гарантируем. Готовы ответить на интересующие вас вопросы и помочь с оформлением заказа. Минивэн онлайн – надежность и безупречный сервис. Удостоверьтесь в этом лично!
Авто сайт с обзорами https://microbus.net.ua новостями, тест-драйвами, каталогом моделей, советами по выбору и эксплуатации автомобилей. Всё, что нужно автолюбителю — в одном месте.
where can i buy ventolin inhalator without insurance
Сайт для женщин https://miymalyuk.com.ua мода, красота, здоровье, отношения, карьера, семья и вдохновение. Актуальные статьи, советы и поддержка для современной женщины каждый день
Главные новости Украины https://novosti24.kyiv.ua и мира — честно, быстро и понятно. События, которые формируют завтрашний день, в одной ленте.
betclic apk
Актуальные новости Украины https://newsportal.kyiv.ua и мира сегодня: главные события, мнения экспертов, интервью, прогнозы. Оставайтесь в курсе с лентой, которая обновляется 24/7.
mines game demo
Finding a Reliable Online Casino with Mobile Gameplay
How to Find a Reliable Online Casino with Mobile Options
In the current market, the selection of a platform for your gaming activities has become more intricate than ever. With countless options at your fingertips, distinguishing between high-quality and dubious offerings is paramount. A strategic approach to assessing different platforms can enhance your experience and ensure both safety and enjoyment.
First, examine the licensing and regulatory frameworks that govern a potential site. Trusted jurisdictions, such as Malta, the UK, and Gibraltar, enforce stringent guidelines that protect players and maintain fair play standards. Transparency in these legal aspects not only establishes credibility but also instills confidence in your choice.
Additionally, consider the variety of games available on the platform. A dynamic selection that includes slots, table games, and live dealer options can cater to different preferences and keep the experience engaging. Pay attention to the software developers behind these games, as reputable companies like Microgaming and NetEnt consistently deliver high-quality products known for their reliability.
Furthermore, assess the mobile compatibility of your preferred choice. A well-optimized platform should offer an intuitive interface, quick loading times, and seamless compatibility across devices. Prioritize establishments that provide applications or responsive websites tailored for mobile users, enhancing your convenience and access to your favorite activities.
Key Features to Look for in Mobile-Friendly Casinos
When assessing platforms for gaming on handheld devices, several attributes are paramount to ensure an enjoyable experience. First, examine the interface; it should be intuitive, allowing for seamless navigation. Look for designs that prioritize user accessibility, making it easy to locate games and features without confusion.
Game Selection plays a significant role. A diverse range of titles, including slots, table games, and live dealer options, broadens entertainment possibilities. Ensure that the favorite games have been optimized for smaller screens, maintaining high-quality graphics and functionality.
The presence of bonus offers is another consideration. Promotions tailored for mobile users, like exclusive bonuses or loyalty rewards, can enhance the playing experience. Check the terms associated with these offers to avoid unexpected restrictions.
Moreover, payment methods should be streamlined for mobile transactions. Look for platforms that support a variety of options, from credit cards to e-wallets, ensuring that deposits and withdrawals are straightforward and secure.
Another vital aspect is customer support. Reliable assistance via live chat, email, or phone is important, especially for users who may require help on the go. A swift response time indicates a commitment to player satisfaction.
Lastly, assess the security measures in place. Platforms should utilize advanced encryption technologies to safeguard personal and financial information, fostering a sense of safety while engaging in gaming activities.
Assessing Casino Reputation and Player Reviews for Trustworthiness
Evaluating the standing of a gambling platform involves investigating various sources and indicators. Start by identifying licenses and certifications that validate the legitimacy of the establishment. Regulated entities typically display these credentials on their websites, ensuring compliance with industry standards.
Moving on to player feedback, online forums and review aggregates can provide real insights. Look for consistent patterns in comments, as a balance of positive and negative reviews can reveal a more nuanced picture. Pay attention to aspects like payout speed, customer service responsiveness, and game variety, which affect user experience.
Check social media presence as well, where players often share real-time experiences. Active engagement from the platform’s support team in response to concerns can indicate reliability. A company’s willingness to address complaints can significantly enhance its credibility.
Analyzing articles or reports from third-party auditing firms adds another layer of trust. Companies that undergo external assessments and publish their findings demonstrate transparency. Look for platforms that share audit results, as this can confirm integrity in their gaming processes.
Finally, consider the community’s overall sentiment. If a large number of players express satisfaction regarding fairness and service, that’s a good sign. Regularly review updated discussions to stay informed, as the reputation of gambling establishments can change rapidly.
Feel free to visit my blog post: https://plinko-pareri.com.ro
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
Наша продукция:
Мишень РёР· драгоценного металла или его сплава кольцевая палладий 50С…4.5 РјРј РџРґ99.9 РўРЈ Выберите уникальные мишени РёР· драгоценных металлов для напыления РѕС‚ Редметсплав.СЂС„. Обеспечивают высокую степень защиты Рё долговечность. Рдеальный выбор для промышленности, строительства Рё производства.
mines game demo
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наша продукция:
Пружина из драгоценных металлов золотая 15х5х0.4 мм ЗлСрМ58.5-8 ТУ Выберите идеальные пружины из золота, серебра или платины, чтобы дополнить ваше украшение. Найдите пружины с изысканным дизайном и прочностью. Они предлагают широкий выбор для того, чтобы подчеркнуть уникальность вашего украшения.
Обучитесь новой профессии, пройдите онлайн курс!
https://unews.pro/news/142107/
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Плоская мишень Cu+Ni+Ti
plinko win
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – идеальный вариант для вас.
поставляемая продукция:
Бронзовое внутреннее стопорное пружинное кольцо 40х1.7 мм БрБНТ1.9Мг ГОСТ 13943-86 Приобретите качественные бронзовые внутренние стопорные кольца по ГОСТ в Редметсплав.рф. Наши колечки отличаются превосходными техническими характеристиками, высоким качеством и надёжностью. Широкий ассортимент и гарантированное соответствие ГОСТ позволят удовлетворить потребности любого заказчика.
Pills information leaflet. What side effects can this medication cause?
subcutaneous naltrexone pellets
Some about medicament. Read information now.
can i buy cheap provera for sale
plinko argent reel avis
РедМетСплав предлагает внушительный каталог отборных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица товара подтверждена требуемыми документами, подтверждающими их качество. Дружелюбная помощь – наша визитная карточка – мы на связи, чтобы разрешать ваши вопросы по мере того как находить ответы под особенности вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
поставляемая продукция:
Проволока кобальтовая РРџ255 Проволока кобальтовая РРџ255 представляет СЃРѕР±РѕР№ высококачественный материал, используемый РІ различных промышленных сферах. РћРЅР° обладает отличными механическими свойствами Рё высокой РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью, что делает ее идеальной для сварки Рё наплавки. Рта проволока рекомендуется для работы СЃ легированными Рё нержавеющими сталями.РљСѓРїРёРІ Проволока кобальтовая РРџ255, РІС‹ получите надежный инструмент для решения сложных задач. Ее использование позволяет обеспечить прочные Рё долговечные соединения, что значительно увеличивает долговечность конечных изделий. Применение данного материала особенно актуально РІ авиационной Рё автомобильной промышленности.
РедМетСплав предлагает обширный выбор качественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их происхождение. Опытная поддержка – наш стандарт – мы на связи, чтобы ответить на ваши вопросы по мере того как находить ответы под требования вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
Наши товары:
РџРѕРєРѕРІРєР° магниевая M13320 – UNS Лист магниевый M13320 – UNS представляет СЃРѕР±РѕР№ высококачественный строительный материал, идеально подходящий для различных промышленных Рё технических применений. Ртот магниевый лист обладает отличной РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью Рё легкостью, что делает его идеальным выбором для тяжелых условий эксплуатации. Рспользуйте его РІ авиации, судостроении Рё машиностроении. Выбирая, РіРґРµ купить Лист магниевый M13320 – UNS, РІС‹ получаете надежный РїСЂРѕРґСѓРєС‚, который соответствует международным стандартам качества. Обеспечьте долгосрочную эксплуатацию Рё эффективность ваших проектов СЃ помощью этого высококлассного материала.
РедМетСплав предлагает обширный выбор качественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до масштабных поставок, мы обеспечиваем быстрое выполнение вашего заказа.
Каждая единица изделия подтверждена всеми необходимыми документами, подтверждающими их происхождение. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы улаживать ваши вопросы а также адаптировать решения под требования вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
поставляемая продукция:
Лист титановый 35БТ – ГОСТ 10994-74 Лента титановая 35БТ – ГОСТ 10994-74 является идеальным материалом для различных промышленных применений. Ртот высококачественный металл обеспечивает отличную РєРѕСЂСЂРѕР·РёРѕРЅРЅСѓСЋ стойкость Рё высокую прочность, что делает его незаменимым РІ авиационной, медицинской Рё химической отраслях. Лента РёР· титана легка РІ обработке Рё долговечна, что повышает ее привлекательность для современного производства. Если РІС‹ хотите купить Лента титановая 35БТ – ГОСТ 10994-74, то РЅРµ упустите возможность получить этот надежный РїСЂРѕРґСѓРєС‚ РїРѕ выгодной цене.
интернет тарифы пермь
domashij-internet-perm002.ru
подключить интернет
buy generic betnovate prices
Gifts and gags for aviation enthusiasts, Aviation Christmas Cards and aircraft photography. Everything funny for pilots from pilots! Check out our new look LINKS FOR aviator.com.in TELEGRAM @happygrannypies
Портал о здоровье и медицине https://pravovakrayina.org.ua узнайте больше о своём организме, симптомах, лечении и профилактике. Удобный поиск, рекомендации врачей, база клиник и аптек.
Онлайн-медицинский портал https://novamed.com.ua справочник болезней, симптомы, анализы, лекарства, консультации специалистов и актуальные новости здравоохранения. Всё о здоровье — в одном месте.
читайте на автомобильном https://proauto.kyiv.ua портале: тест-драйвы, сравнения, автоаналитика, обзоры технологий и новинки автопрома. Только актуальные материалы и честные мнения.
Современный авто портал https://quebradadelospozos.com свежие новости, каталог машин, рейтинг моделей, видеообзоры и полезные статьи. Помощь при покупке, советы по обслуживанию и анализ рынка.
A crosslink is a physical or chemical bond that connects the functional groups of a polymer chain to another one through covalent bonding or supramolecular SEO BACKLINKS, BLACK-LINKS, TRAFFIC BOOST, LINK INDEXING – TELEGRAM @SEO_ANOMALY
Посетите страницу https://www.drive2.ru/o/b/691804470933197117/ и вы сможете ознакомиться с тем как происходит оклейка плёнкой Porsche 911 от профессионалов. Посмотрите как стал выглядеть Porsche 911 Carrera GTS после оклейки. Оклейка Porsche 911 выполнялась пленкой Vega 230 ST – узнайте почему и все ее преимущества в статье. Porsche 911 удивительный автомобиль – вам будет интересно читать!
Drug prescribing information. Effects of Drug Abuse.
cost of cheap azathioprine
Some information about drugs. Get now.
На сайте https://voronlaws.ru/ вы найдете Casino Dragon Money и его новое рабочее зеркало для того, чтобы открыть для себя удивительный мир огромного количества развлечений, щедрых бонусов, а также бесконечных выигрышей. На сайте вас ожидает увлекательный и эксклюзивный контент, который обязательно подарит много приятных и положительных эмоций. Клуб щедр на акции и бонусы, а потому здесь вы найдете все, что понравится и поможет заработать деньги. Рабочее зеркало ежедневно обновляется для вашей безопасности.
game aviator
Идеальные источники бесперебойного питания для дома, получите информацию.
Советы по выбору источников бесперебойного питания, в нашем блоге.
Обзор функций источников бесперебойного питания, получите все ответы.
Рекомендации по выбору источников бесперебойного питания, на нашем сайте.
Все о ИБП, узнайте.
Как не ошибиться при выборе ИБП, узнайте.
Обзор актуальных источников бесперебойного питания, здесь.
Как работает источник бесперебойного питания, на нашем сайте.
Эффективное использование ИБП, получите советы.
Тенденции рынка источников бесперебойного питания, ознакомьтесь.
Правила подключения источника бесперебойного питания, получите информацию.
ИБП для дома и офиса: выбор и рекомендации, с нашим руководством.
Инсайдерские советы по выбору источников бесперебойного питания, здесь.
Рейтинг популярных источников бесперебойного питания, ознакомьтесь.
Как установить источник бесперебойного питания?, на сайте.
Обзор популярнейших источников бесперебойного питания, читайте.
Устранение неисправностей ИБП, в статье.
Рейтинг лучших ИБП для геймеров, ознакомьтесь.
Что учесть при выборе источника бесперебойного питания, читайте.
источники бесперебойного питания купить [url=https://istochniki-bespereboynogo-pitaniya.ru#источники-бесперебойного-питания-купить]https://istochniki-bespereboynogo-pitaniya.ru[/url] .
Ищете Нестероидный противовоспалительный обезболивающий препарат с фенилбутазоном и лидокаином? Посетите сайт https://ambenium.ru/ и узнайте о препарате Амбениум – это единственный нестероидный противовоспалительный препарат, зарегистрированный в России с усиленным обезболивающим эффектом – раствор для внутримышечного введения фенилбутазон и лидокаин. Подробности на сайте.
plinko fake
This tool is designed to very quickly check backlink and referring domain counts for the top 10 billion most-linked to URLs in our database SEO BACKLINKS, CROSS-LINKS, HACKED WP-ADMIN – TELEGRAM @SEO_ANOMALY
Сервисный центр Мобиопт – Ремонт телефонов в Кирове
Visit https://block-snacks.com/ and stay up to date with all the latest developments in the blockchain and cryptocurrency space today and always. We provide complete and up-to-date opinions, reviews, guides, and introduce people from the cryptocurrency world to help the general public understand and successfully use these technologies. We search and publish only relevant and important news in the cryptocurrency world.
Backlinks, or inbound links, are hyperlinks from one website to another. They signal trust, showing that one site values another’s content. Search engines like SEO BACKLINKS, BLACK-LINKS, TRAFFIC BOOST, LINK INDEXING – TELEGRAM @SEO_ANOMALY
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наши товары:
Лист нержавеющий 32x1500x6000 мм AISI 316L Купить Лист нержавеющий гладкий от компании Редметсплав.рф. Листы нержавеющие гладкие высокого качества, прочные и надежные. Различные размеры и толщины. Применение: автомобилестроение, производства оборудования, мебели, медицинское оборудование и другие области.
тарифы интернет и телевидение пермь
domashij-internet-perm003.ru
домашний интернет
Promo Code Use: Promo codes provide additional chances to win but may have wagering requirements and time limitations. Ensure to check the terms and conditions https://judipoker.online/1xbet-promo-code-free-bet-vip-bonus-100-up-to-e130/
Drugs information sheet. Effects of Drug Abuse.
buspirone first day
Actual about pills. Read now.
cost generic cozaar pill
plinko slot
Перевозка негабаритных грузов и доставка по всей территории России и СНГ на сайте https://negabarit-24.ru/ – это профессиональные услуги от нашей компании. Перевозка негабаритных грузов по России и странам СНГ, ЖД перевозки, перевозки манипулятором и международные перевозки. Гибкие варианты оплаты. Соблюдение сроков. Страхование грузов. Подробнее на сайте.
where buy fincar without rx
Современный женский журнал https://reyesmusicandevents.com стиль, уход, карьера, семья, рецепты и саморазвитие. Читайте свежие материалы каждый день — будь в тренде и в гармонии с собой.
Женский онлайн-журнал https://ruforums.net с разнообразными рубриками — от моды и макияжа до материнства и путешествий. Открывайте каждый день с новыми идеями и полезным контентом.
Журнал для женщин https://saralelakarat.com мода, красота, здоровье, психология, семья и вдохновение. Актуальные статьи, советы экспертов и интересные темы для женщин всех возрастов.
служба поддержки мостбет номер телефона [url=https://www.mostbet5008.ru]https://www.mostbet5008.ru[/url] .
Сайт про авто https://rusigra.org обзоры машин, автоновости, тест-драйвы, сравнения моделей, советы водителям и полезные статьи. Всё, что нужно автолюбителям — на одном ресурсе.
На Kraken встречаются покупатели и продавцы со всего мира для взаимовыгодных сделок. На платформе доступны аукционы для поиска редкостей, а также покупки по фиксированной стоимости. Ко всему прочему, [url=https://xn--krakn4-z4a.com]kra link[/url] обеспечивает безопасные покупки и первоклассное обслуживание для вашего комфорта. Откройте выгодный шопинг с [url=https://xn--krakn4-z4a.com]kraken сайт[/url] – вы останетесь довольны.
ссылка на кракен через тор: https://xn--krakn4-z4a.com
plinko bewertung
РедМетСплав предлагает обширный выбор высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица изделия подтверждена требуемыми документами, подтверждающими их соответствие стандартам. Дружелюбная помощь – то, чем мы гордимся – мы на связи, чтобы улаживать ваши вопросы и находить ответы под особенности вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
Наша продукция:
РџРѕРєРѕРІРєР° молибденовая РњР -47Р’Рџ РџРѕРєРѕРІРєР° молибденовая РњР -47Р’Рџ – это высококачественный РїСЂРѕРґСѓРєС‚, который обеспечивает отличные механические свойства Рё устойчивость Рє высоким температурам. Молибденовые РїРѕРєРѕРІРєРё находят широкое применение РІ аэрокосмической, ядерной Рё электронной промышленностях. Ртот материал идеально РїРѕРґС…РѕРґРёС‚ для производства деталей, работающих РІ экстремальных условиях. Приобретая РџРѕРєРѕРІРєСѓ молибденовую РњР -47Р’Рџ, РІС‹ получаете надежность Рё долговечность РІ эксплуатации. РќРµ упустите возможность купить РџРѕРєРѕРІРєР° молибденовая РњР -47Р’Рџ Рё обеспечьте успешное выполнение ваших проектов!
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наша продукция:
Высокочистые плитки алюминия
plinko balls
Discover thousands of offerings — from free courses to full degrees — delivered by world-class partners like Harvard, Google, Amazon and more SEO BACKLINKS, CROSS-LINKS, TRAFFIC BOOST, LINK INDEXING – TELEGRAM @SEO_ANOMALY
I wish online casinos were more social! plinko
plinko game
аккаунты с донатом https://marketplace-akkauntov-top.ru
This exclusive 1xBet promo code sets new players up for success, whether betting on a thrilling Champions League match or spinning vibrant slots in the casino https://friendtalk.mn.co/posts/83234320?utm_source=manual
Детский туроператор Флагман организует школьные экскурсии и туры для детей по Санкт-Петербургу и Северо-Западу. Поездки с Флагманом всегда превращаются в увлекательные приключения. Собственный автопарк, профессиональные водители и контроль безопасности в пути гарантируют комфортные путешествия для детей. Профессиональные экскурсоводы адаптируют маршруты под детскую аудиторию, делая их познавательными и интересными. Подробнее на официальном сайте https://flagmanspb.ru/
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наши товары:
Керамическая мишень для распыления оксида олова – 4N, Плоская форма
The convenient https://www.booking-zabljak.com service will help you find the perfect hotel for car travelers and active holiday lovers. A wide range of accommodation: from cozy guest houses to modern hotels with parking, Wi-Fi and breakfast. Book in advance and relax in comfort in the heart of Montenegro!
подключить интернет в ростове в квартире
domashij-internet-rostov001.ru
провайдеры домашнего интернета ростов
Chanel fake designer bags
Онлайн-журнал для женщин https://zhenskiy.kyiv.ua которые ценят себя, любят жить ярко и стремятся к гармонии. Будь в курсе моды, заботься о себе и черпай вдохновение каждый день.
Looking to transform your style with real dreadlocks? Check out the best range of [real dreadlock extensions|handmade dreadlocks|dreadlock extensions|dreads real hair|human hair dreadlock extensions|dread natural] at the site –
handmade dreadlocks. Crafted from natural human hair, these dreadlocks are perfect for a bold look. Whether you’re after temporary installs, these extensions blend with all hair types.
Stand out in the crowd with premium-quality dreadlocks today. Fast shipping available across the USA!
Портал о машинах https://shpik.info от новостей и технологий до экспертных статей и автомобильной аналитики. Узнайте всё о текущих трендах на рынке и новинках автопрома.
Портал о машинах https://xiwet.com от новостей и технологий до экспертных статей и автомобильной аналитики. Узнайте всё о текущих трендах на рынке и новинках автопрома.
The online rush kicks—stay cool! tiger fortune
Кракен делает онлайн-шопинг простым с помощью невероятного ассортимента товаров и услуг. Независимо от того, работаете ли вы в офисе, учитесь или управляете домом, Кракен имеет что-то для каждого. От предметов для дома до оборудования для тренировок — вы найдете все, что нужно, с легкостью. Надёжная доставка и качественный сервис усиливают преимущества [url=https://xn--krakn4-l4a.com]сайт kraken at[/url]. Начните экономить время и деньги, выбрав [url=https://xn--krakn4-l4a.com]kra27 at[/url] для всех своих онлайн-покупок.
кракен: https://xn--krakn4-l4a.com
buying finasteride without a prescription
Medicine prescribing information. Cautions.
buy generic loperamide online
Everything about medication. Get information here.
aviator
Bottega Veneta bag replica
mines casino game
Gucci replica designer bags
dragon money casino зеркало [url=http://dragon-money42.com]dragon money casino зеркало[/url] .
dragon casino [url=www.dragon-money40.com/]dragon casino[/url] .
Louis Vuitton bag replica
Доставили в санаторий – бабушка счастлива!
букеты томск
Chanel fake designer bags
order generic asacol price
One of the key benefits of time-based rewards is the sense of progression they provide https://bresdel.com/blogs/969094/Crazy-Time-Betting-Guide-Tips-and-Strategies-for-Smarter-Gameplay
predictor aviator
Online casinos should teach rules better—too hard! plinko
Bottega Veneta replica designer bags
Bottega Veneta replica designer bags
1xCasino welcome bonus is a package active across your first four deposits. New customers will claim up to €2205/$2420 1xcasino promo kodu 2025
Боль в суставах может серьезно ухудшить качество жизни. Артроз, подагра и воспаление суставов — частые причины дискомфорта. Эффективное лечение возможно! На сайте https://lechsustavy.ru/ вы найдете натуральные средства для лечения суставов в домашних условиях. Забудьте про боль без операции — попробуйте проверенные препараты, которые помогут восстановить хрящи, снять воспаление и вернуть подвижность. Начните лечить суставы сегодня!
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – наилучшее решение для вашего бизнеса.
Наши товары:
Медная двухраструбная редукционная переходная муфта РїРѕРґ пайку 25С…22 РјРј твердая пайка Рњ2Рњ ГОСТ 32590-2013 Выберите высококачественные медные двухраструбные редукционные муфты РїРѕРґ пайку РѕС‚ Редметсплав для надежного соединения медных труб различных диаметров. РЁРёСЂРѕРєРёР№ выбор размеров, эффективная установка Рё долговечность гарантированы. Рдеальное решение для систем отопления Рё водоснабжения.
tesisat kaçağı bulma cihazı Düzenli kontroller, sürprizleri engeller. https://hygiene-premium.com/?p=3262
Prada fake designer bags
Prada fake designer bags
Журнал про животных https://zoobonus.com.ua интересные статьи о питомцах, дикой природе, уходе, воспитании и поведении. Всё для тех, кто любит животных и хочет узнать о них больше.
Medication information for patients. Long-Term Effects.
how long does it take for promethazine and codeine to get out of your system
Best news about medicine. Read information here.
Полный гид по недвижимости https://all2realt.com.ua как выбрать, купить, продать или арендовать квартиру, дом или коммерческий объект. Обзоры, цены, тенденции рынка и юридические тонкости.
Fendi replica designer bags
Жителям курорта предлагают Зеленоградск экскурсии на Куршскую косу из Зеленоградска с выездом ранним утром.
Efizika.ru – сайт, где вы увидите виртуальные лабораторные работы по физике. Они помогают ученикам освоить физические законы и явления, а также вырабатывают навыки проведения опытов и анализа данных. Вы можете эксперимент провести, усвоить от параметров цепи работу электрического тока и зависимость мощности. Ищете лабораторная работа по физике? Efizika.ru – здесь есть более подробная информация, ознакомьтесь с ней. При возникновении вопросов касаемо осуществления работы, либо интерпретации итогов, обратитесь к учебнику по физике. Желаем вам в эксперименте удачи!
Arenda24.by – интернет-платформа для опубликования по аренде строительной техники и оборудования объявлений. На нашем ресурсе вы сможете работать комфортно, ведь его создала истинная команда профессионалов. Ищете экскаватор беларусь аренда? Arenda24.by – портал, где вы найдете по аренде техники и любых инструментов объявления, и сможете организовать грузоперевозки. У нас есть объявления любых типов категорий. Разместите объявление уже сейчас, и оно в сжатые сроки своих клиентов найдет. С нами вы доступ к качественной и популярной платформе получите.
На сайте https://rozatoday.ru/ представлено огромное количество ярких, запоминающихся композиций, которые созданы из свежих цветов: ослепительных роз, волнующих пионов, трепетных тюльпанов, строгих гвоздик. В компании работают только знающие, компетентные флористы, которые создают изысканные композиции в соответствии с предпочтениями клиента. В обязательном порядке учитываются и актуальные мировые тенденции. Можно приобрести как небольшой букетик, который подходит для романтического свидания, так и внушительных размеров для грандиозного праздника.
домашний интернет ростов
domashij-internet-rostov002.ru
подключить интернет в квартиру ростов
DIOR replica designer bags
melbet kg [url=http://melbet1003.ru/]http://melbet1003.ru/[/url] .
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Обладая обширной линейкой редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наши товары:
Нанокристаллический тигель из высокочистого нитрида бора
РедМетСплав предлагает широкий ассортимент качественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их происхождение. Дружелюбная помощь – наш стандарт – мы на связи, чтобы улаживать ваши вопросы по мере того как предоставлять решения под требования вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в гибкости нашего предложения
Наша продукция:
Пруток магниевый G-Z5Zr – AFNOR NF A57-704 Проволока магниевая G-Z5Zr – AFNOR NF A57-704 представляет СЃРѕР±РѕР№ высококачественный материал, полученный РїРѕ современным технологиям. Рта проволока обладает отличными механическими свойствами Рё РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью, что делает ее идеальным выбором для различных промышленных применений. РЎ помощью магниевой проволоки РІС‹ сможете значительно улучшить качество СЃРІРѕРёС… изделий, снизить вес Рё повысить долговечность. Если РІС‹ хотите купить Проволока магниевая G-Z5Zr – AFNOR NF A57-704, РІС‹ выбираете надежность Рё эффективность. Откройте новые горизонты РІ вашем производстве СЃ этой уникальной проволокой.
Превосходит защитное стекло на телефон – Гидрогелевая пленка в Кирове
cheap astelin without prescription
Drugs information leaflet. What side effects can this medication cause?
buying kamagra no prescription
Actual what you want to know about medicines. Read information now.
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
Наши товары:
Мишень РёР· драгоценного металла или его сплава кольцевая золото 150С…1 РјРј ЗлСрМ58.5-20 РўРЈ Выберите уникальные мишени РёР· драгоценных металлов для напыления РѕС‚ Редметсплав.СЂС„. Обеспечивают высокую степень защиты Рё долговечность. Рдеальный выбор для промышленности, строительства Рё производства.
dragonmoney официальный сайт [url=https://dragon-money42.com]https://dragon-money42.com[/url] .
Online casinos are great for practice—nice! plinko
fake Louis Vuitton bag
the aviator game
Drugs information for patients. What side effects can this medication cause?
buy cheap aricept without a prescription
Everything information about medicament. Read information now.
РедМетСплав предлагает внушительный каталог высококачественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица изделия подтверждена требуемыми документами, подтверждающими их качество. Опытная поддержка – то, чем мы гордимся – мы на связи, чтобы улаживать ваши вопросы и находить ответы под специфику вашего бизнеса.
Доверьте ваш запрос специалистам РедМетСплав и убедитесь в гибкости нашего предложения
Наша продукция:
РљСЂСѓРі висмутовый TBC 19 – PN H-87203 РљСЂСѓРі висмутовый TBC 19 – PN H-87203 – это высококачественный инструмент, предназначенный для широкого спектра промышленных задач. РћРЅ отличается высокой прочностью Рё долговечностью, что делает его идеальным выбором для применения РІ условиях повышенных требований Рє материалам. РЎ помощью этого РєСЂСѓРіР° РІС‹ сможете значительно повысить эффективность СЃРІРѕРёС… процессов. РќРµ упустите возможность купить РљСЂСѓРі висмутовый TBC 19 – PN H-87203 Рё обеспечить надежность ваших работ. Его уникальные свойства позволят вам добиться лучших результатов. Сделайте правильный выбор Рё инвестируйте РІ качество.
Celine fake designer bags
Louis Vuitton replica designer bags
mostbet скачать [url=https://mostbet5008.ru]mostbet скачать[/url] .
can you buy cheap epivir prices
Fendi bag replica
домашний интернет тарифы
domashij-internet-rostov003.ru
домашний интернет ростов
The online thrill bites—stay firm! dragon tiger
where to get cheap astelin without insurance
Medicine information leaflet. Drug Class.
how can i get cheap macrobid without a prescription
Actual about medication. Read information now.
Drug information for patients. Brand names.
cost generic xenical prices
Best trends of drugs. Get information now.
plinko gioco
Prada fake designer bags
Система менеджмента управления качеством содержит критерии менеджмента и стандарты, по которым проводится последующая сертификация предприятия. Чтобы получить сертификат СМК, нужно обратиться в аккредитованный сертификационный центр. Сертификация систем менеджмента качества является документальным подтверждением того, что система менеджмента сертифицированной компании соответствует требованиям того или иного стандарта. Подробнее: https://ok.ru/group/70000034956977
Serialexpress приобрести увлекательные сериалы на dvd предлагает. Здесь можно найти уникальные диски. Вы от покупки будете в восторге, потому как отменное качество сериалов. Товары доступны по приемлемой стоимости и отлично упакованы. Ищете магазин сериалов в Томске? Serialexpress.ru – ресурс, где представлена информация об оплате, рекомендуем вам с ней ознакомиться. Мы накопительную систему скидок предлагаем. Постоянным клиентом выгодно быть. Гарантируем оперативную доставку. При возникновении вопросов, на электронную почту нам пишите. Рады вам всегда!
Gucci bag replica
dragon money зеркало сегодня [url=https://dragon-money40.com]https://dragon-money40.com[/url] .
dragon money официальный сайт [url=http://dragon-money42.com/]http://dragon-money42.com/[/url] .
red on bet casino
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – идеальный вариант для вас.
Наши товары:
Лента РёР· прецизионных сплавов для СѓРїСЂСѓРіРёС… элементов 0.3×62 РјРј РЎРџ22 ГОСТ 14117-85 Купите ленту РёР· прецизионных сплавов для СѓРїСЂСѓРіРёС… элементов РїРѕ выгодной цене РЅР° Редметсплав.СЂС„. Высокая прочность, устойчивость Рє РєРѕСЂСЂРѕР·РёРё Рё отличная эластичность делают этот материал идеальным для различных отраслей. Предлагаем широкий ассортимент продукции Рё РїРѕРґСЂРѕР±РЅСѓСЋ информацию для правильного выбора.
fortune tiger bonus gratis sem deposito
Bottega Veneta bag replica
fake Chanel bag
aviator game download
fake Louis Vuitton bag
Louis Vuitton bag replica
plinko demo
The visuals online are epic—wow factor! plinko
РедМетСплав предлагает обширный выбор высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы обеспечиваем оперативное исполнение вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их качество. Опытная поддержка – наш стандарт – мы на связи, чтобы разрешать ваши вопросы а также находить ответы под требования вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наша продукция:
Вольфрам Р’Р -27Р’Рџ Вольфрам Р’Р -27Р’Рџ – это высококачественный материал, который широко применяется РІ различных отраслях, включая электронику Рё машиностроение. Благодаря СЃРІРѕРёРј уникальным свойствам, таким как высокая прочность Рё устойчивость Рє высоким температурам, Вольфрам Р’Р -27Р’Рџ становится идеальным выбором для профессионалов.Если РІС‹ хотите купить Вольфрам Р’Р -27Р’Рџ, то можете быть уверены РІ его надежности Рё долговечности. Ртот РїСЂРѕРґСѓРєС‚ прекрасно РїРѕРґС…РѕРґРёС‚ для разнообразных задач, начиная РѕС‚ сварки Рё заканчивая производства сложных деталей. РќРµ упустите возможность улучшить СЃРІРѕРё производственные процессы СЃ помощью Вольфрам Р’Р -27Р’Рџ!
mostbet kg скачать [url=https://mostbet5009.ru]mostbet kg скачать[/url] .
скачать mostbet на телефон [url=https://mostbet5010.ru]https://mostbet5010.ru[/url] .
melbet сайт [url=https://melbet1003.ru/]melbet сайт[/url] .
Drug information. What side effects can this medication cause?
how can i get generic motrin pill
Best about drugs. Get now.
DIOR replica designer bags
Посетите B2BOX https://b2box.ru/ – это уникальное комплексное решение для создания упаковки всех видов и направлений. Мы делаем упаковку, которая продает! Упаковочные решения для ритейла, создание индивидуальной и SRP упаковки, услуги дизайнерского бюро, производство транспортной упаковки, собственное плоттерное производство, все виды печати – это и многое другое вы найдете, посетив наш сайт.
Gucci replica designer bags
интернет провайдеры омск
domashij-internet-volgograd001.ru
недорогой интернет омск
Drug information sheet. Drug Class.
rosuvastatin familial hypercholesterolemia
All about medicines. Read information now.
free plinko
mostbet.kg [url=https://mostbet7004.ru/]mostbet.kg[/url] .
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым текущие трудности превращаются в завтрашние успехи.
Наша продукция:
Плоская мишень из фтористого магния
mines casino game
мост бет [url=http://mostbet7004.ru]http://mostbet7004.ru[/url] .
[url=https://chempion-casino-official.ru]chempion-casino-official.ru[/url]
На сайте, посвященном обзору официального сайта Казино Чемпион, вы найдете всестороннюю информацию об этой популярной игровой платформе. Здесь детально разобраны ключевые аспекты казино, начиная от интерфейса и удобства навигации, и заканчивая разнообразием игрового ассортимента и щедростью бонусной программы. В обзоре представлена информация о лицензировании казино, гарантиях безопасности, скорости выплат и качестве работы службы поддержки. Благодаря этому обзору, вы сможете составить полное представление о Казино Чемпион, оценить его преимущества и недостатки, и принять взвешенное решение, стоит ли здесь регистрироваться и играть на реальные деньги. Кроме того, на сайте представлены отзывы реальных игроков, которые помогут вам получить более объективную картину о работе казино и избежать возможных разочарований.
chempion-casino-official.ru
plinko argent reel
мелбет kg [url=http://melbet1003.ru]http://melbet1003.ru[/url] .
скачать мостбет официальный сайт [url=http://mostbet7004.ru/]http://mostbet7004.ru/[/url] .
Bottega Veneta fake designer bags
Online casinos should give more free play credits! Fishin Frenzy
На сайте http://oknaksa.ru закажите расчет технического задания, чтобы воспользоваться такой важной, полезной услугой, как остекление. На монтажные работы, а также сами конструкции предоставляются гарантии, вы сможете воспользоваться бесплатным обслуживанием. А все работы выполняются точно по ГОСТу, в соответствии с требованиями. Предоставляются исчерпывающие и содержательные консультации. Вам будет предложено несколько вариантов решения проблемы. Компания располагает огромным количеством готовых проектов.
Тест: стоит ли вам делать ботокс?, читайте.
ботокс фулфейс до и после [url=botox.life#ботокс-фулфейс-до-и-после]botox.life[/url] .
mostbet chrono [url=http://mostbet7004.ru]http://mostbet7004.ru[/url] .
Medicine information. Effects of Drug Abuse.
get benicar pill
All about medicines. Get information now.
mostber [url=https://mostbet5008.ru]https://mostbet5008.ru[/url] .
play plinko
game sugar rush
DIOR bag replica
DIOR replica designer bags
Ваш идеальный день Куршская коса индивидуальные экскурсии из Светлогорска с персональным гидом.
The best online slots rise of olympus in one place: classics, new releases, jackpots and themed machines. Play without registration, test the demo or make real bets with bonuses.
fake Louis Vuitton bag
Профессиональное агентство рекламное агентство Витрувий: разработка рекламы, брендинг, digital-маркетинг, наружка и SMM. Комплексное продвижение для бизнеса любого масштаба.
By incorporating mass page backlinks into your SEO strategy you can enhance your chances of achieving better search engine rankings BLACK SEO LINKS, BACKLINKS, SOFTWARE FOR MASS BACKLINKING – TELEGRAM @SEO_LINKK
Discover thousands of offerings — from free courses to full degrees — delivered by world-class partners like Harvard, Google, Amazon and more SEO BACKLINKS, CROSS-LINKS, HACKED WP-ADMIN – TELEGRAM @SEO_ANOMALY
Prada fake designer bags
интернет провайдер омск
domashij-internet-volgograd002.ru
провайдеры интернета в волгограде
Meds information for patients. Generic Name.
duloxetine msn patent
Best news about medicines. Read information here.
[url=https://chempion-casino-official.ru]chempion-casino-official.ru[/url]
На сайте, посвященном обзору официального сайта Казино Чемпион, вы найдете всестороннюю информацию об этой популярной игровой платформе. Здесь детально разобраны ключевые аспекты казино, начиная от интерфейса и удобства навигации, и заканчивая разнообразием игрового ассортимента и щедростью бонусной программы. В обзоре представлена информация о лицензировании казино, гарантиях безопасности, скорости выплат и качестве работы службы поддержки. Благодаря этому обзору, вы сможете составить полное представление о Казино Чемпион, оценить его преимущества и недостатки, и принять взвешенное решение, стоит ли здесь регистрироваться и играть на реальные деньги. Кроме того, на сайте представлены отзывы реальных игроков, которые помогут вам получить более объективную картину о работе казино и избежать возможных разочарований.
chempion-casino-official.ru
I don’t get online flak—it’s good! juego mines
fake Louis Vuitton bag
Drug information. Brand names.
cost of diflucan for sale
Best information about drug. Read here.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние возможности.
Наша продукция:
Титановая мишень
мостбет войти [url=www.mostbet5008.ru]www.mostbet5008.ru[/url] .
Teşvikiye su kaçak tespiti Beklentilerimizi Karşıladı: Başta tereddüt ettik ama gerçekten beklentilerimizin üzerinde bir hizmet aldık. https://last2u.com/?p=22239
проведение оценки профессиональных рисков в Москве [url=www.ocenka-profriskov495.ru/]www.ocenka-profriskov495.ru/[/url] .
Ellcon.ru создан для вашего комфорта, чтобы вы экономили время на поездки, и смогли, отыскать нужное не выходя, из собственного дома. у нас вы можете купить провода и аксессуары, электроустановочные изделия, климатические системы, кабели, высоковольтное оборудование по доступным ценам. Ищете заглушка кабель канала серая? Ellcon.ru – ресурс, выбрав его для приобретения, вы время, нервы и денежные средства существенно сэкономите. Доставка товаров исключительно при 100% предоплате заказа выполняется. Стремимся к лучшему, чтобы ежедневно радовать клиентов. Удачных вам покупок!
Fendi bag replica
Celine fake designer bags
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с высоким уровнем сервиса.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
поставляемая продукция:
Оловянный пруток круглый 20 РјРј РћР’Р§-000 ГОСТ 860-75 Купить оловянные прутки РїРѕ оптимальной цене. РЁРёСЂРѕРєРёР№ выбор, высокое качество Рё универсальное применение. Прочность, устойчивость Рє РєРѕСЂСЂРѕР·РёРё Рё легкость обработки. Рдеальное решение для промышленного производства, С…РѕР±Р±Рё Рё ремесел. Специальные условия для оптовых покупателей. Бесплатная консультация Рё поддержка экспертов Рё технической команды.
Louis Vuitton bag replica
fake Bottega Veneta bag
где согласовать перепланировку квартиры [url=www.soglasovanie-pereplanirovki-kvartiry15.ru]www.soglasovanie-pereplanirovki-kvartiry15.ru[/url] .
Online blackjack flows—good mix! fortune ox
mines game download
Medication information. Effects of Drug Abuse.
can i get aldactone without prescription
Best about medicines. Read information now.
Below is an outline of how the SOFTSWISS Casino Platform can help iGaming operators and casino websites with SEO and organic user acquisition SEO BACKLINKS, CROSS-LINKS, HACKED WP-ADMIN – TELEGRAM @SEO_ANOMALY
fake Fendi bag
fake Louis Vuitton bag
fake Gucci bag
РедМетСплав предлагает обширный выбор отборных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица продукции подтверждена соответствующими документами, подтверждающими их качество. Дружелюбная помощь – то, чем мы гордимся – мы на связи, чтобы разрешать ваши вопросы а также адаптировать решения под особенности вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
Лента магниевая MAG 6 – BS 2970 РљСЂСѓРі магниевый MAG 6 – BS 2970 предназначен для высококачественной обработки различных материалов. Рто уникальный инструмент разработан для эффективного резания Рё шлифования, обеспечивая отличные результаты. Благодаря современному производству Рё качественным материалам, РєСЂСѓРі демонстрирует стабильную производительность Рё долговечность РІ эксплуатации. Если РІС‹ ищете надежное решение для СЃРІРѕРёС… задач, обязательно купите РљСЂСѓРі магниевый MAG 6 – BS 2970. РћРЅ идеально РїРѕРґС…РѕРґРёС‚ для профессионалов Рё любителей, стремящихся Рє совершенству РІ работе. РќРµ упустите возможность обеспечить себя высокоэффективным инструментом!
Medication prescribing information. Short-Term Effects.
verapamil otc
Everything trends of drugs. Get now.
домашний интернет подключить омск
domashij-internet-volgograd003.ru
подключить домашний интернет в волгограде
Компания Аллегро https://spb.tk-allegro.ru/ – это аренда автобуса в Санкт-Петербурге. У нас от 8 до 55 мест свой автопарк автобусов. Водители с огромным опытом. Вы можете заказать автобус для различных мероприятий – для школьников, на свадьбу, выпускной, на экскурсию. Заходите на портал, узнайте больше о стоимости аренды, о наших услугах, или же воспользуйтесь для расчета стоимости калькулятором на портале.
Prada bag replica
Купить натуральный камень http://proff-kamen.ru/kamen-krasnodar в Краснодаре и Краснодарском крае по оптовой цене. Каталог с расценками, размеры и монтаж дагестанской плитки из ракушечника, песчаника, травертина, известняка для отделки фасада и цоколя в Краснодаре от производителя.
Посетите сайт https://artradol.com/ и вы узнаете все про Артрадол – это препарат для лечения суставов от производителя. Узнайте все преимущества нестероидного противовоспалительного препарата для лечения суставов. Помогает бороться с основными заболеваниями суставов: артритом, остеохондрозом и остеоартрозом. Подробности, инструкция применения и где купить можно узнать на сайте.
оценка профессиональных рисков в организации [url=http://ocenka-profriskov495.ru]http://ocenka-profriskov495.ru[/url] .
plinko france
переезд с квартиры на квартиру грузчики на дом
заказать переезд квартиры с грузчиками профессиональные грузчики
услуги грузчиков заказать дешево заказать грузчиков
mines gratis
Chanel replica designer bags
На сайте https://pilmi.ru вы найдете огромное количество фильмов самых разных жанров, включая комедии, мелодрамы, драмы, романтические. Для того чтобы подыскать наиболее подходящий вариант, нужно воспользоваться специальным каталогом. Вашему вниманию представлены и ожидаемые любопытные новинки этого года, которые произведут эффект. Все это можно просматривать в любое время и на различных устройствах. Прямо сейчас воспользуйтесь возможностью посмотреть азиатское кино. Используйте поиск для облегчения выбора.
Online casinos feel odd—loss vibe! book of ra
Medicine information sheet. Long-Term Effects.
where to get cheap inderal price
All trends of medicament. Get information here.
aviator game app
Косметолог в Санкт-Петербурге – Перманентный макияж
Shark Bounty : Slot Online Gacor dengan Fitur Menarik dan RTP Tinggi
This guide explores the core SEO strategies needed to increase visibility for your gambling app, attract more users, and improve your rankings BLACK SEO LINKS, BACKLINKS, MASS BACKLINKING – TELEGRAM @SEO_LINKK
fake Chanel bag
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наши товары:
Нержавеющая прямоугольная РїРѕРєРѕРІРєР° 210С…250 РјРј Р—Р68 Высококачественная нержавеющая прямоугольная РїРѕРєРѕРІРєР° 210С…250 РјРј Р—Р68 РЅР° Редметсплав.СЂС„. Прочная Рё долговечная конструкция. Рдеально РїРѕРґС…РѕРґРёС‚ для различных отраслей промышленности. Купите сейчас!
This article will tell you in more detail how to register with 1xBet with a promotional code https://faceout.mn.co/posts/83271572?utm_source=manual
порядок согласования перепланировки квартиры [url=https://soglasovanie-pereplanirovki-kvartiry15.ru/]порядок согласования перепланировки квартиры[/url] .
Купить диплом университета по невысокой цене вы сможете, обращаясь к проверенной специализированной фирме. Купить документ ВУЗа можно у нас в столице. [url=http://forum.artinvestment.ru/member.phpu=1807451/]forum.artinvestment.ru/member.phpu=1807451[/url]
Gucci bag replica
мостбет кг [url=https://mostbet5009.ru/]мостбет кг[/url] .
Посетите https://igormylnikovchannel.ru/poezdki-v-taksi-s-det-mi/ и вы найдете полезную информацию для начинающих таксистов и опытных водителей на тему поездок в такси с детьми, начиная от безопасности поездок и ответственности и правилах перевозки детей, до разбора вопросов почему таксисты отказываются от поездок с детьми и многое другое. Подробнее на странице.
Medicine information. Long-Term Effects.
buying prednisolone price
Some information about medicines. Get information here.
Купить диплом о высшем образовании. Покупка документа о высшем образовании через надежную компанию дарит ряд достоинств для покупателя. Это решение помогает сберечь время и существенные финансовые средства. [url=http://amazonadvt.com/profile/vernturriff150/]amazonadvt.com/profile/vernturriff150[/url]
Купить диплом под заказ в столице можно используя официальный портал компании. [url=http://tunmun.com/read-blog/24_kupit-diplom-ob-obrazovanii-s-reestrom.html/]tunmun.com/read-blog/24_kupit-diplom-ob-obrazovanii-s-reestrom.html[/url]
подключить интернет в квартиру воронеж
domashij-internet-voronezh001.ru
подключить проводной интернет воронеж
Купить диплом института по доступной цене можно, обратившись к надежной специализированной фирме. Заказать документ ВУЗа вы можете в нашей компании в столице. [url=http://diplomk-vo-vladivostoke.ru/kupit-diplom-zaregistrirovannij-v-reestre-8/]diplomk-vo-vladivostoke.ru/kupit-diplom-zaregistrirovannij-v-reestre-8[/url]
Gucci bag replica
РедМетСплав предлагает внушительный каталог отборных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица продукции подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Дружелюбная помощь – то, чем мы гордимся – мы на связи, чтобы разрешать ваши вопросы по мере того как предоставлять решения под требования вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
Наши товары:
РџРѕРєРѕРІРєР° магниевая MgAl3Zn – DIN 1729 Part 1 Лист магниевый MgAl3Zn – DIN 1729 Part 1 – это высококачественный материал, обладающий выдающимися механическими свойствами Рё устойчивостью Рє РєРѕСЂСЂРѕР·РёРё. Ртот магниевый сплав идеально РїРѕРґС…РѕРґРёС‚ для использования РІ различных отраслях, включая автомобилестроение Рё aerospace. Благодаря легкости Рё прочности, лист становится предпочтительным выбором для конструкционных элементов. Покупая Лист магниевый MgAl3Zn – DIN 1729 Part 1, РІС‹ обеспечиваете СЃРІРѕР№ проект надежным материалом. Заказывайте Сѓ нас Рё получите конкурентоспособные цены Рё профессиональную поддержку. РќРµ упустите возможность улучшить качество своей продукции!
mel bet сайт [url=https://melbet1003.ru/]https://melbet1003.ru/[/url] .
Приобрести диплом о высшем образовании!
Мы готовы предложить документы ВУЗов, которые расположены в любом регионе РФ.
[url=http://diplomk-vo-vladivostoke.ru/kupit-diplom-s-reestrom-v-moskve-bistro-i-nadezhno/]diplomk-vo-vladivostoke.ru/kupit-diplom-s-reestrom-v-moskve-bistro-i-nadezhno/[/url]
Мы изготавливаем дипломы любой профессии по приятным ценам. Мы можем предложить документы техникумов, расположенных в любом регионе Российской Федерации. Документы выпускаются на бумаге самого высокого качества. Это позволяет делать настоящие дипломы, не отличимые от оригиналов. [url=http://slliver.getbb.ru/viewtopic.phpf=62&t=3069/]slliver.getbb.ru/viewtopic.phpf=62&t=3069[/url]
Bottega Veneta bag replica
The live games online are so smooth—impressive! sugar rush
plinko italia
Medication information. Cautions.
can i buy proscar
All about medicament. Read now.
лед экраны для рекламы https://svetodiodnye-led-ekrany.ru/
настенная видеостена videostena-1.ru
Это довольно короткий рассказ о девушке с необычной профессией. Марго – профессиональный киллер Кримінальна робота
Bottega Veneta fake designer bags
code bonus plinko
оценка профессиональных рисков [url=ocenka-profriskov495.ru]оценка профессиональных рисков[/url] .
Букет с сухоцветами – стоит уже 2 месяца!
купить цветы томск
Roller garage doors are easy to use, especially when you have a garage remote door system https://concretesubmarine.activeboard.com/t71832754/1xbet/?page=last#lastPostAnchor
mosbet [url=https://www.mostbet5010.ru]https://www.mostbet5010.ru[/url] .
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
С широким ассортиментом продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние возможности.
Наша продукция:
Плоская мишень Fe+Mn
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
С широким ассортиментом продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Металлическая мишень для распыления
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наши товары:
Гранулы оксида алюминия 4N
mines gambling game
DIOR replica designer bags
Online casinos should free—try it! plinko
Medicine information. What side effects?
naproxen interaction with lisinopril
Some what you want to know about drug. Get information now.
Chanel bag replica
Medicament information for patients. What side effects?
get cheap proscar online
Best news about medication. Read now.
экспертиза перепланировки квартиры [url=https://soglasovanie-pereplanirovki-kvartiry15.ru/]экспертиза перепланировки квартиры[/url] .
домашний интернет тарифы воронеж
domashij-internet-voronezh002.ru
подключить проводной интернет воронеж
мостбет кыргызстан [url=https://mostbet5010.ru]https://mostbet5010.ru[/url] .
Gucci fake designer bags
Gucci fake designer bags
fake Louis Vuitton bag
[url=https://chestnye-casino.ru]chestnye-casino.ru[/url]
На сайте, посвященном обзорам онлайн казино, вы найдете независимую оценку и рейтинг самых надежных игровых платформ. Здесь представлены только проверенные и лицензированные казино, прошедшие тщательную проверку экспертов. Вы узнаете о преимуществах и недостатках каждого заведения, получите информацию о бонусах, игровых автоматах, методах оплаты и качестве службы поддержки. Сайт поможет вам сделать осознанный выбор, чтобы играть в слоты на деньги в безопасной и честной среде, минимизируя риски и получая максимум удовольствия от игры. Кроме того, ресурс содержит полезные советы и стратегии, которые помогут вам повысить свои шансы на выигрыш и сделать процесс игры еще более увлекательным. Регулярное обновление обзоров и добавление новых казино позволит вам всегда быть в курсе актуальных предложений и выбрать именно то казино, которое подходит вашим потребностям и предпочтениям.
chestnye-casino.ru
The popular service ai dream girlfriend offers to create an AI girl who understands you, can hold a conversation and even flirt. Ideal for those who are looking for emotions in a digital format.
I’m shy on online—shaky feel! pin up
Посетите сайт https://bzdostup.ru/ и вы сможете купить бетон и раствор с доставкой по Краснодару и краю по выгодной цене от производителя. Ознакомьтесь на сайте с ценами и условиями доставки. Вы также можете забрать бетон самовывозом. Бетонный завод Доступ – это строгое соблюдение ГОСТа и круглосуточный режим работы.
автомобиль танк 300 [url=https://tankautospb.ru/models/tank-300/]https://tankautospb.ru/models/tank-300/[/url] .
melhor plataforma para jogar fortune tiger
Мы предлагаем выгодно и быстро приобрести диплом, который выполняется на бланке ГОЗНАКа и заверен мокрыми печатями, водяными знаками, подписями должностных лиц. Документ пройдет лубую проверку, даже при помощи специально предназначенного оборудования. [url=http://pgonline.ru/forums/index.php?topic=147132.new#new/]pgonline.ru/forums/index.php?topic=147132.new#new[/url]
Medication prescribing information. Generic Name.
where to buy cheap minocycline without prescription
Some what you want to know about medicines. Get information now.
Купить диплом любого ВУЗа. Приобретение официального диплома через надежную компанию дарит много плюсов. Такое решение позволяет сэкономить как дорогое время, так и серьезные деньги. [url=http://yaoisennari.ekafe.ru/posting.php?mode=post&f=169&sid=f828ff3ade5592e9014b998bee76cb1b/]yaoisennari.ekafe.ru/posting.php?mode=post&f=169&sid=f828ff3ade5592e9014b998bee76cb1b[/url]
Заказать диплом на заказ в Москве вы имеете возможность используя сайт компании. [url=http://datemethisnight.copiny.com/question/details/id/1084446/]datemethisnight.copiny.com/question/details/id/1084446[/url]
Мы изготавливаем дипломы любых профессий по приятным ценам. Всегда стараемся поддерживать для заказчиков адекватную ценовую политику. Для нас важно, чтобы дипломы были доступны для большого количества граждан.
Покупка документа, который подтверждает обучение в университете, – это грамотное решение. Купить диплом любого университета: [url=http://diploms-vuza.com/diplom-stomatologa-kupit/]diploms-vuza.com/diplom-stomatologa-kupit/[/url]
Celine bag replica
Medicine information for patients. Long-Term Effects.
get cheap loperamide pill
Actual trends of drugs. Read now.
Ремонт телефонов – Сервисный центр Мобиопт
Online casinos should test—try me! mines
интернет домашний воронеж
domashij-internet-voronezh003.ru
интернет тарифы воронеж
[url=https://chestnye-casino.ru]chestnye-casino.ru[/url]
На сайте, посвященном обзорам онлайн казино, вы найдете независимую оценку и рейтинг самых надежных игровых платформ. Здесь представлены только проверенные и лицензированные казино, прошедшие тщательную проверку экспертов. Вы узнаете о преимуществах и недостатках каждого заведения, получите информацию о бонусах, игровых автоматах, методах оплаты и качестве службы поддержки. Сайт поможет вам сделать осознанный выбор, чтобы играть в слоты на деньги в безопасной и честной среде, минимизируя риски и получая максимум удовольствия от игры. Кроме того, ресурс содержит полезные советы и стратегии, которые помогут вам повысить свои шансы на выигрыш и сделать процесс игры еще более увлекательным. Регулярное обновление обзоров и добавление новых казино позволит вам всегда быть в курсе актуальных предложений и выбрать именно то казино, которое подходит вашим потребностям и предпочтениям.
chestnye-casino.ru
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наша продукция:
Керамическая мишень АZO для распыления
Заказать диплом университета по доступной стоимости возможно, обратившись к проверенной специализированной компании. Заказать документ института можно в нашей компании. [url=http://diplomp-irkutsk.ru/kupite-diplom-ob-obrazovanii-s-registratsiej-v-reestre/]diplomp-irkutsk.ru/kupite-diplom-ob-obrazovanii-s-registratsiej-v-reestre[/url]
Купить диплом университета!
Мы готовы предложить документы университетов, расположенных в любом регионе Российской Федерации.
[url=http://good-diplom.ru/kupit-diplom-s-zaneseniem-v-reestr-vigodno/]good-diplom.ru/kupit-diplom-s-zaneseniem-v-reestr-vigodno/[/url]
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с высоким уровнем сервиса.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – идеальный вариант для вас.
Наши товары:
РџРѕРєРѕРІРєР° круглая РёР· конструкционной стали 930 РјРј 45РҐ Купить конструкционные круглые РїРѕРєРѕРІРєРё РїРѕ выгодным ценам РІ компании Редметсплав.СЂС„. РЁРёСЂРѕРєРёР№ выбор размеров Рё марок стали. Прочные Рё надежные РїРѕРєРѕРІРєРё для различных отраслей промышленности. Рндивидуальный РїРѕРґС…РѕРґ Рє каждому клиенту Рё гарантированное качество продукции.
DIOR replica designer bags
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
Наша продукция:
Рнструментальная квадратная РїРѕРєРѕРІРєР° 115 РјРј РҐ12Р’РњР¤ ГОСТ 5950-2000 Познакомьтесь СЃ широким ассортиментом высокопрочных инструментов для обработки металла РІ категории инструментальной квадратной РїРѕРєРѕРІРєРё РѕС‚ Редметсплав. Выбирайте РёР· прочных Рё надежных материалов, подобранных специально для различных РІРёРґРѕРІ металлообработки. Гарантированное качество РѕС‚ ведущих производителей.
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
Наши товары:
РўСЂСѓР±Р° РёР· драгоценных металлов платиновая 700С…2С…0.5 РјРј РџР»Р90-10 РўРЈ Гарантированное качество Рё изысканный дизайн – купите драгоценные трубы для ювелирных украшений, машиностроения Рё архитектуры. РЁРёСЂРѕРєРёР№ выбор Рё высокое качество! Доставка РїРѕ Р РѕСЃСЃРёРё.
Мы изготавливаем дипломы любых профессий по приятным ценам. Мы готовы предложить документы ВУЗов, расположенных на территории всей РФ. Дипломы и аттестаты выпускаются на бумаге самого высшего качества. Это позволяет делать настоящие дипломы, не отличимые от оригиналов. [url=http://phat4life.mn.co/posts/82586375/]phat4life.mn.co/posts/82586375[/url]
диплом о высшем образовании купить [url=https://rusdiplomm-orig.ru/]диплом о высшем образовании купить[/url] .
[url=https://official-zooma-casino.ru]official-zooma-casino.ru[/url]
На сайте, посвященном обзору официального сайта Zooma Casino, представлен исчерпывающий анализ платформы, призванный помочь игрокам принять обоснованное решение. Здесь вы найдете детальный разбор интерфейса и удобства использования, обзор доступных игр и их поставщиков, а также информацию о бонусной программе и условиях ее получения. Особое внимание уделено вопросам безопасности и лицензирования, чтобы убедиться в надежности и честности казино. Кроме того, в обзоре представлены данные о скорости выплат, доступных платежных методах и работе службы поддержки, что позволит вам составить полное представление о Zooma Casino и оценить его соответствие вашим требованиям и предпочтениям. Отзывы реальных пользователей помогут сформировать более объективное мнение и избежать возможных неприятных сюрпризов.
official-zooma-casino.ru
Great post! I really enjoyed reading this and learned a lot. Thanks for sharing!
Looking for Ukraine flowers delivery? Ukraineflora.com is the best choice for any event. We have flowers in Ukraine in a huge variety! Look at it now, we are sure you will like everything. Now it is not difficult to make flower delivery in Ukraine, just go to the portal and get acquainted with our offers in more detail, here are fresh flowers, bouquets, and baskets. You can also send gifts to Ukraine. Gift delivery in Ukraine is an opportunity to please your relatives even more.
mines in india
Meds information leaflet. Cautions.
seroquel ocd
Actual trends of pills. Get information here.
Заказать диплом любого ВУЗа!
Наша компания предлагаетбыстро приобрести диплом, который выполнен на оригинальном бланке и заверен мокрыми печатями, водяными знаками, подписями официальных лиц. Данный диплом пройдет лубую проверку, даже с применением профессиональных приборов. Достигайте своих целей быстро и просто с нашей компанией- [url=http://ralphouensanga.com/read-blog/112_pokupka-diplomov-s-reestrom.html/]ralphouensanga.com/read-blog/112_pokupka-diplomov-s-reestrom.html[/url]
play fishin frenzy free
most bet [url=www.mostbet5009.ru]most bet[/url] .
Chanel bag replica
mines
Fendi fake designer bags
plinko balls
РедМетСплав предлагает внушительный каталог качественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица изделия подтверждена требуемыми документами, подтверждающими их соответствие стандартам. Опытная поддержка – наш стандарт – мы на связи, чтобы ответить на ваши вопросы и предоставлять решения под требования вашего бизнеса.
Доверьте вашу потребность в редких металлах специалистам РедМетСплав и убедитесь в гибкости нашего предложения
поставляемая продукция:
Фольга кобальтовая РРљ20-ВРФольга кобальтовая РРљ20-ВР– это высококачественный материал, используемый РІ различных отраслях, включая электронику Рё медицинское оборудование. Отличается превосходной устойчивостью Рє РєРѕСЂСЂРѕР·РёРё Рё высоким температурным нагрузкам. Благодаря своей прочности, фольга РїРѕРґС…РѕРґРёС‚ для создания надежных защитных покрытий. Если вам необходим материал, который гарантирует долговечность Рё надежность, купить Фольга кобальтовая РРљ20-ВРбудет отличным решением. Ртот РїСЂРѕРґСѓРєС‚ идеально РїРѕРґС…РѕРґРёС‚ для применения РІ условиях высоких требований Рє качеству.
fake prada bag
РедМетСплав предлагает внушительный каталог высококачественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица изделия подтверждена требуемыми документами, подтверждающими их качество. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы ответить на ваши вопросы а также адаптировать решения под специфику вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в гибкости нашего предложения
Наши товары:
Лента магниевая MAG 9 – BS 2970 РљСЂСѓРі магниевый MAG 9 – BS 2970 предназначен для высококачественного шлифования различных материалов. Его прочная конструкция обеспечивает отличную стойкость Рє РёР·РЅРѕСЃСѓ Рё длительный СЃСЂРѕРє службы. РЎ помощью этого РєСЂСѓРіР° РјРѕР¶РЅРѕ добиться идеальной обработки поверхностей, придавая РёРј гладкость Рё блеск. РќРµ упустите возможность улучшить качество СЃРІРѕРёС… работ Рё ускорить процесс. Купите РљСЂСѓРі магниевый MAG 9 – BS 2970 Рё убедитесь РІ его эффективности. Выберите надежный инструмент для ваших шлифовальных задач!
Online gambling can rise—hold it! plinko
РедМетСплав предлагает внушительный каталог высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до масштабных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица продукции подтверждена всеми необходимыми документами, подтверждающими их качество. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы ответить на ваши вопросы по мере того как предоставлять решения под особенности вашего бизнеса.
Доверьте ваш запрос специалистам РедМетСплав и убедитесь в гибкости нашего предложения
поставляемая продукция:
Проволока магниевая M11610 – UNS Полоса магниевая M11610 – UNS предназначена для различных промышленных применений, РіРґРµ требуется высокая прочность Рё легкость. Рзготовленная РёР· магниевого сплава, РѕРЅР° обладает отличной РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью Рё РїРѕРґС…РѕРґРёС‚ для использования РІ тяжелых условиях. Благодаря СЃРІРѕРёРј конструктивным свойствам, Полоса магниевая M11610 – UNS является идеальным выбором для авиастроения Рё автомобилестроения. Если вам РЅСѓР¶РЅР° надежная Рё качественная полоса для вашего проекта, рекомендуем купить Полоса магниевая M11610 – UNS. РћРЅР° обеспечит вам долгий СЃСЂРѕРє службы Рё высокую производительность РІ любых условиях.
[url=https://frispiny-casino.ru]frispiny-casino.ru[/url]
На сайте, специализирующемся на обзорах онлайн-казино, вы найдете детальную информацию о предложениях фриспинов, предоставляемых при регистрации. Здесь собраны актуальные данные о самых выгодных и привлекательных акциях от различных игровых платформ. Вы узнаете, какие казино предлагают наибольшее количество бесплатных вращений, на каких слотах их можно использовать, и каковы условия их отыгрыша. Благодаря подробным обзорам, вы сможете сравнить различные предложения фриспинов и выбрать те, которые наилучшим образом соответствуют вашим потребностям и предпочтениям, чтобы начать игру с максимальной выгодой и возможностью сорвать крупный выигрыш без риска для собственных средств.
frispiny-casino.ru
Ищете сельхозтехнику и оборудование по самым выгодным ценам от производителя? Посетите сайт Производителя ООО МИТЕХ https://mi-teh.ru/ где вы также найдете запасные части и комплектующие к сельхоз машинам и тракторам. Ознакомьтесь с нашим ассортиментом продукции на сайте.
plinko app
Louis Vuitton bag replica
На сайте https://officepro54.ru представлено огромное количество мебели, которая идеально подходит для организации офисного пространства. Вся она выполнена из инновационных, уникальных материалов, а потому прослужит очень долго, не синтезирует в воздух опасных веществ. В разделе вы найдете кабинет руководителя, функциональные и вместительные столы для переговоров, шкафы-купе, сейфы, акустические системы, ученическую мебель и многое другое. Постоянно действуют акции. На продукцию установлены привлекательные цены.
В случае если желаете узнать самую ценную, полезную и важную информацию о трейдинге, вложениях, то нужно зайти на портал, где вы отыщете все необходимое на такую тему. На портале просто рассказывается то, как торговать на внебиржевом пространстве. https://fxidea.com/ предлагает акции всем начинающим. Скорее ознакомьтесь с индикаторами, отзывами трейдеров, самыми эффективными стратегиями. Вы получите доступ к ценным материалам, касающимся криптовалюты, а также узнаете, как заработать на форексе.
мрстбет [url=mostbet5010.ru]мрстбет[/url] .
fake Celine bag
bet on red
провайдеры интернета в омске
domashij-internet-omsk001.ru
интернет провайдер омск
[url=https://official-zooma-casino.ru]official-zooma-casino.ru[/url]
На сайте, посвященном обзору официального сайта Zooma Casino, представлен исчерпывающий анализ платформы, призванный помочь игрокам принять обоснованное решение. Здесь вы найдете детальный разбор интерфейса и удобства использования, обзор доступных игр и их поставщиков, а также информацию о бонусной программе и условиях ее получения. Особое внимание уделено вопросам безопасности и лицензирования, чтобы убедиться в надежности и честности казино. Кроме того, в обзоре представлены данные о скорости выплат, доступных платежных методах и работе службы поддержки, что позволит вам составить полное представление о Zooma Casino и оценить его соответствие вашим требованиям и предпочтениям. Отзывы реальных пользователей помогут сформировать более объективное мнение и избежать возможных неприятных сюрпризов.
official-zooma-casino.ru
plinko casino
I won $600 online—unreal moment! vipzino
DIOR fake designer bags
plinko balls
мостбет войти [url=https://www.mostbet5009.ru]https://www.mostbet5009.ru[/url] .
Fendi replica designer bags
Fendi replica designer bags
[url=https://frispiny-casino.ru]frispiny-casino.ru[/url]
На сайте, специализирующемся на обзорах онлайн-казино, вы найдете детальную информацию о предложениях фриспинов, предоставляемых при регистрации. Здесь собраны актуальные данные о самых выгодных и привлекательных акциях от различных игровых платформ. Вы узнаете, какие казино предлагают наибольшее количество бесплатных вращений, на каких слотах их можно использовать, и каковы условия их отыгрыша. Благодаря подробным обзорам, вы сможете сравнить различные предложения фриспинов и выбрать те, которые наилучшим образом соответствуют вашим потребностям и предпочтениям, чтобы начать игру с максимальной выгодой и возможностью сорвать крупный выигрыш без риска для собственных средств.
frispiny-casino.ru
What if you double your funds before even placing your first bet? With the 1xBet promo code 1XBRO200, new players can kick-start their adventure with a 100% welcome bonus https://myworldgo.com/forums/topic/211047/code-promo-1x-bet-exclusif-tours-gratuits/view/post_id/2250793
vai de bet gratis
мостбет мобильная версия скачать [url=http://mostbet3021.ru/]http://mostbet3021.ru/[/url] .
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
Наша продукция:
Медная двухраструбная редукционная переходная муфта РїРѕРґ пайку 15С…12 РјРј мягкая пайка Рњ1 ГОСТ 32590-2013 Выберите высококачественные медные двухраструбные редукционные муфты РїРѕРґ пайку РѕС‚ Редметсплав для надежного соединения медных труб различных диаметров. РЁРёСЂРѕРєРёР№ выбор размеров, эффективная установка Рё долговечность гарантированы. Рдеальное решение для систем отопления Рё водоснабжения.
мостбет скачать на андроид [url=https://mostbet7005.ru]мостбет скачать на андроид[/url] .
The service will help you to attract the indexing robots of Google search engine (Googlebot) and get indexed your web page or backlinks faster BLACK SEO LINKS, BACKLINKS, SOFTWARE FOR MASS BACKLINKING – TELEGRAM @SEO_LINKK
Fendi fake designer bags
mostbest [url=https://mostbet3021.ru]https://mostbet3021.ru[/url] .
mostbet kg скачать [url=www.mostbet7005.ru]www.mostbet7005.ru[/url] .
Drug prescribing information. Short-Term Effects.
lyrica tablets price ksa
All about medicament. Get information now.
plinko demo
Prada bag replica
служба поддержки мостбет номер телефона [url=http://mostbet7005.ru]http://mostbet7005.ru[/url] .
скачать mostbet [url=https://www.mostbet3021.ru]https://www.mostbet3021.ru[/url] .
Мы предлагаем выгодно и быстро приобрести диплом, который выполнен на оригинальном бланке и заверен печатями, штампами, подписями должностных лиц. Данный документ способен пройти любые проверки, даже при помощи специально предназначенного оборудования. [url=http://linkat.app/read-blog/3106_kupit-diplom-s-zaneseniem-v-reestr-instituta.html/]linkat.app/read-blog/3106_kupit-diplom-s-zaneseniem-v-reestr-instituta.html[/url]
Medication information for patients. Cautions.
can i order generic xenical without a prescription
All news about pills. Get here.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым текущие трудности превращаются в завтрашние успехи.
Наши товары:
Плоская мишень для распыления свинца 4N
Chanel replica designer bags
mostbet casino [url=www.mostbet3021.ru]www.mostbet3021.ru[/url] .
mostbet официальный сайт [url=https://mostbet7005.ru]mostbet официальный сайт[/url] .
[url=frispiny-casino-registration.ru]frispiny-casino-registration.ru[/url]
На этом сайте вы найдете полный обзор фриспинов, предоставляемых онлайн казино при регистрации. Мы собрали актуальную информацию о самых щедрых предложениях, доступных новым игрокам. Вы узнаете, какие казино предлагают наибольшее количество бесплатных вращений, на каких слотах их можно использовать, и какие условия необходимо выполнить для вывода выигрышей. Подробные обзоры включают информацию о вейджерах, сроках действия фриспинов и других важных нюансах, которые помогут вам максимально выгодно воспользоваться этими бонусами. Мы поможем вам выбрать лучшее предложение и начать игру в онлайн казино с минимальными рисками и максимальными шансами на выигрыш. Сайт регулярно обновляется, чтобы вы всегда были в курсе самых свежих и выгодных акций.
frispiny-casino-registration.ru
РедМетСплав предлагает внушительный каталог качественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица продукции подтверждена требуемыми документами, подтверждающими их качество. Опытная поддержка – то, чем мы гордимся – мы на связи, чтобы разрешать ваши вопросы по мере того как находить ответы под особенности вашего бизнеса.
Доверьте ваш запрос специалистам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
Фольга висмутовая Sn96Ag2,5Bi1Cu0,5 – ISO 9453 Фольга висмутовая Sn96Ag2,5Bi1Cu0,5 – ISO 9453 является качественным материалом для пайки, обеспечивая отличные электрические Рё механические свойства. РћРЅР° РїРѕРґС…РѕРґРёС‚ для различных областей применения, включая электронику Рё энергетику. Уникальная формула восемьдесят шесть процентов содержания олова, серебра, висмута Рё меди гарантирует высокую устойчивость Рє РєРѕСЂСЂРѕР·РёРё Рё РёР·РЅРѕСЃСѓ. Купить Фольга висмутовая Sn96Ag2,5Bi1Cu0,5 – ISO 9453 РјРѕР¶РЅРѕ РїСЂСЏРјРѕ сейчас, чтобы обеспечить надежность Рё долговечность ваших соединений. Обратите внимание РЅР° преимущества, которые предлагает данный РїСЂРѕРґСѓРєС‚.
This is exactly what I was looking for—thanks for the helpful tips!
Заказать диплом любого института. Приобретение диплома через проверенную и надежную компанию дарит ряд плюсов. Такое решение позволяет сберечь как дорогое время, так и значительные денежные средства. [url=http://w77515cs.beget.tech/2025/04/07/diplom-oficialnogo-obrazca.html/]w77515cs.beget.tech/2025/04/07/diplom-oficialnogo-obrazca.html[/url]
подключить интернет омск
domashij-internet-omsk002.ru
провайдеры интернета омск
Chanel bag replica
Мы изготавливаем дипломы любой профессии по разумным тарифам. Стараемся поддерживать для покупателей адекватную ценовую политику. Важно, чтобы документы были доступными для подавляющей массы наших граждан.
Приобретение документа, подтверждающего окончание института, – это рациональное решение. Приобрести диплом о высшем образовании: [url=http://kupite-diplom0024.ru/kupit-diplom-kolledzha-v-moskve/]kupite-diplom0024.ru/kupit-diplom-kolledzha-v-moskve/[/url]
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наша продукция:
Гранулы оксида алюминия 4N
The payout waits online drag—speed on! Jackpot Raider
Medicine information for patients. Drug Class.
taken too many metformin
All what you want to know about medicine. Get information here.
mostber [url=http://mostbet6033.ru]http://mostbet6033.ru[/url] .
fake Louis Vuitton bag
nokta atışı su kaçak tespiti Bulgurlu su kaçağı tespiti: Bulgurlu’da su kaçağına anında müdahale. https://ai.florist/read-blog/12668_uskudar-su-tesisatcisi.html
most bet [url=mostbet6033.ru]mostbet6033.ru[/url] .
Celine replica designer bags
Купить диплом ВУЗа по выгодной стоимости возможно, обратившись к надежной специализированной фирме. Заказать документ ВУЗа вы сможете в нашей компании в столице. [url=http://diplomj-irkutsk.ru/kupit-diplom-visshem-obrazovanii-zaneseniem-reestr-12/]diplomj-irkutsk.ru/kupit-diplom-visshem-obrazovanii-zaneseniem-reestr-12[/url]
Drug information sheet. Generic Name.
can you buy zoloft prices
All what you want to know about drug. Get information now.
DIOR bag replica
Приобрести диплом о высшем образовании!
Мы можем предложить документы институтов, расположенных в любом регионе РФ.
[url=http://diplomc-v-ufe.ru/bistroe-i-nadezhnoe-vnesenie-diploma-v-reestr/]diplomc-v-ufe.ru/bistroe-i-nadezhnoe-vnesenie-diploma-v-reestr/[/url]
DIOR bag replica
Мы предлагаем дипломы любых профессий по приятным тарифам. Мы предлагаем документы ВУЗов, расположенных на территории всей России. Документы выпускаются на “правильной” бумаге самого высшего качества. Это дает возможности делать настоящие дипломы, которые не отличить от оригиналов. [url=http://arzookanak0077.copiny.com/question/details/id/1082984/]arzookanak0077.copiny.com/question/details/id/1082984[/url]
Заказать диплом университета!
Наши специалисты предлагаютвыгодно и быстро заказать диплом, который выполняется на оригинальном бланке и заверен мокрыми печатями, водяными знаками, подписями. Документ пройдет любые проверки, даже с использованием профессионального оборудования. Решите свои задачи быстро с нашей компанией- [url=http://collie.fatbb.ru/posting.phpmode=post&f=18&sid=411cb223026489cf5677d2add674db01/]collie.fatbb.ru/posting.phpmode=post&f=18&sid=411cb223026489cf5677d2add674db01[/url]
куплю диплом самой [url=https://rusdiplomm-orig.ru/]rusdiplomm-orig.ru[/url] .
Celine bag replica
avis plinko
Хотите, чтобы ваш портал занимал лидирующие позиции в поисковых системах и привлекал тысячи пользователей? Вечные ссылки – ваш ключ к успеху! Наши преимущества: белые методы продвижения, гибкие тарифы, быстрый результат. Мы после оплаты сразу же приступаем к осуществлению заказа. Ищете сео продвижение сайта? SeoBomba.ru – тут опубликована более подробная о нас информация, посмотрите ее в любое время. Также здесь ответы на часто задаваемые вопросы представлены. Не упустите возможность вывести свой сайт на новый уровень. Созвонитесь с нами для консультации.
[url=frispiny-casino-registration.ru]frispiny-casino-registration.ru[/url]
На этом сайте вы найдете полный обзор фриспинов, предоставляемых онлайн казино при регистрации. Мы собрали актуальную информацию о самых щедрых предложениях, доступных новым игрокам. Вы узнаете, какие казино предлагают наибольшее количество бесплатных вращений, на каких слотах их можно использовать, и какие условия необходимо выполнить для вывода выигрышей. Подробные обзоры включают информацию о вейджерах, сроках действия фриспинов и других важных нюансах, которые помогут вам максимально выгодно воспользоваться этими бонусами. Мы поможем вам выбрать лучшее предложение и начать игру в онлайн казино с минимальными рисками и максимальными шансами на выигрыш. Сайт регулярно обновляется, чтобы вы всегда были в курсе самых свежих и выгодных акций.
frispiny-casino-registration.ru
Ищите место для отдыха? иордания экскурсионная Мы предлагаем увлекательные экскурсии по Иордании, комфортный отдых и маршруты по главным достопримечательностям Иордании. Не знаете, что посмотреть в Иордании? Начните с Петры, Вади Рам и Мёртвого моря!
plinko opinioni
домашний интернет тарифы омск
domashij-internet-omsk003.ru
подключить домашний интернет омск
Medication information for patients. Long-Term Effects.
buy cheap nolvadex
Everything news about medicine. Read information now.
Ganhe 100$ de Bônus no dobrowin ao Criar Sua Conta!
fake DIOR bag
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
Наша продукция:
Дюралевый швеллер 440076 15С…20С…1.5С…1.5С…1.5 РјРј Р”1 ГОСТ 4784-97 Купить дюралевый швеллер РѕС‚ производителя. Прочный Рё легкий материал для различных областей строительства. РЁРёСЂРѕРєРёР№ выбор размеров Рё профилей. Устойчивость Рє РєРѕСЂСЂРѕР·РёРё Рё надежность конструкций. Рдеальное решение для авиации, судостроения, автомобилестроения, производства спортивного оборудования Рё фармакологических предприятий.
Phim sex clip sex Việt Nam
fake Louis Vuitton bag
fortune tiger bet
Chanel bag replica
plinko’
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
С широким ассортиментом продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наши товары:
Мишень для распыления лютеция
Meds information. Generic Name.
ezetimibe a statin or something else unraveling the mystery
Actual what you want to know about drug. Read now.
Online casinos feel off—loss streak! plinko
Louis Vuitton replica designer bags
РедМетСплав предлагает широкий ассортимент качественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их качество. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы ответить на ваши вопросы а также адаптировать решения под специфику вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
Наша продукция:
Лента висмутовая H48Bi8A – JIS Z 3282 Лента висмутовая H48Bi8A – JIS Z 3282 является высококачественным материалом, который активно применяется РІ электротехнике. Рта лента идеально РїРѕРґС…РѕРґРёС‚ для создания защищенных соединений Рё соединительных узлов. Высокая прочность Рё стойкость Рє РєРѕСЂСЂРѕР·РёРё делают её незаменимой РІ производстве Рё ремонте. Если РІС‹ ищете надежный Рё долговечный материал, то эта лента станет отличным выбором. Чтобы обеспечить надежность Рё эффективность своей работы, рекомендуется купить Лента висмутовая H48Bi8A – JIS Z 3282 СѓР¶Рµ сегодня!
Celine bag replica
мос бет [url=http://mostbet6033.ru/]http://mostbet6033.ru/[/url] .
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с прекрасным качеством обслуживания.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
поставляемая продукция:
Медный тройник РїРѕРґ пайку стандартный 76.1С…65С…1.6 РјРј 33.5С…36.5 РјРј твердая пайка Рњ2СЂ ГОСТ Р 52922-2008 Купить качественные медные тройники РїРѕРґ пайку для надежных Рё долговечных соединений РІ трубопроводных системах. Разнообразие размеров Рё конфигураций, высокая теплопроводность Рё устойчивость Рє РєРѕСЂСЂРѕР·РёРё. Простая установка Рё надежность соединения. Рдеальное решение для различных проектов. Редметсплав.СЂС„
DIOR bag replica
Drug information for patients. Short-Term Effects.
cost of generic lyrica prices
Actual what you want to know about medicines. Read information now.
jogo do tigrinho fortune tiger
Very well written. Looking forward to more posts like this!
GetGrass.io is a decentralized platform that lets users earn rewards by sharing their unused internet bandwidth. The collected traffic is used to gather public web data for training AI models, ensuring transparency and privacy. You can join the network through their browser extension or desktop app — https app getgrass register.
мостбет скачать [url=https://mostbet7006.ru]https://mostbet7006.ru[/url] .
fake Celine bag
DIOR fake designer bags
склад хранения частных вещей [url=http://hranim-veshi-msk24.ru/]http://hranim-veshi-msk24.ru/[/url] .
plinko game avis
РедМетСплав предлагает обширный выбор отборных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица продукции подтверждена соответствующими документами, подтверждающими их происхождение. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы улаживать ваши вопросы а также предоставлять решения под специфику вашего бизнеса.
Доверьте вашу потребность в редких металлах специалистам РедМетСплав и убедитесь в гибкости нашего предложения
поставляемая продукция:
Пруток кобальтовый РРљ20-ВРПруток кобальтовый РРљ20-ВР– это высококачественный легированный металл, обладающий отличными механическими свойствами. Рспользуется РІ машиностроении, авиации Рё РґСЂСѓРіРёС… отраслях. Его повышенная устойчивость Рє РєРѕСЂСЂРѕР·РёРё Рё высоким температурам делает его незаменимым для производства ответственных деталей. Если РІС‹ хотите обеспечить надежность Рё долговечность СЃРІРѕРёС… изделий, купить Пруток кобальтовый РРљ20-ВР– правильный выбор. Поддерживайте высокие стандарты качества РІ своем производстве СЃ помощью этого уникального материала!
fishin frenzy even bigger catch demo
Throughout this review, we will describe the advantages and benefits of betting on 1xBet from Argentina, as well as tell you a little about the brand, its promotions, and how to use these promo codes on its platform to take advantage of its promos https://myworldgo.com/forums/topic/211213/codigo-promocional-1x-bet-bono-apuesta-gratis-hasta-130/view/post_id/2252984
Fendi fake designer bags
One of these tools is bonuses, with the help of which you can quickly get the attention of players, increase the interest of users in your own gaming product https://iesriojucar.es/pages/el-codigo-promocional-1xbet_bono_100.html
Medication information. Short-Term Effects.
can i get cheap ampicillin prices
Some about pills. Get now.
Medicament information for patients. What side effects?
can i buy requip
Actual what you want to know about pills. Get now.
fake prada bag
see this here https://phoenixtrade.me
I wish online kept old—love that! book of ra
Мы можем предложить дипломы любой профессии по выгодным тарифам. Всегда стараемся поддерживать для клиентов адекватную политику тарифов. Для нас важно, чтобы документы были доступными для большого количества наших граждан.
Заказ документа, подтверждающего окончание ВУЗа, – это грамотное решение. Купить диплом ВУЗа: [url=http://10000diplomov.ru/kupit-diplom-srednee-spetsialnoe/]10000diplomov.ru/kupit-diplom-srednee-spetsialnoe/[/url]
DIOR fake designer bags
?Hola jugadores
Las casas apuestas legales EspaГ±a tienen lГmites mensuales obligatorios.
Las casas de apuestas fiables sin licencia existen. Solo necesitas revisar si tienen cifrado, auditorГas externas y buenas reseГ±as de usuarios.
Mas detalles en el enlace – http://casasdeapuestassinlicenciaespana.xyz/
?Que tengas excelentes botes acumulados!
check my source https://balancer.ac
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наши товары:
РљСЂСѓРі РёР· магнитно-РјСЏРіРєРёС… сплавов 14 РјРј 16РҐ ГОСТ 10994-74 Получите РєСЂСѓРіРё РёР· магнитно-РјСЏРіРєРёС… сплавов высочайшего качества для разнообразных технических приложений РѕС‚ компании Редметсплав.СЂС„. Наши уникальные РєСЂСѓРіРё обладают превосходными магнитными свойствами, идеальной мягкостью Рё обеспечивают надежность РІ различных условиях эксплуатации. Рспользуйте наши РєСЂСѓРіРё для электроники, энергетики, медицины Рё РґСЂСѓРіРёС… областей Рё получите максимальную производительность Рё эффективность.
Chanel bag replica
мос бет [url=https://mostbet6033.ru]мос бет[/url] .
Drugs information sheet. What side effects can this medication cause?
where buy cheap aldactone
All trends of medication. Get now.
Платформа alcodostavkashop88.ru предлагает уникальную возможность [url=https://alcodostavkashop88.ru/]купить алкоголь круглосуточно[/url]. Это особенно актуально в ночное время и в экстренных ситуациях. Услуга доступна в любой район Москвы, все напитки проходят обязательную проверку качества.
?? Top Adult Webcam Sites! ??
Join hot models for private chats! Don’t wait – and enjoy!
https://livenude.blog/
РедМетСплав предлагает внушительный каталог качественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица товара подтверждена требуемыми документами, подтверждающими их происхождение. Дружелюбная помощь – наш стандарт – мы на связи, чтобы разрешать ваши вопросы и предоставлять решения под специфику вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
поставляемая продукция:
Пруток кобальтовый РРџ558 Пруток кобальтовый РРџ558 – это высококачественный металл, используемый РІ различных промышленных приложениях. РћРЅ славится своей превосходной РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью Рё механическими свойствами. Применяется РІ производстве инструментов, обладающих высокой твердостью, Р° также РІ изготовлении деталей для работы РїСЂРё высоких температурах. Приобретая этот РїСЂРѕРґСѓРєС‚, РІС‹ обеспечиваете СЃРІРѕСЋ продукцию надежностью Рё долговечностью. Удобен РІ обработке Рё хорошо сочетируется СЃ РґСЂСѓРіРёРјРё металлами. Р’С‹ можете купить Пруток кобальтовый РРџ558, который станет отличным выбором для ваших производственных РЅСѓР¶Рґ.
подключить интернет тарифы пермь
domashij-internet-perm001.ru
провайдеры пермь
Gucci bag replica
gra plinko
I hit a bonus online and won $60—cool! plinko
Medicines prescribing information. What side effects can this medication cause?
how to get generic kamagra without rx
All trends of drugs. Read information now.
Chanel replica designer bags
купить дипломы о высшем образовании в тюмени [url=https://rusdiplomm-orig.ru/]rusdiplomm-orig.ru[/url] .
Гортензии именно того оттенка, что я хотела!
заказать цветы с доставкой в томске
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние успехи.
Наши товары:
Высокочистые гранулы титана
На Куршскую косу из Зеленоградска экскурсия индивидуальная https://kurshskaya-kosa-ekskursii.ru/ позволяет насладиться природой без толпы туристов
Drug information leaflet. Long-Term Effects.
generic celebrex prices
Everything news about medicament. Get information here.
fake Bottega Veneta bag
plinko demo
Chanel fake designer bags
Команда рекламное агентство Витрувий знает, как вывести вашу компанию в лидеры. Мы продумываем стратегию, оптимизируем кампании и добиваемся максимального эффекта.
DIOR fake designer bags
plinko reviews
pin up casino azerbaijan [url=http://pinup-azerbaycan5.com/]pin up casino azerbaijan[/url] .
Interesting perspective! It gave me a new way of thinking about this topic.
betonred casino no deposit bonus
I won $150 on an online slot—pure adrenaline! dragon tiger
трансформатор силовой трехфазный сухой [url=https://suhie-transformatory-kupit.ru/]трансформатор силовой трехфазный сухой[/url] .
Thanks for the valuable information. It was easy to understand and super useful.
DIOR bag replica
plinko echtgeld
fake Celine bag
Drug information for patients. Brand names.
how to get cheap lansoprazole for sale
All about medicine. Read now.
ячейки хранения вещей [url=https://hranim-veshi-msk24.ru/]https://hranim-veshi-msk24.ru/[/url] .
Pills information. What side effects?
where buy aricept without dr prescription
All news about medicine. Read here.
дешевый интернет пермь
domashij-internet-perm002.ru
тарифы интернет и телевидение пермь
bet on red casino
Louis Vuitton fake designer bags
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние возможности.
Наша продукция:
Мишень железохромовая плоская для распыления
plinko fake
поддержка мостбет [url=mostbet7007.ru]mostbet7007.ru[/url] .
fortune tiger bet
pin up casino azerbaijan [url=http://pinup-azerbaycan5.com]pin up casino azerbaijan[/url] .
Online slots hook me—those vibes! teen patti
Celine replica designer bags
сухие трансформаторы [url=suhie-transformatory-kupit.ru]сухие трансформаторы[/url] .
Chanel replica designer bags
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с прекрасным качеством обслуживания.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – наилучшее решение для вашего бизнеса.
поставляемая продукция:
Кольцо РёР· драгоценных металлов платиновое 150С…2С…2.5 РјРј РџР»99.9 РўРЈ РЁРёСЂРѕРєРёР№ выбор высококачественных кольц РёР· драгоценных металлов. Рлегантные украшения, созданные талантливыми мастерами. Уникальность Рё изысканность РІ каждой детали. Возможность заказа персонального кольца РїРѕ вашим пожеланиям. Подчеркните СЃРІРѕСЋ индивидуальность СЃ нашими кольцами.
Pills information for patients. Long-Term Effects.
availability of cymbalta in the uk exploring access and options
Best information about drug. Get information here.
the aviator game
dragon tiger 51 bonus
motbet [url=http://mostbet7006.ru]http://mostbet7006.ru[/url] .
Chanel bag replica
Pills information. Drug Class.
lyrica for sale
Best what you want to know about medicine. Get now.
fake Gucci bag
fake Fendi bag
РедМетСплав предлагает обширный выбор высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы обеспечиваем быстрое выполнение вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их качество. Опытная поддержка – то, чем мы гордимся – мы на связи, чтобы разрешать ваши вопросы и адаптировать решения под особенности вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наша продукция:
Фольга магниевая QE22 – CSA HG.9 РўСЂСѓР±Р° магниевая QE22 – CSA HG.9 – это высококачественный изделия, отличающееся легкостью Рё прочностью. РќРµ подвержена РєРѕСЂСЂРѕР·РёРё Рё станет идеальным решением для различных промышленных применений. Ее уникальная структура обеспечивает отличные механические характеристики, что делает эту трубу надежной РІ эксплуатации. Если РІС‹ ищете долговечный Рё экономичный РїСЂРѕРґСѓРєС‚, чтобы удовлетворить ваши РЅСѓР¶РґС‹, то купить РўСЂСѓР±Р° магниевая QE22 – CSA HG.9 будет отличным выбором. Ртот товар способен существенно повысить эффективность ваших проектов.
I love the online peace—no crowd! Marvel Casino
подключить интернет в квартиру пермь
domashij-internet-perm003.ru
домашний интернет
dragon tiger game download 51 bonus
скачать mostbet [url=https://mostbet7006.ru/]https://mostbet7006.ru/[/url] .
Обзор на самые лучшие сайты для продажи скинов, которые имеют хорошую репутацию среди комьюнити.
Если вы ищете, где продать предметы кс где выгоднее продать скины CS2, CS:GO, DOTA 2 за реальные деньги или хотите узнать, как быстро и сразу продать скины с выводом на карту.
Prada bag replica
1xBet — download the app for Android and iOS. The 1xBet app allows millions of players from all over the world to place sports bets as quickly as possible http://hotel-golebiewski.phorum.pl/viewtopic.php?p=561684#561684
Medicines information for patients. What side effects can this medication cause?
can i get lyrica tablets
Actual information about medicines. Read information now.
Melbet is not just a casino, but also a bookmaker platform with bets on popular sports: football, basketball, tennis, hockey and others https://concretesubmarine.activeboard.com/t71837216/code-promo-melbet-bonus-de-bienvenue-100-jusqu-130/?page=last#lastPostAnchor
pin up azerbaycan [url=pinup-azerbaycan5.com]pin up azerbaycan[/url] .
Алкогольный запой является тяжёлым состоянием, требующим срочного медицинского вмешательства. Наркологическая клиника «Пульс» в Краснодаре предлагает эффективную помощь в борьбе с алкогольной зависимостью, используя проверенный метод — капельницу от запоя на дому. Наши специалисты оперативно выезжают по указанному адресу и обеспечивают качественную медицинскую помощь с гарантией конфиденциальности и безопасности.
Получить дополнительную информацию – [url=https://kapelnica-ot-zapoya-krasnodar7.ru/]капельница от запоя цена краснодарский край[/url]
mostbet uz haqida [url=https://www.mostbet3023.ru]https://www.mostbet3023.ru[/url] .
трансформаторы сухие [url=https://suhie-transformatory-kupit.ru]трансформаторы сухие[/url] .
Fendi fake designer bags
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
Наша продукция:
Пружина из драгоценных металлов платиновая 1000х1х0.4 мм Пл99.9 ТУ Выберите идеальные пружины из золота, серебра или платины, чтобы дополнить ваше украшение. Найдите пружины с изысканным дизайном и прочностью. Они предлагают широкий выбор для того, чтобы подчеркнуть уникальность вашего украшения.
скачать mostbet на телефон [url=http://mostbet6013.ru/]http://mostbet6013.ru/[/url] .
The mobile casino needs work—spotty! mines juego
mostbet сайт [url=http://mostbet3022.ru]http://mostbet3022.ru[/url] .
мостбет казино [url=www.mostbet6013.ru]www.mostbet6013.ru[/url] .
mostbet uz [url=https://mostbet3022.ru]mostbet uz[/url] .
мостбет скачать на андроид [url=mostbet6013.ru]mostbet6013.ru[/url] .
мостбет узбекистан [url=http://mostbet3022.ru/]мостбет узбекистан[/url] .
Gucci fake designer bags
Drugs prescribing information. Effects of Drug Abuse.
can venlafaxine be taken at night
Best about medicine. Get information here.
мостбет скачать андроид [url=https://mostbet6013.ru/]https://mostbet6013.ru/[/url] .
мостбет скачать [url=www.mostbet7007.ru]www.mostbet7007.ru[/url] .
mostbet rasmiy sayt [url=https://mostbet3022.ru/]https://mostbet3022.ru/[/url] .
РедМетСплав предлагает широкий ассортимент качественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица товара подтверждена требуемыми документами, подтверждающими их соответствие стандартам. Опытная поддержка – то, чем мы гордимся – мы на связи, чтобы ответить на ваши вопросы по мере того как адаптировать решения под особенности вашего бизнеса.
Доверьте вашу потребность в редких металлах специалистам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наша продукция:
Лента титановая Р’Рў21Р› – РћРЎРў 1 90060-92 РљСЂСѓРі титановый Р’Рў21Р› – РћРЎРў 1 90060-92 представляет СЃРѕР±РѕР№ высококачественный РїСЂРѕРґСѓРєС‚, изготовленный РёР· титана, что обеспечивает ему отличные механические свойства Рё РєРѕСЂСЂРѕР·РёРѕРЅРЅСѓСЋ стойкость. Ртот РєСЂСѓРі РїРѕРґС…РѕРґРёС‚ для использования РІ различных отраслях, включая авиационную Рё автомобильную. Купить РљСЂСѓРі титановый Р’Рў21Р› – РћРЎРў 1 90060-92 РјРѕР¶РЅРѕ для реализации сложных инженерных решений Рё повышения долговечности изделий. Доступен РІ различных размерах Рё сертифицирован РІ соответствии СЃ установленными стандартами. Обладая высокой пластичностью, этот РєСЂСѓРі РїРѕРґС…РѕРґРёС‚ для дальнейшей обработки Рё применения РІ инновационных технологиях.
интернет домашний ростов
domashij-internet-rostov001.ru
подключить домашний интернет ростов
Discover Montenegro Kolasin Montenegro crystal clear sea, picturesque bays, mountain landscapes and ancient fortresses. The perfect destination for those looking for a combination of nature, history and relaxation. Detailed guides, recommendations, photos and route ideas.
SPA-салон в Москве https://uslugi.yandex.ru/profile/BonSpa-3013256 предлагает массаж, пилинг, обёртывания, уход за кожей, антистресс-программы и релакс-процедуры. Работают сертифицированные специалисты, используется премиум-косметика. Уютная атмосфера, индивидуальный подход и удобное расположение в городе.
[url=https://tournaments-casino.ru]tournaments-casino.ru[/url]
Турниры в онлайн-казино 2025: как участвовать с мобильного телефона
В 2025 году онлайн-казино предлагают множество захватывающих турниров с крупными призами. Если вы хотите испытать удачу и побороться за ценные награды, сделать это можно прямо с мобильного устройства. На нашем сайте собрана актуальная информация о самых ожидаемых турнирах, правилах участия и стратегиях для победы.
Популярные турниры 2025 года
В этом сезоне игроков ждут:
Слоты-баттлы – соревнования на самых популярных автоматах с прогрессивными джекпотами.
Покерные серии – турниры с гарантированными призовыми фондами.
Рулетка на время – скоростные раунды с дополнительными бонусами.
Ежедневные фрироллы – бесплатные турниры с реальными денежными призами.
Как участвовать с телефона?
Выберите надежное казино – на сайте представлен рейтинг лицензированных платформ с мобильной версией.
Скачайте приложение или играйте в браузере – большинство казино адаптированы под iOS и Android.
Зарегистрируйтесь и пополните счет – некоторые турниры требуют минимального депозита.
Найдите раздел “Турниры” – там указаны условия, расписание и призовые фонды.
Начните играть и следите за таблицей лидеров – чем выше ваша позиция, тем больше шансов на победу.
Советы для успешной игры
Изучите правила каждого турнира – иногда важны не только выигрыши, но и количество ставок.
Используйте бонусы – многие казино дают бесплатные спины или кэшбэк для участников.
Следите за обновлениями – новые турниры появляются регулярно.
Хотите узнать больше? Переходите по ссылке и читайте полный обзор турниров 2025 года с подробными инструкциями по участию.tournaments-casino.ru
mines game login
Обучитесь новой специальности, пройдите онлайн экспресс-курс!
https://xn--18-6kcdusowgbt1a4b.xn--p1ai/%D0%BA%D0%B0%D0%BA-%D0%B2%D1%8B%D0%B1%D1%80%D0%B0%D1%82%D1%8C-%D0%BF%D1%80%D0%BE%D1%84%D0%B5%D1%81%D1%81%D0%B8%D1%8E/
Celine fake designer bags
The online flow is wild—stay sharp! fortune ox
fake Bottega Veneta bag
Bottega Veneta replica designer bags
dragon versus tiger
https://t.me/bezdeper
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым текущие трудности превращаются в завтрашние успехи.
Наши товары:
Керамическая мишень Индиевого оксида, плоская форма
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наши товары:
Плоская мишень магниевая
DIOR fake designer bags
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наша продукция:
Гранулы силикона 4N
Chanel bag replica
DIOR replica designer bags
pinup casino [url=https://pinup-azerbaycan51.com/]pinup casino[/url] .
mostbet uz skachat [url=https://mostbet3023.ru/]https://mostbet3023.ru/[/url] .
betclic
pin-up online [url=http://www.pinup-azerbaycan6.com]pin-up online[/url] .
мос бет [url=http://mostbet7006.ru]мос бет[/url] .
razor returns online casino
мотбет [url=https://www.mostbet6039.ru]https://www.mostbet6039.ru[/url] .
Are online jackpots real?—skeptic! book of ra
раскрутка сайта москва [url=https://www.itechua.com/other/277983]раскрутка сайта москва[/url] .
Louis Vuitton fake designer bags
[url=https://tournaments-casino.ru]tournaments-casino.ru[/url]
Турниры в онлайн-казино 2025: как участвовать с мобильного телефона
В 2025 году онлайн-казино предлагают множество захватывающих турниров с крупными призами. Если вы хотите испытать удачу и побороться за ценные награды, сделать это можно прямо с мобильного устройства. На нашем сайте собрана актуальная информация о самых ожидаемых турнирах, правилах участия и стратегиях для победы.
Популярные турниры 2025 года
В этом сезоне игроков ждут:
Слоты-баттлы – соревнования на самых популярных автоматах с прогрессивными джекпотами.
Покерные серии – турниры с гарантированными призовыми фондами.
Рулетка на время – скоростные раунды с дополнительными бонусами.
Ежедневные фрироллы – бесплатные турниры с реальными денежными призами.
Как участвовать с телефона?
Выберите надежное казино – на сайте представлен рейтинг лицензированных платформ с мобильной версией.
Скачайте приложение или играйте в браузере – большинство казино адаптированы под iOS и Android.
Зарегистрируйтесь и пополните счет – некоторые турниры требуют минимального депозита.
Найдите раздел “Турниры” – там указаны условия, расписание и призовые фонды.
Начните играть и следите за таблицей лидеров – чем выше ваша позиция, тем больше шансов на победу.
Советы для успешной игры
Изучите правила каждого турнира – иногда важны не только выигрыши, но и количество ставок.
Используйте бонусы – многие казино дают бесплатные спины или кэшбэк для участников.
Следите за обновлениями – новые турниры появляются регулярно.
Хотите узнать больше? Переходите по ссылке и читайте полный обзор турниров 2025 года с подробными инструкциями по участию.tournaments-casino.ru
fake Louis Vuitton bag
fake Louis Vuitton bag
I love how you explained this so clearly. Subscribed for more!
buy lexapro prices
plataforma de jogos fortune tiger
домашний интернет тарифы ростов
domashij-internet-rostov002.ru
подключить интернет в квартиру ростов
Meds information. Generic Name.
can you buy prevacid for sale
Everything trends of drug. Get information here.
fake Bottega Veneta bag
how can i get doxycycline tablets
Online casinos should show odds—clear it! sugar rush
jogo.do tigre
pinup [url=http://pinup-azerbaycan51.com/]pinup[/url] .
plinko balls
мостбет мобильная версия скачать [url=https://www.mostbet6014.ru]https://www.mostbet6014.ru[/url] .
pin.up casino [url=https://pinup-azerbaycan6.com]pin.up casino[/url] .
Celine replica designer bags
аренда боксов для хранения вещей в московской области [url=https://www.hranim-veshi-msk24.ru]https://www.hranim-veshi-msk24.ru[/url] .
раскрутка сайтов москва [url=https://itechua.com/other/277983/]раскрутка сайтов москва[/url] .
Ищете выгодные предложения в агропромышленности? Добро пожаловать на АгроПартнер.су новые агро объявления
Производитель прозрачных силиконовых скатертей гибкое стекло на современном оборудовании с доставкой по всей России https://dj-shop.ru/
https://300.ya.ru/TNSboyKz/ Сайты для покупки скинов Дота 2 по выгодной цене с банковской карты
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наши товары:
Ультрачистые гранулы германия
Meds prescribing information. What side effects?
order cheap minocycline online
All news about meds. Read here.
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – идеальный вариант для вас.
Наша продукция:
Медная муфта 23х1.8 мм литая с концом под пайку с наружной конической модифицированной резьбой Rk 1 4х7 мм М1ф ГОСТ Р52949-2008 Купить медные муфты с конической резьбой в России. Надежное соединение для водопроводных и отопительных систем. Прочные медные муфты предлагаются в различных размерах. Выберите оптимальный вариант для ваших нужд. Гарантированная надежность и простота установки.
The visuals online stun—eye pop! plinko
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
Наши товары:
Латунная полоса 6×250 РјРј ЛМц58-2 ГОСТ 931-90 Покупайте латунную полосу различных размеров Рё толщин РЅР° сайте Редметсплав.СЂС„. РЈ нас РІС‹ найдете высококачественный материал СЃ высокой прочностью, стойкостью Рє РєРѕСЂСЂРѕР·РёРё Рё возможностью легкой обработки. Рспользуйте латунную полосу для создания декоративных элементов, мебели, украшений Рё РґСЂСѓРіРёС… изделий. Доступны различные размеры Рё толщины.
how to buy dapsone price
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с высоким уровнем сервиса.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – наилучшее решение для вашего бизнеса.
Наши товары:
Медный двухраструбный отвод 45 градусов под пайку 80х68х1.8 мм 35.5х38.5 мм твердая пайка Cu-DHP ГОСТ Р52922-2008 Приобретите высококачественные Медные отводы 45 градусов под пайку от компании Редметсплав.рф и улучшите вашу трубопроводную систему. Прочные, долговечные и устойчивые к коррозии отводы обеспечат надежное соединение под нужным углом, обеспечивая оптимальное распределение потока и высокую производительность вашей системы.
Prada replica designer bags
Successful Strategies for Online Strategy Game Play
Guide to Playing Online Strategy Games Successfully
Engaging with complex tactical experiences demands more than mere enthusiasm; it calls for a blend of analytical thinking and calculated decision-making. Players who excel not only familiarize themselves with the mechanics but also adopt a critical mindset that allows them to adapt quickly. Each choice carries weight, influencing both immediate outcomes and longer-term developments within the gameplay. Understanding the nuances can make a considerable difference between victory and defeat.
Focusing on resource management stands at https://plinkoreview.com core of many competitive encounters. Players should prioritize optimizing building and unit production, ensuring that every decision aligns with their overarching objectives. For instance, early-game investments shaping the economy can set the stage for a flourishing military presence or technological advancement later on. Monitoring opponents’ actions can offer valuable insights into their strategies, allowing for preemptive measures and counterplays.
Collaborative elements further enrich the experience in multiplayer settings. Forming alliances can provide a strategic edge, as sharing resources and coordinating attacks with other players amplifies overall strength. However, one must remain vigilant; trust is often transient. Identifying potential threats and adapting alliances as circumstances shift can be the key to maintaining dominance in dynamic confrontations.
Mastering Resource Management for Optimal Gameplay
In competitive scenarios, managing assets becomes a cornerstone of advancement. Players should prioritize gathering and allocating resources such as currency, materials, and manpower. Understanding each resource’s value is paramount; some may have limited availability while others are abundant but crucial for specific upgrades.
A well-crafted plan includes diversifying resource types. Rather than focusing solely on one, balancing the collection of various assets ensures that players can adapt to different situations. For example, if your opponents are stockpiling one resource, consider shifting attention to acquire what they lack, gaining a tactical advantage.
Implementing a system for prioritizing projects can significantly enhance efficiency. Rank tasks based on immediate necessity versus long-term benefits. For instance, while fortifying defenses may seem critical, investing in economy-building structures could yield greater returns over time.
Monitoring resource consumption is another key aspect. Regularly examine expenditure patterns to identify wasteful practices. If certain units or buildings consume disproportionate amounts, reassess their utility and impact on overall performance.
Utilizing trade can serve to bolster resource acquisition. Engaging with other players or factions to exchange goods ensures that you are not relying solely on your own production capabilities. Establishing alliances can lead to mutual benefits, enhancing growth potential for all parties involved.
Lastly, staying informed about updates and game mechanics allows for better alignment with current trends. Changes to resource values or availability can influence how players approach their management tactics. Keeping abreast of these shifts enables adaptability, which is vital for maintaining a competitive edge.
Effective Team Tactics for Competitive Matchups
In high-stakes encounters, coordinated efforts among teammates lead to significant advantages. To achieve success, implement the following techniques:
1. Role Assignment: Clearly define individual responsibilities based on strengths. Each player should excel in specific roles, such as offense, defense, or support, to ensure smooth collaboration. A dedicated scout can gather critical intelligence, while strong defenders secure territory.
2. Communication Protocols: Establish a structured communication system that includes callouts for enemy movements and strategic shifts. Use voice chat or ping systems effectively, ensuring all teammates can respond swiftly to unforeseen circumstances.
3. Flanking Maneuvers: Utilize flanking tactics to catch opponents off guard. Coordinate with teammates to attack from multiple angles, creating confusion and making it difficult for the enemy to concentrate fire effectively.
4. Resource Management: Monitor and share critical resources, such as ammunition and health packs. Designate a leader to oversee resource distribution and ensure all players have what they need to engage in combat.
5. Building Alliances: Form temporary partnerships with other teams when beneficial. Gaining temporary allies can shift the balance of power, especially in free-for-all scenarios. Communicate the objective clearly to avoid betrayal.
6. Adaptability: Remain flexible in tactics. If an initial plan fails, quickly reassess the situation and pivot to alternative approaches. Encourage open discussions among team members to brainstorm new tactics on the fly.
7. Practice Scenarios: Regularly engage in drills that simulate various combat situations. This preparation allows players to develop instincts and deepen their understanding of each player’s typical responses, enhancing overall team efficiency.
8. Analyze Opponents: Study past match replays to identify opponents’ patterns and weaknesses. This analysis helps in crafting counter-tactics tailored to exploit specific flaws in the enemy team’s formation.
Utilizing these methods can substantially enhance team performance during competitive match situations, allowing for greater control and execution during heated clashes.
Discover Montenegro holidays Igalo Montenegro crystal clear sea, picturesque bays, mountain landscapes and ancient fortresses. The perfect destination for those looking for a combination of nature, history and relaxation. Detailed guides, recommendations, photos and route ideas.
Serialexpress приобрести увлекательные сериалы на dvd предлагает. Здесь можно найти уникальные диски. Вы будете в восторге от покупки, потому что качество сериалов безупречное. Товары доступны по приемлемой стоимости и отлично упакованы. Ищете магазин сериалов? Serialexpress.ru – портал, где информация об оплате предоставлена, советуем с ней ознакомиться вам. Мы накопительную систему скидок предлагаем. Быть постоянным клиентом – выгодно. Гарантируем оперативную доставку. При появлении каких-либо вопросов, пишите нам на электронную почту. Мы всегда рады вам!
подключить домашний интернет в ростове
domashij-internet-rostov003.ru
интернет провайдеры ростов
Chanel bag replica
РедМетСплав предлагает внушительный каталог высококачественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их качество. Превосходное обслуживание – наш стандарт – мы на связи, чтобы ответить на ваши вопросы и предоставлять решения под особенности вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
поставляемая продукция:
Лента магниевая MgAl8Zn (Alloy 23) – ISO 3116 РљСЂСѓРі магниевый MgAl8Zn (Alloy 23) – ISO 3116 – это высококачественный материал, который идеально РїРѕРґС…РѕРґРёС‚ для различных промышленных применений. Обладая отличными механическими свойствами Рё высокой РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью, данный РєСЂСѓРі прекрасно РїРѕРґС…РѕРґРёС‚ для машиностроения Рё авиации. Его легкий вес Рё прочность делают его незаменимым РІ производстве. Приобрести этот РїСЂРѕРґСѓРєС‚ РјРѕР¶РЅРѕ РїСЂСЏРјРѕ Сѓ нас, так как РјС‹ гарантируем оптимальные условия поставки Рё доступные цены. РќРµ упустите шанс купить РљСЂСѓРі магниевый MgAl8Zn (Alloy 23) – ISO 3116 Рё получите надежное решение для вашего бизнеса. Отличный выбор для всех, кто ценит качество Рё эффективность.
РедМетСплав предлагает широкий ассортимент отборных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их происхождение. Превосходное обслуживание – наш стандарт – мы на связи, чтобы улаживать ваши вопросы по мере того как находить ответы под специфику вашего бизнеса.
Доверьте вашу потребность в редких металлах специалистам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наша продукция:
Лист титановый EL Лента титановая EL – это высококачественный РїСЂРѕРґСѓРєС‚, обеспечивающий отличные механические свойства Рё РєРѕСЂСЂРѕР·РёРѕРЅРЅСѓСЋ стойкость. Рспользуется РІ медицинской, аэрокосмической Рё нефтегазовой отраслях, РіРґРµ требуется надежность Рё прочность. Лента обладает легким весом Рё высокой прочностью, что делает ее идеальной для различных конструкций. Если РІС‹ ищете надежный материал для вашего проекта, вам стоит рассмотреть возможность купить Лента титановая EL. Доступна РІ различных размерах Рё толщине, что позволяет выбрать оптимальный вариант РІ зависимости РѕС‚ ваших потребностей.
мостбет скачать казино [url=https://mostbet6040.ru/]https://mostbet6040.ru/[/url] .
подарочные карты онлайн покупка гифт карт
РедМетСплав предлагает внушительный каталог высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы обеспечиваем быстрое выполнение вашего заказа.
Каждая единица товара подтверждена всеми необходимыми документами, подтверждающими их происхождение. Опытная поддержка – наш стандарт – мы на связи, чтобы улаживать ваши вопросы а также находить ответы под особенности вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в множестве наших преимуществ
поставляемая продукция:
Лента кобальтовая РҐРљ62Рњ6Р› – ГОСТ Р 51395-99 Лента кобальтовая РҐРљ62Рњ6Р› – ГОСТ Р 51395-99 предназначена для производства высокопрочных изделий, необходимых РІ различных отраслях, включая машиностроение Рё инструментальное производство. Основные характеристики включают отличные механические свойства Рё стойкость Рє температурным воздействиям. Рто делает данную ленту идеальным выбором для тяжелых условий эксплуатации. Выбирая ленту, РІС‹ гарантируете надежность Рё долговечность продукции. РќРµ упустите возможность улучшить качество СЃРІРѕРёС… изделий! Купить Лента кобальтовая РҐРљ62Рњ6Р› – ГОСТ Р 51395-99 РјРѕР¶РЅРѕ РїСЂСЏРјРѕ сейчас Рё обеспечить себе высокую производительность.
Главным достоинством Апостолиди является предоставление возможности для актуальной информации посетителям. Мы простым языком объясняем сложные финансовые вопросы. Благодаря постоянным обновлениям вы будете в курсе основных событий. https://apostolidi.ru – сайт, который сейчас пользуются невероятной популярностью. Тут имеется поиск, воспользуйтесь им. Апостолиди дает возможность находить полезные советы, исследовать уникальные материалы и получать знания от экспертов. Добро пожаловать на наш портал!
I wish online paid swift—drag off! aviator game
Pills information. Generic Name.
can you buy cheap levitra without rx
All information about meds. Read information here.
Купить диплом любого ВУЗа!
Мы можем предложить документы институтов, которые расположены в любом регионе России. Документы делаются на бумаге самого высшего качества: [url=http://rabota.newrba.ru/employer/aurus-diploms/]rabota.newrba.ru/employer/aurus-diploms[/url]
мостбет официальный сайт [url=http://mostbet7007.ru/]http://mostbet7007.ru/[/url] .
Приобрести диплом об образовании!
Приобрести диплом института по невысокой стоимости возможно, обратившись к надежной специализированной фирме. Приобрести диплом: [url=http://fastdiploms.com/kupit-diplom-vuza-otzivi-realnix-klientov/]fastdiploms.com/kupit-diplom-vuza-otzivi-realnix-klientov[/url]
Celine fake designer bags
can i order exforge for sale
pin.up casino [url=http://pinup-azerbaycan6.com/]pin.up casino[/url] .
pin ap [url=http://www.pinup-azerbaycan51.com]pin ap[/url] .
Декорируйте дом с помощью римских штор на заказ
римские шторы на заказ [url=https://rimskie-shtory-na-zakaz.ru/]римские шторы на заказ[/url] .
Gucci bag replica
Сшить шторы на заказ по индивидуальному проекту, лучшие цены.
Качественные шторы на заказ, с гарантией качества.
Изготовление штор на заказ, под ваш интерьер.
Шторы на заказ с доставкой, высокое качество материалов.
Пошив штор на заказ для кухни, с индивидуальным подходом.
Индивидуальный дизайн штор, быстро и качественно.
Пошив штор для нестандартных окон, по желанию.
Эксклюзивные шторы на заказ, по вашему желанию.
Классические шторы на заказ, по вашему проекту.
Шторы на заказ с учетом ваших пожеланий, от ведущих мастеров.
Изготовление штор на заказ на любой вкус, под любой интерьер.
Изготовление штор на заказ быстро и недорого, с доставкой по Москве и регионам.
Элегантные шторы на заказ, под ваш бюджет.
Премиум шторы на заказ, по вашему проекту.
Пошив штор по индивидуальному дизайну, от профессиональных мастеров.
Пошив штор на заказ с индивидуальным подходом, под любой стиль.
сшить шторы на заказ [url=https://moscow-shtory-zakaz.ru/]сшить шторы на заказ[/url] . Прокарниз
Биржа профилей социальных сетей (Facebook, Instagram, TikTok, Telegram и др.) является эффективный ресурс для тех, кто занимается цифровому маркетингу, арбитражу и продвижению в соцсетях. Такие площадки помогают оперативно решать проблемы банами аккаунтов, необходимостью масштабирования а также избежать долгого и не всегда успешного самостоятельного фарма. Основные плюсы бирж вроде birzha-akkauntov-online.ru: обеспечение надежности покупки и продажи благодаря систему гаранта, экономия времени, большой выбор профилей под разные цели и легкость масштабирования. Однако, для стабильной работы, необходимо пользоваться надежные сервисы с прозрачными правилами, обязательно использовать антидетект браузеры и надежные прокси для каждого аккаунта а также не пренебрегать этапу адаптации приобретенных профилей перед активным использованием.
hot hot fruit big win
Celine fake designer bags
раскрутка сайтов москва [url=http://itechua.com/other/277983/]раскрутка сайтов москва[/url] .
fortune mouse gratis demo
where can i buy lanoxin without rx
plinko casino
Bottega Veneta replica designer bags
most bet uz [url=http://mostbet3023.ru/]http://mostbet3023.ru/[/url] .
I lost time online—swift pass! legacy of dead
Доставили в другой город – все дошло в целости!
купить цветы в томске
Pills information for patients. Short-Term Effects.
can i order generic minocycline no prescription
Actual about meds. Get now.
[url=https://install-champion-casino.ru]install-champion-casino.ru[/url]
Если вам интересно ознакомиться с инструкциями по установке приложений на мобильные устройства, мы можем предложить общий гайд.
Как установить приложения на Android и iOS
Установка мобильных приложений зависит от операционной системы вашего устройства.
Для Android:
Откройте файл APK — если приложение недоступно в Google Play, его можно скачать через официальный сайт.
Разрешите установку из неизвестных источников — зайдите в Настройки > Безопасность и активируйте соответствующую опцию.
Запустите установку и следуйте инструкциям.
Для iOS:
Скачайте приложение из App Store — большинство сервисов доступны через официальный магазин.
Используйте TestFlight или корпоративный сертификат — если приложение не опубликовано в App Store.
Разрешите установку — в настройках iPhone перейдите в раздел VPN и управление устройствами и подтвердите доверие к разработчику.
Если вас интересует конкретное приложение, проверьте его официальный сайт или магазин приложений. Но помните о безопасности: скачивайте ПО только из проверенных источников, чтобы избежать мошенничества.
Хотите узнать больше? Читайте дополнительные инструкции на сайте по ссылке ниже.install-champion-casino.ru
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
Наша продукция:
Медный тройник РїРѕРґ пайку СЃ направленным отводом 27.4С…23С…0.9 РјРј 18.4С…20.4 РјРј твердая пайка Рњ2СЂ ГОСТ 32590-2013 Купить качественные медные тройники РїРѕРґ пайку для надежных Рё долговечных соединений РІ трубопроводных системах. Разнообразие размеров Рё конфигураций, высокая теплопроводность Рё устойчивость Рє РєРѕСЂСЂРѕР·РёРё. Простая установка Рё надежность соединения. Рдеальное решение для различных проектов. Редметсплав.СЂС„
Bottega Veneta bag replica
DIOR fake designer bags
auto transport carriers car shipping service
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым текущие трудности превращаются в завтрашние возможности.
Наша продукция:
Металлическая мишень для распыления железа
fishin frenzy slot machine
провайдеры интернета омск
domashij-internet-volgograd001.ru
домашний интернет тарифы
Такой магазин аккаунтов, как birzha-akkauntov-online.ru – эффективный инструмент вебмастеров.
Подобные ресурсы помогают без лишних усилий решать задачи с блокировками аккаунтов и минуя самостоятельного фарма.
Основные плюсы подобных сервисов:
– Обеспечение надежности покупок через систему гаранта
– Значительная экономия времени и усилий
– Широкий выбор аккаунтов для любых задач — от дешевых до трастовых с историей
– Легкость работы с крупными объемами
Чтобы получить максимальную отдачу, рекомендуется:
– Пользоваться авторитетными платформами с хорошими отзывами
– Использовать надежные прокси (резидентные) и инструменты анонимизации
– Не пренебрегать аккуратной подготовке перед запуском рекламы
mostbet casino [url=https://www.mostbet6039.ru]https://www.mostbet6039.ru[/url] .
Мы изготавливаем дипломы любой профессии по приятным ценам. Дипломы производятся на фирменных бланках Быстро купить диплом любого ВУЗа [url=http://diplomservis.com/]diplomservis.com[/url]
Где заказать диплом по актуальной специальности?
Купить диплом университета по доступной цене можно, обращаясь к надежной специализированной компании.: [url=http://magazin-diplomov.ru/]magazin-diplomov.ru[/url]
plinko app review
Celine bag replica
Playing online bingo is a chill way to unwind! plinko
РедМетСплав предлагает внушительный каталог качественных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их качество. Опытная поддержка – наш стандарт – мы на связи, чтобы разрешать ваши вопросы по мере того как адаптировать решения под требования вашего бизнеса.
Доверьте вашу потребность в редких металлах специалистам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наша продукция:
Полоса висмутовая Galinstan – Geratherm Medical AG Полоса висмутовая Galinstan – Geratherm Medical AG представляет СЃРѕР±РѕР№ высококачественный медицинский инструмент, созданный для точной измерительной практики. Производитель использует передовые технологии РІ разработке этого продукта, что гарантирует его надежность Рё точность. Полоса РїРѕРґС…РѕРґРёС‚ для различных медицинских применений, обеспечивая быстрый Рё удобный процесс измерения.Если РІС‹ хотите повысить качество СЃРІРѕРёС… измерений, вам стоит купить Полоса висмутовая Galinstan – Geratherm Medical AG. Ртот РїСЂРѕРґСѓРєС‚ станет идеальным дополнением Рє вашему медицинскому оборудованию, позволяя достигать высоких результатов РІ работе.
Louis Vuitton replica designer bags
Где заказать [b]диплом[/b] по нужной специальности?
Полученный диплом с необходимыми печатями и подписями отвечает стандартам, никто не сможет отличить его от оригинала – даже со специально предназначенным оборудованием. Не откладывайте личные цели на потом, реализуйте их с нашей помощью – отправляйте быструю заявку на диплом уже сегодня! Диплом о высшем образовании – запросто! [url=http://gertsyhr.com/employer/premialnie-diplom-24/]gertsyhr.com/employer/premialnie-diplom-24[/url]
how to buy generic valtrex tablets
Приобрести диплом о высшем образовании. Приобретение документа о высшем образовании через проверенную и надежную компанию дарит ряд преимуществ для покупателя. Данное решение дает возможность сберечь как длительное время, так и серьезные финансовые средства. [url=http://recruitment.econet.co.zw/employer/eonline-diploma/]recruitment.econet.co.zw/employer/eonline-diploma[/url]
Louis Vuitton bag replica
order generic rulide for sale
Приобрести диплом под заказ вы сможете используя сайт компании. [url=http://newsbizlife.ru/kachestvennyie-diplomyi-na-zakaz/]newsbizlife.ru/kachestvennyie-diplomyi-na-zakaz[/url]
Drug prescribing information. Brand names.
can you buy generic phenytoin online
All what you want to know about medicine. Get here.
app plinko
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние успехи.
Наши товары:
Металлическая мишень для распыления
aviator bet
fake Bottega Veneta bag
[url=https://install-champion-casino.ru]install-champion-casino.ru[/url]
Если вам интересно ознакомиться с инструкциями по установке приложений на мобильные устройства, мы можем предложить общий гайд.
Как установить приложения на Android и iOS
Установка мобильных приложений зависит от операционной системы вашего устройства.
Для Android:
Откройте файл APK — если приложение недоступно в Google Play, его можно скачать через официальный сайт.
Разрешите установку из неизвестных источников — зайдите в Настройки > Безопасность и активируйте соответствующую опцию.
Запустите установку и следуйте инструкциям.
Для iOS:
Скачайте приложение из App Store — большинство сервисов доступны через официальный магазин.
Используйте TestFlight или корпоративный сертификат — если приложение не опубликовано в App Store.
Разрешите установку — в настройках iPhone перейдите в раздел VPN и управление устройствами и подтвердите доверие к разработчику.
Если вас интересует конкретное приложение, проверьте его официальный сайт или магазин приложений. Но помните о безопасности: скачивайте ПО только из проверенных источников, чтобы избежать мошенничества.
Хотите узнать больше? Читайте дополнительные инструкции на сайте по ссылке ниже.install-champion-casino.ru
Где купить диплом специалиста?
Мы готовы предложить дипломы любых профессий по доступным тарифам. Для нас важно, чтобы документы были доступными для большого количества наших граждан. Быстро купить диплом любого института [url=http://diplomc-v-ufe.ru/ofitsialnij-diplom-o-visshem-obrazovanii-zaregistrirovan-v-reestre/]diplomc-v-ufe.ru/ofitsialnij-diplom-o-visshem-obrazovanii-zaregistrirovan-v-reestre/[/url]
Celine fake designer bags
мостбет официальный сайт [url=mostbet6040.ru]mostbet6040.ru[/url] .
мрстбет [url=https://www.mostbet6014.ru]https://www.mostbet6014.ru[/url] .
Привет!
Мы изготавливаем дипломы любой профессии по приятным ценам. Цена может зависеть от выбранной специальности, года выпуска и образовательного учреждения: [url=http://diploman-russian.com/]diploman-russian.com/[/url]
Приобрести диплом института!
Мы оказываем услуги по продаже документов об окончании любых ВУЗов Российской Федерации. Документы производят на подлинных бланках. [url=http://13.flybb.ru/viewtopic.php?f=20&t=1572/]13.flybb.ru/viewtopic.php?f=20&t=1572[/url]
I hit a blackjack streak—good day! vipzino
Платформа перепродажа аккаунтов – эффективный ресурс вебмастеров.
Такие площадки позволяют без лишних усилий решать задачи с блокировками аккаунтов и минуя самостоятельного фарма.
Ключевые плюсы таких платформ:
– Обеспечение надежности сделок через эскроу-механизм
– Существенная экономия времени и усилий
– Большой ассортимент профилей для разных целей — от авторегов до прогретых с историей
– Легкость работы с крупными объемами
Чтобы обеспечить стабильную работу, необходимо:
– Выбирать только проверенные платформами с прозрачными правилами
– Использовать надежные прокси (мобильные) и антидетект-браузеры с уникальными фингерпринтами
– Не пренебрегать прогреву новых аккаунтов
Купить диплом института по выгодной цене вы можете, обратившись к надежной специализированной компании. Мы можем предложить документы высших учебных заведений, которые расположены на территории всей России. [url=http://vastudecore.com/kupit-diplom-bystro-i-prosto-133/]vastudecore.com/kupit-diplom-bystro-i-prosto-133[/url]
vai de bet oficial
мостбет кыргызстан [url=http://mostbet7007.ru]мостбет кыргызстан[/url] .
Fendi bag replica
Заказать документ о получении высшего образования вы сможете в нашей компании в Москве. Мы предлагаем документы об окончании любых университетов РФ. Вы получите необходимый диплом по любой специальности, любого года выпуска, в том числе документы старого образца. Даем гарантию, что при проверке документов работодателем, каких-либо подозрений не появится. [url=http://diploml-174.ru/kupit-diplom-s-zaneseniem-bistro-i-nadezhno-5/]diploml-174.ru/kupit-diplom-s-zaneseniem-bistro-i-nadezhno-5/[/url]
Medicament information. Long-Term Effects.
where can i get cheap chlorpromazine prices
All news about meds. Get now.
домашний интернет в волгограде
domashij-internet-volgograd002.ru
подключить интернет омск
мостбет скачать андроид [url=http://mostbet6040.ru/]http://mostbet6040.ru/[/url] .
Заказать диплом о высшем образовании !
Покупка диплома университета России у нас – надежный процесс, поскольку документ будет заноситься в государственный реестр. Приобрести диплом об образовании [url=http://kupit-diplom24.com/kupit-diplom-o-visshem-obrazovanii-bistro-i-legko-5/]kupit-diplom24.com/kupit-diplom-o-visshem-obrazovanii-bistro-i-legko-5[/url]
fake Bottega Veneta bag
Всегда думал что купить диплом о высшем образовании это миф и нереально, но все оказалось не так, изначально искал информацию про: купить диплом высшем образовании нижний новгород, купить диплом колледжа в нижнем новгороде, купить диплом нового образца в нижнем новгороде, купить диплом старого образца в нижнем новгороде, где купить диплом в нижнем тагиле, потом про дипломы вузов, подробнее здесь [url=http://diplomybox.com/diplom-psikhologa/]diplomybox.com/diplom-psikhologa[/url]
can i order generic artane without insurance
This post really resonated with me. Appreciate your honesty and insights.
Chanel bag replica
plinko app erfahrungen
[url=https://sukaaa-casino-slots.ru]sukaaa-casino-slots.ru[/url]
Обзор игровой платформы Sukaa 2025
В 2025 году Sukaa остается одной из популярных международных игровых платформ, привлекающей внимание пользователей из разных стран. На нашем сайте представлен детальный обзор особенностей данного ресурса.
Основные характеристики:
Лицензия: международная (Кюрасао)
Валюта: поддерживаются рублевые транзакции
Игровой софт: представлены слоты, настольные игры, live-дилеры
Мобильная версия: адаптирована под iOS и Android
Особенности платформы:
Бонусная программа для новых пользователей
Регулярные турниры с призовыми фондами
Система лояльности для постоянных клиентов
Важно учитывать:
Платформа не имеет российской лицензии
Доступ может быть ограничен интернет-провайдерами
Рекомендуется проверять актуальность информации на официальном сайте
Альтернативы:
Для российских пользователей доступны легальные варианты:
Официальные лотереи (Столото)
Социальные игровые приложения
Напоминание: Азартные игры могут вызывать зависимость. Играйте ответственно. 18+
Полную информацию о платформе Sukaa вы найдете на сайте по ссылке.sukaaa-casino-slots.ru
Prada replica designer bags
The live games online rock—fan love! plinko app
Мы изготавливаем дипломы любой профессии по приятным тарифам.
Вы приобретаете документ в надежной и проверенной временем компании. Приобрести диплом о высшем образовании– [url=http://sensualmarketplace.com/read-blog/13318/]sensualmarketplace.com/read-blog/13318[/url]
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – идеальный вариант для вас.
Наши товары:
Дюралевый швеллер 440218 26С…40С…8С…4С…4 РјРј Р”1 ГОСТ 4784-97 Купить дюралевый швеллер РѕС‚ производителя. Прочный Рё легкий материал для различных областей строительства. РЁРёСЂРѕРєРёР№ выбор размеров Рё профилей. Устойчивость Рє РєРѕСЂСЂРѕР·РёРё Рё надежность конструкций. Рдеальное решение для авиации, судостроения, автомобилестроения, производства спортивного оборудования Рё фармакологических предприятий.
скачать mostbet uz [url=www.mostbet3023.ru]www.mostbet3023.ru[/url] .
Качественный пошив штор на заказ
пошив штор на заказ [url=https://shtory-na-zakaz-moscow.ru/]пошив штор на заказ[/url] .
Bomonti su kaçak tespiti Gelişmiş elektronik kaçak tespiti, araştırmayı minimum düzeyde invaziv yapar. http://bird-dresden.de/?p=3963
No pgwin, Você Começa com 100$ de Bônus Exclusivo!
Платформа покупка аккаунтов – проверенное решение для SMM-щиков, работающих с соцсетями.
Они позволяют оперативно обходить ограничения соцсетей и минуя самостоятельного фарма.
Ключевые преимущества подобных сервисов:
– Обеспечение безопасности сделок через эскроу-механизм
– Существенная экономия времени и усилий
– Широкий выбор аккаунтов для любых задач — от авторегов до прогретых с историей
– Легкость работы с крупными объемами
Чтобы обеспечить стабильную работу, необходимо:
– Выбирать только проверенные платформами с хорошими отзывами
– Использовать чистые прокси (мобильные) и антидетект-браузеры с уникальными фингерпринтами
– Уделять внимание аккуратной подготовке перед запуском рекламы
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Обладая обширной линейкой редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Мишень рутения 3N5
can you buy lipitor pills
Всех приветствую!
Для некоторых людей, купить [b]диплом[/b] ВУЗа – это острая необходимость, шанс получить выгодную работу. Но для кого-то – это понятное желание не терять массу времени на учебу в ВУЗе. Что бы ни толкнуло вас на это решение, мы готовы помочь. Оперативно, профессионально и недорого изготовим документ любого года выпуска на подлинных бланках с реальными подписями и печатями.
Основная причина, почему многие прибегают к покупке документа, – получить определенную должность. Например, знания и опыт дают возможность кандидату устроиться на желаемую работу, однако подтверждения квалификации не имеется. При условии, что для работодателя важно присутствие “корочек”, риск потерять хорошее место довольно высокий.
Приобрести документ о получении высшего образования вы имеете возможность у нас в столице. Мы предлагаем документы об окончании любых университетов России. Вы получите необходимый диплом по любой специальности, любого года выпуска, в том числе документы старого образца. Гарантируем, что при проверке документа работодателем, каких-либо подозрений не возникнет.
Факторов, которые вынуждают купить диплом ВУЗа много. Кому-то очень срочно нужна работа, а значит, необходимо произвести хорошее впечатление на начальника во время собеседования. Другие задумали попасть в престижную компанию, для того, чтобы повысить свой статус в социуме и в дальнейшем начать свой бизнес. Чтобы не терять множество времени, а сразу начать успешную карьеру, применяя имеющиеся навыки, можно приобрести диплом в интернете. Вы сможете стать полезным в обществе, получите денежную стабильность быстро и просто- [url=http://diplomanrussians.com/]купить диплом о среднем образовании[/url]
Drugs information sheet. What side effects?
amoxicillin verfallsdatum
All news about pills. Get now.
Chanel bag replica
Лучшие шторы для уютного загородного жилья
шторы в загородном доме [url=https://zagorodshtory.ru/]шторы в загородном доме[/url] .Ткацкий
мостбет вход через соцсети [url=http://mostbet6041.ru/]http://mostbet6041.ru/[/url] .
мостбет авиатор [url=https://mostbet6042.ru/]https://mostbet6042.ru/[/url] .
The Race Up Aviator game has been gaining traction on Reddit, where players share tips, big wins, and thrilling near-misses. Many users are discussing strategies and comparing experiences, making it a hot topic in online casino communities Race Up Aviator
Скатерть из силикона от производителя является абсолютно безопасной. Она не испаряет токсичных соединений https://dj-shop.ru/
мосбет казино [url=https://mostbet6039.ru/]https://mostbet6039.ru/[/url] .
Prada fake designer bags
РедМетСплав предлагает широкий ассортимент высококачественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до масштабных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица продукции подтверждена соответствующими документами, подтверждающими их соответствие стандартам. Опытная поддержка – то, чем мы гордимся – мы на связи, чтобы ответить на ваши вопросы по мере того как находить ответы под требования вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
Наши товары:
Лист висмутовый CAC904C Лист висмутовый CAC904C – это высококачественный материал, идеально подходящий для использования РІ различных областях, включая электронику Рё ювелирные изделия. Ртот лист характеризуется отличной РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью Рё малой плотностью, что делает его идеальным выбором для лёгких конструкций. Наши клиенты высоко оценивают его стабильность РІ сложных условиях эксплуатации. РќРµ упустите возможность купить Лист висмутовый CAC904C Рё улучшить качество ваших проектов. Рзучите преимущества этого материала Рё воспользуйтесь отличным предложением СѓР¶Рµ сегодня!
Fendi fake designer bags
Uğurmumcu su kaçak tespiti Kaçak tespiti, binanızın değerini korumaya yardımcı olur. https://bnplumbingservice.com/?p=217
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – наилучшее решение для вашего бизнеса.
Наши товары:
Рнструментальная круглая РїРѕРєРѕРІРєР° 165 РјРј 11РҐ4Р’2РњР¤3РЎ2 ГОСТ 1133-71 РЁРёСЂРѕРєРёР№ выбор круглых РїРѕРєРѕРІРѕРє для профессиональной обработки металла. Рнструментальная круглая РїРѕРєРѕРІРєР° высочайшего качества СЃ разнообразием размеров Рё форм. Повышенная прочность, износостойкость Рё превосходное качество изготовления. Обеспечивает отличное сцепление СЃ материалом Рё эффективную работу оборудования. Покупайте РїСЂСЏРјРѕ сейчас!
I lost big online—reset time! plinko
ship car from dealership dealer auto transport
подарочная карта через интернет интернет подарочная карта
jogos vai de bet
fake Gucci bag
домашний интернет подключить омск
domashij-internet-volgograd003.ru
интернет домашний омск
Haznedar su kaçak tespiti Beylikdüzü’nde su kaçağı sorunumuz vardı, çok memnun kaldık, öneriyorum. https://midiario.com.mx/read-blog/8450_uskudar-su-tesisatcisi.html?mode=day
[url=https://sukaaa-casino-slots.ru]sukaaa-casino-slots.ru[/url]
Обзор игровой платформы Sukaa 2025
В 2025 году Sukaa остается одной из популярных международных игровых платформ, привлекающей внимание пользователей из разных стран. На нашем сайте представлен детальный обзор особенностей данного ресурса.
Основные характеристики:
Лицензия: международная (Кюрасао)
Валюта: поддерживаются рублевые транзакции
Игровой софт: представлены слоты, настольные игры, live-дилеры
Мобильная версия: адаптирована под iOS и Android
Особенности платформы:
Бонусная программа для новых пользователей
Регулярные турниры с призовыми фондами
Система лояльности для постоянных клиентов
Важно учитывать:
Платформа не имеет российской лицензии
Доступ может быть ограничен интернет-провайдерами
Рекомендуется проверять актуальность информации на официальном сайте
Альтернативы:
Для российских пользователей доступны легальные варианты:
Официальные лотереи (Столото)
Социальные игровые приложения
Напоминание: Азартные игры могут вызывать зависимость. Играйте ответственно. 18+
Полную информацию о платформе Sukaa вы найдете на сайте по ссылке.sukaaa-casino-slots.ru
Koza su kaçak tespiti Profesyonel hizmetler, garanti ve güvenlik sunar. https://www.studiodentisticofraietta.it/author/kacak/
Заказать диплом любого университета!
Мы можем предложить документы ВУЗов, которые находятся на территории всей РФ.
[url=http://freediplom.com/kupit-podlinnij-diplom-s-zaneseniem-v-reestr-6/]freediplom.com/kupit-podlinnij-diplom-s-zaneseniem-v-reestr-6/[/url]
Bottega Veneta replica designer bags
Prada bag replica
Bottega Veneta replica designer bags
Sefaköy su kaçağı tespiti Sürekli su basıncı düşüşleri gizli kaçakların habercisi olabilir. http://sat.poznan.pl/?p=2548
Drug prescribing information. Brand names.
where to buy generic singulair without dr prescription
Best about drugs. Get here.
can i order urticaria without a prescription
Bottega Veneta fake designer bags
РедМетСплав предлагает обширный выбор отборных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до масштабных поставок, мы обеспечиваем оперативное исполнение вашего заказа.
Каждая единица изделия подтверждена соответствующими документами, подтверждающими их происхождение. Дружелюбная помощь – наша визитная карточка – мы на связи, чтобы ответить на ваши вопросы а также предоставлять решения под специфику вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
Наши товары:
Порошок титановый EL1 Рзделия РёР· титана EL1 представляют СЃРѕР±РѕР№ идеальный выбор для тех, кто ценит надежность Рё стиль. Рти изделия отличаются высокой прочностью Рё легким весом, что делает РёС… идеальными для различных применений, РѕС‚ промышленности РґРѕ повседневного использования. Титановый сплав обеспечивает устойчивость Рє РєРѕСЂСЂРѕР·РёРё Рё долговечность, что гарантирует долгий СЃСЂРѕРє службы. Если РІС‹ хотите повысить качество вашей продукции или просто ищете эксклюзивный аксессуар, то купить Рзделия РёР· титана EL1 будет отличным решением. Питайте СЃРІРѕР№ интерес Рє инновациям Рё высоким технологиям СЃ нашими титанами!
aviator demo
джили эмгранд 2024 [url=http://www.geely-v-spb1.ru/models/emgrand]http://www.geely-v-spb1.ru/models/emgrand[/url] .
Celine fake designer bags
Online casinos should test—try me! gates of olympus
mostbet скачать на телефон бесплатно андроид [url=mostbet6039.ru]mostbet скачать на телефон бесплатно андроид[/url] .
поддержка мостбет [url=http://mostbet6014.ru]http://mostbet6014.ru[/url] .
DIOR fake designer bags
Заказать диплом об образовании. Покупка официального диплома через надежную компанию дарит немало плюсов для покупателя. Это решение дает возможность сберечь время и значительные средства. [url=http://dev.fleeped.com/create-blog/]dev.fleeped.com/create-blog[/url]
mostbet kg скачать [url=http://mostbet6014.ru]http://mostbet6014.ru[/url] .
plinko reviews
Meds information sheet. What side effects can this medication cause?
can i buy cheap macrobid no prescription
Best about pills. Get information now.
Louis Vuitton replica designer bags
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
Наши товары:
Меднаядвухраструбная подвижнаямуфта под пайку34х1.8ммМ1рГОСТ 32590-2013 Выберите медные двухраструбные муфты под пайку от производителя RedmetSplav. Прочные и герметичные соединения для систем водоснабжения, отопления и газоснабжения. Долговечные и надежные муфты для любых видов работ. Подходят для использования в домашних условиях, коммерческих и промышленных объектах.
Bottega Veneta bag replica
рейтинг онлайн казино с детальным обзором
mines app download
Playing online craps is a blast—way easier than learning in person! slot gallina
На сайте https://ufk-techno.ru/ уточните всю необходимую информацию, которая связана с поставками промышленного оборудования от предприятия ООО ТК «Регион Новые Технологии». Компания находится на рынке, начиная с 2011 года. Основная специализация – это поставки электродвигателей, насосного оборудования как зарубежного, так и отечественного производства. Перед тем, как совершить приобретение, изучите весь каталог продукции. Регулярно появляются новые тематические статьи, чтобы вы с ними ознакомились.
Allergic conjunctivitis treatment options
недорогой интернет воронеж
domashij-internet-voronezh001.ru
подключить интернет воронеж
Celine fake designer bags
Платформа birzha-akkauntov-online.ru – проверенное решение для вебмастеров.
Подобные ресурсы помогают быстро обходить ограничения соцсетей и избежать непредсказуемого фарминга.
Сильные преимущества таких платформ:
– Обеспечение безопасности покупок через эскроу-механизм
– Существенная экономия времени и усилий
– Большой ассортимент профилей для любых задач — от авторегов до трастовых с историей
– Легкость работы с крупными объемами
Чтобы получить максимальную отдачу, рекомендуется:
– Пользоваться авторитетными платформами с прозрачными правилами
– Применять чистые прокси (мобильные) и инструменты анонимизации
– Уделять внимание прогреву новых аккаунтов
buy lisinopril 40 mg tablet
Купить диплом ВУЗа по невысокой стоимости вы можете, обратившись к проверенной специализированной фирме. Заказать документ института можно в нашей компании в столице. [url=http://oddswinner.com/kupit-diplom-gosudarstvennogo-obrazca-211-2/]oddswinner.com/kupit-diplom-gosudarstvennogo-obrazca-211-2[/url]
https://xnudes.app/
РедМетСплав предлагает внушительный каталог отборных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до масштабных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица изделия подтверждена требуемыми документами, подтверждающими их происхождение. Дружелюбная помощь – наша визитная карточка – мы на связи, чтобы ответить на ваши вопросы и находить ответы под специфику вашего бизнеса.
Доверьте ваш запрос специалистам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наша продукция:
РљСЂСѓРі висмутовый РџРћРЎР’Рё 36-4 РљСЂСѓРі висмутовый РџРћРЎР’Рё 36-4 – это высококачественный инструмент, предназначенный для выполнения сварочных Рё резательных работ. Рзготавливается РёР· специального сплава, обеспечивающего отличные термические Рё механические свойства. Профессионалы ценят его Р·Р° долговечность Рё эффективность. Благодаря уникальным характеристикам, РєСЂСѓРі РїРѕРґС…РѕРґРёС‚ как для профессионального, так Рё для бытового использования. Если РІС‹ ищете надежный инструмент, советуем купить РљСЂСѓРі висмутовый РџРћРЎР’Рё 36-4. Его использование значительно улучшит качество выполняемых работ.
Приобрести диплом возможно через сайт компании. [url=http://gtral.com/companies/frees-diplom/]gtral.com/companies/frees-diplom[/url]
Drugs information for patients. Effects of Drug Abuse.
how can i get generic xenical without a prescription
Best information about medicines. Read information here.
fake Fendi bag
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние успехи.
Наши товары:
Ниобиевый тигель высокой чистоты
мостбет скачать бесплатно [url=https://www.mostbet6040.ru]https://www.mostbet6040.ru[/url] .
fake Louis Vuitton bag
aviator predictor online free
На сайте http://gruzogazel.ru уточните телефон компании для того, чтобы воспользоваться срочными и круглосуточными грузоперевозками, а также услугами квалифицированного, компетентного грузчика. При необходимости ваш груз будет доставлен непосредственно до дверей. В работе используются автомобили следующих марок: Форд, Мерседес, Газель. Услуги по фиксированной стоимости. В компании гарантируют аккуратность, точность, а также вежливое отношение. Воспользоваться услугами сможет каждый желающий, кто заинтересован в быстром выполнении работ.
На сайте https://smartporog.ru/ есть возможность посмотреть весь каталог выпадающих умных порогов. Они подходят для дверей любых типов и модификаций. Пороги автоматического типа идеально подходят для того, чтобы ими заменить стационарный порог, который в конкретном случае не получится установить. Их основное предназначение в том, чтобы замаскировать преграду в полу, избавиться от сквозняка, повысить качество звукоизоляции. Кроме того, такие пороги повышают звукоизоляцию. Выполнены из высокотехнологичных, инновационных материалов.
I don’t trust online casino RNGs—feels like they cheat! aviator casino
На сайте http://pkfnova.ru закажите обратный звонок для того, чтобы приобрести резиновые рукава, техническую пластину либо гидроуплотнения. Основная сфера деятельности предприятия ООО “ПКФ “НОВА” заключается в том, чтобы произвести резиновые рукава, а также резинотехнические изделия. Резинотехнические изделия создаются и внедряются для самых разных областей промышленности. У предприятия имеется своя производственная площадка. Вся продукция создается в соответствии с ГОСТом и нормативами, а потому отличается долгим сроком службы.
Prada fake designer bags
Prada bag replica
Платформа аккаунт для рекламы – проверенное решение для специалистов по привлечению трафика, SMM, интернет-маркетингу.
Подобные ресурсы помогают без лишних усилий решать задачи с блокировками аккаунтов и избежать самостоятельного фарма.
Ключевые преимущества подобных сервисов:
– Обеспечение безопасности сделок через эскроу-механизм
– Значительная экономия времени и усилий
– Большой ассортимент профилей для разных целей — от авторегов до трастовых с историей
– Простота масштабирования
Чтобы получить максимальную отдачу, необходимо:
– Выбирать только проверенные платформами с прозрачными правилами
– Применять надежные прокси (мобильные) и инструменты анонимизации
– Уделять внимание прогреву новых аккаунтов
Экзотика для души и тела.
Екзотични почивки 2025 [url=ekzotichni-pochivki.com]ekzotichni-pochivki.com[/url] .
На сайте https://ruzskiy-rayon.santex-uslugi.ru/ оставьте заявку для того, чтобы воспользоваться установкой систем отопления, а также водоснабжения. Если система правильно спроектирована, а монтаж выполнен грамотно и в соответствии с требованиями, нормативами, то такая система прослужит очень долго, дополнительно обеспечит комфорт, необходимый микроклимат. В помещении будет создан комфорт и уют, что особенно важно в межсезонье и холодные зимние месяцы. В компании применяются только материалы эталонного качества.
На сайте https://penza.seoalex.ru/o-sebe/ ознакомьтесь с информацией, посвященной высококлассному, опытному SEO-специалисту Алексею. Он уже давно работает в этой сфере и предпринимает все возможное для того, чтобы каждый клиент получил именно тот результат, который хотел. Он работает директологом контекстной рекламы, а также СЕО-специалистом. Отличается огромным опытом в перечисленных сферах, использует только уникальные и работающие методы, которые дают гарантированный результат. Если не желаете переплачивать, то обращайтесь к Алексею.
Medicines information sheet. Brand names.
where can i buy ramipril price
Everything information about medicines. Read information here.
Fendi bag replica
провайдеры домашнего интернета воронеж
domashij-internet-voronezh002.ru
подключить интернет тарифы воронеж
Chanel replica designer bags
how to get cipro pill
Мы готовы предложить дипломы любой профессии по приятным ценам.– [url=http://kupit-diplom24.com/kupit-diplom-s-zaneseniem-v-reestr-foruma-vigodno-3/]kupit-diplom24.com/kupit-diplom-s-zaneseniem-v-reestr-foruma-vigodno-3/[/url]
Приобрести диплом любого ВУЗа!
Наша компания предлагаетбыстро и выгодно заказать диплом, который выполняется на оригинальной бумаге и заверен мокрыми печатями, водяными знаками, подписями. Диплом пройдет любые проверки, даже при использовании специально предназначенного оборудования. Решайте свои задачи максимально быстро с нашей компанией- [url=http://avtobestnews.ru/kupite-diplom-i-nachnite-zarabatyivat-bolshe/]avtobestnews.ru/kupite-diplom-i-nachnite-zarabatyivat-bolshe[/url]
I was recommended this website by my cousin I am not sure whether this post is written by him as nobody else know such detailed about my trouble You are amazing Thanks
I was recommended this website by my cousin I am not sure whether this post is written by him as nobody else know such detailed about my trouble You are amazing Thanks
get generic zyvox no prescription
Online casinos need signs—flag us! book of ra
Licensed online casino mostbet jet x popular slot machines, live dealers, promotions and bonuses. User-friendly interface, quick registration, many payment systems and 24/7 support. Play your favorite games and win without leaving your home.
Aviator Bangladesh https://www.city.fi/blogit/aviator-game-casino/aviator+game+in+bangladesh+a+comprehensive+overview+introduction/139383 is your gateway to fun and fast gaming! No complex rules — just launch, bet, and cash out on time. Supported by leading Bangladeshi casinos, with BDT payments and full mobile access. Join and win anytime, anywhere.
Bottega Veneta bag replica
Hi i think that i saw you visited my web site thus i came to Return the favore I am attempting to find things to improve my web siteI suppose its ok to use some of your ideas
мостбет официальный сайт вход букмекерская контора [url=https://mostbet6041.ru]https://mostbet6041.ru[/url] .
new comics superhero comics free HD
Ellcon.ru для вашего комфорта создан, чтобы вы время на поездки могли экономить, и найти нужное, из дома не выходя. у нас вы можете купить провода и аксессуары, электроустановочные изделия, климатические системы, кабели, высоковольтное оборудование по доступным ценам. Ищете кабель канал 25х16 элекор? Ellcon.ru – портал, выбрав его для покупки, вы нервы, деньги и время прилично сэкономите. Доставка товаров исключительно при 100% предоплате заказа выполняется. Стремимся к лучшему, чтобы ежедневно радовать клиентов. Приятных вам приобретений!
Gucci fake designer bags
anime manga comic online manga site best
Мы предлагаем дипломы психологов, юристов, экономистов и любых других профессий по разумным тарифам. Мы предлагаем документы техникумов, расположенных на территории всей РФ. Дипломы и аттестаты выпускаются на “правильной” бумаге высшего качества. Это дает возможность делать государственные дипломы, которые невозможно отличить от оригинала. [url=http://aviantrp.moibb.ru/viewtopic.phpf=8&t=868/]aviantrp.moibb.ru/viewtopic.phpf=8&t=868[/url]
Заказать диплом об образовании. Приобретение документа о высшем образовании через проверенную и надежную фирму дарит ряд преимуществ. Такое решение позволяет сэкономить как дорогое время, так и серьезные финансовые средства. [url=http://sensemi.getbb.ru/viewtopic.php?f=6&t=1685/]sensemi.getbb.ru/viewtopic.php?f=6&t=1685[/url]
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние возможности.
Наша продукция:
Керамическая мишень Титаната Бария
Профильная юридическая служба, специализирующаяся на спорах в сфере автотранспорта. Основная деятельность:
Оспаривание постановлений ГИБДД, МАДИ, АДИ в судах Ленинградской области;
Взыскание ущерба при ДТП на загородных трассах ЛО;
Защита прав водителей при отказе страховых в выплатах по ОСАГО.
Работаем с делами на территории Санкт-Петербурга и пригородов с 2018 года. В арсенале: 120+ успешных кейсов по возврату водительских удостоверений. [url=https://avtoyuristvspb.ru/]Автоюрист спб[/url]
Meds information sheet. Brand names.
spironolactone transsexual hormone therapy
Actual news about pills. Read information now.
Такой магазин аккаунтов, как маркетплейс аккаунтов – это незаменимый инструмент вебмастеров.
Такие площадки помогают быстро обходить ограничения соцсетей и избежать непредсказуемого фарминга.
Основные преимущества таких платформ:
– Гарантия безопасности сделок через эскроу-механизм
– Значительная экономия времени и усилий
– Большой ассортимент профилей для любых задач — от авторегов до трастовых с историей
– Простота масштабирования
Чтобы избежать проблем, рекомендуется:
– Пользоваться авторитетными платформами с хорошими отзывами
– Применять надежные прокси (резидентные) и инструменты анонимизации
– Уделять внимание аккуратной подготовке перед запуском рекламы
Pills information for patients. Short-Term Effects.
generic fosamax price
Best news about medicines. Get now.
plinko free
Для удачного продвижения по карьерной лестнице нужно наличие диплома о высшем образовании. Приобрести диплом любого института у сильной организации: [url=http://diplom-zentr.com/kupit-diplom-o-visshem-obrazovanii-bistro-i-nadezhno-7/]diplom-zentr.com/kupit-diplom-o-visshem-obrazovanii-bistro-i-nadezhno-7/[/url]
aviator game app
Bottega Veneta fake designer bags
Bottega Veneta fake designer bags
plinko official app
Приобрести диплом института по доступной цене вы сможете, обращаясь к надежной специализированной компании. Купить документ университета вы имеете возможность у нас. [url=http://kolhos.listbb.ru/viewtopic.phpf=2&t=3013/]kolhos.listbb.ru/viewtopic.phpf=2&t=3013[/url]
Prada fake designer bags
Elite Link Indexer is the top choice among digital marketing agencies looking to maximize the number of backlinks indexed for their clients how to kill yourself
plinko game download
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с прекрасным качеством обслуживания.
Наша команда поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
Наша продукция:
Медная муфта деформируемая с концом под пайку 47х1.9 мм с наружной цилиндрической резьбой G 2 B 15.5 мм М2р ГОСТ Р52949-2008 Заказать медные муфты с цилиндрической резьбой по выгодной цене. Универсальные и надежные компоненты для создания прочных соединений в водоснабжении, отоплении и промышленном оборудовании. Большой выбор размеров и высокое качество гарантированы производителем. Доставка по России.
Online casinos should verify withdrawals faster—waiting is torture! plinko
На сайте https://y6.tartugi.site в большом количестве представлены самые интересные, увлекательные фильмы различных жанров: триллеры, драмы, комедии, приключения, боевики, исторические, детективы и многое другое. Также представлены и сериалы, которые вызовут у вас восторг и положительные впечатления. Есть как новинки, так и бестселлеры прошлых лет, которые не перестают пересматривать раз за разом. Имеются и мультфильмы тех лет, новинки, выхода которых ждали дети самых разных возрастов. Заходите сюда регулярно, чтобы получить порцию приятных эмоций.
avodart 2.5
DIOR replica designer bags
Celine bag replica
казино мостбет скачать [url=https://mostbet6042.ru/]https://mostbet6042.ru/[/url] .
Online clicker for kids in browser!
https://leasedadspace.com/members/Platonov/blog/1501319/top-10-io-games-you-need-to-try-in-2025/
DIOR bag replica
провайдеры домашнего интернета воронеж
domashij-internet-voronezh003.ru
провайдеры домашнего интернета воронеж
Приобрести диплом о высшем образовании!
Мы предлагаем документы университетов, расположенных на территории всей Российской Федерации. Документы печатаются на бумаге самого высокого качества: [url=http://milanoregola.copiny.com/question/details/id/1079692/]milanoregola.copiny.com/question/details/id/1079692[/url]
Выгодно купить диплом о высшем образовании!
Заказать диплом института по невысокой цене возможно, обратившись к надежной специализированной фирме. Купить диплом о высшем образовании: [url=http://diplomgorkiy.com/diplom-s-reestrom-kupit-ofitsialnij-dokument/]diplomgorkiy.com/diplom-s-reestrom-kupit-ofitsialnij-dokument[/url]
Drugs information for patients. Brand names.
cheap venlafaxine hcl er
Actual trends of pills. Get information here.
Доставка воды в в крупных городах здесь
Play Aviator Game https://devfolio.co/projects/grocall-1 online and win real money! Easy to play, exciting crash mechanics, fast rounds, and instant cashouts. Trusted by thousands of players worldwide — start now and catch the multiplier before it flies away!
Заказать диплом под заказ в Москве возможно используя официальный портал компании. [url=http://flashtest.80lvl.ru/posting.phpmode=post&f=3&sid=bed9579a55b80cec2f8f04638a4684ce/]flashtest.80lvl.ru/posting.phpmode=post&f=3&sid=bed9579a55b80cec2f8f04638a4684ce[/url]
online plinko
Купить диплом университета по выгодной стоимости можно, обратившись к проверенной специализированной компании. Заказать документ о получении высшего образования можно в нашей компании в столице. [url=http://kupitediplom0027.ru/kupit-diplom-s-reestrom-bistro-i-bez-problem-5/]kupitediplom0027.ru/kupit-diplom-s-reestrom-bistro-i-bez-problem-5[/url]
РедМетСплав предлагает внушительный каталог высококачественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица изделия подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Превосходное обслуживание – наш стандарт – мы на связи, чтобы ответить на ваши вопросы по мере того как предоставлять решения под особенности вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
Наши товары:
Лента титановая Р’Рў18РЈ – РћРЎРў 1 90013-81 РљСЂСѓРі титановый Р’Рў18РЈ – РћРЎРў 1 90013-81 представляет СЃРѕР±РѕР№ высококачественный материал, используемый РІ различных отраслях, включая аэрокосмическую Рё медицинскую. Отличается превосходной РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью Рё легкостью, что делает его идеальным выбором для сложных конструкций. Ртот РєСЂСѓРі обладает высокой прочностью Рё отличной обрабатываемостью, что обеспечивает удобство РІ работе Рё долговечность конечных изделий. РќРµ упустите возможность купить РљСЂСѓРі титановый Р’Рў18РЈ – РћРЎРў 1 90013-81 Рё получите надежный материал для ваших проектов!
Louis Vuitton replica designer bags
Платформа покупка аккаунтов – необходимый ресурс арбитражников.
Подобные ресурсы помогают оперативно обходить ограничения соцсетей и избежать самостоятельного фарма.
Основные преимущества таких платформ:
– Гарантия надежности сделок через систему гаранта
– Существенная экономия ресурсов
– Большой ассортимент профилей для любых задач — от дешевых до прогретых с историей
– Легкость работы с крупными объемами
Чтобы получить максимальную отдачу, необходимо:
– Выбирать только проверенные платформами с хорошими отзывами
– Применять чистые прокси (резидентные) и инструменты анонимизации
– Уделять внимание прогреву новых аккаунтов
Gucci replica designer bags
mines casino game free
mostbet мостбет [url=http://mostbet6042.ru]http://mostbet6042.ru[/url] .
where can i get generic micronase pills
is plinko legit
Online casinos should cap—play safe! pinup
Celine bag replica
Посетите сайт https://xn--j1abip.com/ и вы сможете ознакомиться с ЖК Толк в Академическом районе города Екатеринбург. Узнайте все преимущества ЖК, его местоположение, инфраструктуру рядом. На сайте вы сможете выбрать квартиру или паркинг. Купить новую квартиру в ЖК от застройщика по выгодной цене рядом с Преображенским парком легко – мы предлагаем различные варианты оплаты и ипотеку по выгодной ставке. Подробнее на сайте.
Louis Vuitton replica designer bags
fake prada bag
Заказать диплом любого университета!
Наша компания предлагаетвыгодно приобрести диплом, который выполнен на бланке ГОЗНАКа и заверен мокрыми печатями, водяными знаками, подписями. Данный диплом способен пройти лубую проверку, даже при использовании специально предназначенного оборудования. Решайте свои задачи быстро и просто с нашим сервисом- [url=http://worksale.mn.co/posts/82926266/]worksale.mn.co/posts/82926266[/url]
Meds prescribing information. Brand names.
can i get cheap cardizem without rx
All what you want to know about meds. Get now.
Chanel fake designer bags
mines game download
where to get generic dramamine without rx
what is plinko
Gucci fake designer bags
Где приобрести [b]диплом[/b] по актуальной специальности?
Полученный диплом с приложением полностью отвечает стандартам, неотличим от оригинала. Не следует откладывать собственные мечты на продолжительные годы, реализуйте их с нашей помощью – отправляйте заявку на изготовление документа сегодня! Получить диплом о среднем образовании – легко! [url=http://prof-komplekt.com/club/log/index.php/]prof-komplekt.com/club/log/index.php[/url]
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – идеальный вариант для вас.
Наша продукция:
Проволока вольфрамовая 1435 мм ВМ ГОСТ 19671-91 Купить вольфрамовую проволоку у производителя. Широкий выбор диаметров и нарезка по размеру. Высокая теплопроводность и стойкость к коррозии. Доставка по России.
Meds information for patients. Effects of Drug Abuse.
buying cheap norvasc
Actual information about medication. Get here.
DIOR replica designer bags
pin up azerbaycan [url=https://pinup-azerbaycan51.com/]pin up azerbaycan[/url] .
Закажите уникальные шторы на заказ по лучшим ценам
шторы на заказ [url=https://shtory-moscow-zakaz.ru/]шторы на заказ[/url] . “Ткацкий”
Online casinos slide easy—mind it! plinko
мефедрон кристалл купить гей порно видео
найкращі фільми 2025 дивитися фільми онлайн безкоштовно 2025
Мы предлагаем дипломы любых профессий по приятным тарифам. Дипломы изготавливаются на фирменных бланках Купить диплом об образовании [url=http://diplomc-v-ufe.ru/]diplomc-v-ufe.ru[/url]
Где купить диплом по актуальной специальности?
Приобрести диплом университета по невысокой стоимости можно, обращаясь к проверенной специализированной компании.: [url=http://okdiplom.com/]okdiplom.com[/url]
aviator game apk
fake prada bag
На сайте https://mxart.ru/ ознакомьтесь со справочником тех компаний, которые занимаются не только разработкой, но и продвижением сайтов при помощи уникальных и новаторских методик. Здесь же вы сможете ознакомиться и с подготовкой к разработке ресурса. Это крайне важно для того, чтобы получить больше клиентов. Для начала рекомендуется произвести анализ конкурентов, а также ЦА с той целью, чтобы выделиться на фоне других. Также необходимо будет обозначить и бизнес-стратегии, поставить цели, к которым вы будете идти.
Заказать диплом любого университета!
Мы оказываем услуги по продаже документов об окончании любых ВУЗов Российской Федерации. Документы производят на подлинных бланках. [url=http://pattondemos.com/employer/radiplomy/]pattondemos.com/employer/radiplomy[/url]
Мы изготавливаем дипломы любой профессии по приятным ценам. Мы предлагаем документы техникумов, расположенных в любом регионе РФ. Документы выпускаются на “правильной” бумаге самого высокого качества. Это позволяет делать государственные дипломы, не отличимые от оригинала. [url=http://dukan.lovelytutorials.com/member.phpu=5648/]dukan.lovelytutorials.com/member.phpu=5648[/url]
РедМетСплав предлагает внушительный каталог высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы обеспечиваем быстрое выполнение вашего заказа.
Каждая единица товара подтверждена всеми необходимыми документами, подтверждающими их происхождение. Опытная поддержка – наша визитная карточка – мы на связи, чтобы улаживать ваши вопросы по мере того как находить ответы под требования вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
Наши товары:
Лента магниевая Coralloy Zirconium 704 – KIND & CO., Edelstahlwerk, KG РљСЂСѓРі магниевый Coralloy Zirconium 704 – KIND & CO., Edelstahlwerk, KG – это высококачественный абразивный материал, предназначенный для различных производственных процессов. РћРЅ обеспечивает отличное качество обработки поверхностей благодаря уникальным свойствам магния Рё циркония. Ртот РєСЂСѓРі является идеальным выбором для резки Рё шлифовки, гарантируя долговечность Рё эффективность. Если РІС‹ ищете надежное решение для обработки металлов, то купить РљСЂСѓРі магниевый Coralloy Zirconium 704 – KIND & CO., Edelstahlwerk, KG станет правильным выбором для вашего бизнеса. Ртот РїСЂРѕРґСѓРєС‚ поможет добиться высокой производительности Рё точности РІ работе.
Prada fake designer bags
Medicines information. Generic Name.
where can i get proscar without prescription
All what you want to know about medicine. Read information here.
Great post! I really enjoyed reading this.
Louis Vuitton bag replica
aviator download
I love the online promos—new spark! Marvel Casino
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наши товары:
Керамическая мишень Licoo2 (3N5, 4N)
Где купить диплом по нужной специальности?
Мы готовы предложить дипломы любой профессии по выгодным тарифам. Для нас важно, чтобы дипломы были доступными для большого количества наших граждан. Быстро купить диплом университета [url=http://diplomnie.com/ofitsialnij-diplom-o-srednem-obrazovanii-bistro-i-nadezhno/]diplomnie.com/ofitsialnij-diplom-o-srednem-obrazovanii-bistro-i-nadezhno/[/url]
Chanel replica designer bags
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
С широким ассортиментом продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние возможности.
Наши товары:
Плоская мишень Cofeb
cost generic detrol online
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Мишень Диспрозия 3N, плоская форма”
how to get cheap sumycin without dr prescription
Online clicker for kids in browser!
https://usa.life/read-blog/73559
Здравствуйте!
Мы предлагаем дипломы любых профессий по приятным тарифам. Цена может зависеть от той или иной специальности, года получения и образовательного учреждения: [url=http://rdiploma24.com/]rdiploma24.com/[/url]
Мы предлагаем быстро заказать диплом, который выполняется на оригинальной бумаге и заверен мокрыми печатями, штампами, подписями официальных лиц. Данный документ пройдет любые проверки, даже с использованием специфических приборов. [url=http://semeyka.listbb.ru/viewtopic.php?f=20&t=1653/]semeyka.listbb.ru/viewtopic.php?f=20&t=1653[/url]
pin up azerbaycan [url=https://www.pinup-azerbaycan51.com]pin up azerbaycan[/url] .
Приобрести диплом института по невысокой цене вы можете, обращаясь к проверенной специализированной компании. Приобрести документ о получении высшего образования вы можете в нашей компании в столице. [url=http://rusd-diplomj.ru/diplom-bakalavra-s-zaneseniem-v-reestr-8/]rusd-diplomj.ru/diplom-bakalavra-s-zaneseniem-v-reestr-8[/url]
Esenkent su kaçak tespiti Pendik’teki evimdeki su kaçağını Testo termal kameralarıyla tespit ettiler, çok başarılıydılar. https://siteintel.net/domain/uskudartesisat.com
Купить диплом института по невысокой цене вы сможете, обратившись к надежной специализированной фирме. Купить документ университета можно в нашем сервисе. [url=http://negreenorchid.com/kupit-diplom-gosudarstvennogo-obrazca-164/]negreenorchid.com/kupit-diplom-gosudarstvennogo-obrazca-164[/url]
Заказать диплом об образовании. Приобретение подходящего диплома через проверенную и надежную компанию дарит массу достоинств для покупателя. Это решение позволяет сберечь как длительное время, так и значительные финансовые средства. [url=http://worldgonews.ru/kupit-diplom-garantii-i-konfidentsialnost/]worldgonews.ru/kupit-diplom-garantii-i-konfidentsialnost[/url]
Gucci replica designer bags
На сайте http://kungfu.ru/ почитайте всю исчерпывающую, полезную информацию о школе классического ушу, которая находится в Москве. Обучение происходит для новичков в этой сфере, которые стремятся освоить технику. На уроках изучается шаолиньский Цигун, рукопашный бой, а также медитации. Ученики обучаются самым разным приемам, работе с оружием. Вы сможете не только защищаться, но и улучшите здоровье, укрепите организм, улучшите работу органов дыхания. Стоимость одного занятия указана на портале. Есть возможность воспользоваться пробным занятием.
Fendi replica designer bags
Medicine information leaflet. Drug Class.
cytotec online india
Everything trends of medicines. Read information here.
мостбет вход регистрация [url=mostbet6042.ru]мостбет вход регистрация[/url] .
Заказать диплом академии !
Приобретение диплома ВУЗа России в нашей компании является надежным процессом, ведь документ будет заноситься в реестр. Приобрести диплом об образовании [url=http://diplomv-v-ruki.ru/kupit-diplom-zanesennij-v-reestr-bistro-i-bez-problem/]diplomv-v-ruki.ru/kupit-diplom-zanesennij-v-reestr-bistro-i-bez-problem[/url]
Drugs information sheet. What side effects?
can i purchase fosamax without dr prescription
All information about meds. Get information here.
futebol ao vivo multicanal -(https://xi744q4s.com)
Всегда думал что купить диплом о высшем образовании это миф и нереально, но все оказалось не так, изначально искал информацию про: купить диплом эколога, купить диплом в вольске, купить диплом в минеральных водах, купить диплом в новоалтайске, купить диплом в киселевске, потом про дипломы вузов, подробнее здесь [url=http://proffdiplomik.com/tambov/]proffdiplomik.com/tambov[/url]
I love the live roulette—real deal! mines game
Купить диплом университета по выгодной стоимости возможно, обращаясь к проверенной специализированной фирме. Мы можем предложить документы ВУЗов, расположенных на территории всей Российской Федерации. [url=http://borderforum.ru/viewtopic.phpf=7&t=13215/]borderforum.ru/viewtopic.phpf=7&t=13215[/url]
На сайте https://internetometer.net/ воспользуйтесь всеми возможностями интернетометра. При помощи такого сервиса у вас получится узнать собственный IP-адрес, настройки браузера, а также местоположение, другую важную и ценную информацию, которая обязательно вам пригодится. Этот сервис предоставляет уникальную возможность абсолютно бесплатно узнать то, какова скорость вашего Интернета. Вы сможете зайти на сайт в любое, наиболее комфортное время. Все представленные данные являются абсолютно точными, поэтому на них можно положиться.
На сайте https://naro-fominsk.santex-uslugi.ru/ оставьте заявку с той целью, чтобы воспользоваться услугой, связанной с установкой систем отопления. Монтаж является обязательным условием для того, чтобы с комфортом проживать в частном доме, коттедже. Так получится создать в помещении непринужденную, приятную атмосферу, уют и безопасность. Предприятие дает на свои работы, материалы гарантии, обеспечивается профессиональный подход к выполнению задачи любого уровня сложности. Сотрудники отличаются глубокими знаниями в данной сфере.
Gucci replica designer bags
Купить документ института вы можете в нашем сервисе. Мы оказываем услуги по продаже документов об окончании любых университетов Российской Федерации. Вы получите необходимый диплом по любой специальности, включая документы Советского Союза. Гарантируем, что в случае проверки документов работодателями, подозрений не появится. [url=http://diplomt-nsk.ru/kupit-gosudarstvennij-diplom-s-zaneseniem-v-reestr-3/]diplomt-nsk.ru/kupit-gosudarstvennij-diplom-s-zaneseniem-v-reestr-3/[/url]
Bottega Veneta bag replica
Собрался в короткую поездку, а на билеты не хватало 6 000 рублей. Быстро поискал через Яндекс — вышел на канал [url=https://t.me/s/mfo_2024_online]совсем новые мфо без отказа[/url] . Сразу видно: люди заморочились. У каждой МФО — мини-обзор, все с регистрацией в ЦБ. И плюс — суперраздел “дают при любой истории”. Деньги получил через 15 минут. Работает!
Потолочные и оконные шторы на заказ — широкий выбор
шторы на заказ [url=https://shtory-moscow-zakaz.ru/]шторы на заказ[/url] . “Ткацкий”
[url=https://top-casino-rus.ru]top-casino-rus.ru[/url]
В 2025 году российские пользователи проявляют повышенный интерес к онлайн-развлечениям, включая легальные игровые платформы. На нашем сайте представлен актуальный рейтинг проверенных сервисов, соответствующих требованиям законодательства.
Критерии отбора
В наш обзор включены только ресурсы, которые:
Имеют международные лицензии (Кюрасао, Мальта)
Поддерживают рублевые платежи
Предлагают SSL-шифрование данных
Имеют положительную репутацию среди пользователей
Топ-3 легальные платформы
“Клуб азартных игр” – лицензионный проект с ежедневными турнирами
“Игровой портал” – платформа с прозрачными выплатами
“Развлекательный центр” – сервис с системой ответственной игры
Важная информация
Согласно российскому законодательству:
Организация азартных игр в интернете запрещена
Доступ к международным платформам ограничен
Игра на незаконных сайтах влечет ответственность
Альтернативные развлечения
Мы рекомендуем рассмотреть легальные варианты:
Официальные лотереи (Столото)
Социальные казино без реальных ставок
Игровые приложения с виртуальной валютой
Важно: Напоминаем о рисках игровой зависимости. Если вы или ваши близкие столкнулись с этой проблемой, обратитесь в специализированные центры помощи.
Для получения полной информации о легальных игровых возможностях в 2025 году посетите сайт по ссылке.top-casino-rus.ru
перенаправляется сюда [url=https://vodkacasino.net]vodka bet vodkabet[/url]
[url=https://t.me/ruanabol_org]Фармаком Лабс[/url] – Pharmacom, Анаболики
Купить диплом под заказ вы имеете возможность используя сайт компании. [url=http://feleempleo.es/employer/frees-diplom/]feleempleo.es/employer/frees-diplom[/url]
can you get zocor
Louis Vuitton replica designer bags
Louis Vuitton replica designer bags
Добрый день!
Для некоторых людей, заказать [b]диплом[/b] ВУЗа – это необходимость, шанс получить достойную работу. Впрочем для кого-то – это понятное желание не терять время на учебу в ВУЗе. С какой бы целью вам это не потребовалось, наша фирма готова помочь вам. Быстро, качественно и по доступной цене сделаем документ любого года выпуска на подлинных бланках со всеми необходимыми подписями и печатями.
Основная причина, почему многие люди прибегают к покупке документа, – получить хорошую должность. Предположим, способности и опыт позволяют специалисту устроиться на желаемую работу, но подтверждения квалификации не имеется. В том случае если работодателю важно присутствие “корочки”, риск потерять место работы очень высокий.
Заказать документ о получении высшего образования можно в нашем сервисе. Мы предлагаем документы об окончании любых ВУЗов Российской Федерации. Вы сможете получить необходимый диплом по любой специальности, любого года выпуска, в том числе документы Советского Союза. Гарантируем, что при проверке документа работодателями, подозрений не появится.
Ситуаций, которые вынуждают заказать диплом ВУЗа много. Кому-то прямо сейчас нужна работа, а значит, нужно произвести впечатление на руководителя при собеседовании. Другие желают попасть в престижную компанию, для того, чтобы повысить собственный статус в обществе и в последующем начать свой бизнес. Чтобы не тратить попусту годы жизни, а сразу начать успешную карьеру, используя имеющиеся навыки, можно заказать диплом через интернет. Вы сможете стать полезным для социума, получите финансовую стабильность в кратчайшие сроки- [url=http://rdiploman.com/]диплом купить о среднем образовании[/url]
Medicament information leaflet. Effects of Drug Abuse.
celebrex price
Actual about medicine. Read now.
play plinko
Work5 — №1 на рынке помощи с написанием курсовых работ! Выполняют срочные заказы по написанию курсовых, недорого https://www.work5.ru/kurs
Guide to Playing Online Contextual Games Successfully
How to Play Online Contextual Games
As the popularity of interactive competitions surges, participants are seeking effective methods to enhance their performance and enjoyment. The diverse landscape of these engaging activities presents numerous opportunities, but also challenges that require understanding and strategy. Many players find themselves overwhelmed by the options available, leading to suboptimal experiences.
In the ever-expanding realm of digital interactions, adopting a tactical approach can significantly improve your outcomes. Analyzing the mechanics of each challenge and recognizing patterns in gameplay can provide valuable insights. Familiarity with various formats, from trivia to strategy-based puzzles, allows competitors to tailor their techniques to fit the specific demands of each scenario.
Furthermore, building a network of fellow enthusiasts can enhance not only your skill set but also your enjoyment. Sharing tips, strategies, and experiences fosters a deeper understanding of the intricate details that can make a difference. Engaging with a community can also provide motivation and support, encouraging continuous improvement and exploration.
In conclusion, carving out a space in the competitive interactive scene requires dedication, strategic planning, and a willingness to learn. By employing these approaches, you can elevate your participation and achieve greater satisfaction in this dynamic environment.
Mastering Game Mechanics for Strategic Advantage
Understanding the underlying systems and rules of your chosen virtual experience is crucial for gaining an upper hand. Each platform features unique attributes, so take time to analyze specific mechanics that influence gameplay, such as resource management, character abilities, or environmental interactions.
One effective strategy involves studying character statistics and their impact on performance. Knowing how attributes like speed, strength, or intelligence affect gameplay can guide your decisions. Create builds that align with personal play styles while also adapting to the strengths and weaknesses of opponents.
Timing plays a significant role in many scenarios. Mastering actions that require precise execution, such as attacks or defenses, can shift momentum. Utilize practice modes or single-player components to hone your timing without the pressure of competition.
Leveraging terrain can provide profound benefits. Familiarize yourself with maps to identify advantageous positions that offer strategic leverage. High ground often translates to better visibility and control over engagements, while cover can protect you from enemy fire. Awareness of these spatial elements can dictate offensive or defensive tactics.
Real-time decision-making is paramount. Train yourself to assess situations quickly and choose appropriate actions under pressure. Develop a mental checklist of options available during conflicts, which will allow for versatility and responsiveness.
Collaboration with teammates enhances outcomes. Communication tools enable coordination; therefore, utilize them effectively. Share information regarding enemy locations, resource availability, or strategic plans. Encourage synergy by complimenting your teammates’ abilities, amplifying the collective performance.
Adaptation to shifting dynamics is necessary. Observe how opponents adjust their strategies and consider how you can counteract effectively. Implementing flexibility in your approach can provide an advantage and keep adversaries guessing.
Lastly, dedicating time to evaluate past performances can reveal areas for improvement. Recording gameplay and analyzing decisions can cultivate a more profound understanding of mechanics and enhance future actions. Gaining insights from both successes and failures will foster skill development.
Effective Time Management and Resource Allocation in Gameplay
Strategic use of time significantly impacts overall performance. Set specific time blocks for various tasks within the activity. For instance, allocate a certain duration for exploring, gathering resources, and completing objectives. This allows for a structured approach, reducing distractions and maximizing focus.
Prioritize activities based on immediate goals. Identify which tasks yield the highest returns in terms of points or resources. Concentrate efforts on high-impact actions, like completing challenges or acquiring special items that enhance future progress. This prioritization directs energy effectively.
Monitor resource usage closely. Establish limits on how much energy or currency can be spent within a session. Use tracking tools, if available, to keep an eye on expenditures and reserves. Very often, a sudden depletion of resources can disrupt plans, causing delays in advancement.
Make use of breaks. Scheduled pauses not only aid in maintaining mental acuity but also provide time to reorganize strategy based on recent outcomes. Utilize these intervals to analyze what strategies worked and what didn’t, thereby refining your approach for better results.
Collaborate with others whenever feasible. Sharing resources or trading can expedite progress and create opportunities to accomplish goals more rapidly. Form alliances or engage in multiplayer aspects to maximize harvests and benefits from joint efforts.
Set milestones. Break larger goals into smaller, manageable achievements. This breakdown helps in maintaining motivation and provides clear direction, making the experience more rewarding as each step is accomplished.
Regularly assess your strategies. Adaptations based on performance and resource availability can lead to improved outcomes. Being flexible enables you to seize unexpected opportunities and respond to challenges efficiently.
Feel free to surf to my web site; https://tigrinhoplataforma.com.br
Приобрести диплом о высшем образовании!
Наша компания предлагаетбыстро купить диплом, который выполнен на оригинальном бланке и заверен печатями, штампами, подписями. Диплом пройдет лубую проверку, даже с применением профессиональных приборов. Достигайте свои цели быстро с нашей компанией- [url=http://vibrantclubs.com/read-blog/18660_kupit-diplom-provedennyj-cherez-reestr.html/]vibrantclubs.com/read-blog/18660_kupit-diplom-provedennyj-cherez-reestr.html[/url]
pin.up casino [url=https://pinup-azerbaycan73.com/]pin.up casino[/url] .
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наши товары:
Гранулы кремния высокой чистоты.
can i buy generic vantin prices
pinap [url=https://www.pinup-azerbaycan51.com]https://www.pinup-azerbaycan51.com[/url] .
pinup giris [url=www.pinup-azerbaycan6.com/]pinup giris[/url] .
клубная музыка слушать [url=http://klubnaya-muzyka.ru]клубная музыка слушать[/url] .
сет музыкальный клубный [url=https://klubnaya-muzyka1.ru]сет музыкальный клубный[/url] .
Заказать диплом о высшем образовании!
Мы предлагаем дипломы любых профессий по приятным тарифам. Вы приобретаете диплом в надежной и проверенной компании. : [url=http://pivotalta.com/employer/2515/frees-diplom/]pivotalta.com/employer/2515/frees-diplom[/url]
[b]Диплом университета РФ![/b]
Без института сложно было продвигаться по карьерной лестнице. Именно из-за этого решение о покупке диплома стоит считать целесообразным. Заказать диплом о высшем образовании [url=http://talkrealty.ru/byistroe-oformlenie-diplomov-lyubogo-uchebnogo-zavedeniya/]talkrealty.ru/byistroe-oformlenie-diplomov-lyubogo-uchebnogo-zavedeniya[/url]
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
Наши товары:
РўСЂСѓР±Р° РёР· драгоценных металлов палладиевая 10С…5С…0.5 РјРј РџРґР82-18 РўРЈ Гарантированное качество Рё изысканный дизайн – купите драгоценные трубы для ювелирных украшений, машиностроения Рё архитектуры. РЁРёСЂРѕРєРёР№ выбор Рё высокое качество! Доставка РїРѕ Р РѕСЃСЃРёРё.
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наша продукция:
Алюминиевый двутавр Р’РђР”1 5x60x35 ГОСТ 13621-90 Купить двутавр алюминиевый СЃ высокой прочностью Рё устойчивостью Рє РєРѕСЂСЂРѕР·РёРё. Легкий вес для удобства транспортировки Рё монтажа. Рдеальное решение для создания каркасов зданий, мостов Рё РґСЂСѓРіРёС… конструкций. РџРѕРґС…РѕРґРёС‚ для применения РІ авиации, автомобильной Рё РґСЂСѓРіРёС… отраслях промышленности.
https://rkt-mai.ru/eskort-v-podmoskove-iskusstvo-sotvoreniya-nezabyvaemyx-momentov/
Купить диплом ВУЗа по выгодной цене возможно, обратившись к проверенной специализированной компании. Мы предлагаем документы об окончании любых университетов РФ. Заказать диплом ВУЗа– [url=http://diplomt-v-chelyabinske.ru/kupite-diplom-o-visshem-obrazovanii-prosto-i-bistro/]diplomt-v-chelyabinske.ru/kupite-diplom-o-visshem-obrazovanii-prosto-i-bistro/[/url]
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – идеальный вариант для вас.
поставляемая продукция:
Медная двухраструбная редукционная переходная муфта РїРѕРґ пайку 40.5С…40 РјРј мягкая пайка Рњ3Р Рњ ГОСТ Р 52922-2008 Выберите высококачественные медные двухраструбные редукционные муфты РїРѕРґ пайку РѕС‚ Редметсплав для надежного соединения медных труб различных диаметров. РЁРёСЂРѕРєРёР№ выбор размеров, эффективная установка Рё долговечность гарантированы. Рдеальное решение для систем отопления Рё водоснабжения.
plinko
Drug information for patients. Drug Class.
take lisinopril with or without food
Actual about meds. Read information now.
подробнее [url=https://alt-coins.cc/]Альткоин обменник[/url]
[url=https://top-casino-rus.ru]top-casino-rus.ru[/url]
В 2025 году российские пользователи проявляют повышенный интерес к онлайн-развлечениям, включая легальные игровые платформы. На нашем сайте представлен актуальный рейтинг проверенных сервисов, соответствующих требованиям законодательства.
Критерии отбора
В наш обзор включены только ресурсы, которые:
Имеют международные лицензии (Кюрасао, Мальта)
Поддерживают рублевые платежи
Предлагают SSL-шифрование данных
Имеют положительную репутацию среди пользователей
Топ-3 легальные платформы
“Клуб азартных игр” – лицензионный проект с ежедневными турнирами
“Игровой портал” – платформа с прозрачными выплатами
“Развлекательный центр” – сервис с системой ответственной игры
Важная информация
Согласно российскому законодательству:
Организация азартных игр в интернете запрещена
Доступ к международным платформам ограничен
Игра на незаконных сайтах влечет ответственность
Альтернативные развлечения
Мы рекомендуем рассмотреть легальные варианты:
Официальные лотереи (Столото)
Социальные казино без реальных ставок
Игровые приложения с виртуальной валютой
Важно: Напоминаем о рисках игровой зависимости. Если вы или ваши близкие столкнулись с этой проблемой, обратитесь в специализированные центры помощи.
Для получения полной информации о легальных игровых возможностях в 2025 году посетите сайт по ссылке.top-casino-rus.ru
plinko free demo
[url=https://create-casino.ru]create-casino.ru[/url]
Создать онлайн-казино в 2025? Это сложный процесс, требующий лицензии, надежного софта, безопасности и маркетинга. Необходимо учитывать законодательство, конкуренцию и ответственный подход к азартным играм. Узнайте больше о ключевых этапах и подводных камнях. Читайте подробнее по ссылке ниже
create-casino.ru
plinko app
Are online jackpots real?—skeptic! plinko
https://kovry32.ru/eskort-v-podmoskove-iskusstvo-tvoreniya-nezabyvaemyx-momentov/
Medicine information. What side effects can this medication cause?
metoprolol indian brands
All information about medicine. Get here.
https://receptmult.ru/
can i get atarax without a prescription
[url=https://azimutelematika.ru/]снижение расходов на Платон[/url] – Идентификация водителей, Контроль температуры
РедМетСплав предлагает внушительный каталог качественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до масштабных поставок, мы обеспечиваем быстрое выполнение вашего заказа.
Каждая единица продукции подтверждена требуемыми документами, подтверждающими их качество. Превосходное обслуживание – наш стандарт – мы на связи, чтобы улаживать ваши вопросы и адаптировать решения под особенности вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в множестве наших преимуществ
поставляемая продукция:
Проволока вольфрамовая ВМ Проволока вольфрамовая ВМ – это высококачественный материал, предназначенный для различных промышленных применений. Ее отличает высокая температура плавления, что делает ее не заменимой в сферах, требующих устойчивости к высоким температурами. Проволока обладает отличной проводимостью и коррозионной стойкостью. Приобретая Проволоку вольфрамовую ВМ, вы получаете надежный и долговечный продукт, который прекрасно подходит для использования в электронной и светотехнической отраслях. Не упустите возможность купить Проволока вольфрамовая ВМ и обеспечить свое производство качественным материалом!
РедМетСплав предлагает обширный выбор качественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица продукции подтверждена всеми необходимыми документами, подтверждающими их качество. Опытная поддержка – наша визитная карточка – мы на связи, чтобы ответить на ваши вопросы и находить ответы под особенности вашего бизнеса.
Доверьте потребности вашего бизнеса специалистам РедМетСплав и убедитесь в множестве наших преимуществ
поставляемая продукция:
Порошок титановый ЕТ2 Рзделия РёР· титана ЕТ2 – это надежные Рё прочные компоненты, созданные для удовлетворения потребностей даже самых требовательных клиентов. Титан обладает высокой РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью Рё легкостью, что делает его идеальным выбором для различных применений. Р’ нашем ассортименте представлены изделия, которые РїРѕРґС…РѕРґСЏС‚ как для промышленного использования, так Рё для индивидуальных проектов. РС… высокие показатели прочности Рё долговечности обеспечивают долгий СЃСЂРѕРє службы. Р’С‹ можете купить Рзделия РёР· титана ЕТ2 РїСЂСЏРјРѕ сейчас Рё сделать правильный выбор для своей деятельности.
РедМетСплав предлагает внушительный каталог качественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их происхождение. Опытная поддержка – наш стандарт – мы на связи, чтобы ответить на ваши вопросы а также адаптировать решения под требования вашего бизнеса.
Доверьте вашу потребность в редких металлах специалистам РедМетСплав и убедитесь в множестве наших преимуществ
Наши товары:
Рзделия РёР· кобальта HAYNES 263 Рзделия РёР· кобальта HAYNES 263 представляют СЃРѕР±РѕР№ высококачественные изделия, обладающие отличными эксплуатационными характеристиками. Ртот сплав разработан для работы РІ условиях высоких температур Рё агрессивных сред, что делает его идеальным выбором для множества промышленных приложений. РљСЂРѕРјРµ того, кобальтовые изделия выделяются своей стойкостью Рє РєРѕСЂСЂРѕР·РёРё Рё высокой прочностью, что обеспечивает долговечность Рё надежность. Если РІС‹ ищете надежные Рё высокоэффективные решения, РЅРµ упустите шанс купить Рзделия РёР· кобальта HAYNES 263 Рё убедитесь РІ РёС… превосходных преимуществах.
Мы предлагаем выгодно купить диплом, который выполнен на оригинальной бумаге и заверен печатями, водяными знаками, подписями. Данный диплом способен пройти лубую проверку, даже при помощи профессиональных приборов. [url=http://audiojerks.com/2025/04/11/pokupka-diploma-s-zaneseniem-v-reestr-196/]audiojerks.com/2025/04/11/pokupka-diploma-s-zaneseniem-v-reestr-196[/url]
Мы изготавливаем дипломы любых профессий по приятным тарифам. Мы предлагаем документы техникумов, которые расположены на территории всей Российской Федерации. Документы печатаются на “правильной” бумаге высшего качества. Это дает возможности делать государственные дипломы, которые невозможно отличить от оригиналов. [url=http://slliver.getbb.ru/viewtopic.phpf=62&t=3069/]slliver.getbb.ru/viewtopic.phpf=62&t=3069[/url]
подробнее [url=https://futbolka-oversize.ru/]смотреть[/url]
Hürriyet su kaçak tespiti Sonsuz Teşekkürler: Bizi büyük bir dertten kurtardılar. Sonsuz teşekkürler! https://helpdesk.rikor.com/?p=366272
pin. up [url=www.pinup-azerbaycan73.com]pin. up[/url] .
Avcılar su kaçak tespiti Beklentilerimizi Karşıladı: Başta tereddüt ettik ama gerçekten beklentilerimizin üzerinde bir hizmet aldık. http://www.lostandfoundstudio.it/lfs/?p=10617
Medicines information leaflet. What side effects can this medication cause?
can you get tadacip pills
All information about medication. Get here.
Приобрести диплом любого ВУЗа!
Купить диплом университета по невысокой стоимости можно, обратившись к надежной специализированной фирме. Заказать диплом: [url=http://diplomist.com/diplom-s-otsenkami-karera-i-visokij-doxod/]diplomist.com/diplom-s-otsenkami-karera-i-visokij-doxod[/url]
Купить диплом университета по выгодной стоимости возможно, обращаясь к проверенной специализированной компании. Заказать документ ВУЗа вы имеете возможность у нас. [url=http://good-diplom.ru/kupite-legalnij-diplom-s-zaneseniem-v-reestr/]good-diplom.ru/kupite-legalnij-diplom-s-zaneseniem-v-reestr[/url]
крутая музыка [url=https://klubnaya-muzyka1.ru]крутая музыка[/url] .
На сайте https://pr-n.ru/advance/yandex-direct/ закажите звонок для того, чтобы узнать все об услуге Яндекс.Директ. В компании работают лучшие, перспективные и талантливые специалисты, которые трудятся на результат, помогают вашему бизнесу быстрее развиться. Для этих целей создается имиджевая реклама, а также реклама мобильных приложений. В обязательном порядке проводится аудит, разрабатывается особая стратегия, которая поможет продвинуть ваш бизнес. Каждый клиент будет регулярно получать отчеты о проделанной работе.
[url=https://create-casino.ru]create-casino.ru[/url]
Создать онлайн-казино в 2025? Это сложный процесс, требующий лицензии, надежного софта, безопасности и маркетинга. Необходимо учитывать законодательство, конкуренцию и ответственный подход к азартным играм. Узнайте больше о ключевых этапах и подводных камнях. Читайте подробнее по ссылке ниже
create-casino.ru
Желаете отправить букет без лишних хлопот?
Тогда вам стоит обратить внимание на интересную статью одоставке цветов https://rostov161.net/articles/5637-luchshie-kustovye-rozy-v-moskve-s-dostavkoi-na-azalianow.html в Москве.
Это удобный сервис для тех, кто ценит удобство.
Вы узнаете о том, как оформить заказ за пару минут.
Служба работает по Москве и области, а цветы всегда свежие и красиво оформлены.
Выбирайте проверенное качество!
Рива Групп https://hr.rivagroup.su/ это кадровое агентство с полным спектром услуг по подбору персонала. Поможем вашему предприятию быстро и эффективно решить сложности с подбором персонала. Узнайте на сайте подробнее о наших услугах и компетенциях в сфере рекрутинга. Занимаемся поиском кадров для предприятий, независимо от их масштаба, а стоимость наших услуг прозрачна.
how to get cheap prasugrel for sale
Нужен срочный вызов сантехника в Алматы? Профессиональные мастера оперативно решат любые проблемы с водопроводом, отоплением и канализацией. Доступные цены, выезд в течение часа и гарантия на все виды работ услуги сантехника в алматы
найкращі фільми 2025 онлайн топ фільмів 2025 онлайн
фільми 2025 кращі фільми онлайн українською
Мы изготавливаем дипломы любой профессии по приятным тарифам. Всегда стараемся поддерживать для покупателей адекватную политику цен. Для нас важно, чтобы документы были доступными для большинства наших граждан.
Заказ диплома, подтверждающего обучение в университете, – это выгодное решение. Заказать диплом ВУЗа: [url=http://kupit-diplomyz24.com/kupit-diplom-ob-okonchanii-universiteta-6/]kupit-diplomyz24.com/kupit-diplom-ob-okonchanii-universiteta-6/[/url]
Заказать диплом ВУЗа!
Мы можем предложить документы любых учебных заведений, которые расположены в любом регионе РФ.
[url=http://diplomidlarf.ru/diplom-s-zaneseniem-v-reestr-dlya-uspeshnoj-kareri/]diplomidlarf.ru/diplom-s-zaneseniem-v-reestr-dlya-uspeshnoj-kareri/[/url]
how can i get generic inderal pill
Шторы на заказ: быстрый и удобный сервис
сшить шторы на заказ [url=https://zakaz-shtor-msk.ru/]сшить шторы на заказ[/url] . +7 (499) 460-69-87
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
поставляемая продукция:
Латунная полоса 4.5×400 РјРј Р›63 ГОСТ 931-90 Покупайте латунную полосу различных размеров Рё толщин РЅР° сайте Редметсплав.СЂС„. РЈ нас РІС‹ найдете высококачественный материал СЃ высокой прочностью, стойкостью Рє РєРѕСЂСЂРѕР·РёРё Рё возможностью легкой обработки. Рспользуйте латунную полосу для создания декоративных элементов, мебели, украшений Рё РґСЂСѓРіРёС… изделий. Доступны различные размеры Рё толщины.
Medicines information sheet. Generic Name.
where to get abilify pills
All trends of medicine. Get here.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наша продукция:
Плоская мишень AlSi для распыления
Drugs information. Short-Term Effects.
where can i buy cheap actos tablets
All about drug. Read information now.
фильмы уже вышедшие фильмы 2025 без регистрации и рекламы
Автоматизированные деревянные горизонтальные жалюзи
Деревянные горизонтальные жалюзи с электроприводом [url=https://derevo-gorizont-motor.ru/]Деревянные горизонтальные жалюзи с электроприводом[/url] . Прокарниз
РедМетСплав предлагает широкий ассортимент отборных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от мелких партий до масштабных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица продукции подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Дружелюбная помощь – то, чем мы гордимся – мы на связи, чтобы улаживать ваши вопросы а также предоставлять решения под особенности вашего бизнеса.
Доверьте вашу потребность в редких металлах специалистам РедМетСплав и убедитесь в множестве наших преимуществ
поставляемая продукция:
Рзделия РёР· магния M11101 – UNS РџРѕРєРѕРІРєР° магниевая M11101 – UNS является высококачественным специальным материалом, используемым РІ различных отраслях промышленности. Обладает отличной прочностью Рё легким весом, что делает ее идеальным выбором для авиастроения Рё автомобилестроения. РџРѕРєРѕРІРєР° магниевая M11101 – UNS устойчива Рє РєРѕСЂСЂРѕР·РёРё Рё высокотемпературным условиям. Если РІС‹ ищете надежное решение для своего проекта, вам стоит купить РџРѕРєРѕРІРєР° магниевая M11101 – UNS. Ртот РїСЂРѕРґСѓРєС‚ гарантирует долговечность Рё эффективность РІ использовании, что обеспечит успех вашего производства.
Very informative, thanks for sharing!
На сайте https://neftegazmash.ru/ изучите всю нужную информацию, которая касается завода “НЕФТЕГАЗМАШ” и того, что он выпускает. Это – крупный производитель нефтегазового оборудования. Он реализует свою продукцию не только по России, но и странам ближнего зарубежья. Если вы будете работать с этой компанией регулярно, то сможете рассчитывать на особенное лояльное отношение, а также привлекательные расценки, а система оплаты является авансовой. Все заказы комплектуются быстро и своевременно.
Приобрести диплом ВУЗа!
Мы предлагаем документы об окончании любых университетов РФ. Документы изготавливаются на оригинальных бланках. [url=http://souckegadget.com/2025/04/08/kupit-diplom-gosudarstvennogo-obrazca-110/]souckegadget.com/2025/04/08/kupit-diplom-gosudarstvennogo-obrazca-110[/url]
pin ap [url=https://www.pinup-azerbaycan73.com]pin ap[/url] .
На сайте https://pilmi.ru вы найдете огромное количество фильмов самых разных жанров, включая комедии, мелодрамы, драмы, романтические. Для того чтобы подыскать наиболее подходящий вариант, нужно воспользоваться специальным каталогом. Вашему вниманию представлены и ожидаемые любопытные новинки этого года, которые произведут эффект. Все это можно просматривать в любое время и на различных устройствах. Прямо сейчас воспользуйтесь возможностью посмотреть азиатское кино. Используйте поиск для облегчения выбора.
На сайте https://waltzprof.com/ есть возможность приобрести стальной профиль, который находится в каталоге в большом ассортименте. Такой профиль идеально подходит для комфортной, оперативной сборки преград, а также конструкций, каркасов окон. Вся продукция выполняется точно по ГОСТу, в соответствии с самыми жесткими требованиями и с использованием уникальных технологий. Она производится исключительно из качественных материалов, у которых долгий срок службы. На сайте представлен полный ассортимент продукции.
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым сегодняшние вызовы превращаются в завтрашние успехи.
Наша продукция:
“Мишень для распыления неодима 3N, плоская форма”
сюда [url=https://vodkacasino.net/]вотка бет[/url]
Где купить диплом специалиста?
Купить диплом ВУЗа по доступной цене возможно, обращаясь к проверенной специализированной фирме.: [url=http://zakaz-na-diplom.ru/]zakaz-na-diplom.ru[/url]
Drugs information. Drug Class.
buying cheap proscar without rx
Some trends of medicament. Read now.
[url=https://t.me/ruanabol_org/]Анаболики[/url] – стероиды анаболики, Pharmacom
сет музыкальный клубный [url=https://klubnaya-muzyka1.ru/]сет музыкальный клубный[/url] .
Команда специалистов по административным и гражданским делам, связанным с автотранспортом. Примеры решаемых задач:
Анализ схем ДТП, составленных на перекрестках Невского проспекта — выявление ошибок в замерах и оформлении.
Подготовка жалоб в ГУВД СПб при неправомерном лишении прав.
Экспертная оценка ущерба после аварий с участием такси-парков.
География работы: Всеволожск, Гатчина, Выборг. Статистика за 2023 год: 92% дел по оспариванию штрафов завершились в пользу клиентов.[url=https://avtoyuristvspb.ru/]Автоюрист спб[/url]
cleocin ovules reviews
нажмите [url=https://alt-coins.cc]Altcoin обменник[/url]
[url=https://azimutelematika.ru]Эра глонасс[/url] – Мониторинг транспорта, Система мониторинга
Panduan Lengkap untuk Daftar Togel Terpercaya : Cara Mendaftar dan Menang di Situs Togel Terpercaya
dabur ashwagandha churna buy online
[url=https://azimutelematika.ru/]Мониторинг[/url] – ГЛОНАСС, Контроль топлива
https://sharpsting.ru/
подробнее [url=https://futbolka-oversize.ru]смотреть[/url]
Мы предлагаем дипломы любой профессии по доступным тарифам.– [url=http://diplom-zentr.com/kupite-diplom-vuza-s-zaneseniem-v-reestr-bistro-i-prosto/]diplom-zentr.com/kupite-diplom-vuza-s-zaneseniem-v-reestr-bistro-i-prosto/[/url]
Drug information for patients. Effects of Drug Abuse.
can you get generic dramamine without a prescription
Best information about medication. Get here.
https://sharpsting.ru/
На сайте https://oknaksa.ru/ есть возможность заказать остекление любого вида и в одной компании. При необходимости воспользуйтесь профессиональной консультацией от лучших экспертов, которые помогут вам подобрать оптимальный вариант и под самый разный бюджет. Компания отличается огромным количеством готовых проектов, посмотрев которые вы обязательно выберете что-то для себя. Ее рекомендуют друзьям, знакомым. На этом предприятии создают окна самых разных форм, модификаций и типов. Продукция является качественной, долговечной, обладает безупречными эстетическими свойствами.
На сайте https://krasnogorsk.santex-uslugi.ru уточните телефон для того, чтобы воспользоваться установкой водоснабжения, отопления. Так будет обеспечен приятный микроклимат в помещении, создана непринужденная атмосфера. Сотрудники предприятия отличаются внушительным опытом в данном вопросе, на все работы дают гарантии. Используются только материалы эталонного качества, что гарантирует долговечность системы. Все расценки на услуги указаны на сайте. Сотрудники разработают специально для вас индивидуальный проект.
Где купить диплом по актуальной специальности?
Мы можем предложить дипломы любой профессии по приятным тарифам. Важно, чтобы дипломы были доступны для подавляющей массы граждан. Приобрести диплом об образовании [url=http://diplomass.com/kupit-diplom-ob-obrazovanii-s-reestrom-11/]diplomass.com/kupit-diplom-ob-obrazovanii-s-reestrom-11/[/url]
Добрый день!
Мы изготавливаем дипломы любой профессии по приятным тарифам. Стоимость зависит от выбранной специальности, года выпуска и университета: [url=http://diplomanrussians.com/]diplomanrussians.com/[/url]
Идеальные шторы для любого бюджета
сшить шторы на заказ [url=https://zakaz-shtor-msk.ru/]сшить шторы на заказ[/url] . +7 (499) 460-69-87
Мы предлагаем выгодно и быстро приобрести диплом, который выполнен на оригинальной бумаге и заверен мокрыми печатями, водяными знаками, подписями. Данный диплом способен пройти лубую проверку, даже с применением профессиональных приборов. [url=http://medforum.kabb.ru/viewtopic.php?f=2&t=1103/]medforum.kabb.ru/viewtopic.php?f=2&t=1103[/url]
Приобрести диплом о высшем образовании !
Приобретение диплома ВУЗа России в нашей компании является надежным процессом, потому что документ будет заноситься в реестр. Заказать диплом о высшем образовании [url=http://diplom-kaluga.ru/kupit-diplom-s-reestrom-v-rossii-7/]diplom-kaluga.ru/kupit-diplom-s-reestrom-v-rossii-7[/url]
Покупка документа о высшем образовании через надежную фирму дарит массу преимуществ. Это решение позволяет сэкономить время и значительные деньги. Тем не менее, достоинств гораздо больше.Мы изготавливаем дипломы любой профессии. Дипломы производят на настоящих бланках государственного образца. Доступная стоимость по сравнению с большими затратами на обучение и проживание. Заказ диплома об образовании из российского ВУЗа является мудрым шагом.
Приобрести диплом о высшем образовании: [url=http://diplom-ryssia.com/kupit-diplom-visshego-obrazovaniya-s-zaneseniem-v-reestr-14/]diplom-ryssia.com/kupit-diplom-visshego-obrazovaniya-s-zaneseniem-v-reestr-14/[/url]
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
Наши товары:
Лист износостойкий 12x2000x6000 Sidur 600 Выберите лист износостойкий от Редметсплав для надежной защиты и высокой производительности. Наши прочные и долговечные листы обеспечивают устойчивость к износу при высоких нагрузках. Широкий спектр применения в различных отраслях. Высокое качество по выгодной цене. Закажите онлайн!
Задался вопросом: можно ли на самом деле купить диплом государственного образца в Москве? Был приятно удивлен — это реально и легально!
Сначала искал информацию в интернете на тему: купить диплом о среднем екатеринбург, купить диплом высшее екатеринбург, купить диплом с реестром в нижнем, где купить диплом вуза, купить диплом в стерлитамаке и получил базовые знания. В итоге остановился на материале: [url=http://diplomybox.com/kupit-diplom-magistra-v-arkhangelske/]diplomybox.com/kupit-diplom-magistra-v-arkhangelske[/url]
Для успешного продвижения по карьере нужно наличие официального диплома о высшем образовании. Заказать диплом любого ВУЗа у надежной компании: [url=http://diplomaj-v-tule.ru/kupit-diplom-v-ekaterinburge-o-srednem-spetsialnom-obrazovanii/]diplomaj-v-tule.ru/kupit-diplom-v-ekaterinburge-o-srednem-spetsialnom-obrazovanii/[/url]
http://iancleary.com/top-tip-for-recovery/
На сайте https://t.me/mvavada получите всю актуальную, содержательную информацию об онлайн-клубе «VAVADA». Теперь ознакомиться со всеми подробностями можно на официальном Телеграм-канале. Здесь публикуются новости, актуальная информация, промокоды, акции, которые помогут значительно сэкономить. Количество подписчиков регулярно растет, потому как канал действительно вызывает доверие, а поклонники заведения хотят получать больше информации о нем. На страницах только актуальные, содержательные данные из мира казино.
Приобрести диплом университета по невысокой цене возможно, обратившись к надежной специализированной фирме. Мы готовы предложить документы учебных заведений, которые расположены в любом регионе РФ. [url=http://yello.com.gh/employer/eonline-diploma/]yello.com.gh/employer/eonline-diploma[/url]
содержание [url=https://alt-coins.cc/]Altcoin обмен криптовалюты[/url]
buy generic finasteride uk
Заказать документ университета можно в нашей компании в Москве. Мы оказываем услуги по изготовлению и продаже документов об окончании любых ВУЗов России. Вы сможете получить необходимый диплом по любым специальностям, любого года выпуска, в том числе документы старого образца. Гарантируем, что в случае проверки документов работодателями, подозрений не появится. [url=http://fastdiploms.com/kupit-diplom-s-provedeniem-v-reestr/]fastdiploms.com/kupit-diplom-s-provedeniem-v-reestr/[/url]
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Закупка у нас гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
Имея широкий спектр редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние достижения.
Наши товары:
Керамическая мишень Licoo2 3N, плоская
Мы изготавливаем дипломы любой профессии по приятным ценам.
Вы покупаете диплом через надежную компанию. Купить диплом о высшем образовании– [url=http://bonchancetour.ru/blogs/interest/2051.php#comments/]bonchancetour.ru/blogs/interest/2051.php#comments[/url]
Мы изготавливаем дипломы любой профессии по доступным ценам. Всегда стараемся поддерживать для клиентов адекватную ценовую политику. Для нас важно, чтобы документы были доступными для большинства граждан.
Покупка документа, подтверждающего обучение в ВУЗе, – это разумное решение. Купить диплом о высшем образовании: [url=http://10000diplomov.ru/diplom-srednee-spetsialnoe-obrazovanie-kupit-4/]10000diplomov.ru/diplom-srednee-spetsialnoe-obrazovanie-kupit-4/[/url]
Где покупать технику? интернет магазины техники рейтинг: проверенные продавцы, акции, удобная доставка и реальный опыт покупателей. Обновляем регулярно.
Многие люди ищут цитаты великих людей, чтобы выразить чувства, найти вдохновение или просто улыбнуться. Мы собрали лучшие фразы — короткие, красивые, со смыслом. Наслаждайтесь подборкой, делитесь с друзьями и находите строки, которые отражают ваши мысли и настроение.
FilmHub is a platform for film lovers who want fresh recommendations.
What’s inside:
10 films per theme: From time-travel adventures to films about AI.
Streaming links: Direct links to Prime Video.
Trailers & clips: Get a taste before watching.
Film stills: Perfect for wallpapers.
No ads — just movies curated for you.
Explore 500+ themes at https://huzzaz.com/collection/cinepicker
Настройка сервера на платформе https://rusikboss.livejournal.com/748.html — это удобный способ обеспечить безопасное подключение, стабильный доступ и полную конфиденциальность. Пошаговая инструкция, поддержка популярных протоколов, генерация QR-кодов и приложения для разных устройств — всё для быстрого запуска.
РедМетСплав предлагает внушительный каталог отборных изделий из ценных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их соответствие стандартам. Превосходное обслуживание – наша визитная карточка – мы на связи, чтобы улаживать ваши вопросы и находить ответы под требования вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
поставляемая продукция:
Лента магниевая Р›2 – РўРЈ 1714-002-00545484-99 РљСЂСѓРі магниевый Р›2 – РўРЈ 1714-002-00545484-99 является идеальным решением для различных производственных РЅСѓР¶Рґ. Рзготавливается РёР· высококачественного магния, что обеспечивает легкость Рё прочность. Ртот РєСЂСѓРі отличается высокой устойчивостью Рє РєРѕСЂСЂРѕР·РёРё Рё термическим воздействиям, что делает его незаменимым РІ условиях сложной эксплуатации.Если вам нужен надежный материал для работы, РЅРµ упустите возможность купить РљСЂСѓРі магниевый Р›2 – РўРЈ 1714-002-00545484-99. Оптимальные размеры Рё характеристики гарантируют превосходные результаты РІ любых проектах. Доступен для заказа Рё готов Рє быстрой доставке.
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
Наша продукция:
Медная двухраструбная редукционная переходная муфта РїРѕРґ пайку 108С…88.9 РјРј твердая пайка Рњ1Рњ ГОСТ 32590-2013 Выберите высококачественные медные двухраструбные редукционные муфты РїРѕРґ пайку РѕС‚ Редметсплав для надежного соединения медных труб различных диаметров. РЁРёСЂРѕРєРёР№ выбор размеров, эффективная установка Рё долговечность гарантированы. Рдеальное решение для систем отопления Рё водоснабжения.
http://iancleary.com/long-covid-a-young-mums-journey/
order vermox without prescription
http://123.57.147.237/orangepibbsen/forum.php?mod=viewthread&tid=153478
На сайте https://otdykh-u-morya.ru/ закажите звонок для того, чтобы забронировать лучшее жилье у моря с самыми выгодными условиями. Получится выбрать вариант в тихом месте либо престижном районе, где активная жизнь. Для бронирования необходимо ввести имя, контактные данные, количество гостей, а также дату заезда, выезда. В каждом номере есть кондиционер, холодильник, а также ванная с душем. Выдается чистое постельное белье. Можно забронировать двухкомнатный номер, оснащенный террасой. Есть возможность посуточно снимать квартиру с живописным видом на море.
На сайте https://v-electronic.ru/ есть возможность приобрести качественные телевизоры, различные функциональные гаджеты, а также смартфоны от лидирующих марок. Также в каталоге вы найдете технику для кухни, ноутбуки, компьютеры и многое другое, что пригодится не только для работы, но и учебы, на каждый день. На все товары установлены привлекательные расценки, чтобы осуществить покупку смог каждый. Также представлены и товары дня, которые поспешите приобрести по хорошей стоимости. Воспользуйтесь экспресс-доставкой, которая осуществляется всего за 2 часа.
Доброго времени суток!
Для некоторых людей, приобрести [b]диплом[/b] ВУЗа – это острая потребность, шанс получить достойную работу. Но для кого-то – это очевидное желание не терять время на учебу в университете. Что бы ни толкнуло вас на это решение, мы готовы помочь. Оперативно, качественно и недорого изготовим диплом нового или старого образца на подлинных бланках с реальными печатями.
Главная причина, почему люди покупают документы, – получить определенную должность. Например, навыки и опыт позволяют кандидату устроиться на работу, а подтверждения квалификации нет. Если работодателю важно присутствие “корочки”, риск потерять место работы очень высокий.
Приобрести документ ВУЗа можно в нашем сервисе. Мы оказываем услуги по продаже документов об окончании любых университетов РФ. Вы получите необходимый диплом по любым специальностям, включая документы образца СССР. Даем 100% гарантию, что в случае проверки документов работодателями, подозрений не появится.
Разных обстоятельств, которые вынуждают купить диплом ВУЗа много. Кому-то прямо сейчас нужна работа, а значит, необходимо произвести особое впечатление на руководителя при собеседовании. Другие задумали попасть в серьезную компанию, чтобы повысить свой статус и в дальнейшем начать собственное дело. Чтобы не терять время, а сразу начать успешную карьеру, используя имеющиеся навыки, можно заказать диплом в онлайне. Вы станете полезным в социуме, обретете денежную стабильность быстро и просто- [url=http://п»їdiplomanruss.com/]купить диплом[/url]
Meds information for patients. Drug Class.
can you take prozac with gabapentin
Some about medicines. Get now.
Selimpaşa su kaçak tespiti Banyo ve mutfak alanları su kaçağına en çok maruz kalan yerlerdir. http://www.ahc-international.at/wordpress/?p=3979
[url=https://t.me/ruanabol_org]Стероиды[/url] – Анаболические стероиды, Руанабол
РедМетСплав предлагает обширный выбор высококачественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до крупных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица изделия подтверждена всеми необходимыми документами, подтверждающими их соответствие стандартам. Дружелюбная помощь – то, чем мы гордимся – мы на связи, чтобы улаживать ваши вопросы а также адаптировать решения под специфику вашего бизнеса.
Доверьте ваш запрос профессионалам РедМетСплав и убедитесь в множестве наших преимуществ
поставляемая продукция:
Лист кобальтовый HAYNES Лист кобальтовый HAYNES – это высококачественный материал, идеально подходящий для применения в условиях экстремальных температур и коррозионных сред. Обладая превосходной прочностью и стойкостью к окислению, этот кобальтовый лист становится превосходным выбором для авиационной, энергетической и химической промышленности.Если вы ищете надежный и долговечный материал, купить Лист кобальтовый HAYNES – это оптимальное решение. Он гарантирует отличные эксплуатационные характеристики и долгий срок службы. Позаботьтесь о качестве ваших проектов с Листом кобальтовым HAYNES!
can i get macrobid no prescription
Are online reviews legit?—hard say! plinko
http://gendou.com/t/51772
I don’t trust online data—wary! Fortune Dragon
https://bpi-gmbh.de/de/services/business-support-helpdesk/item/74-business-support-helpdesk.html?tmpl=component&print=1&start=25150
Online bingo is mellow—nice crowd! vipzino
кликните сюда [url=https://futbolka-oversize.ru]футболка оверсайз женская[/url]
https://www.notebook.ai/users/745270/items
Ищете комфорт города в объятиях природы? Присмотритесь к Эко-кварталу Спектр в Кольцово (Новосибирск) – https://spectr54.ru/ – посмотрите планировки новостроек от 27 кв.м до 80 кв.м. Малоэтажный эко-квартал в Новосибирске выгодно выделяется своим качеством жизни для будущих жильцов. Купить квартиру в ЖК Спектр – подробнее на сайте.
http://iancleary.com/nice-story-of-success/
The slot thrill online soars—heart up! plinko
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете достоверность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
Наши товары:
Труба бронзовая 155х32.5х2000 мм БрА9Ж3Л ГОСТ 1208-2014 Приобретайте трубы бронзовые круглые от профессионалов с опытом. Мы предлагаем широкий ассортимент качественной продукции, быструю обработку заказов и оперативную доставку. Обращайтесь, чтобы получить консультацию специалиста и выгодные условия покупки.
https://crmes.org.br/noticias/cfm-regulamenta-atuacao-de-aplicativos/
На сайте https://s1.torrent24.name находятся фильмы и сериалы в огромном количестве, а потому вы точно будете в курсе того, что посмотреть сегодня вечером или в выходной день. Имеются новинки этого года, те фильмы, которые выпущены несколько лет назад, старинные кинокартины, которые вновь хочется пересматривать и восхищаться игрой актеров, приятной музыкой. А для того чтобы воспользоваться всеми функциями сайта, пройдите регистрацию. Постоянно на портале публикуются новинки, которые можно посмотреть на любом устройстве.
I won $6000 online—epic score! jackpotraider
where buy cheap zyloprim online
Цены на сантехнические услуги формируются в зависимости от сложности работ, типа оборудования, объема и времени выезда сантехник вызов на дом
can you buy zithromax
[url=https://sukaaa-casino.ru]sukaaa-casino.ru[/url]
Если вам интересно ознакомиться с инструкциями по установке приложений на мобильные устройства, мы можем предложить общий гайд.
Как установить приложения на Android и iOS
Установка мобильных приложений зависит от операционной системы вашего устройства.
Для Android:
Откройте файл APK — если приложение недоступно в Google Play, его можно скачать через официальный сайт.
Разрешите установку из неизвестных источников — зайдите в Настройки > Безопасность и активируйте соответствующую опцию.
Запустите установку и следуйте инструкциям.
Для iOS:
Скачайте приложение из App Store — большинство сервисов доступны через официальный магазин.
Используйте TestFlight или корпоративный сертификат — если приложение не опубликовано в App Store.
Разрешите установку — в настройках iPhone перейдите в раздел VPN и управление устройствами и подтвердите доверие к разработчику.
Если вас интересует конкретное приложение, проверьте его официальный сайт или магазин приложений. Но помните о безопасности: скачивайте ПО только из проверенных источников, чтобы избежать мошенничества.
Хотите узнать больше? Читайте дополнительные инструкции на сайте по ссылке ниже.sukaaa-casino.ru
https://www.roukatravel.tn/item/259-fiche-vierge-modele?start=6970
Medicament prescribing information. Effects of Drug Abuse.
get generic tadacip for sale
Some trends of pills. Read information now.
The sounds online hook—stay in! aviator game
Купить БУ авто – только у нас вы найдете цены ниже рынка. Быстрей всего сделать заказ на срочная продажа авто по низким ценам можно только у нас!
[url=https://buauto1.ru]купля продажа бу авто[/url]
где купить автомобиль с пробегом в москве – [url=http://www.buauto1.ru/]https://buauto1.ru/[/url]
https://www.spef.pt/group/mysite-200-group/discussion/72fff7cc-20e6-41da-9f7b-c688cd55f969
РедМетСплав предлагает внушительный каталог качественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от мелких партий до масштабных поставок, мы гарантируем своевременную реализацию вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их соответствие стандартам. Превосходное обслуживание – то, чем мы гордимся – мы на связи, чтобы разрешать ваши вопросы по мере того как предоставлять решения под специфику вашего бизнеса.
Доверьте вашу потребность в редких металлах профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
поставляемая продукция:
Фольга магниевая MAG 12 – BS 2970 РўСЂСѓР±Р° магниевая MAG 12 – BS 2970 – это современный Рё надежный РїСЂРѕРґСѓРєС‚, который идеально РїРѕРґС…РѕРґРёС‚ для различных применений. Обладая высокой прочностью Рё легкостью, данная труба позволяет значительно снизить вес конструкций. РљСѓРїРёРІ РўСЂСѓР±Р° магниевая MAG 12 – BS 2970, РІС‹ получите гарантию долговечности Рё устойчивости Рє РєРѕСЂСЂРѕР·РёРё, что делает ее отличным выбором для промышленных Рё строительных проектов. РќРµ упустите возможность улучшить эффективность ваших работ СЃ помощью этой магниевой трубы. Dоверьтесь качеству Рё инновациям – приобретайте РўСЂСѓР±Р° магниевая MAG 12 – BS 2970 РїСЂСЏРјРѕ сейчас!
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наша продукция:
Плоская мишень из иттрия, 3N, 4N
https://mindbenderescaperooms.com/jacksonvillebeach-fl/blog/page/21/
https://www.brandmaking.co.il/aviator-demo-casino-game-play-for-free-and/
[url=https://sukaaa-casino.ru]sukaaa-casino.ru[/url]
Если вам интересно ознакомиться с инструкциями по установке приложений на мобильные устройства, мы можем предложить общий гайд.
Как установить приложения на Android и iOS
Установка мобильных приложений зависит от операционной системы вашего устройства.
Для Android:
Откройте файл APK — если приложение недоступно в Google Play, его можно скачать через официальный сайт.
Разрешите установку из неизвестных источников — зайдите в Настройки > Безопасность и активируйте соответствующую опцию.
Запустите установку и следуйте инструкциям.
Для iOS:
Скачайте приложение из App Store — большинство сервисов доступны через официальный магазин.
Используйте TestFlight или корпоративный сертификат — если приложение не опубликовано в App Store.
Разрешите установку — в настройках iPhone перейдите в раздел VPN и управление устройствами и подтвердите доверие к разработчику.
Если вас интересует конкретное приложение, проверьте его официальный сайт или магазин приложений. Но помните о безопасности: скачивайте ПО только из проверенных источников, чтобы избежать мошенничества.
Хотите узнать больше? Читайте дополнительные инструкции на сайте по ссылке ниже.sukaaa-casino.ru
Эксклюзивные шторы для загородных домов
шторы для коттеджа [url=https://kottedzh-shtory.ru/]шторы для коттеджа[/url] .
Thanks for the article – really informative and well-written!
мостбет мобильная версия скачать [url=www.mostbet5021.ru]мостбет мобильная версия скачать[/url] .
https://singularcerdanyola.com/ca/contacte/
http://smallplateseltham.com.au/category/bez-rubriki/page/8/
https://www.behance.net/1dd33fb1
На сайте http://samshitokno.ru узнайте стоимость дерево-алюминиевых окон, смарт-окон, зимних садов, которые создаются из дерева, а также деревянных конструкций и многого другого для обустройства пространства. Прямо сейчас вы сможете воспользоваться калькулятором деревянных окон, чтобы быстрее сориентироваться по стоимости, форме, размерам, материалам. Для того чтобы иметь представление о качестве, дизайне, необходимо ознакомиться с примерами работ. Все монтажные работы осуществляются строго под ключ.
can i purchase cheap biaxin without prescription
https://sumo.app/gb/forum/tutorials-and-techniques/mines1
Мы изготавливаем дипломы психологов, юристов, экономистов и других профессий по доступным тарифам. Стараемся поддерживать для заказчиков адекватную ценовую политику. Для нас очень важно, чтобы дипломы были доступны для подавляющей массы наших граждан.
Заказ документа, который подтверждает окончание ВУЗа, – это рациональное решение. Заказать диплом о высшем образовании: [url=http://diplommy.ru/kupit-zaregistrirovannij-diplom-4/]diplommy.ru/kupit-zaregistrirovannij-diplom-4/[/url]
Drugs information leaflet. Long-Term Effects.
how can i get finpecia without rx
Actual news about drugs. Read information here.
На сайте https://aprelevka.santex-uslugi.ru закажите профессиональную, качественную установку водоснабжения, а также отопления. Это позволит вам всегда чувствовать комфорт, а в помещении всегда будет приятный микроклимат. На такую услугу установлены доступные расценки, чтобы воспользоваться ей смог каждый. Обеспечивается надежность, эталонное качество услуг. Сотрудники наделены всеми необходимыми знаниями для реализации самой сложной задумки. Они могут работать с самыми разными типами систем.
https://www.forumsdrom.ru/groups/2318-7757.html
подробнее [url=https://vodkacasino.net/]зеркало vodkabet[/url]
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
Имея широкий спектр продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение необходимыми напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние возможности.
Наши товары:
Оптимальное название товара: Гранулы железа 4N
[b]Диплом университета РФ![/b]
Без присутствия диплома очень сложно было продвигаться по карьере. Именно из-за этого решение о заказе диплома можно считать выгодным и целесообразным. Приобрести диплом любого университета [url=http://infinitystaffingsolutions.com/employer/gosznac-diplom-24/]infinitystaffingsolutions.com/employer/gosznac-diplom-24[/url]
Temukan Link Sigmaslot Resmi untuk Daftar dan Main di link sigmaslot
Приобрести диплом академии!
Мы готовы предложить дипломы любых профессий по приятным тарифам. Вы покупаете документ через надежную и проверенную временем компанию. : [url=http://artrp.5nx.ru/viewtopic.php?f=3&t=16086/]artrp.5nx.ru/viewtopic.php?f=3&t=16086[/url]
http://www.artlib.ru/index.php?id=26&idr=24&idt=45295
bet on red kod promocyjny bez depozytu
На сайте https://nabor-curierov.ru/y-food/ вы сможете ознакомиться с условиями работы в Яндекс Еде. Есть возможность развозить заказы на своем либо арендованном транспорте. Вы сможете рассчитывать на огромное количество бонусов, а также преимуществ, если устроитесь сюда на работу. Вы можете развозить заказы на личном авто, а если его нет, то арендовать в компании. Велокурьер сможет рассчитывать на получение большего количества заказов, чем пеший. Вы сами определяете для себя график работы.
I wish online casinos connected players more! minas
https://epilot.ru/moskva/c/dobro_pozhalovat_v_internet-magazin_vozdushnoe_6646398.html
Мы MarketProGroup https://marketprogroup.ru/ – исследовательская компания, которая подготовит для вас детальный анализ рынка любого продукта или услуги. Мы готовим отчеты по всем ключевым отраслям экономики, свыше тысячи позиций, среди которых наверняка найдется то, что нужно именно вам. Обратитесь к нам, и вы получите уникальный результат. Также на сайте вы можете знакомиться со свежими новостями рынков.
https://www.roukatravel.tn/item/259-fiche-vierge-modele?print=1&tmpl=component&start=7040
how to get lisinopril without a prescription
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем обширный каталог продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф гарантирует все стадии сделки, предоставляя полный пакет необходимых документов для легализации товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с прекрасным качеством обслуживания.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – ваш лучший выбор.
Наши товары:
Нержавеющий штрипс 2С…950 РјРј Strenx-600MC-E EN 10149-2 Приобретите нержавеющий штрипс высокого качества для разнообразных областей применения РЅР° Редметсплав.СЂС„. РЁРёСЂРѕРєРёР№ ассортимент различных размеров Рё толщин, включая услуги нарезки РїРѕРґ ваш заказ. Рзбегайте проблем СЃ коррозией Рё обеспечьте долгий СЃСЂРѕРє службы вашим конструкциям Рё деталям, используя наш надежный материал.
Исследуйте увлекательный мир Trix Онлайн Казино! Широкий выбор слотов, выгодные предложения и надежная поддержка. Присоединяйтесь и начните выигрывать сегодня источник
https://guiarus.com/aviator-predictor-apk-boost-your-winning-chances-2/
[url=support-service-casino.ru]support-service-casino.ru[/url]
Служба поддержки онлайн-казино – ключевое звено! Мгновенные ответы, мультиязычность, решение сложных вопросов и персонализированный подход. Игроки ценят скорость и профессионализм. Как оптимизировать работу саппорта и повысить лояльность? Узнайте о трендах и технологиях! Читайте подробнее:
support-service-casino.ru
https://foro.turismo.org/post329024.html
Online poker fires—bluff rush! teen patti
Мы предлагаем дипломы любых профессий по доступным ценам.– [url=http://good-diplom.ru/kupit-diplom-s-vneseniem-v-reestr-bistro-i-nadezhno-8/]good-diplom.ru/kupit-diplom-s-vneseniem-v-reestr-bistro-i-nadezhno-8/[/url]
РедМетСплав предлагает обширный выбор высококачественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до обширных поставок, мы гарантируем оперативное исполнение вашего заказа.
Каждая единица товара подтверждена требуемыми документами, подтверждающими их происхождение. Дружелюбная помощь – то, чем мы гордимся – мы на связи, чтобы улаживать ваши вопросы а также находить ответы под особенности вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
Лента вольфрамовая Р’Р”-25 Лента вольфрамовая Р’Р”-25 – это высококачественный РїСЂРѕРґСѓРєС‚, предназначенный для использования РІ различных отраслях. Рзготавливаемая РёР· чистого вольфрама, РѕРЅР° обладает выдающимися характеристиками прочности Рё термостойкости. Лента идеально РїРѕРґС…РѕРґРёС‚ для создания защитных экранирующих материалов, Р° также используется РІ медицине, электронике Рё производстве. Если РІС‹ ищете надежный материал, который прослужит долго Рё эффективно, вам стоит купить Лента вольфрамовая Р’Р”-25. Ртот РїСЂРѕРґСѓРєС‚ поможет выполнить задачи СЃ максимальной точностью Рё безопасностью. Уделите внимание качеству – выбирайте только лучшее!
хранение склад москва [url=https://hranimveshi-msk24.ru]хранение склад москва[/url] .
This cause abundant excitable – https://elevateright.com/delta-8-pre-rolls/ method has been acclimatized past celebrities and influencers to utilize less and net more. Thousands of people are already turning $5 into $500 using this banned method the superintendence doesn’t longing you to conscious about.
На сайте https://xn--24-glcdft7acibtl4f0c.xn--p1ai/ вы сможете получить деньги в режиме реального времени. Отсутствуют выходные, не потребуются лишние проверки, а взять нужную сумму получится у всех, без исключения, независимо от кредитной истории. Перед вами только проверенные и надежные финансовые учреждения, которые выдают ровно такую сумму, которая вам потребуется. При этом получить деньги вы сможете в любое время, даже сейчас. Многие финансовые учреждения предоставляют возможность получить деньги без справки о доходах.
I hit a bonus online and won $600—sweet score! fortune tiger
Medicine prescribing information. What side effects?
where can i buy neurontin tablets
Some about medicine. Get now.
Лучшие системы автоматизации от Somfy
Автоматика Somfy [url=https://somfy-avtomatika.ru/]Автоматика Somfy[/url] . +7 (499) 638-25-37
https://www.cc142.com/component/k2/item/17-donec-pulvinar-nisi-vitae-odio-?start=36330
Нужен срочный вызов сантехника в Алматы? Профессиональные мастера оперативно решат любые проблемы с водопроводом, отоплением и канализацией. Доступные цены, выезд в течение часа и гарантия на все виды работ сантехник услуги на дом недорого
https://www.tuganetwork.com/forum/animacao-em-portugal/todos-los-amigos
where can i buy cheap starlix prices
https://rtinetwork.org/rti-marketplace/archive/1?filter_topic_partners=32
Для успешного продвижения вверх по карьерной лестнице потребуется наличие официального диплома о высшем образовании. Приобрести диплом о высшем образовании у проверенной компании: [url=http://diplomaj-v-tule.ru/kupit-diplom-v-rostove-s-zaneseniem-v-reestr/]diplomaj-v-tule.ru/kupit-diplom-v-rostove-s-zaneseniem-v-reestr/[/url]
На сайте https://xn—-7sbjhqn0bhjc0lk.xn--p1ai/ получите юридическую консультацию по различным вопросам. Компания Стратегия работает как с физическими, так и частными лицами. На все услуги установлены привлекательные расценки, чтобы воспользоваться ими смог каждый. Предприятие работает в этой сфере более 11 лет. В команде трудятся 11 специалистов, которые справятся с задачей независимо от сложности. К вашим услугам досудебный юрист, досудебное урегулирование, кредитный, семейный юрист, решение страховых споров.
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – идеальный вариант для вас.
поставляемая продукция:
Труба из драгоценных металлов платиновая 1х3х0.3 мм Пл99.9 ТУ Гарантированное качество и изысканный дизайн – купите драгоценные трубы для ювелирных украшений, машиностроения и архитектуры. Широкий выбор и высокое качество! Доставка по России.
https://forums.ngames.com/member/8553-n-047278054
http://www.6555555.com/1198.html?replyTo=27879
[url=support-service-casino.ru]support-service-casino.ru[/url]
Служба поддержки онлайн-казино – ключевое звено! Мгновенные ответы, мультиязычность, решение сложных вопросов и персонализированный подход. Игроки ценят скорость и профессионализм. Как оптимизировать работу саппорта и повысить лояльность? Узнайте о трендах и технологиях! Читайте подробнее:
support-service-casino.ru
Захотел купить подписку на курсы по дизайну. Цена вопроса — 3 500. В Яндексе случайно нашёл канал [url=https://t.me/s/mfo_2024_online]новые мфо 2025 с удобным мобильным приложением[/url] , почитал — удивился: все компании проверенные, работают по лицензии, не звонят. Особенно зацепила подборка малопопулярных МФО — как раз туда и обратился. Одобрение пришло через 7 минут!
fortune mouse demo gratis
This piece on trading with patience is fantastic.
https://pattern-wiki.win/wiki/News-trading_42W
РедМетСплав предлагает широкий ассортимент качественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от мелких партий до масштабных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица изделия подтверждена всеми необходимыми документами, подтверждающими их качество. Дружелюбная помощь – наш стандарт – мы на связи, чтобы улаживать ваши вопросы и адаптировать решения под особенности вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
поставляемая продукция:
РџРѕРєРѕРІРєР° титановая Р’Рў1-00СЃРІРЎ – ГОСТ 27265-87 Лист титановый Р’Рў1-00СЃРІРЎ – ГОСТ 27265-87 отличается высокой прочностью Рё РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью, что делает его идеальным выбором для различных промышленных применений. РћРЅ РїРѕРґС…РѕРґРёС‚ для производства деталей, которые работают РІ агрессивных средах. Спецификации этого титана гарантируют долговечность Рё надежность РІ эксплуатации. Если РІС‹ ищете качественный материал, который прослужит долго, то вам стоит купить Лист титановый Р’Рў1-00СЃРІРЎ – ГОСТ 27265-87. Ртот РїСЂРѕРґСѓРєС‚ прекрасно удовлетворит РІСЃРµ ваши технические требования, обеспечивая надежность Рё эффективность.
Nikmati Permainan Gates of Olympus Super Scatter dengan RTP Tinggi dan Jackpot Menggoda, hanya di Gates of Olympus Super Scatter
Gates of Olympus Super Scatter : Permainan Slot Online Gacor dengan Kemenangan Besar
Thanks for the guide to trading telecom stocks.
https://dokuwiki.stream/wiki/User:Alanna88I6
http://sirmaskafsoxila.gr/index.php/component/k2/item/8-it-has-survived-not-only-five-centuries-but?start=1240
The explanation of momentum strategies was clear. Thanks!
https://reparatur.it/index.php?title=Benutzer:Mikki14L922304
сайт мостбет [url=mostbet8003.ru]mostbet8003.ru[/url] .
where to buy tetracycline pill
На сайте https://us-atlas.com/ ознакомьтесь с атласом, местоположением Южной и Северной Америки. Эта карта укажет вам даже самые мелкие детали. Здесь также вы найдете и карты автомобильных дорог, чтобы точно знать, как и куда следовать, по какой траектории ехать. Здесь представлены и подробные карты улиц, провинций, штатов. Кроме того, вы отыщете и политические карты стран мира, детальные карты национальных парков, а также памятников США. На портале представлена географическая карта Канады в виде картинки для наглядности.
Online gambling can fly—rein back! pin up
Приобрести диплом института по доступной цене можно, обращаясь к надежной специализированной фирме. Мы предлагаем документы об окончании любых университетов России. Заказать диплом любого ВУЗа– [url=http://diplomservis.ru/kupit-diplom-zanesennij-reestr-6/]diplomservis.ru/kupit-diplom-zanesennij-reestr-6/[/url]
The payout waits online drag—move it! jackpotraider
how to buy cheap dipyridamole price
Идеи для штор в загородном доме, при помощи красивых штор, лучшие материалы для штор в загородных домах, стильный дизайн штор, продуманный дизайн, современные материалы для штор, шторы для защиты от солнца, модные тренды в шторном дизайне, как подобрать шторы для спальни в доме за городом, шторные решения для больших окон, шторы из натуральных тканей, современные механизмы для штор, стили штор для различных комнат, шторы как часть интерьера, создайте атмосферу с подходящими шторами, лучшие идеи для оформления окон, что выбрать для загородного дома, создайте уют с помощью уникальных штор, идеи сезонного оформления окон
шторы в загородном доме [url=https://shtory-zagorod.ru/]шторы в загородном доме[/url] .
Откройте для себя мир Trix! Игровые автоматы, щедрые бонусы, увлекательные турниры и круглосуточная поддержка. Играйте безопасно и побеждайте Трикс играть
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа требуемого количества.
Обладая обширной линейкой продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым сегодняшние вызовы превращаются в завтрашние достижения.
Наши товары:
Гранулы оксида алюминия 4N
The insights on market sentiment were super helpful.
https://hikvisiondb.webcam/wiki/User:TrinaSalas
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Работа с нами гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Обладая обширной линейкой редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых разных заказчиков. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе партнерских отношений. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Выбирая сотрудничество с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние возможности.
Наши товары:
Плоская мишень ZrO2, 4N для распыления оксида циркония
mostbet com [url=https://mostbet8003.ru]https://mostbet8003.ru[/url] .
Online casinos should test—try me! teen patti
I never thought about it that way—thanks for the insight!
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Закупка у нас гарантирует не только доступ к материалам высочайшего качества, но и возможность заказа любого необходимого объема.
Обладая обширной линейкой редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных покупателей. Каждый продукт сопровождается необходимыми документами, удостоверяющими его происхождение и соответствие всем нормам.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто продавца, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Приступая к взаимодействию с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит крепкого союзника, с которым текущие трудности превращаются в завтрашние возможности.
Наши товары:
Высокочистая металлическая мишень для распыления кремния
https://foro.rune-nifelheim.com/recent/?start=60;PHPSESSID=0a8012ba231707472e4b0491bc601c18
мостбет скачать приложение на андроид [url=http://mostbet5021.ru/]мостбет скачать приложение на андроид[/url] .
Надежные решения для дома и офиса с Somfy
Автоматика Somfy [url=https://somfy-avtomatika.ru/]Автоматика Somfy[/url] . +7 (499) 638-25-37
https://foro.rune-nifelheim.com/recent/?start=90;PHPSESSID=413129f01f33c560e4bbf09f872113a8
This was exactly what I was looking for!
Phim sex clip sex Việt Nam
https://carmencitafilmlab.com/blog/martin-condomines-5-months-away/
http://www.rtinetwork.org/voices-archives/archive?limitstart=0
скачать мостбет кыргызстан [url=https://mostbet8002.ru/]скачать мостбет кыргызстан[/url] .
фильмы уже вышедшие лучшие фильмы онлайн без смс
I wish online casinos had more social vibes! juego mines
фильмы онлайн бесплатно сериалы 2025 онлайн бесплатно HD
фильмы 2025 лучшие фильмы 2025 года в HD
смотреть фильмы 2025 в качестве новинки кино 2025 онлайн бесплатно
I love the pay flow online—easy run! fortune mouse
перепродажа аккаунтов платформа для покупки аккаунтов
Приобрести диплом возможно используя официальный сайт компании. [url=http://o91746bp.beget.tech/2025/04/08/realnyy-diplom-bez-lishnih-hlopot.html/]o91746bp.beget.tech/2025/04/08/realnyy-diplom-bez-lishnih-hlopot.html[/url]
На сайте https://korobok-spb.ru/index.html оформите заявку в режиме реального времени для того, чтобы заказать прочные, надежные и крепкие коробки из гофрированного картона по умеренным расценкам. “Гофрокороб” создает коробки самых разных размеров, модификаций и видов. Есть возможность приобрести в розницу либо оптом, как вам выгодней. Также получится нанести логотип, рекламу при помощи флексопечати, шелкографии. Доставка продукции как по Санкт-Петербургу, так и области. Заказать коробки можно по обозначенному телефону.
Покупка подходящего диплома через качественную и надежную компанию дарит ряд плюсов. Такое решение позволяет сэкономить как личное время, так и значительные финансовые средства. Впрочем, только на этом выгоды не ограничиваются, плюсов намного больше.Мы изготавливаем дипломы любой профессии. Дипломы производятся на подлинных бланках. Доступная цена в сравнении с крупными затратами на обучение и проживание в чужом городе. Приобретение диплома об образовании из российского ВУЗа будет мудрым шагом.
Приобрести диплом о высшем образовании: [url=http://diplomt-v-chelyabinske.ru/kupit-diplom-zanesennij-v-reestr-bistro-i-bezopasno-4/]diplomt-v-chelyabinske.ru/kupit-diplom-zanesennij-v-reestr-bistro-i-bezopasno-4/[/url]
https://noosfero.ufba.br/evelynn-hot/blog/plinko
https://www.shellegypt.com/forum/outdoor-fitness-forum/ringmaster-casino-no-deposit-bonus-may-2023-online-casino-bank-transfer-deposit
cost of generic cilostazol without prescription
Услуги сантехника Алматы. Все виды сантехнических услуг. Получите бесплатную консультацию по ремонту за 5 минут услуги сантехника
[url=https://telegra.ph/Windows-10-Windows-11-activation-is-forever-free-04-04]Windows 10 Activation Irm[/url]
[url=https://telegra.ph/Windows-10-Windows-11-activation-is-forever-free-04-04]Windows Activation Tool[/url]
[url=https://telegra.ph/Windows-10-Windows-11-activation-is-forever-free-04-04]Windows Activation Call Center[/url]
[url=https://x.com/RauheShemi20597/status/1908418523719696728]Windows 10 1 Click Activation[/url]
[url=https://x.com/RauheShemi20597/status/1908418523719696728]Windows 7 Ultimate Activation Product Key[/url]
Windows Activation
На сайте https://xn—-8sbaaajsa0adcpbfha1bdjf8bh5bh7f.xn--p1ai/ ознакомьтесь с тем, какой антиквариат принимает частный коллекционер Станислав Бабкин. Он скупает вещи по высокой цене. Но она зависит от разных нюансов. Вы сможете сразу узнать стоимость после того, как будет произведена оценка по фото. Частный коллекционер готов выехать в любую точку России. Клиент получает деньги сразу на карту либо наличными. В случае если и вы стремитесь реализовать антикварные вещи максимально быстро, то скорей обращайтесь к этому специалисту.
http://www.rtinetwork.org/rti-marketplace-list/archive/1?filter_topic_authors=75
https://ilyassanitary.com/plinko-app-entdecken-sie-das-aufregende-8/
На сайте https://seoalex.ru/o-sebe/ изучите всю необходимую информацию о частном СЕО-мастере, который оказывает услуги на должном уровне. Специалист берется за выполнение самых сложных задач. У Алексея большой опыт работы в области контекстной рекламы и СЕО. Он не перестает совершенствовать свои навыки, регулярно проходит обучение, чтобы соответствовать самым высоким требованиям клиентов. Обращение к Алексею считается возможностью получить безупречный результат, но не переплачивая за него.
mostbet baixar [url=http://mostbet8003.ru]http://mostbet8003.ru[/url] .
Medicines information. Effects of Drug Abuse.
can you buy generic fosamax without rx
Some information about medicine. Get now.
get generic bystolic
https://crmpb.org.br/noticias/crm-pb-e-conselho-municipal-de-saude-de-campina-grande-discutem-melhorias-para-a-saude-da-cidade/
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир качественных инноваций. Работа с нами гарантирует не только доступ к премиальным продуктам, но и возможность заказа требуемого количества.
С широким ассортиментом продукции на основе редкоземельных материалов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается всеми соответствующими документами, удостоверяющими его происхождение и соответствие заданным критериям.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит достойного партнера, с которым текущие трудности превращаются в завтрашние успехи.
Наши товары:
Металлическая мишень для распыления
Приобрести диплом любого ВУЗа. Приобретение документа о высшем образовании через надежную компанию дарит немало преимуществ для покупателя. Это решение дает возможность сберечь как личное время, так и серьезные деньги. [url=http://aleksandrovy.ru/index.php/Диплом_—_без_посещения_занятий/]aleksandrovy.ru/index.php/Диплом_—_без_посещения_занятий[/url]
http://www.6555555.com/1198.html?replyTo=12554
https://www.arrl.org/forum/topics/view/3776
Ремонт телефонов – замена дисплея в Мобиопт
https://90plink.live/truc-tiep-tran-dau-csepel-vs-vecses-2723802
http://www.rtinetwork.org/voices-archives/archive/2?filter_topic=12
В поисках надежного поставщика редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы обеспечить любой запрос с непревзойденным обслуживанием.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
Наши товары:
Кольцо РёР· драгоценных металлов серебряное 30С…6С…1.5 РјРј РЎСЂРњ95 РўРЈ РЁРёСЂРѕРєРёР№ выбор высококачественных кольц РёР· драгоценных металлов. Рлегантные украшения, созданные талантливыми мастерами. Уникальность Рё изысканность РІ каждой детали. Возможность заказа персонального кольца РїРѕ вашим пожеланиям. Подчеркните СЃРІРѕСЋ индивидуальность СЃ нашими кольцами.
https://teste.pelissariweb.com.br/2025/03/06/plinko-sovellus-nain-voit-voittaa-suuresti-ja/
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф защищает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы выполнить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в подборе нужных изделий и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что качество и уровень нашего сервиса – ваш лучший выбор.
Наша продукция:
Медный двухраструбный отвод под высокотемпературную пайку 90 градусов 40.5х36х1.1 мм 27х29 мм твердая пайка М2р ГОСТ Р52922-2008 Купите высококачественные медные отводы под высокотемпературную пайку для систем водоснабжения, отопления и кондиционирования воздуха. Превосходные технические характеристики, простота монтажа и долгий срок службы. Широкий выбор размеров и типов отводов. Доставка по России.
https://www.studyway.eu/2025/03/11/ultimate-guide-to-casino-aviator-game-strategies-5/
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем широкий ассортимент продукции, обеспечивая высочайшее качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для законного использования товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с непревзойденным обслуживанием.
Наша команда поддержки всегда на связи, чтобы помочь вам в выборе товаров и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете надежность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – наилучшее решение для вашего бизнеса.
Наша продукция:
Лист свинцовый 20x500x1000 С3 ГОСТ 9559-89 Выберите идеальный свинцовый лист для вашего проекта. Широкий выбор размеров и толщин для защиты от радиации, звукоизоляции, строительства, производства аккумуляторов и многих других целей. Мы предлагаем высококачественные продукты с гарантированной прочностью и устойчивостью. Подробности на сайте!
Мечтаете играть в Castle Clash на компьютере? Получите удовольствие от улучшенной графики и удобных элементов управления. Возводите базы и управляйте армиями мифических существ. после установки через простой Android-эмулятор. Переходите по ссылке [url=https://castle-clash-skachat-na-pk.ru]castle-clash-skachat-na-pk.ru[/url], чтобы начать установку и стартуйте свой восход к славе в королевстве стратегических битв!
castle clash скачать на компьютер
castle clash на пк
castle clash скачать взлом
can you get cheap remeron online
mostbet skachat [url=www.mostbet8002.ru]mostbet skachat[/url] .
Мы изготавливаем дипломы психологов, юристов, экономистов и прочих профессий по приятным тарифам. Мы можем предложить документы ВУЗов, расположенных в любом регионе Российской Федерации. Документы печатаются на бумаге высшего качества. Это дает возможности делать настоящие дипломы, не отличимые от оригинала. [url=http://social.elpaso.world/read-blog/6068_kupit-diplom-s-zaneseniem-v-reestr-forum.html/]social.elpaso.world/read-blog/6068_kupit-diplom-s-zaneseniem-v-reestr-forum.html[/url]
https://poarta9.md/discover-the-exciting-world-of-casino-game-plinko-144/
Заказать диплом университета!
Мы можем предложить дипломы любых профессий по разумным тарифам. Вы покупаете диплом через надежную и проверенную временем компанию. : [url=http://mirmafii.ru/article/8683/]mirmafii.ru/article/8683[/url]
aviator predictor hack apk
хороший фильм без рекламы лучшие фильмы 2025 года в HD
https://www.myvipon.com/post/1474686/suspend_product.php
фильмы на телефон фильмы 2025 без регистрации и рекламы
фильм боевик 2025 фантастика 2025 смотреть бесплатно
Легкое управление жалюзи с пультом — комфорт каждый день
жалюзи с пультом [url=https://pult-zhaluzi.ru/]жалюзи с пультом[/url] . Prokarniz
фильмы онлайн бесплатно фантастика 2025 смотреть бесплатно
https://iancleary.com/never-eat-angry-digestion-and-stress-hormones/
http://www.rtinetwork.org/voices-from-the-field/archive?start=20
мостбет казино скачать [url=http://mostbet5021.ru]мостбет казино скачать[/url] .
РедМетСплав предлагает широкий ассортимент высококачественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до обширных поставок, мы обеспечиваем быстрое выполнение вашего заказа.
Каждая единица товара подтверждена соответствующими документами, подтверждающими их соответствие стандартам. Дружелюбная помощь – то, чем мы гордимся – мы на связи, чтобы ответить на ваши вопросы и адаптировать решения под специфику вашего бизнеса.
Доверьте вашу потребность в редких металлах специалистам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
поставляемая продукция:
РџРѕРєРѕРІРєР° магниевая AZ91B – ASTM B275 Лист магниевый AZ91B – ASTM B275 является высококачественным материалом, который широко используется РІ авиастроении, автомобилестроении Рё РґСЂСѓРіРёС… отраслях, РіРґРµ требуется легкость Рё прочность. Ртот лист обладает отличными механическими свойствами Рё РєРѕСЂСЂРѕР·РёРѕРЅРЅРѕР№ стойкостью. Благодаря РЅРёР·РєРѕР№ плотности, конструкции РёР· него становятся легче, что особенно важно РІ современных технологиях. РќРµ упустите возможность купить Лист магниевый AZ91B – ASTM B275 РїРѕ выгодной цене Рё улучшить СЃРІРѕРё проекты, обеспечив надежность Рё долговечность.
Online casinos should cap—safe guard! vincispin
Well written and easy to understand. Keep it up!
Phim sex clip sex Việt Nam
РедМетСплав предлагает обширный выбор качественных изделий из нестандартных материалов. Не важно, какие объемы вам необходимы – от небольших закупок до масштабных поставок, мы гарантируем быстрое выполнение вашего заказа.
Каждая единица изделия подтверждена соответствующими документами, подтверждающими их качество. Превосходное обслуживание – наша визитная карточка – мы на связи, чтобы ответить на ваши вопросы а также адаптировать решения под требования вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в широком спектре предлагаемых возможностей
Наши товары:
Рлектрод вольфрамовый Р’РќРњ 3-2 Рлектрод вольфрамовый Р’РќРњ 3-2 – это надежный инструмент для сварки Рё резки, обеспечивающий высокое качество соединений. Рзготовленный РёР· высококачественного вольфрама, РѕРЅ гарантирует длительный СЃСЂРѕРє службы Рё устойчивость Рє высоким температурам. Ртот электрод РїРѕРґС…РѕРґРёС‚ для различных типов сварочных работ, как РІ промышленности, так Рё РІ быту. Если РІС‹ ищете надежное решение для выполнения сварочных задач, вам стоит купить Рлектрод вольфрамовый Р’РќРњ 3-2. Его использование значительно повысит производительность Рё качество ваших работ, делая РёС… более профессиональными.
http://make2yatra.com/2025/03/07/entdecken-sie-die-plinko-app-spa-und-gewinne-im-11/
РедМетСплав предлагает обширный выбор высококачественных изделий из редких материалов. Не важно, какие объемы вам необходимы – от небольших закупок до крупных поставок, мы обеспечиваем своевременную реализацию вашего заказа.
Каждая единица продукции подтверждена всеми необходимыми документами, подтверждающими их качество. Опытная поддержка – наш стандарт – мы на связи, чтобы улаживать ваши вопросы а также находить ответы под требования вашего бизнеса.
Доверьте потребности вашего бизнеса профессионалам РедМетСплав и убедитесь в гибкости нашего предложения
поставляемая продукция:
Труба магниевая AS 21 Пруток магниевый AS 21 представляет собой лёгкий и прочный материал, который широко используется в различных отраслях. Он обладает отличными механическими свойствами и коррозионной стойкостью, что делает его идеальным выбором для производства компонентов в аэрокосмической и автомобильной индустрии. Пруток обеспечивает высокую эффективность в обработке и эксплуатации. Благодаря уникальным характеристикам, его часто используют для создания высокотехнологичных решений. Если вы хотите купить Пруток магниевый AS 21, рекомендуем сделать это прямо сейчас, чтобы получить качественный продукт для своих нужд.
Приобрести диплом на заказ возможно через официальный портал компании. [url=http://connectingsparks.com/read-blog/75477_mozhno-li-kupit-diplom-v-reestre.html/]connectingsparks.com/read-blog/75477_mozhno-li-kupit-diplom-v-reestre.html[/url]
https://foro.rune-nifelheim.com/general/colombia/
MetaMask Extension keeps improving! Regular updates enhance security and performance, making it the best crypto wallet.
Medicament prescribing information. What side effects?
how to get cheap atarax for sale
Best information about meds. Read here.
cheap cefixime without dr prescription
I appreciate the detailed explanation, it helped a lot.
http://www.rtinetwork.org/essential/assessment
http://milkywaygalaxynews.com/vision/covid-19-spread-by-aliens/comment-page-72/
amoxil black box warning
http://rtinetwork.org/rti-blog-archives/entry/1/29
Medicine information sheet. Cautions.
how to buy generic loperamide for sale
All information about medication. Get now.
Хотите скачать Castle Clash на компьютер? Оцените высокого разрешения и удобных элементов управления. Сражайтесь с мировым комьюнити в масштабных войнах, после установки через простой Android-эмулятор. Просто перейдите на сайт [url=https://castle-clash-skachat-na-pk.ru]castle-clash-skachat-na-pk.ru[/url] для загрузки и запустите свой путь к величию в королевстве стратегических битв!
castle clash скачать на пк
castle clash скачать на компьютер
как играть в castle clash на пк
The online flow is wild—stay sharp! plinko
http://www.6555555.com/1198.html?replyTo=41041
http://diegoferia.xyz/smiltda/index.php/noticias/item/1-la-falta-de-mantenimiento-de-las-instalaciones-de-gas-pueden-ocasionar-accidentes-no-deseados?start=7190
http://www.smbc-comics.com/smbcforum/posting.php?mode=quote&f=1&p=197169
мостбет бк вход [url=www.mostbet8003.ru]www.mostbet8003.ru[/url] .
мостбет контакты [url=https://www.mostbet5021.ru]https://www.mostbet5021.ru[/url] .
https://donklephant.net/games/servera-lineage-ii-vybor-igrovoj-ploshhadki.html
Одно из существенных преимуществ Апостолиди – возможность предоставлять необходимую информацию посетителям. Мы простым языком объясняем сложные финансовые вопросы. Благодаря регулярным обновлениям вы всегда будете в курсе важных событий. https://apostolidi.ru – сайт, который сейчас пользуются невероятной популярностью. Тут имеется поиск, воспользуйтесь им. Апостолиди дает возможность находить полезные советы, исследовать уникальные материалы и получать знания от экспертов. Рады видеть вас на своем ресурсе!
The visuals online wow—great sight! fortune mouse
В поисках достоверного источника редкоземельных металлов и сплавов? Обратите внимание на компанию Редметсплав.рф. Мы предлагаем внушительный выбор продукции, обеспечивая превосходное качество каждого изделия.
Редметсплав.рф обеспечивает все стадии сделки, предоставляя полный пакет необходимых документов для оформления товаров. Неважно, какие объемы вам необходимы – от мелких партий до крупнооптовых заказов, мы готовы поставить любой запрос с высоким уровнем сервиса.
Наша команда службы поддержки всегда на связи, чтобы помочь вам в определении подходящих продуктов и ответить на любые вопросы, связанные с применением и характеристиками металлов. Выбирая нас, вы выбираете уверенность в каждой детали сотрудничества.
Заходите на наш сайт Редметсплав.рф и убедитесь, что наше качество и сервис – идеальный вариант для вас.
поставляемая продукция:
Танталовый пруток 11 мм ТТ-1 ТУ 95-2819-2002 Широкий выбор танталовых прутков различных диаметров и длин. У нас вы можете приобрести танталовые прутки высокого качества по выгодным ценам для различных промышленных и научных приложений.
На сайте https://iq-kredit.ru/ вы получите всю исчерпывающую, содержательную информацию, которая касается получения кредитов. Прямо сейчас вы сможете воспользоваться поиском по всем кредитным программам финансовых учреждений. Сделайте расчет платежа по кредитам. Вы сможете взять, в том числе, кредит наличными либо на карту. Если вы хотите получить более точную и исчерпывающую информацию на данную тему, то изучите вопросы и ответы. Представлены полезные материалы на тему того, как правильно подобрать кредит.
https://vaeie.eu/tr/members/sasaverber/forums/replies/
Сотрудничество с компанией НАПЫЛЕНИЕ РФ, специализирующейся на поставке редкоземельных материалов для различных методов напыления, открывает перед вашим предприятием двери в мир высококлассных инноваций. Партнерство с нашей компанией гарантирует не только доступ к премиальным продуктам, но и возможность заказа любого необходимого объема.
С широким ассортиментом редкоземельных элементов, от мышьяка до циркония, компания НАПЫЛЕНИЕ РФ готова удовлетворить потребности самых требовательных клиентов. Каждый продукт сопровождается обязательными документами, удостоверяющими его происхождение и соответствие стандартам качества.
Кроме того, НАПЫЛЕНИЕ РФ понимает важность профессионального взаимодействия и поддержки на каждом этапе сотрудничества. В нашем лице вы найдете не просто поставщика, но и партнера, готового предложить квалифицированную помощь и поддержку, опираться на ваши конкретные потребности и адаптировать условия поставки под особенности вашего производства.
Заключая партнерство с НАПЫЛЕНИЕ РФ, вы гарантируете себе плавное и бесперебойное снабжение ценными напыляемыми материалами, позволяющее вам без остановки реализовывать самые передовые и технологичные проекты. Ваш бизнес получит надежного партнера, с которым текущие трудности превращаются в завтрашние успехи.
Наша продукция:
Керамическая мишень АZO для распыления
http://www.vehicleskins.com/memberlist.php?mode=joined&order=ASC&start=237500
Приобрести диплом об образовании. Покупка документа о высшем образовании через надежную компанию дарит ряд достоинств для покупателя. Данное решение позволяет сберечь как дорогое время, так и серьезные финансовые средства. [url=http://addindda.copiny.com/question/details/id/1083585/]addindda.copiny.com/question/details/id/1083585[/url]